The blank identifier in Golang
Sometimes, a programming language needs to ignore some values for multiple reasons. Go has an identifier for this. If any variable is declared but not used or any import has the same issue, the Go compiler throws an error. Golang blank identifier helps prevent that.
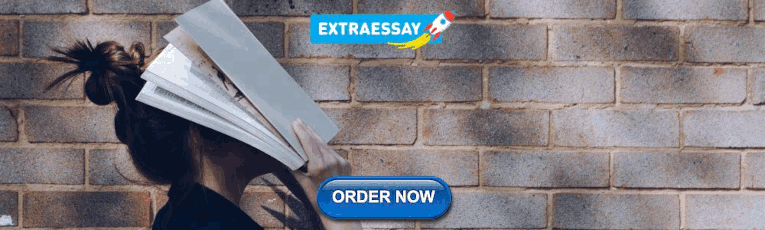
What is the blank identifier?
The blank identifier is the single underscore (_) operator. It is used to ignore the values returned by functions or import for side-effects.
Why is it needed?
Go compiler throws an error whenever it encounters a variable declared but not used. Now we can simply use the blank identifier and not declare any variable at all.
Uses of blank identifier
The blank identifier can ignore any value. The main use cases for this identifier is to ignore some of the values returned by a function or for import side-effects.
1. Ignore values
The blank identifier ignores any value returned by a function. It can be used any number of times in a Go program. The code below shows how to use the blank identifier.
2. Side effects of import
Sometimes, a package in Go needs to be imported solely for side effects i.e. initialization. The blank identifier if used before does that. It allows us to import the package without using anything from it. It also stops the compiler from throwing error messages like “ unused import “.
import _ "fmt"
3. Ignore Compiler Errors
The blank identifier can be used as a placeholder where the variables are going to be ignored for some purpose. Later those variables can be added by replacing the operator. In many cases, it helps to debug code.
The Blank Identifier in Go: A Swiss Army Knife for the Discerning Developer
Go is a programming language that is known for its simplicity, efficiency, and ease of use. One of the most interesting features of Go is the blank identifier, also known as the underscore character ( _ ). In this article, we will explore how the blank identifier can be used in multiple assignment, unused imports and variables, import for side effect, and interface checks.
Multiple Assignment
In Go, we can use the blank identifier to ignore values returned by functions during multiple assignment. For example:
In this example, the second value returned by foo() is ignored by using the blank identifier. This is a common idiom in Go, where functions can return multiple values, but sometimes only some of those values are needed.
Unused Imports and Variables
Go is a statically typed language, which means that variables must be declared before they are used. However, sometimes we may need to import a package or declare a variable, but not actually use it in our code. In such cases, we can use the blank identifier to indicate that the value is not needed.
For example, consider the following code:
In this example, we import the fmt package for printing messages, but we also import the net/http/pprof package for profiling purposes. However, we don’t actually use the net/http/pprof package in our code. To indicate that we don’t need the package, we use the blank identifier in the import statement.
Similarly, we can use the blank identifier to declare variables that are not used. For example:
In this example, the second value returned by foo() is not needed, so we use the blank identifier to indicate that it should be discarded.
Import for Side Effect
In Go, packages can have init functions that are called automatically when the package is imported. Sometimes we may need to import a package only for its init function, and not actually use any of its exported functions or variables. In such cases, we can use the blank identifier to import the package for its side effects.
In this example, we import the github.com/mattn/go-sqlite3 package for its init function, which registers the SQLite driver with the database/sql package. We don’t actually use any of the exported functions or variables from the package, so we use the blank identifier to indicate that they are not needed.
Interface Checks
In Go, we can use the blank identifier to perform interface checks. For example:
In this example, we use the blank identifier to ignore the first value returned by the type assertion a.(MyInterface) . The second value is a boolean that indicates whether the assertion succeeded or not. By ignoring the first value, we can perform the interface check without having to store the result in a variable.
The blank identifier in Go is a versatile and useful feature that can help us write more expressive and efficient code. By using the blank identifier judiciously and with care, we can make our code more readable and maintainable, while also taking advantage of the unique features of the Go language.
Go » Blank Identifiers
In above example, you have nothing to do with result and you don’t use it. The compiler won’t allow this by saying
result declared but not used
In this case, you can replace result with an underscore or a blank identifier as shown below
Even if you declare a variable , you can ignore it with a blank identifier later as in below code.
imported and not used “<package name>”
Blank identifier can be used to resolve this compiler error. There are two ways to use blank identifiers to resolve unused package error as shown below.
A. Declare a global blank identifier(before main() function) that access a symbol from the unused package such as Open in below code.
B. Prefix the unused import with a blank identifier as below.
Blank identifiers make the code more readable by avoiding unused variable declarations through out the code.
What is _ (underscore) in Go?
Underscore _ explained in Go.
Underscore _ demisified in Go.
Blank identifier
The blank identifier is represented by the underscore character _. It serves as an anonymous placeholder instead of a regular (non-blank) identifier and has special meaning in declarations, as an operand, and in assignment statements.
Official Docs:
- The Go Programming Language Specification
- Effective Go
Why we use it?
As you know, in Golang, every variable should be used in its declaration scope, But what if you have a variable that is not used, and also irrelevant to you? The compiler will throw an exception, so to Example Ignoring array index
Its also common if you use a function that returns more than one value, and you want to ignore one of the values (commonly to test things out you ignore the error) Underscore will be your friend, and if you want more details.
The blank identifier may be used whenever syntax requires a variable name but program logic does not, for instance to discard an unwanted loop index when we require only the element value.
- Copywrite The Go Programming Language (Addison-Wesley Professional Computing Series)
Common use case
If you see an import statement with underscore, Jon Explains it here Why we import SQL drivers as the blank identifier
- Datadog Site
- Serverless for AWS Lambda
- Autodiscovery
- Datadog Operator
- Assigning Tags
- Unified Service Tagging
- Service Catalog
- Session Replay
- Continuous Testing
- Browser Tests
- Private Locations
- Incident Management
- Database Monitoring
- Cloud Security Management
- Software Composition Analysis
- Workflow Automation
- CI Visibility
- Test Visibility
- Intelligent Test Runner
- Learning Center
- Standard Attributes
- Amazon Linux
- Oracle Linux
- Rocky Linux
- From Source
- Architecture
- Supported Platforms
- Advanced Configurations
- Configuration Files
- Status Page
- Network Traffic
- Proxy Configuration
- FIPS Compliance
- Dual Shipping
- Secrets Management
- Remote Configuration
- Fleet Automation
- Upgrade to Agent v7
- Upgrade to Agent v6
- Upgrade Between Agent Minor Versions
- Container Hostname Detection
- Agent Flare
- Agent Check Status
- Permission Issues
- Integrations Issues
- Site Issues
- Autodiscovery Issues
- Windows Container Issues
- Agent Runtime Configuration
- High CPU or Memory Consumption
- Data Security
- Getting Started
- OTLP Metrics Types
- Configuration
- Integrations
- Resource Attribute Mapping
- Metrics Mapping
- Infrastructure Host Mapping
- Hostname Mapping
- Service-entry Spans Mapping
- Ingestion Sampling
- OTLP Ingestion by the Agent
- W3C Trace Context Propagation
- OpenTelemetry API Support
- Correlate RUM and Traces
- Correlate Logs and Traces
- Troubleshooting
- Visualizing OTLP Histograms as Heatmaps
- Migrate to OpenTelemetry Collector version 0.95.0+
- Producing Delta Temporality Metrics
- Sending Data from OpenTelemetry Demo
- OAuth2 in Datadog
- Authorization Endpoints
- Datagram Format
- Unix Domain Socket
- High Throughput Data
- Data Aggregation
- DogStatsD Mapper
- Writing a Custom Agent Check
- Writing a Custom OpenMetrics Check
- Create an Agent-based Integration
- Create an API Integration
- Create a Log Pipeline
- Integration Assets Reference
- Build a Marketplace Offering
- Create a Tile
- Create an Integration Dashboard
- Create a Recommended Monitor
- Create a Cloud SIEM Detection Rule
- OAuth for Integrations
- Install Agent Integration Developer Tool
- UI Extensions
- Submission - Agent Check
- Submission - DogStatsD
- Submission - API
- JetBrains IDEs
- Visual Studio
- Account Management
- Components: Common
- Components: Azure
- Components: AWS
- AWS Accounts
- Azure Accounts
- Dashboard List
- Interpolation
- Correlations
- Scheduled Reports
- Template Variables
- Configure Monitors
- Recommended Monitors
- Audit Trail
- Error Tracking
- Integration
- Live Process
- Network Performance
- Process Check
- Real User Monitoring
- Service Check
- Search Monitors
- Monitor Status
- Check Summary
- Monitor Settings
- Host and Container Maps
- Infrastructure List
- Container Images View
- Orchestrator Explorer
- Kubernetes Resource Utilization
- Increase Process Retention
- Cloud Resources Schema
- Metric Type Modifiers
- Historical Metrics Ingestion
- Submission - Powershell
- OTLP Metric Types
- Metrics Types
- Distributions
- Metrics Units
- Advanced Filtering
- Metrics Without Limits™
- Impact Analysis
- Faulty Deployment Detection
- Managing Incidents
- Natural Language Querying
- Navigate the Service Catalog
- Investigate a Service
- Create Entries
- Import Entries from Datadog
- Import Entries from Integrations
- Manage Entries
- Service Definitions
- Service Scorecards
- Troubleshooting and Best Practices
- Exploring APIs
- Assigning Owners
- Monitoring APIs
- Adding Entries to API Catalog
- Adding Metadata to APIs
- API Catalog API
- Endpoint Discovery from APM
- Issue States
- Default Grouping
- Custom Grouping
- Identify Suspect Commits
- Monitor-based SLOs
- Metric-based SLOs
- Time Slice SLOs
- Error Budget Alerts
- Burn Rate Alerts
- Incident Details
- Incident Settings
- Incident Analytics
- Datadog Clipboard
- Ingest Events
- Arithmetic Processor
- Date Remapper
- Category Processor
- Grok Parser
- Lookup Processor
- Service Remapper
- Status Remapper
- String Builder Processor
- Navigate the Explorer
- Customization
- Notifications
- Saved Views
- Triaging & Notifying
- Create a Case
- View and Manage
- Enterprise Configuration
- Build Workflows
- Authentication
- Trigger Workflows
- Workflow Logic
- Data Transformation
- HTTP Requests
- Save and Reuse Actions
- Connections
- Actions Catalog
- Embedded Apps
- Log collection
- Tag extraction
- Data Collected
- Installation
- Further Configuration
- Integrations & Autodiscovery
- Prometheus & OpenMetrics
- Control plane monitoring
- Data collected
- Data security
- Commands & Options
- Cluster Checks
- Endpoint Checks
- Admission Controller
- AWS Fargate
- Duplicate hosts
- Cluster Agent
- HPA and Metrics Provider
- Lambda Metrics
- Distributed Tracing
- Log Collection
- Advanced Configuration
- Continuous Profiler
- Securing Functions
- Deployment Tracking
- Libraries & Integrations
- Enhanced Metrics
- Linux - Code
- Linux - Container
- Windows - Code
- Azure Container Apps
- Google Cloud Run
- Overview Page
- Network Analytics
- Network Map
- DNS Monitoring
- SNMP Metrics
- NetFlow Monitoring
- Network Device Topology Map
- Google Cloud
- Custom Costs
- SaaS Cost Integrations
- Tag Pipelines
- Container Cost Allocation
- Cost Recommendations
- APM Terms and Concepts
- Automatic Instrumentation
- Custom Instrumentation
- Library Compatibility
- Library Configuration
- Configuration at Runtime
- Trace Context Propagation
- Serverless Application Tracing
- Proxy Tracing
- Span Tag Semantics
- Trace Metrics
- Runtime Metrics
- Ingestion Mechanisms
- Ingestion Controls
- Generate Metrics
- Trace Retention
- Usage Metrics
- Correlate DBM and Traces
- Correlate Synthetics and Traces
- Correlate Profiles and Traces
- Search Spans
- Query Syntax
- Span Facets
- Span Visualizations
- Trace Queries
- Request Flow Map
- Service Page
- Resource Page
- Service Map
- APM Monitors
- Expression Language
- Error Tracking Explorer
- Exception Replay
- Tracer Startup Logs
- Tracer Debug Logs
- Connection Errors
- Agent Rate Limits
- Agent APM metrics
- Agent Resource Usage
- Correlated Logs
- PHP 5 Deep Call Stacks
- .NET diagnostic tool
- APM Quantization
- Supported Language and Tracer Versions
- Profile Types
- Profile Visualizations
- Investigate Slow Traces or Endpoints
- Compare Profiles
- Setup Architectures
- Self-hosted
- Google Cloud SQL
- Autonomous Database
- Connecting DBM and Traces
- Exploring Database Hosts
- Exploring Query Metrics
- Exploring Query Samples
- Data Jobs Monitoring
- Monitoring Page Performance
- Monitoring Resource Performance
- Collecting Browser Errors
- Tracking User Actions
- Frustration Signals
- Crash Reporting
- Mobile Vitals
- Web View Tracking
- Integrated Libraries
- Sankey Diagrams
- Funnel Analysis
- User Retention
- Generate Custom Metrics
- Connect RUM and Traces
- Search RUM Events
- Search Syntax
- Watchdog Insights for RUM
- Feature Flag Tracking
- Track Browser Errors
- Track Mobile Errors
- Multistep API Tests
- Recording Steps
- Test Results
- Advanced Options for Steps
- Authentication in Browser Tests
- Dimensioning
- Search Test Batches
- Search Test Runs
- Test Coverage
- Browser Test
- Test Summary
- APM Integration
- Testing Multiple Environments
- Testing With Proxy, Firewall, or VPN
- Azure DevOps Extension
- CircleCI Orb
- GitHub Actions
- Results Explorer
- AWS CodePipeline
- Custom Commands
- Custom Tags and Measures
- Custom Pipelines API
- Search and Manage
- Search Test Runs or Pipeline Executions
- CI Providers
- Java and JVM Languages
- JavaScript and TypeScript
- JUnit Report Uploads
- Tests in Containers
- Developer Workflows
- Code Coverage
- Instrument Browser Tests with RUM
- Instrument Swift Tests with RUM
- Early Flake Detection
- How It Works
- CircleCI Orbs
- Generic CI Providers
- Static Analysis Rules
- GitHub Pull Requests
- Deployment events
- Incident events
- Suppressions
- Security Inbox
- Threat Intelligence
- Account Takeover Protection
- Log Detection Rules
- Signal Correlation Rules
- Security Signals
- Investigator
- CSM Enterprise
- CSM Cloud Workload Security
- CSM Agentless Scanning
- Detection Rules
- Investigate Security Signals
- Creating Custom Agent Rules
- CWS Events Formats
- Manage Compliance Rules
- Create Custom Rules
- Manage Compliance Posture
- Explore Misconfigurations
- Signals Explorer
- Identity Risks
- Vulnerabilities
- Agentless Scanning
- Mute Issues
- Automate Security Workflows
- Create Jira Issues
- Severity Scoring
- Terms and Concepts
- Using Single Step Instrumentation
- Using Datadog Tracing libraries
- Enabling ASM for Serverless
- Code Security
- User Monitoring and Protection
- Custom Detection Rules
- In-App WAF Rules
- Trace Qualification
- Attack Summary
- Attacker Explorer
- API Security Inventory
- Splunk HTTP Event Collector
- Splunk Forwarders (TCP)
- Sumo Logic Hosted Collector
- Datadog Agent
- Update Existing Pipelines
- Best Practices for Scaling Observability Pipelines
- React Native
- OpenTelemetry
- Other Integrations
- Pipeline Scanner
- Attributes and Aliasing
- Rehydrate from Archives
- PCI Compliance
- Connect Logs and Traces
- Search Logs
- Advanced Search
- Transactions
- Log Side Panel
- Watchdog Insights for Logs
- Track Browser and Mobile Errors
- Track Backend Errors
- Manage Data Collection
- Switching Between Orgs
- User Management
- Login Methods
- Custom Organization Landing Page
- Service Accounts
- IP Allowlist
- Granular Access
- Permissions
- User Group Mapping
- Active Directory
- API and Application Keys
- Team Management
- Multi-Factor Authentication
- Cost Details
- Usage Details
- Product Allotments
- Multi-org Accounts
- Log Management
- Synthetic Monitoring
- HIPAA Compliance
- Library Rules
- Investigate Sensitive Data Issues
- Regular Expression Syntax
Remove unnecessary blank identifiers
ID: go-best-practices/unnecessary-blank-identifier
Language: Go
Severity: Info
Category: Best Practices
Description
In Go, when using range iterations or receiving values from channels, it is recommended to avoid assigning the iteration or received value to the blank identifier _ . Instead, it is preferred to omit the assignment entirely.
Here’s why it is best to use for range s {} , x = someMap[key] , and <-ch instead of using the blank identifier _ :
- Clarity and Readability: By omitting the assignment entirely, it makes the code more readable and self-explanatory. Using _ can introduce confusion and make it less clear what the purpose of the assignment is or if the value is discarded intentionally or accidentally.
- Avoiding Variable Pollution: Using _ as an assignment can unnecessarily pollute the variable space. Although Go allows the use of the blank identifier _ to disregard a value, it is a good practice to avoid introducing unnecessary variables, especially if they are never used.
- Linting and static analysis: Some linting tools and static analyzers may flag the use of varName = _ as an indication of accidental assignment or failure to handle errors or returned values properly. Removing these assignments eliminates such warnings or false-positive detections.
For example, consider the following code snippets:
Both snippets achieve the same result, but the second one that omits the assignments using _ is preferred for its simplicity, readability, and adherence to Go’s best practices.
By using for range s {} , x = someMap[key] , and <-ch instead of assigning to _ , you can write cleaner and more readable Go code while avoiding unnecessary variable assignments and potential confusion.
Non-Compliant Code Examples
Compliant code examples.
Seamless integrations. Try Datadog Code Analysis
Try this rule and analyze your code with Datadog Code Analysis
How to use this rule.
- Create a static-analysis.datadog.yml with the content above at the root of your repository
- Use our free IDE Plugins or add Code Analysis scans to your CI pipelines
- Get feedback on your code
For more information, please read the Code Analysis documentation
VS Code Extension
Identify code vulnerabilities directly in your VS Code editor
JetBrains Plugin
Identify code vulnerabilities directly in JetBrains products
Use Datadog Code Analysis to catch code issues at every step of your development process
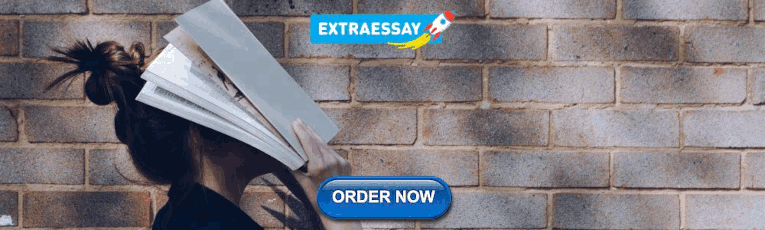
Request a personalized demo
Get started with datadog.
- Data Types in Go
- Go Keywords
- Go Control Flow
- Go Functions
- GoLang Structures
- GoLang Arrays
- GoLang Strings
- GoLang Pointers
- GoLang Interface
- GoLang Concurrency
- Go Programming Language (Introduction)
- How to Install Go on Windows?
- How to Install Golang on MacOS?
- Hello World in Golang
Fundamentals
- Identifiers in Go Language
- Go Variables
- Constants- Go Language
- Go Operators
Control Statements
- Go Decision Making (if, if-else, Nested-if, if-else-if)
- Loops in Go Language
- Switch Statement in Go
Functions & Methods
- Functions in Go Language
- Variadic Functions in Go
- Anonymous function in Go Language
- main and init function in Golang
What is Blank Identifier(underscore) in Golang?
- Defer Keyword in Golang
- Methods in Golang
- Structures in Golang
- Nested Structure in Golang
- Anonymous Structure and Field in Golang
- Arrays in Go
- How to Copy an Array into Another Array in Golang?
- How to pass an Array to a Function in Golang?
- Slices in Golang
- Slice Composite Literal in Go
- How to sort a slice of ints in Golang?
- How to trim a slice of bytes in Golang?
- How to split a slice of bytes in Golang?
- Strings in Golang
- How to Trim a String in Golang?
- How to Split a String in Golang?
- Different ways to compare Strings in Golang
- Pointers in Golang
- Passing Pointers to a Function in Go
- Pointer to a Struct in Golang
- Go Pointer to Pointer (Double Pointer)
- Comparing Pointers in Golang
Concurrency
- Goroutines - Concurrency in Golang
- Select Statement in Go Language
- Multiple Goroutines
- Channel in Golang
- Unidirectional Channel in Golang
_ (underscore) in Golang is known as the Blank Identifier. Identifiers are the user-defined name of the program components used for the identification purpose. Golang has a special feature to define and use the unused variable using Blank Identifier. Unused variables are those variables that are defined by the user throughout the program but he/she never makes use of these variables. These variables make the program almost unreadable. As you know, Golang is a more concise and readable programming language so it doesn’t allow the programmer to define an unused variable if you do such, then the compiler will throw an error.
The real use of Blank Identifier comes when a function returns multiple values, but we need only a few values and want to discard some values. Basically, it tells the compiler that this variable is not needed and ignored it without any error. It hides the variable’s values and makes the program readable. So whenever you will assign a value to Blank Identifier it becomes unusable.
Example 1: In the below program, the function mul_div is returning two values and we are storing both the values in mul and div identifier. But in the whole program, we are using only one variable i.e. mul . So compiler will throw an error div declared and not used
Output:
Example 2: Let’s make use of the Blank identifier to correct the above program. In place of div identifier just use the _ (underscore). It allows the compiler to ignore declared and not used error for that particular variable.
Important Points:
- You can use multiple Blank Identifiers in the same program. So you can say a Golang program can have multiple variables using the same identifier name which is the blank identifier.
- There are many cases that arise the requirement of assignment of values just to complete the syntax even knowing that the values will not be going to be used in the program anywhere. Like a function returning multiple values. Mostly blank identifier is used in such cases.
- You can use any value of any type with the Blank Identifier.
Please Login to comment...
Similar reads.
- Go Language
Improve your Coding Skills with Practice
What kind of Experience do you want to share?

- golangci-lint工具
如何安装:golangci-lint 使用文档 go linter如何与GoLand联动
- 安装go linter plugin
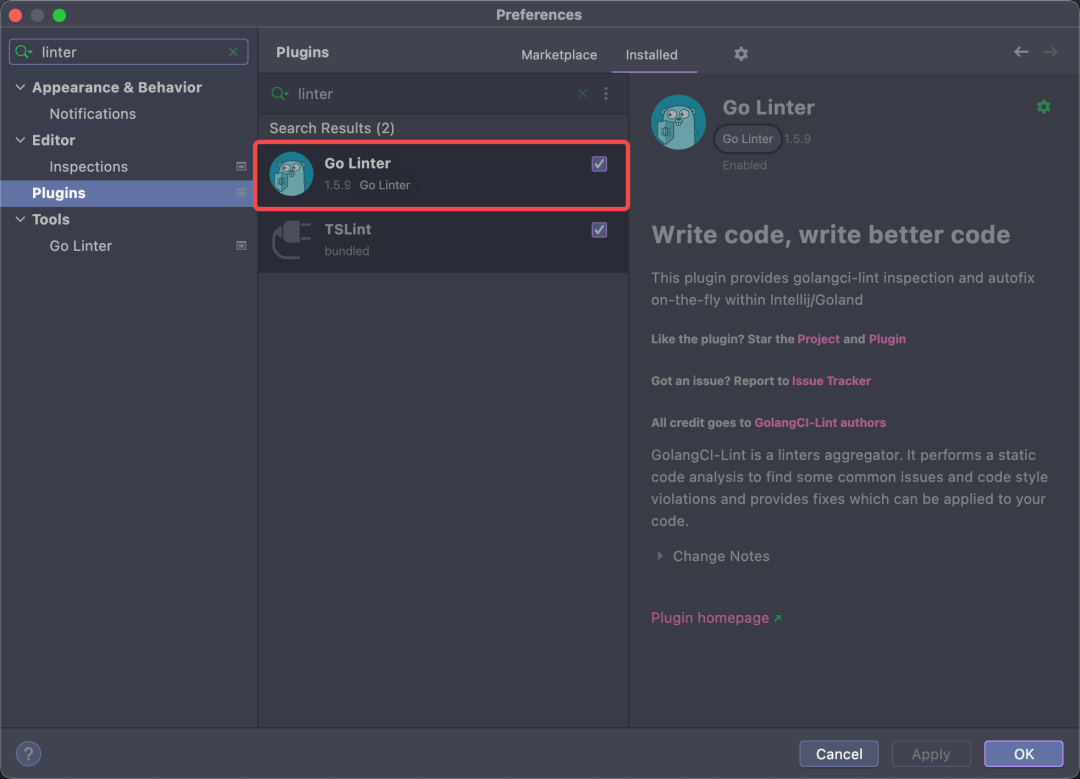
- 将go linter的执行文件的path指定为上面下载的golangci-lint工具
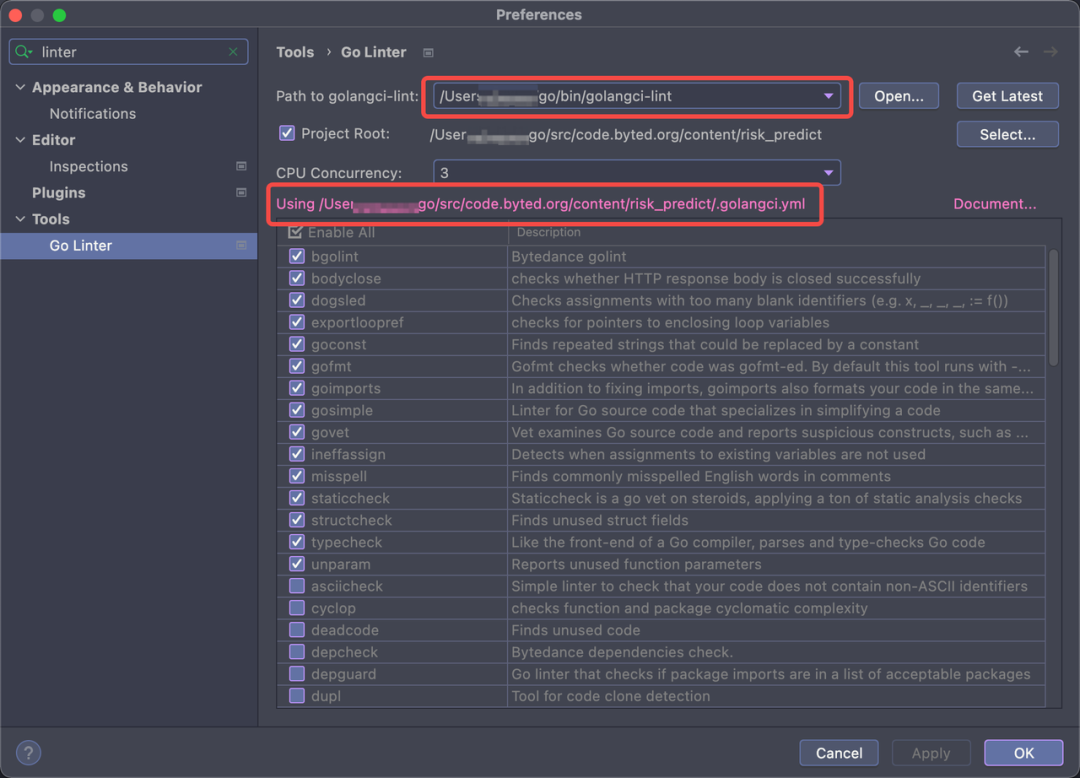
- 这样,就可以在IDE里看到lint问题
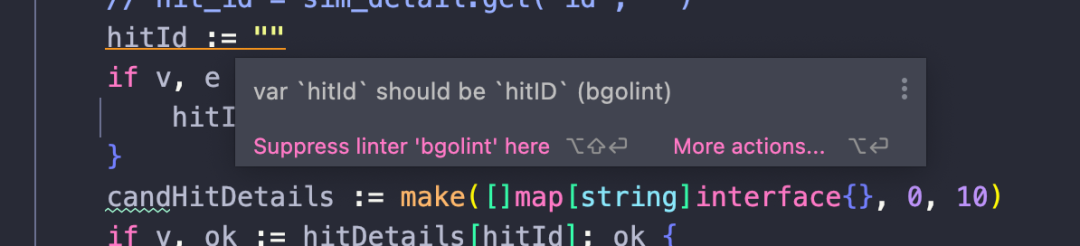
注意 :lint检测应该建立在项目build成功的基础上 不然,在codebase CI的检测结果中,可能会出现一些意料之外的错误信息。一般情况下,因lint问题导致检测 GolngCI-lint检测失败的Exit Code为1。
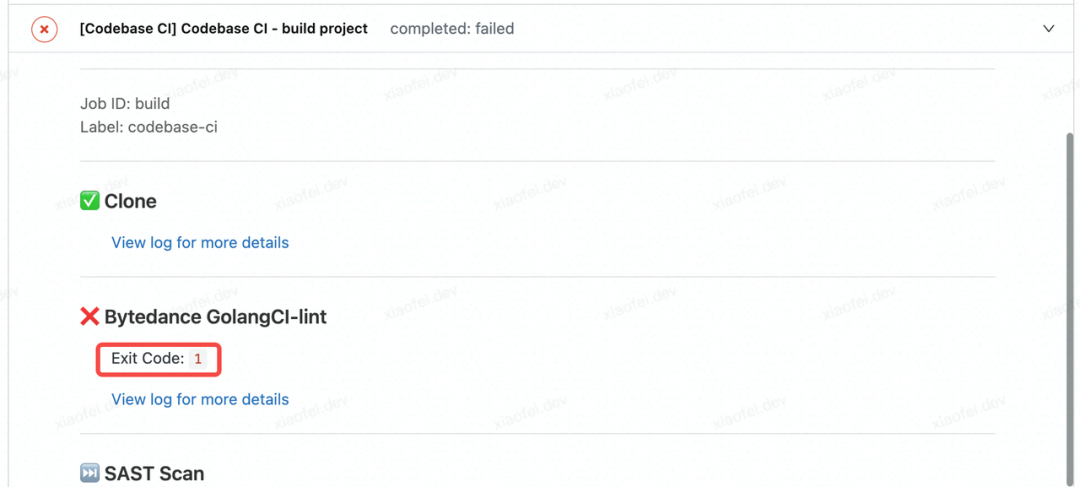
全部使用小写字母。若必须是多个单词组合,则直接拼接在一起,例如videopredict,而非video_predict。
使用ID,而非Id;使用RPC,而非Rpc。
常量的注释,记得带上变量名的前缀,这会提醒你只添加该常量相关的注释,或者,它会提醒你不要添加“非必要”的注释。如果要做某些常量的注释,那么在定义常量集合的时候,请这样写:
camel格式。不要全部大写
缩进格式化 File is not gofmt -ed with -s (gofmt) 执行下面的命令做格式化: gofmt -s -w consts/consts.go
gosimple S1034(related information): could eliminate this type assertion 对比一下这两段代码
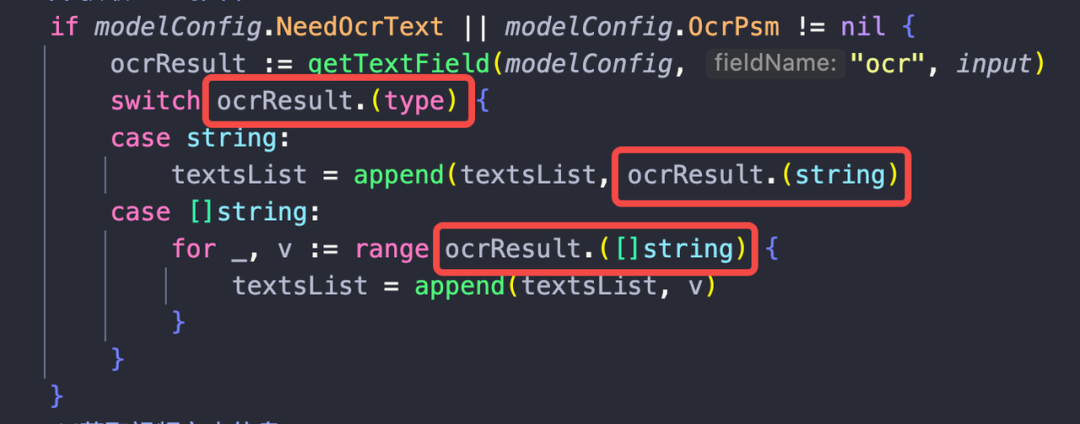
S1005: unnecessary assignment to the blank identifier 参见GoDoc
If the last iteration variable is the blank identifier, the range clause is equivalent to the same clause without that identifier.
Bad practice
Recommended
S1023: redundant return statement 方法最后的无效return,请移除。
S1033: unnecessary guard around call to delete Bad practice
S1009: should omit nil check; len() for nil slices is defined as zero Bad practice
S1008: should use 'return ok == nil' instead of 'if ok == nil { return true }; return false'
S1011: should replace loop with textsList = append(textsList, ocrResult...)
方法的注释,请紧挨着方法体 对比一下这两种写法,在方法提示上的区别
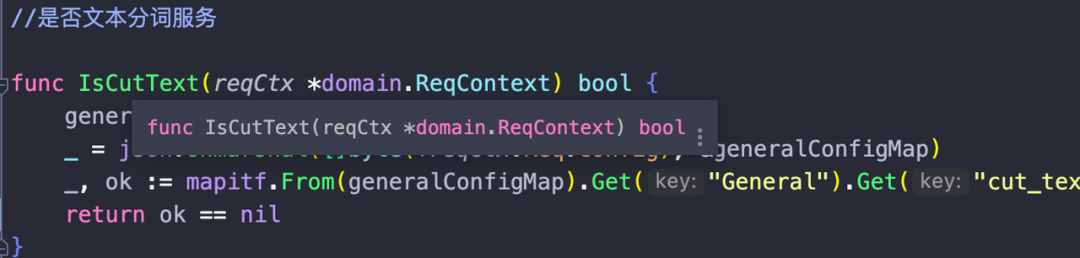
File is not goimports -ed (goimports) 调整import顺序,按照“块”import,不同“块”之间用空行隔开。官方库放在最前面,其他的放在后面。按照前缀做排序。特殊情况下(特殊的package名),可能需要取别名。
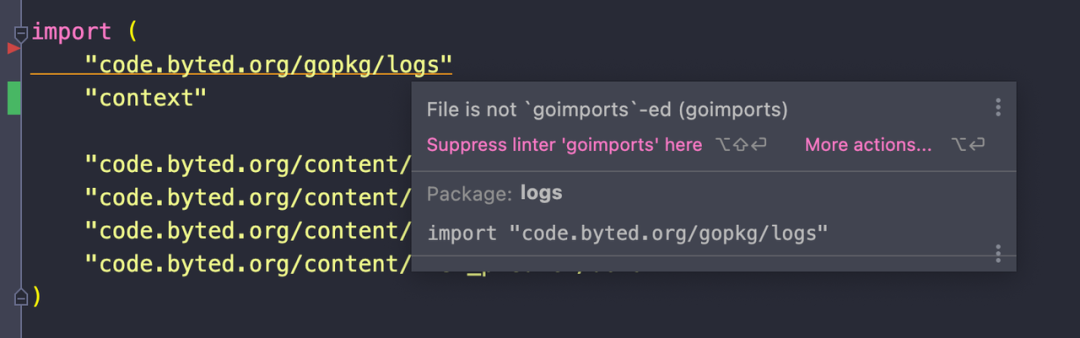
如果实在没办法解决的话,比如,有已经在使用的关键词,但是关键词拼写错误了,必须要豁免lint检测,那么,可以使用//nolint注释来豁免。
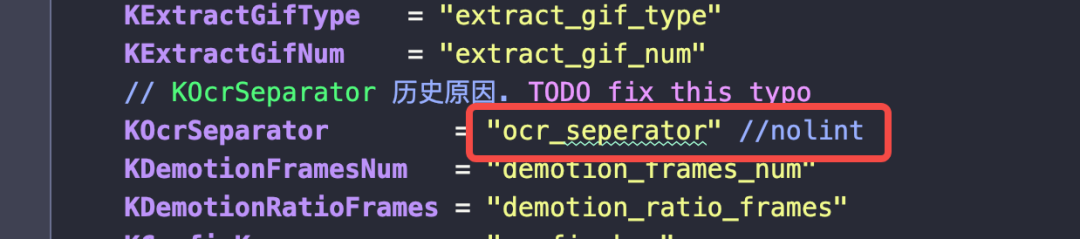
对于不同的代码实体(例如,单行代码、方法、package等)nolint注释的位置也不相同。可以参照此链接。
- Attempt to use unknown class
在mac os上,执行go test带上-race参数时,会出现此错误。看起来,是因为mac OS上执行的原因,可以看看这里,自己再判断下。真正部署到CI实例上,执行codebase CI流程时,并不会出现这样的问题。
单元测试的能力边界应该控制在哪里?比如,一个执行调度的方法,对于超时导致的异常,需要使用单元测试的能力做测试覆盖吗?还是说,应该走用例测试?
- https://staticcheck.io/docs/checks
本文分享自 程序员奇点 微信公众号, 前往查看
如有侵权,请联系 [email protected] 删除。
本文参与 腾讯云自媒体分享计划 ,欢迎热爱写作的你一起参与!
Copyright © 2013 - 2024 Tencent Cloud. All Rights Reserved. 腾讯云 版权所有
深圳市腾讯计算机系统有限公司 ICP备案/许可证号: 粤B2-20090059 深公网安备号 44030502008569
腾讯云计算(北京)有限责任公司 京ICP证150476号 | 京ICP备11018762号 | 京公网安备号11010802020287
Copyright © 2013 - 2024 Tencent Cloud.
All Rights Reserved. 腾讯云 版权所有
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
x/tools/go/analysis/passes/copylock: allow assignment to blank identifier #50551
buglloc commented Jan 11, 2022
bcmills commented Jan 11, 2022
Sorry, something went wrong.
timothy-king commented Jan 11, 2022
Buglloc commented jan 12, 2022, bcmills commented jan 12, 2022 • edited.
- 👍 1 reaction
buglloc commented Jan 14, 2022
No branches or pull requests
Blank identifier (underscore)
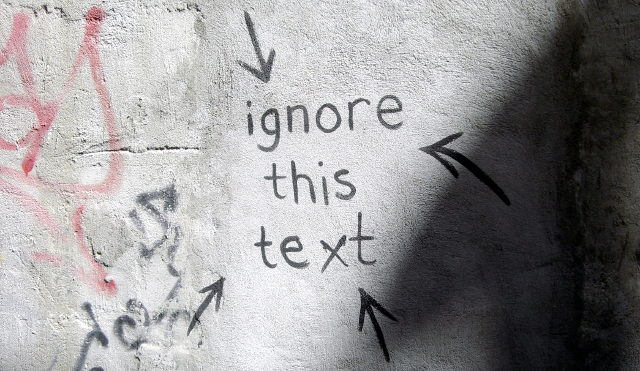
The blank identifier _ is an anonymous placeholder. It may be used like any other identifier in a declaration, but it does not introduce a binding.
Ignore values
The blank identifier provides a way to ignore left-hand side values in an assignment.
Import for side effects
It can also be used to import a package solely for its side effects.
Silence the compiler
It can be used to during development to avoid compiler errors about unused imports and variables in a half-written program.
For an automatic solution, use the goimports tool, which rewrites a Go source file to have the correct imports. Many Go editors and IDEs run this tool automatically.
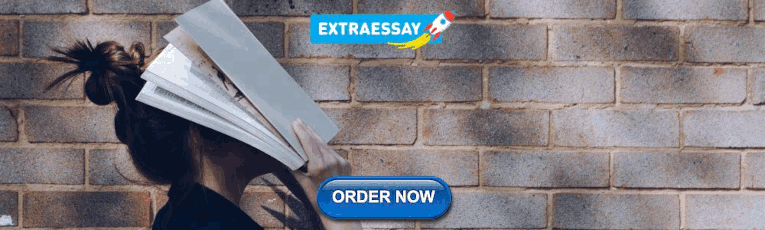
IMAGES
VIDEO
COMMENTS
The T {} is the value that would be assigned to "_", just like "Shelly" is assigned to variable "a". In go, we can assign empty struct to a variable. e.g. type XYZ struct {} var emptyXYZ = XYZ{} fmt.Print("emptyXYZ:", emptyXYZ). var emptyXYZ = XYZ{} equals to var emptyXYZ XYZ = XYZ{}. If emptyXYZ is not XYZ type, say var emptyXYZ string = XYZ ...
The blank identifier ignores any value returned by a function. It can be used any number of times in a Go program. The code below shows how to use the blank identifier. 2. Side effects of import. Sometimes, a package in Go needs to be imported solely for side effects i.e. initialization.
However, you can tell it to trigger on _ assignments with the -blank flag. This is documented in the Use section of the errcheck documentation: The -blank flag enables checking for assignments of errors to the blank identifier. It takes no arguments. At the golang-ci level, this can be specified in the config file: linters-settings ...
Multiple Assignment. In Go, we can use the blank identifier to ignore values returned by functions during multiple assignment. For example: a, _, c := foo() In this example, the second value returned by foo() is ignored by using the blank identifier. This is a common idiom in Go, where functions can return multiple values, but sometimes only ...
The way of making this work is assigning the value that you don't care for to the blank identifier. This isn't limited to range, it works with any multiple assignment, for example functions that return multiple values.
Blank identifier. The blank identifier is represented by the underscore character _. It serves as an anonymous placeholder instead of a regular (non-blank) identifier and has special meaning in declarations, as an operand, and in assignment statements. Official Docs: Why we use it? As you know, in Golang, every should be used in its declaration ...
What is blank identifier. A Blank identifier is a placeholder for unused values. It is represented by an underscore ( _ ). Since blank identifiers have no name, they are also called anonymous placeholders. Golang does not permit declaring unused variables or import statements. That is, you cannot declare a variable and leave it unused.
The blank identifier is represented by the underscore character _. It serves as an anonymous placeholder instead of a regular (non-blank) identifier and has special meaning in declarations, as an operand, and in assignment statements. Official Docs: The Go Programming Language Specification. Effective Go.
While debugging code, one (at least I do) often temporarily stops using a variable and assigns it to the blank identifier to shut up the compiler. For example: x1, x2 := fn() _ = x1 // use(x1) use(x2) As debugging continues and code is u...
Instead, it is preferred to omit the assignment entirely. Here's why it is best to use for range s {} , x = someMap[key], and <-ch instead of using the blank identifier _: Clarity and Readability: By omitting the assignment entirely, it makes the code more readable and self-explanatory. Using _ can introduce confusion and make it less clear ...
Last Updated : 30 Aug, 2022. _ (underscore) in Golang is known as the Blank Identifier. Identifiers are the user-defined name of the program components used for the identification purpose. Golang has a special feature to define and use the unused variable using Blank Identifier. Unused variables are those variables that are defined by the user ...
The type field is a string representing the AST variant type. Each subtype of No…. unnecessary assignment to the blank identifier (s1005)go-staticcheck技术、学习、经验文章掘金开发者社区搜索结果。. 掘金是一个帮助开发者成长的社区,unnecessary assignment to the blank identifier (s1005)go-staticcheck技术 ...
不要全部大写. 缩进格式化 File is not gofmt -ed with -s (gofmt) 执行下面的命令做格式化: gofmt -s -w consts/consts.go. gosimple S1034 (related information): could eliminate this type assertion 对比一下这两段代码. S1005: unnecessary assignment to the blank identifier 参见GoDoc.
The reason there's any assignment allowed at all is for: type T struct {x, _, z int} var t1, t2 T t1 = t2. where the _ is padding. The literal assignment fell out from changing the rules to allow the whole-struct assignment but was probably a mistake. If we had it to do over again, we could probably disallow:
After copylock was able to detect copylock in multi-assignment (golang/tools#319) it starts report false positives on test type assertions. ... allow assignment to blank identifier #50551. buglloc opened this issue Jan 11, 2022 · 5 ... just to ignore unnecessary struct. Unfortunately, the code is private, but I have tried to rewrite it as ...
The blank identifier (_) in Go is a special placeholder used to discard values returned by functions or to ignore specific variables. It's particularly handy when you need to focus on a subset of ...
The blank identifier _ is an anonymous placeholder. It may be used like any other identifier in a declaration, but it does not introduce a binding. Ignore values. The blank identifier provides a way to ignore left-hand side values in an assignment. _, present := timeZone["CET"] sum := 0 for _, n := range a { sum += n } Import for side effects
unnecessary assignment to the blank identifier. pkg/proc/bininfo.go. 250 supportArchs = append (supportArchs, linuxArch) 251} 252 case "windows": 253 for windowArch, _:= range supportedWindowsArch {254 supportArchs = append (supportArchs, windowArch) 255} 256 case "darwin": ... Assigning to the blank identifier is unnecessary. From the Go spec:
The blank identifier _ is used because the variable does not need to be referenced elsewhere in the package. The same result can be achieved with a non-blank identifier: @Theuserwithnohat it's not in the spec. It's an unofficial convention. @Karrot Kake now I understand how it works.
27. The Go programming language specification states: "To import a package solely for its side-effects (initialization), use the blank identifier as explicit package name." For example: import _ "foo/bar". I am having difficulty imagining a use case for this construct. The accepted answer for Usage of the `import` statement mentions a use case ...