Java Coding Practice
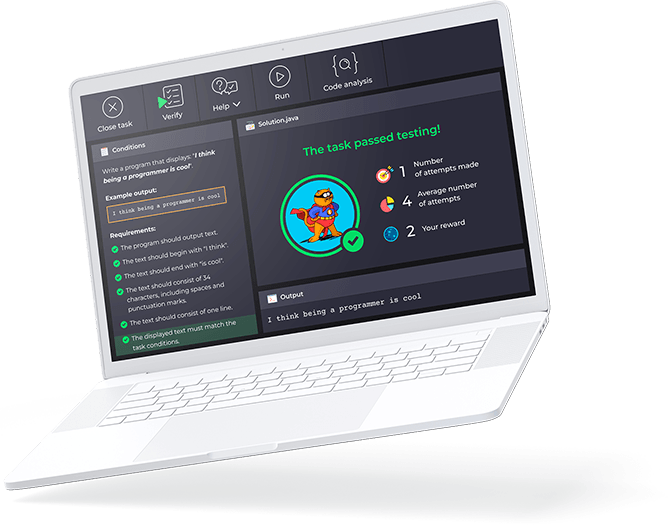
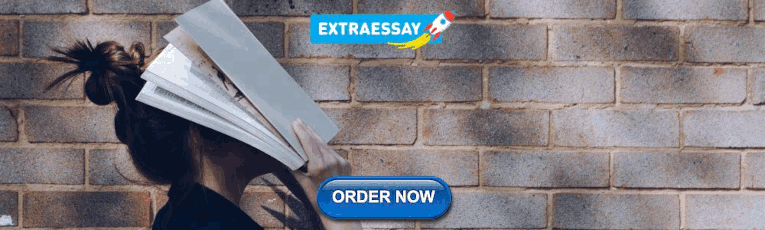
What kind of Java practice exercises are there?
How to solve these java coding challenges, why codegym is the best platform for your java code practice.
- Tons of versatile Java coding tasks for learners with any background: from Java Syntax and Core Java topics to Multithreading and Java Collections
- The support from the CodeGym team and the global community of learners (“Help” section, programming forum, and chat)
- The modern tool for coding practice: with an automatic check of your solutions, hints on resolving the tasks, and advice on how to improve your coding style
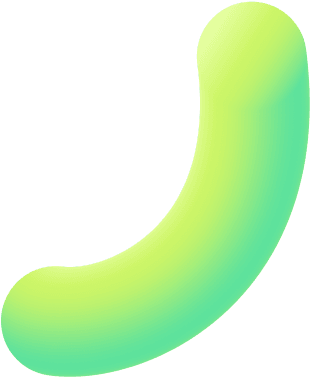
Click on any topic to practice Java online right away
Practice java code online with codegym.
In Java programming, commands are essential instructions that tell the computer what to do. These commands are written in a specific way so the computer can understand and execute them. Every program in Java is a set of commands. At the beginning of your Java programming practice , it’s good to know a few basic principles:
- In Java, each command ends with a semicolon;
- A command can't exist on its own: it’s a part of a method, and method is part of a class;
- Method (procedure, function) is a sequence of commands. Methods define the behavior of an object.
Here is an example of the command:
The command System.out.println("Hello, World!"); tells the computer to display the text inside the quotation marks.
If you want to display a number and not text, then you do not need to put quotation marks. You can simply write the number. Or an arithmetic operation. For example:
Command to display the number 1.
A command in which two numbers are summed and their sum (10) is displayed.
As we discussed in the basic rules, a command cannot exist on its own in Java. It must be within a method, and a method must be within a class. Here is the simplest program that prints the string "Hello, World!".
We have a class called HelloWorld , a method called main() , and the command System.out.println("Hello, World!") . You may not understand everything in the code yet, but that's okay! You'll learn more about it later. The good news is that you can already write your first program with the knowledge you've gained.
Attention! You can add comments in your code. Comments in Java are lines of code that are ignored by the compiler, but you can mark with them your code to make it clear for you and other programmers.
Single-line comments start with two forward slashes (//) and end at the end of the line. In example above we have a comment //here we print the text out
You can read the theory on this topic here , here , and here . But try practicing first!
Explore the Java coding exercises for practicing with commands below. First, read the conditions, scroll down to the Solution box, and type your solution. Then, click Verify (above the Conditions box) to check the correctness of your program.
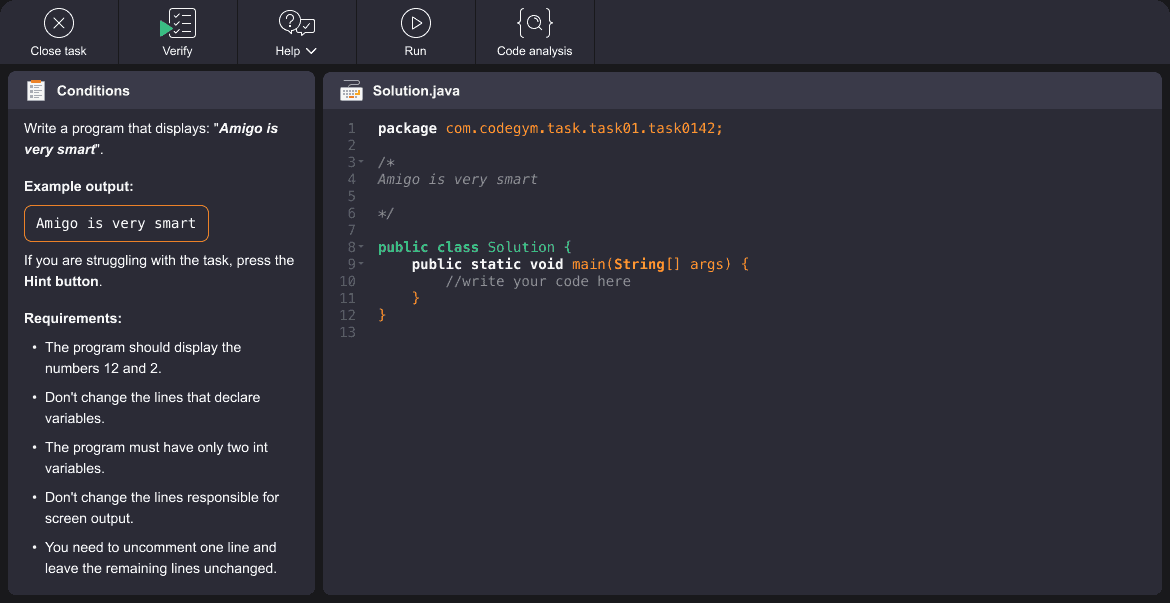
The two main types in Java are String and int. We store strings/text in String, and integers (whole numbers) in int. We have already used strings and integers in previous examples without explicit declaration, by specifying them directly in the System.out.println() operator.
In the first case “I am a string” is a String in the second case 5 is an integer of type int. However, most often, in order to manipulate data, variables must be declared before being used in the program. To do this, you need to specify the type of the variable and its name. You can also set a variable to a specific value, or you can do this later. Example:
Here we declared a variable called a but didn't give it any value, declared a variable b and gave it the value 5 , declared a string called s and gave it the value Hello, World!
Attention! In Java, the = sign is not an equals sign, but an assignment operator. That is, the variable (you can imagine it as an empty box) is assigned the value that is on the right (you can imagine that this value was put in the empty box).
We created an integer variable named a with the first command and assigned it the value 5 with the second command.
Before moving on to practice, let's look at an example program where we will declare variables and assign values to them:
In the program, we first declared an int variable named a but did not immediately assign it a value. Then we declared an int variable named b and "put" the value 5 in it. Then we declared a string named s and assigned it the value "Hello, World!" After that, we assigned the value 2 to the variable a that we declared earlier, and then we printed the variable a, the sum of the variables a and b, and the variable s to the screen
This program will display the following:
We already know how to print to the console, but how do we read from it? For this, we use the Scanner class. To use Scanner, we first need to create an instance of the class. We can do this with the following code:
Once we have created an instance of Scanner, we can use the next() method to read input from the console or nextInt() if we should read an integer.
The following code reads a number from the console and prints it to the console:
Here we first import a library scanner, then ask a user to enter a number. Later we created a scanner to read the user's input and print the input out.
This code will print the following output in case of user’s input is 5:
More information about the topic you could read here , here , and here .
See the exercises on Types and keyboard input to practice Java coding:
Conditions and If statements in Java allow your program to make decisions. For example, you can use them to check if a user has entered a valid password, or to determine whether a number is even or odd. For this purpose, there’s an 'if/else statement' in Java.
The syntax for an if statement is as follows:
Here could be one or more conditions in if and zero or one condition in else.
Here's a simple example:
In this example, we check if the variable "age" is greater than or equal to 18. If it is, we print "You are an adult." If not, we print "You are a minor."
Here are some Java practice exercises to understand Conditions and If statements:
In Java, a "boolean" is a data type that can have one of two values: true or false. Here's a simple example:
The output of this program is here:
In addition to representing true or false values, booleans in Java can be combined using logical operators. Here, we introduce the logical AND (&&) and logical OR (||) operators.
- && (AND) returns true if both operands are true. In our example, isBothFunAndEasy is true because Java is fun (isJavaFun is true) and coding is not easy (isCodingEasy is false).
- || (OR) returns true if at least one operand is true. In our example, isEitherFunOrEasy is true because Java is fun (isJavaFun is true), even though coding is not easy (isCodingEasy is false).
- The NOT operator (!) is unary, meaning it operates on a single boolean value. It negates the value, so !isCodingEasy is true because it reverses the false value of isCodingEasy.
So the output of this program is:
More information about the topic you could read here , and here .
Here are some Java exercises to practice booleans:
With loops, you can execute any command or a block of commands multiple times. The construction of the while loop is:
Loops are essential in programming to execute a block of code repeatedly. Java provides two commonly used loops: while and for.
1. while Loop: The while loop continues executing a block of code as long as a specified condition is true. Firstly, the condition is checked. While it’s true, the body of the loop (commands) is executed. If the condition is always true, the loop will repeat infinitely, and if the condition is false, the commands in a loop will never be executed.
In this example, the code inside the while loop will run repeatedly as long as count is less than or equal to 5.
2. for Loop: The for loop is used for iterating a specific number of times.
In this for loop, we initialize i to 1, specify the condition i <= 5, and increment i by 1 in each iteration. It will print "Count: 1" to "Count: 5."
Here are some Java coding challenges to practice the loops:
An array in Java is a data structure that allows you to store multiple values of the same type under a single variable name. It acts as a container for elements that can be accessed using an index.
What you should know about arrays in Java:
- Indexing: Elements in an array are indexed, starting from 0. You can access elements by specifying their index in square brackets after the array name, like myArray[0] to access the first element.
- Initialization: To use an array, you must declare and initialize it. You specify the array's type and its length. For example, to create an integer array that can hold five values: int[] myArray = new int[5];
- Populating: After initialization, you can populate the array by assigning values to its elements. All elements should be of the same data type. For instance, myArray[0] = 10; myArray[1] = 20;.
- Default Values: Arrays are initialized with default values. For objects, this is null, and for primitive types (int, double, boolean, etc.), it's typically 0, 0.0, or false.
In this example, we create an integer array, assign values to its elements, and access an element using indexing.
In Java, methods are like mini-programs within your main program. They are used to perform specific tasks, making your code more organized and manageable. Methods take a set of instructions and encapsulate them under a single name for easy reuse. Here's how you declare a method:
- public is an access modifier that defines who can use the method. In this case, public means the method can be accessed from anywhere in your program.Read more about modifiers here .
- static means the method belongs to the class itself, rather than an instance of the class. It's used for the main method, allowing it to run without creating an object.
- void indicates that the method doesn't return any value. If it did, you would replace void with the data type of the returned value.
In this example, we have a main method (the entry point of the program) and a customMethod that we've defined. The main method calls customMethod, which prints a message. This illustrates how methods help organize and reuse code in Java, making it more efficient and readable.
In this example, we have a main method that calls the add method with two numbers (5 and 3). The add method calculates the sum and returns it. The result is then printed in the main method.
All composite types in Java consist of simpler ones, up until we end up with primitive types. An example of a primitive type is int, while String is a composite type that stores its data as a table of characters (primitive type char). Here are some examples of primitive types in Java:
- int: Used for storing whole numbers (integers). Example: int age = 25;
- double: Used for storing numbers with a decimal point. Example: double price = 19.99;
- char: Used for storing single characters. Example: char grade = 'A';
- boolean: Used for storing true or false values. Example: boolean isJavaFun = true;
- String: Used for storing text (a sequence of characters). Example: String greeting = "Hello, World!";
Simple types are grouped into composite types, that are called classes. Example:
We declared a composite type Person and stored the data in a String (name) and int variable for an age of a person. Since composite types include many primitive types, they take up more memory than variables of the primitive types.
See the exercises for a coding practice in Java data types:
String is the most popular class in Java programs. Its objects are stored in a memory in a special way. The structure of this class is rather simple: there’s a character array (char array) inside, that stores all the characters of the string.
String class also has many helper classes to simplify working with strings in Java, and a lot of methods. Here’s what you can do while working with strings: compare them, search for substrings, and create new substrings.
Example of comparing strings using the equals() method.
Also you can check if a string contains a substring using the contains() method.
You can create a new substring from an existing string using the substring() method.
More information about the topic you could read here , here , here , here , and here .
Here are some Java programming exercises to practice the strings:
In Java, objects are instances of classes that you can create to represent and work with real-world entities or concepts. Here's how you can create objects:
First, you need to define a class that describes the properties and behaviors of your object. You can then create an object of that class using the new keyword like this:
It invokes the constructor of a class.If the constructor takes arguments, you can pass them within the parentheses. For example, to create an object of class Person with the name "Jane" and age 25, you would write:
Suppose you want to create a simple Person class with a name property and a sayHello method. Here's how you do it:
In this example, we defined a Person class with a name property and a sayHello method. We then created two Person objects (person1 and person2) and used them to represent individuals with different names.
Here are some coding challenges in Java object creation:
Static classes and methods in Java are used to create members that belong to the class itself, rather than to instances of the class. They can be accessed without creating an object of the class.
Static methods and classes are useful when you want to define utility methods or encapsulate related classes within a larger class without requiring an instance of the outer class. They are often used in various Java libraries and frameworks for organizing and providing utility functions.
You declare them with the static modifier.
Static Methods
A static method is a method that belongs to the class rather than any specific instance. You can call a static method using the class name, without creating an object of that class.
In this example, the add method is static. You can directly call it using Calculator.add(5, 3)
Static Classes
In Java, you can also have static nested classes, which are classes defined within another class and marked as static. These static nested classes can be accessed using the outer class's name.
In this example, Student is a static nested class within the School class. You can access it using School.Student.
More information about the topic you could read here , here , here , and here .
See below the exercises on Static classes and methods in our Java coding practice for beginners:
- C Program : Remove Vowels from A String | 2 Ways
- C Program : Remove All Characters in String Except Alphabets
- C Program : Sorting a String in Alphabetical Order – 2 Ways
- C Program To Check If Vowel Or Consonant | 4 Simple Ways
- C Program To Print Number Of Days In A Month | Java Tutoring
- C Program To Check Whether A Number Is Even Or Odd | C Programs
- C Program To Find Reverse Of An Array – C Programs
- C Program To Count The Total Number Of Notes In A Amount | C Programs
- C Program To Check A Number Is Negative, Positive Or Zero | C Programs
- C Program To Input Any Alphabet And Check Whether It Is Vowel Or Consonant
- C Program Inverted Pyramid Star Pattern | 4 Ways – C Programs
- C Program To Check Whether A Character Is Alphabet or Not
- C Program To Check Character Is Uppercase or Lowercase | C Programs
- C Program To Check If Alphabet, Digit or Special Character | C Programs
- C Program To Find Maximum Between Three Numbers | C Programs
- C Program To Check Whether A Year Is Leap Year Or Not | C Programs
- C Program Area Of Triangle | C Programs
- C Program To Check If Triangle Is Valid Or Not | C Programs
- C Program Find Circumference Of A Circle | 3 Ways
- C Program To Calculate Profit or Loss In 2 Ways | C Programs
- C Program Area Of Rectangle | C Programs
- X Star Pattern C Program 3 Simple Ways | C Star Patterns
- C Program Hollow Diamond Star Pattern | C Programs
- C Program To Check Number Is Divisible By 5 and 11 or Not | C Programs
- Mirrored Rhombus Star Pattern Program In c | Patterns
- C Program Area Of Rhombus – 4 Ways | C Programs
- C Program Area Of Isosceles Triangle | C Programs
- C Program Area Of Square | C Programs
- C Program To Find Area Of Semi Circle | C Programs
- C Program To Find Volume of Sphere | C Programs
- C Program Area Of Trapezium – 3 Ways | C Programs
- C Program Area Of Parallelogram | C Programs
- C Program Check A Character Is Upper Case Or Lower Case
- Hollow Rhombus Star Pattern Program In C | Patterns
- C Program To Count Total Number Of Notes in Given Amount
- C Program Area Of Equilateral Triangle | C Programs
- C Program to find the Area Of a Circle
- C Program To Find Volume Of Cone | C Programs
- C Program To Calculate Volume Of Cube | C Programs
- C Program To Calculate Perimeter Of Rectangle | C Programs
- C Program To Calculate Perimeter Of Rhombus | C Programs
- C Program Volume Of Cuboid | C Programs
- C Program To Calculate Perimeter Of Square | C Programs
- C Program To Search All Occurrences Of A Character In String | C Programs
- C Program Count Number Of Words In A String | 4 Ways
- C Program To Left Rotate An Array | C Programs
- C Program To Copy All Elements From An Array | C Programs
- C Program To Toggle Case Of Character Of A String | C Programs
- C Program To Delete Duplicate Elements From An Array | 4 Ways
- C Program Inverted Right Triangle Star Pattern – Pattern Programs
- C Program Volume Of Cylinder | C Programs
- C Square Star Pattern Program – C Pattern Programs | C Programs
- C Program To Remove First Occurrence Of A Character From String
- C Program To Compare Two Strings – 3 Easy Ways | C Programs
- C Program To Search All Occurrences Of A Word In String | C Programs
- C Mirrored Right Triangle Star Pattern Program – Pattern Programs
- C Program To Count Occurrences Of A Word In A Given String | C Programs
- C Program To Find Reverse Of A string | 4 Ways
- C Program To Delete An Element From An Array At Specified Position | C Programs
- C Program To Reverse Words In A String | C Programs
- C Program To Remove Last Occurrence Of A Character From String
- C Programs – 500+ Simple & Basic Programming Examples & Outputs
- C Pyramid Star Pattern Program – Pattern Programs | C
- C Program Replace First Occurrence Of A Character With Another String
- C Program Replace All Occurrences Of A Character With Another In String
- C Program To Remove Repeated Characters From String | 4 Ways
- C Plus Star Pattern Program – Pattern Programs | C
- C Program To Find Last Occurrence Of A Word In A String | C Programs
- Hollow Square Pattern Program in C | C Programs
- C Program To Check A String Is Palindrome Or Not | C Programs
- C Program To Remove Blank Spaces From String | C Programs
- Rhombus Star Pattern Program In C | 4 Multiple Ways
- C Program To Find Last Occurrence Of A Character In A Given String
- C Program To Trim Leading & Trailing White Space Characters From String
- C Program To Copy One String To Another String | 4 Simple Ways
- C Program Number Of Alphabets, Digits & Special Character In String | Programs
- Merge Two Arrays To Third Array C Program | 4 Ways
- C Program To Find Maximum & Minimum Element In Array | C Prorams
- C Program To Sort Even And Odd Elements Of Array | C Programs
- C Program To Search An Element In An Array | C Programs
- C Program Right Triangle Star Pattern | Pattern Programs
- C Program To Count Frequency Of Each Character In String | C Programs
- C Program Count Number Of Vowels & Consonants In A String | 4 Ways
- C Program Find Maximum Between Two Numbers | C Programs
- C Program To Count Frequency Of Each Element In Array | C Programs
- C Program To Trim Trailing White Space Characters From String | C Programs
- C Program To Trim White Space Characters From String | C Programs
- Highest Frequency Character In A String C Program | 4 Ways
- C Program To Find First Occurrence Of A Word In String | C Programs
- C Program To Concatenate Two Strings | 4 Simple Ways
- C Program To Remove First Occurrence Of A Word From String | 4 Ways
- C Program To Insert Element In An Array At Specified Position
- C Program To Convert Lowercase String To Uppercase | 4 Ways
- C Program To Print All Unique Elements In The Array | C Programs
- C Program To Put Even And Odd Elements Of Array Into Two Separate Arrays
- C Program To Count Occurrences Of A Character In String | C Programs
- C Program To Count Number Of Even & Odd Elements In Array | C Programs
- C Program To Sort Array Elements In Ascending Order | 4 Ways
- C Program To Count Number Of Negative Elements In Array
- C Program Hollow Inverted Right Triangle Star Pattern
- C Program To Convert Uppercase String To Lowercase | 4 Ways
- C Program To Print Number Of Days In A Month | 5 Ways
- Diamond Star Pattern C Program – 4 Ways | C Patterns
- C Program To Input Week Number And Print Week Day | 2 Ways
- C Program Hollow Inverted Mirrored Right Triangle
- C Program To Remove All Occurrences Of A Character From String | C Programs
- C Program Count Number of Duplicate Elements in An Array | C Programs
- C Program To Find Sum Of All Array Elements | 4 Simple Ways
- C Program To Find Lowest Frequency Character In A String | C Programs
- C Program To Sort Array Elements In Descending Order | 3 Ways
- 8 Star Pattern – C Program | 4 Multiple Ways
- C Program To Read & Print Elements Of Array | C Programs
- C Program To Replace Last Occurrence Of A Character In String | C Programs
- C Program To Find Length Of A String | 4 Simple Ways
- C Program To Right Rotate An Array | 4 Ways
- C Program To Find First Occurrence Of A Character In A String
- C Program Hollow Mirrored Rhombus Star Pattern | C Programs
- C Program Half Diamond Star Pattern | C Pattern Programs
- C Program Hollow Mirrored Right Triangle Star Pattern
- Hollow Inverted Pyramid Star Pattern Program in C
- C Program To Print All Negative Elements In An Array
- Right Arrow Star Pattern Program In C | 4 Ways
- C Program : Capitalize First & Last Letter of A String | C Programs
- C Program Hollow Right Triangle Star Pattern
- C Program : Check if Two Arrays Are the Same or Not | C Programs
- C Program : Check if Two Strings Are Anagram or Not
- Left Arrow Star Pattern Program in C | C Programs
- C Program Mirrored Half Diamond Star Pattern | C Patterns
- C Program Inverted Mirrored Right Triangle Star Pattern
- Hollow Pyramid Star Pattern Program in C
- C Program : Non – Repeating Characters in A String | C Programs
- C Program : Sum of Positive Square Elements in An Array | C Programs
- C Program : Find Longest Palindrome in An Array | C Programs
- C Program : To Reverse the Elements of An Array | C Programs
- C Program Lower Triangular Matrix or Not | C Programs
- C Program : Maximum Scalar Product of Two Vectors
- C Program Merge Two Sorted Arrays – 3 Ways | C Programs
- C Program : Check If Arrays are Disjoint or Not | C Programs
- C program : Find Median of Two Sorted Arrays | C Programs
- C Program : Convert An Array Into a Zig-Zag Fashion
- C Program : Minimum Scalar Product of Two Vectors | C Programs
- C Program : Find Missing Elements of a Range – 2 Ways | C Programs
- C Program Transpose of a Matrix 2 Ways | C Programs
- C Program Patterns of 0(1+)0 in The Given String | C Programs
- C Program : To Find Maximum Element in A Row | C Programs
- C Program : To Find the Maximum Element in a Column
- C Program : Rotate the Matrix by K Times | C Porgrams
- C Program To Check Upper Triangular Matrix or Not | C Programs
- C Program : Check if An Array Is a Subset of Another Array
- C Program : Non-Repeating Elements of An Array | C Programs
- C Program : Rotate a Given Matrix by 90 Degrees Anticlockwise
- C Program Sum of Each Row and Column of A Matrix | C Programs
Learn Java Java Tutoring is a resource blog on java focused mostly on beginners to learn Java in the simplest way without much effort you can access unlimited programs, interview questions, examples
Java programs – 500+ simple & basic programs with outputs.
in Java Programs , Java Tutorials March 6, 2024 Comments Off on Java Programs – 500+ Simple & Basic Programs With Outputs
Java programs: Basic Java programs with examples & outputs. Here we covered over the list of 500+ Java simple programs for beginners to advance, practice & understood how java programming works. You can take a pdf of each program along with source codes & outputs.
In case if you are looking out for C Programs , you can check out that link.
We covered major Simple to basic Java Programs along with sample solutions for each method. If you need any custom program you can contact us.
All of our Sample Java programs with outputs in pdf format are written by expert authors who had high command on Java programming. Even our Java Tutorials are with rich in-depth content so that newcomers can easily understand.
1. EXECUTION OF A JAVA PROGRAM
Static loading : A block of code would be loaded into the RAM before it executed ( i.e after being loaded into the RAM it may or may not get executed )
Dynamic loading: A block of code would be loaded into the RAM only when it is required to be executed.
Note: Static loading took place in the execution of structured programming languages. EX: c- language
Java follows the Dynamic loading
– JVM would not convert all the statements of the class file into its executable code at a time.
– Once the control comes out from the method, then it is deleted from the RAM and another method of exe type will be loaded as required.
– Once the control comes out from the main ( ), the main ( ) method would also be deleted from the RAM. This is why we are not able to view the exe contents of a class file.
Simple Hello Word Program
Out of 500+ Simple & Basic Java Programs: Hello world is a first-ever program which we published on our site. Of course, Every Java programmer or C programmer will start with a “Hello World Program”. Followed by the rest of the programs in different Categories.
Basic Java Programs – Complete List Here
Advanced simple programming examples with sample outputs, string, array programs.
Sort Programs
Conversion Programs:
Star & Number Pattern Programs
Functions of JVM:
- It converts the required part if the bytecode into its equivalent executable code.
- It loads the executable code into the RAM.
- Executes this code through the local operating system.
- Deletes the executable code from the RAM.
We know that JVM converts the class file into its equivalent executable code. Now if a JVM is in windows environment executable code that is understood by windows environment only.
Similarly, same in the case with UNIX or other or thus JVM ID platform dependent.
Java, With the help of this course, students can now get a confidant to write a basic program to in-depth algorithms in C Programming or Java Programming to understand the basics one must visit the list 500 Java programs to get an idea.
Users can now download the top 100 Basic Java programming examples in a pdf format to practice.
But the platform dependency of the JVM is not considered while saying Java is platform independent because JVM is supplied free of cost through the internet by the sun microsystems.
Platform independence :
Compiled code of a program should be executed in any operating system, irrespective of the as in OS in which that code had been generated. This concept is known as platform independence.
- The birth of oops concept took place with encapsulation.
- Any program contains two parts.
- Date part and Logic part
- Out of data and logic the highest priority we have given to data.
- But in a structured programming language, the data insecurity is high.
- Thus in a process, if securing data in structured prog. lang. the concept of encapsulation came into existence.
Note: In structured programming lang programs, the global variable play a vital role.
But because of these global variables, there is data insecurity in the structured programming lang programs. i.e functions that are not related to some variables will have access to those variables and thus data may get corrupted. In this way data is unsecured.
“This is what people say in general about data insecurity. But this is not the actual reason. The actual concept is as follows”.
Let us assume that, we have a ‘C’ program with a hundred functions. Assume that it is a project. Now if any upgradation is required, then the client i.e the user of this program (s/w) comes to its company and asks the programmers to update it according to his requirement.
Now we should note that it is not guaranteed that the programmers who developed this program will still be working with that company. Hence this project falls into the hands of new programmers.
Automatically it takes a lot of time to study. The project itself before upgrading it. It may not be surprising that the time required for writing the code to upgrade the project may be very less when compared to the time required for studying the project.
Thus maintenance becomes a problem.
If the new programmer adds a new function to the existing code in the way of upgrading it, there is no guarantee that it will not affect the existing functions in the code. This is because of global variables. In this way, data insecurity is created.
- To overcome this problem, programmers developed the concept of encapsulation .
- For example, let us have a struc.prog.lang. program with ten global variables and twenty functions.
- It is sure that all the twenty functions will not use all the global variables .
Three of the global variables may be used only by two functions. But in a structured prog. Lang like ‘C’ it is not possible to restrict the access of global variables by some limited no of functions.
Every function will have access to all the global variables.
To avoid this problem, programmers have designed a way such that the variables and the functions which are associated with or operate on those variables are enclosed in a block and that bock is called a class and that class and that class is given a name, Just as a function is given a name.
Now the variables inside the block cannot be called as the local variable because they cannot be called as global variables because they are confined to a block and not global.
Hence these variables are known as instance variables
_______________________________________________________________
Example 2 :
Therefore a class is nothing but grouping data along with its functionalities.
Note 1: E ncapsulation it’s the concept of binding data along with its corresponding functionalities.
Encapsulations came into existence in order to provide security for the data present inside the program.
Note 2: Any object oriental programming language file looks like a group of classes. Everything is encapsulated. Nothing is outside the class.
- Encapsulation is the backbone of oop languages.
- JAVA supports all the oop concepts ( i.e. encapsulation, polymorphism, inheritance) and hence it is known as an object-oriented programming language.
- C++ breaks the concept of encapsulation because the main ( ) method in a C++ program is declared outside a class. Hence it is not a pure oop language, in fact, it is a poor oop language.
Related Posts !
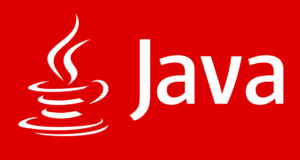
Java Program To Calculate Perimeter Of Rhombus | 3 Ways
April 10, 2024
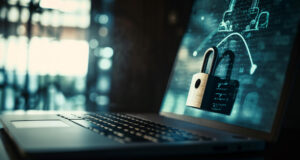
VPN Blocked by Java Security on PC? Here’s How to Fix That
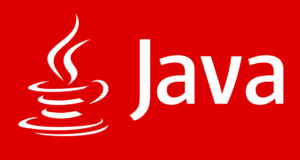
HCF Of Two & N Numbers Java Program | 3 Ways
April 7, 2024
LCM Of Two Numbers Java Program | 5 Ways – Programs
April 4, 2024
Java Program Convert Fahrenheit To Celsius | Vice Versa
April 1, 2024
Java Program Count Vowels In A String | Programs
March 29, 2024
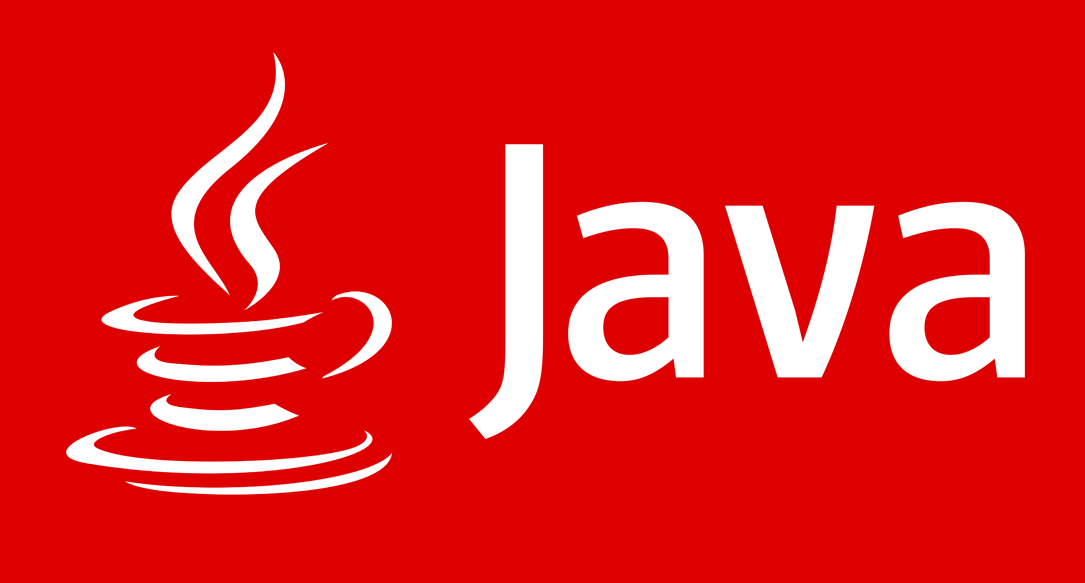
X Star Pattern Java Program – Patterns
Java program to print X star pattern program – We have written the below print/draw ...
The Java Handbook – Learn Java Programming for Beginners

Java has been around since the 90s. And despite its massive success in many areas, this cross-platform, object-oriented programming language is often maligned.
Regardless of how people feel about Java, I can tell you from experience is that it is an excellent programming language. After its first appearance back in 1995, it's still widely-used – and chances are it's not going anywhere anytime soon.
You can use Java to build servers, create desktop applications, games, mobile applications and more. There are also other JVM (we'll discuss what that means very soon) languages such as Kotlin , Groovy , Scala , and Clojure that you can use for different purposes.
Java is also cross-platform which means code you write and compile on one platform can run on any other platform that has Java installed on it. We'll discuss this topic in much more detail later on.
For now, I can tell you that although Java has its fair share of flaws, it also a has a lot to offer.
Table of Contents
Prerequisites, what’s going on in the code, what is jvm, what is jre and jdk.
- How To Setup Java on Your Computer?
- How To Install a Java IDE on Your Computer?
- How To Create a New Project on IntelliJ IDEA
What Are the Rules for Declaring Variables?
What are final variables, what is type conversion or casting.
- What are Wrapper Classes in Java
What Are the Arithmetic Operators?
What are the assignment operators, what are the relational operators, what are the logical operators, what are the unary operators, how to format a string, how to get the length of a string or check if it's empty or not, how to split and join strings, how to convert a string to upper or lowercase, how to compare two strings.
- How to Replace Characters or Substring in a String
How to Check If a String Contains a Substring or Not
What are the different ways of inputting and outputting data, how to use conditional statements in java, what is a switch-case statement, what is variable scope in java, what are default values of variables in java, how to sort an array, how to perform binary search on an array, how to fill an array, how to make copies of an array, how to compare two arrays, for-each loop, do-while loop, how to add or remove multiple elements, how to remove elements based on a condition, how to clone and compare array lists.
- How To Check if an Element Is Present or the Array List Is Empty
How to Sort an Array List
- How To Keep Common Elements From Two Array Lists
- How To Perform an Action on All Elements of an Array List
- How To Put or Replace Multiple Elements in a Hash Map
- How To Check if a Hash Map Contains an Item or if It’s Empty
- How To Perform an Action on All Elements of a Hash Map
What is a Method?
What is method overloading, what are constructors in java, what are access modifiers in java, what are the getter and setter methods in java, what is inheritance in java, how to override a method in java.
The only re-requisite for this course is familiarity with any other programming language such as Python, JavaScript, and so on.
Although I'll explain crucial programming concepts in the context of Java, I'll not explain things like what a variable is in the context of programming in general.
How to Write Hello World in Java
Ideally the first step should've been setting up Java on your computer, but I don't want to bore you with downloading and installing a bunch of software right at the beginning. For this example, you'll use https://replit.com/ as your platform.
First, head over to https://replit.com/ and create a new account if you don't already have one. You can use your existing Google/GitHub/Facebook account to login. Once logged in, you'll land on your home. From there, use the Create button under My Repls to create a new repl.
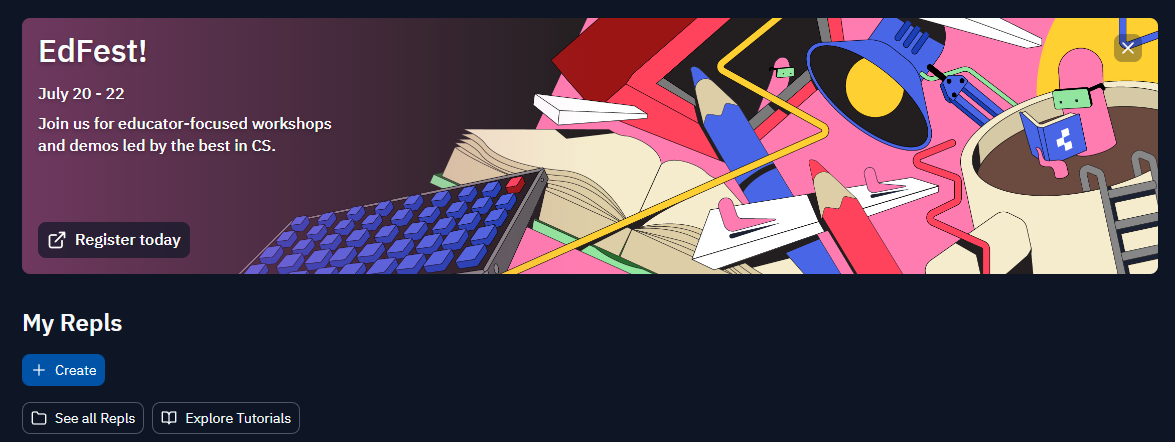
In the Create a Repl modal, choose Java as Template, set a descriptive Title such as HelloWorld and hit the Create Repl button.

A code editor will show up with an integrated terminal as follows:
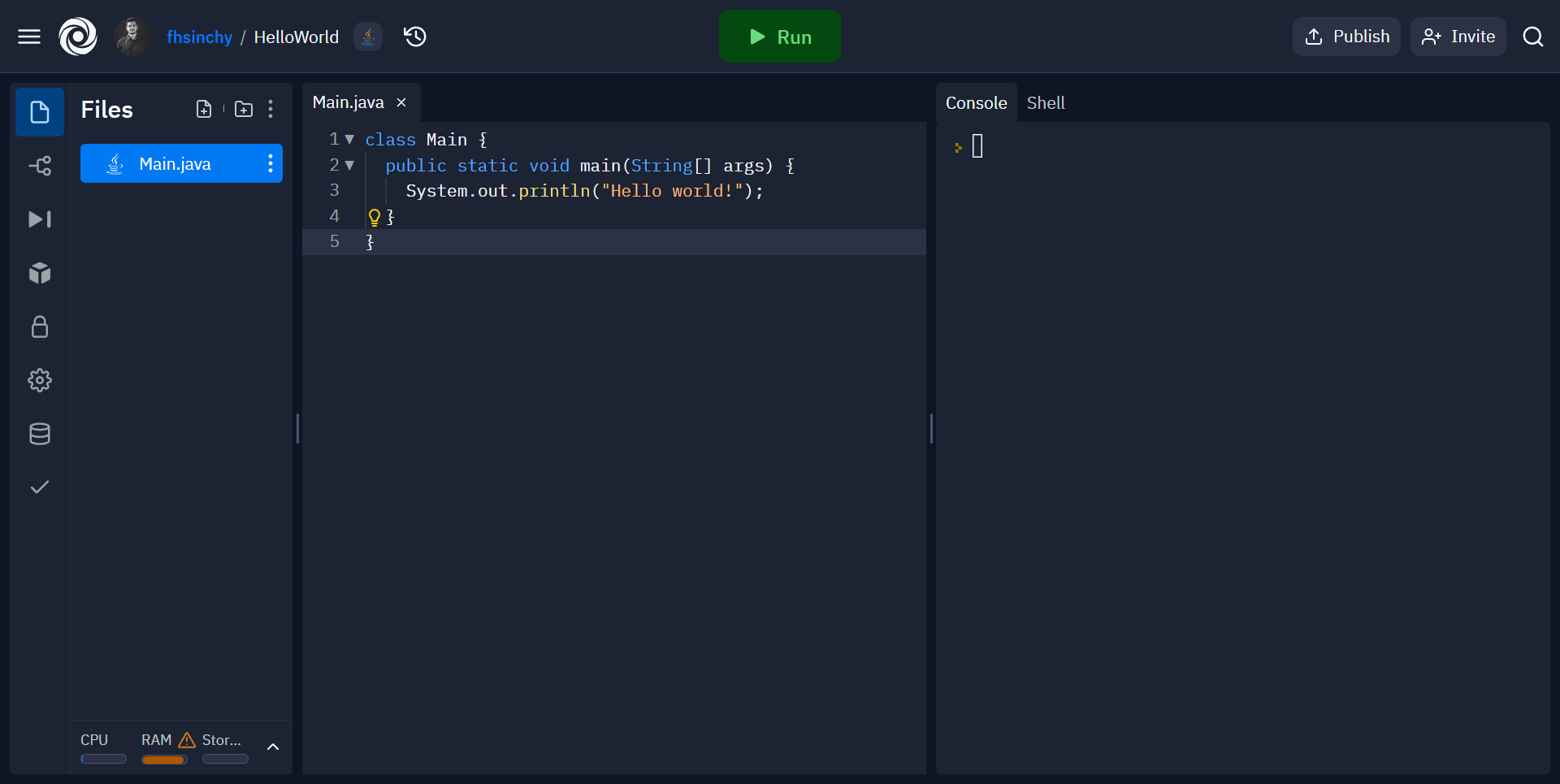
On the left side is the list of files in this project, in the middle is the code editor, and on the right side is the terminal.
The template comes with some code by default. You can run the code by hitting the Run button. Go ahead and do that, run the program.

If everything goes fine, you'll see the words "Hello world!" printed on the right side. Congratulations, you've successfully run your first Java program.
The hello world program is probably the most basic executable Java program that you can possibly write – and understanding this program is crucial.
Let's start with the first line:
This line creates a Main class. A class groups together a bunch of related code within a single unit.
This is a public class, which means this class is accessible anywhere in the codebase. One Java source file (files with the .java extension) can contain only one top level public class in it.
This top level public class has to be named exactly the same as the source code filename. That's why the file named Main.java contains the main class in this project.
To understand why, click on the three dots in the list of files and click on the Show hidden files option.
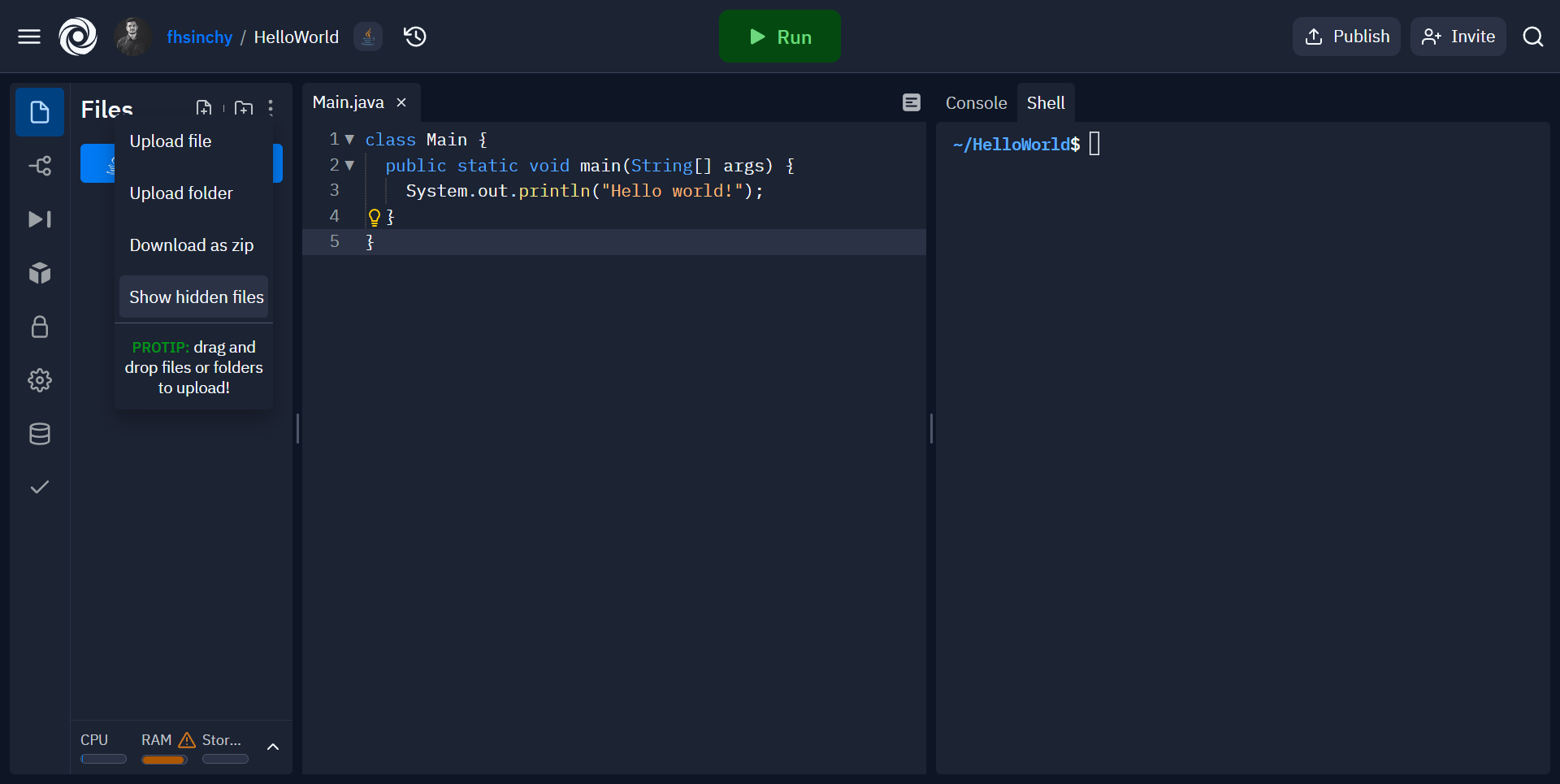
This will unveil some new files within the project. Among them is the Main.class file. This is called a bytecode. When you hit the Run button, the Java compiler compiled your code from the Main.java file into this bytecode.
Now, modify the existing Hello World code as follows:
As you can see, a new class called NotMain has been added. Go ahead and hit the Run button once more while keeping your eyes on the Files menu.
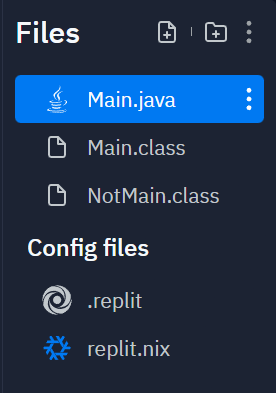
A new bytecode named NotMain.class has showed up. This means that for every class you have within your entire codebase, the compiler will create a separate bytecode.
This creates confusion about which class is the entry-point to this program. To solve this issue, Java uses the class that matches the source code file name as the entry-point to this program.
Enough about the class, now let's look at the function inside it:
The public static void main (String[] args) function is special in Java. If you have experience with languages like C, C++ or Go, you should already know that every program in those languages has a main function. The execution of the program begins from this main function.
In Java, you have to write this function as exactly public static void main (String[] args) otherwise it won't work. In fact, if you change it even a little bit Java will start to scream.
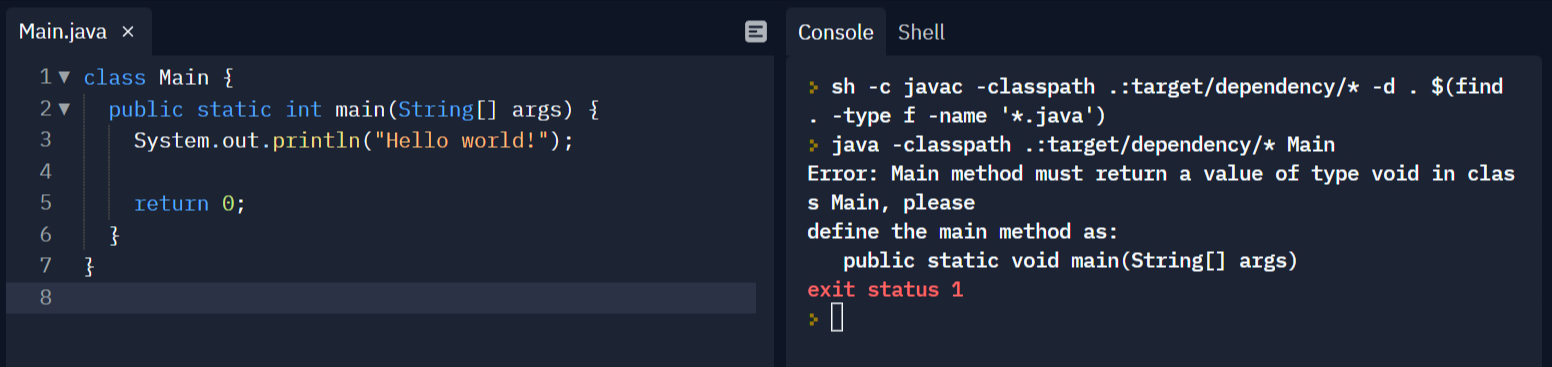
The return type has changed from void to int and the function now returns 0 at the end. As you can see in the console, it says:
Listen to that suggestion and revert your program back to how it was before.
The main method is a public method and the static means, you can call it without instantiating its class.
The void means that the function doesn't return any value and the String[] args means that the function takes an array of strings as an argument. This array holds command line arguments passed to the program during execution.
The System.out.println prints out strings on the terminal. In the example above, "Hello world!" has been passed to the function, so you get Hello world! printed on the terminal.
In Java, every statement ends with a semicolon. Unlike JavaScript or Python, semicolons in Java are mandatory . Leaving one out will cause the compilation to fail.
That's pretty much it for this program. If you didn't understand every aspect of this section word by word, don't worry. Things will become much clearer as you go forward.
For now, remember that the top level public class in a Java source file has to match the file name, and the main function of any Java program has to be defined as public static void main(String[] args) .
I've uttered the word "bytecode" a few times already in the previous section. I've also said that Java is "cross-platform" which means code written and compiled in one platform can run on any platform that has Java installed on it.
You see, your processor doesn't understand English. In fact the only thing it understands are zeros and ones, aka binary.
When you write and compile a C++ program it results in a binary file. Your processor understands it and based on the program's targeted platform, this file can be different.
Take an AMD64 and an ARMv8-A processor for example. These processors have different instruction sets. So in order to run your program on these two different platforms, you'll have to compile them separately.
But a Java program can be written once and run anywhere. I hope you remember the bytecodes we talked about in the previous section. When you compile Java code it doesn't result in binary but rather in bytecode.
This bytecode is not entirely binary but it's also not human readable. In fact, your processor can't read it either.
So instead of throwing this bytecode at the CPU, we instead run it through the Java Virtual Machine or JVM for short. JVM then reads and interprets the bytecode to the CPU.
If you would like to understand the architecture of JVM at a deeper level, I would suggest Siben Nayak 's in-depth article on the topic.
JRE stands for Java Runtime Environment and JDK stands for Java Development Kit.
The JRE or Java Runtime Environment packages together an implementation of the JVM along with a set of libraries required for running Java programs.
The JDK, on the other hand, packages the JRE along with all the necessary libraries for developing Java programs.
So if you want to run Java programs on your computer you install the JRE. If you want to develop Java programs yourself, you install the JDK. There are multiple implementation of the JDK.
There is the Java SE (Standard Edition) Development Kit from Oracle, then there is the OpenJDK , an official reference implementation of Java SE (Standard Edition) Development Kit.
As you can tell from the name of OpenJDK, it's open-source. So there are multiple builds of it. If you're on a Linux machine and use your distro's package manager to install JDK, it's highly likely that you'll install an OpenJDK build such as Adoptium , Microsoft Build of OpenJDK and so on.
I hope that you understand that JRE is a superset of JVM and JDK is a superset of JRE. Don't worry about different implementations or builds at the moment, you'll get your hands on them when the time comes.
How to Setup Java on Your Computer
First, head over to https://www.oracle.com/java/technologies/downloads/ and download the latest version of the Java SE Development Kit according to the platform you're on:
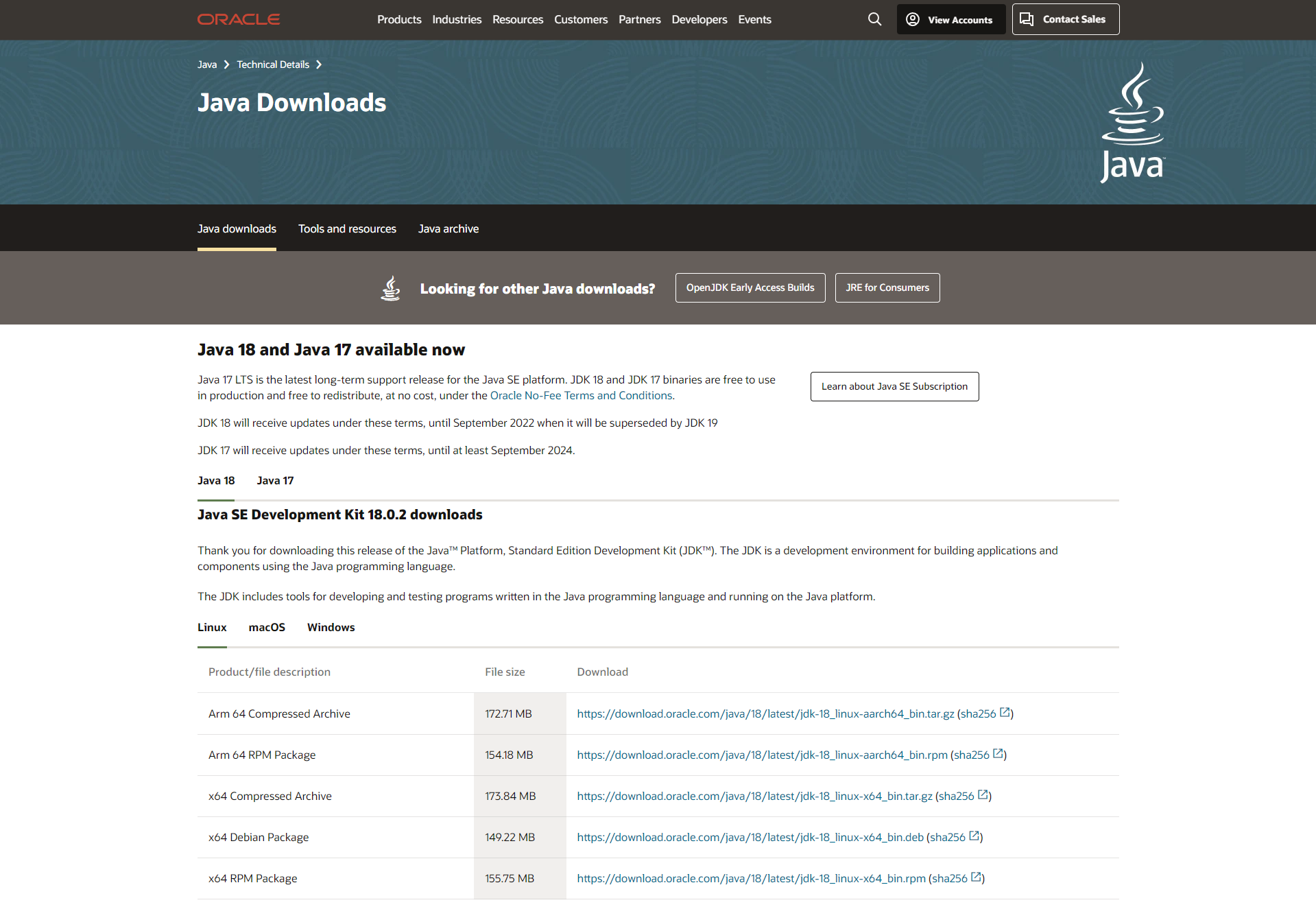
Once the download has finished, start the installer and go through the installation process by hitting the Next buttons. Finish it by hitting the Close button on the last page.

The installation process may vary on macOS and Linux but you should be able to figure it out by yourself.
Once the installation has finished, execute the following command on your terminal:
If it works, you've successfully install Java SE Development Kit on your computer. If you want to use OpenJDK instead, feel free to download Microsoft Build of OpenJDK or Adoptium and go through the installation process.
For the simple example programs that we're going to write in this article, it won't matter which JDK you're using. But in real life, make sure that your JDK version plays nicely with the type of project you're working on.
How to Install a Java IDE on Your Computer
When it comes to Java, IntelliJ IDEA is undeniably the best IDE out there. Even Google uses it as a base for their Android Studio .
The ultimate version of the IDE can cost an individual up-to $149.00 per year . But if you're student, you can get educational licenses for all JetBrains products for free.
There is also the completely free and open-source community edition. This is the one we'll be using throughout the entire book.
Head over to the IntelliJ IDEA download page , and download the community edition for your platform.
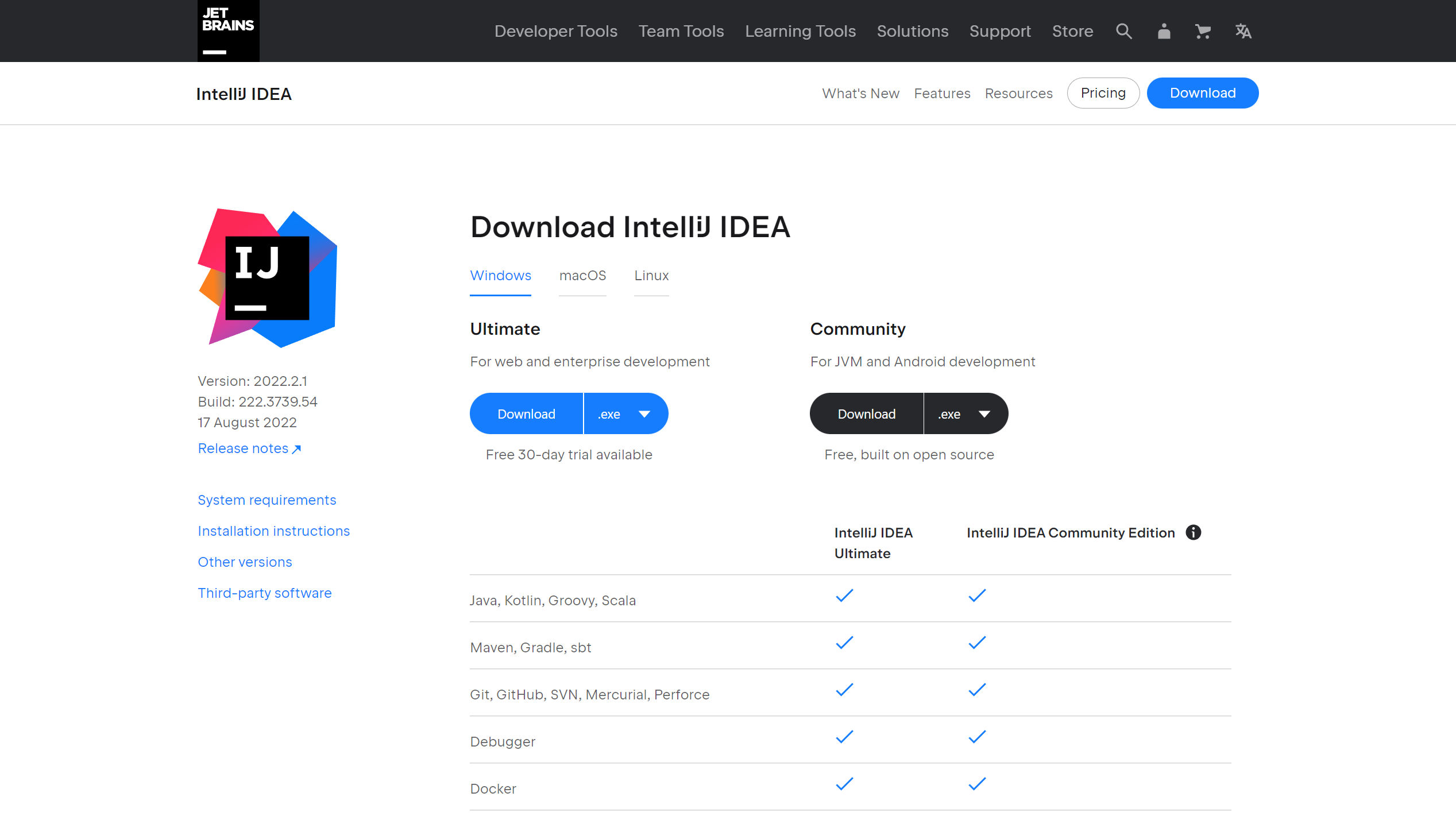
Once the download finishes, use the installer to install IntelliJ IDEA like any other software.
How to Create a New Project on IntelliJ IDEA
Use the shortcut from your start menu to start IntelliJ IDEA. The following window will show up:

Use the New Project button and a New Project window will show up:
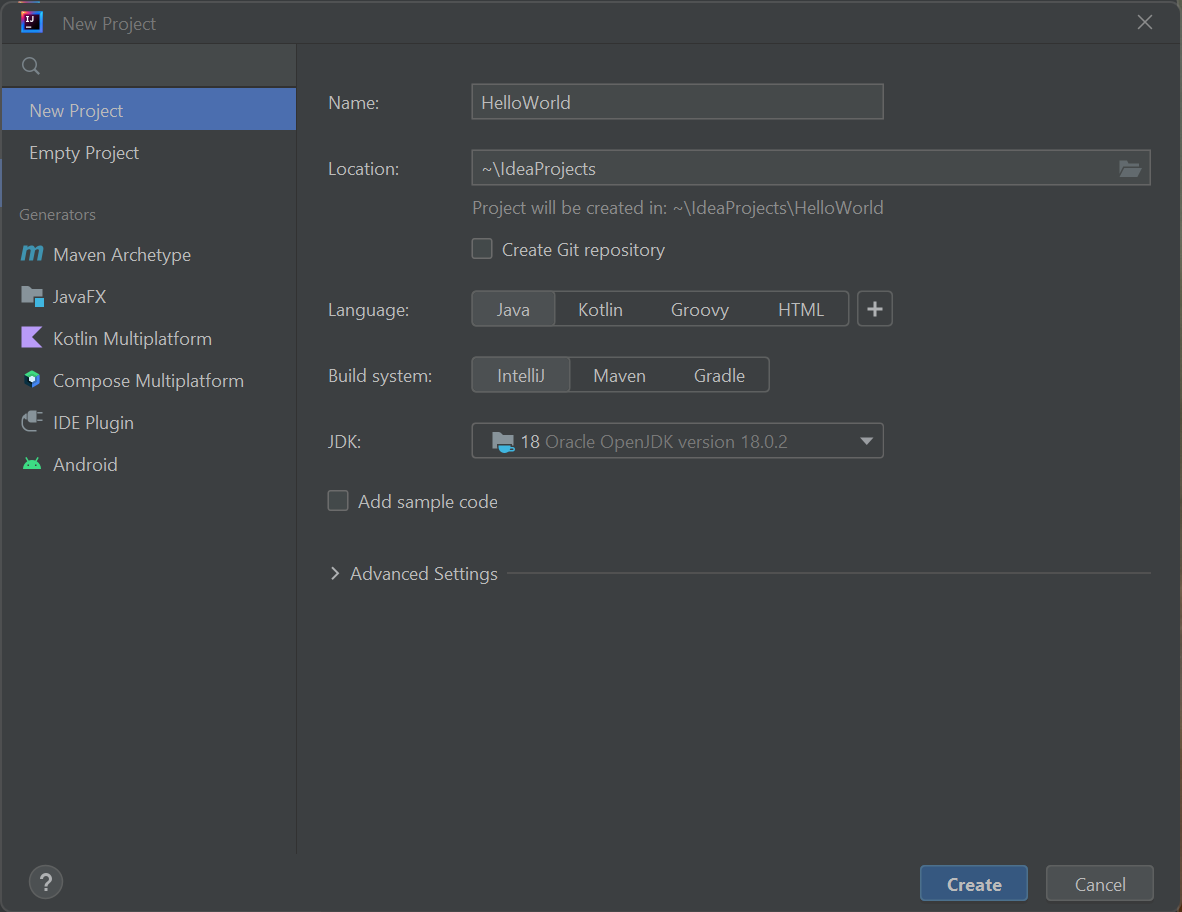
Put a descriptive name for your project. Leave the rest of the options as they are and press the Create button.
The project creation shouldn't take longer than a moment and once it's done, the following window will show up:
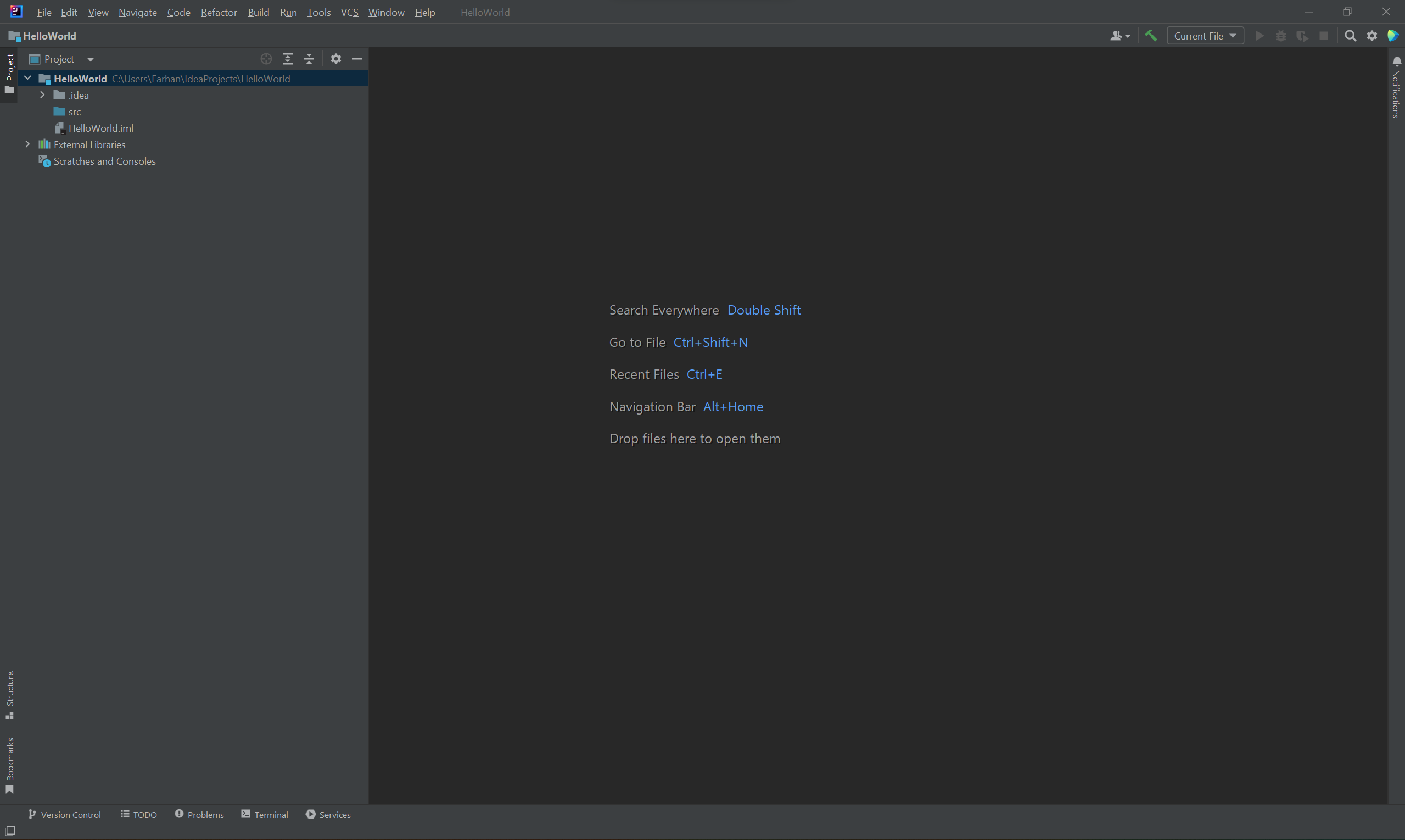
That's the project tool window on the left side. All your source code will live inside that src folder.
Right click on the src folder and go to New > Java Class .
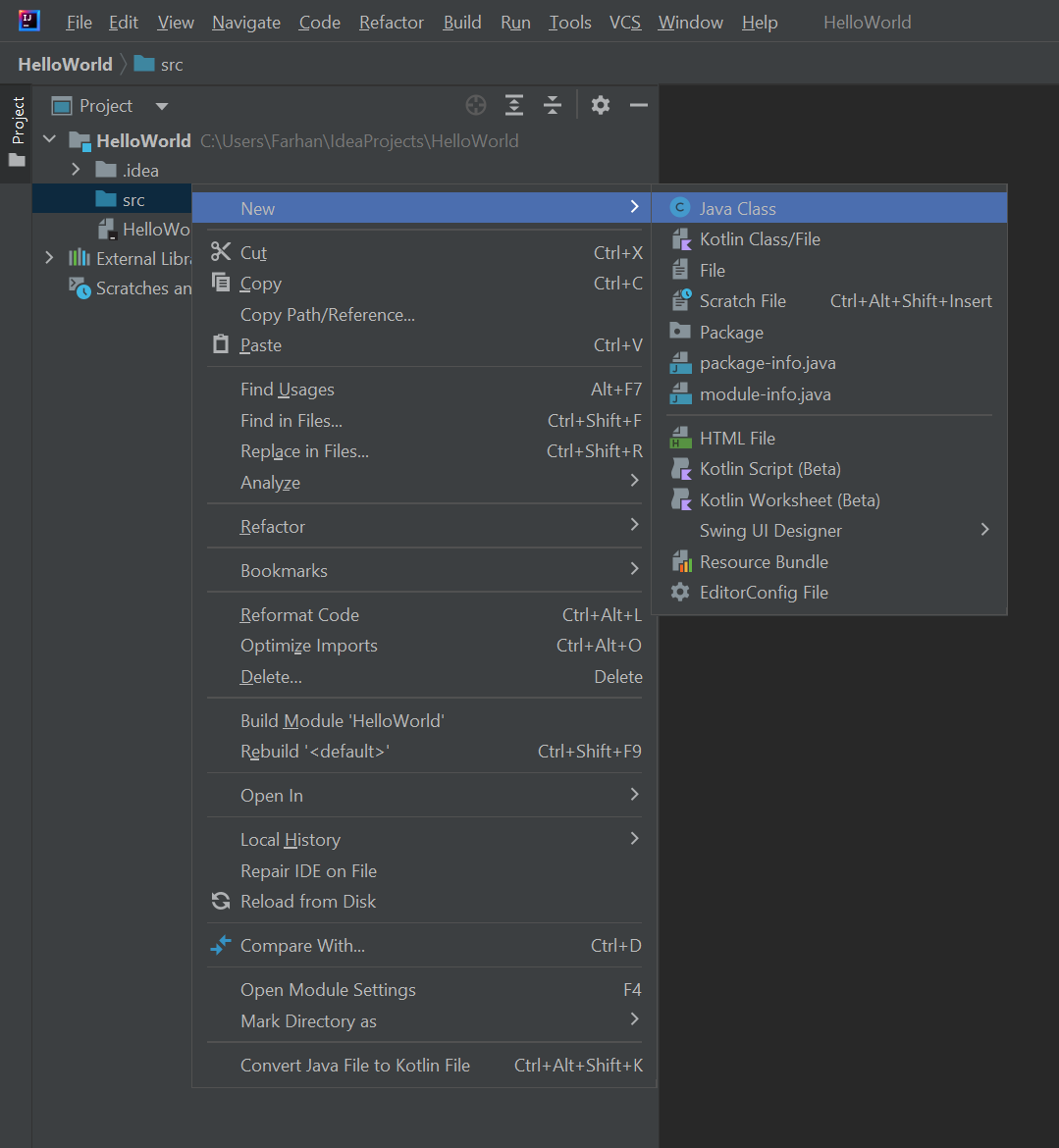
In the next step, put a name, such as Main for your class and make sure Class is highlighted as the type.
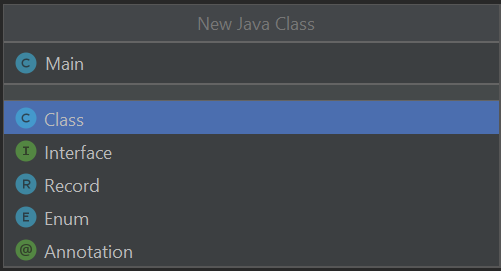
A new class will be created with a few lines of code.

Update the code as follows:
To run this code, use the green play button on the right side of the top bar.

The code will run and the output will be shown in the integrated terminal at the bottom of the window.
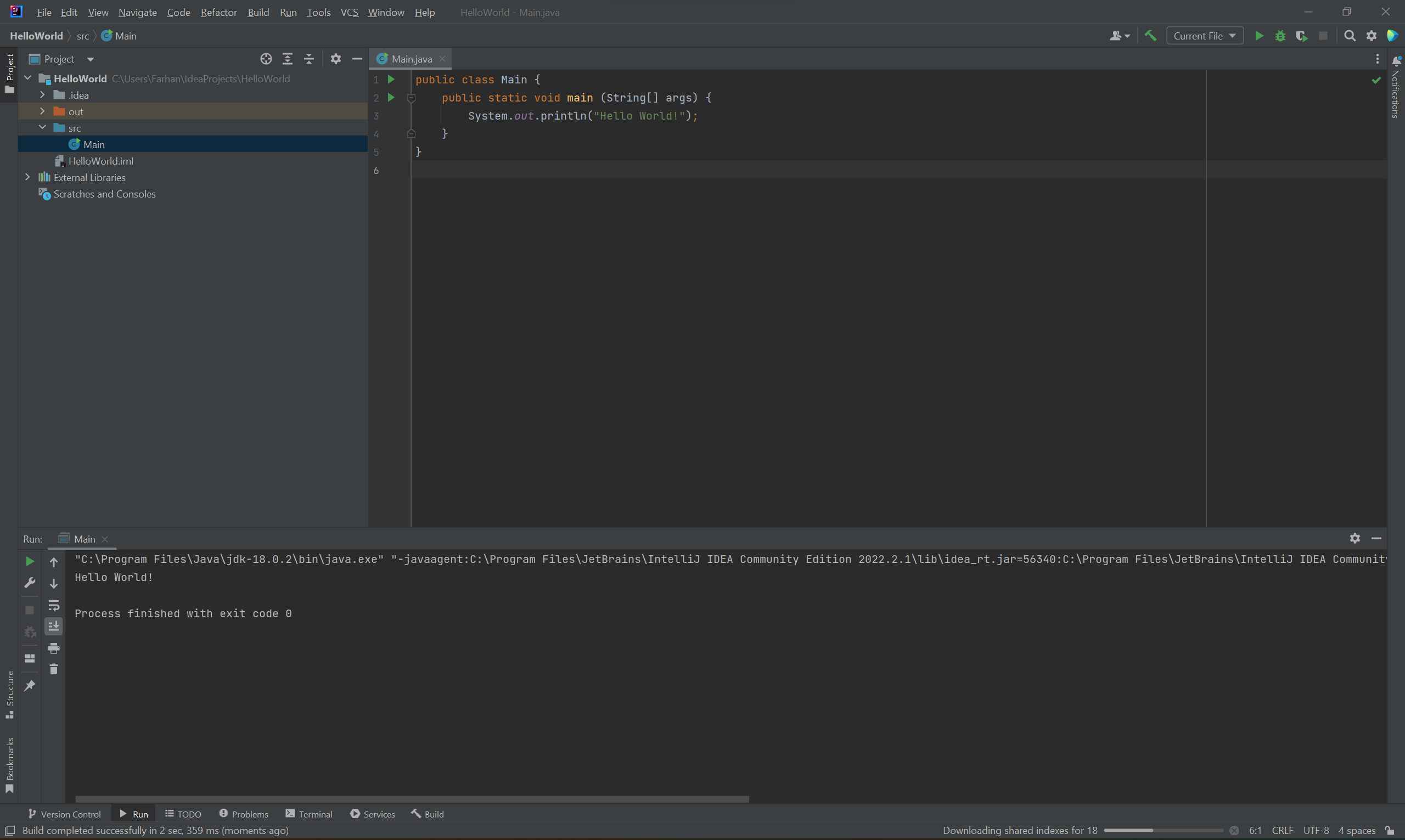
Congratulations, you've successfully recreated the previously discussed HelloWorld program in IntelliJ IDEA.
How to Work with Variables in Java
To work with different kinds of data in Java, you can create variables of different types. For example, if you want to store your age in a new variable, you can do so like this:
You start by writing out the type of data or variable. Since age is a whole number, its type will be integer or int for short, followed by the name of the variable age and a semicolon.
At the moment, you've declared the variable but you haven't initialized it. In other words, the variable doesn't have any value. You can initialize the variable as follows:
When assigning a value, you start by writing the name of the variable you want to initialize, followed by an equal sign (it's called the assignment operator) then the value you want to assign to the variable. And don't forget the semicolon at the end.
The System.out.println(); function call will print the line I am 27 years old. to the console. In case you're wondering, using a plus sign is one of the many ways to dynamically print out variables in the middle of a sentence.
One thing that you have to keep in mind is you can not use an uninitialized variable in Java. So if you comment out the line age = 27 by putting two forward slashes in front of it and try to compile the code, the compiler will throw the following error message at you:
The line The local variable age may not have been initialized indicates that the variable has not been initialized.
Instead of declaring and initializing the variable in different lines, you can do that in one go as follows:
The code should be back to normal again. Also, you can change the value of a variable as many times as you want in your code.
In this code, the value of age will change from 27 to 28 because you're overwriting it just before printing.
Keep in mind, while you can assign values to a variables as many times as you want, you can not declare the same variable twice.
If you try to compile this code, the compiler will throw the following error message at you:
The line Duplicate local variable age indicates that the variable has already been declared.
Apart from variables, you may find the term "literal" on the internet. Literals are variables with hardcoded values.
For example, here, age = 27 and it's not dynamically calculated. You've written the value directly in the source code. So age is an integer literal.
There are some rules when it comes to naming your variables in Java. You can name it anything as long as it doesn't start with a number and it can't contain any spaces in the name.
Although, you can start a variable name with an underscore (_) or a dollar sign ($), not being mindful of their usage can make your code hard to read. Variable names are also case sensitive. So age and AGE are two different variables.
Another important thing to remember is you can not use any of the keywords reserved by Java. There are around 50 of them at present. You can learn about these keywords from the official documentation but don't worry about memorizing them.
As you keep practicing, the important ones will slip into your neurons automatically. And if you still manage to mess up a variable declaration, the compiler will be there to remind you that something's wrong.
Apart from the rules, there are some conventions that you should follow:
- Start your variable name with small letter and not any special character (like an underscore or dollar sign).
- If the variable name has multiple words, use camel case: firstName , lastName
- Don't use single letter names: f , l
As long as you follow these rules and conventions, you're good to go. If you'd like to learn more about naming conventions in general, checkout my article on the topic .
A final variable in Java can be initialized only once. So if you declare a variable as final , you can not reassign it.
Since the age variable has been declared as final , the code will throw the following error message at you:
However, if you leave the variable uninitialized while declaring, the code will work:
So, declaring a variable as final will limit your ability to reassign its value. If you leave it uninitialized, you'll be able to initialize it as usual.
What are the Primitive Data Types in Java?
At a high level, there are two types of data in Java. There are the "primitives types" and the "non-primitive" or "reference types".
Primitive types store values. For example, int is a primitive type and it stores an integer value.
A reference type, on the other hand, stores the reference to a memory location where a dynamic object is being stored.
There are eight primitive data types in Java.
Yeah yeah I know the table looks scary but don't stress yourself. You don't have to memorize them.
You will not need to think about these ranges very frequently, and even if you do, there are ways to print them out within your Java code.
However, if you do not understand what a bit is, I would recommend this short article to learn about binary.
You've already learned about declaring an integer in the previous section. You can declare a byte , short , and long in the same way.
Declaring a double also works the same way, except you can assign a number with a decimal point instead of an integer:
If you assign an int to the double , such as 4 instead of 4.8 , the output will be 4.0 instead of 4 , because double will always have a decimal point.
Since double and float are similar, you may think that replacing the double keyword with float will convert this variable to a floating point number – but that's not correct. You'll have to append a f or F after the value:
This happens because, by default, every number with a decimal point is treated as a double in Java. If you do not append the f , the compiler will think you're trying to assign a double value to a float variable.
boolean data can hold either true or false values.
As you can imagine, false can be treated as a no and true can be treated as a yes.
Booleans will become much more useful once you've learned about conditional statements. So for now, just remember what they are and what they can hold.
The char type can hold any Unicode character within a certain range.
In this example, you've saved the percent sign within a char variable and printed it out on the terminal.
You can also use Unicode escape sequences to print out certain symbols.
The Unicode escape sequence for the copyright symbol, for example, is \u00A9 and you can find more Unicode escape sequences on this website .
Among these 8 types of data, you'll be working with int , double , boolean , and char majority of the time.
Type conversion in Java can be either "implicit" or "explicit". When the compiler converts a smaller type of data to a larger one automatically, it's known as an implicit or narrowing type conversion.
Since a double is larger than an integer, the compiler could easily perform the conversion. If you try to do the reverse however, you'll face the following error from the compiler:
When performing an implicit conversion, the flow of conversion should be as follows:
You can of course go from a short to a double , for example, skipping the others in between.
You can also go from smaller data types to larger ones. That's called an explicit or widening type conversion.
Previously you've seen that if you try to convert a larger data type to a smaller one, the compiler complains. But when you add the (int) cast operator explicitly, you show the compiler who's boss.
In doing so, you lose a part of your data. If you change the initial double number from 8.5 to just 8.0 , you'll not lose any information. So whenever you're performing an explicit conversion, be careful.
You can also convert a char to an int as follows:
70 is the ASCII code for the character F – that's why the output was like this. If you'd like to learn more about ASCII codes, my colleague Kris Koishigawa has written an excellent article on the topic.
The flow of conversion in this case will be the opposite of what you've seen already.
I'd suggest you to experiment by converting various values from one type to another and see what happens. This will deepen your understanding and make you confident.
What are Wrapper Classes in Java?
Wrapper classes can wrap around primitive datatypes and turn them into reference types. Wrapper classes are available for all eight primitive data types.
You can use these wrapper classes as follows:
All you have to do is replace the primitive data type with the equivalent wrapper class. These reference types also have methods for extracting the primitive type from them.
For example, age.intValue() will return the age as a primitive integer and the gpa.doubleValue() will return the GPA in a primitive double type.
There are such methods for all eight datatypes. Although you'll use the primitive types most of the time, these wrapper classes will be handy in some scenarios we'll discuss in a later section.
How to Use Operators in Java
Operators in programming are certain symbols that tell the compiler to perform certain operations such as arithmetic, relational, or logical operations.
Although there are six types of operators in Java, I won't talk about bitwise operators here. Discussing bitwise operators in a beginner guide can make it intimidating.
Arithmetic operators are the ones that you can use to perform arithmetic operations. There are five of them:
Addition, subtraction, multiplication, and division operations are pretty self-explanatory. Have a look at the following code example to understand:
Outputs from the first four operations need no explanation. In the last operation, you've performed a modulo/modulus operation using the % symbol. The result is 0 because if you divide 10 by 2, there'll be nothing left (no remainder).
Addition and multiplication operations are quite simple. But, when performing a subtraction, if the first operand is larger than the second operand, the result will be a negative number, just like in real life.
The type of data you're working with makes a difference in the result of division and modulo operations.
Although the result of this operation should've been 1.6 it didn't happen because in Java, if you divide an integer by another integer, the result will be an integer. But if you change both or one of them to a float/double, everything will be back to normal.
This principle applies to the modulo operations as well. If both or one of the operands are a float/double, the result will be a float/double.
You've already worked with the assignment operator in a previous section.
When you use the = symbol to assign a value to a variable, it works as an assignment operator. But, this is not the only form of this operator.
Combining the regular assignment operator with the arithmetic operators, you can achieve different results.
The following code example should make things clearer:
The other operators work the same. They operate and then assign the resultant value to the left operand.
I could demonstrate the other ones using code but I think if you try them out yourself, you'll get a better understanding. After all, experimentation and practice are the only ways to solidify your knowledge.
Relational operators are used to check the relation between operands. Such as whether an operand is equal to another operand or not.
These relational operators return either true or false depending on the operation you've performed.
There are six relational operators in Java.
The following code example demonstrates the usage of these operators:
Practical usage of these operators will become much apparent to you once you've learned about conditional statements in a later section.
You can also use these operators with characters.
What do you think the output of this code will be? Find out for yourself. Remember the ASCII values of the characters? They play a role in the output of this program.
Imagine a scenario where a program you've made can only be used by people who are 18 and up but not over 40 years old. So the logic should be as follows:
Or in another scenario, a user has to be a student of your school or member of the library to borrow books. In this case the logic should be as follows:
These logical decisions can be made using logical operators. There are three such operators in Java.
Let's see these operators in code. First, the logical and operator:
In this case, there are two conditions on either side of the && operator. If and only if both conditions evaluate to true , the and operation evaluates to true .
If the first condition evaluates to false , the computer will not evaluate the rest of the conditions and return false . Because if the first one evaluates to false , then there is no way for the entire operation to evaluate to true .
The logical or operator works similarly, but in this case, if any of the conditions are true then the entire operation will evaluate to true:
If the first condition of a logical or operation evaluates to true , the computer will not evaluate the rest of the conditions and return true . Because if the first condition evaluates to true the operation will evaluate to true regardless of what the other conditions evaluate to.
Finally the not operator evaluates to the opposite of whatever its condition evaluates to. Take a look at the following code example:
As you can see, the not operator returns the opposite of the given boolean value. The not operator is a unary operator, meaning it operates on a single operand.
In this example, the not operator turns isSchoolStudent into true , so the operation evaluates to true . However, if you modify the code as follows:
First, the logical or operation will take place and evaluate to true . The not operator will turn it into false .
Although you've used two operands with each operator, you can use as many as you want. You can also mix and match multiple operators together.
What do you think the output of this code will be? I'd recommend you find out by yourself. :)
There are some operators that are used with one operand at a time and these are called the unary operators. Although there are five of them, I'll only discuss two.
The following code example will demonstrate them nicely:
You can also use the operators as prefixes:
So far this is simple. But there are some slight differences between the postfix and prefix syntaxes that you need to understand. Look at the following code:
This is expected behavior. The prefix decrement operator will work the same. But look what happens if you switch to the postfix version:
Confusing, isn't it? What do you think is the actual value of the variable right now? It's 96. Let me explain.
When using the postfix syntax within a print function, the print function encounters the variable first and then increments it. That's why the second line prints out the newly updated value.
In case of the prefix syntax, the function encounters the increment operator first and performs the operation. Then it goes on to printing the updated value.
This little difference may catch you off guard if you're not careful. Or you try to avoid incrementing or decrementing within function calls.
How to Work with Strings in Java
The String type in Java is one of the most commonly used reference types. It's a collection of characters that you can use to form lines of text in your program.
There are two ways of creating new strings in Java. The first one is the literal way:
As you can see, declaring and using a String this way is not very different from declaring the primitive types in Java.
The second way to create a new String is by using the new operator.
This program will work exactly like the previous one but there's a slight difference between the two.
The JVM maintains a portion of your computer's memory for storing strings. This portion is called the string pool.
Whenever you create a new String in the literal way, the JVM first checks if that String already exists in the pool. If it does, JVM will reuse it. If it doesn't, then the JVM will create it.
On the other hand, when you use the new operator, the JVM will always create a new String object no matter what. The following program demonstrates this concept clearly:
As you may already know, the == operator is used for checking equality. The output of this program will be:
Since abc was already in the string pool, the literalString2 variable reuses that. In case of the object strings however, both of them are different entities.
You've already seen the usage of the + operator to sew strings together or format them in a specific way.
That approach works until you have a lot of additions to a string. It's easy to mess up the placements of the quotation marks.
A better way to format a string is the String.format() method.
The method takes a string with format specifiers as its first argument and arguments to replace those specifiers as the later arguments.
In the code above, the %s , and %d characters are format specifiers. They're responsible for telling the compiler that this part of the string will be replaced with something.
Then the compiler will replace the %s with the name and the %d with the age . The order of the specifiers needs to match the order of the arguments and the arguments need to match the type of the specifier.
The %s and %d are not random. They are specific for string data and decimal integers. A chart of the commonly used specifiers are as follows:
There is also %o for octal integers, %x or %X for hexadecimal numbers, and %e or %E for scientific notations. But since, we won't discuss them in this book, I've left them out.
Just like the %s and %d specifiers you saw, you can use any of these specifiers for their corresponding data type. And just in case you're wondering, that %f specifier works for both floats and doubles.
Checking the length of a string or making sure its not empty before performing some operation is a common task.
Every string object comes with a length() method that returns the length of that string. It's like the length property for arrays.
The method returns the length as an integer. So you can freely use it in conjunction with the integer format specifier.
To check if a string is empty or not, you can use the isEmpty() method. Like the length() method, it also comes with every string object.
The method returns a boolean value so you can use it directly in if statements. The program checks if the name is empty or not and prints out different responses based off of that.
The split() method can split a string based on a regular expression.
The method returns an array of strings. Each string in that array will be a substring from the original string. Here for example, you're breaking the string Farhan Hasin Chowdhury at each space. So the output will be [Farhan, Hasin, Chowdhury] .
Just a reminder that arrays are collections of multiple data of the same type.
Since the method takes a regex as argument, you can use regular expressions to perform more complex split operations.
You can also join this array back into a string like this:
The join() method can also help you in joining multiple strings together outside of an array.
Converting a string to upper or lower case is very straightforward in Java. There are the aptly named toUpperCase() and toLowerCase() methods to perform the tasks:
Since strings are reference types, you can not compare them using the = operator.
The equals() method checks whether two strings are equal or not and the equalsIgnoreCase() method ignores their casing when comparing.
How to Replace Characters or Substrings in a String
The replace() method can replace characters or entire substrings from a given string.
Here, the loremIpsumStd string contains a portion of the original lorem ipsum text. Then you're replacing the first line of that string and saving the new string in the loremIpsumHalfTranslated variable.
The contains() method can check whether a given string contains a certain substring or not.
The method returns a boolean value, so you can use the function in any conditional statement.
There were some of the most common string methods. If you'd like to learn about the other ones, feel free to consult the official documentation .
So far you've learned about the System.out.println() method to print out information on the terminal. You've also learned about the String.format() method in a previous section.
In this section, you'll learn about some siblings of the System.out.println() method. You'll also learn about taking input from the user.
Taking input from user is extremely easy in languages like Python. However in Java, it takes a few more lines of code.
The java.util.Scanner class is necessary for taking user inputs. You can bring the class to your program using the import keyword.
Then, you'll need to create a new instance of the Scanner class using the new keyword. While creating the new instance, you'll have to let it know your desired input stream.
You may want to take input from the user or from a file. Whatever it is, you'll have to let the compiler know about it. The System.in stream is the standard input and output stream.
The scanner object has methods like nextLine() for taking string input, nextInt() for taking integer input, nextDouble() for taking double input and so on.
In the code above, the scanner.nextLine() method will ask for a string from the user and return the given input with a newline character appended.
Then the scanner.nextInt() method will ask for an integer and return the given number from the user.
You may be seeing the System.out.printf() method for the first time here. Well, apart from the System.out.println() method, there is also the System.out.print() method that prints out a given string without appending a newline character to it.
The System.out.printf() is kind of a combination of the System.out.print() and String.format() methods. You can use the previously discussed format specifiers in this method as well.
Once you're done with taking input, you'll need to close the scanner object. You can do that by simply calling the scanner.close() method.
Simple right? Let me complicate it a bit.
I've added a new scanner.nextLine() statement after the scanner.nextInt() method call. Will it work?
No, it won't. The program will simply skip the last input prompt and print out the last line. This behavior is not exclusive to just scanner.nextInt() . If you use scanner.nextLine() after any of the other nextWhatever() methods, you'll face this issue.
In short, this happens because when you press enter on the scanner.nextInt() method, it consumes the integer and leaves the newline character in the input buffer.
So when scanner.nextLine() is invoked, it consumes that newline character as the end of the input. The easiest solution to this problem is writing an additional scanner.nextLine() call after the other scanner method calls.
There is another way of solving this problem. But I won't get into that here. If you're interested, checkout my article on this topic .
You use conditional statements for making decision based on conditions.
It's done using the if statement as follows:
The statement starts with an if and then there is the condition inside a pair of parenthesis. If the condition evaluates to true, the code within the curly braces will be executed.
Code enclosed between a set of curly braces is known as a code block.
If you change the value of age to 50 the print statement will not be executed and there'll be no output on the console. For these kind of situations where the condition evaluates to false , you can add an else block:
Now if the condition evaluates to false , the code within the else block will execute and you'll see you can not use the program printed on your terminal.
You can also have multiple conditions within an if-else if-else ladder:
Now, if the first condition evaluates to false then the second condition will be tested. If the second one evaluates to true then the code within curly braces will be executed. If the conditions in both if statements evaluate to false , then the else block will be executed.
You can also nest if statements within other if statements as follows:
In this case, only if the first if statement evaluates to true will the inner if statement be tested.
Apart from the if-else blocks, there are also switch cases where you can define multiple cases based on a single switch.
This is a very simple calculator program. The program prompts the user for two numbers and then asks what operation they would like to perform.
Every switch-case statement will have one switch and multiple cases. When you say case "sum" , the program checks whether the value of the switch or the operation variable in this is sum or not.
If it matches, the case body will execute. If none of the cases match, the default case will be executed.
And about that break statement. It does what it sounds like: stops the program from going into the next case.
If you remove the break statements, all the cases will be executed one after the other until the default case has been reached.
Scope is the lifetime and accessibility of a variable. Depending on where you declare a variable you may or may not be able to access it from other places.
Take the following code snippet as an example:
Here, the age variable is declared within the class code block. That means you can access this variable within the entire class without any issue. Since the variable is accessible in the entire class instance, it's an instance variable.
However, the isSchoolStudent and isLibraryMember variables have been declared within the first if statement code block. So it'll not be accessible outside of that code block.
But, it'll be accessible within any nested code block inside the first if block. These are called local variables.
There are also class variables declared using the static keyword but you'll learn about them in the object-oriented programming sections.
So for now, the rule of thumb is, a variable will be accessible within the code block it was declared at and any other code block nested inside the parent block.
You've learned that in Java, you need to initialize a variable after declaring it. Otherwise, you'll not be able to use that. Well that's not true in all cases.
If you declare a variable in the class level, that variable will be assigned a default value by the compiler.
Since the main method is static , it can only access static variables in the class level. I'll discuss static in greater detail in the object-oriented programming section.
But if you move the variable declaration inside the main method, it becomes local to that method and doesn't get any default value.
This code will throw the The local variable age may not have been initialized error on compilation.
Variables get their default values based on their type. In most cases, it'll be 0 or null . I'm giving a list of all the primitive types and their default values:
Any reference type will be assigned the value null by default. We'll discuss reference types, classes, and objects in the object-oriented programming sections.
How to Work with Arrays in Java
You've already learned about declaring single variables and using them in your program. This is where arrays come in.
Arrays are data structures containing multiple value sof the same data type in sequential memory locations. Arrays can be of any primitive or non-primitive data type.
You can create an array in Java as follows:
You start by typing out the type of data you want to hold in the array, char in this case. Then you write out the name of the array, vowels followed by a pair of square braces here. This pair of braces tells Java that you're declaring an array of characters and not a regular character variable.
Then you put an equal sign followed by the new operator used for creating new objects in Java. Since arrays are reference types in Java, new is required to create new instances.
You finish off the declaration by writing out the type again, followed by another pair of square braces enclosing the length of the array. Here, 5 means the array will hold five elements and not more than that.
When working with a single variable, you can refer to the variable simply by its name. But in case of an array, each element will have an index and arrays are zero-based. This means the first element in an array will have 0 as its index and not 1 .
To access an element in an array, you start by writing out the name of the array – in this case vowels followed by a pair of square braces enclosing your desired index. So if you want to access the first element in the array, you can do so as follows:
At this point, vowels[0] is similar to a regular character variable. You can print it out, assign new value to it, perform calculations in case of number types, and so on.
Since the array is empty at this moment, I'm assigning the character a to the first index. You can assign the rest of the vowels to the rest of the indices as follows:
Since the indices start at 0 they'll end at the length of the array - 1, which in this case is 4 . If you try to assign another element to the array like vowels[4] = 'x'; the compiler will throw the following error:
Arrays can not be printed like regular variables. You'll have to use a loop or you'll have to convert the array into a string. Since, I haven't discussed loops yet, I'll use the second method.
You'll need to first import the java.util.Arrays; and use the Arrays.toString() method to convert the array to a string. This class has a bunch of other interesting methods, but before discussing them, I'd like to show you how you can declare and initialize an array in one go.
The left side of the declaration syntax remains unchanged. However, after the assignment operator, instead of using new you write out the individual array elements separated by comma and enclosed within a pair of curly braces.
In this case the compiler will count the number of elements in the array and use that as the length of the array.
If you do not know the length of an array, you can take a look at the length property.
In this case, vowels.length will be 5 since there are five elements in the array. The length property is an integer and is present in every array in Java.
Arrays can also be multidimensional. So far you've worked with arrays that look like this:
Single dimensional arrays like this are perfect when you want to store a series of values. But imagine someone's daily medicine routine in the form of a table:
The first row represents the seven days in a week and the columns represent how many times the patient should take their medicine out of three times a day. 0 means no and 1 means yes.
You can map this routine using a multidimensional array in your program:
Multidimensional arrays can not be printed out using the regular Arrays.toString() method, you have to dig deeper.
Although the output doesn't look anything like the table, you can make it look like a table using some clever programming:
You've already learned about the replace() method for strings. You're just replacing the ending square brace in each line with a square brace and a newline character.
The first row represents the 7 weekdays and the rest of the rows are medicine routines for each day. Each line in this table represents an array.
To access a single value from a multidimensional array, you'll need two indices. The first index determines the row and the second one determines the column.
So medicineRoutine[2][3] will select the element in index 3 of the third array. That element will be 0 . Working with multidimensional array can seem a bit tricky but practicing will make it much easier.
Since you can create arrays of any type in Java, why don't you try creating some other types of arrays by yourself, huh?
One of the most common tasks that you will perform on arrays is sorting them. The java.utils.Arrays comes with the Arrays.sort() method to do just that:
The Arrays.sort() method takes the unsorted array as its argument and sorts it in place. So instead of getting a new sorted array in return, your original array itself will be sorted in ascending order.
By default, the method treats the first index of the array as its starting index and the length of the array as its ending index.
You can specify these two indices manually. For example, if you want to sort only u, o, i in ascending order and leave e, a as is, you can do so as follows:
This time, the method takes the array as the first parameter, the starting index as the second parameter, and the ending index as the third parameter. The rest of the behaviors stay the same as before.
Searching for values within a sorted value is another common task. The Arrays.binarySearch() method lets you search for items in a sorted array using the binary search algorithm.
The Arrays.binarySearch() method takes an array as its first parameter and the the search key (aka the item you're looking for) as its second parameter. It'll return the index of the found item as an integer.
You can store that index in an int and use that to access the element from the array as vowels[foundItemIndex] .
Note that the array has to be sorted in ascending order. If you're unsure of the array's ordering, use the Arrays.sort() method to sort it first.
By default, the method treats the first index of the array as its starting index and the length of the array as its ending index. But you can also specify those indices manually.
For example, if you want the search to take place from index 2 to index 4 , you can do so as follows:
This time the method takes the array you want to search on as the first parameter, the starting index as the second parameter, the ending index as the third parameter, and the search key as the fourth parameter.
Now the search will take place within i , o , and u . So if you look for a , it'll not be found. In cases where the given item is not found, you'll get a negative index. The resultant negative index will vary based on a number of factors but I won't get into those here. If you're interested in learning more, check out my article on the topic .
You've already learned about initializing an array with values but you may sometimes want to fill an entire array with the same value. The Arrays.fill() method can do that for you:
Like the Arrays.sort() method, Arrays.fill() also performs its operation in place. It takes your array as the first parameter, the value you want to fill the array with as the second parameter, and updates the original array in place.
This method also treats the first index as the starting index and the length of the array as the ending index. You can specify these indices manually as follows:
This time the method takes your array as the first argument, the starting index as the second argument, the ending index as the third argument, and the filler as the fourth argument.
Since arrays in Java are of reference types, copying them using the assignment operator can cause some unexpected behavior.
Although you've made changes to the source array, the copy reflects them as well. This happens because when you use the assignment operator to copy an array, the copy references the original array in the memory.
To properly copy an array, you can use the Arrays.copyOf() method as follows:
The method takes the source array as its first argument and the desired length of the new array as the second argument. If you want the length to be the same, simply pass the length of the original array using the length property.
If you put a smaller length, any value after that will be cut off and if you put a larger length, the new indices will be filled with the default value of the array data type.
There is another method Arrays.copyOfRange() that can copy a portion of an array to a new one:
This method takes the source array as its first argument, then the start index and finally the end index.
Keep in mind, the ending index is not inclusive. That's why 15 is absent from the new array. But if you want to include the last index of the array, use the length of the original array as the ending index.
Now the new array will also include 15 in it. You can also put a higher number than the length of the source array. In that case the newly added indices will contain the default value of the array data type.
If you try two check if two arrays are the same or not in Java using the equal relational operator, you'll get some unexpected results.
Even though the two arrays are identical, the output of the program is false . Since arrays are reference types, the relational operator will check whether they are the same instance or not.
To compare two arrays in Java, you can use the Arrays.equals() method:
However, if you change even a single element in either of these arrays, the output will be false since the arrays will not remain identical anymore.
You can also compare multidimensional arrays, but for that you'll have to use the Arrays.deepEquals() method instead of the regular one.
This method calls itself each time it encounters a new array inside the parent array.
These were some of the most common methods inside the java.util.Arrays class. You can consult the official documentation if you'd like to learn more.
How to Use Loops in Java
If you ever need to repeat a task for a set number of times, you can use a loop. Loops can be of three types: they are for loops, for...each loops, and while loops.
For loops are probably the most common types of loops that you'll see on the internet.
Every for loop consists of three parts. The initialization, condition, and update expression. The looping happens in multiple steps.
If you want to print the numbers from 0 to 10 using a for loop, you can do so as follows:
The flowchart for this loop looks like this:
- In the beginning of the loop, you initialize a new integer named number with the initial value of 0 .
- Then you check whether the number is less than or equal to 10 or not.
- If its less than or equal to 10 , you execute the statement inside the loop block and print out the number on the terminal.
- Then you update the number variable by incrementing its value by 1.
- The loop goes back to checking whether the value of number is still less than or equal or not.
As long as the value of number remains less than or equal to 10, the loop goes on. The moment the value of the number variable becomes 11 , the loop ends.
You can use a for loop to loop over an array as follows:
The flowchart for this loop will be as follows:
Since the last index of the array is one less than its length, you run the loop as long as the index is less than the array length. The moment the index becomes equal to the length of the array, you exit the loop.
One of the fun things that you can do with loops is printing out multiplication tables. For example, the multiplication table for 5 will be as follows:
Loops can also be nested. Which means you can put one loop inside another. You can print out the multiplication table of all the numbers from 1 to 10 using nested loops:
I wouldn't dare printing out the output here. Instead, try out the code by yourself. Draw out each iteration of the loop on a piece of paper so that you understand what's happening on each step.
If you want to iterate over a collection like an array and perform some operation on each element of that collection, you can use a for...each loop.
In the case of a for-each loop, the type of the item needs to match the type of the collection you're working with. Here, the array is of type integer so the item in the loop is of type integer.
This does the same task as the previously shown for loop. But in this one, you don't have to keep track of the index or use the square braces to access the elements. It looks cleaner and is less error prone.
If you want to execute a bunch of code until a certain condition is met, you can use a while loop.
There are no initialization or update steps in a while loop. Whatever happens, happens within the loop body. If you rewrite the program for printing out the multiplication table of 5 using a while loop, it'll be as follows:
Although, while loops are not as common as for loops in the real world, learning about them is worth it.
The final type of loop you'll learn about is the do-while loop. It kind of reverses the order of the regular while loop – so instead of checking the condition before executing the loop body, you execute the loop body first and then check the condition.
The multiplication table code implemented using a do-while loop will be as follows:
Do-while loops are very useful when you need to perform some operation until the user gives a specific input. Such as, show a menu until user presses the "x" key.
How to Work with Array Lists in Java
Arrays in Java are not resizable. Once you've set a length for an array, you can not change it any way. The ArrayList class in Java mitigates this limitation.
To create array lists, you'll need to import the java.util.ArrayList class at the top of your source file.
Then you start by writing ArrayList and then inside a pair of less than-greater than signs, you'll write the data type for the elements. Then you'll add the name of the array list itself followed by the assignment operator and new ArrayList<>() .
You can not create array lists of primitive types, so you'll have to use the corresponding wrapper class.
Although these elements have zero-based indices like arrays, you can not use the square brace notation to access them. Instead, you'll have to use the get() method:
The get() method will get the value in the given index. Just like get() you can use the set() method to update the value of an element.
The first parameter to the set() method is the index and the second one is the updated value.
There is no length property like in an array but you can use the size() method on any array list to find out its length.
You can remove elements from an array list using the remove method:
The remove() method can remove an element by value or by index. If you pass a primitive integer value to the method, it'll remove the element in the given index.
But if you pass an object like in this code, the method will find and delete that given element. The valueOf() method is present in all wrapper classes and it can convert a primitive value to a reference type.
You've already seen examples of the add() and remove() methods. There are two other methods addAll() and removeAll() for working with multiple elements.
Both methods accept collections as their parameter. In the code above, you're creating two separate array lists and joining them using the addAll() method.
Then you remove the elements from the second array list using the removeAll() method and the array list goes back to its original state.
You can also drop all elements from an array list using the clear() method:
The method doesn't require any parameter at all and also doesn't return any value. It just empties your array list in a single call.
The removeIf() method can remove elements from an array list if they meet a certain condition:
The method takes a lambda expression as a parameter. Lambda expressions are like unnamed methods. They can receive parameters and work with them.
Here, the removeIf() method will loop over the array list and pass each element to the lambda expression as the value of the number variable.
Then the lambda expression will check whether the given number is divisible by 2 or not and return true or false based on that.
If the lambda expression returns true , the removeIf() method will keep the value. Otherwise the value will be deleted.
To make a duplicate of an array list, you can use the clone() method.
The clone() method returns an object, so you'll have to cast it to a proper array list manually. You can compare two array lists using the equals() method just like in arrays.
How to Check if an Element Is Present or the Array List Is Empty
You can use the contains() method to check if an array list contains a given element or not:
If you want to check if an array list is empty or not, just call the isEmpty() method on it and you'll get a boolean in return.
You can sort an array list in different orders using the sort() method:
The sort() method takes a comparator as its parameter. A comparator imposes the order of sorting on the array list.
You can sort the array list in reverse order just by changing the passed comparator:
Comparators have other usages as well but those are out of the scope of this book.
How to Keep Common Elements From Two Array Lists
Think of a scenario where you have two array lists. Now you'll have to find out which elements are present in both array lists and remove the rest from the first array list.
The retainAll() method can get rid of the uncommon elements from the first array list for you. You'll need to call the method on the array list you want to operate on and pass the second array list as a parameter.
How to Perform an Action on All Elements of an Array List
You've already learned about looping in previous sections. Well, array lists have a forEach() method of their own that takes a lambda expression as parameter and can perform an action on all the elements of the array list.
Last time, the lambda expression you saw was a single line – but they can be bigger. Here, the forEach() method will loop over the array list and pass each element to the lambda expression as the value of the number variable.
The lambda expression will then multiply the supplied value by 2 and print it out on the terminal. However, the original array list will be unchanged.
How to Work With Hash Maps in Java
Hash maps in Java can store elements in key-value pairs. This collection type is comparable to dictionaries in Python and objects in JavaScript.
To create hash maps, you'll first have to import the java.util.HashMap class at the top of your source file.
Then you start by writing HashMap and then inside a pair of less than-greater than signs, you'll write the data type for the key and the value.
Here, the keys will be strings and values will be doubles. After that the assignment operator, followed by new HashMap<>() .
You can use the put() method to put a record in the hash map. The method takes the key as the first parameter and its corresponding value as the second parameter.
There is also the putIfAbsent() method that adds the given element only if it already doesn't exist in the hash map.
You can use the get() method to bring out a value from the hash map. The method takes the key as its parameter.
There is another variation of the this method. The getOrDefault() method works like get() but if the given key is not found, it'll return a specified default value.
The default value has to match the type of the values in the hash map. You can update a value in a hash map using the replace() method:
For removing elements from a hash map, you can use the aptly named remove() method:
If you ever need to know how many entries are there in a hash map, you can do so by using the size() method:
Finally if you want to clear a hash map in Java, you can do so by using the clear() method.
Just like in the array lists, the method doesn't take any argument or return any value.
How to Put in or Replace Multiple Elements in a Hash Map
If you want to put multiple elements into a hash map in a single go, you can do so by using the putAll() method:
The method takes another hash map as its parameter and adds its elements to the one the method has been called upon.
You can also use the replaceAll() method to update multiple values in a hash map.
The replace all method iterates over the hashmap and passes each key value pair to the lambda expression.
The first parameter to the lambda expression is the key and the second one is the value. Inside the lambda expression, you perform your actions.
How to Check if a Hash Map Contains an Item or if It’s Empty
You can use the methods containsKey() and containsValue() for checking if a hash map contains a value or not.
Difference between the two methods is that the containsKey() method checks if the given key exists or not and the containsValue() method checks if the given value exists or not.
And if you want to check if a hash map is empty or not, you can do so by using the isEmpty() method:
Since the method returns a boolean value, you can use it within if-else statements.
How to Perform an Action on All Elements of a Hash Map
Like the array lists, hash maps also have their own forEach() method that you can use to loop over the hash map and repeat a certain action over each entry.
The method loops over each entry and passes the key and value to the lambda expression. Inside the lambda expression body, you can do whatever you want.
Classes and Objects in Java
Here's a helpful definition of object-oriented programming :
OOP (Object-oriented programming) is a programming paradigm based on the concept of "objects", which can contain data and code: data in the form of fields (often known as attributes or properties), and code, in the form of procedures (often known as methods).
Imagine a library management system where members of the library can log in, take a look at the books they have already borrowed, request new ones, and so on.
In this system the users and the books can all be objects. These objects will have their own properties such as name and birthday (in case of a user) and title and author in case of the books.
Classes in object oriented programming are blueprints for the aforementioned objects. We've already discussed the possible properties of the user and book objects.
To create a new User class, right click on the src folder once again. Then go to New > Java Class , name it User , and hit enter.
Keeping the previously discussed properties in mind, your code for the User class should be as follows:
The LocalDate is a reference data type that represents a date. Now go back to the Main.java file and create a new instance of this class:
Creating a new user is not very different from creating a new string or array. You start by writing out the name of the class then the instance or object name.
Then you put the assignment operator followed by the new keyword and the constructor call. The constructor is a special method that initializes the object.
The constructor has initialized the object properties with default values which is null for all these reference types.
You can access the properties of the object by writing out the name of the object followed by a dot and then the name of the property.
The LocalDate.parse() method can parse a date from a given string. Since the birthDay is a reference type, you'll have to use the toString() method to print out on the console.
The variables or properties of a class describe the state of its objects. Methods on the other hand describes the behavior.
For example, you can have a method within your User class that calculates the user's age.
Here, the this keyword represents the current instance of the class. You start by writing out the return type of the method. Since the age of a user is an integer, the return type of this method will be int .
After the return type, you write the name of the method, followed by a pair of parenthesis.
Then you write the method body within a pair of curly braces. The Period class in Java expresses a time frame in the ISO-8601 calendar system. The LocalDate.now() method returns the current date.
So the Period.between(this.birthDay, LocalDate.now()).getYears() method call will return the difference between the current date and the date of birth in years.
Now back in the Main.java file, you can call this method as follows:
Methods can also accept parameters. For example, if you want to create a method borrow() for inserting new books into the list of borrowed books for this user, you can do so as follows:
Back in the Main.java file, you can call this method as follows:
Let's create a class for the books as well:
Books often have multiple authors. Now you can create a new book instance back in the Main.java file.
Now let's go back to the User.java file and create a relationship between the users and the books:
Instead of using an array list of strings, you're now using an array list of books to store the books borrowed by this user.
Since the argument type of the method has changed, you'll have to update the code in the Main.java file accordingly:
Everything works out fine except the fact that book information has not been printed properly.
I hope you remember the toString() method. When you call user.borrowedBooks.toString() the compiler realizes that the items stored in the arraylist are objects or reference types. So it starts calling the toString() methods inside those items.
The problem is, there is no proper implementation of toString() in your Book class. Open Book.java and update its code as follows:
The toString() method now returns a nicely formatted string instead of the object reference. Run the code once again and this time the output should be Farhan has borrowed these books: [Carmilla by [Sheridan Le Fanu]] .
As you can see, being able to design your software around real-life entities makes it a lot more relatable. Although there is just an array list and a bunch of strings in play, it feels like as if a real book borrowing operation is going on.
In Java, multiple methods can have the same name if their parameters are different. This is called method overloading.
One example can be the borrow() method on the User class. Right now, it accepts a single book as its parameter. Let's make an overloaded version which can accept an array of books instead.
The return type and name of the new method is identical to the previous one, but this one accepts an array of Book objects instead of a single object.
Let's update the Main.java file to make use of this overloaded method.
As you can see, the borrow() method now accepts an array of books or a single book object without any issue.
Constructors are a special kind of method that exists in every class, and whenever you create a new object from a class, the compiler calls it.
Since the method is called during the construction of an object, it's called a constructor. By default, a constructor assigns default values to all its properties.
To override the default constructor, you need to create a new method under your classes with the same name as the class.
Now that you have a constructor, instead of parsing the date from a string in the Main.java file, you can do that here.
This is because the format of the birthday is the concern of the User class and the Main class doesn't need to bother about it.
Same treatment for the book class as well:
Again, the type of the authors collection is not a concern of the Main class. The most basic way of working with a bunch of values in Java is an array.
So you'll receive the author names as an array from the Main class and create an array list out of it in the Book class.
Now you'll have to pass those parameters to the constructor when creating a new user or book object in the Main.java file.
Look how much cleaner it already looks. But soon it'll look better.
You've already seen the keyword public multiple times. This is one of the access modifiers in Java.
There are four access modifiers in Java:
For now, I'll discuss the Default , Public and Private access modifiers. Protected will be discussed in a later section.
You've already learned about classes. Packages are collections of multiple classes separated by their functionality.
For example, if you're making a game, you can put all the physics-related classes in a separate package and the graphics-related ones in a different one.
Packages are outside of the scope of this book, but as you keep working on larger and larger projects, you'll get the hang of them.
The Public access modifier is pretty self-explanatory. These variables, methods, or classes are accessible from any other class or package in your project.
The Private ones, on the other hand, are the opposite. They're only available within their class.
Take the User class, for example. The name and birthday of a user shouldn't be accessible from the outside.
That's better. Update the Book class as well to hide the title and author information from the outside world.
Since the properties have become private now, the System.out.println() line in the Main.java file will fail to directly access them and will cause an issue.
The solution to this program is writing public methods that other classes can use to access these properties.
Getters and setters are public methods in classes used to read and write private properties.
The getName() and getBorrowedBooks() are responsible for returning the value of the name and borrowedBooks variables.
You never actually access the birthday variable out of the age() method, so a getter is not necessary.
Since the type of the borrowedBooks variable is not a concern of the Main class, the getter makes sure to return the value in the proper format.
Now update the code in the Main.java file to make use of these methods:
Excellent. It has become even cleaner and easier to read. Like getters, there are setters for writing values to the private properties.
For example, you may want to allow the user to change their name or birthday. The borrow() method already works as a setter for the borrowedBooks array list.
Now you can call the setName() method with whatever name you want to set to the user. Similarly, the setBirthDay() method can set the birthday.
You can implement some getters and setters for the Book class as well.
Now you can not access those properties directly. Instead you'll have to use one of the getters or setters.
Inheritance is another big feature of object oriented programming. Imagine you have three kinds of books. The regular ones, e-books, and audio books.
Although they have similarities such as title and author, they also have some differences. For example, the regular books and e-books have page count whereas audio books have run time. The e-books also have format such as PDF or EPUB.
So using the same class for all three of them is not an option. That doesn't mean you'll have to create three separate classes with minor differences, though. You can just create separate classes for e-books and audio books and make them inherit the properties and methods from the Book class.
Let's begin by adding the page count in the Book class:
Since you're not going to use getters and setters in these examples, cleaning up seemed like a good idea. The length() method returns the length of the book as a string.
Now create a new Java class named AudioBook and put the following code in it:
The extends keyword lets the compiler know that this class is a subclass of the Book class. This means that this class inherits all the properties and methods from the parent class.
Inside the AudioBook constructor method, you set the run time for the audio book which is fine – but you'll also have to manually call the constructor of the parent class.
The super keyword in Java refers to the parent class, so super(title, 0, authors) essentially calls the parent constructor method with the necessary parameters.
Since the audio books don't have any pages, setting the page count to zero can be an easy solution.
Or you can create an overloaded version of the Book constructor method that doesn't require the page count.
Next, create another Java class named Ebook with the following code:
This class is largely identical to the Book class except the fact that it has a format property.
So far everything is working fine. But do you remember the length() method you wrote inside the Book class? It'll work for the regular books but will break in the e-books.
That's because the page count property is marked as private and no other class except Book will be able to access it. The title is also a private property.
Open the Book.java file and mark the title and pageCount properties as protected.
This'll make them accessible from the subclasses. The audio books have another problem with the length() method.
Audio books don't have a page count. They have run times and this difference will break the length method.
One way to solve this problem is by overriding the length() method.
As the name suggests, overriding means cancelling the effect of a method by replacing it with something else.
You override a method from the parent class by rewriting the method in the subclass. The @Override keyword is an annotation. Annotations in Java are metadata.
It's not mandatory to annotate the method like this. But if you do, the compiler will know that the annotated method overrides a parent method and will make sure you're following all the rules of overriding.
For example, if you make a mistake in the method name and it doesn't match any method from the parent, the compiler will let you know that the method is not overriding anything.
Cool, isn't it? Whenever overriding a method in Java, keep in mind that both the original and overridden method must have the same return type, same name, and same parameters.
I would like to thank you from the bottom of my heart for the time you've spent on reading this book. I hope you've enjoyed your time and have learned all the fundamental concepts of Java.
This handbook is not frozen in time. I'll keep working on it and I'll update it with improvements, new content, and more. You can provide anonymous opinions and suggestion on the handbook in this form .
Apart from this one, I've written full-length handbooks on other complicated topics available for free on freeCodeCamp .
These handbooks are part of my mission to simplify hard to understand technologies for everyone. Each of these handbooks takes a lot of time and effort to write.
If you've enjoyed my writing and want to keep me motivated, consider leaving starts on GitHub and endorse me for relevant skills on LinkedIn .
I'm always open to suggestions and discussions on Twitter or LinkedIn . Hit me up with direct messages.
In the end, consider sharing the resources with others, because:
In open source, we feel strongly that to really do something well, you have to get a lot of people involved. — Linus Torvalds
Till the next one, stay safe and keep learning.
Software developer with a knack for learning new things and writing about them
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
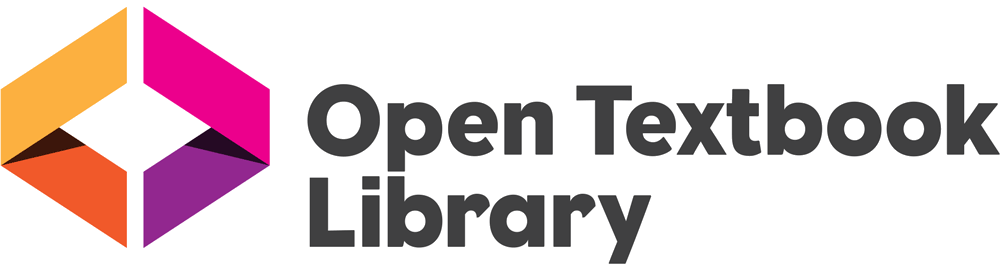
Introduction to Programming Using Java - Eighth Edition
(6 reviews)

David J. Eck, Hobart and William Smith Colleges
Copyright Year: 2015
Publisher: David J. Eck
Language: English
Formats Available
Conditions of use.

Learn more about reviews.
Reviewed by Tanvir Irfan Chowdhury, Assistant Professor, Marshall University on 2/1/24
This book covers all the essential topics in Java development. It is not just for beginners; experienced programmers can also review the chapters for a quick reference. It can be used both as an introductory programming class using Java and also... read more
Comprehensiveness rating: 5 see less
This book covers all the essential topics in Java development. It is not just for beginners; experienced programmers can also review the chapters for a quick reference. It can be used both as an introductory programming class using Java and also as upper level class that covers GUI programming using Java.
Content Accuracy rating: 5
I haven't seen any example with an obvious error. Having the source code available online for practice is a nice feature.
Relevance/Longevity rating: 5
Java is updated regularly. Although it is hard to keep up with the updates, I still think the book is using a fairly new version of Java. The reader will not miss much with the used version and if they want to learn the latest version, they can do it easily.
Clarity rating: 4
The book is easy to follow and flows naturally from beginning to end. It introduces various concepts not generally seen in a programming language book, such as section 8.5, Analysis of Algorithms. While I appreciate the author's initiative, the example used in the first paragraph is rather exaggerated/dramatic ("..correct answer after ten thousand years...").
Consistency rating: 5
The book uses consistent terminology throughout. All the codes/code segments were formatted using the same style. While the authors provided an explanation for using the term "subroutine" in chapter 4, I personally still think using the name "method" would be more beneficial for the reader.
Modularity rating: 5
The book's modularity enables instructors of introductory Java programming courses to teach with ease. Instructors have the flexibility to select essential chapters while reserving more advanced topics for students at higher levels of proficiency.
Organization/Structure/Flow rating: 5
The book is organized in a logical fashion.
Interface rating: 5
Navigating the book is incredibly straightforward. The website provides various options such as online access, PDF downloads, and other convenient methods to explore its contents.
Grammatical Errors rating: 5
I did not notice any obvious grammatical errors in my reading.
Cultural Relevance rating: 5
While, I do not see examples of the inclusion of various races, ethnicities, and backgrounds. The most important thing is I also did not see any insensitive, or offensive content either. For the subject matter, I believe the book is perfectly fine.
Reviewed by Zhenguang Gao, Professor, Framingham State University on 6/15/21
The book covers all parts needed for a freshman course. It is can be used as one semester or one year book for Java programming. read more
The book covers all parts needed for a freshman course. It is can be used as one semester or one year book for Java programming.
The syntax explanations are precise. The Java examples are error free.
Relevance/Longevity rating: 4
The book doesn't use the most recent version of Java Environment. However, I do not think that is a problem. Beginners do not need the up-to-date new syntax to do well in a problem solving course.
Clarity rating: 5
The book is very concise, and easy to follow.
The book is very well organized in style. Similar formats are used from the beginning to the end.
Modularity rating: 4
The book follows the standard modularity for a first programming course. It begins with an introduction to computation, then followed by Java basics. It is a great book for a fast paced course. However, for some schools with a slow paced programming course, covering more basic programming skills and more examples could improve the learning experience.
Organization/Structure/Flow rating: 4
I feel the book should cover the basics in more details and more examples. The order of the some topics are not conventional. For example, "if" structure is covered after the looping structures; and programming environment is in the last section of Chapter 2, not in the first section.
The book is very easy to use. The website offers online, PDF, and other ways to navigate.
I do not see any grammatical errors.
Cultural Relevance rating: 4
The book doesn't not make use of a lot of examples relevant to races, ethnicity, or diversity. However, I did not see any culturally insensitive, or offensive content.
I'd recommend the book for any one looking for a first year programming course.
Reviewed by Eric Jiang, Professor, University of San Diego on 4/18/21
This book is quite comprehensive and it provides all foundational topics for beginners to learn the Java programming language. In addition, it offers a nice overview of Java programming environments and includes chapters on graphical user... read more
This book is quite comprehensive and it provides all foundational topics for beginners to learn the Java programming language. In addition, it offers a nice overview of Java programming environments and includes chapters on graphical user interfaces and programming with multi-threading. The book is suitable for a typical first programming course in Java and some of the book’s material can also be used for a subsequent programming course.
I did not find any technical errors or inaccuracies in the book.
The current 8th edition of the book is based on Java 8 and it uses JavaFX for GUI programming, so the book’s content is quite up-to-date. Of course, as more features are periodically added to the Java language, the book will need to be revised accordingly.
The book is extremely well-written and easy to follow. In fact, the author uses a conversational writing style and provides clear explanations of concepts and principles throughout the book. All of these make it a good text book for beginners.
The author uses consistent terminology internally. But the term “subroutine” introduced in the book would not be consistent with other Java sources including Oracle’s Java Documentation where the term “method” is used. This external term inconsistency may cause some confusions to readers.
The book is generally modular. One could cluster and rearrange some of its chapters or sections to fit an intro to Java class. However, the book’s modularity could be further enhanced by re-organizing its coverage of some topics. For instance, it would be good to separate the section on recursion from linked data structures and to consider expanding it to a chapter because the recursion concept and recursive algorithms are usually difficult to comprehend for many beginners.
The author does a good job presenting the material in a logical manner. The book starts basic language constructs and then covers methods, program design, classes, arrays and some more advanced topics. At the end of each chapter, it also includes programming exercises and quizzes. One suggestion I have for book’s organization is to delay the coverage of some class related topics including inheritance, polymorphism and interfaces to a later chapter. In addition, the coverage on data structures (stacks, queues and binary trees) may be expanded so the book could also be used in a second programming course in Java.
Interface rating: 4
The book’s interface is good and it is quite easy to navigate all book components. However, I feel the interface could be improved by adding a glossary and also an index.
This is a very well-edited book.
All examples and exercises from the book are very good and they are not culturally insensitive.
Overall, this is a great book for introducing Java programming. It has a very decent coverage of all important foundational topics and provides clear explanations and nice examples and exercises. Plus, it is freely accessible.
I will definitely consider using the book in some of my future classes.
Reviewed by Joey Cho, Professor, Colorado State University - Pueblo on 12/24/19
The book covers all important and necessary topics for beginners in 13 chapters. read more
The book covers all important and necessary topics for beginners in 13 chapters.
The content is very accurate but here's minor things: 1. On page 14, the word "but" was typed twice (...but but has recently been removed...) 2. In section 10.1.4, the abbreviation for Java Collection Framework should be JCF not JFC.
Content is very fresh with a full of good examples and exercises. The previous version describes Swing libraries for the graphical user interface but the current version describes the up-to-date JavaFX.
The text was written with very easy words and explains concepts clearly with appropriate examples and pictures.
The text has a very good flow with a consistent jargon and framework. For example, in section 3.8, the concept of Arrays is introduced and explained later in detail in chapter 7. Also, the GUI programming is introduced in section 3.9 as an appetizer and described fully in chapter 6 and 13 with consistency.
The text is consisted of 13 chapters and many sub sections to facilitate any instructor/user to reconstruct/reorganize the content without any difficulties.
The essential topics of Java programming are organized in a way for beginners to read and study easily.
Any interface issues are found. The PDF file that can be downloaded from the open book site provides bookmark links to specific topics of interest, and the table of contents are hyperlinked. Also, all of the name of example code listed in the text have a link to actual code.
No grammatical errors are found.
The text is full of good examples and exercises without having any bias culturally and ethnically.
The introduction to the Conway’s Game of Life and the Checkers in chapter 7, and the Towers of Hanoi and the Blob Counting in chapter 8 are very useful examples for students who are interested in game programming. Also, 3 network programming examples (a simple chat room, a networked Tic-tac-toe game, and a networked Poker game) in section 12.5 could attract a lot of student's attention.
In section 6.1.3, when a BorderPane is explained, it would be good to show a picture of a BorderPane of the 5 section as shown in section 6.5.2 or place a link to that section.
Reviewed by John McManus, Assistant Professor, Randolph-Macon College on 3/27/18
This textbook is comprehensive. It covers a significant amount of material in a well-thought out and logical manner. The text starts with basic programming concepts introducing variables, types and input and output. The author covers the basics... read more
This textbook is comprehensive. It covers a significant amount of material in a well-thought out and logical manner. The text starts with basic programming concepts introducing variables, types and input and output. The author covers the basics of a variety of programming environments including Eclipse, NetBeans, and the command line environment. The next section introduces control structures, exception handling, and introduces arrays and GUI programming. The early introduction of arrays and GUI program is a nice feature, allow students to add address slightly more complex problems. The book introduces objects and classes late, allowing introductory students to focus on syntax and basic problem solving before adding objects. I believe the text is well suited to a two-semester introductory sequence, or an upper level Software Design Course. The text includes quizzes at the end of each chapter, as well as programming exercises.
I did not see any technical errors or inaccuracies in the book.
The book uses the Swing library used to build GUI applications. Swing has been replaced with JavaFX. Swing is still widely used and okay for an introductory courses; the text should be updated to cover JavaFX.
The author uses an easy to read, conversational writing style and provides very thorough explanations. The flow is very logical, with sections building on the prior section.
The author uses consistent, and for the most part, modern terminology. The use of “subroutine” can be a confusing to students. The more correct term “methods” is also used and it’s not clear why “subroutines” is introduced. I appreciate the use of JavaDoc.
The text is as modular, and the order that the modules are introduced in is very logical. It is possible to re-order the modules to match your preferences for introducing specific topics.
I like the organization of the book for an introductory course, and for a course on software design. approach. Objects and classes are covered in chapter five, after the basic programming building blocks such as control structures and methods have been covered. This allows you to choose the depth that you cover topics, going slower in an introductory class, but faster in a course on Software design. I would recommend moving some sections around. I like to introduce arrays early, and I defer exceptions until a bit later.
I did not find any interface issues. The text includes PDF links in the table of contents, and also when the text makes a reference to another section. The author also includes links to the full code examples on the book's web site. Figures are easy to read and high resolution.
The text is well edited. I found a very small number of spelling or grammatical errors in the book. The book is “cleaner” that many professional edited textbooks.
I didn't notice any instances of the text being culturally insensitive. It is difficult to always find neutral examples or references. The sample problems are appropriate.
This is one of the best Java programming texts that I have reviewed. I am currently using a different text and plan to switch to this text next semester. It is very detailed. The author provides explanations of the core concepts and provides great examples. The modular approach allows it to be used in an introductory CS class, with Java as a first language; and in a software design class focusing on object-oriented design.
Reviewed by Ian Finlayson, Assistant Professor, The University of Mary Washington on 6/20/17
This textbook is remarkably comprehensive. It covers a tremendous amount of material, including nearly every facet of the Java programming language (such as anonymous inner classes, lambdas, variable arity methods etc.). It also includes a... read more
This textbook is remarkably comprehensive. It covers a tremendous amount of material, including nearly every facet of the Java programming language (such as anonymous inner classes, lambdas, variable arity methods etc.). It also includes a chapter covering basic data structures such as lists, stacks, queues and binary trees, as well as chapters on multi-threading and networking, in addition to its thorough and integrated coverage of graphical user interfaces. When using this text for a one semester CS 1 course, I use roughly half of the content. I would probably not use it for a non-major's CS 0 course, as it could be an overwhelming amount of material for students.
The book is excellent for self-study - many students love having all the extra material available even if we don't cover it in class.
One area where I would have like to have seen more content is in the books coverage of recursion. There is one section in chapter nine dealing with recursion which contains four examples. Recursion is also used for implementing lists and trees, but it would be nice to have a slightly longer treatment as it is a confusing topic for many beginning students.
The text does not include an index. The book itself also does not contain a glossary, but there is one on the companion web site. The text includes quizzes at the end of each chapter, as well as programming exercises.
I did not notice any technical errors or inaccuracies in the book.
The book mostly covers Java 7, with some treatment of Java 8 features, so as of now, the book is perfectly up to date. Future changes to Java likely won't necessitate major changes to the text, and the author has updated the text several times (currently on version 7).
The one area of slight concern is with the Swing library used to build GUI applications. Oracle has replaced Swing with JavaFX, which is the new preferred way of writing GUIs in Java. Still, Swing is widely used and a fine thing to use for introductory courses. Moreover, Swing will be a supported part of Java for a long time as it is still so widely used.
I think the clarity of writing is the best feature of this text. The author uses an easy to read, conversational writing style. The text is also very thorough in its explanations.
The author does a good job using consistent terminology. He explains new terms which are introduced and is very careful about phrasing in general. For instance when talking about objects he has this to say:
"It is certainly not at all true to say that the object is 'stored in the variable std.' The proper terminology is that 'the variable std refers to or points to the object".
Actually speaking about the terminology explicitly like this is really helpful.
The text does use the term "subroutine". While it is internally consistent about this, it is not really consistent with other sources which nearly always refer to them as "methods" in the context of Java. It is not a big point, but students may be confused because they are not called subroutines in other resources they may consult.
The text is as modular as any other introductory programming text book I have seen. It wouldn't be possible to make sense of the example programs in later chapters without a solid mastery of the material in earlier chapters, but that's expected with this type of text. That said, the book does a good job of being as modular as it can. For instance, several of the later sections in a chapter can be skipped over, and, as I describe below, I re-arrange the material slightly to fit my course.
One caveat to this is that many of the examples in later chapters make use of the GUI programming from chapter six. When considering this text, you should be aware that its use of graphics is pretty fundamental and not something you could easily skip over. I see this as a positive as it adds some interest to the example programs which are graphical.
I like the organization of the book quite a lot. It does not use the "objects first" approach. Objects and classes are covered in chapter five, after the basic programming building blocks such as control structures and methods. I did find that some of the later sections within a chapter were things I didn't want to introduce to students at that point. For instance I skip the section on exceptions at the end of chapter three, and the sections on interfaces and nested classes at the end of chapter five.
I do think that the putting off arrays until chapter seven is a mistake, mostly because it is just hard to give good assignments that don't use arrays. When teaching with this book, I cover chapter seven after chapter four.
Overall though, the book does a fine job of transitioning from one topic to the next as it covers not only the particulars of the Java programming language, but the art of programming itself.
I did not find any interface issues at all. The text includes helpful PDF links in the table of contents, and also when the text makes a reference to another section. When discussing the example programs, the author also includes links to the full code on the book's web site, which is helpful. Figures are easy to read and high resolution. One suggestion I would have to improve the interface would be to include syntax highlighting for code listings.
The text is remarkably well edited for its length. I only noticed a handful of spelling or grammatical errors in the book.
I didn't notice any instances of the text being culturally insensitive. The text did not refer to people very often at all. In the few times it mentions the user or programmer, the author uses male and female pronouns equally. For instance at one point the text will mention a guess the number program telling the user "he lost", and later on saying a blackjack program should quit when the user wants to or when "she runs out of money".
I think this book is superior to other introductory programming texts that I have used, even without considering the fact that it is open and free. It is very detailed and provides clear expositions and great examples on everything that can be included in an introductory course (and then some). The few criticisms I have for the book can be easily worked around.
Table of Contents
- Chapter 1: Overview: The Mental Landscape
- Chapter 2: Programming in the Small I: Names and Things
- Chapter 3: Programming in the Small II: Control
- Chapter 4: Programming in the Large I: Subroutines
- Chapter 5: Programming in the Large II: Objects and Classes
- Chapter 6: Introduction to GUI Programming
- Chapter 7: Arrays and ArrayLists
- Chapter 8: Correctness, Robustness, Efficiency
- Chapter 9: Linked Data Structures and Recursion
- Chapter 10: Generic Programming and Collection Classes
- Chapter 11: Input/Output Streams, Files, and Networking
- Chapter 12: Threads and Multiprocessing
- Chapter 13: GUI Programming Continued
Ancillary Material
- David J. Eck
About the Book
Welcome to the Eighth Edition of Introduction to Programming Using Java, a free, on-line textbook on introductory programming, which uses Java as the language of instruction. This book is directed mainly towards beginning programmers, although it might also be useful for experienced programmers who want to learn something about Java. It is not meant to provide complete coverage of the Java language.
The eighth edition requires Java 8 or later, and it uses JavaFX for GUI programming. Version 8.1 is a small update of Version 8.0. This version briefly covers some of the new features in Java 11 and makes it clearer how to use this book with Java 11 and later.
About the Contributors
David J. Eck Ph.D. is a Professor at Department of Mathematics and Computer Science at the Hobart and William Smith Colleges.
Contribute to this Page
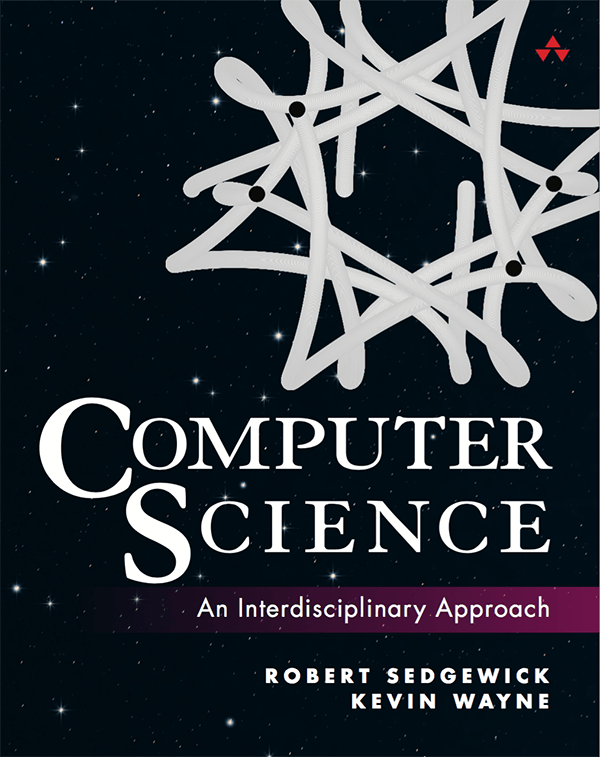
Programming in Java · Computer Science · An Interdisciplinary Approach
Online content. , introduction to programming in java., computer science., for teachers:, for students:.
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Java program for beginners.
Last Updated on May 9, 2023 by Prepbytes
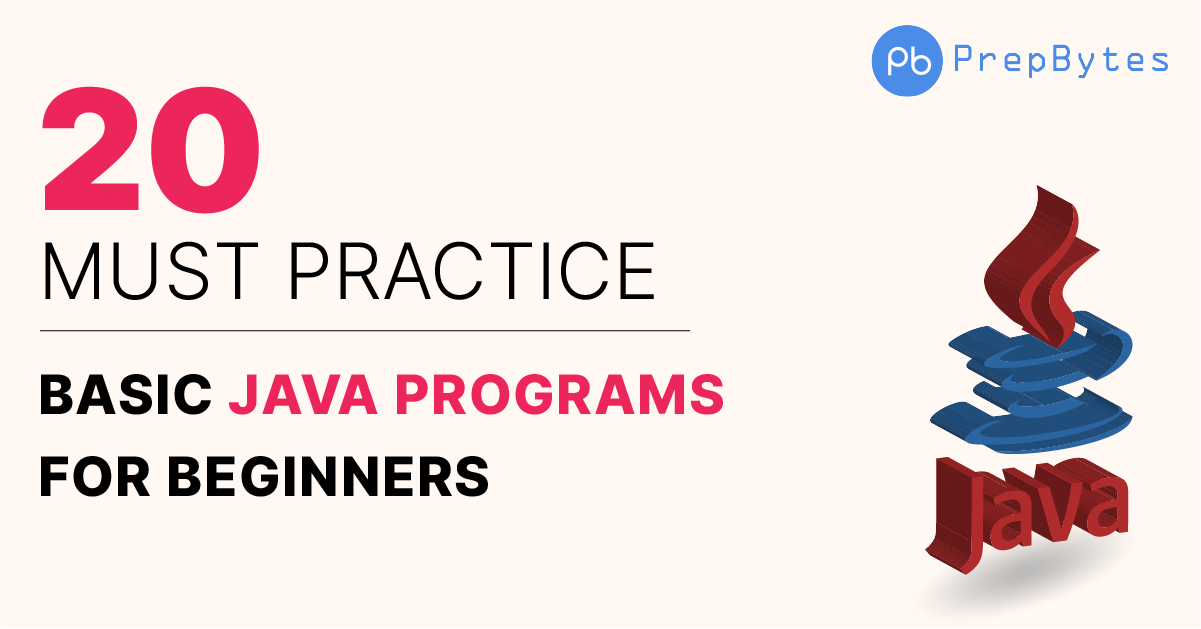
Java program examples are the most effective way to practice the Java programming language. This page contains basic Java practice program examples. It is suggested that you use the examples and try them on your own.
What is Java?
Java, a programming language and computing platform, was first introduced by Sun Microsystems in 1995. It has grown from humble beginnings to power a significant portion of today’s digital world by providing a solid foundation for the development of numerous services and applications. Java is still used in the development of cutting-edge goods and digital services for the future.
What is Java used for?
The following are some important Java applications:
- It is used in the development of Android applications.
- It enables you to create a wide range of mobile Java applications and enterprise software.
- Scientific Computing Applications
- Java Programming on Hardware Devices for Big Data Analytics
- Server-side technologies such as GlassFish, JBoss, and Apache make use of it.
We’ve talked about the Java programming language so far, and we hope you’ve gotten a good understanding of it. Now, let’s get back to our main topic: simple Java programs for beginners to practice, which will help you get a good understanding of the Java programming language.
Simple Java Programs for Beginners
Interviewers frequently ask about Java programs. oops, array, string, and control statements can all be used to access these programs. Questions about the Fibonacci series, prime numbers, factorial numbers, and palindrome numbers are frequently asked in interviews and tests. Most of the simple Java programs for beginners are provided below. Take a look at the list of Java programs and their logic and solutions.
Write a program to swap two numbers in Java. There are two ways to do this -with the third variable and without a third variable.
Write a program to print all the elements of Fibonacci series. A Fibonacci series is given by Fib(n)=Fib(n-1)+Fib(n-2)
Check if a given number is palindrome or not. A palindrome is a number which is when reversed give the same number. We find the reverse and check whether it is equal to the given number or not.
Write a program to find whether a number is an Armstrong number or not. Armstrong number – Sum of the cubes of its digit is equal to the number itself. E.g – 153
Find the GCD of two numbers in logn time. The symbol gcd (a, b) represents the greatest common divisor of two integers, a and b. To determine the gcd of the numbers, we must first list all of their factors and then identify the most common factor among them. As a result, we can say that of the three numbers, 4 has the most in common.
Write a program to find the sum of n natural numbers The formula for the product of n natural numbers is [n(n+1)]/2. Natural numbers are those that begin at 1 and go all the way to infinity. Except for the number 0, natural numbers only contain whole integers.
Write a program to find the lcm of two numbers. The lowest number that may be divided by both numbers is the LCM (Least Common Multiple) of two numbers. LCM of 15 and 20 is 60, for instance, while LCM of 5 and 7 is 35. Finding the union of all the elements contained in both numbers is a straightforward way to solve the problem.
Calculate the sum of digits of a given number. By adding a number’s digits without considering for place values, we may determine the amount of digits that make up the sum. Therefore, using the number 567 as a starting point, we may compute the digit total as 5 + 6 + 7 = 18.
Write a program to reverse a string. Reversing the characters in a string is possible using the built-in function reverse() of the StringBuilder or StringBuffer class. By using this technique, the characters are substituted in the opposite order. In Java, the static method with the necessary logic to reverse a string is called reverse.
Can you find the second highest number in given array? Running two loops is a simple way to find the second largest member of an array. The first loop will look for the largest member of the array. The second loop will then search the array for the largest member whose size is less than the first.
Find the first repeating element In the array. The HashSet data structure is the usual method for locating duplicate entries in an array. If you recall, duplicates are not permitted with the Set abstract data type. This attribute may be used to filter out duplicate items.
Write a program to find two elements in the array whose sum is near to zero. Given below is the solution where we use a two pointer approach.
What is Singleton and write a program to describe it. Ans. Singleton class in Java has only one instance in the whole program. We can easily implement a singleton class with thread safe property. We can see the implementation below –
Write a code to print all the first n prime numbers, where n will be given as input. The formal definition of a prime number "n" is one that can only be divided by 1 and n. In other words, a number is considered prime if it cannot be divided by any integer between 2 and n-1.
Can you write the Bubble Sort Algorithm? The idea is to bubble out the greater numbers to the right.
Implement the merge Sort algorithm. This is a Divide and conquer algorithm.
Write a program to get the sum of even and odd numbers in an array. Make an array of elements first. Next, define and initialize three variables with the values i evenSum, and oddSum to get the sum; Then, grab each member from the array individually using the "for loop" command.
Write a program to add two binary numbers. Begin adding from the right side, and when more than one is returned, store the carry for the next numbers.
Conclusion We discussed the must-practice simple Java programs for beginners in this article, as well as a brief introduction to Java. The Java programs for practice that are being discussed in this article are a must for a beginner.
Frequently Asked Questions (FAQs)
Q1. What is the basic program of Java? Ans. Fibonacci series, prime numbers, factorial numbers, and palindrome numbers are examples of Java basic programs that are frequently asked in interviews and exams.
Q2. What is Java, for example? Ans. Java is a programming language that focuses on objects. In Java, everything is associated with classes and objects, as well as their attributes and methods.
Q3. Is Java easy for beginners? Ans. It is also considered to be one of the easiest coding languages to learn. Because many of the processes of this high-level language are automated, you won’t need to do as much research into how everything works as you would with a low-level language.
Q4. What are the two types of Java? Ans. Java programming language types are classified into two sorts: basic types and reference types. The boolean and numeric types are the primitive types (4.2). The integral types are byte, short, int, long, and char, and the floating-point types are float and double.
Q5. What are 5 uses of Java? Ans. Here are a few of the most common tasks for which Java is best suited:
- Building and running mobile applications.
- Building and scaling cloud applications.
- Developing chatbots and other marketing tools.
- Powering enterprise-level web applications.
- Supporting artificial intelligence (AI) and internet of things (IoT) devices.
You May Also Like to Read
Calculator Program in Java Java Program to Add Two Numbers Java Program to Find the Factorial of a Number Java Program to Check Prime Number Menu Driven Program in Java Caesar Cipher Program in Java Anagram Program in Java Fizzbuzz Program in Java Package Program in Java Java Program to search an element in a Linked List Java Program to Reverse a Number Stack Program in Java Pyramid Program in Java Multiple Inheritance Program in Java Matrix Program in Java Series program in java Pattern Program in Java Program to convert ArrayList to LinkedList in Java Even Odd Program in Java Number Pattern Program in Java Binary Search Program in Java Leap Year Program in Java String Palindrome Program in Java Inheritance Program in Java Linear Search Program in Java Interface Program in Java JDBC Program in Java Method Overloading Program in Java Applet Program in Java Constructor Program in Java Recursion Program in Java For Loop Program In Java While Loop Program in Java If else Program in Java Java Program to Reverse a linked list Quick Sort Program in Java Hello World Program in Java Star Program in Java How to Run Java Program? String Programs in Java Multithreading Program in Java Thread Program in JAVA Switch Case Program in Java Collection Programs in Java Linked List Program in Java Exception Handling Program in Java
One thought on “ Java Program for Beginners ”
Nice and helful for begineers.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Java interview questions, concrete class in java, what is the difference between interface and concrete class in java, taking character input in java, difference between list and set in java, java 8 stream.
{{ activeMenu.name }}
- Python Courses
- JavaScript Courses
- Artificial Intelligence Courses
- Data Science Courses
- React Courses
- Ethical Hacking Courses
- View All Courses
Fresh Articles
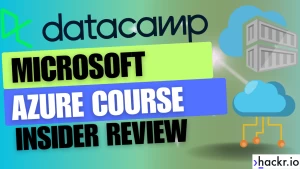
- Python Projects
- JavaScript Projects
- Java Projects
- HTML Projects
- C++ Projects
- PHP Projects
- View All Projects
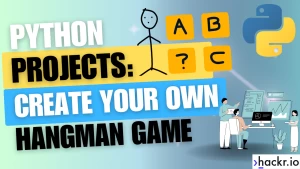
- Python Certifications
- JavaScript Certifications
- Linux Certifications
- Data Science Certifications
- Data Analytics Certifications
- Cybersecurity Certifications
- View All Certifications
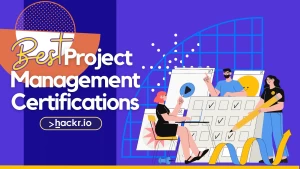
- IDEs & Editors
- Web Development
- Frameworks & Libraries
- View All Programming
- View All Development
- App Development
- Game Development
- Courses, Books, & Certifications
- Data Science
- Data Analytics
- Artificial Intelligence (AI)
- Machine Learning (ML)
- View All Data, Analysis, & AI
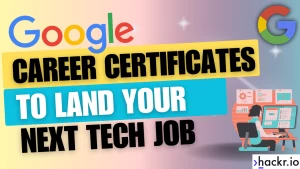
- Networking & Security
- Cloud, DevOps, & Systems
- Recommendations
- Crypto, Web3, & Blockchain
- User-Submitted Tutorials
- View All Blog Content
- JavaScript Online Compiler
- HTML & CSS Online Compiler
- Certifications
- Programming
- Development
- Data, Analysis, & AI
- Online JavaScript Compiler
- Online HTML Compiler
Don't have an account? Sign up
Forgot your password?
Already have an account? Login
Have you read our submission guidelines?
Go back to Sign In
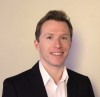
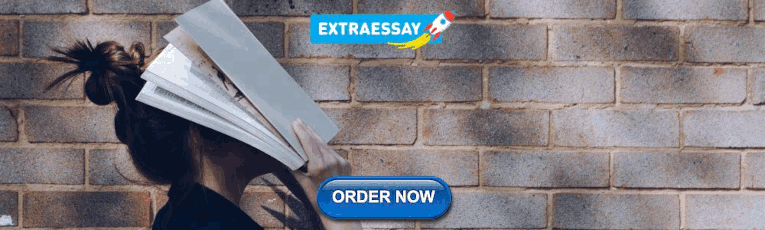
10 Best Java Projects for Beginners 2024 [With Source Code]
If I could go back in time to help my younger self learn Java, I'd tell him to build more Java projects!
That's exactly why I wrote this article: to share 10 Java projects to help beginners like you.
Whether you’re looking to start a career in Java development or enhance your portfolio, these Java projects are perfect for leveling up your Java skills.
I’ve also personally designed the first three Java projects to be step-by-step tutorials so you can follow along with me to get hands-on and code some cool stuff.
You can think of these tutorial projects as taking a free Java course while growing your Java portfolio!
I'm also regularly adding new Java projects with step-by-step tutorials, so make sure you bookmark this page and check back for the latest Java projects to grow your skills.
Without further ado, let’s dive in and start building with Java!
- 10 Best Java Projects for Beginners in 2024
1. Java Chat Application
What is this Java project?
In this Java project, you'll build a chat application, a dynamic and engaging tool that facilitates real-time communication between users
I've also designed this project to be a step-by-step tutorial so you can follow along with me to build something very cool and very practical.
This project also goes beyond merely creating a functional application; it serves as an excellent example of utilizing Java's networking capabilities and Swing framework to create interactive and responsive desktop applications.
It's a perfect addition to your portfolio, particularly if you're looking to demonstrate your proficiency in Java development, as it showcases essential programming concepts within a context that is both interactive and practically valuable.
So get ready and fire up your favorite Java IDE , and let's get building!
Java Skills Covered:
- Networking Logic: Develop the core logic for establishing client-server connections, including handling multiple client connections simultaneously.
- Dynamic UI Updates: Utilize the Swing framework to dynamically update the chat interface, reflecting messages and user actions in real-time, thus enhancing the overall user experience.
- Event Handling: Manage action and window events to capture user inputs such as sending a message or exiting the chat.
- User Interface Design: Apply principles of Swing GUI design to create a clean, user-friendly interface for the chat application, demonstrating skills in creating appealing desktop applications.
- Multithreading: Implement multithreading to handle concurrent tasks, such as listening for incoming messages while allowing the user to type new messages.
- Best Practices in Java: Write clean, efficient, and well-structured Java code, adhering to best practices for readability, maintainability, and performance.
Build This Java Project Here
2. Java Chess Game
In this project, you will create a fully interactive chess game, a classic strategy game that brings to life the timeless battle between two opposing forces on a checkered board.
I've also designed this Java project to be a step-by-step tutorial so you can follow along with me to build something fun, challenging, and very impressive!
This project also goes beyond creating a basic application; it's about mastering the intricacies of Java's Swing framework to create a visually appealing and user-interactive gaming experience.
It's also a perfect addition to your portfolio, particularly if you want to demonstrate your ability to handle complex logic and state management, problem-solving skills, and an understanding of GUI development.
So, warm up those coding fingers, and let’s get started with this exciting project!
- Game Logic Implementation: Craft the fundamental logic for chess, including piece movements, capturing mechanics, and game-ending conditions like checkmate.
- Graphical User Interface (GUI) Design: Utilize the Swing framework to design and implement a user-friendly and visually appealing interface for the chess game.
- Event-Driven Programming: Manage user interactions through event listeners, enabling players to move pieces, respond to game states, and interact with the game dynamically.
- Advanced State Management: Develop sophisticated game state management to handle the various states of a chess game, including tracking turns, game status, and special moves.
- Problem-Solving: Demonstrate advanced problem-solving abilities in implementing chess rules, strategizing piece movements, and validating legal moves.
- Best Practices in Java: Write clean, efficient, and well-structured Java code, following best practices for software design, maintainability, and GUI development.
3. Java Email Client
In this Java project, you're going to build an email client application, allowing users to interact with their emails through a desktop interface.
This is another Java project that I've designed to be a step-by-step tutorial, so you can follow along with me to build something practical, challenging, and quite cool! I'll also guide you on how to setup your email client to connect with Gmail.
This tutorial is not just about coding; it's about applying Java's powerful capabilities in networking and the Swing framework to craft applications that are both interactive and efficient.
So, stretch out those digits and get started with this exciting project!
- Email Protocols: Learn to use JavaMail API for handling SMTP and IMAP protocols, enabling the sending and receiving of emails.
- Dynamic UI Updates: Utilize the Swing framework to dynamically update the email client interface, reflecting changes in real-time and improving the user experience.
- Event Handling: Implement action listeners to capture user interactions like composing emails, adding attachments, and authenticating users.
- User Interface Design: Employ Swing GUI design to develop a clean, intuitive interface for the email client, showcasing your ability to craft appealing and functional desktop applications.
- Session Management: Handle email session management efficiently, ensuring secure and persistent connections to email servers.
- Best Practices in Java: Write clean, effective, and well-organized Java code, adhering to best practices for code readability, maintainability, and application performance.
4. Brick Breaker Game
This brick breaker game is one of many fun Java projects that has you trying to break bricks at the top of the screen. The player controls a tiny ball placed on a small platform at the bottom of the screen, which can be moved around from left to right using the arrow keys. The goal is to break the bricks without missing the ball with your platform. The project makes use of Java swing and OOPS concepts , among other things.
Source Code
5. Data Visualization Software
Data Visualization has become important as it displays data visually using statistical graphics and scientific visualization, to the point where data visualization software has been created. This project displays the node connectivity in networking in data visualization form. This node connectivity can be located at different locations via mouse or trackpad.
6. ATM Interface
This somewhat complex Java project consists of five different classes and is a console-based application. When the system starts the user is prompted with a user id and user pin. After entering the details successfully, the ATM functionalities are unlocked.
7. Web Server Management System
This web server management system project deals with the information, maintenance, and information management of the web server. It covers several concepts, including tracing the physical location of an entity, and identifying URL authorities and names.
8. Airline Reservation System
The project is a web-based one featuring open architecture that keeps up with the dynamic needs of the airline business by the addition of new systems & functionality. The project includes online transactions, fares, inventory, and e-ticket operations.
The software consists of four key modules, i.e., user registration, login, reservation, and cancellation. The app allows communication through a TCP/IP network protocol thereby facilitating the usage of internet & intranet communication globally.
9. Online Book Store
This project is mainly developed for bookstores and shops to digitize the book-purchasing process. The aim is to create an efficient and reliable online bookselling platform. It also records sold and stock books automatically in the database.
10. Snake Game in Java
If you are a ’90s kid or an adult you have probably played this game on your phone. The goal of this game is to make the snake eat the tokens without the snake being touched to the boundary on the screen. Every time the snake eats the token the score is updated. The player loses when the snake touches the boundary and the final score is displayed.
- How To Setup Your Java Environment
Before you start coding in Java, it's essential to have your coding environment properly set up and ready for action.
Being a compiled language that runs on a wide range of devices through the Java Virtual Machine (JVM), Java requires a bit more setup compared to interpreted languages like JavaScript that run directly in web browsers.
Here's how to set up a Java development environment on most operating systems.
Install a Java Development Kit (JDK)
First and foremost, you need the JDK, which includes the Java Runtime Environment (JRE) and the compilers and tools needed to compile and run Java applications.
- Download the JDK: Visit AdoptOpenJDK or Oracle's official Java site to download the JDK. I recommend choosing the LTS (Long-Term Support) version for stability.
- Install the JDK: Run the installer and follow the instructions. Make sure to set the JAVA_HOME environment variable to the JDK installation path and add the JDK's bin directory to your system's PATH . This is crucial for making Java commands accessible from your command line or terminal.
Verify Installation
After setting up, verify that everything is working correctly.
To begin, open a terminal or command prompt and run these commands to check the installed Java and Java compiler versions, respectively:
Then, try creating a simple Java program and compile it using:
Then run it with:
This should confirm your JDK is correctly set up.
Install a Java IDE or Code Editor
You'll need an IDE or code editor that supports Java syntax highlighting and potentially IntelliSense for code completion.
Eclipse, NetBeans, and IntelliJ IDEA are some of the most popular choices and three of my personal favorites.
That said, I'd also consider Visual Studio Code (VSCode) as this is a hugely popular choice among developers for various languages thanks to its extensive and lightweight feature set and vast library of extensions.
If you do go the VSCode route, head to the VSCode extension marketplace and install the ‘Extension Pack for Java’ from Microsoft, and you’ll be good to go.
Install Git [Optional but Recommended]
If you're really new to coding, you might want to skip this step, but even then, I'd really recommend becoming familiar with Git as soon as you can.
If you want the TL-DR, Git is a version control system that lets you track changes in your code and collaborate with others.
While this step is not strictly necessary for Java development, it's a best practice, especially for larger projects or when working in a team.
Simply download Git from the official Git website , and during installation, you can accept most default settings. That said, you might want to choose your preferred text editor and ensure that Git is added to your system's PATH.
- Wrapping Up
And there we have it! If you've taken the time to build these 10 Java projects, you should be feeling much more competent and confident with Java.
You'll also have a burgeoning Java portfolio that's packed full of interesting and practical Java projects, each demonstrating your dedication and abilities.
I also hope you enjoyed following along with my step-by-step tutorial on the first three Java projects!
My motivation with these Java tutorials is to guide you through the nuances of Java development while also giving you hands-on experience that you'd usually only get when taking a Java course.
Here at hackr.io , we're huge fans of project-based learning, so I hope these Java projects have bolstered your confidence and sparked a deeper interest in web development or any other form of Java development.
Remember, the journey doesn't end here!
With new projects and step-by-step tutorials regularly added to this page, be sure to check back often for new opportunities to refine your Java skills and expand your portfolio.
Happy coding!
Want to sharpen up your Java development skills in 2024? Check out:
Udemy's Top Rated Course: Java 17 Masterclass: Start Coding in 2024
People are also reading:
- Top Java Certifications
- Best Java Books
- Top Java Programming Interview Questions
- Core Java Cheatsheet
- Top Java Frameworks
- Best Way to Learn Java
- Constructor in java
- Prime Number Program in Java
- Difference between Java vs Javascript
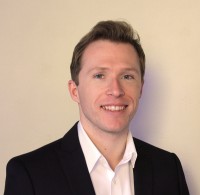
Technical Editor for Hackr.io | 15+ Years in Python, Java, SQL, C++, C#, JavaScript, Ruby, PHP, .NET, MATLAB, HTML & CSS, and more... 10+ Years in Networking, Cloud, APIs, Linux | 5+ Years in Data Science | 2x PhDs in Structural & Blast Engineering
Subscribe to our Newsletter for Articles, News, & Jobs.
Disclosure: Hackr.io is supported by its audience. When you purchase through links on our site, we may earn an affiliate commission.
In this article
- DataCamp Azure Fundamentals Course: Insider Review Azure Cloud Cloud Platforms Learning Platforms
- How To Create A Python Hangman Game With GUI for Beginners App Development Game Development Projects Python
- How To Create A Java Email Client App for Beginners App Development Java Projects
Please login to leave comments
Always be in the loop.
Get news once a week, and don't worry — no spam.
- Help center
- We ❤️ Feedback
- Advertise / Partner
- Write for us
- Privacy Policy
- Cookie Policy
- Change Privacy Settings
- Disclosure Policy
- Terms and Conditions
- Refund Policy
Disclosure: This page may contain affliate links, meaning when you click the links and make a purchase, we receive a commission.
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
- Java Tutorial
Overview of Java
- Introduction to Java
- The Complete History of Java Programming Language
- C++ vs Java vs Python
- How to Download and Install Java for 64 bit machine?
- Setting up the environment in Java
- How to Download and Install Eclipse on Windows?
- JDK in Java
- How JVM Works - JVM Architecture?
- Differences between JDK, JRE and JVM
- Just In Time Compiler
- Difference between JIT and JVM in Java
- Difference between Byte Code and Machine Code
- How is Java platform independent?
Basics of Java
- Java Basic Syntax
- Java Hello World Program
- Java Data Types
- Primitive data type vs. Object data type in Java with Examples
- Java Identifiers
Operators in Java
- Java Variables
- Scope of Variables In Java
Wrapper Classes in Java
Input/output in java.
- How to Take Input From User in Java?
- Scanner Class in Java
- Java.io.BufferedReader Class in Java
- Difference Between Scanner and BufferedReader Class in Java
- Ways to read input from console in Java
- System.out.println in Java
- Difference between print() and println() in Java
- Formatted Output in Java using printf()
- Fast I/O in Java in Competitive Programming
Flow Control in Java
- Decision Making in Java (if, if-else, switch, break, continue, jump)
- Java if statement with Examples
- Java if-else
- Java if-else-if ladder with Examples
- Loops in Java
- For Loop in Java
- Java while loop with Examples
- Java do-while loop with Examples
- For-each loop in Java
- Continue Statement in Java
- Break statement in Java
- Usage of Break keyword in Java
- return keyword in Java
- Java Arithmetic Operators with Examples
- Java Unary Operator with Examples
Java Assignment Operators with Examples
- Java Relational Operators with Examples
- Java Logical Operators with Examples
- Java Ternary Operator with Examples
- Bitwise Operators in Java
- Strings in Java
- String class in Java
- Java.lang.String class in Java | Set 2
- Why Java Strings are Immutable?
- StringBuffer class in Java
- StringBuilder Class in Java with Examples
- String vs StringBuilder vs StringBuffer in Java
- StringTokenizer Class in Java
- StringTokenizer Methods in Java with Examples | Set 2
- StringJoiner Class in Java
- Arrays in Java
- Arrays class in Java
- Multidimensional Arrays in Java
- Different Ways To Declare And Initialize 2-D Array in Java
- Jagged Array in Java
- Final Arrays in Java
- Reflection Array Class in Java
- util.Arrays vs reflect.Array in Java with Examples
OOPS in Java
- Object Oriented Programming (OOPs) Concept in Java
- Why Java is not a purely Object-Oriented Language?
- Classes and Objects in Java
- Naming Conventions in Java
- Java Methods
Access Modifiers in Java
- Java Constructors
- Four Main Object Oriented Programming Concepts of Java
Inheritance in Java
Abstraction in java, encapsulation in java, polymorphism in java, interfaces in java.
- 'this' reference in Java
- Inheritance and Constructors in Java
- Java and Multiple Inheritance
- Interfaces and Inheritance in Java
- Association, Composition and Aggregation in Java
- Comparison of Inheritance in C++ and Java
- abstract keyword in java
- Abstract Class in Java
- Difference between Abstract Class and Interface in Java
- Control Abstraction in Java with Examples
- Difference Between Data Hiding and Abstraction in Java
- Difference between Abstraction and Encapsulation in Java with Examples
- Difference between Inheritance and Polymorphism
- Dynamic Method Dispatch or Runtime Polymorphism in Java
- Difference between Compile-time and Run-time Polymorphism in Java
Constructors in Java
- Copy Constructor in Java
- Constructor Overloading in Java
- Constructor Chaining In Java with Examples
- Private Constructors and Singleton Classes in Java
Methods in Java
- Static methods vs Instance methods in Java
- Abstract Method in Java with Examples
- Overriding in Java
- Method Overloading in Java
- Difference Between Method Overloading and Method Overriding in Java
- Differences between Interface and Class in Java
- Functional Interfaces in Java
- Nested Interface in Java
- Marker interface in Java
- Comparator Interface in Java with Examples
- Need of Wrapper Classes in Java
- Different Ways to Create the Instances of Wrapper Classes in Java
- Character Class in Java
- Java.Lang.Byte class in Java
- Java.Lang.Short class in Java
- Java.lang.Integer class in Java
- Java.Lang.Long class in Java
- Java.Lang.Float class in Java
- Java.Lang.Double Class in Java
- Java.lang.Boolean Class in Java
- Autoboxing and Unboxing in Java
- Type conversion in Java with Examples
Keywords in Java
- Java Keywords
- Important Keywords in Java
- Super Keyword in Java
- final Keyword in Java
- static Keyword in Java
- enum in Java
- transient keyword in Java
- volatile Keyword in Java
- final, finally and finalize in Java
- Public vs Protected vs Package vs Private Access Modifier in Java
- Access and Non Access Modifiers in Java
Memory Allocation in Java
- Java Memory Management
- How are Java objects stored in memory?
- Stack vs Heap Memory Allocation
- How many types of memory areas are allocated by JVM?
- Garbage Collection in Java
- Types of JVM Garbage Collectors in Java with implementation details
- Memory leaks in Java
- Java Virtual Machine (JVM) Stack Area
Classes of Java
- Understanding Classes and Objects in Java
- Singleton Method Design Pattern in Java
- Object Class in Java
- Inner Class in Java
- Throwable Class in Java with Examples
Packages in Java
- Packages In Java
- How to Create a Package in Java?
- Java.util Package in Java
- Java.lang package in Java
- Java.io Package in Java
- Java Collection Tutorial
Exception Handling in Java
- Exceptions in Java
- Types of Exception in Java with Examples
- Checked vs Unchecked Exceptions in Java
- Java Try Catch Block
- Flow control in try catch finally in Java
- throw and throws in Java
- User-defined Custom Exception in Java
- Chained Exceptions in Java
- Null Pointer Exception In Java
- Exception Handling with Method Overriding in Java
- Multithreading in Java
- Lifecycle and States of a Thread in Java
- Java Thread Priority in Multithreading
- Main thread in Java
- Java.lang.Thread Class in Java
- Runnable interface in Java
- Naming a thread and fetching name of current thread in Java
- What does start() function do in multithreading in Java?
- Difference between Thread.start() and Thread.run() in Java
- Thread.sleep() Method in Java With Examples
- Synchronization in Java
- Importance of Thread Synchronization in Java
- Method and Block Synchronization in Java
- Lock framework vs Thread synchronization in Java
- Difference Between Atomic, Volatile and Synchronized in Java
- Deadlock in Java Multithreading
- Deadlock Prevention And Avoidance
- Difference Between Lock and Monitor in Java Concurrency
- Reentrant Lock in Java
File Handling in Java
- Java.io.File Class in Java
- Java Program to Create a New File
- Different ways of Reading a text file in Java
- Java Program to Write into a File
- Delete a File Using Java
- File Permissions in Java
- FileWriter Class in Java
- Java.io.FileDescriptor in Java
- Java.io.RandomAccessFile Class Method | Set 1
- Regular Expressions in Java
- Regex Tutorial - How to write Regular Expressions?
- Matcher pattern() method in Java with Examples
- Pattern pattern() method in Java with Examples
- Quantifiers in Java
- java.lang.Character class methods | Set 1
- Java IO : Input-output in Java with Examples
- Java.io.Reader class in Java
- Java.io.Writer Class in Java
- Java.io.FileInputStream Class in Java
- FileOutputStream in Java
- Java.io.BufferedOutputStream class in Java
- Java Networking
- TCP/IP Model
- User Datagram Protocol (UDP)
- Differences between IPv4 and IPv6
- Difference between Connection-oriented and Connection-less Services
- Socket Programming in Java
- java.net.ServerSocket Class in Java
- URL Class in Java with Examples
JDBC - Java Database Connectivity
- Introduction to JDBC (Java Database Connectivity)
- JDBC Drivers
- Establishing JDBC Connection in Java
- Types of Statements in JDBC
- JDBC Tutorial
- Java 8 Features - Complete Tutorial
Operators constitute the basic building block of any programming language. Java too provides many types of operators which can be used according to the need to perform various calculations and functions, be it logical, arithmetic, relational, etc. They are classified based on the functionality they provide.
Types of Operators:
- Arithmetic Operators
- Unary Operators
- Assignment Operator
- Relational Operators
- Logical Operators
- Ternary Operator
- Bitwise Operators
- Shift Operators
This article explains all that one needs to know regarding Assignment Operators.
Assignment Operators
These operators are used to assign values to a variable. The left side operand of the assignment operator is a variable, and the right side operand of the assignment operator is a value. The value on the right side must be of the same data type of the operand on the left side. Otherwise, the compiler will raise an error. This means that the assignment operators have right to left associativity, i.e., the value given on the right-hand side of the operator is assigned to the variable on the left. Therefore, the right-hand side value must be declared before using it or should be a constant. The general format of the assignment operator is,
Types of Assignment Operators in Java
The Assignment Operator is generally of two types. They are:
1. Simple Assignment Operator: The Simple Assignment Operator is used with the “=” sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
2. Compound Assignment Operator: The Compound Operator is used where +,-,*, and / is used along with the = operator.
Let’s look at each of the assignment operators and how they operate:
1. (=) operator:
This is the most straightforward assignment operator, which is used to assign the value on the right to the variable on the left. This is the basic definition of an assignment operator and how it functions.
Syntax:
Example:
2. (+=) operator:
This operator is a compound of ‘+’ and ‘=’ operators. It operates by adding the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
Note: The compound assignment operator in Java performs implicit type casting. Let’s consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5 Method 2: x += 4.5 As per the previous example, you might think both of them are equal. But in reality, Method 1 will throw a runtime error stating the “i ncompatible types: possible lossy conversion from double to int “, Method 2 will run without any error and prints 9 as output.
Reason for the Above Calculation
Method 1 will result in a runtime error stating “incompatible types: possible lossy conversion from double to int.” The reason is that the addition of an int and a double results in a double value. Assigning this double value back to the int variable x requires an explicit type casting because it may result in a loss of precision. Without the explicit cast, the compiler throws an error. Method 2 will run without any error and print the value 9 as output. The compound assignment operator += performs an implicit type conversion, also known as an automatic narrowing primitive conversion from double to int . It is equivalent to x = (int) (x + 4.5) , where the result of the addition is explicitly cast to an int . The fractional part of the double value is truncated, and the resulting int value is assigned back to x . It is advisable to use Method 2 ( x += 4.5 ) to avoid runtime errors and to obtain the desired output.
Same automatic narrowing primitive conversion is applicable for other compound assignment operators as well, including -= , *= , /= , and %= .
3. (-=) operator:
This operator is a compound of ‘-‘ and ‘=’ operators. It operates by subtracting the variable’s value on the right from the current value of the variable on the left and then assigning the result to the operand on the left.
4. (*=) operator:
This operator is a compound of ‘*’ and ‘=’ operators. It operates by multiplying the current value of the variable on the left to the value on the right and then assigning the result to the operand on the left.
5. (/=) operator:
This operator is a compound of ‘/’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the quotient to the operand on the left.
6. (%=) operator:
This operator is a compound of ‘%’ and ‘=’ operators. It operates by dividing the current value of the variable on the left by the value on the right and then assigning the remainder to the operand on the left.
Please Login to comment...
Similar reads.
- Java-Operators
- Google Introduces New AI-powered Vids App
- Dolly Chaiwala: The Microsoft Windows 12 Brand Ambassador
- 10 Best Free Remote Desktop apps for Android in 2024
- 10 Best Free Internet Speed Test apps for Android in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples.
Java is a popular programming language.
Java is used to develop mobile apps, web apps, desktop apps, games and much more.
Examples in Each Chapter
Our "Try it Yourself" editor makes it easy to learn Java. You can edit Java code and view the result in your browser.
Try it Yourself »
Click on the "Run example" button to see how it works.
We recommend reading this tutorial, in the sequence listed in the left menu.
Java is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
Java Exercises
Test yourself with exercises.
Insert the missing part of the code below to output "Hello World".
Start the Exercise
Advertisement
Test your Java skills with a quiz.
Start Java Quiz
Learn by Examples
Learn by examples! This tutorial supplements all explanations with clarifying examples.
See All Java Examples
My Learning
Track your progress with the free "My Learning" program here at W3Schools.
Log in to your account, and start earning points!
This is an optional feature. You can study at W3Schools without using My Learning.

Java Keywords
Java String Methods
Java Math Methods
Download Java
Download Java from the official Java web site: https://www.oracle.com
Java Exam - Get Your Diploma!
Kickstart your career.
Get certified by completing the course

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
Browse Course Material
Course info, instructors.
- Adam Marcus
Departments
- Electrical Engineering and Computer Science
As Taught In
- Programming Languages
- Software Design and Engineering
Learning Resource Types
Introduction to programming in java, assignments.

You are leaving MIT OpenCourseWare
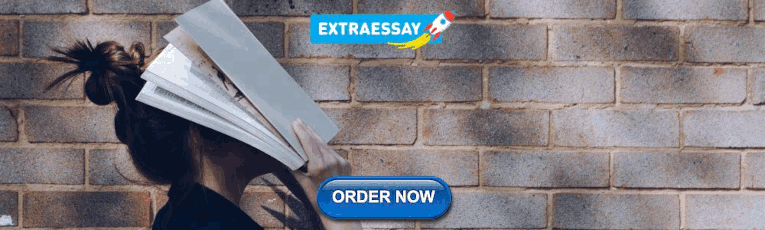
IMAGES
VIDEO
COMMENTS
Here you have the opportunity to practice the Java programming language concepts by solving the exercises starting from basic to more complex exercises. A sample solution is provided for each exercise. It is recommended to do these exercises by yourself first before checking the solution. Hope, these exercises help you to improve your Java ...
1. How to do Java projects for beginners? To do Java projects you need to know the fundamentals of Java programming. Then you need to select the desired Java project you want to work on. Plan and execute the code to finish the project. Some beginner-level Java projects include: Reversing a String; Number Guessing Game; Creating a Calculator
Write a Java program to compute the area of a polygon. Area of a polygon = (n*s^2)/ (4*tan (π/n)) where n is n-sided polygon and s is the length of a side Input Data: Input the number of sides on the polygon: 7 Input the length of one of the sides: 6 Expected Output. The area is: 130.82084798405722.
W3Schools offers a wide range of services and products for beginners and professionals, helping millions of people everyday to learn and master new skills. ... We have gathered a variety of Java exercises (with answers) for each Java Chapter. Try to solve an exercise by editing some code, or show the answer to see what you've done wrong.
Explore the Java coding exercises for practicing with commands below. First, read the conditions, scroll down to the Solution box, and type your solution. Then, click Verify (above the Conditions box) to check the correctness of your program. Exercise 1 Exercise 2 Exercise 3. Start task.
Simple Hello Word Program. Out of 500+ Simple & Basic Java Programs: Hello world is a first-ever program which we published on our site. Of course, Every Java programmer or C programmer will start with a "Hello World Program". Followed by the rest of the programs in different Categories. 1.
The course is designed to introduce the Java programming language to beginners. It covers the basics of Java, including syntax, data types, and operators. The course dives deeper into exception handling, file I/O, working with arrays, and object-oriented programming concepts. ... To access graded assignments and to earn a Certificate, you will ...
Get started with Java by learning about the basics of a Java program and variables! Get started with Java by learning about the basics of a Java program and variables! ... Beginner Friendly. 19 hours. Free course. Learn Java: Object-Oriented Programming Explore classes and objects in this introduction to object-oriented programming with Java.
Learn to code in Java — a robust programming language used to create software, web and mobile apps, and more. ... Beginner Friendly. 6 hours. Free course. Learn Java: Loops and Arrays Take your programming skills to the next level by learning about arrays and loops.
Java Tutorial for Beginners. This java tutorial would help you learn Java like a pro. I have shared 1000+ tutorials on various topics of Java, including core java and advanced Java concepts along with several Java programming examples to help you understand better. All the tutorials are provided in a easy to follow systematic manner.
10 Java code challenges to practice your new skills. 1. Word reversal. For this challenge, the input is a string of words, and the output should be the words in reverse but with the letters in the original order. For example, the string "Dog bites man" should output as "man bites Dog.".
You start by writing out the return type of the method. Since the age of a user is an integer, the return type of this method will be int. After the return type, you write the name of the method, followed by a pair of parenthesis. Then you write the method body within a pair of curly braces.
Welcome to the Eighth Edition of Introduction to Programming Using Java, a free, on-line textbook on introductory programming, which uses Java as the language of instruction. This book is directed mainly towards beginning programmers, although it might also be useful for experienced programmers who want to learn something about Java. It is not meant to provide complete coverage of the Java ...
Programming assignments. Creative programming assignments that we have used at Princeton. You can explore these resources via the sidebar at left. Introduction to Programming in Java. Our textbook Introduction to Programming in Java [ Amazon · Pearson · InformIT] is an interdisciplinary approach to the traditional CS1 curriculum with Java. We ...
Java Beginners Tutorial is probably the best Java learning platform for beginners as well as experienced programmers. It is divided into three sections. ... Along with the course content, it also provides your assignments according to the topics. For example, after completing variables in Java, you will get 5 assignments. Some key topics ...
Java Tutorial Summary. This Java tutorial for beginners is taught in a practical GOAL-oriented way. It is recommended you practice the code assignments given after each core Java tutorial to learn Java from scratch. This Java programming for beginners course will help you learn basics of Java and advanced concepts.
This course is an introduction to software engineering, using the Java™ programming language. It covers concepts useful to 6.005. Students will learn the fundamentals of Java. The focus is on developing high quality, working software that solves real problems. The course is designed for students with some programming experience, but if you have none and are motivated you will do fine.
Most of the simple Java programs for beginners are provided below. Take a look at the list of Java programs and their logic and solutions. Write a program to swap two numbers in Java. There are two ways to do this -with the third variable and without a third variable. public static void swapNumberswithtemp(int a, int b) { //using 3rd variable.
How Edabit Works. This is an introduction to how challenges on Edabit work. In the Code tab above you'll see a starter function that looks like this: public static boolean returnTrue () { } All you have to do is type return true; between the curly braces { } and then click the Check button. If you did this correctly, the button will turn re ...
Best Practices in Java: Write clean, effective, and well-organized Java code, adhering to best practices for code readability, maintainability, and application performance. Build This Java Project Here. 4. Brick Breaker Game. This brick breaker game is one of many fun Java projects that has you trying to break bricks at the top of the screen.
variable operator value; Types of Assignment Operators in Java. The Assignment Operator is generally of two types. They are: 1. Simple Assignment Operator: The Simple Assignment Operator is used with the "=" sign where the left side consists of the operand and the right side consists of a value. The value of the right side must be of the same data type that has been defined on the left side.
Example Get your own Java Server. Click on the "Run example" button to see how it works. We recommend reading this tutorial, in the sequence listed in the left menu. Java is an object oriented language and some concepts may be new. Take breaks when needed, and go over the examples as many times as needed.
This section provides the assignments for the course, supporting files, and a special set of assignment files that can be annotated. Browse Course Material ... GravityCalculator.java 2 FooCorporation 3 Marathon Marathon.java 4 Library Book.java . Library.java . 5 Graphics! initial.png . SimpleDraw.java ...