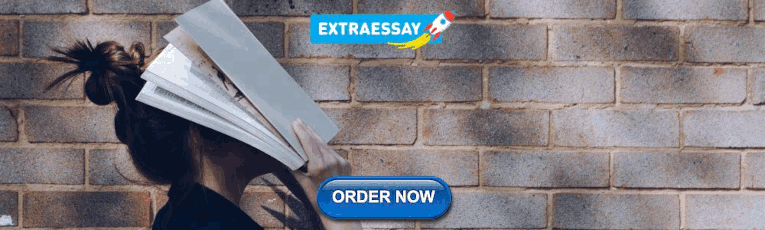
Practice 146 exercises in JavaScript
Learn and practice JavaScript by completing 146 exercises that explore different concepts and ideas.
Explore the JavaScript exercises on Exercism
Unlock more exercises as you progress. They’re great practice and fun to do!
Free Javascript challenges
Learn Javascript online by solving coding exercises.
Javascript for all levels
Solve Javascript tasks from beginner to advanced levels.
Accross various subjects
Select your topic of interest and start practicing.
Start your learning path here
Why jschallenger, a hands-on javascript experience.
JSchallenger provides a variety of JavaScript exercises, including coding tasks, coding challenges, lessons, and quizzes.
Structured learning path
JSchallenger provides a structured learning path that gradually increases in complexity. Build your skills and knowledge at your own pace.
Build a learning streak
JSchallenger saves your learning progress. This feature helps to stay motivated and makes it easy for you to pick up where you left off.
Type and execute code yourself
Type real JavaScript and see the output of your code. JSchallenger provides syntax highlighting for easy understanding.
Join 1.000s of users around the world
Most popular challenges, most failed challenges, what users say about jschallenger.
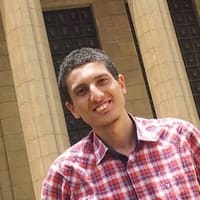
Mohamed Ibrahim
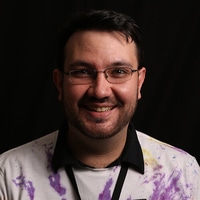
The Modern JavaScript Tutorial
How it's done now. From the basics to advanced topics with simple, but detailed explanations.
Table of contents
Main course contains 2 parts which cover JavaScript as a programming language and working with a browser. There are also additional series of thematic articles.
The JavaScript language
Here we learn JavaScript, starting from scratch and go on to advanced concepts like OOP.
We concentrate on the language itself here, with the minimum of environment-specific notes.
- An Introduction to JavaScript
- Manuals and specifications
- Code editors
- Developer console
- Hello, world!
- Code structure
- The modern mode, "use strict"
- Interaction: alert, prompt, confirm
- Type Conversions
- Basic operators, maths
- Comparisons
- Conditional branching: if, '?'
- Logical operators
- Nullish coalescing operator '??'
- Loops: while and for
- The "switch" statement
- Function expressions
- Arrow functions, the basics
- JavaScript specials
- Debugging in the browser
- Coding Style
- Automated testing with Mocha
- Polyfills and transpilers
- Object references and copying
- Garbage collection
- Object methods, "this"
- Constructor, operator "new"
- Optional chaining '?.'
- Symbol type
- Object to primitive conversion
- Methods of primitives
- Array methods
- Map and Set
- WeakMap and WeakSet
- Object.keys, values, entries
- Destructuring assignment
- Date and time
- JSON methods, toJSON
- Recursion and stack
- Rest parameters and spread syntax
- Variable scope, closure
- The old "var"
- Global object
- Function object, NFE
- The "new Function" syntax
- Scheduling: setTimeout and setInterval
- Decorators and forwarding, call/apply
- Function binding
- Arrow functions revisited
- Property flags and descriptors
- Property getters and setters
- Prototypal inheritance
- F.prototype
- Native prototypes
- Prototype methods, objects without __proto__
- Class basic syntax
- Class inheritance
- Static properties and methods
- Private and protected properties and methods
- Extending built-in classes
- Class checking: "instanceof"
- Error handling, "try...catch"
- Custom errors, extending Error
- Introduction: callbacks
- Promises chaining
- Error handling with promises
- Promise API
- Promisification
- Async/await
- Async iteration and generators
- Modules, introduction
- Export and Import
- Dynamic imports
- Proxy and Reflect
- Eval: run a code string
- Reference Type
- Unicode, String internals
- WeakRef and FinalizationRegistry
Browser: Document, Events, Interfaces
Learning how to manage the browser page: add elements, manipulate their size and position, dynamically create interfaces and interact with the visitor.
- Browser environment, specs
- Walking the DOM
- Searching: getElement*, querySelector*
- Node properties: type, tag and contents
- Attributes and properties
- Modifying the document
- Styles and classes
- Element size and scrolling
- Window sizes and scrolling
- Coordinates
- Introduction to browser events
- Bubbling and capturing
- Event delegation
- Browser default actions
- Dispatching custom events
- Mouse events
- Moving the mouse: mouseover/out, mouseenter/leave
- Drag'n'Drop with mouse events
- Pointer events
- Keyboard: keydown and keyup
- Form properties and methods
- Focusing: focus/blur
- Events: change, input, cut, copy, paste
- Forms: event and method submit
- Page: DOMContentLoaded, load, beforeunload, unload
- Scripts: async, defer
- Resource loading: onload and onerror
- Mutation observer
- Selection and Range
- Event loop: microtasks and macrotasks
Additional articles
- Popups and window methods
- Cross-window communication
- The clickjacking attack
- ArrayBuffer, binary arrays
- TextDecoder and TextEncoder
- File and FileReader
- Fetch: Download progress
- Fetch: Abort
- Fetch: Cross-Origin Requests
- URL objects
- XMLHttpRequest
- Resumable file upload
- Long polling
- Server Sent Events
- Cookies, document.cookie
- LocalStorage, sessionStorage
- Bezier curve
- CSS-animations
- JavaScript animations
- From the orbital height
- Custom elements
- Template element
- Shadow DOM slots, composition
- Shadow DOM styling
- Shadow DOM and events
- Patterns and flags
- Character classes
- Unicode: flag "u" and class \p{...}
- Anchors: string start ^ and end $
- Multiline mode of anchors ^ $, flag "m"
- Word boundary: \b
- Escaping, special characters
- Sets and ranges [...]
- Quantifiers +, *, ? and {n}
- Greedy and lazy quantifiers
- Capturing groups
- Backreferences in pattern: \N and \k<name>
- Alternation (OR) |
- Lookahead and lookbehind
- Catastrophic backtracking
- Sticky flag "y", searching at position
- Methods of RegExp and String
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
40 JavaScript Projects for Beginners – Easy Ideas to Get Started Coding JS
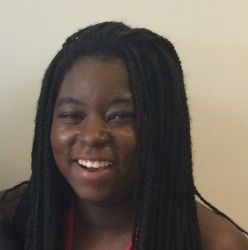
The best way to learn a new programming language is to build projects.
I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript.
My advice for tutorials would be to watch the video, build the project, break it apart and rebuild it your own way. Experiment with adding new features or using different methods.
That will test if you have really learned the concepts or not.
You can click on any of the projects listed below to jump to that section of the article.
Vanilla JavaScript Projects
How to create a color flipper.
- How to create a counter
- How to create a review carousel
- How to create a responsive navbar
- How to create a sidebar
- How to create a modal
How to create a FAQ page
How to create a restaurant menu page, how to create a video background, how to create a navigation bar on scroll, how to create tabs that display different content, how to create a countdown clock, how to create your own lorem ipsum, how to create a grocery list, how to create an image slider, how to create a rock paper scissors game, how to create a simon game, how to create a platformer game.
- How to create Doodle Jump
- How to create Flappy Bird
- How to create a Memory game
- How to create a Whack-a-mole game
- How to create Connect Four game
- How to create a Snake game
- How to create a Space Invaders game
- How to create a Frogger game
- How to create a Tetris game
React Projects
How to build a tic-tac-toe game using react hooks, how to build a tetris game using react hooks, how to create a birthday reminder app.
- How to create a tours page
How to create an accordion menu
How to create tabs for a portfolio page, how to create a review slider, how to create a color generator, how to create a stripe payment menu page, how to create a shopping cart page, how to create a cocktail search page, typescript projects, how to build a quiz app with react and typescript, how to create an arkanoid game with typescript.
If you have not learned JavaScript fundamentals, then I would suggest watching this course before proceeding with the projects.
Many of the screenshots below are from here .

In this John Smilga tutorial , you will learn how to create a random background color changer. This is a good project to get you started working with the DOM.
In Leonardo Maldonado's article on why it is important to learn about the DOM, he states:
By manipulating the DOM, you have infinite possibilities. You can create applications that update the data of the page without needing a refresh. Also, you can create applications that are customizable by the user and then change the layout of the page without a refresh.
Key concepts covered:
- document.getElementById()
- document.querySelector()
- addEventListener()
- document.body.style.backgroundColor
- Math.floor()
- Math.random()
- array.length
Before you get started, I would suggest watching the introduction where John goes over how to access the setup files for all of his projects.
How to create a Counter
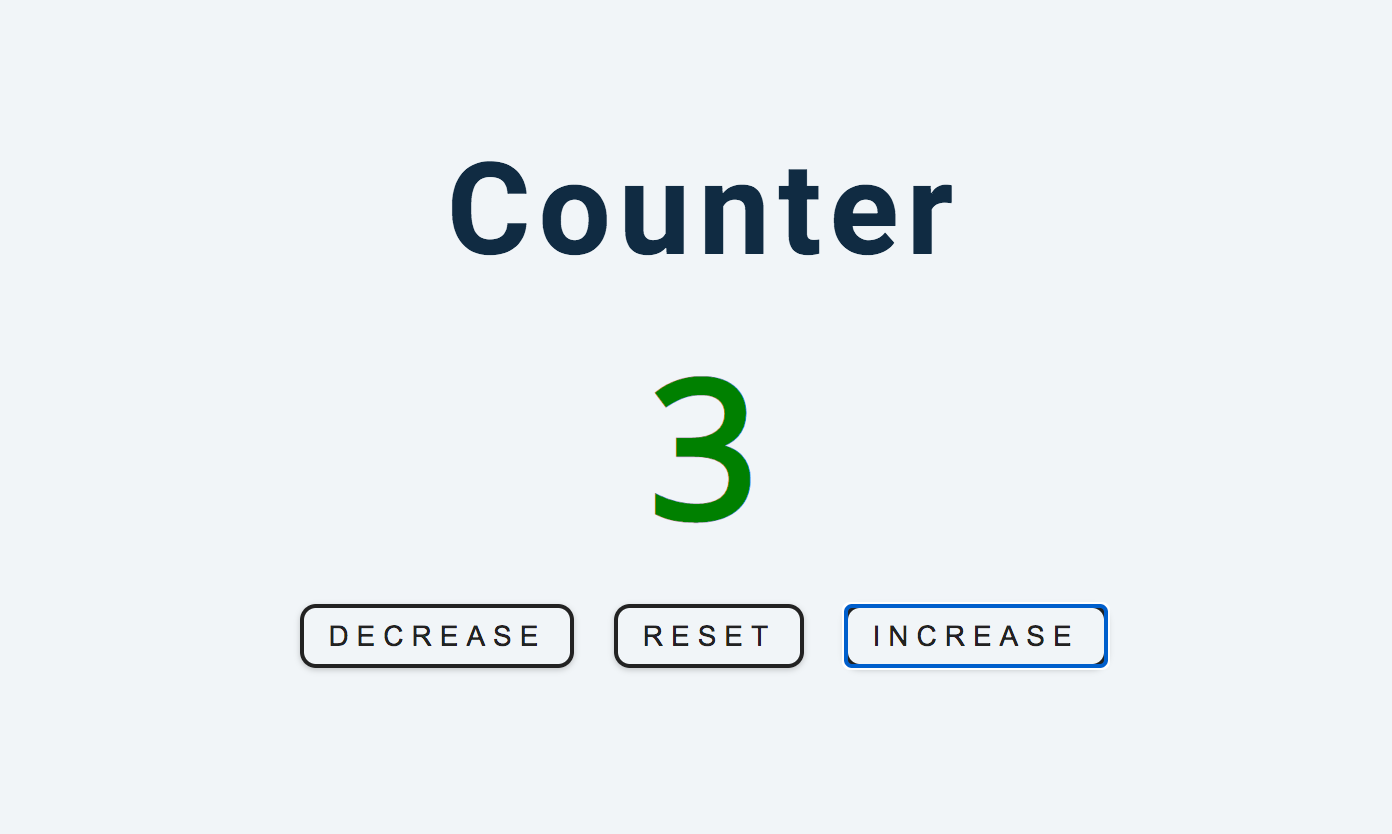
In this John Smilga tutorial , you will learn how to create a counter and write conditions that change the color based on positive or negative numbers displayed.
This project will give you more practice working with the DOM and you can use this simple counter in other projects like a pomodoro clock.
- document.querySelectorAll()
- currentTarget property
- textContent
How to create a Review carousel

In this tutorial , you will learn how to create a carousel of reviews with a button that generates random reviews.
This is a good feature to have on an ecommerce site to display customer reviews or a personal portfolio to display client reviews.
- DOMContentLoaded
How to create a responsive Navbar
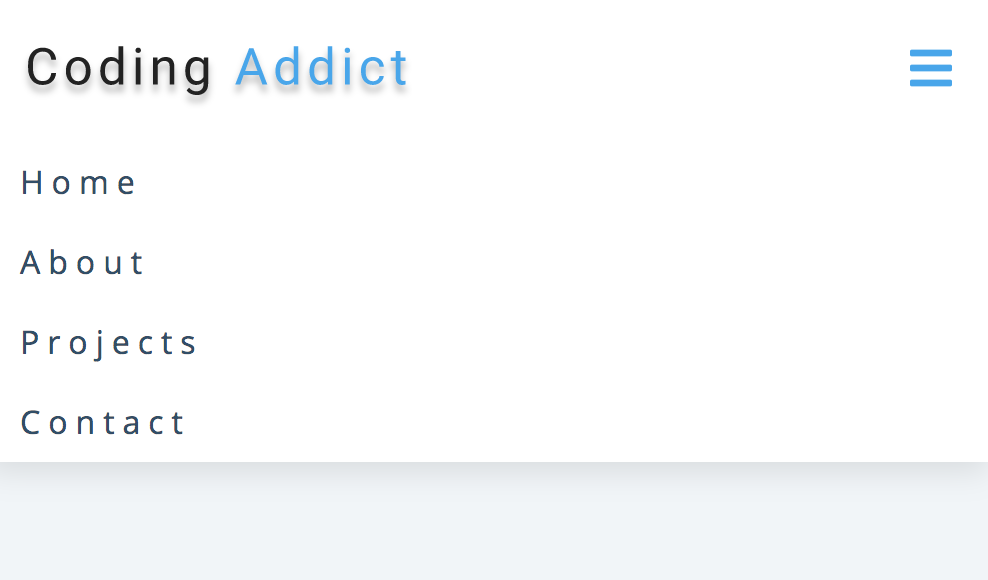
In this tutorial , you will learn how to create a responsive navbar that will show the hamburger menu for smaller devices.
Learning how to develop responsive websites is an important part of being a web developer. This is a popular feature used on a lot of websites.
- classList.toggle()
How to create a Sidebar

In this tutorial , you will learn how to create a sidebar with animation.
This is a cool feature that you can add to your personal website.
- classList.remove()
How to create a Modal
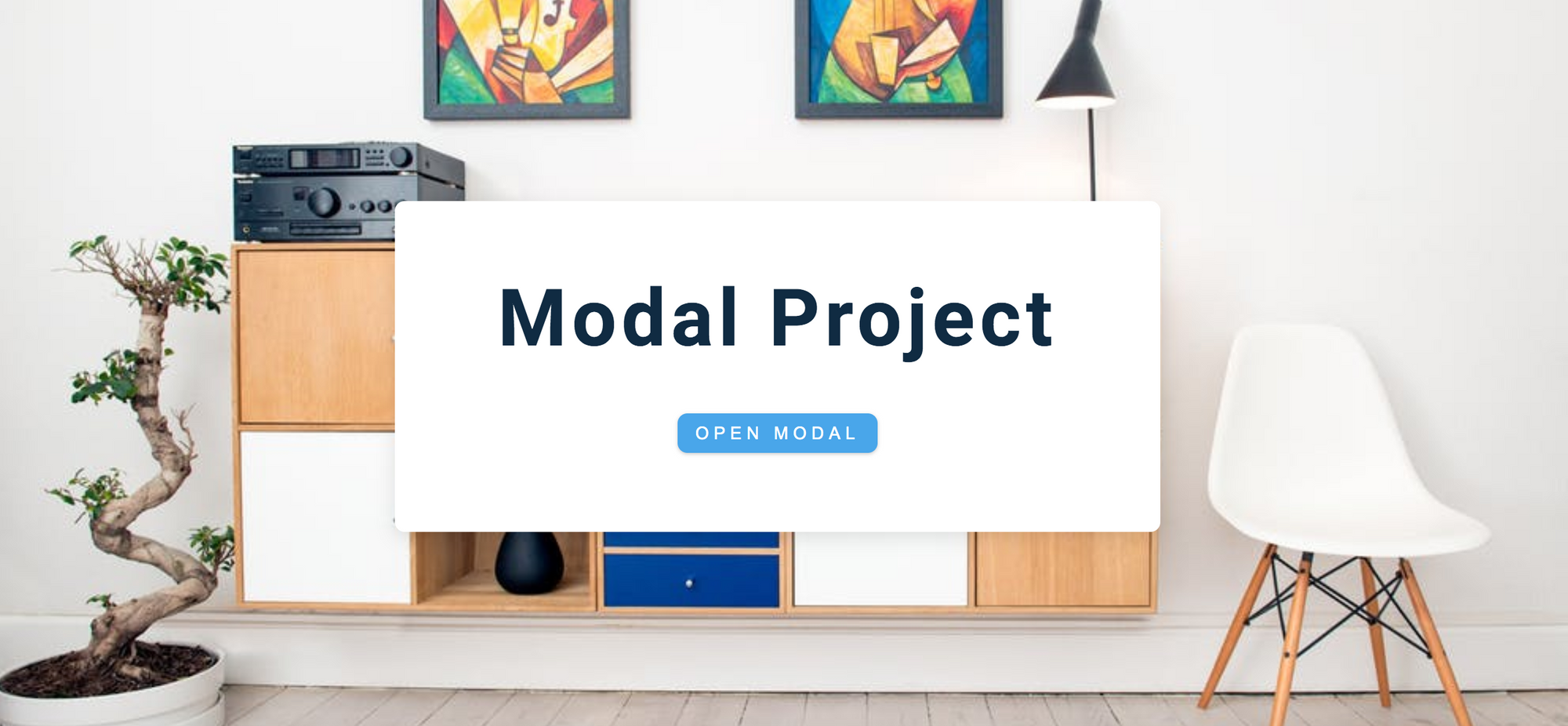
In this tutorial , you will learn how to create a modal window which is used on websites to get users to do or see something specific.
A good example of a modal window would be if a user made changes in a site without saving them and tried to go to another page. You can create a modal window that warns them to save their changes or else that information will be lost.
- classList.add()
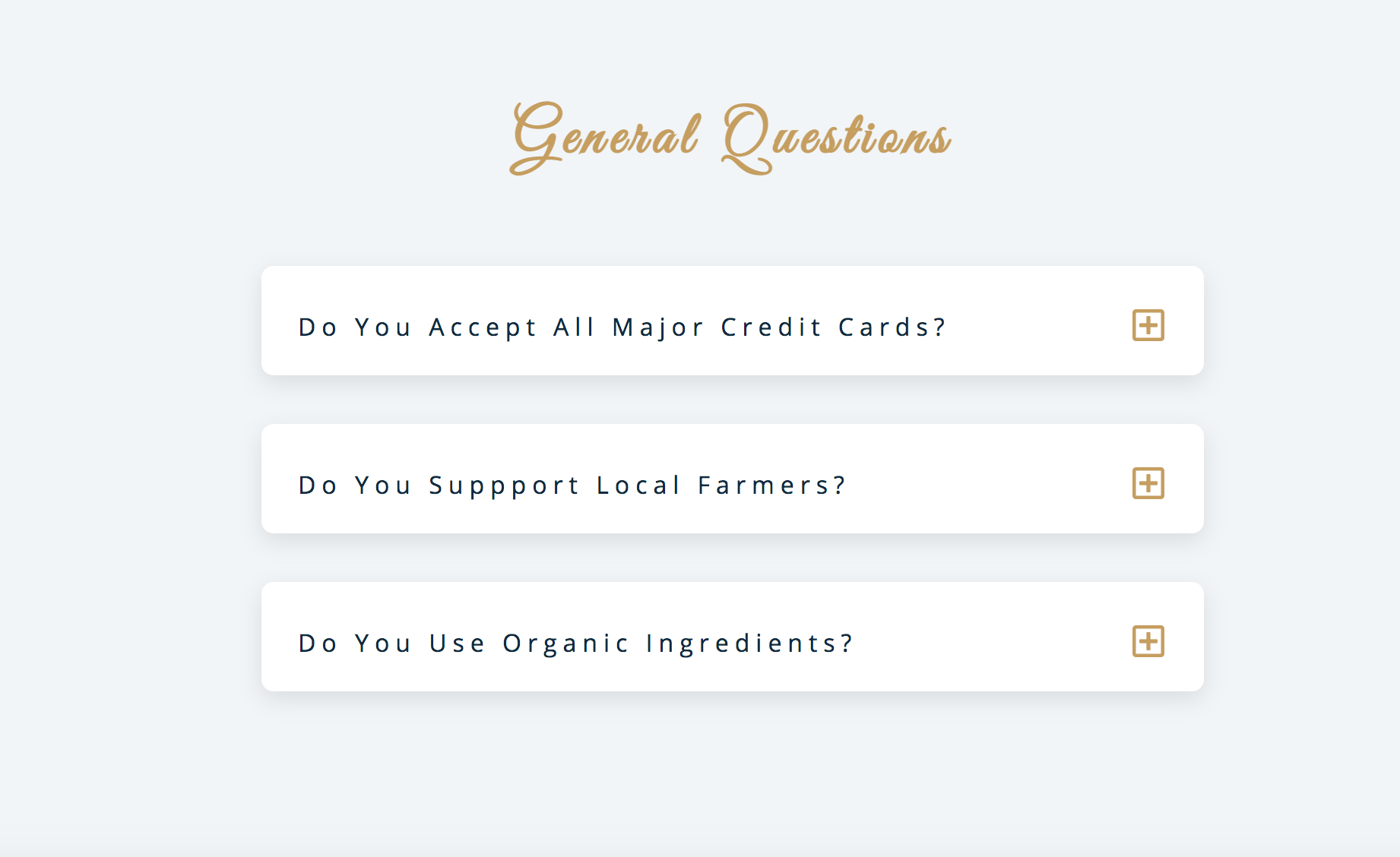
In this tutorial , you will learn how to create a frequently asked questions page which educates users about a business and drives traffic to the website through organic search results.

In this tutorial , you will learn how to make a restaurant menu page that filters through the different food menus. This is a fun project that will teach you higher order functions like map, reduce, and filter.
In Yazeed Bzadough's article on higher order functions, he states:
the greatest benefit of HOFs is greater reusability.
- map, reduce, and filter
- includes method

In this tutorial , you will learn how to make a video background with a play and pause feature. This is a common feature found in a lot of websites.
- classList.contains()
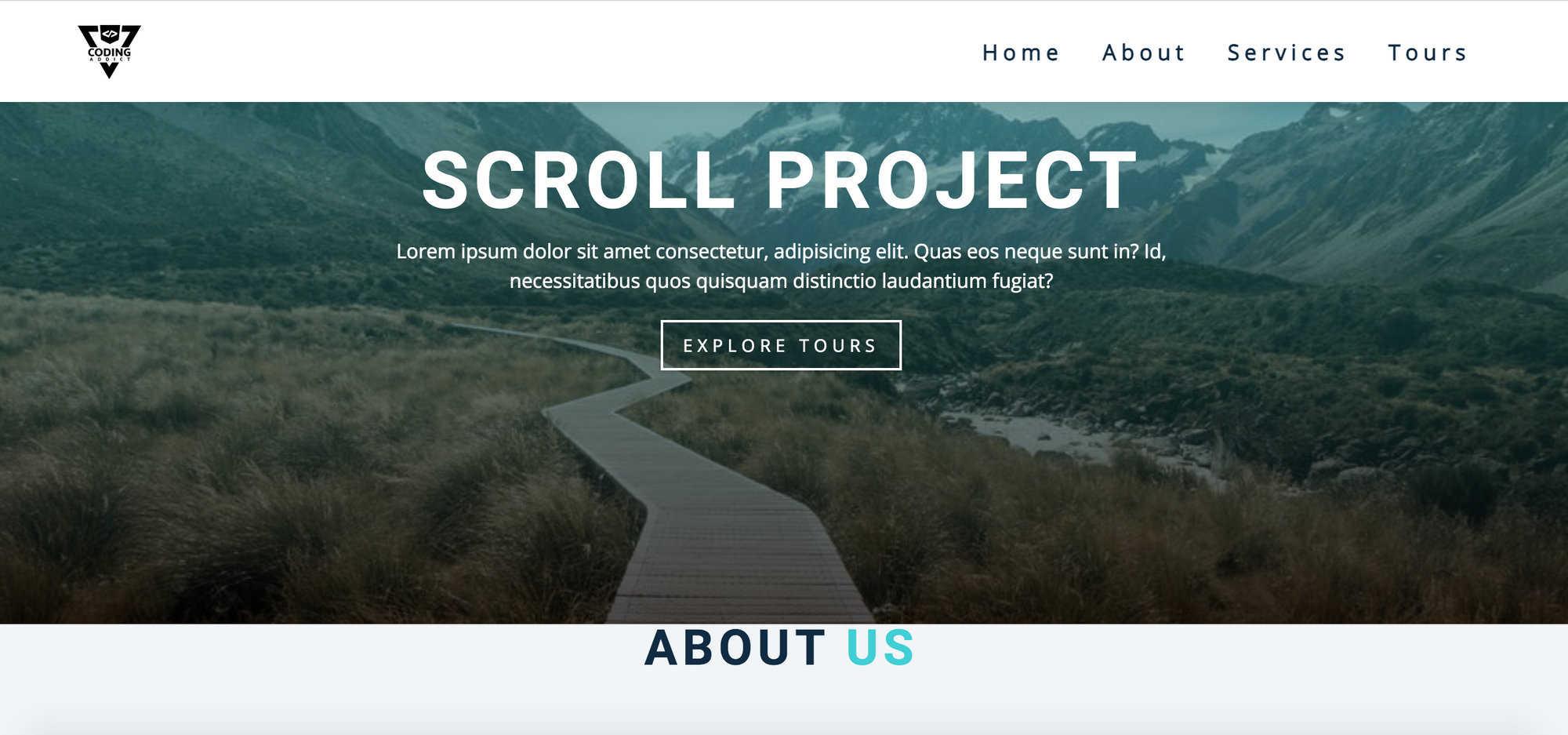
In this tutorial , you will learn how to create a navbar that slides down when scrolling and then stays at a fixed position at a certain height.
This is a popular feature found on many professional websites.
- getFullYear()
- getBoundingClientRect()
- slice method
- window.scrollTo()

In this tutorial , you will learn how to create tabs that will display different content which is useful when creating single page applications.
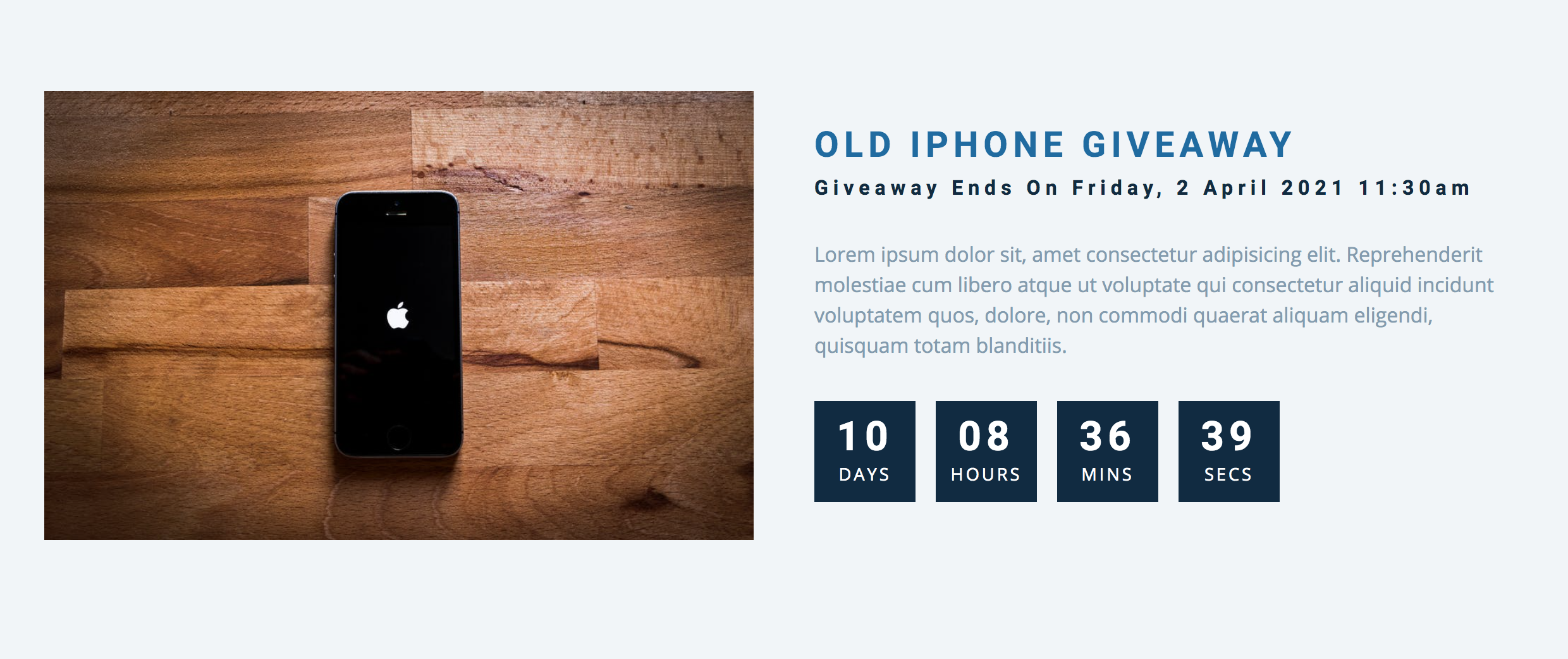
In this tutorial , you will learn how to make a countdown clock which can be used when a new product is coming out or a sale is about to end on an ecommerce site.
- setInterval()
- clearInterval()
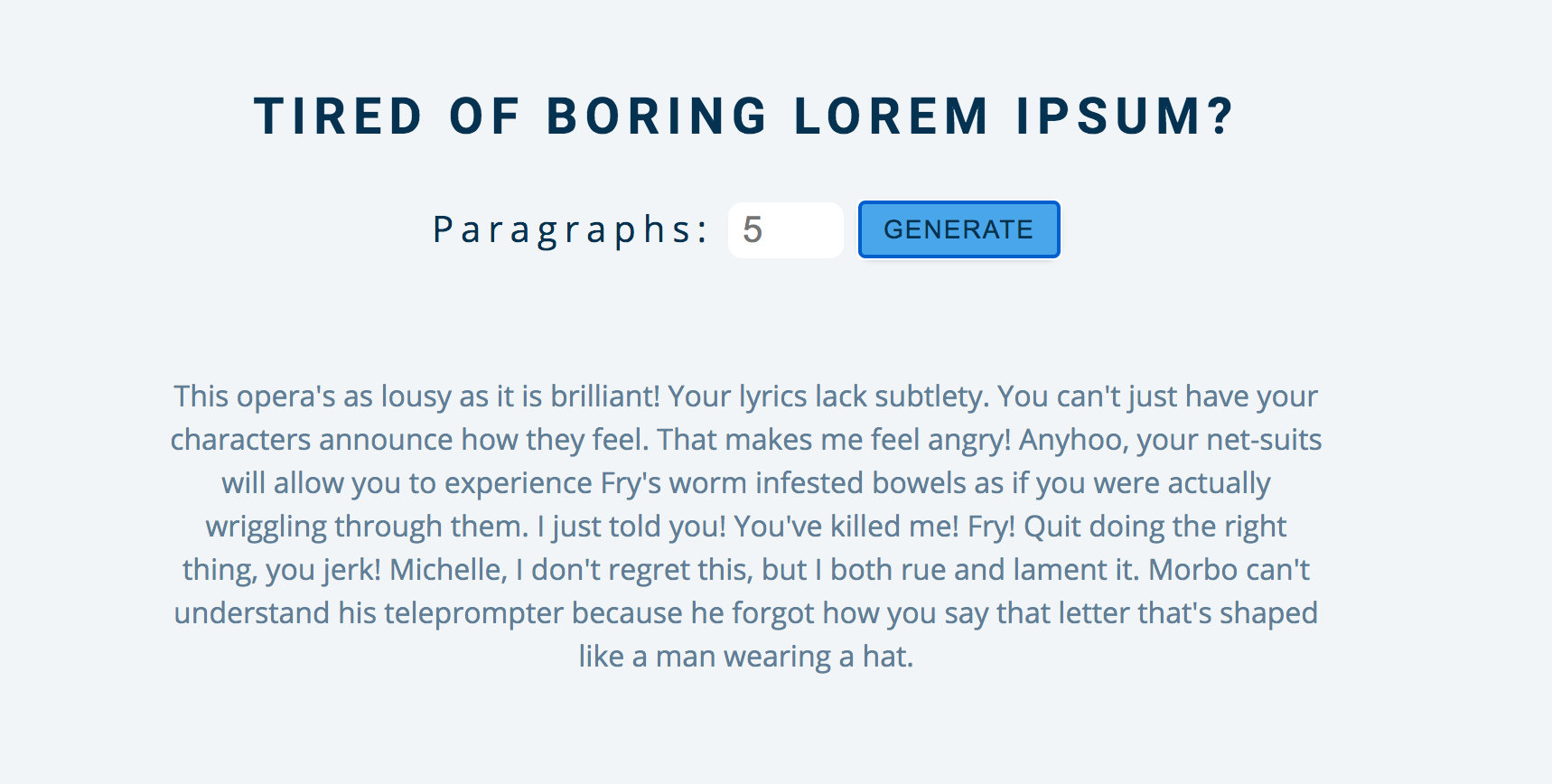
In this tutorial , you will learn how to create your own Lorem ipsum generator.
Lorem ipsum is the go to placeholder text for websites. This is a fun project to show off your creativity and create your own text.
- event.preventDefault()

In this tutorial , you will learn how to update and delete items from a grocery list and create a simple CRUD (Create, Read, Update, and Delete) application.
CRUD plays a very important role in developing full stack applications. Without it, you wouldn't be able to do things like edit or delete posts on your favorite social media platform.
- createAttribute()
- setAttributeNode()
- appendChild()
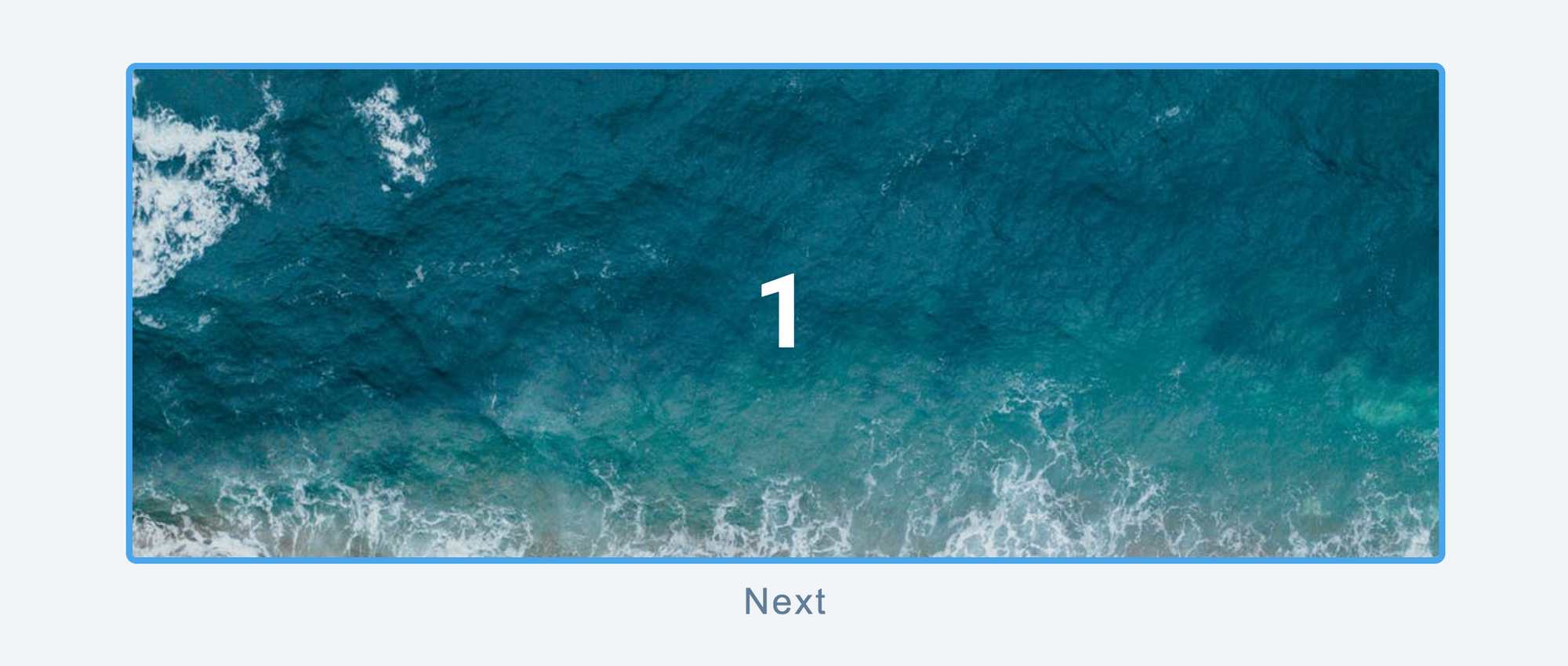
In this tutorial , you will learn how to build an image slider that you can add to any website.
- querySelectorAll()
- if/else statements
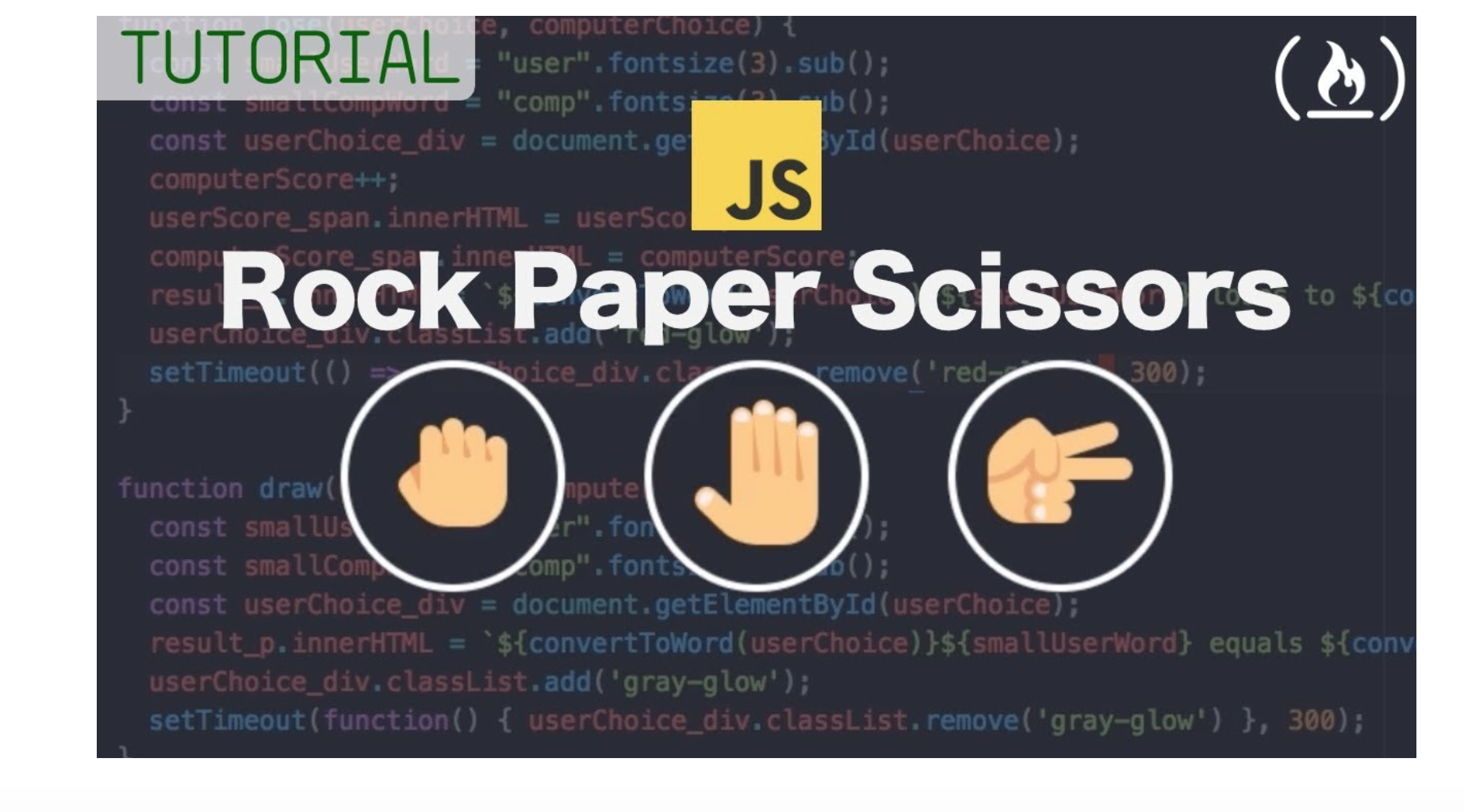
In this tutorial , Tenzin will teach you how to create a Rock Paper Scissors game. This is a fun project that will give more practice working with the DOM.
- switch statements

In this tutorial , Beau Carnes will teach you how to create the classic Simon Game. This is a good project that will get you thinking about the different components behind the game and how you would build out each of those functionalities.
- querySelector()
- setTimeout()
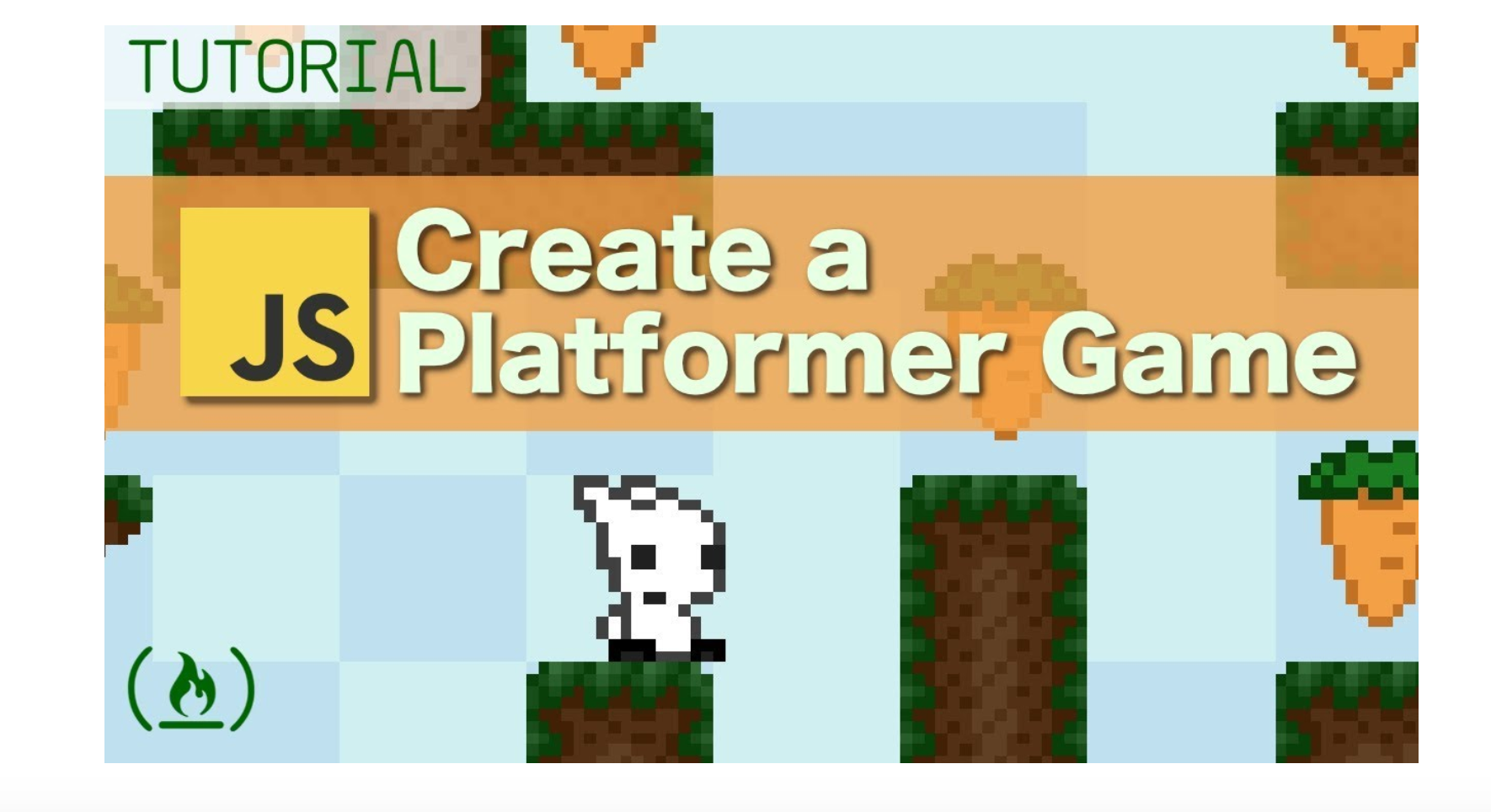
In this tutorial , Frank Poth will teach you how to build a platformer game. This project will introduce you to Object Oriented Programming principles and the Model, View, Controller software pattern.
- this keyword
- OOP principles
- MVC pattern
How to create Doodle Jump and Flappy Bird

In this video series , Ania Kubow will teach you how to build Doodle Jump and Flappy Bird .
Building games are a fun way to learn more about JavaScript and will cover many popular JavaScript methods.
- createElement()
- removeChild()
- removeEventListener()
How to create seven classic games with Ania Kubow

You will have a lot of fun creating seven games in this course by Ania Kubow:
- Memory Game
- Whack-a-mole
- Connect Four
- Space Invaders
- onclick event
- arrow functions
If you are not familiar with React fundamentals, then I would suggest taking this course before proceeding with the projects.
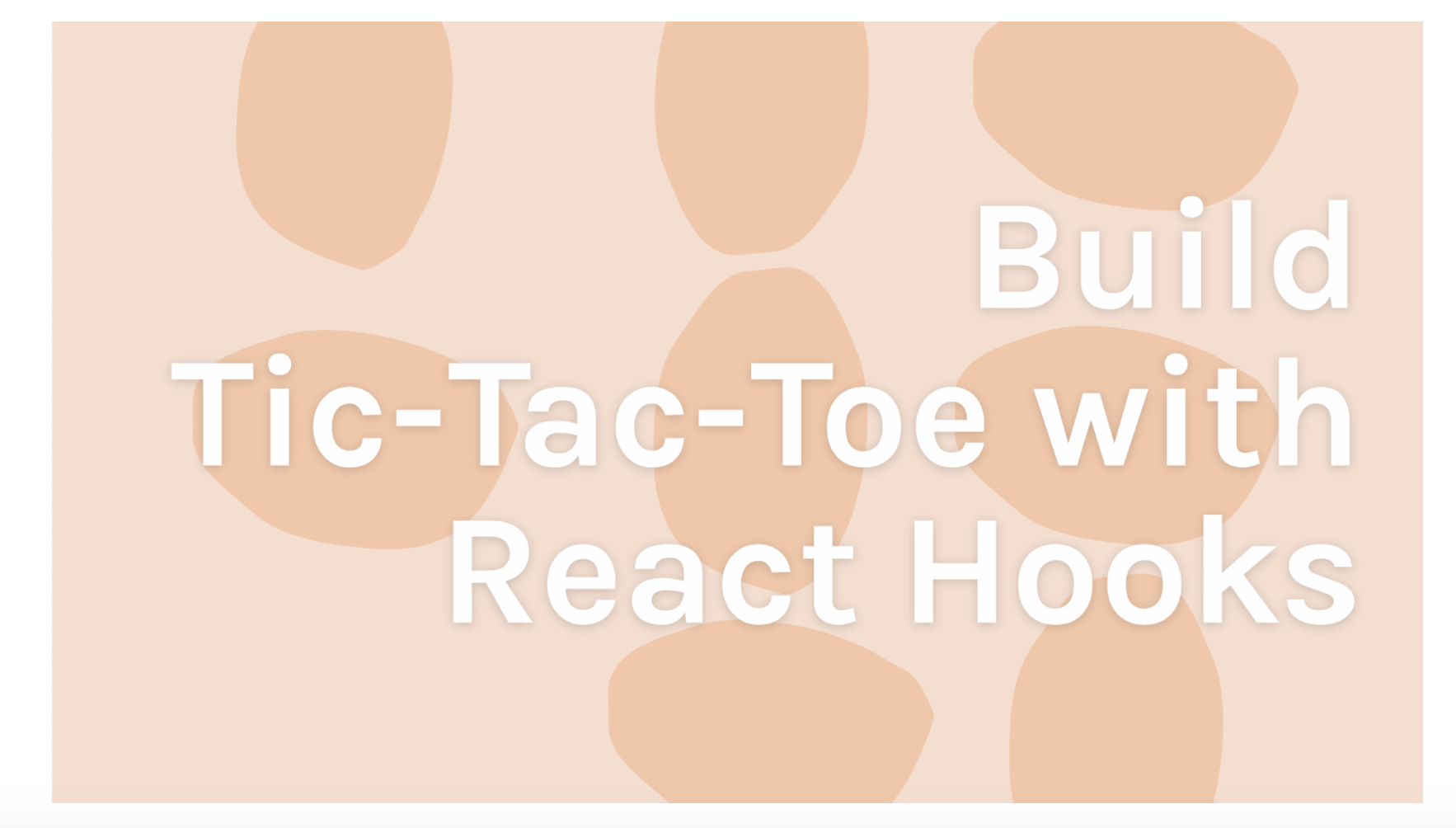
In this freeCodeCamp article , Per Harald Borgen talks about Scrimba's Tic-Tac-Toe game tutorial led by Thomas Weibenfalk. You can view the video course on Scimba's YouTube Channel.
This is a good project to start getting comfortable with React basics and working with hooks.
- import / export
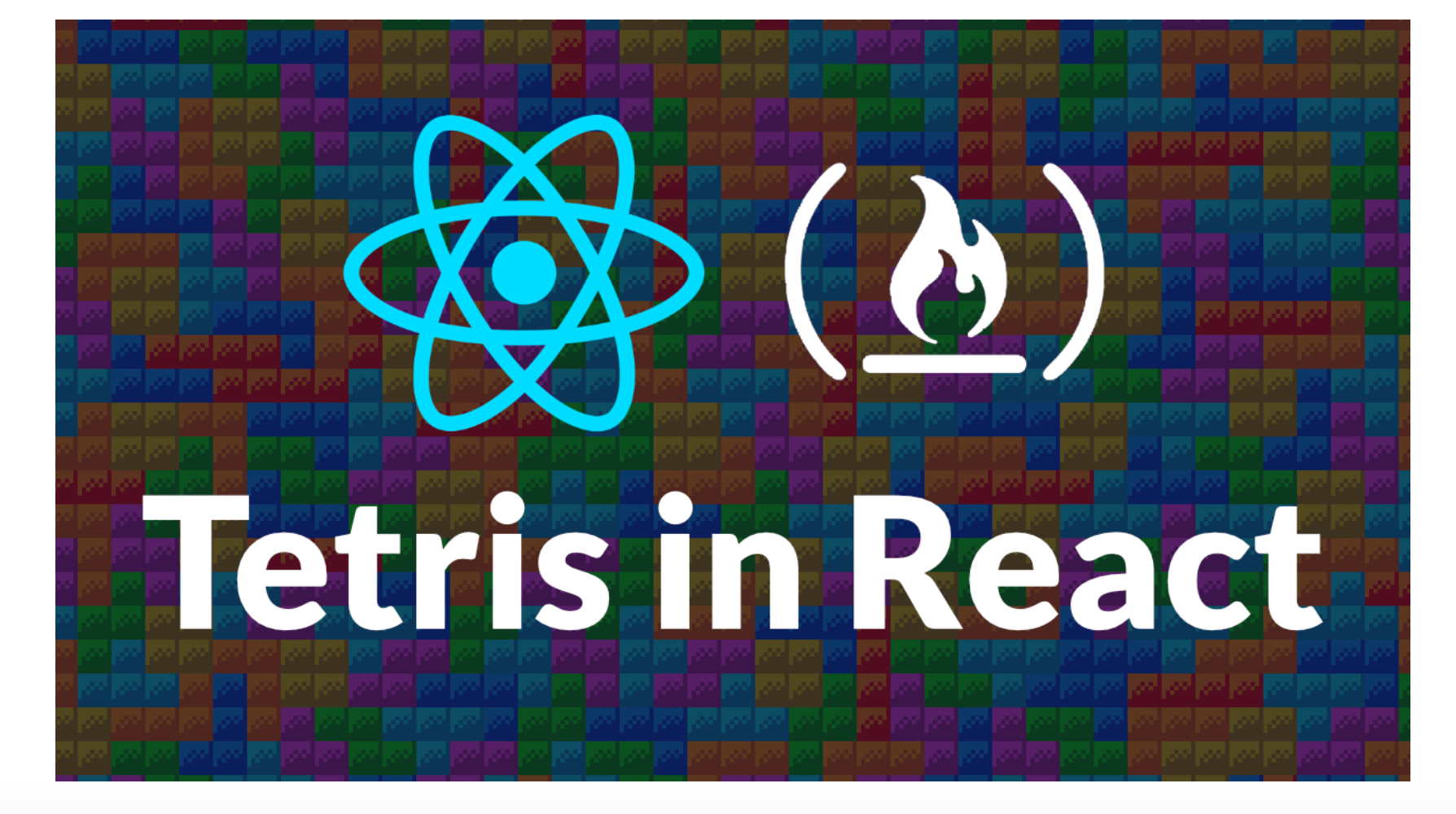
In this tutorial , Thomas Weibenfalk will teach you how to build a Tetris game using React Hooks and styled components.
- useEffect()
- useCallback()
- styled components
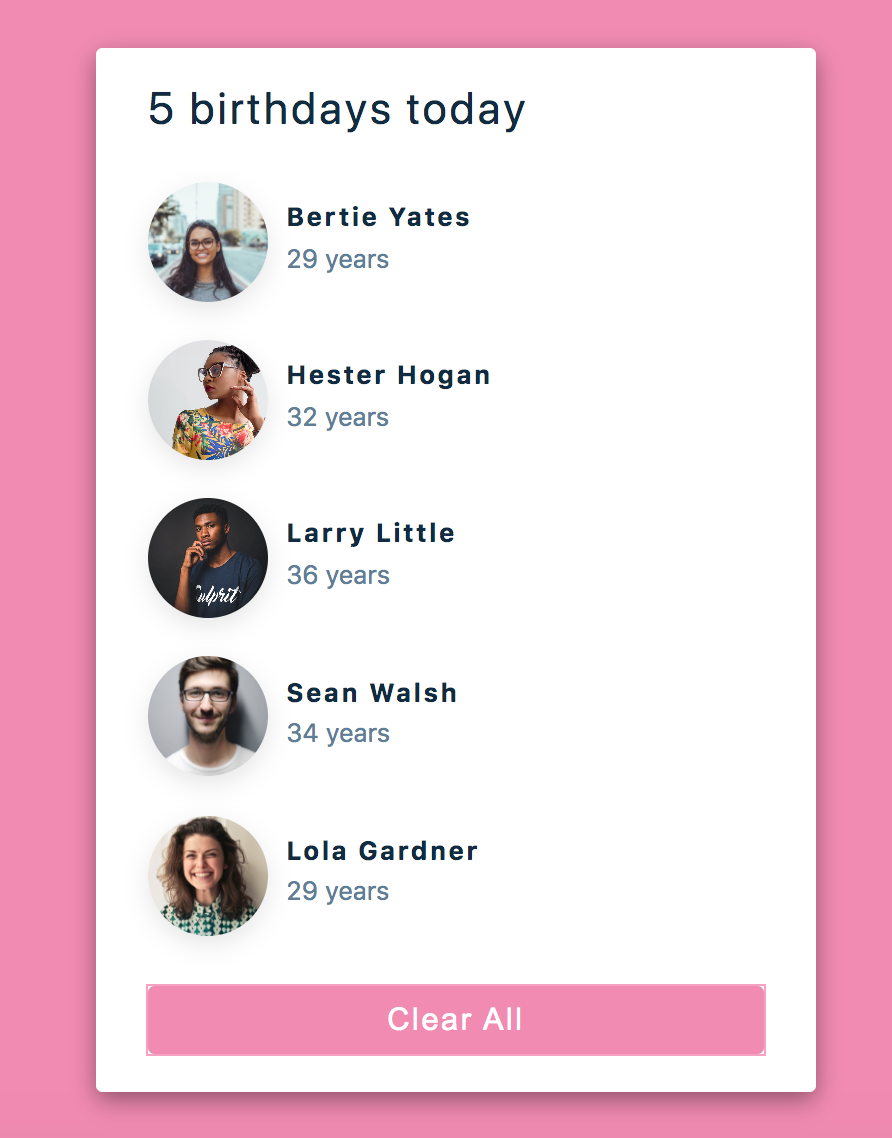
In this John Smilga course, you will learn how to create a birthday reminder app. This is a good project to start getting comfortable with React basics and working with hooks.
I would also suggest watching John's video on the startup files for this project.
How to create a Tours Page

In this tutorial , you will learn how to create a tours page where the user can delete which tours they are not interested in.
This will give you practice with React hooks and the async/await pattern.
- try...catch statement
- async/await pattern

In this tutorial , you will learn how to create a questions and answers accordion menu. These menus can be helpful in revealing content to users in a progressive way.
- React icons

In this tutorial , you will learn how to create tabs for a mock portfolio page. Tabs are useful when you want to display different content in single page applications.

In this tutorial , you will learn how to create a review slider that changes to a new review every few seconds.
This is a cool feature that you can incorporate into an ecommerce site or portfolio.

In this tutorial , you will learn how to create a color generator. This is a good project to continue practicing working with hooks and setTimeout.
- clearTimeout()

In this tutorial , you will learn how to create a Stripe payment menu page. This project will give you good practice on how to design a product landing page using React components.
- useContext()

In this tutorial , you will learn how to create a shopping cart page that updates and deletes items. This project will also be a good introduction to the useReducer hook.
- <svg> elements
- useReducer()
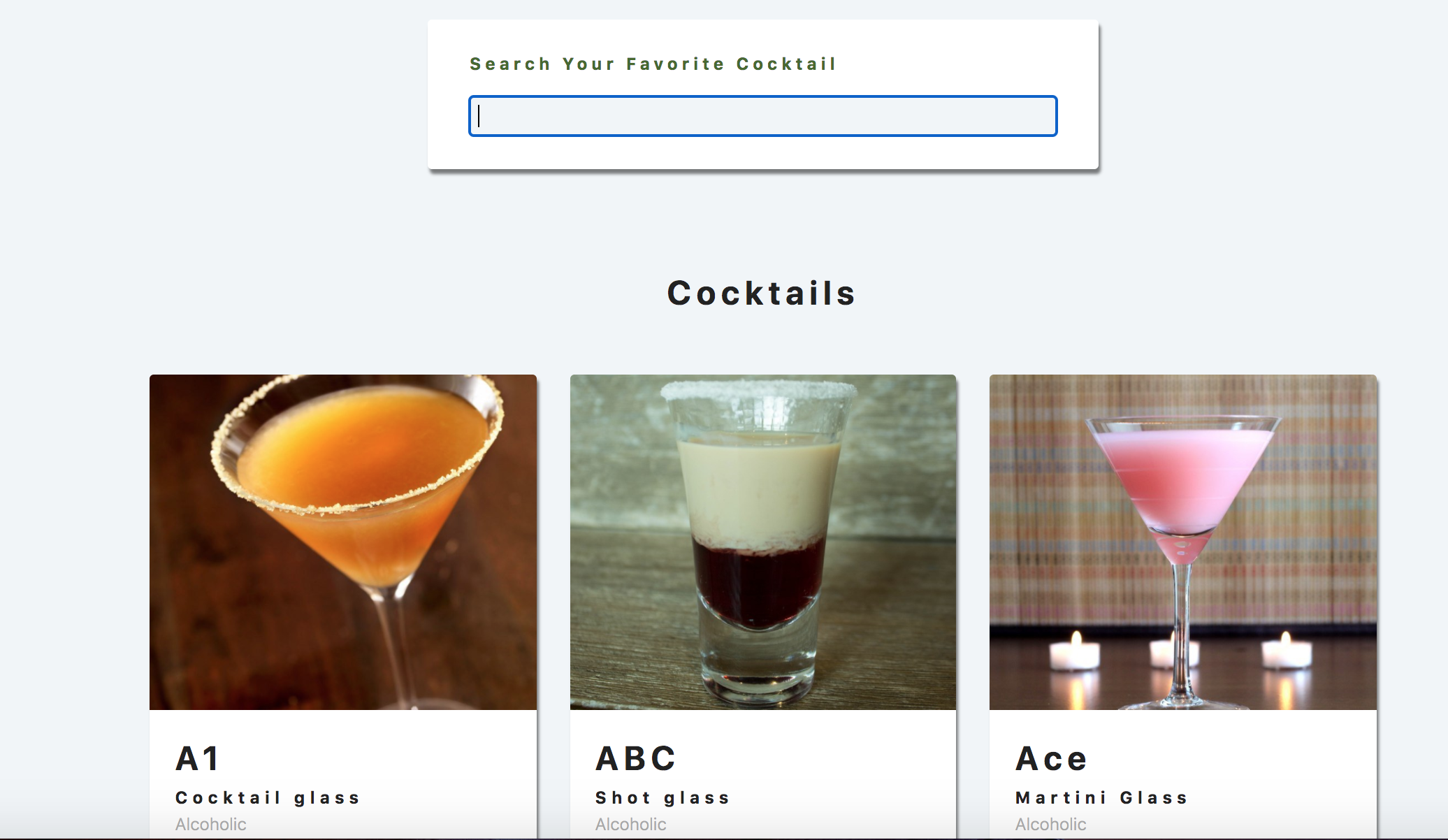
In this tutorial , you will learn how to create a cocktail search page. This project will give you an introduction to how to use React router.
React router gives you the ability to create a navigation on your website and change views to different components like an about or contact page.
- <Router>
- <Switch>
If you are unfamiliar with TypeScript, then I would suggest watching this course before proceeding with this project.
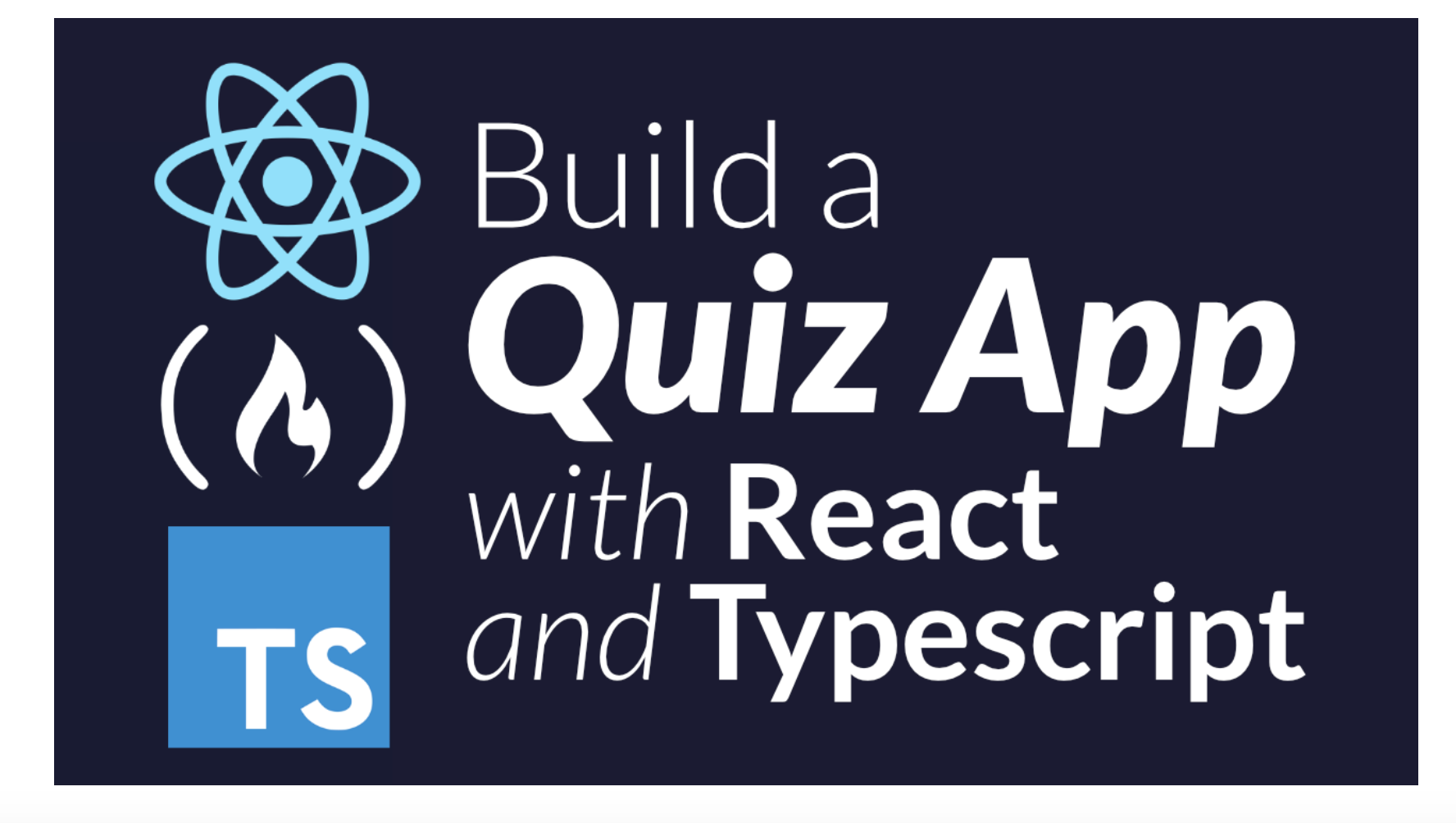
In this tutorial , Thomas Weibenfalk will teach you how to build a quiz app with React and TypeScript. This is a good opportunity to practice the basics of TypeScript.
- dangerouslySetInnerHTML

In this tutorial , Thomas Weibenfalk will teach you how to build the classic Arkanoid game in TypeScript. This is a good project that will give you practice working with the basic concepts for TypeScript.
- HTMLCanvasElement
I hope you enjoy this list of 40 project tutorials in Vanilla JavaScript, React and TypeScript.
Happy coding!
I am a musician and a programmer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
JavaScript Practice Exercises for All Levels
Louis herman content writer.
JavaScript is a language that can be intimidating for newcomers. But it’s actually easier than it seems.
One of the ways to get started is to do practice exercises. But there are so many online that it can be hard to choose the right ones for a beginner.
Before you go and do exercises, it’s best to learn a little about the subject. In this article, we’ll be introducing JavaScript and give you a curated list of the best JavaScript practice exercises to get started.
What is JavaScript?
JavaScript (not to be confused with Java ) scripting programming language that appeared in 1995 and is at the core of the modern World Wide Web. While HTML takes care of the content and structure of a page and CSS of the style, JS is used on the client-side for the behavior of a page—it makes it come to life.
However, JavaScript is more complex than the first two. It lets you do things like:
Auto-update content, like a social media feed
Animate visual elements
Add visual feedback on user interactions
Add interactive maps
Trigger pop-ups/offers after user actions
It’s a high-level, interpreted language just like Python. High-level means that a lot of abstraction is made, so you don’t have to deal with memory management as you would with low-level languages like C or C++. Interpreted means that you don’t need to run it through a compiler, and the language can be directly executed by your computer’s processor.
JavaScript is also multi-paradigm so that you can write your code in a variety of ways, like object-oriented or functional .
Behind JavaScript exist a convention called ECMAScript . It is the scripting language specification it’s based on. It defines language lexicon & syntax and the APIs that should be made available in it.
With time, many frameworks have been developed to improve the functionalities and development of JS. The most popular ones are React (and Next.js its sub-framework), Vue (and Nuxt.js its sub-framework), and Angular.
What can it be used for?
It can be used in the front-end and run on the client-side/browser or in the back-end with node.js.
It’s your browser’s programming language, meaning it's the only language browser can execute. But JavaScript isn't only restricted to websites. With it, you can build:
Complete full-stack web apps
Mobile apps (with React Native or Ionic , for example)
Desktop apps (with Electron.js),
Complete shopping cart solutions
Why learn JavaScript?
Well, as you probably already observed, JavaScript is a powerful programming language that can be used to do a lot of things. It’s also very fast and relatively easy to learn compared to other languages.
Here are the reasons why you should learn JS:
Highly in demand
75% of companies are looking for JavasScript web developers.
As we’ve seen earlier, JavaScript can be used to build almost anything for the modern world. Learning this language will enable you to be versatile in the different tasks you’ll have in your career.
Beginners-friendly
JavaScript is one of the easiest scripting languages out there. A lot of tools for debugging, for example, are also available to make your life as a newcomer a lot easier.
Free to learn
Since it’s one of the most popular programming languages, there are a lot of resources to get started. From books, blog tutorials, and videos, you can learn the way that suits you most.
Learning the JavaScript fundamentals
Before you dive into the JavaScript exercises, it’s best to learn the fundamentals. Here are a few resources I recommend to get started:
If you are an absolute beginner:
Learn JavaScript repository from Snipcart
A first splash into JavaScript from Mozilla
You SHOULD Learn Vanilla JavaScript Before JS Frameworks from Snipcart
Eloquent JavaScript eBook by Marijn Haverbeke
JavaScript exercises for all levels
So, now let’s get into it and explore how to get started when you have understood the basics.
Whatever your JavaScript skills are, here's are some JavaScript coding exercises you can do at your own pace.
1. W3 School JavaScript exercises
67 exercises to practice the JavaScript basics.
2. JavaScript - Exercises, Practice, Solution
Free exercises covering the basics to the more advanced aspect of JS like the DOM (document object modal) async function, and more.
3. Show user IP address with JavaScript
An exercise created by our co-founder Charles. Ideal for beginners to practice the basic fetch function in the CodePen code editor.
4. Add items to a wishlist with JavaScript
An exercise created by our co-founder Charles. Ideal for beginners. You’ll practice creating a simple wishlist with JS in the CodePen code editor.
5. Some JavaScript Challenges
A resource with 47 exercises to practice whatever your level is, With exercises from well-known universities.
6. Debugging Vue.js
Tutorial and exercise on how to debug your Vue.js frontend. Ideal for intermediate developers.
7. Build a React E-Commerce Web App
JavaScript tutorial and exercise on how to create a React dynamic website.
8. Progressive Web Application Development with Nuxt
JavaScript tutorial and exercise on how to build a PWA with a JavaScript framework.
9. Create a Node.js for E-Commerce
Tutorial and exercise about creating a complete eCommerce website using Snipcart, Node.js, and Koa.js as the frontend.
10. 5 Typical JavaScript Interview Exercises
5 exercises to practice for a JavaScript web developer interview.
JavaScript courses
Intro to js: drawing & animation.
This is a free course by Khan Academy. Each section is covered with an explanatory video and then you have exercises to practice what you just learned.
JavaScript Algorithms and Data Structures
Free course by freecodecamp. With 113 exercises that cover the basics of JavaScript.
Learn JavaScript
Free course by Learn JavaScript. Cover the fundamentals with exercises to practice at every step.
This is a course by Code Academy. It’s highly interactive, however, you need a subscription in order to have access to the whole course.
JavaScript courses on mobile
If you are looking for a way to learn and practice JavaScript while on the go, I suggest your look at two free apps.
Grasshopper
This is an app (mobile and web) developed by a team at Google. It’s a fun way to learn JavaScript by doing exercises. The course is divided into small sections that you can do when you have a few minutes.
Mimo is an app to learn web development. While it’s not strictly limited to JavaScript you can select to learn and practice only JavaScript. While they offer a free version, you can also subscribe to Mimo Pro to unlock more exercises.
As you may have noted, learning JavaScript is pretty accessible. Practice exercises are an important part of the learning experience. Because it’s a constantly evolving language, It’s best to keep up to date with the latest developments in the ecosystem. There are even some JavaScript podcasts if you want to learn on the go.
Let us know in the comments how it went if you’ve tried any of the exercises. If you enjoyed it, consider sharing it.

About the author
Louis is a content writer. He is specialized in technology and marketing.
Add a Shopping Cart to a Website in 4 Simple Steps
Recent articles in web development, the 6 best javascript frameworks to use in 2022.

A Beginner's Guide to APIs: How to Integrate and Use Them

5 Best Angular Tutorials for Beginners
Microservices vs. api: the benefits of using microservices, webhook vs. api: how do they compare.

36 000+ geeks are getting our monthly newsletter: join them!
Advanced JavaScript Topics
Discover the depths of advanced JavaScript concepts. Explore closures, ES6, hoisting, OOP, prototypes, and more in this collection of expert-written tutorials.
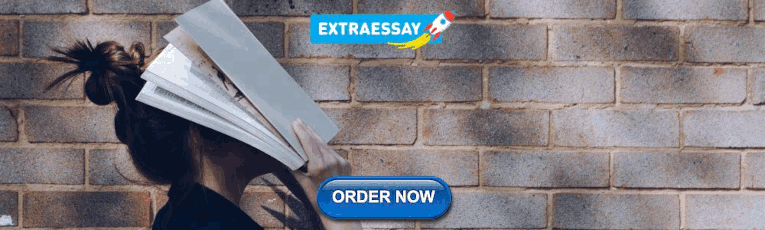
Closures and Scope
Learn the ins and outs of closures and scope in JavaScript. From the basics to advanced techniques, this guide will help you understand how they work and how to use them to write more efficient and maintainable code
ES6 and Beyond
Discover the latest features of ES6 in Javascript and what the future holds for this programming language. Explore code examples and understand the benefits of using the newest version. Stay ahead of the game with this insightful article.
Hoisting in JavaScript
Understand hoisting in JavaScript with our in-depth guide. Learn how hoisting works and avoid common mistakes in your code.
JavaScript Object Oriented Programming
Discover the power of object-oriented programming with Javascript. Learn the fundamentals and explore practical examples.
Prototypes and Inheritance
Discover the power of prototypes and inheritance in JavaScript with our in-depth guide. Learn how they work and gain a solid understanding with real-world examples. Perfect for beginners and advanced programmers alike.
- Skip to main content
- Select language
- Skip to search
- Assignment operators
An assignment operator assigns a value to its left operand based on the value of its right operand.
The basic assignment operator is equal ( = ), which assigns the value of its right operand to its left operand. That is, x = y assigns the value of y to x . The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
Simple assignment operator which assigns a value to a variable. The assignment operation evaluates to the assigned value. Chaining the assignment operator is possible in order to assign a single value to multiple variables. See the example.
Addition assignment
The addition assignment operator adds the value of the right operand to a variable and assigns the result to the variable. The types of the two operands determine the behavior of the addition assignment operator. Addition or concatenation is possible. See the addition operator for more details.
Subtraction assignment
The subtraction assignment operator subtracts the value of the right operand from a variable and assigns the result to the variable. See the subtraction operator for more details.
Multiplication assignment
The multiplication assignment operator multiplies a variable by the value of the right operand and assigns the result to the variable. See the multiplication operator for more details.
Division assignment
The division assignment operator divides a variable by the value of the right operand and assigns the result to the variable. See the division operator for more details.
Remainder assignment
The remainder assignment operator divides a variable by the value of the right operand and assigns the remainder to the variable. See the remainder operator for more details.
Exponentiation assignment
This is an experimental technology, part of the ECMAScript 2016 (ES7) proposal. Because this technology's specification has not stabilized, check the compatibility table for usage in various browsers. Also note that the syntax and behavior of an experimental technology is subject to change in future version of browsers as the spec changes.
The exponentiation assignment operator evaluates to the result of raising first operand to the power second operand. See the exponentiation operator for more details.
Left shift assignment
The left shift assignment operator moves the specified amount of bits to the left and assigns the result to the variable. See the left shift operator for more details.
Right shift assignment
The right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the right shift operator for more details.
Unsigned right shift assignment
The unsigned right shift assignment operator moves the specified amount of bits to the right and assigns the result to the variable. See the unsigned right shift operator for more details.
Bitwise AND assignment
The bitwise AND assignment operator uses the binary representation of both operands, does a bitwise AND operation on them and assigns the result to the variable. See the bitwise AND operator for more details.
Bitwise XOR assignment
The bitwise XOR assignment operator uses the binary representation of both operands, does a bitwise XOR operation on them and assigns the result to the variable. See the bitwise XOR operator for more details.
Bitwise OR assignment
The bitwise OR assignment operator uses the binary representation of both operands, does a bitwise OR operation on them and assigns the result to the variable. See the bitwise OR operator for more details.
Left operand with another assignment operator
In unusual situations, the assignment operator (e.g. x += y ) is not identical to the meaning expression (here x = x + y ). When the left operand of an assignment operator itself contains an assignment operator, the left operand is evaluated only once. For example:
Specifications
Browser compatibility.
- Arithmetic operators
Document Tags and Contributors
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Iterators and generators
- Meta programming
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- ArrayBuffer
- AsyncFunction
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- Intl.Collator
- Intl.DateTimeFormat
- Intl.NumberFormat
- ParallelArray
- ReferenceError
- SIMD.Bool16x8
- SIMD.Bool32x4
- SIMD.Bool64x2
- SIMD.Bool8x16
- SIMD.Float32x4
- SIMD.Float64x2
- SIMD.Int16x8
- SIMD.Int32x4
- SIMD.Int8x16
- SIMD.Uint16x8
- SIMD.Uint32x4
- SIMD.Uint8x16
- SharedArrayBuffer
- StopIteration
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Array comprehensions
- Bitwise operators
- Comma operator
- Comparison operators
- Conditional (ternary) Operator
- Destructuring assignment
- Expression closures
- Generator comprehensions
- Grouping operator
- Legacy generator function expression
- Logical Operators
- Object initializer
- Operator precedence
- Property accessors
- Spread syntax
- async function expression
- class expression
- delete operator
- function expression
- function* expression
- in operator
- new operator
- void operator
- Legacy generator function
- async function
- for each...in
- function declaration
- try...catch
- Arguments object
- Arrow functions
- Default parameters
- Method definitions
- Rest parameters
- constructor
- element loaded from a different domain for which you violated the same-origin policy.">Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the 'delete' operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: More arguments needed
- TypeError: can't access dead object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't redefine non-configurable property "x"
- TypeError: cyclic object value
- TypeError: invalid 'in' operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
- ECMAScript 2015 support in Mozilla
- ECMAScript 5 support in Mozilla
- ECMAScript Next support in Mozilla
- Firefox JavaScript changelog
- New in JavaScript 1.1
- New in JavaScript 1.2
- New in JavaScript 1.3
- New in JavaScript 1.4
- New in JavaScript 1.5
- New in JavaScript 1.6
- New in JavaScript 1.7
- New in JavaScript 1.8
- New in JavaScript 1.8.1
- New in JavaScript 1.8.5
- Documentation:
- All pages index
- Methods index
- Properties index
- Pages tagged "JavaScript"
- JavaScript doc status
- The MDN project
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
- Conditional Statements in JavaScript
- JavaScript Continue Statement
- Garbage Collection in JavaScript
- Introduction to Three.js
- Synchronous and Asynchronous in JavaScript
- JavaScript Label Statement
- JavaScript | DataView()
- Javascript Error and Exceptional Handling With Examples
- Dynamic User Interfaces in JavaScript
- Control Statements in JavaScript
- JavaScript Loops
- Global and Local variables in JavaScript
- Static Methods in JavaScript
- JavaScript Versions
- JavaScript Variables
- Difference Between Variables and Objects in JavaScript
- Interesting Facts About Javascript
- Top 7 One liners of JavaScript
- JavaScript Code Execution
JavaScript Basics
JavaScript is a versatile, lightweight, client-side scripting language used in web development. It can be used for both Client-side as well as Server-side developments. JavaScript is also known as a scripting language for web pages, It supports variables, data types, operators, conditional statements, loops , functions, arrays , and objects . With JavaScript, you can create dynamic, interactive, and engaging websites.
These are the basics that you need to learn before deep dive into JavaScript:
Table of Content
Variables in JavaScript
- Data type in JavaScript
Conditional statements in javascript
Function in javascript, looping in javascript, comments in javascript, operators in javascript.
JavaScript Variables are the building blocks of any programming language. In JavaScript, variables can be used to store reusable values.
List of variables:
Example: Here is the basic example of using var, let, and const variables.
Data types in JavaScript
Data type in JavaScript defines the type of data stored in a variable.
List of data types in javascript
Example: In this example, we are using the above-mentioned data types.
Example: Here is the basic example of using if statement and an else statement and an else if statement.
A function in JavaScript is a block of reusable code that performs a specific task, can take inputs (parameters), and returns a result using the return statement .
Example: In this example, we are performing the addition of two numbers with the help of the javascript function.
Looping in programming languages is a feature that facilitates the execution of a set of instructions/functions repeatedly while some condition evaluates to true.
List of loops in javascript:
Example: Here is the basic example of for loop.
JavaScript comments are used to explain the code to make it more readable. It can be used to prevent the execution of a section of code if necessary.
List of comments
Example: In this example, we are using single-line comments and multiline comments.
J avaScript operators operate the operands, these are symbols that are used to manipulate a certain value or operand. Operators are used to performing specific mathematical and logical computations on operands.
List of operators
Example: Here are some basic examples of the above-mentioned operator.
We have a complete list of JavaScript Operators, to check those, please go through this JavaScript Operators Reference article.
Please Login to comment...
Similar reads.
- javascript-basics
- Web Technologies
- What are Tiktok AI Avatars?
- Poe Introduces A Price-per-message Revenue Model For AI Bot Creators
- Truecaller For Web Now Available For Android Users In India
- Google Introduces New AI-powered Vids App
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- Read Tutorial
- Watch Guide Video
- Complete the Exercise
Now that we've talked about operators. Let's talk about something called the compound assignment operator and I'm going make one little change here in case you're wondering if you ever want to have your console take up the entire window you come up to the top right-hand side here you can undock it into a separate window and you can see that it takes up the entire window.
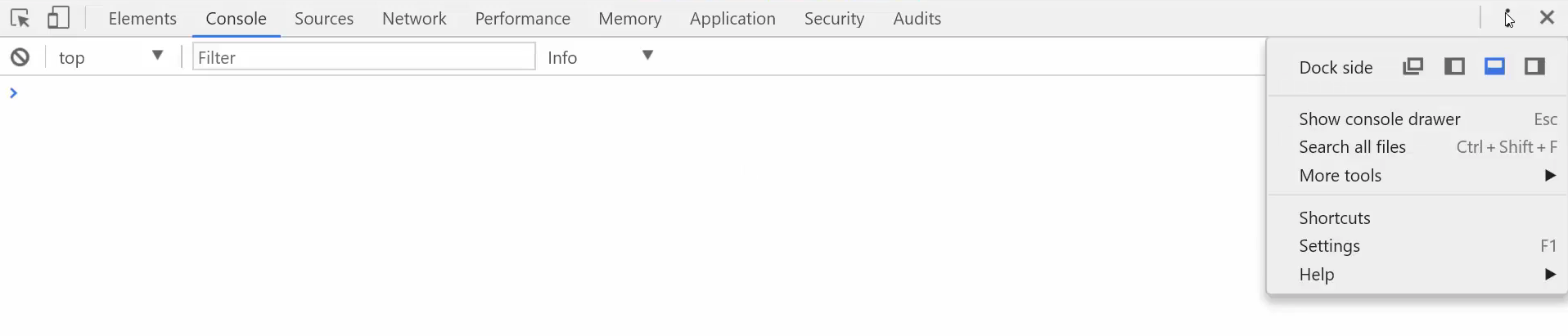
So just a little bit more room now.
Additionally, I have one other thing I'm going to show you in the show notes. I'm going to give you access to this entire set of assignment operators but we'll go through a few examples here. I'm going to use the entire window just to make it a little bit easier to see.
Let's talk about what assignment is. Now we've been using assignment ever since we started writing javascript code. You're probably pretty used to it. Assignment is saying something like var name and then setting up a name
And that is assignment the equals represents assignment.
Now javascript gives us the ability to have the regular assignment but also to have that assignment perform tasks. So for example say that you want to add items up so say that we want to add up a total set of grades to see the total number of scores. I can say var sum and assign it equal to zero.
And now let's create some grades.
I'm going to say var gradeOne = 100.
and then var gradeTwo = 80.
Now with both of these items in place say that we wanted to add these if you wanted to just add both of them together you definitely could do something like sum = (gradeOne + gradeTwo); and that would work.
However, one thing I want to show you is, there are many times where you don't have gradeOne or gradeTwo in a variable. You may have those stored in a database and then you're going to loop through that full set of records. And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do.
Let's use one of the more basic ones which is to have the addition assignment.
Now you can see that sum is equal to 100.
Then if I do
If we had 100 grades we could simply add them just like that.
Essentially what this is equal to is it's a shorthand for saying something like
sum = sum + whatever the next one is say, that we had a gradeThree, it would be the same as doing that. So it's performing assignment, but it also is performing an operation. That's the reason why it's called a compound assignment operator.
Now in addition to having the ability to sum items up, you could also do the same thing with the other operators. In fact literally, every one of the operators that we just went through you can use those in order to do this compound assignment. Say that you wanted to do multiplication you could do sum astrix equals and then gradeTwo and now you can see it equals fourteen thousand four hundred.
This is that was the exact same as doing sum = whatever the value of sum was times gradeTwo. That gives you the exact same type of process so that is how you can use the compound assignment operators. And if you reference the guide that is included in the show notes. You can see that we have them for each one of these from regular equals all the way through using exponents.
Then for right now don't worry about the bottom items. These are getting into much more advanced kinds of fields like bitwise operators and right and left shift assignments. So everything you need to focus on is actually right at the top for how we're going to be doing this. This is something that you will see in a javascript code. I wanted to include it, so when you see it you're not curious about exactly what's happening.
It's a great shorthand syntax for whenever you want to do assignment but also perform an operation at the same time.
- Documentation for Compound Assignment Operators
- Source code
devCamp does not support ancient browsers. Install a modern version for best experience.
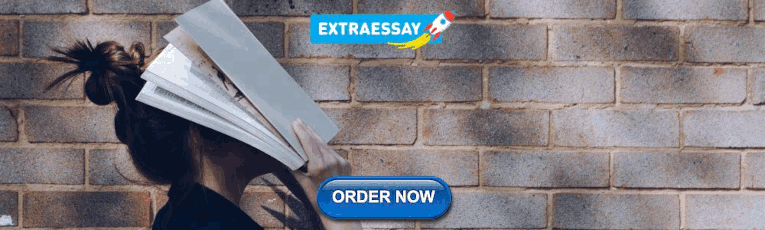
IMAGES
VIDEO
COMMENTS
JavaScript is a cross-platform, object-oriented scripting language. It is a small and lightweight language. Inside a host environment ( a web browser), JavaScript can be connected to the objects of its environment to provide programmatic control over them. JavaScript contains a standard library of objects, such as Array, Date, and Math, and a ...
Embark on your JavaScript learning journey with our online practice portal. Start by selecting quizzes tailored to your skill level. Engage in hands-on coding exercises, receive real-time feedback, and monitor your progress. With our user-friendly platform, mastering JavaScript becomes an enjoyable and personalized experience.
How Edabit Works. This is an introduction to how challenges on Edabit work. In the Code tab above you'll see a starter function that looks like this: function hello () { } All you have to do is type return "hello edabit.com" between the curly braces { } and then click the Check button. If you did this correctly, the button will turn red and ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
JavaScript Programming Essentials. This course is part of multiple programs. Learn more ... Access to lectures and assignments depends on your type of enrollment. If you take a course in audit mode, you will be able to see most course materials for free. To access graded assignments and to earn a Certificate, you will need to purchase the ...
Develop fluency in 69 programming languages with our unique blend of learning, practice and mentoring. Exercism is fun, effective and 100% free, forever. Explore the 146 JavaScript exercises on Exercism.
JSchallenger recognizes what skill level you're on and adjusts the difficulty of the next challenges automatically. Making you continuously improve your Javascript skills in a short amount of time. JSchallenger. Free Javascript challenges. Learn Javascript online by solving coding exercises. Javascript for all levels.
Catastrophic backtracking. Sticky flag "y", searching at position. Methods of RegExp and String. Modern JavaScript Tutorial: simple, but detailed explanations with examples and tasks, including: closures, document and events, object oriented programming and more.
JavaScript is the world's most popular programming language. JavaScript is the programming language of the Web. JavaScript is easy to learn. This tutorial will teach you JavaScript from basic to advanced. Start learning JavaScript now »
JavaScript is the programming language that powers the modern web. In this course, you will learn the basic concepts of web development with JavaScript. You will work with functions, objects, arrays, variables, data types, the HTML DOM, and much more. ... 2 videos 2 readings 2 quizzes 1 programming assignment 2 discussion prompts.
40 JavaScript Projects for Beginners - Easy Ideas to Get Started Coding JS. The best way to learn a new programming language is to build projects. I have created a list of 40 beginner friendly project tutorials in Vanilla JavaScript, React, and TypeScript. My advice for tutorials would be to watch the video, build the project, break it apart ...
Explore our Javascript Projects for practical assignments in web development, front-end and back-end programming, data structures, algorithms, and API integrations. These projects are designed to hone your coding skills and prepare you for a vibrant career in Javascript programming.
Free exercises covering the basics to the more advanced aspect of JS like the DOM (document object modal) async function, and more. 3. Show user IP address with JavaScript. An exercise created by our co-founder Charles. Ideal for beginners to practice the basic fetch function in the CodePen code editor. 4.
Discover the power of prototypes and inheritance in JavaScript with our in-depth guide. Learn how they work and gain a solid understanding with real-world examples. Perfect for beginners and advanced programmers alike. Discover the depths of advanced JavaScript concepts. Explore closures, ES6, hoisting, OOP, prototypes, and more in this ...
This chapter describes JavaScript's expressions and operators, including assignment, comparison, arithmetic, bitwise, logical, string, ternary and more. At a high level, an expression is a valid unit of code that resolves to a value. There are two types of expressions: those that have side effects (such as assigning values) and those that ...
Assignment (=) The assignment ( =) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
An assignment operator assigns a value to its left operand based on the value of its right operand.. Overview. The basic assignment operator is equal (=), which assigns the value of its right operand to its left operand.That is, x = y assigns the value of y to x.The other assignment operators are usually shorthand for standard operations, as shown in the following definitions and examples.
JavaScript is one of the core technologies of the World Wide Web. In this course, you will learn to use JavaScript to add behavior to your web pages. You will create web content that is more interactive by adding animations, menus, scrolling text, interactive maps, and other kinds of lively content. ... 2 programming assignments ...
JavaScript Basics. JavaScript is a versatile, lightweight, client-side scripting language used in web development. It can be used for both Client-side as well as Server-side developments. JavaScript is also known as a scripting language for web pages, It supports variables, data types, operators, conditional statements, loops, functions, arrays ...
W3Schools offers free online tutorials, references and exercises in all the major languages of the web. Covering popular subjects like HTML, CSS, JavaScript, Python, SQL, Java, and many, many more.
The addition assignment (+=) operator performs addition (which is either numeric addition or string concatenation) on the two operands and assigns the result to the left operand. Try it Syntax
JavaScript programming assignments can be challenging, but they are also an opportunity for growth. Seek help when needed, practice consistently, and stay updated with the latest trends to excel ...
And so you need to be able to add them on the fly. And so that's what a compound assignment operator can do. Let's use one of the more basic ones which is to have the addition assignment. sum += gradeOne; // 100. Now you can see that sum is equal to 100. Then if I do. sum += gradeTwo; // 180.