Introduction To OS
- Introduction to Operating System
- Evolution of Operating System
- Types of Operating System
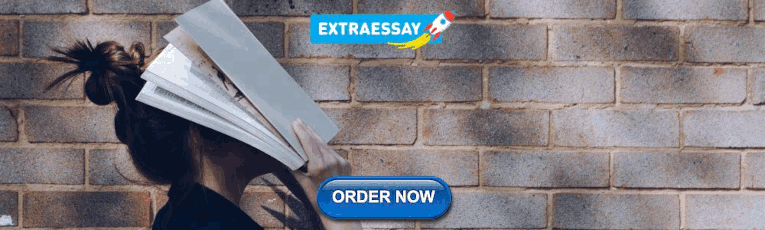
Process & MultiThreading
- Operating System Processes
- Process Scheduling
CPU Scheduling
- First Come First Serve
- Shortest Job First
- Priority Scheduling
- Round Robin Scheduling
- Multilevel Queue Scheduling
- Multilevel Feedback Queue Scheduling
- Comparision of Scheduling Algorithms
- Introduction to Threads
- Process Synchronization
- Classical Synchronization Problems
- Bounded Buffer Problem
- Dining Philosophers Problem
- Readers Writer Problem
- Semaphores in OS
- Classical Problems of Synchronization
- Deadlock Prevention in OS
- Deadlock Avoidance in OS
- Deadlock Detection and Recovery
- HRRN Scheduling
- Shortest Remaining Time First
- Longest Job First Scheduling
- Longest Remaining Time First Scheduling
- Memory Management
- Partition Allocation Methods
- Virtual Memory
- File System
- Banker's Algorithm
- Secondary Storage
- Resource Allocation Graph
- System Calls
- Logical and Physical Address
- Swapping in OS
- Contiguous Memory Allocation
- Paging in OS
- Page Table in OS
- Segmentation in OS
- Paging Vs Segmentation
- Contiguous Vs Non-Contiguous
- Paging Vs Swapping
- Internal Vs External Fragmentation
- Virtual Memory in OS
- Demand Paging in OS
- Copy on Write in OS
- Page Fault in OS
- Page Replacement Algorithm
- Thrashing in OS
Banker's Algorithm in Operating System
Banker's algorithm is a deadlock avoidance algorithm . It is named so because this algorithm is used in banking systems to determine whether a loan can be granted or not.
Consider there are n account holders in a bank and the sum of the money in all of their accounts is S . Every time a loan has to be granted by the bank, it subtracts the loan amount from the total money the bank has. Then it checks if that difference is greater than S . It is done because, only then, the bank would have enough money even if all the n account holders draw all their money at once.
Banker's algorithm works in a similar way in computers.
Whenever a new process is created, it must specify the maximum instances of each resource type that it needs, exactly.
Characteristics of Banker's Algorithm
The characteristics of Banker's algorithm are as follows:
If any process requests for a resource, then it has to wait.
This algorithm consists of advanced features for maximum resource allocation.
There are limited resources in the system we have.
In this algorithm, if any process gets all the needed resources, then it is that it should return the resources in a restricted period.
Various resources are maintained in this algorithm that can fulfill the needs of at least one client.
Let us assume that there are n processes and m resource types.
Data Structures used to implement the Banker’s Algorithm
Some data structures that are used to implement the banker's algorithm are:
1. Available
It is an array of length m . It represents the number of available resources of each type. If Available[j] = k , then there are k instances available, of resource type Rj .
It is an n x m matrix which represents the maximum number of instances of each resource that a process can request. If Max[i][j] = k , then the process Pi can request atmost k instances of resource type Rj .
3. Allocation
It is an n x m matrix which represents the number of resources of each type currently allocated to each process. If Allocation[i][j] = k , then process Pi is currently allocated k instances of resource type Rj .
It is a two-dimensional array. It is an n x m matrix which indicates the remaining resource needs of each process. If Need[i][j] = k , then process Pi may need k more instances of resource type Rj to complete its task.
Banker’s algorithm comprises of two algorithms:
Safety algorithm
Resource request algorithm
Safety Algorithm
A safety algorithm is an algorithm used to find whether or not a system is in its safe state. The algorithm is as follows:
This means, initially, no process has finished and the number of available resources is represented by the Available array.
If there is no such i present, then proceed to step 4.
It means, we need to find an unfinished process whose needs can be satisfied by the available resources. If no such process exists, just go to step 4.
Go to step 2.
When an unfinished process is found, then the resources are allocated and the process is marked finished. And then, the loop is repeated to check the same for all other processes.
That means if all processes are finished, then the system is in safe state.
This algorithm may require an order of mxn² operations in order to determine whether a state is safe or not.
Resource Request Algorithm
Now the next algorithm is a resource-request algorithm and it is mainly used to determine whether requests can be safely granted or not.
Let Requesti be the request vector for the process Pi. If Requesti[j]==k, then process Pi wants k instance of Resource type Rj.When a request for resources is made by the process Pi, the following are the actions that will be taken:
1. If Requesti <= Needi, then go to step 2;else raise an error condition, since the process has exceeded its maximum claim.
2.If Requesti <= Availablei then go to step 3; else Pi must have to wait as resources are not available.
3.Now we will assume that resources are assigned to process Pi and thus perform the following steps:
Available= Available-Requesti;
Allocationi=Allocationi +Requesti;
Needi =Needi - Requesti;
If the resulting resource allocation state comes out to be safe, then the transaction is completed and, process Pi is allocated its resources. But in this case, if the new state is unsafe, then Pi waits for Requesti, and the old resource-allocation state is restored.
Disadvantages of Banker's Algorithm
Some disadvantages of this algorithm are as follows:
During the time of Processing, this algorithm does not permit a process to change its maximum need.
Another disadvantage of this algorithm is that all the processes must know in advance about the maximum resource needs.
This algorithm permits the requests to be provided in constrained time, but for one year which is a fixed period.
Now its time to take a look at the Example of Banker's Algorithm:
Let us consider the following snapshot for understanding the banker's algorithm:
- calculate the content of the need matrix?
- Check if the system is in a safe state?
- Determine the total sum of each type of resource?
1 . The Content of the need matrix can be calculated by using the formula given below:
Need = Max – Allocation
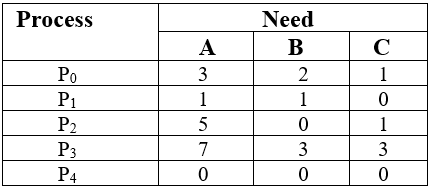
Safe sequence:
- For process P0, Need = (3, 2, 1) and
Available = (2, 1, 0)
Need <=Available = False
So, the system will move to the next process.
2. For Process P1, Need = (1, 1, 0)
Need <= Available = True
Request of P1 is granted.
Available = Available +Allocation
= (2, 1, 0) + (2, 1, 2)
= (4, 2, 2) (New Available)
3. For Process P2, Need = (5, 0, 1)
Available = (4, 2, 2)
4. For Process P3, Need = (7, 3, 3)
5. For Process P4, Need = (0, 0, 0)
Request of P4 is granted.
Available = Available + Allocation
= (4, 2, 2) + (1, 1, 2)
= (5, 3, 4) now, (New Available)
6. Now again check for Process P2, Need = (5, 0, 1)
Available = (5, 3, 4)
Request of P2 is granted.
= (5, 3, 4) + (4, 0, 1)
= (9, 3, 5) now, (New Available)
7. Now again check for Process P3, Need = (7, 3, 3)
Available = (9, 3, 5)
Need <=Available = True
The request for P3 is granted.
= (9, 3, 5) + (0, 2, 0) = (9, 5, 5)
8. Now again check for Process P0, = Need (3, 2, 1)
= Available (9, 5, 5)
So, the request will be granted to P0.
Safe sequence: < P1, P4, P2, P3, P0>
The system allocates all the needed resources to each process. So, we can say that the system is in a safe state.
3. The total amount of resources will be calculated by the following formula:
The total amount of resources= sum of columns of allocation + Available
= [8 5 7] + [2 1 0] = [10 6 7]
Implementation of Banker's Algorithm in C
Given below is the code for Banker's algorithm implementation:
Here is the output of the above program:

With this we end the banker's algorithm tutorial. We hope you understood it, if not go to our forum and ask your doubt.
- ← Prev
- Next →
OS MCQ Tests
gate mcq tests.
- Introduction
- Scripts and Compilation
- The TOC/TOU Bug
- Vulnerable Root Program
Banker's Algorithm
- Submission Procedure
You need Python 3.10.x or above to complete this lab.
In deadlock avoidance , before granting a resource request (even if the request is valid and the requested resources are now available), we need to check that the request will not cause the system to enter a deadlock state in the future or immediately.
The Banker’s Algorithm is a resource allocation and deadlock avoidance algorithm. It can be used to predict and compute whether or not the current request will lead to a deadlock .
- If yes, the request for the resources will be rejected ,
- Otherwise, it will be granted .
There are two parts of the Banker’s Algorithm:
- Resource Allocation Algorithm
- Safety Algorithm
You will be implementing the Safety Algorithm and calling it in Resource Allocation Algorithm in this lab.
Starter Code
Install python 3.10.x or above.
If you don’t have Python 3.10.x installed already, here’s a list of commands you can enter to install:
When done, check that it is installed, and install pip as well:
Download this repository:
You should have the following files:
You will be required to modify only certain sections in banker.py .
Leave ALL other files untouched. Also, DO NOT print anything else in banker.py . Only type your answers in the given space labeled in the starter code as TASK 1 and TASK 2 . Remember, DO NOT print anything else, and DO NOT import any other modules, and DO NOT modify any other instructions.
Banker’s Algorithm
Prerequisite.
Suppose we have N processes competing for M different types of resources. We call these processes “ customers ” (to these available resources).
In order for the Banker’s Algorithm to work, need to know the maximum demand of process i for resource j instances.
System State
We also need to represent the system STATE , such as the amount of resources available per-type-per-process using the following data structures:
- available: 1 by M vector
- available[i] : the available instances of resource i
- max: N by M matrix
- max[i][j] : maximum demand of process i for resource j instances
- allocation: N by M matrix
- allocation[i][j] : current allocation of resource j instances for process i
- need: N by M matrix
- need[i][j] : how much more of resource j instances might be needed by process i
For example, the following arrays display the available , max , allocation , and need values of a current state of a system with 5 processes: P0 , P1 , P2 , P3 , and P4 , and 3 types of resources: A , B , C ;
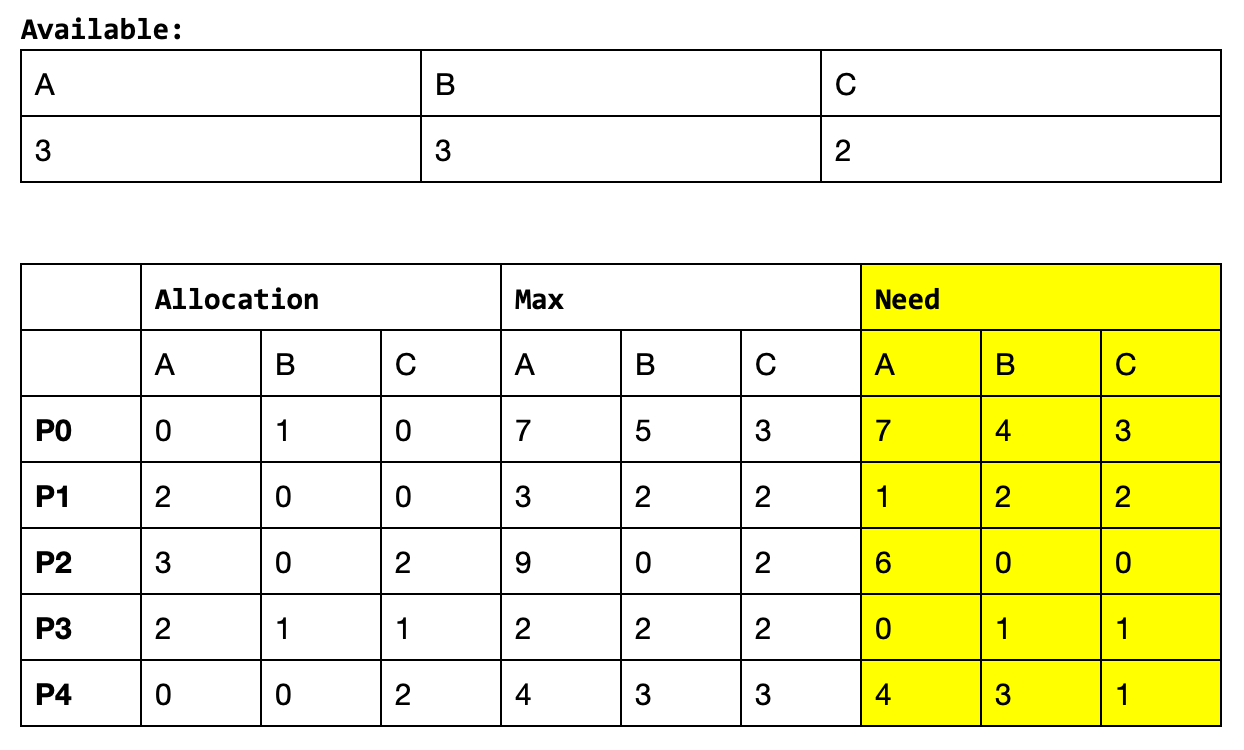
Open Banker.py and give it a quick read.
There are a few methods defined in Class Banker :
- set_maximum_demand : implemented for you, this populates the corresponding row of max matrix
- request_resources : request resource algorithm implementation. Task 1 is here .
- release_resources : releases resources borrowed by a customer. Assume release is valid for simplicity
- check_safe : safety check algorithm implementation. Task 2 is here .
- print_state and run_file : functions to load the input files and printing the states of the system: available, max, allocation, need values.
Input Format
The inputs to banker.py are inside test_files/ . All .txt files named as qi.txt are various input files.
The format are as follows:
- The first three lines contain values of N (number of processes), M (number of resource type), and the contents of the available vector.
- The format is c,pid,rid_1 rid_2 rid_3 ...
- The format is: r,pid,rid_1 rid_2 rid_3 ...
- The format is: f,pid,rid_1 rid_2 rid_3 ...
- For lines with just p , we print the state of the system
The lines in the input are read from top to bottom, and are treated as incoming requests or releases by each process as time progresses .
For instance, open test_files/q0.txt :
From the first two lines, we know that there are 3 distinct processes ( N ) competing for 3 different types of resources ( M ).
- Let’s name them as P0, P1, and P2 so that it is easier for us to address them.
- Also label each of the 3 resources as A, B, and C.
From the third line, we know that NONE of the resources are available: available = [0,0,0] .
In the fourth to sixth line, we can initialise the max matrix as follows:
This means P0 will need at most 2 instances of Resource A, 2 instances of Resource B, and 4 instances of Resource C during its lifetime of execution. Similar logic for P1 and P2.
In the seventh line r,0,1 2 1 , P0 starts requesting for 1 instance of Resource A, 2 instances of Resource B, and 1 instance of Resource C simultanously.
We will need to run the Banker’s algorithm now to determine whether this request is safe , and to be granted .
This is already done for you inside run_file function. Whenever line with r is encountered, the function request_resources is called.
- If the request is granted, we need to update available , allocation and need . This is already done for you inside request_resources function.
- If the request is not granted, we simply ignore that request. The values of available , allocation and need remains the same. This is already done for you inside request_resources function.
The same is done for all lines starting with r .
At the end, we print the state of the system with p .
In this example, NO resources are available. So obviously we are met with the same state of available , allocation and need after running the file.
You can run the file using the command:
The output is as such as expected, with allocation matrix remaining at 0.
Experiment with the other 5 files as well. Try them one by one and study the result.
Resource Request Algorithm
This algorithm is already implemented for you inside request_resources function. This algorithm is called each time we encounter a resource request (line starting with r ). The function receives two parameters and returns a bool:
The algorithm goes as follows:
If request[i] <= need[customer_index][i] for all i < M , go to step 2. Otherwise, return False (request rejected) since the process has exceeded its maximum claim.
If request[i] <= available[i] for all i < M , go to step 3. Otherwise, return False .
- Process i must wait since its requested resources are not immediately available (request rejected) .
At this point, the requested resources are available, but we check if granting the request is safe (will not lead to deadlock).
- Create a deepcopy of available , allocation , and need
- Pass these new data structures to the safety_check algorithm, which will return True (safe) or False (unsafe)
If the outcome of Step(3) is:
- available[i] = available[i] - request[i] for all i<M
- need[customer_index][i] = need[customer_index][i] - request[i] for all i<M
- allocation[customer_index][i] = allocation[customer_index][i] + request[i] for all i<M
- False : This means request rejected , and process i has to try again in the future. This is because granting the request results in deadlock in the future.
You may run: python3.10 banker.py test_files/q1.txt and study the output.
Initially, we know that allocation was initialised as 0 and that available=[5,5,5] from the third line in test_files/q1.txt . The first 3 requests are granted :
After granting the three requests above, we have the system state:
Further request by Process 0: request=[0,1,0] is rejected because Process 0 requests MORE than what’s been declared at maximum .
- It declared that it needs at maximum of 2 resources B (the second type of resource)
- It already held 2 types of resources B –> need[0][1]: 2
You may run: python3.10 banker.py test_files/q2.txt and study the output.
This time round, we have N=5 processes and M=3 different types of resources, and available=[10,5,7] .
After such requests from all customers,
…we should have only 3 counts of Resource A available (the first type of resource).
However here we witness Process 0 releasing 1 instance of Resource A, and hence the updated system state:
TASK 1: Call check_safe algorithm inside request_resources in banker.py . Right now the variable safe is always set to True , hence we always grant the request as long as the resources are available and the processes do not violate their max . For input q0, q1, q2 , we are lucky because we never had any requests that might result in deadlock.
However, if you try running the program with q3 :
…you will notice that the printed output is not the same as the answer: test_files/q3_answer.txt . We need to implement and call the check_safe algorithm to ensure that we get the right answer.
Let’s start easy. Since check_safe function is already defined (although not yet implemented), call check_safe method and assign their return value to safe :
DO NOT PRINT ANYTHING ELSE . Please remove your debug messages.
The Safety Algorithm
This algorithm is also called each time we encounter a resource request (line starting with r ), but only if we managed to enter Step 3 of the Resource Request Algorithm. The function receives five parameters and returns a bool :
Create a vector finish: list[int] of size N , initialised to be False for all elements in finish .
- work[i] = work[i] - request[i] for all i<M
- allocation[customer_index][i] = allocation[customer_index][] + request[i] for all i<M
- This request granting is hypothetical because work is a copy of available (not the actual available ). Similar argument with need, allocation . In reality, we haven’t granted the request yet, we simply compute this hypothetical situation and decide whether it will be safe or unsafe .
Find an index i (which is a customer ) such that:
- finish[i] == False and
- need[i][j] <= work[j] for all j<M .
- The two above condition signifies that an incomplete Customer i can complete even after this request by customer_index is granted
If such index i from Step 2 exists do the following, else go to Step 4.
- This signifies that a Customer i that can complete will free its currently allocated resources.
- Update: finish[i] = True
You might want to store the values of i each time you execute this Step 3 elsewhere to backtrack a possible safe execution sequence, but that’s not required for this lab.
If no such index i in Step 2 exists:
- If finish[i] == True for all i<N , then it means the system is in a safe state . Return True .
- Else, the system is not in a safe state . Return False .
Careless Mistake
Common careless mistake: A lot of people missed the “REPEAT step 2” instruction in step 3. Step 2 and 3 must be implemented in a while loop, as you might NOT necessarily obtain i in sequential (increasing) order.
Why is that so? Does it mean we can have a safe execution sequence e.g: 1,0,2 for a 3-process system? Yes of course! That simply means P1 can be executed first, then P0 , then P2 . Think about what i represents (just arbitrary naming of consumer processes), of course a safe execution sequence has nothing to do with their naming!
Run the Banker algorithm with q3 :
At first, P0 is making the request [1,0,3] and it is granted as shown in the allocation matrix:
You can verify whether any requests so far is granted by checking that the allocation matrix value adds up to the requests made.
Then, P1 requests for [1,1,1] . This is a legal request, since if the request is granted, then P1 allocation ( allocation[1] is initially [0,0,0] ) will still be not more than its maximum: max[1]: [2,1,3] .
If your implementation is correct , this should however lead to an unsafe state , and hence the request is rejected and the state of the system remain the same:
The same logic applies to samples in q4 and q5 as well:
- In q4, the last request by P0: [0,2,0] leads to an unsafe state and therefore rejected .
- In q4, the request by P1: [1,0,2] is rejected because it leads to an unsafe state . However, after P0 release the resources it held: [1,3,4] , a repeated request by P1 for the same set of resources [1,0,2] is granted.
TASK 2: Implement the safety check algorithm inside check_safe function in banker.py :
Again, do NOT print any other stuffs as part of your answer.
Further Notes
Rejecting requests.
If a resource request is rejected , dont panic, it’s not the end of the world. The process/consumer just need to try to request it again in the future.
How can this be implemented? Usually schedulers are programmed to tackle this kind of cases; e.g: they can be placed to a special queue and will periodically prompt for resource request until granted, or there exists some kind of event-driven solution – it’s free-for-all to implement.
Resource Release Caveat
We also assume that (for simplicity of this lab) a process will not release more than what has been allocated to them (that the value of release is valid). We wrote this detail under release_resources function:
Synchronisation
Finally, since max, allocation, need and available are shared data structures among all these methods, we protect each method that modifies these values using a reentrant lock : bank_lock = threading.RLock() .
We guard the critical sections with bank_lock.acquire() and bank_lock.release() to make it thread safe .
4010-441 Principles of Concurrent System Design The Banker's Algorithm
This page is accessible at www.se.rit.edu/~se441/Assignments/Bankers_Algorithm.html.
Here are pointers to other discussions of the Banker's Algorithm; the Web is full of them.
- University of Idaho
- Colorado State
One of the trickiest problems in concurrency is system resource management; the Banker's Algorithm is a classic deadlock free solution that is appropriate when (a) the resources are mutable, (b) processes / threads may request multiple units of a resource (e.g., printers), and (c) each process / thread can determine, at startup, the maximum number of units it needs of the resource (actually, we can relax these restrictions a bit at the cost of some additional complexity, but we won't).
The banker is initially configured with the number of resources it has to loan. Newly created threads register their maximum resource claim; obviously, this cannot exceed the number of resources the banker has. For each registered thread the banker maintains the maximum claim and the number of resources on loan to the thread (initially 0).
Once registered, a thread can request and release resources. We will ignore the details of how specific units (e.g., specific printers) are associated with a thread; all we care about is the following:
- The number of resource units currently allocated to each thread.
- The number of resource units remaining in each thread's request.
- The number of unallocated resource units.
The information above defines the state of the system from the perspective of the Banker. Note the following state invariants:
- The unallocated units plus the sum of the units allocated to each thread equals the total number of units available.
- For each thread, its allocation plus its remaining claim equals its maximum claim.
Safe States
The design of the system revolves around the notion of a safe state . In a safe state, there is some order in which threads could repay all outstanding loans (return all allocated resources) even if every thread requests all the remaining resources it can claim. Any other state is unsafe , as it may lead to deadlock (deadlock is not certain, because threads are not required to request all the resources in their claim). Below are examples of safe and unsafe states in a system with two threads and four allocatable resources. The columns are (C)laim (as initially sent to the banker), (A)llocated and (R)emaining claim. Note that, for each thread, C = A + R is an invariant.
Thus state 1 is safe .
Assume T_2 requests one unit; if granted, the system state would look like:
Thus, state 2a is also safe and the request can proceed.
Now assume we are in state 1 again and T_1 requests 1 unit; if granted, the system state would look like:
- The initial state, where no threads have registered a claim, is safe.
- If the system is in a safe state and new thread registers a claim for no more than the available resources, the resulting state is safe (in the worst case we can run all the threads in the original state to completion, and then run the new thread to completion).
- Thus, the only time we can create an unsafe state is when a thread attempts to acquire more resources (up to its remaining claim). In this case, we "pretend" to allocate the resource(s) by creating a copy of the current state, and altering the copy to reflect an increase of the requesting thread's allocation (and decrease of its remaining claim) by the number of units requested.
- Run the bankers algorithm (see below) on the pretend sate, and
- If the pretend state is safe, use it as the current state and return the resources to the requester.
- If the pretend state is unsafe, block the requester until some resources are released, and repeat the "pretend" allocation again.
- If the system is in a safe state and a thread releases some resources, the resulting state is safe (why?). As this may allow a blocked requester to get the resources it needs, we unblock any and all blocked requesters in the hope that at least one will be able to proceed.
The Banker's Algorithm: Determining State Safety
Banker class.
- The method calls System.exit(1) if:
- the thread already has a claim registered, or
- nUnits is not strictly positive, or
- nUnits exceeds the number of resources in the system.
- Associate the thread with a current claim equal to nUnits and a current allocation of 0.
- Print a message of the form: Thread name sets a claim for nUnits units. where name is the thread name (via Thread.currentThread().getName() ) and nUnits is the number of resources claimed, and return.
- The method calls System.exit(1) if (a) the current thread has no claim registered, (b) nUnits is not strictly positive or (c) nUnits exceeds the invoking thread's remaining claim.
- Print the message Thread name requests nUnits units.
- If allocating the resources results in a safe state, Print a message Thread name has nUnits units allocated. Update the banker's state and return to the caller.
- Otherwise enter a loop that Prints the message Thread name waits . Waits for notification of a change. When reawakened, prints the message Thread name awakened. If allocating the resources results in a safe state, prints Thread name has nUnits units allocated. Updates the banker's state and returnz .
- The method calls System.exit(1) if (a) the current thread has no claim registered, (b) nUnits is not strictly positive or (c) nUnits exceeds the number of units allocated to the current thread.
- Print the message Thread name releases nUnits units.
- Release nUnits of the units allocated to the current thread, notify all waiting threads, and return .
Client Class
The Client class extends Thread ; multiple Client objects content for the pool of resources by calling methods in a shared Banker object.
- If the banker.remaining() == 0 , then the client will release all of its units, otherwise the client will request some units.
- At the end of each loop, use Thread.sleep(millis) to sleep a random number of milliseconds from minSleepMillis to maxSleepMillis . This will introduce another dose of non-determinism into your program.
Driver Class
The driver class contains the public static void main( ... ) method that (a) creates a Banker object, (b) creates several Client objects, (c) starts all of the Client s, and (d) waits for all the clients to complete via the instance method join( ) . With proper setting of the configuration parameters you should be able to produce runs where a Client is delayed because granting its request would lead to an unsafe state.
The Driver should also contain a set of well-documented final private static int variables that specify the configuration parameters (number of resources for the Banker , number of Clients , arguments to the Client constructors, etc.). I may use these to experiment with your solution to see if I can break it.
Deliverables
Submit three files (and only three files), Driver.java , Client.java , and Banker.java to the Banker Activity dropbox by due date. Of the 10 points available for this activity:
- 2 will be granted on an all-or-nothing basis for a correct submission in accordance with the preceding specification,
- 5 will be based on the correctness of the solution,
- 2 will be based on the quality of the solution (naming, spacing, indentation, etc.), and
- 1 will be based on whether or not the internal documentation is of acceptable quality.

Banker’s Algorithm in Operating System [Example]

What is Banker’s Algorithm?
Banker’s Algorithm is used majorly in the banking system to avoid deadlock. It helps you to identify whether a loan will be given or not.
This algorithm is used to test for safely simulating the allocation for determining the maximum amount available for all resources. It also checks for all the possible activities before determining whether allocation should be continued or not.
For example, there are X number of account holders of a specific bank, and the total amount of money of their accounts is G.
When the bank processes a car loan, the software system subtracts the amount of loan granted for purchasing a car from the total money ( G+ Fixed deposit + Monthly Income Scheme + Gold, etc.) that the bank has.
It also checks that the difference is more than or not G. It only processes the car loan when the bank has sufficient money even if all account holders withdraw the money G simultaneously.
Banker’s Algorithm Notations
Here is an important notation used in Banker’s algorithm:
- X: Indicates the total number of processes of the system.
- Y: Indicates the total number of resources present in the system.
[I: Y] indicate which resource is available.
[l:X,l: Y]: Expression of the maximum number of resources of type j or process i
[l:X,l:Y]. Indicate where process you have received a resource of type j
Express how many more resources can be allocated in the future
Example of Banker’s algorithm
Assume that we have the following resources:
- 5 Pen drives
- 3 Hard disks
Here, we have created a vector representing total resources: Available = (5, 2, 4, 3).
Assume there are four processes. The available resources are already allocated as per the matrix table below.
Here, the allocated resources is the total of these columns:
Allocated = (4, 2, 2, 3).
We also create a Matrix to display the number of each resource required for all the processes. This matrix is called Need =(3,0,2,2)
The available vector will be :
Available=Available- Allocated
= (5, 2, 4, 3) -(4, 2, 2, 3)
=(1, 0, 2, 0)
Resource Request Algorithm
- Resource request algorithm enables you to represent the system behavior when a specific process makes a resource request.
Let understand this by the following steps:
Step 1) When a total requested instance of all resources is lesser than the process, move to step 2.
Step 2) When a requested instance of each and every resource type is lesser compared to the available resources of each type, it will be processed to the next step. Otherwise, the process requires to wait because of the unavailability of sufficient resources.
Step 3) Resource is allocated as shown in the below given Pseudocode.
This final step is performed because the system needs to assume that resources have been allocated. So that there should be less resources available after allocation.
Characteristics of Banker’s Algorithm
Here are important characteristics of banker’s algorithm:
- Keep many resources that satisfy the requirement of at least one client
- Whenever a process gets all its resources, it needs to return them in a restricted period.
- When a process requests a resource, it needs to wait
- The system has a limited number of resources
- Advance feature for max resource allocation
Disadvantage of Banker’s algorithm
Here, are cons/drawbacks of using banker’s algorithm
- Does not allow the process to change its Maximum need while processing
- It allows all requests to be granted in restricted time, but one year is a fixed period for that.
- All processes must know and state their maximum resource needs in advance.
- Banker’s algorithm is used majorly in the banking system to avoid deadlock . It helps you to identify whether a loan will be given or not.
- Notations used in banker’s algorithms are 1) Available 2) Max 3) Allocation 4) Need
- Banker’s algorithm keeps many resources that satisfy the requirement of at least one client
- The biggest drawback of banker’s algorithm this that it does not allow the process to change its Maximum need while processing.
- Inter Process Communication (IPC) in OS
- Round Robin Scheduling Algorithm with Example
- Process Synchronization: Critical Section Problem in OS
- Process Scheduling in OS: Long, Medium, Short Term Scheduler
- Priority Scheduling Algorithm: Preemptive, Non-Preemptive EXAMPLE
- Memory Management in OS: Contiguous, Swapping, Fragmentation
- Shortest Job First (SJF): Preemptive, Non-Preemptive Example
- Difference Between SSD and HDD
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Process Management in Distributed System
- Concurrency Control in Distributed Transactions
- Bully Algorithm in Distributed System
- MBR v/s GPT Partition in OS
- What is a Monitor?
- Error Correction in Computer Networks
- Prototyping in Product Management | Importance, Benefits, Methodologies and Tools
- How to Stop ffmpeg Remotely?
- Product Management Process | 7 stages of product management
- Digital Product Manager | Introduction, Skills, Roles and Responsibilities
- Using Masquerading with Iptables for Network Address Translation (NAT)
- Integrating Google Cloud Storage with WordPress: Storing Media Files
- SAP ERP: Components, Working Process and Advantages
- Semrush Toolbar | Installation, Purpose and Features
- How Should Resources Be Managed in Agile Project Management?
- On-policy vs off-policy methods Reinforcement Learning
- Estimation by Analogy and Relative Sizing
- How Does Kanban Facilitate Workflow Optimization?
- Information Radiators
Distributed System – Banker’s Algorithm
Distributed systems have a banker’s algorithm which serves as a deadlock avoidance algorithm. Bankers algorithm depicts the resource allocation strategy which can help in determining the availability of resources in the present or future and how these availability of resources will lead a Bankers’ system to go into deadlock. Different data structures can be used to implement the banker’s algorithm.
Banking Algorithm Terminologies
1. Available:
- This is used to define resource availability.
- It is depicted by Available[j] notation.
- This is used to depict the maximum resources a matrix can store.
3. Allocation:
- A matrix depicting the resources allocated to complete the assigned work.
- A matrix depicting the resources needed to complete the assigned work.
- Matrix is depicted with Need[i][j]
- This is used to define a boolean value to determine if process has the required resources and if resources get released after task finished.
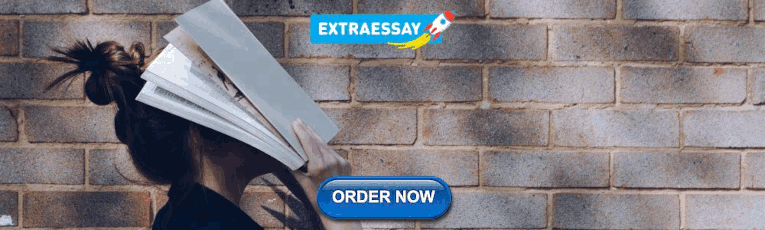
Algorithms Used in Banking Algorithms
To avoid deadlock in a system, Banker’s Algorithm includes the below two algorithms:
Resource Request Algorithm
Safety algorithm.
The safety algorithm as the name suggests is used to determine and calculate the safety of the system. It checks on the state and sequence of the system to analyze system safety.
To determine the safety of the system it is important to understand: Work, Finish[i], and Need[i] w.r.t the available resources.
Here, Finish[i], and Need[i] include arrays of length n.
Step 1: Set Work as Available.
Step 2: Check the availability of resources and continue the loop till Finish[i] is valued as false.
Step 3: When Finish[i] is valued as true we can consider the system to be safe.
The resource request algorithm as the name suggests is used to determine and calculate the system behavior when process makes request to resources.
To determine the resource request of the system it is important to understand: Allocation[i], Resource[i], Process[i] w.r.t the resources.
Let’s create a resource request array R[i] for each process P[i]. If the Resource Requesti [j] is equal to ‘K’, which means the process P[i] requires ‘k’ instances of Resources type R[j] in the system.
Step 1: Continue and go to step 2 if the condition is met else wait till the need meets the request.
Step 2: Continue and go to step 3 if the condition is met else wait till the resources become available.
Step 3: Here, the requested resource is made available to the system process
These two algorithms as discussed in the blog together constituted Banker’s algorithm.
- Banker’s algorithm plays a very important part role in ensuring customer safety.
- It ensures resource requirements are fulfilled keeping in mind all the necessary deadlock conditions .
- Multi-process environments using a banker’s algorithm grant safety, and security and ensure adequate resources to process are made available.
FAQ on Banker’s Algorithm in a Distributed System
Q.1: name the two algorithms that are important in banking algorithm.
The two algorithsms are as below: 1. Resource Request Algorithm 2. Safety Algorithm
Q.2: Are banking algorithms safe?
Yes, banking algorithm is very much safe and the algorithms takes care of respecting privacy and security.
Q.3: Comment on how a banking algorithms impact customers.
Banking algorithms enhance customer experience while ensuring safety and security.
Q.4: State the benefits of the banking algorithm.
Benefits of banking algorithm include fraud detection, ensuring security and safety.
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- Distributed System
- Geeks Premier League
- 10 Best Slack Integrations to Enhance Your Team's Productivity
- 10 Best Zendesk Alternatives and Competitors
- 10 Best Trello Power-Ups for Maximizing Project Management
- Google Rolls Out Gemini In Android Studio For Coding Assistance
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Banker's Algorithm Simulator

- Design Pattern
- Interview Q
C Control Statements
C functions, c dynamic memory, c structure union, c file handling, c preprocessor, c command line, c programming test, c interview.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

Search code, repositories, users, issues, pull requests...
Provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
bankers-algorithm
Here are 91 public repositories matching this topic..., mishal23 / os-lab.
Codes pertaining to OS Lab for Course CO254 - Operating Systems Lab
- Updated Jun 7, 2023
iguit0 / BankersAlgorithm
🚦 Dijkstra's famous algorithm
- Updated May 21, 2019
AmanCSE-1 / Operating-System
This repository contains the Python Programs for various algorithms of Operating Systems
- Updated Oct 11, 2021
Shruthi-Sivagnanam / Os-Concepts
Basic operating system concepts in c language.
- Updated Jun 24, 2022
geekswaroop / OS-Simulator
Operating System Simulator built using JS, HTML and CSS
- Updated Jun 8, 2021
notwld / bankers-algorithm
Banker's Algorithm to avoid deadlock among the processes.
- Updated Jan 23, 2023
Rakibul73 / Operating_System_Code
Operating System Code in Python 3
- Updated Aug 8, 2023
- Jupyter Notebook
iamrohitsuthar / SPOS
SPPU Computer Engineering Codes - SPOS (System Programming and Operating System Programs)
- Updated Sep 27, 2022
Swap76 / Bankers-Algorithm
C++ Program to Simulate Banker's Algorithm
- Updated Dec 4, 2019
radinshayanfar / os-lab
AUT Operating Systems Lab course
- Updated Feb 8, 2021
moazmohamed20 / OS-Algorithms
Operating System Algorithms Implementations in C++
- Updated Mar 5, 2024
madhurchhajed / Bankers-Algorithm-Implementation
Banker’s Algorithm is a resource allocation and deadlock avoidance algorithm.
- Updated Oct 1, 2020
signature95 / UBION_Project
UBION_Project 부실기업예측 알고리즘 개발입니다.
- Updated Jan 1, 2022
jspw / OS-Lab-Final
To whom it may concern
- Updated Dec 20, 2019
Anish-U / Bankers-Algorithm-Js
Visualizer for Deadlock Avoidance Algorithm (Bankers Algorithm / Advance Claim Algorithm)
- Updated Oct 23, 2020
Kapedinc / Underwriting-Module
Our underwriting python module for underwriting credit card accounts. For enterprise partners wanting to do their own underwriting in-house.
- Updated Feb 9, 2023
KhaledAshrafH / Deadlock-Handler
This repository contains a C++ implementation of the Banker's algorithm, which is used to avoid deadlock in a system. The program allows processes to request and release resources, and the banker will grant a request only if it leaves the system in a safe state. If a request would lead to an unsafe state, it will be denied. The program also include
- Updated Aug 23, 2023
amir78729 / Operating-Systems-Lab
OS course lab
- Updated Dec 23, 2020
JeffreyC1998 / CS330-Project-Deadlock-avoidance
- Updated Apr 11, 2020
Tomiwa-Ot / cs-assignments
computer science assignments
- Updated Apr 3, 2024
Improve this page
Add a description, image, and links to the bankers-algorithm topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the bankers-algorithm topic, visit your repo's landing page and select "manage topics."
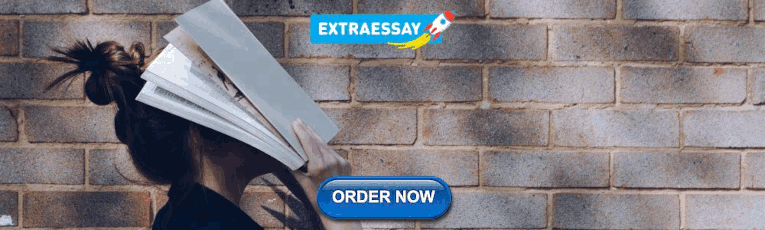
IMAGES
VIDEO
COMMENTS
The banker's algorithm is a resource allocation and deadlock avoidance algorithm that tests for safety by simulating the allocation for the predetermined maximum possible amounts of all resources, then makes an "s-state" check to test for possible activities, before deciding whether allocation should be allowed to continue.
Banker's algorithm is a deadlock avoidance algorithm.It is named so because this algorithm is used in banking systems to determine whether a loan can be granted or not. Consider there are n account holders in a bank and the sum of the money in all of their accounts is S.Every time a loan has to be granted by the bank, it subtracts the loan amount from the total money the bank has.
Banker's Algorithm: Data Structures • Available: Vector of length m. If available [j] = k, there are k instances of resource type R j available. • Max: n x m matrix. If Max [i,j] = k, then process P i may request at most k instances of resource type R j. • Allocation: n x m matrix. If Allocation[i,j] = k then P i is currently allocated ...
7 Banker's Algorithm Applicable to resources with multiple instances. Less efficient than the resource-allocation graph scheme. Each process declares its needs (number of resources) When a process requests a set of resources: Will the system be at a safe state after the allocation? - Yes → Grant the resources to the process. - No → Block the process until the resources are
tutorial on implementing the Banker's Algorithm in C. Whether you're a computer science student or a programming enthusiast, this video breaks down the compl...
The Banker's Algorithm is a resource allocation and deadlock avoidance algorithm. It can be used to predict and compute whether or not the current request will lead to a deadlock. If yes, the request for the resources will be rejected, Otherwise, it will be granted. There are two parts of the Banker's Algorithm:
Overview. One of the trickiest problems in concurrency is system resource management; the Banker's Algorithm is a classic deadlock free solution that is appropriate when (a) the resources are mutable, (b) processes / threads may request multiple units of a resource (e.g., printers), and (c) each process / thread can determine, at startup, the ...
Banker's algorithm is used majorly in the banking system to avoid deadlock. It helps you to identify whether a loan will be given or not. Notations used in banker's algorithms are 1) Available 2) Max 3) Allocation 4) Need. Resource request algorithm enables you to represent the system behavior when a specific process makes a resource request.
Considering a system with five processes P0 through P4 and three resources of type A, B, C. Resource type A has 10 instances, B has 5 instances and type C has 7 instances. Suppose at time t0 following snapshot of the system has been taken: Implement the Banker's algorithm to answer the following question:Is the system in a safe state?
multithreaded program that implements the banker's algorithm discussed in Section 7.5.3. Several customers request and release resources from the bank. The banker will grant a request only if it leaves the system in a safe state. A request that leaves the system in an unsafe state will be denied. This programming assignment combines three separate topics: (1) multithreading, (2) preventing ...
Hence, we created the context of need matrix. Ans. 2: Apply the Banker's Algorithm: Available Resources of A, B and C are 3, 3, and 2. Now we check if each type of resource request is available for each process. Step 1: For Process P1: Need <= Available. 7, 4, 3 <= 3, 3, 2 condition is false.
Prerequisite - Resource Allocation Graph (RAG), Banker's Algorithm, Program for Banker's Algorithm Banker's Algorithm is a resource allocation and deadlock avoidance algorithm. This algorithm test for safety simulating the allocation for predetermined maximum possible amounts of all resources, then makes an "s-state" check to test for possible activities, before deciding whether ...
BANKER'S ALGORITHMS PROGRAMMING ASSIGNMENT - 2. OPERATING SYSTEMS. HARPREET SINGH ARORA. 5838428. WHAT IS BANKER'S ALGORITHM? It is sometimes referred to as the detection algorithm, is a resource allocation and deadlock avoidance algorithm that tests for safety by simulating the allocation of predetermined maximum possible amounts of all ...
This project demonstrates the Banker's Algorithm by using two-dimensional arrays in order to store data each process' allocation, max, avaliable, and need. The program stores this data in an input file, and reads it to determine whether or not the system is in a safe state.
Learn more about Banker's Algorithm and how it works with the help of examples. Practice. Data Structures and Algorithms. Machine Coding Round (LLD) System Design & Architecture (HLD) Frontend UI Machine Coding. Resources. Career Advice and Roadmaps. Data Structures and Algorithms. Machine Coding Round (LLD) ...
Distributed systems have a banker's algorithm which serves as a deadlock avoidance algorithm. Bankers algorithm depicts the resource allocation strategy which can help in determining the availability of resources in the present or future and how these availability of resources will lead a Bankers' system to go into deadlock. Different data ...
No. of instances of A: No. of instances of B: No. of instances of C: Sample Example Find available Find need Find process sequence Reset
Banker's algorithm is a useful technique for managing multiple resources and avoiding deadlock. In this tutorial, you will learn how to implement banker's algorithm in C language with examples and explanations. You will also learn the concepts of c pointers, c structures, c union, c strings and more.
Cannot retrieve latest commit at this time. Although the repository is named "Shell-Scripts", it might not be limited to the same. I'll also include my assignments on Operating Systems concepts, which happens to cover shell-scripting. - Operating-Systems/Bankers Algorithm/Bankers.java at master · tinvaan/Operating-Systems.
Download Bankers Algorithm examples and explan and more Operating Systems Assignments in PDF only on Docsity! ABSTRACT In an operating system, there exist at least three strategies dealing with deadlocks for concurrent processes namely; deadlock prevention, avoidance and detection, in the increasing order of handling extent.
Programming Assignment #2 - Banker's Algorithm. Contribute to jmccloskey622/BankersAlgorithm development by creating an account on GitHub.
This repository contains a C++ implementation of the Banker's algorithm, which is used to avoid deadlock in a system. The program allows processes to request and release resources, and the banker will grant a request only if it leaves the system in a safe state. If a request would lead to an unsafe state, it will be denied. The program also include