Ruby assignment operators return the right-hand side value
Memoizing in ruby is pretty straight forward, but sometimes ruby puts limitations. This limitation is apparent when you override behavior of parent class, and the solution, let alone your mistake, is not so obvious.
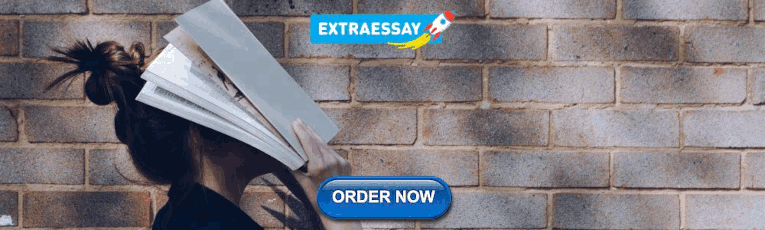
The Problem
Ran into an interesting ruby problem the other day. Basically, we were trying to memoize a hash like so:
You are probably expecting my_hash to have 4 values, right? Wrong.
This is what we got, and Waldo is missing!
Why is this happening?
Ruby specs tell you that, when using assignment operations in ruby, the right side must be returned. This allows chained assignment of variables like so:
When we assign variables in this matter, we expect variable a to be assigned 42 and not be modified somewhere in that assignment process. Suppose we overrode the definition of = on c , and returned a modified value (outcome of c= ), we will not have consistent assignment, and a will not be 42 . For this reason, ruby does not return the result of the assignment, but rather the value we are assigning.
Unfortunately, this leads to confusion in some cases. In our case, it’s use of ActiveSupport::HashWithIndifferentAccess , which inherits from ruby’s native Hash and overrides the []= assignment operator .
The problem with our code is in this line:
When initial assignment of hash happens, we are passing a regular ruby hash into HashWithIndifferentAccess . It modifies a regular ruby hash into a HashWithIndifferentAccess object during the assignment. Since ruby returns the right side of assignemnt, my_hash method will return our regular ruby hash, while memoizing the modified value. The stored object and the returned object will be different. So, when we first access the hash, we actually get the wrong object, and when we assign WALDO to it; we are essentially assigning it to a ruby hash, and not our memoized hash.
The Solution / Workaround
There are two ways of solving this problem:
1. Memoize with HashWithIndifferentAccess
Call hash_with_indifferent_access on your hash to memoize a non-ruby hash, and make sure the right hand side and the left side are same objects when returned.
2. Call my_hash once before assignment
Access the hash getter method to run the memoization before you do any assignments. This will make sure that HashWithIndifferentAccess hash is returned when you do the assignment itself, and you will be accessing the same object.
You can read a smarter answer by Matt on Rails Core mailinglist, and refer to this answer on Stackoverflow .
Expressions
Operator expressions, miscellaneous expressions, command expansion, backquotes are soft, parallel assignment, nested assignments, other forms of assignment, conditional execution, boolean expressions, defined, and, or, and not, if and unless expressions, if and unless modifiers, case expressions, break, redo, and next, variable scope and loops.
Everything You Need to Know About Destructuring in Ruby 3
January 6, 2021 Permalink

Welcome to our first article in a series all about the exciting new features in Ruby 3 ! Today we’re going to look how improved pattern matching and rightward assignment make it possible to “destructure” hashes and arrays in Ruby 3—much like how you’d accomplish it in, say, JavaScript—and some of the ways it goes far beyond even what you might expect. December 2021: now updated for Ruby 3.1 — see below!
First, a primer: destructuring arrays
For the longest time Ruby has had solid destructuring support for arrays. For example:
So that’s pretty groovy. However, you haven’t been able to use a similar syntax for hashes. This doesn’t work unfortunately:
Now there’s a method for Hash called values_at which you could use to pluck keys out of a hash and return in an array which you could then destructure:
But that feels kind of clunky, y’know? Not very Ruby-like.
So let’s see what we can do now in Ruby 3!
Introducing rightward assignment
In Ruby 3 we now have a “rightward assignment” operator. This flips the script and lets you write an expression before assigning it to a variable. So instead of x = :y , you can write :y => x . (Yay for the hashrocket resurgence!)
What’s so cool about this is the smart folks working on Ruby 3 realized that they could use the same rightward assignment operator for pattern matching as well. Pattern matching was introduced in Ruby 2.7 and lets you write conditional logic to find and extract variables from complex objects. Now we can do that in the context of assignment!
Let’s write a simple method to try this out. We’ll be bringing our A game today, so let’s call it a_game :
Now we can pass some hashes along and see what happens!
But what happens when we pass a hash that doesn’t contain the “a” key?
Darn, we get a runtime error. Now maybe that’s what you want if your code would break horribly with a missing hash key. But if you prefer to fail gracefully, rescue comes to the rescue. You can rescue at the method level, but more likely you’d want to rescue at the statement level. Let’s fix our method:
And try it again:
Now that you have a nil value, you can write defensive code to work around the missing data.
What about all the **rest?
Looking back at our original array destructuring example, we were able to get an array of all the values besides the first ones we pulled out as variables. Wouldn’t it be cool if we could do that with hashes too? Well now we can!
But wait, there’s more! Rightward assignment and pattern matching actually works with arrays as well! We can replicate our original example like so:
In addition, we can do some crazy stuff like pull out array slices before and after certain values:
Rightward assignment within pattern matching 🤯
Ready to go all Inception now?!
You can use rightward assignment techniques within a pattern matching expression to pull out disparate values from an array. In other words, you can pull out everything up to a particular type, grab that type’s value, and then pull out everything after that.
You do this by specifying the type (class name) in the pattern and using => to assign anything of that type to the variable. You can also put types in without rightward assignment to “skip over” those and move on to the next match.
Take a gander at these examples:
Powerful stuff!
And the pièce de résistance: the pin operator
What if you don’t want to hardcode a value in a pattern but have it come from somewhere else? After all, you can’t put existing variables in patterns directly:
But in fact you can! You just need to use the pin operator ^ . Let’s try this again!
You can even use ^ to match variables previously assigned in the same pattern. Yeah, it’s nuts. Check out this example from the Ruby docs :
In case you didn’t follow that mind-bendy syntax, it first assigns the value of school (in this case, "high" ), then it finds the hash within the schools array where level matches school . The id value is then assigned from that hash, in this case, 2 .
So this is all amazingly powerful stuff. Of course you can use pattern matching in conditional logic such as case which is what all the original Ruby 2.7 examples showed, but I tend to think rightward assignment is even more useful for a wide variety of scenarios.
“Restructuring” for hashes and keyword arguments in Ruby 3.1
New with the release of Ruby 3.1 is the ability to use a short-hand syntax to avoid repetition in hash literals or when calling keyword arguments.
First, let’s see this in action for hashes:
What’s going on here is that {a:} is shorthand for {a: a} . For the sake of comparison, JavaScript provides the same feature this way: const a = 1; const obj = {a} .
I like {a:} because it’s a mirror image of the hash destructuring feature we discussed above. Let’s round-trip-it!
Better yet, this new syntax doesn’t just work for hash literals. It also works for keyword arguments when calling methods!
Prior to Ruby 3.1, you would have needed to write say_hello(first_name: first_name) . Now you can DRY up your method calls!
Another goodie: the values you’re passing via a hash literal or keyword arguments don’t have to be merely local variables. They can be method calls themselves. It even works with method_missing !
What’s happening here is we’re instantiating a new MissMe object and calling print_message . That method in turn calls miss_you which actually prints out the message. But wait, where is dear actually being defined?! print_message certainly isn’t defining that before calling miss_me . Instead, what’s actually happening is the reference to dear in print_message is triggering method_missing . That in turn supplies the return value of "my dear" .
Now this all may seem quite magical, but it would have worked virtually the same way in Ruby 3.0 and prior—only you would have had to write miss_you(dear: dear) inside of print_message . Is dear: dear any clearer? I don’t think so.
In summary, the new short-hand hash literals/keyword arguments in Ruby 3.1 feels like we’ve come full circle in making both those language features a lot more ergonomic and—dare I say it—modern.
Topic: Ruby 3 Fundamentals
If the Ruby interpreter encounters a line beginning with =begin , it skips that line and all remaining lines through and including a line that begins with =end .
The string expressions begin with % are the special form to avoid putting too many backslashes into quoted strings. The %q/STRING/ expression is the generalized single quote. The %Q/STRING/ (or %/STRING/ ) expression is the generalized double quote. Any non-alphanumeric delimiter can be used in place of / , including newline. If the delimiter is an opening bracket or parenthesis, the final delimiter will be the corresponding closing bracket or parenthesis. (Embedded occurrences of the closing bracket need to be backslashed as usual.)
Backslash notation
\t tab(0x09) \n newline(0x0a) \r carriage return(0x0d) \f form feed(0x0c) \b backspace(0x08) \a bell(0x07) \e escape(0x1b) \s whitespace(0x20) \nnn character in octal value nnn \xnn character in hexadecimal value nn \cx control x \C-x control x \M-x meta x (c | 0x80) \M-\C-x meta control x \x character x itself
The %x/STRING/ is the another form of the command output expression.
The %r/STRING/ is the another form of the regular expression.
^ beginning of a line or string $ end of a line or string . any character except newline \w word character[0-9A-Za-z_] \W non-word character \s whitespace character[ \t\n\r\f] \S non-whitespace character \d digit, same as[0-9] \D non-digit \A beginning of a string \Z end of a string, or before newline at the end \z end of a string \b word boundary(outside[]only) \B non-word boundary \b backspace(0x08)(inside[]only) [ ] any single character of set * 0 or more previous regular expression *? 0 or more previous regular expression(non greedy) + 1 or more previous regular expression +? 1 or more previous regular expression(non greedy) {m,n} at least m but most n previous regular expression {m,n}? at least m but most n previous regular expression(non greedy) ? 0 or 1 previous regular expression | alternation ( ) grouping regular expressions (?# ) comment (?: ) grouping without backreferences (?= ) zero-width positive look-ahead assertion (?! ) zero-width negative look-ahead assertion (?ix-ix) turns on (or off) `i' and `x' options within regular expression. These modifiers are localized inside an enclosing group (if any). (?ix-ix: ) turns on (or off) `i' and `x' options within this non-capturing group.
line-oriented string literals (Here document)
There's a line-oriente form of the string literals that is usually called as `here document'. Following a << you can specify a string or an identifier to terminate the string literal, and all lines following the current line up to the terminator are the value of the string. If the terminator is quoted, the type of quotes determines the type of the line-oriented string literal. Notice there must be no space between << and the terminator.
If the - placed before the delimiter, then all leading whitespcae characters (tabs or spaces) are stripped from input lines and the line containing delimiter. This allows here-documents within scripts to be indented in a natural fashion.
The local variables assigned first time in the blocks are only valid in that block. They are called `dynamic variables.' For example: i0 = 1 loop { i1 = 2 print defined?(i0), "\n" # true print defined?(i1), "\n" # true break } print defined?(i0), "\n" # true print defined?(i1), "\n" # false
self the receiver of the current method nil the sole instance of the Class NilClass(represents false) true the sole instance of the Class TrueClass(typical true value) false the sole instance of the Class FalseClass(represents false) __FILE__ the current source file name. __LINE__ the current line number in the source file.
%w expressions make creation of the arrays of strings easier. They are equivalent to the single quoted strings split by the whitespaces. For example:
is equivalent to ["foo", "bar", "baz"] . Note that parenthesis right after %s is the quote delimiter, not usual parenthesis.
If the last argument expression preceded by & , the value of the expression, which must be a Proc object, is set as the block for the calling method.
Some methods are private , and can be called from function form invocations (the forms that omits receiver).
There may be no space between operators and = .
Multiple assignment acts like this:
Behavior of the === method varies for each Object. See docutmentation for each class.
The word ` raise ' is not the reserved word in Ruby. raise is the method of the Kernel module. There is an alias named fail .
If an exception occurs in the begin body, the rescue clause with the matching exception type is executed (if any). The match is done by the kind_of? . The default value of the rescue clause argument is the StandardError , which is the superclass of most built-in exceptions. Non-local jumps like SystemExit or Interrupt are not subclass of the StandardError .
The begin statement has an optional else clause, which must follow all rescue clauses. It is executed if the begin body does not raise any exception.
For the rescue clauses, the error_type is evaluated just like the arguments to the method call, and the clause matches if the value of the variable $! is the instance of any one of the error_type of its subclass. If error_type is not class nor module, the rescue clause raises TypeError exception.
If retry appears in the iterator, the block, or the body of the for expression, restarts the invocation of the iterator call. Arguments to the iterator is re-evaluated.
Registers the initialize routine. The block followed after BEGIN is evaluated before any other statement in that file (or string). If multiple BEGIN blocks are given, they are evaluated in the appearing order.
The BEGIN block introduce new local-variable scope. They don't share local variables with outer statements.
The BEGIN statement can only appear at the toplevel.
Registers finalize routine. The block followed after END is evaluated just before the interpreter termination. Unlike BEGIN , END blocks shares their local variables, just like blocks.
The END statement registers its block only once at the first execution. If you want to register finalize routines many times, use at_exit .
The END statement can only appear at the toplevel. Also you cannot cancel finalize routine registered by END .
Defines the new method. Method_name should be either identifier or re-definable operators (e.g. ==, +, -, etc.). Notice the method is not available before the definition. For example: foo def foo print "foo\n" end will raise an exception for undefined method invoking.
The return value of the method is the value given to the return , or that of the last evaluated expression.
In addition, the methods named initialize are always defined as private methods.
Making aliases for the numbered global variables ( $1 , $2 ,...) is prohibited. Overriding the builtin global variables may cause serious problems.
Ruby 2.7 Reference SAVE UKRAINE
In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v :
Assignment creates a local variable if the variable was not previously referenced.
Abbreviated Assignment
You can mix several of the operators and assignment. To add 1 to an object you can write:
This is equivalent to:
You can use the following operators this way: + , - , * , / , % , ** , & , | , ^ , << , >>
There are also ||= and &&= . The former makes an assignment if the value was nil or false while the latter makes an assignment if the value was not nil or false .
Here is an example:
Note that these two operators behave more like a || a = 0 than a = a || 0 .
Multiple Assignment
You can assign multiple values on the right-hand side to multiple variables:
In the following sections any place “variable” is used an assignment method, instance, class or global will also work:
You can use multiple assignment to swap two values in-place:
If you have more values on the right hand side of the assignment than variables on the left hand side, the extra values are ignored:
You can use * to gather extra values on the right-hand side of the assignment.
The * can appear anywhere on the left-hand side:
But you may only use one * in an assignment.
Array Decomposition
Like Array decomposition in method arguments you can decompose an Array during assignment using parenthesis:
You can decompose an Array as part of a larger multiple assignment:
Since each decomposition is considered its own multiple assignment you can use * to gather arguments in the decomposition:
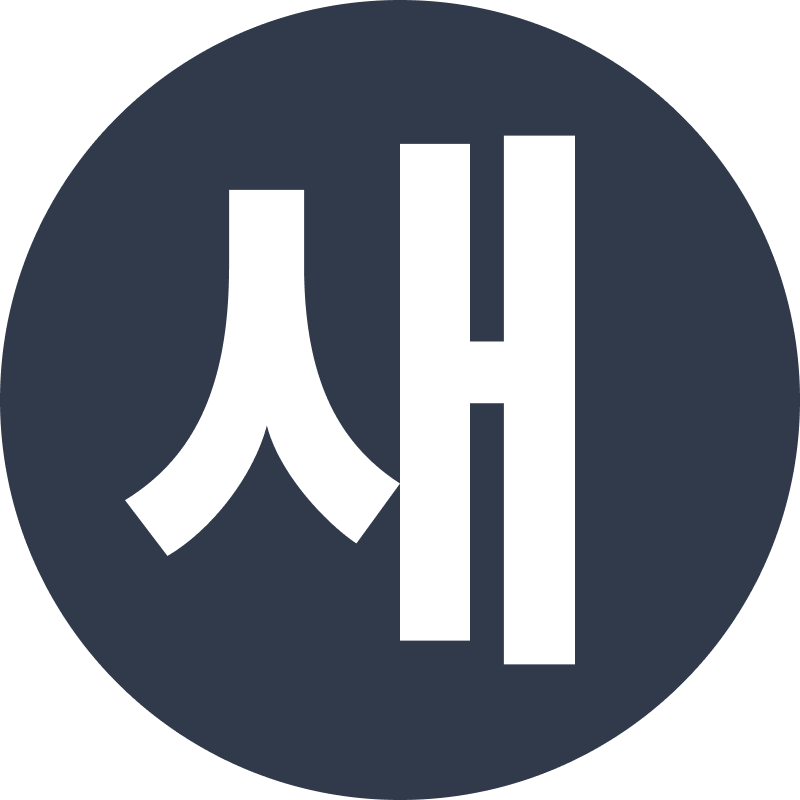
Ruby adds experimental support for Rightward assignments
Vamsi is a Ruby on Rails and ReactJS Developer.
This blog post discusses the support for Rightward assignments in ruby.
Historically, all of the early programming languages were designed by Mathematicians. It’s a common practice in mathematics to say let x = 4y + z , which is read as let x be equal to 4y + z .
So the same pattern is followed in programming languages as well.
For assigning a value to a variable, we typically do age = 42 in ruby. Here we are assigning the Rvalue 42 to an Lvalue age .
While the above pattern has become standardized, it feels somewhat unnatural as we read most of the spoken languages from left to right.
We were not able to do something like 42 => age .
With the recent changes , Ruby supports the Right ward assignments as demonstrated below.
Let’s take a look at few more examples:
which is equivalent to
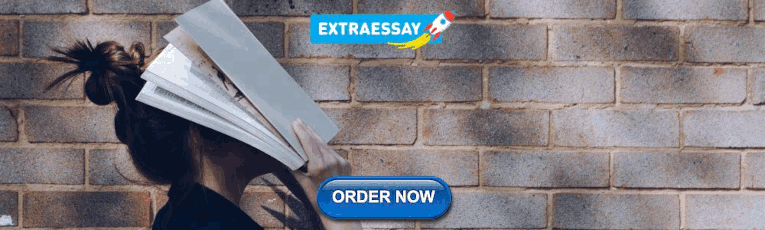
Additional notes:
There are already some languages which support rightward assignment.
TI-BASIC’s uses STO (“store”) operation to achieve this, as demonstrated below:
R language also has a similar way of doing this 42 -> age .
Note : This is added as an experimental feature in Ruby, which means it could be removed depending on the feedback received.
Need help on your Ruby on Rails or React project?
If you enjoyed this post, you might also like:
The Ultimate Guide to Gemfile and Gemfile.lock
August 16, 2022
Integrate OpenAI API in Ruby applications
May 22, 2023
Ruby 3.1 introduces pattern matching pin operator against expression
July 7, 2021
Join Our Newsletter
Thanks! Your subscription has been confirmed.
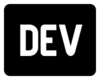
DEV Community

Posted on Aug 3, 2022
Destructuring in Ruby
Destructuring refers to the process of unpacking a small part of something from the main part. In Ruby, we use destructuring to conveniently extract multiple values from data stored in Arrays or Hashes. It provides a way for us to group or ungroup data and assign them to variables where needed.
In this article we’re going to discuss destructuring for assignments, arrays and keyword args. We’ll also touch on why you would utilize destructuring.
The most straightforward form of destructuring is through multiple assignments. This form of destructuring assigns the variables on the left to the values on the right in the order that they are provided.
As an example:
In the above example the variables on the left are assigned to the values on the right. If we have a mismatch between the number of variables ready for assignment on the left and the number of values on the right then some of the variables will remain unassigned like below:
If we have a mismatch in the number of values on the left, with fewer variables to assign to them, then some of the values will remain unassigned like below:
More complex destructuring can be done using an operator in Ruby called the the splat (*) operator. Splat performs different operations depending on how it is used, let’s discuss how splat works.
Slurp/Collect
When the splat operator appears on the left hand side of an assignment then we can refer to the operation as slurp or collect. Slurping takes a variable number of values and collects it into an array.
The example below with a slurp on a variable at the end will collect the rest of the values (into an array) that have not been assigned to the first two variables:
Splat is pretty smart - it can slurp up the “rest” of the values depending where the splat operator is positioned. Let’s look at some more examples.
A splat operator somewhere in the middle will get the “rest” of the values once the other non-splatted variables are assigned. In this case, a gets assigned to the first value, then c gets assigned to the last value and b gets assigned the remainder of the values in the form of an array.
Now, think about what you would expect a slurp operator on a variable at the start of the assignment to yield?
Well, we’d look at the non splatted values first and work our way backwards - c will take the value of 4, b will take the value of 3 and a would collect the “rest” of the values into an array i.e. [1, 2] . Does that make sense to you?
Just like above, an array can also be destructured into multiple parts and assigned to variables.
Up until now we talked about a splat on the left hand side of the assignment which slurps or collects up the values on the right hand side. How does it then work when the splat operator (*) is on the right hand side? It will split up the array into individual components.
Destructuring on the right hand side allows us to split the collection into a list of values being assigned.
So in the case above the splat on *list will change the statement into:
and so the assignments happen as per usual:
Let’s look at a more complex example - what if we had to split on the right side of the assignment and slurp up values on the left side, how would that work?
Slurp and Split
You’re already equipped with the tools for dealing with them separately so let’s apply that knowledge.
First, let’s look at the right side of this assignment. Based on previous knowledge we know that the splat operator on the right hand side will split, meaning that it will convert the array into a list of values like below:
Now that we’ve done that, let's look at the left hand side of the assignment. As per the rules that we learned earlier, we will allocate the first variable a to the first value 1 . We have the splat in the middle so let's leave that for last. We then allocate out the last variable to the last value, so c is assigned to 5 . Finally, we’ll focus on the splat operator which indicates that we should slurp up the rest of the values [2, 3, 4] to assign to b .
Implicit and Explicit Splats
If we have an assignment containing just one variable on the left with a number of values on the right, it will slurp up all of the values into a new array.
This is equivalent to
Notice that in the first example we didn’t need the asterisk, the value was just implicitly spatted . In some cases where the assignments are more straightforward, this is acceptable.
However, if you wanted to perform destructuring where there is more than one variable on the left you would need to explicitly splat the variable. You would also have noticed in some of the above sections that there are cases whereby an array will be listed alongside individual values, and hence you would need an explicit splat to break down the array into its component values.
Keyword Arguments
In a rails app you’ll often come across a splat on argument methods, like *args below:
Abiding to our previous rules outlined, the splat operator on *args collects the rest of the parameters except the one assigned to name . It then converts those “rest” of the arguments to an array within the method, hence, allowing us to loop through the args.
In this manner, we don’t need to specify the number of arguments that we are providing to the method. Instead, we can deal with them as an array that we loop through.
The parameter with the splat operator is also optional, which means that if we omit these arguments to a method, Ruby will not complain about it.
It is important to note that a method cannot have two parameters with a splat operator, and you can only splat the last parameter to a method.
Now that we’ve got a good foundation on how destructuring is used, let’s talk about when we would use destructuring.
Destructuring can be used:
- When you do not want to specify the number of parameters that you have.
- When you want to make the parameter optional - a parameter with a splat operator is optional.
- When you’d like to convert a list of arguments to an array within the method and vice versa - when you want to convert an array into several arguments..
- You can use destructuring when programming in a functional, recursive manner whereby you can assign the first parameter to one variable and the rest of the variables can be assigned to another variable using a splat.
The scenarios above are only some instances where destructuring can be useful, let me know how you’ve used destructuring in the past.
Top comments (1)
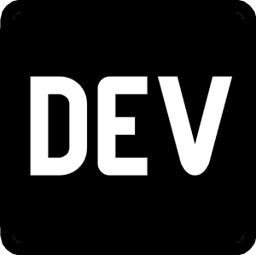
Templates let you quickly answer FAQs or store snippets for re-use.

- Email [email protected]
- Location Bengaluru, India
- Education B.Tech. (Computer Engineering)
- Work Software Engineer at Deepsource
- Joined Aug 20, 2018
Wait so the way positional arguments work in Ruby is also used for variable destructuring in a simple code block? Thats such an elegant language implementation!
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
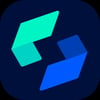
ServBay 1.3.0 Release Notes
ServBay - Mar 29
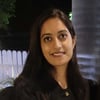
LOOPS - FOR LOOP
Sakshi - Mar 29
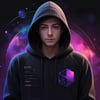
What is dependency injection
mahdi - Apr 8
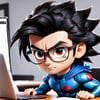
Unity UI Optimization Workflow: Step-by-Step full guide for everyone
Devs Daddy - Apr 2
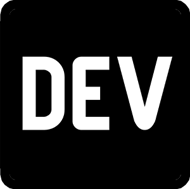
We're a place where coders share, stay up-to-date and grow their careers.
Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
Ruby right-hand assignment syntax bug #4902
willcosgrove commented Mar 19, 2023
- 👍 1 reaction
joeldrapper commented Apr 6, 2024
Sorry, something went wrong.
willcosgrove commented Apr 6, 2024
No branches or pull requests
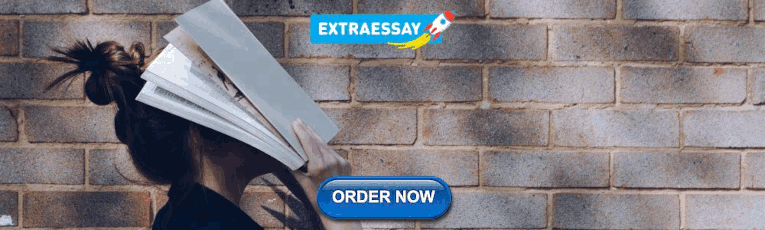
IMAGES
VIDEO
COMMENTS
Assignment ¶ ↑. In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: ... If you have more values on the right hand side of the assignment than variables on the left hand side, the extra values are ignored: a, b = 1, 2, ...
When initial assignment of hash happens, we are passing a regular ruby hash into HashWithIndifferentAccess.It modifies a regular ruby hash into a HashWithIndifferentAccess object during the assignment. Since ruby returns the right side of assignemnt, my_hash method will return our regular ruby hash, while memoizing the modified value. The stored object and the returned object will be different.
I'm having trouble understanding these two sections in ruby-doc: Implicit Array Assignment; Multiple Assignment; When it says left-hand side, the splat operator is on the right side, and when it says right-hand side, the operator is on the left side. For example: The * can appear anywhere on the right-hand side:
The values on the right-hand side are evaluated in the order in which they appear before any assignment is made to variables or attributes on the left. A somewhat contrived example illustrates this. ... As of Ruby 1.6.2, if an assignment has one lvalue and multiple rvalues, the rvalues are converted to an array and assigned to the lvalue.
Introducing rightward assignment. In Ruby 3 we now have a "rightward assignment" operator. ... New with the release of Ruby 3.1 is the ability to use a short-hand syntax to avoid repetition in hash literals or when calling keyword arguments. ... podcast & article updates sent right to your email inbox.
This from is evaluated to the invocation of the method named identifier= with the right hand side expression as a argument. self assignment. Examples: foo += 12 Syntax: expr op= expr # left hand side must be assignable. This form evaluated as expr = expr op expr. But right hand side expression evaluated once. op can be one of:
Ruby has a variety of ways to control execution. All the expressions described here return a value. ... When used as a modifier the left-hand side is the "then" statement and the right-hand side is the "test" expression: a = 0 a += 1 if a. zero? p a. This will print 1. ... then later it sees the assignment to a in the "test ...
You can use multiple assignment to swap two values in-place: old_value = 1 new_value, old_value = old_value, 2 p new_value: new_value, old_value: old_value # prints {:new_value=>1, :old_value=>2} If you have more values on the left hand side of the assignment than variables on the right hand side the extra values are ignored:
priority= called on the result of the tag. After. Starting with Ruby 3.1, multiple assignments evaluation order has been made consistent with single assignment evaluation order. For the above expression, the left-hand side is evaluated before the right-hand side and the order is as below: lists. tag.
You can assign multiple values on the right-hand side to multiple variables: a , b = 1 , 2 p a: a , b: b # prints {:a=>1, :b=>2} In the following sections any place "variable" is used an assignment method, instance, class or global will also work:
a = 1. method `a' called. a = 99. During the parse, Ruby sees the use of a in the first puts statement and, as it hasn't yet seen any assignment to a, assumes that it is a method call. By the time it gets to the second puts statement, though, it has seen an assignment, and so treats a as a variable.
Additional notes: There are already some languages which support rightward assignment. TI-BASIC's uses STO ("store") operation to achieve this, as demonstrated below:. 42→age. R language also has a similar way of doing this 42 -> age.. Note: This is added as an experimental feature in Ruby, which means it could be removed depending on the feedback received.
There's a new weird feature that's been added experimentally to the latest version of ruby: The "Right-ward Assignment Operator" Normally in a ruby program you's assign values to variables with the traditional: variable_name = variable_value. But this new experimental feature, true to Ruby form, gives you yet another possible way to ...
More complex destructuring can be done using an operator in Ruby called the the splat (*) operator. Splat performs different operations depending on how it is used, let's discuss how splat works. Slurp/Collect When the splat operator appears on the left hand side of an assignment then we can refer to the operation as slurp or collect.
The "pipeline operator" is not the only syntactic construct where data flow goes from left to right. Indeed, Ruby's most basic construct, method invocation, leads to a data flow from left to right in the form of method chains. If an R-assign operator is suitable after some pipeline operator(s), it sure should be suitable after a method chain.
Overview. This cop checks the indentation of the first line of the right-hand-side of a multi-line assignment. The indentation of the remaining lines can be corrected with other cops such as 'IndentationConsistency` and `EndAlignment`.
a && = b is also a conditional assignment operator. It is considered to be a shorthand for a && a = b.The a && a assigns the value on the right to the variable on the left, if the variable on the left, in this case a, is not nil or false.. If a is NOT undefined or falsey (false or nil), then evaluate band set a to the result. Scenario 5 (x is defined and evaluates to false):
Seeing something like a = 123, the interpreter assumes that this is always an assignment operation.However, you can call self.a = 123- as this cannot be a proper assignment (variable name cannot include a dot); it will invoke a method you defined.. Note that the same happens inside the class, so it is not a different behaviour: class Foo def foo=(x) puts "OK: x=#{x}" end def bar foo = 1 end ...
Check for existing issues Completed Describe the bug / provide steps to reproduce it Ruby like this will break syntax highlighting for the remainder of the file: def a_method a_method_call => a_variable, another_variable end It handles r...
Ruby arrays: right hand of statement changing through assignment even though I didn't tell it to. Ask Question Asked 4 years, 2 months ago. Modified 4 years, ... Ruby variable (Array) assignment misunderstanding (with push method) 1 Ruby incorrect array management. 0 ...