- Contributors
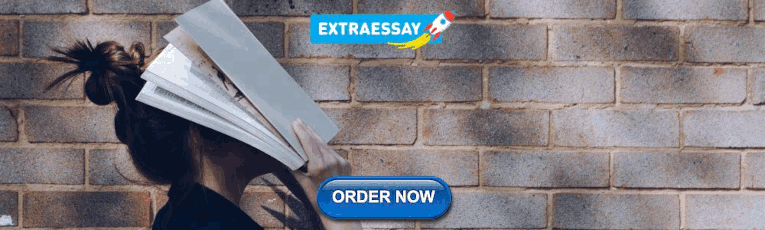
Array Variables
Table of contents, python's array, array vs list in python, how to create an array, a length of an array in python, array indexing in python, iterating over an array using "for" loop, python array or dataframe, how to print an array in python, how to find an element in an array, the map() function, working with json arrays, how to get the last element of an array in python, how to save a numpy array in python, bitarray library, associative arrays in python, dynamic array in python.
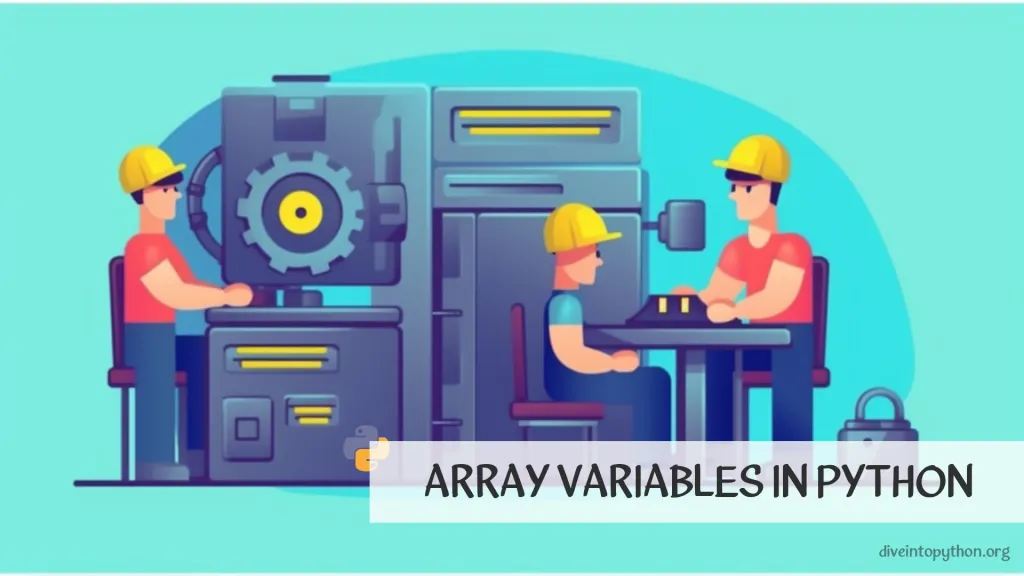
The official Python documentation contains information about arrays . However, in Python, the built-in data structure commonly used to represent arrays is the list . The official documentation primarily focuses on lists rather than a separate array data type. Lists are ordered, mutable, and can contain elements of different data types . You can access elements in a list using their indices, and you can perform various operations on lists such as appending, inserting, or removing elements. With these facts in mind, we'll look at lists from, say, an array's point of view.
Let's look at what an array is in Python. An array is a data structure that stores a collection of elements of the same type. It is a container that holds a fixed number of items, and the elements can be accessed using their indices. Python provides several ways to work with arrays, including built-in data structures like lists and the NumPy library's ndarray.
There are several possibilities how to make an array in Python. As we already mentioned, lists are usually used as arrays in Python. But if you want to improve performance and reduce memory consumption for certain use cases, you can use the array module in Python. It provides a way to create arrays that are more efficient and compact compared to traditional lists, it allows you to define arrays of a specific data type.
To use the array module, you first need to import it:
Next, you can create an array in Python by specifying the desired data type code and initializing it with values. Here's an example of creating an array of integers:
In the above example, 'i' represents the data type code for signed integers. You can choose different data type codes based on your specific needs (e.g., 'f' for floats, 'd' for doubles, 'b' for bytes, etc.).
Once you have created an array, you can access its elements using indexing, just like with regular lists. You can also modify the values in the array or perform various operations available for arrays.
Note: the array module is particularly useful when you are working with large amounts of numerical data or when you need to interface with low-level libraries that expect data in a specific format. For general-purpose collections of heterogeneous elements, the built-in list type is usually more flexible and commonly used in Python.
In Python, the terms "array" and "list" are often used interchangeably, but they refer to different data structures with some distinctions. Let's explore the differences between them:
Memory Allocation : Arrays in Python are provided by the array module and represent a fixed-size, homogeneous collection of elements. They are generally more memory-efficient compared to lists because they store elements of the same type contiguously in memory. Lists, on the other hand, are heterogeneous and can store elements of different types. Lists are implemented as dynamic arrays that automatically resize themselves to accommodate new elements.
Data Types : Arrays are constrained to a specific data type. When creating an array, you need to specify the type of elements it will hold (e.g., integers, floats, characters). This constraint allows arrays to provide more efficient storage and operations on their elements. Lists, being heterogeneous, can contain elements of different data types within the same list.
Flexibility : Lists are more flexible compared to arrays. They can grow or shrink dynamically, as elements can be added or removed at any position. Arrays, once created, have a fixed size and cannot be changed. If you need to modify an array's size, you would have to create a new array with the desired size and copy the elements from the old array.
Operations and Methods : Both arrays and lists provide common operations like indexing, slicing, and iteration. However, arrays have additional methods provided by the array module, such as efficient mathematical operations on the array as a whole (e.g., sum, product), which can be faster compared to equivalent operations on lists. External Libraries: Some external libraries, such as NumPy, provide multidimensional arrays that are widely used for numerical computations. NumPy arrays offer efficient storage and vectorized operations on arrays, making them highly optimized for numerical computations. Lists do not have such built-in functionality.
In summary, arrays are fixed-size, homogeneous collections of elements that are memory-efficient and provide specific operations, while lists are dynamic, heterogeneous collections that offer more flexibility and versatility. The choice between arrays and lists depends on the specific requirements of your program, such as memory usage, data type constraints, and the need for dynamic resizing or specialized operations.
In Python, arrays can be created using various methods and libraries. There are also some other parameters which should be taken into account at the moment of array creation.
Simple Array with Integers
You can create an array in Python using the built-in array module or by simply initializing an empty list. Here are two examples of creating arrays:
- Initializing an array of integers using the array module:
- The second approach is to declare a list instead of an array:
To create an empty array, you can follow the approaches mentioned above. Next, we'll look at the defining of an array of size n.
Array of Size N
To create an array of a specific size in Python, you can use various methods, including using a list comprehension or using NumPy. Here are a few examples of arrays' declaring:
Using a list comprehension:
Using NumPy:
Random-Generated Array
To generate a random array in Python, you can use the random module from the Python standard library or the numpy library. Here are examples using both approaches:
Using the random module:
Using the numpy library:
Both approaches allow you to generate random arrays of integers. Adjust the parameters ( a , b , and size ) based on your specific requirements to control the range and size of the random array.
2D Array in Python
Here is an example how to initialize a multi-dimensional array in Python using np.array() function:
You can also create a two-dimensional array using a list of lists, where each inner list represents a row. Here's an example of how to create and initialize a 2D array using nested lists:
How to Create a NumPy Array in Python
To create a NumPy array in Python, you can use the numpy.array() function. Here's an example of np array initialization:
In the above code, import numpy as np imports the NumPy module, allowing us to use its functions and classes .
Array of Strings in Python
To create an array of strings in Python , you can use a list where each element of the list represents a string. Here's an example:
In the above example, we create an array of strings called array using a list. Each element of the list represents a string. The resulting array contains four strings: 'apple', 'banana', 'orange', and 'grape'.
Array of Dictionaries
In Python, you can create an array (or list) of dictionaries by simply initializing a list and adding dictionaries as its elements. Each dictionary can contain key-value pairs representing different properties or attributes. Here's an example:
Array of Tuples in Python
In Python, you can create an array of tuples using different data structures. Here are a few examples:
- List of Tuples:
You can create an array of tuples using a list. Each tuple represents an element in the array. Here's an example:
- NumPy Array of Tuples:
If you are working with NumPy arrays, you can create an array of tuples using the np.array() function. Here's an example:
- Array module:
If you are using the built-in array module, you can create an array of tuples using the array constructor. Here's an example:
Array of Bytes
In Python, you can create an array of bytes using the built-in bytearray or bytes types. Here's an example of creating and working with an array of bytes:
Using bytearray :
Using bytes :
Both bytearray and bytes represent sequences of bytes and can be used interchangeably in many contexts. Choose the appropriate one based on whether you need a mutable or immutable sequence of bytes.
The range() Function for Array in Python
In Python, you can create an array or list of numbers using the range() function. The range() function generates a sequence of numbers within a specified range.
Here are a few examples of using the range() function to create arrays or lists of numbers:
- Creating a range of numbers as a list:
- Creating a range of numbers with a specified start and end:
- Creating a range of numbers with a specified start, end, and step size:
The range() function can be used to create arrays or lists of numbers based on different start, end, and step size values. By converting the range object to a list using the list() function, you can obtain a list representation of the range.
Array of Zeros
In Python, you can create an array of zeros using various libraries and data structures. Here are a few examples:
If you have NumPy installed, you can use the zeros() function from the NumPy library to create an array of zeros. Here's an example:
You can also create multi-dimensional arrays of zeros by specifying the shape as a tuple. For example:
- List comprehension:
If you prefer working with lists, you can use list comprehension to create an array of zeros. Here's an example:
For multi-dimensional arrays, you can nest list comprehensions. Here's an example:
You can get the length of an array (or any sequence) using the len() function. The len() function returns the number of elements in the sequence.
Here's an example of how to use len() to get the length of an array:
In this example, len(my_array) counts array elements and returns the length of the my_array list, which is 5. The length variable stores this value, and it is then printed to the console.
Note: The len() function works not only with arrays but with any iterable object, such as lists, tuples, strings, or sets.
In Python, an indexed array is typically represented using a list. The indices of a list are used to access and manipulate the elements within it, so you can access individual elements of an array (or list) using indexing. Array indexing allows you to retrieve a specific element from the array by referring to its position or index within the array.
Array indexes start at 0, so the first element of an array is at index 0, the second element is at index 1, and so on.
Here's an example of how to use array indexing in Python:
In this example, my_array[2] retrieves the element at index 2 of my_array , which is 30. The value is then stored in the element variable and printed to the console.
You can also use negative indexing to access elements from the end of the array. With negative indexing, -1 refers to the last element, -2 refers to the second-to-last element, and so on.
In this case, my_array[-1] retrieves the last element of my_array , which is 50. The value is stored in the element variable and printed to the console.
You can also use indexing to modify the value of an element or to extract a subset of elements from an array using slicing.
In Python, you can use a "for" loop to iterate over the elements of an array and perform operations on each element. There are different ways to iterate over an array, depending on the type of array you are working with. Here are a few examples of looping through arrays:
- Using a for loop with a standard Python list:
- Using a "for" loop with a NumPy array:
- Using a "for" loop with a multidimensional NumPy array:
We have already seen what an array is, let's look at DataFrame.
A DataFrame (pandas) is a two-dimensional tabular data structure provided by the pandas library. It is highly versatile and widely used for data manipulation and analysis tasks. DataFrames can hold data of different types (e.g., integers, floats, strings) and provide powerful indexing, slicing, grouping, and aggregation functionalities. DataFrames are particularly useful when working with large datasets, performing complex operations, or when you need to work with labeled or structured data.
Here's an example of creating a DataFrame:
In this example, we create a DataFrame df using a dictionary data and then print the resulting DataFrame.
DataFrames offer many features, such as indexing, filtering, merging, and handling missing values, making them a popular choice for data analysis and manipulation tasks.
In summary, if you need a simple data structure for basic numerical computations, a Python array can be sufficient. However, if you require more advanced data manipulation, analysis, and a tabular structure, a DataFrame (such as pandas DataFrame) would be a better choice.
To print an array in Python, you can use the print() function. The specific syntax will depend on the type of array you are working with. Here are a few examples:
- Printing a standard Python list:
- Printing a NumPy array:
- Printing a multidimensional NumPy array:
To find an element in an array in Python, you can use various methods depending on the type of array you are working with. Here are a few examples:
If you have a standard Python list, you can use the in operator or the index() method to find an element:
- NumPy array:
For a NumPy array, you can use boolean indexing or the where() function to find the indices or values that match a condition:
In Python, you can use the map() function to apply a given function to each element of an array or iterable. The map( ) function returns an iterator that contains the results of applying the provided function to each element. Here's an example of how to use map() with an array:
In this example, the map( ) function is used to apply the square() function to each element of the my_array . The square() function squares each input number, and the map() function returns an iterator containing the squared values. Finally, the result is converted to a list using the list() function.
Alternatively, you can use a lambda function with map() to achieve the same result in a more concise way:
In this case, the lambda function lambda x: x ** 2 is used to square each element of the array.
The map() function is a useful tool for applying a function to every element of an array or iterable in Python. It simplifies the process of transforming the elements and provides a concise way to perform element-wise operations.
In Python, you can work with JSON arrays using the json module, which provides functions for working with JSON data. Here's an example of how to work with a JSON array in Python:
You can also convert a Python list into a JSON array using the json.dumps() function. Here's an example:
To get the last element of an array in Python, you can use indexing or built-in functions depending on the data structure you are working with. Here are a few approaches:
If you have a list, you can use negative indexing to access the last element. Here's an example:
If you are working with a NumPy array, you can use the [-1] index to access the last element. Here's an example:
If you are using the built-in array module, you can use indexing to access the last element. Here's an example:
To save a NumPy array in Python, you can use the numpy.save() function or the numpy.savez() function. Here's how you can use each of them:
- numpy.save() : This function saves a single NumPy array to a binary file with a .npy extension. You can specify the filename along with the array you want to save. Here's an example:
- numpy.savez() : This function saves multiple NumPy arrays into a single compressed .npz file. You can provide a filename and pass the arrays as arguments. Here's an example:
In Python, you can use the bitarray library to work with bit arrays. The bitarray library provides a flexible and efficient way to manipulate arrays of boolean values, where each boolean value represents a single bit.
To use the bitarray library, you first need to install it. You can install it using pip by running the following command:
Once installed, you can start working with bit arrays using the bitarray class from the library. Here's an example:
In Python, associative arrays are typically implemented using dictionaries. Dictionaries are unordered collections of key-value pairs, where each key is unique and associated with a value. They provide a way to store and retrieve data based on a specific key rather than numerical indices. Here's an example of how to work with dictionaries as associative arrays in Python:
In Python, you can use the built-in list data structure to create a dynamic array. A dynamic array in Python is a resizable array that can grow or shrink in size as needed. The list data structure provides dynamic resizing automatically, allowing you to add or remove elements dynamically without explicitly managing the array's size.
Here's an example of how to create and use a dynamic array in Python:
Dive deep into the topic
- How to Convert an Array to Other Types
- Operations with Arrays
Contribute with us!
Do not hesitate to contribute to Python tutorials on GitHub: create a fork, update content and issue a pull request.
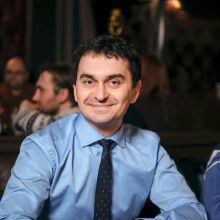
Multiple assignment in Python: Assign multiple values or the same value to multiple variables
In Python, the = operator is used to assign values to variables.
You can assign values to multiple variables in one line.
Assign multiple values to multiple variables
Assign the same value to multiple variables.
You can assign multiple values to multiple variables by separating them with commas , .
You can assign values to more than three variables, and it is also possible to assign values of different data types to those variables.
When only one variable is on the left side, values on the right side are assigned as a tuple to that variable.
If the number of variables on the left does not match the number of values on the right, a ValueError occurs. You can assign the remaining values as a list by prefixing the variable name with * .
For more information on using * and assigning elements of a tuple and list to multiple variables, see the following article.
- Unpack a tuple and list in Python
You can also swap the values of multiple variables in the same way. See the following article for details:
- Swap values in a list or values of variables in Python
You can assign the same value to multiple variables by using = consecutively.
For example, this is useful when initializing multiple variables with the same value.
After assigning the same value, you can assign a different value to one of these variables. As described later, be cautious when assigning mutable objects such as list and dict .
You can apply the same method when assigning the same value to three or more variables.
Be careful when assigning mutable objects such as list and dict .
If you use = consecutively, the same object is assigned to all variables. Therefore, if you change the value of an element or add a new element in one variable, the changes will be reflected in the others as well.
If you want to handle mutable objects separately, you need to assign them individually.
after c = []; d = [] , c and d are guaranteed to refer to two different, unique, newly created empty lists. (Note that c = d = [] assigns the same object to both c and d .) 3. Data model — Python 3.11.3 documentation
You can also use copy() or deepcopy() from the copy module to make shallow and deep copies. See the following article.
- Shallow and deep copy in Python: copy(), deepcopy()
Related Categories
Related articles.
- NumPy: arange() and linspace() to generate evenly spaced values
- Chained comparison (a < x < b) in Python
- pandas: Get first/last n rows of DataFrame with head() and tail()
- pandas: Filter rows/columns by labels with filter()
- Get the filename, directory, extension from a path string in Python
- Sign function in Python (sign/signum/sgn, copysign)
- How to flatten a list of lists in Python
- None in Python
- Create calendar as text, HTML, list in Python
- NumPy: Insert elements, rows, and columns into an array with np.insert()
- Shuffle a list, string, tuple in Python (random.shuffle, sample)
- Add and update an item in a dictionary in Python
- Cartesian product of lists in Python (itertools.product)
- Remove a substring from a string in Python
- pandas: Extract rows that contain specific strings from a DataFrame

Nick McCullum
Software Developer & Professional Explainer
NumPy Indexing and Assignment
Hey - Nick here! This page is a free excerpt from my $199 course Python for Finance, which is 50% off for the next 50 students.
If you want the full course, click here to sign up.
In this lesson, we will explore indexing and assignment in NumPy arrays.
The Array I'll Be Using In This Lesson
As before, I will be using a specific array through this lesson. This time it will be generated using the np.random.rand method. Here's how I generated the array:
Here is the actual array:
To make this array easier to look at, I will round every element of the array to 2 decimal places using NumPy's round method:
Here's the new array:
How To Return A Specific Element From A NumPy Array
We can select (and return) a specific element from a NumPy array in the same way that we could using a normal Python list: using square brackets.
An example is below:
We can also reference multiple elements of a NumPy array using the colon operator. For example, the index [2:] selects every element from index 2 onwards. The index [:3] selects every element up to and excluding index 3. The index [2:4] returns every element from index 2 to index 4, excluding index 4. The higher endpoint is always excluded.
A few example of indexing using the colon operator are below.
Element Assignment in NumPy Arrays
We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step).
arr[2:5] = 0.5
Returns array([0. , 0. , 0.5, 0.5, 0.5])
As you can see, modifying second_new_array also changed the value of new_array .
Why is this?
By default, NumPy does not create a copy of an array when you reference the original array variable using the = assignment operator. Instead, it simply points the new variable to the old variable, which allows the second variable to make modification to the original variable - even if this is not your intention.
This may seem bizarre, but it does have a logical explanation. The purpose of array referencing is to conserve computing power. When working with large data sets, you would quickly run out of RAM if you created a new array every time you wanted to work with a slice of the array.
Fortunately, there is a workaround to array referencing. You can use the copy method to explicitly copy a NumPy array.
An example of this is below.
As you can see below, making modifications to the copied array does not alter the original.
So far in the lesson, we have only explored how to reference one-dimensional NumPy arrays. We will now explore the indexing of two-dimensional arrays.
Indexing Two-Dimensional NumPy Arrays
To start, let's create a two-dimensional NumPy array named mat :
There are two ways to index a two-dimensional NumPy array:
- mat[row, col]
- mat[row][col]
I personally prefer to index using the mat[row][col] nomenclature because it is easier to visualize in a step-by-step fashion. For example:
You can also generate sub-matrices from a two-dimensional NumPy array using this notation:
Array referencing also applies to two-dimensional arrays in NumPy, so be sure to use the copy method if you want to avoid inadvertently modifying an original array after saving a slice of it into a new variable name.
Conditional Selection Using NumPy Arrays
NumPy arrays support a feature called conditional selection , which allows you to generate a new array of boolean values that state whether each element within the array satisfies a particular if statement.
An example of this is below (I also re-created our original arr variable since its been awhile since we've seen it):
You can also generate a new array of values that satisfy this condition by passing the condition into the square brackets (just like we do for indexing).
An example of this is below:
Conditional selection can become significantly more complex than this. We will explore more examples in this section's associated practice problems.
In this lesson, we explored NumPy array indexing and assignment in thorough detail. We will solidify your knowledge of these concepts further by working through a batch of practice problems in the next section.
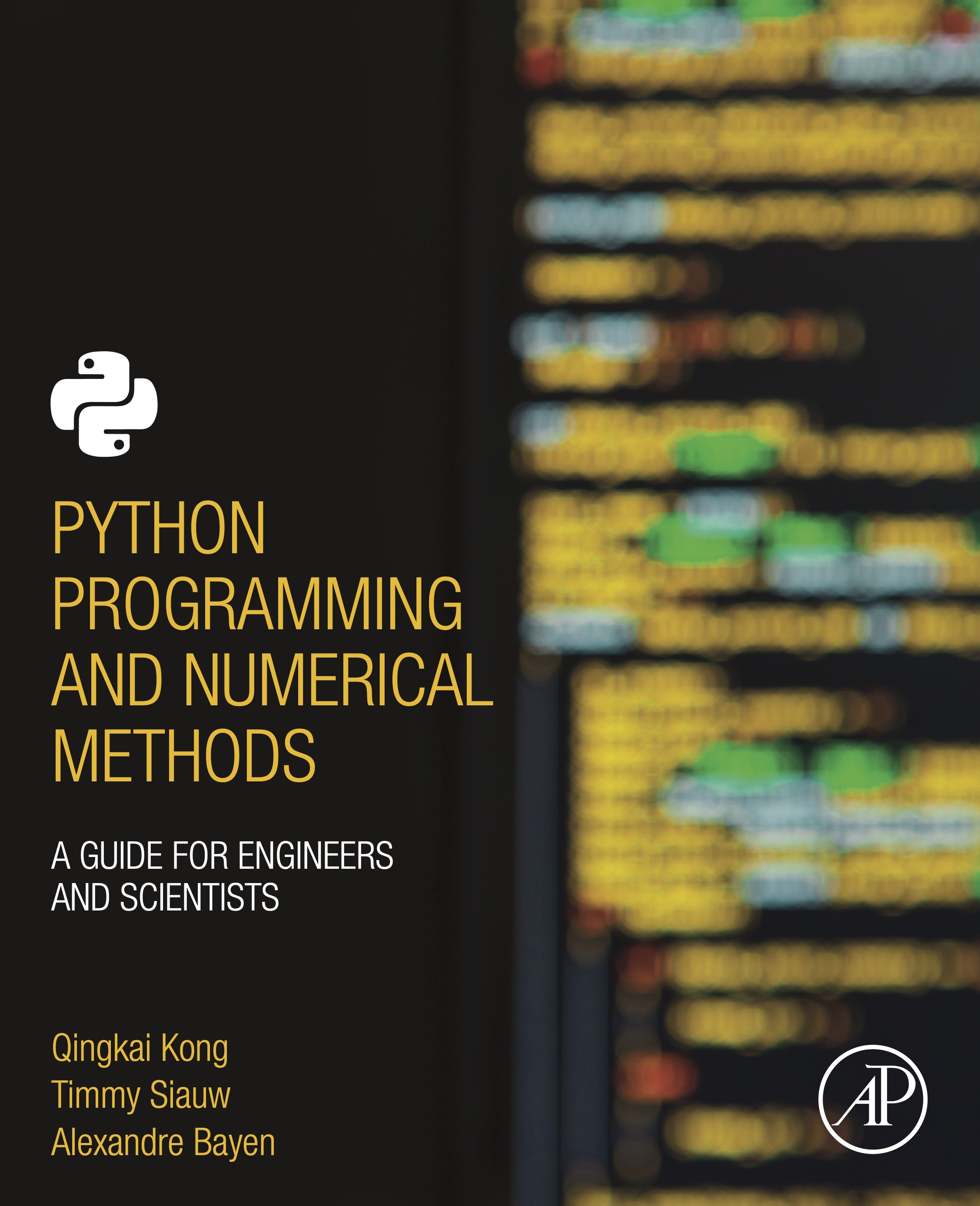
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.0 Variables and Basic Data Structures | Contents | 2.2 Data Structure - Strings >
Variables and Assignment ¶
When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator , denoted by the “=” symbol, is the operator that is used to assign values to variables in Python. The line x=1 takes the known value, 1, and assigns that value to the variable with name “x”. After executing this line, this number will be stored into this variable. Until the value is changed or the variable deleted, the character x behaves like the value 1.
TRY IT! Assign the value 2 to the variable y. Multiply y by 3 to show that it behaves like the value 2.
A variable is more like a container to store the data in the computer’s memory, the name of the variable tells the computer where to find this value in the memory. For now, it is sufficient to know that the notebook has its own memory space to store all the variables in the notebook. As a result of the previous example, you will see the variable “x” and “y” in the memory. You can view a list of all the variables in the notebook using the magic command %whos .
TRY IT! List all the variables in this notebook
Note that the equal sign in programming is not the same as a truth statement in mathematics. In math, the statement x = 2 declares the universal truth within the given framework, x is 2 . In programming, the statement x=2 means a known value is being associated with a variable name, store 2 in x. Although it is perfectly valid to say 1 = x in mathematics, assignments in Python always go left : meaning the value to the right of the equal sign is assigned to the variable on the left of the equal sign. Therefore, 1=x will generate an error in Python. The assignment operator is always last in the order of operations relative to mathematical, logical, and comparison operators.
TRY IT! The mathematical statement x=x+1 has no solution for any value of x . In programming, if we initialize the value of x to be 1, then the statement makes perfect sense. It means, “Add x and 1, which is 2, then assign that value to the variable x”. Note that this operation overwrites the previous value stored in x .
There are some restrictions on the names variables can take. Variables can only contain alphanumeric characters (letters and numbers) as well as underscores. However, the first character of a variable name must be a letter or underscores. Spaces within a variable name are not permitted, and the variable names are case-sensitive (e.g., x and X will be considered different variables).
TIP! Unlike in pure mathematics, variables in programming almost always represent something tangible. It may be the distance between two points in space or the number of rabbits in a population. Therefore, as your code becomes increasingly complicated, it is very important that your variables carry a name that can easily be associated with what they represent. For example, the distance between two points in space is better represented by the variable dist than x , and the number of rabbits in a population is better represented by nRabbits than y .
Note that when a variable is assigned, it has no memory of how it was assigned. That is, if the value of a variable, y , is constructed from other variables, like x , reassigning the value of x will not change the value of y .
EXAMPLE: What value will y have after the following lines of code are executed?
WARNING! You can overwrite variables or functions that have been stored in Python. For example, the command help = 2 will store the value 2 in the variable with name help . After this assignment help will behave like the value 2 instead of the function help . Therefore, you should always be careful not to give your variables the same name as built-in functions or values.
TIP! Now that you know how to assign variables, it is important that you learn to never leave unassigned commands. An unassigned command is an operation that has a result, but that result is not assigned to a variable. For example, you should never use 2+2 . You should instead assign it to some variable x=2+2 . This allows you to “hold on” to the results of previous commands and will make your interaction with Python must less confusing.
You can clear a variable from the notebook using the del function. Typing del x will clear the variable x from the workspace. If you want to remove all the variables in the notebook, you can use the magic command %reset .
In mathematics, variables are usually associated with unknown numbers; in programming, variables are associated with a value of a certain type. There are many data types that can be assigned to variables. A data type is a classification of the type of information that is being stored in a variable. The basic data types that you will utilize throughout this book are boolean, int, float, string, list, tuple, dictionary, set. A formal description of these data types is given in the following sections.
Python Slicing – How to Slice an Array and What Does [::-1] Mean?
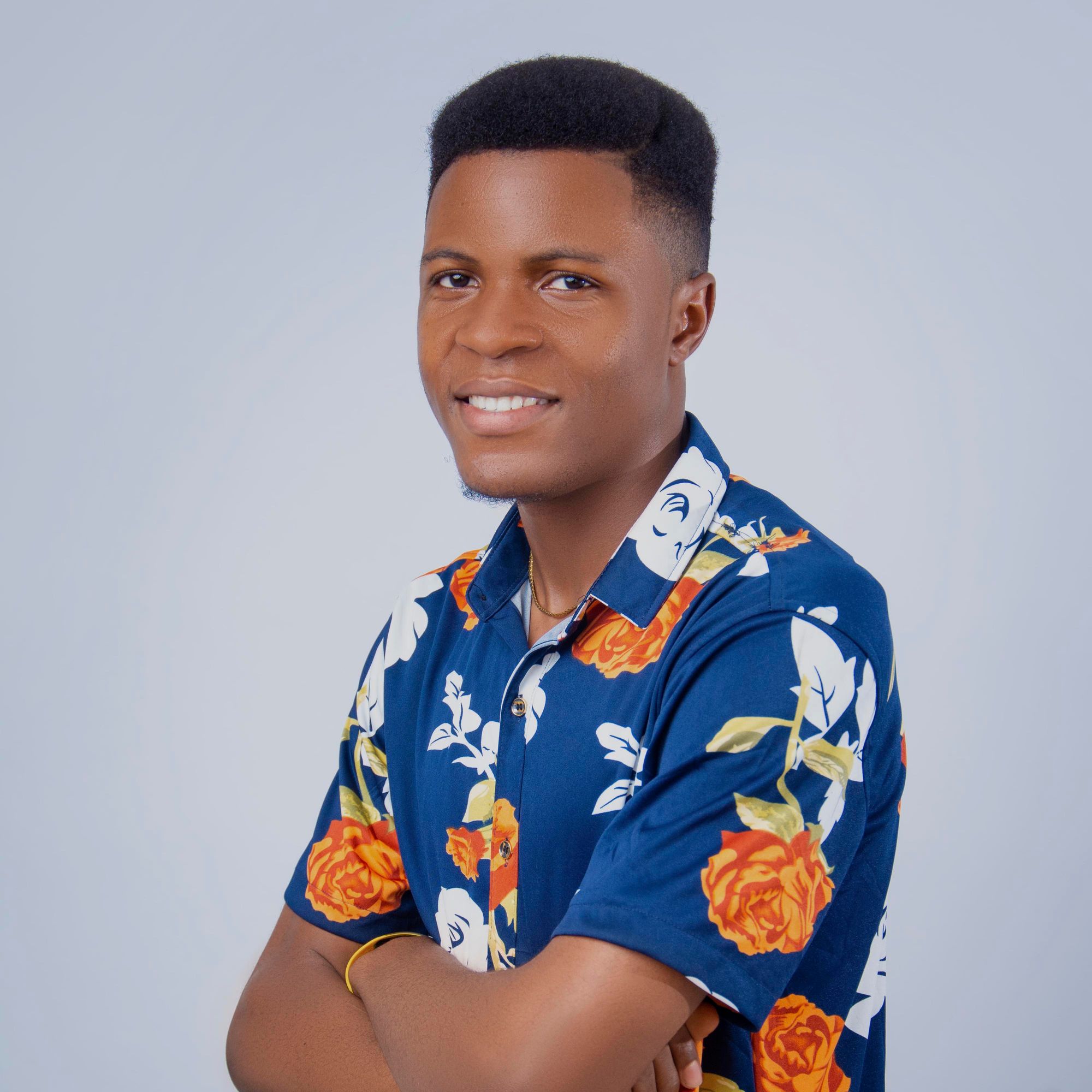
Slicing an array is the concept of cutting out – or slicing out – a part of the array. How do you do this in Python? I'll show you how in this article.
If you like watching video content to supplement your reading, here's a video version of this article as well.
What is an Array?
An array is a data structure that allows you to store multiple items of the same data type in order in a variable at the same time. You can access each of these items by their index (location in the order).
They are a bit similar to lists in Python, it's just that lists allow you to store multiple items of different data types . Also, while lists are built-in, arrays aren't.
How to Access Values in an Array in Python
Here's the syntax to create an array in Python:
As the array data type is not built into Python by default, you have to import it from the array module. We import this module as arr .
Using the array method of arr , we can create an array by specifying a typecode (data type of the values) and the values stored in the array.
Here's a table showing the acceptable typecodes:
Typecodes gotten from the Python documentation .
Here's an example array in Python:
We created an array of integer values from 1 to 5 here. We also accessed the second value by using square brackets and its index in the order, which is 1 .
How to Slice an Array in Python
Let's say you want to slice a portion of this array and assign the slice to another variable. You can do it using colons and square brackets. The syntax looks like this:
The start index specifies the index that the slicing starts from. The default is 0 .
The end index specifies the index where the slicing ends (but excluding the value at this index). The default is the length of the array.
The step argument specifies the step of the slicing. The default is 1 .
Let's see some examples that cover different ways in which arrays can be sliced.
How to slice without a start or end index
When you slice without a start or end index, you basically get a whole copy of the array:
As you can see here, we have a copy of the numbers array.
It's also worth noting that the slicing action does not affect the original array. With slicing, you only "copy a portion" of the original array.
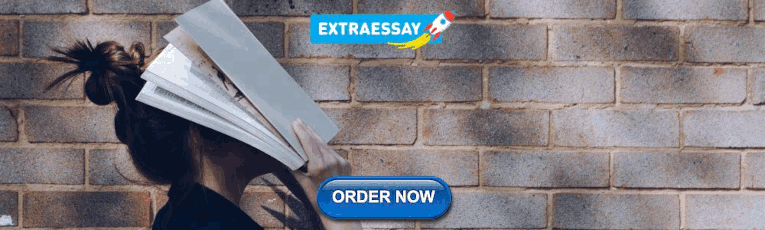
How to slice with a start index
For example, if you want to slice an array from a specific start value to the end of the array, here's how:
By passing 2: in the square brackets, the slicing starts from index 2 (which holds value 3) up until the end of the array, as you can see in the results.
How to slice with an end index
For example, if you want to slice an array from the first value to the third, here's how:
By passing :3 in the square brackets, slicing starts from the 0 index (by default, since not specified) up until the third index we specified.
As mentioned earlier, the slicing excludes the value at the third index. So in the results, as you find in the copy variable, the values returned are from index 0 through index 2 .
How to slice with a start and end index
What if you want to specify the starting and ending points of the slicing? Here's how:
By using a number, then a colon, followed by a number in square brackets, you can specify a starting and ending indexes, respectively.
In our case, we used 1 and 4 as in [1:4] . From the results, you see that the slicing starts from the value at index 1 which is 2 , up until the value before the index 4 , which is 4 (at index 3).
How to slice with steps
When you specify a start and end index of 1 and 5, respectively, slicing will select values at index 1 , index 2 (1 increment to the previous index), index 3 (1 increment to the previous index) and index 4 (and one increment to the previous index).
In this slicing, a step of 1 is used by default. But you can provide a different step. Here's how:
By adding another colon, you can specify a step. So we have [start:stop:step] .
In our example, the start is 1 , the end is 4 and the step is 2. Slicing starts from the value at index 1 which is 2 , then the next value will be at the previous index plus the step, which is 1 + 2 equals 3. The value at index 3 is 4 so that is added to the slice. The next index will be 5 ( 3 + 2 ) but since 5 exceeds the stop index, it will not be added to the slice.
As you can see in the code, the sliced copy is just 2 and 4.
How to slice with negative start and end indexes
The start or stop indexes can also be negative. Negative indexes count from the end of the array. This means a negative index is the last value in an array:
By using a negative 1 here, you see that 5 is returned, which is from the end of an array.
With a slice expression like [-3:-1] , this means a start index of -3 and end index of -1 . Let's see how that works with our array:
The slice starts from index -3 (which is the third value from the end of the array, that is 3) and stops at index -1 (which is the last value in the array, that is 5). Slicing doesn't include the last value so we have 3 and 4.
Combining a negative start index and a positive end index (or vice versa) will not work as you'll be going different directions at once.
How to slice with negative steps
You can use negative steps, which means the steps decrement for the slicing. Here's an example:
Here, we specify a start of index 2 , no end, and a step of -1 . Slicing here will start from index 2 which is 3. The negative steps mean the next value in the slice will be at an index smaller than the previous index by 1. This means 2 - 1 which is 1 so the value at this index, which is 2 will be added to the slice.
This goes on reversed until it gets to the end of the array which is index 0 . The sliced array results in values of 3, 2, and 1.
What does [::-1] mean?
Have you seen this expression anywhere in Python before? Well, here's what it means: there's no start index specified, nor an end index, only a negative step of -1 .
The start index defaults to 0 , so by using -1 step, the slicing will contain values at the following indexes: -1 ( 0 - 1 ), -2 ( -1 - 1 ), -3 ( -2 - 1 ), -4 ( -3 - 1 ) and -5 ( -4 - 1 ). Pretty much a reversed copy of the array.
Here's the code for it:
As you can see, this is a simple way to reverse an array.
Wrapping Up
In this article, we've briefly looked at how to declare arrays in Python, how to access values in an array, and also how to cut – or slice – a part of an array using a colon and square brackets.
We also learned how slicing works with steps and positive/negative start and stop indexes.
You can learn more about arrays here: Python Array Tutorial – Define, Index, Methods .
Developer Advocate and Content Creator passionate about sharing my knowledge on Tech. I simplify JavaScript / ReactJS / NodeJS / Frameworks / TypeScript / et al My YT channel: youtube.com/c/deeecode
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Python Variables and Assignment
Python variables, variable assignment rules, every value has a type, memory and the garbage collector, variable swap, variable names are superficial labels, assignment = is shallow, decomp by var.
Multiple Assignment Syntax in Python
- python-tricks
The multiple assignment syntax, often referred to as tuple unpacking or extended unpacking, is a powerful feature in Python. There are several ways to assign multiple values to variables at once.
Let's start with a first example that uses extended unpacking . This syntax is used to assign values from an iterable (in this case, a string) to multiple variables:
a : This variable will be assigned the first element of the iterable, which is 'D' in the case of the string 'Devlabs'.
*b : The asterisk (*) before b is used to collect the remaining elements of the iterable (the middle characters in the string 'Devlabs') into a list: ['e', 'v', 'l', 'a', 'b']
c : This variable will be assigned the last element of the iterable: 's'.
The multiple assignment syntax can also be used for numerous other tasks:
Swapping Values
This swaps the values of variables a and b without needing a temporary variable.
Splitting a List
first will be 1, and rest will be a list containing [2, 3, 4, 5] .
Assigning Multiple Values from a Function
This assigns the values returned by get_values() to x, y, and z.
Ignoring Values
Here, you're ignoring the first value with an underscore _ and assigning "Hello" to the important_value . In Python, the underscore is commonly used as a convention to indicate that a variable is being intentionally ignored or is a placeholder for a value that you don't intend to use.
Unpacking Nested Structures
This unpacks a nested structure (Tuple in this example) into separate variables. We can use similar syntax also for Dictionaries:
In this case, we first extract the 'person' dictionary from data, and then we use multiple assignment to further extract values from the nested dictionaries, making the code more concise.
Extended Unpacking with Slicing
first will be 1, middle will be a list containing [2, 3, 4], and last will be 5.
Split a String into a List
*split, is used for iterable unpacking. The asterisk (*) collects the remaining elements into a list variable named split . In this case, it collects all the characters from the string.
The comma , after *split is used to indicate that it's a single-element tuple assignment. It's a syntax requirement to ensure that split becomes a list containing the characters.
Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python variables, varia b l e s.
Variables are containers for storing data values.
Creating Variables
Python has no command for declaring a variable.
A variable is created the moment you first assign a value to it.
Variables do not need to be declared with any particular type , and can even change type after they have been set.
If you want to specify the data type of a variable, this can be done with casting.
Advertisement
Get the Type
You can get the data type of a variable with the type() function.
Single or Double Quotes?
String variables can be declared either by using single or double quotes:
Case-Sensitive
Variable names are case-sensitive.
This will create two variables:

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- How to Fix: ValueError: Unknown Label Type: 'Continuous' in Python
- How To Check If Variable Is Empty In Python?
- How To Fix - Python RuntimeWarning: overflow encountered in scalar
- How To Resolve Issue " Threading Ignores Keyboardinterrupt Exception" In Python?
- AttributeError: can’t set attribute in Python
- How to Fix ImportError: Cannot Import name X in Python
- AttributeError: __enter__ Exception in Python
- Modulenotfounderror: No Module Named 'httpx' in Python
- How to Fix - SyntaxError: (Unicode Error) 'Unicodeescape' Codec Can't Decode Bytes
- SyntaxError: ‘return’ outside function in Python
- How to fix "SyntaxError: invalid character" in Python
- No Module Named 'Sqlalchemy-Jsonfield' in Python
- Python Code Error: No Module Named 'Aiohttp'
- Fix Python Attributeerror: __Enter__
- Python Runtimeerror: Super() No Arguments
- Filenotfounderror: Errno 2 No Such File Or Directory in Python
- ModuleNotFoundError: No module named 'dotenv' in Python
- Nameerror: Name Plot_Cases_Simple Is Not Defined in Python
- How To Avoid Notimplementederror In Python?
How to Fix – UnboundLocalError: Local variable Referenced Before Assignment in Python
Developers often encounter the UnboundLocalError Local Variable Referenced Before Assignment error in Python. In this article, we will see what is local variable referenced before assignment error in Python and how to fix it by using different approaches.
What is UnboundLocalError: Local variable Referenced Before Assignment?
This error occurs when a local variable is referenced before it has been assigned a value within a function or method. This error typically surfaces when utilizing try-except blocks to handle exceptions, creating a puzzle for developers trying to comprehend its origins and find a solution.
Below, are the reasons by which UnboundLocalError: Local variable Referenced Before Assignment error occurs in Python :
Nested Function Variable Access
Global variable modification.
In this code, the outer_function defines a variable ‘x’ and a nested inner_function attempts to access it, but encounters an UnboundLocalError due to a local ‘x’ being defined later in the inner_function.
In this code, the function example_function tries to increment the global variable ‘x’, but encounters an UnboundLocalError since it’s treated as a local variable due to the assignment operation within the function.
Solution for Local variable Referenced Before Assignment in Python
Below, are the approaches to solve “Local variable Referenced Before Assignment”.
In this code, example_function successfully modifies the global variable ‘x’ by declaring it as global within the function, incrementing its value by 1, and then printing the updated value.
In this code, the outer_function defines a local variable ‘x’, and the inner_function accesses and modifies it as a nonlocal variable, allowing changes to the outer function’s scope from within the inner function.
Please Login to comment...
Similar reads.
- Python Errors
- Python How-to-fix
- Google Introduces New AI-powered Vids App
- Dolly Chaiwala: The Microsoft Windows 12 Brand Ambassador
- 10 Best Free Remote Desktop apps for Android in 2024
- 10 Best Free Internet Speed Test apps for Android in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
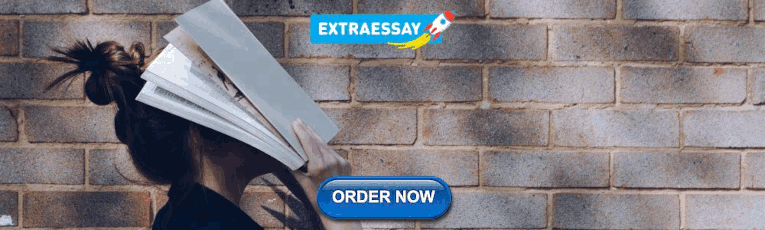
IMAGES
VIDEO
COMMENTS
14. Yes you can separate each variable with a , to perform unpacking. repost_pid, repost_permalink, repost_domain, repost_title, repost_submitter = row. If there is a particular value that you are not concerned about, the convention is to assign it to an underscore variable, e.g. repost_pid, repost_permalink, _, repost_title, repost_submitter ...
Python Variables Variable Names Assign Multiple Values Output Variables Global Variables Variable Exercises. ... to work with arrays in Python you will have to import a library, like the NumPy library. Arrays are used to store multiple values in one single variable: Example. Create an array containing car names: cars = ["Ford", "Volvo", "BMW"]
How to Save a NumPy Array in Python. To save a NumPy array in Python, you can use the numpy.save() function or the numpy.savez() function. Here's how you can use each of them: numpy.save(): This function saves a single NumPy array to a binary file with a .npy extension. You can specify the filename along with the array you want to save.
Here, variable represents a generic Python variable, while expression represents any Python object that you can provide as a concrete value—also known as a literal—or an expression that evaluates to a value. To execute an assignment statement like the above, Python runs the following steps: Evaluate the right-hand expression to produce a concrete value or object.
They act like a variable that can hold a collection of values, all of which are the same type. These values are stored together in bordering memory. Python Array Methods. You can use various built-in Python methods when working with lists and arrays. Below are the methods you can use on arrays and lists in Python. The Append() method
Python Array Tutorial - Define, Index, Methods. Dionysia Lemonaki. In this article, you'll learn how to use Python arrays. You'll see how to define them and the different methods commonly used for performing operations on them. The article covers arrays that you create by importing the array module. We won't cover NumPy arrays here.
Python supports numbers, strings, sets, lists, tuples, and dictionaries. These are the standard data types. I will explain each of them in detail. Declare And Assign Value To Variable. Assignment sets a value to a variable. To assign variable a value, use the equals sign (=) myFirstVariable = 1 mySecondVariable = 2 myFirstVariable = "Hello You"
You can also swap the values of multiple variables in the same way. See the following article for details: Swap values in a list or values of variables in Python; Assign the same value to multiple variables. You can assign the same value to multiple variables by using = consecutively.
Variable Assignment. Think of a variable as a name attached to a particular object. In Python, variables need not be declared or defined in advance, as is the case in many other programming languages. To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ).
In Python, an array is used to store multiple values or elements of the same datatype in a single variable. The extend () function is simply used to attach an item from iterable to the end of the array. In simpler terms, this method is used to add an array of values to the end of a given or existing array.
Try: variable = []; x = [variable]; x[0] = 10;print variable; The point is, it is not possible to modify the other references to an object when you assign one of the references to something else. - Ashwini Chaudhary
Element Assignment in NumPy Arrays. We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step). array([0.12, 0.94, 0.66, 0.73, 0.83])
To create a variable, you just assign it a value and then start using it. Assignment is done with a single equals sign ( = ): Python. >>> n = 300. This is read or interpreted as " n is assigned the value 300 .". Once this is done, n can be used in a statement or expression, and its value will be substituted: Python.
Variables and Assignment¶. When programming, it is useful to be able to store information in variables. A variable is a string of characters and numbers associated with a piece of information. The assignment operator, denoted by the "=" symbol, is the operator that is used to assign values to variables in Python.The line x=1 takes the known value, 1, and assigns that value to the variable ...
How to Slice an Array in Python. Let's say you want to slice a portion of this array and assign the slice to another variable. You can do it using colons and square brackets. The syntax looks like this: array[start:stop:step] The start index specifies the index that the slicing starts from. The default is 0.
Python Variables. A Python variable is a named bit of computer memory, keeping track of a value as the code runs. A variable is created with an "assignment" equal sign =, with the variable's name on the left and the value it should store on the right: x = 42 In the computer's memory, each variable is like a box, identified by the name of the ...
Use numpy.meshgrid () to make arrays of indexes that you can use to index into both your original array and the array of values for the third dimension. import numpy as np. import scipy as sp. import scipy.stats.distributions. a = np.zeros((2,3,4)) z = sp.stats.distributions.randint.rvs(0, 4, size=(2,3))
The multiple assignment syntax, often referred to as tuple unpacking or extended unpacking, is a powerful feature in Python. There are several ways to assign multiple values to variables at once. Let's start with a first example that uses extended unpacking. This syntax is used to assign values from an iterable (in this case, a string) to ...
Example Get your own Python Server. x = 5. y = "John". print(x) print(y) Try it Yourself ». Variables do not need to be declared with any particular type, and can even change type after they have been set.
Python Array Variable Assign. 0. assigning a value to a list of arrays. 2. How to assign multiple variables from multidimensional array in python. 0. Assigning new variable to a list of arrays in Python. Hot Network Questions What contemporary hardware was available for the development of Atari 2600 (or other 2nd gen) games?
So always use the == operator to check if any two Python objects have the same value. 4. Tuple Assignment and Mutable Objects . If you're familiar with built-in data structures in Python, you know that tuples are immutable. So you cannot modify them in place. Data structures like lists and dictionaries, on the other hand, are mutable.
Output. Hangup (SIGHUP) Traceback (most recent call last): File "Solution.py", line 7, in <module> example_function() File "Solution.py", line 4, in example_function x += 1 # Trying to modify global variable 'x' without declaring it as global UnboundLocalError: local variable 'x' referenced before assignment Solution for Local variable Referenced Before Assignment in Python
Python Array Variable Assign. Ask Question Asked 4 years, 10 months ago. Modified 4 years, 10 months ago. Viewed 595 times 0 I have the following array in Python in the following format: Array[('John', '123'), ('Alex','456'),('Nate', '789')] Is there a way I can assign the array variables by field as below? ...