Clean One-Line JavaScript Conditionals
August 12, 2021 - 3 minutes read
Writing shorter conditionals in JavaScript, when appropriate, can help keep our code concise yet clean.
I will demonstrate three different shorthand JavaScript conditionals:
Condensed if...else block
One-line ternary expression
One-line binary expression
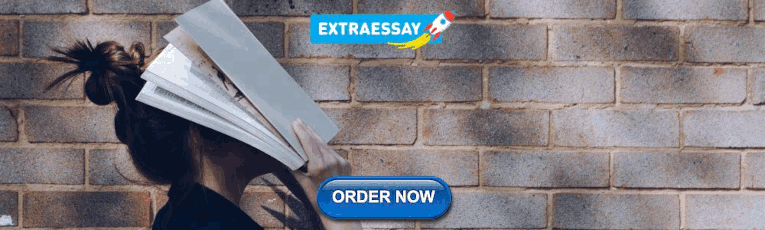
Link to this section Condensed if…else block
Here’s a standard if...else block in JavaScript:
And here’s one way it can be shortened into a single line:
It’s worth mentioning this method also works for else if and else conditions like this one:
Just place each condition on its one line:
However, there is a caveat to this approach .
Consider the following code:
It won’t work. If you’re going to use this type of one-line conditional as the body of an ES6 arrow function , then you’ll need to enclose it in brackets:
Link to this section One-line ternary expression
JavaScript’s ternary expression is a much more versatile one-line conditional. However, it only supports if and else conditions (but not else if conditions).
Here’s a typical block of code using an if and else condition:
Here’s an example of shortening that block using a ternary expression:
A ternary expression contains two special symbols, “ ? ” and “ : ”.
These two special symbols separate the expression into three parts:
The code before the ? is the condition.
The code between the ? and : is the expression to evaluate if the condition is truthy .
The code after the : is the expression to evaluate if the condition is falsy .
Ternary expressions can be used in variable assignments:
And they can also be interlopated within strings:
Link to this section One-line binary expression
Now this is the one that I’ve found especially useful.
The binary expression only supports if conditions, but neither else if nor else conditions.
Here’s a typical if condition:
And here’s how we can use one of JavaScript’s binary expressions to shorten it:
This binary expression is separated by the “ && ” binary operator into two parts:
The code before the && is the condition.
The code after the && is the expression to evaluate if the condition is truthy.
Link to this section Conclusion
You made it to the end!
I hope you find at least one of these shortened conditionals useful for cleaning and shortening your code.
Personally, I use them all the time.
Slim Down Your Code with Single-Line Conditionals
January 23, 2016.
Reading time ~8 minutes
So you’ve been programming in JavaScript for a little while now. Your code is getting lean and mean and DRY as a California reservoir. Maybe you’ve even started to take advantage of some of JavaScript’s single-line conditionals and you’re wondering just how deep this rabbit hole goes. Maybe you have no idea what I’m talking about when I say “single-line conditionals”. Whether you’re a doe-eyed newbie or a hardened professional, this post has some fun tricks you may be interested in.
Braces Optional
The traditional way to write an if statement looks something like this:
This is all fine and good, sticks to practices we’re used to, makes a lot of sense. But what if we only have a single line of code in between those brackets?
Three lines of code for what amounts to one simple if/then statement? I don’t know about you, but this is starting to feel downright wasteful. Well, the fix in this case is rather simple. Just kill the braces:
This is a totally valid JS statement and will execute just fine. Anytime an if is not followed by curly braces, JavaScript will look for the next statement, and consider that the then part of your conditional. Even better, since whitespace is ignored, let’s kill that too:
BAM! Single-line conditional. Not only is it less code, but by removing a bunch of extraneous braces, I think we’ve actually made our code more readable too. And what if our simple if/then has a simple else ? Not a problem, else works the same way:
Simple. Readable. Short. My favorite kind of code. Technically you can do the same thing with else if ’s too, though in that case I might add a bit of white space back in to help with readability. Of course, if your plan was if/else all along, there may be a better tool:
The Ternary Operator
The ternatory operator (so named because it takes three operands), is one of the more intimidating pieces of JavaScript syntax a new coder is likely to encounter. It looks strange and alien, and the way it works is sometimes profoundly unclear. However, if you really want to save space, you can write the above if else statement in one single line:
Frankly, I find that the ternary operator really hurts readability, and I generally avoid it for that reason. You could add some white space to help clear things up:
This is a debatable improvement, and no longer satisfies our single line desires. Why not just go back to an explicit if else at this point? Well, I usually do. BUT, there is a scenario in which there is no substitute for our ternary frienemy: assignment.
Unlike an if else statement, the ternary operator is an operator . That means you are free to use it to the right of an assignment statement, which would throw one heck of a syntax error if you tried it with if else . Though this isn’t necessarily any more readable than other ternary uses, it saves an amazing amount of code when you compare it to the alternative:
The Case For Defaults
It turns out that there are more operators we can press into service to make our conditionals cleaner. One common example is to use the logical OR ( || ) to create default values in functions. For example:
If you’ve never seen it before, this construct may be a little confusing, though it does read in a remarkably sensible way: “input equals input or five.” In other words, if there’s an input, input should be that, if not, it should be five. Just like with a ternary, the beauty of this set up is that we can put this conditional in places you couldn’t put an if statement. Like for instance, as part of the return statement:
Same effect. Less Code. More readable. And imagine the alternate version using if else . Might as well go back to punch cards at that point.
But how does this bizarre hack of the OR operator actually work? The secret is in how JavaScript handles logical operators. In the case of || , JS is trying to determine whether either of the two operands is “truthy”. As soon as it sees the first one is, there is no reason to bother with the second. So it doesn’t. Furthermore, JS never bothers converting a truthy value to true , or a falsey value to false . Why bother? If the first operand is truthy, just return it. If not, the truthiness of the second operand will determine if the overall expression is truthy or not. So don’t even check it, just return it and be done.
One big gotcha to watch out for here: be sure the value of input can’t be falsey value that you want to keep. In the above code for example, if we passed in an input of 0 , we would ignore it and return 5 . There are six falsey values, false , 0 , NaN , '' , undefined , and null , and if our input evaluates to any of them, our default will be returned. But if that is the sort of behvior you are looking for, you can really clean up your code this way. Does that mean we can use && to write single-line conditionals too?
Using && to Write Single-Line Conditionals
Similar to the logical OR, && checks to see if either of two operands is falsey . If the first operand is, there is no point in checking the second. This behavior is not taken advantage of nearly as often as || , but I did just write some actual server code that I couldn’t have been done any other way:
The above helper function may seem a little daunting out of context, so allow me to offer a brief explanation. Using an array of users defined elsewhere, I am creating a series of User objects in my database. The order is important here, so I can’t iterate through users with a simple for loop. If one User happens to be slow to save, the next one would end up being created first. Not good. By using a recursive function I can ensure that each iteration will wait for the one before it.
And what about that insane (read: beautiful) single-line base case? I might have written it more clearly (read: uglily) like this:
If you’ve never seen this usage before, a return statement is a handy way to break out of a function. No more code will be executed once you run that return . It’s perfect for a recursive base case. Even better, if you need to call a function on your way out, rather than write them on seperate lines, you can just return the function call itself. So I might have used my own curly brace lesson from before and written:
But, I have one more problem problem. This helper function can be called in both asynchronus and synchronus environments, and so I do not know ahead of time whether or not next will be defined. The typical construct for calling a function only if it exists is the simple and readable if (fun) fun(); , but if you tried to return if (fun) fun(); , you would be rewarded with a big fat syntax error. Why? Remember, if and else are a statements , ?: , || , and && are operators . You cannot return a statement. But you can return the results of an operation. Which brings us back to my original implementation:
If next is undefined, JavaScript has no need to evaluate next() , and will simply skip it, returning the value to the left ( undefined , which is fine for my purposes). On the other hand, if next is a function (and therefore truthy), JS will look at the value on the right, see that there is a function that needs to be executed, and do so. A fairly complex series of operations have been reduced to one simple (okay, not that simple) line.
With Great Power…
To me, JavaScript is the ultimate “eh, sure I guess”, language. Can I just call undefined false? “Eh, sure I guess.” Can I get rid of these curly braces? “Eh, sure I guess.” What about this OR operator, seems like I could use it to set a default value. “Eh, sure I guess.”
That sort of flexibility can be very freeing, but there are pitfalls too. Remember that human beings still need to read your code. Before I pull out any of these tricks I always try to ask myself: does this make my code cleaner or messier? Does it make it more or less readable?
Shorter is nice, but clearer is better. It’s when you have the opportunity to do both that these tricks really shine.
Scraping the Web for Fun and Profit
So you and your team have been talking about your new social web app. Development has been going well, and someone had the bright idea: "...… Continue reading
The How's Why's and WTF's of Mongoose Hooks
Config files for security and convenience.

How to create one-line if statements in JavaScript self.__wrap_n=self.__wrap_n||(self.CSS&&CSS.supports("text-wrap","balance")?1:2);self.__wrap_b=(r,n,e)=>{e=e||document.querySelector(`[data-br="${r}"]`);let o=e.parentElement,l=u=>e.style.maxWidth=u+"px";e.style.maxWidth="";let s,i=o.clientWidth,p=o.clientHeight,a=i/2-.25,d=i+.5;if(i){for(l(a),a=Math.max(e.scrollWidth,a);a+1 {self.__wrap_b(0,+e.dataset.brr,e)})).observe(o)};self.__wrap_n!=1&&self.__wrap_b(":R16976:",1)

Kris Lachance
Head of Growth
What is Basedash?
Sign up ->
November 6, 2023
One-line if statements in JavaScript are solid for concise conditional execution of code. They’re particularly good for simple conditions and actions that can be expressed neatly in a single line. We’ll go into more detail on all of this below.
What is the basic if statement?
Before diving into one-line if statements, you should know the basic if syntax:
Use the one-line if without curly braces
For a single statement following the if condition, braces can be omitted:
Employ the ternary operator for if-else
The ternary operator is the true one-liner for if-else statements:
Combine methods or operations
Chaining methods or operations in a one-liner if statement can keep your code terse:
Leverage short-circuit evaluation
Short-circuiting with logical operators allows if-else constructs in one line:
Handle assignment within one-line if
You can assign a value based on a condition in one line:
Use arrow functions for inline execution
Incorporate arrow functions for immediate execution within your one-liner:
How to handle multiple one-line if statements
When dealing with several conditions that require one-liners, ensure they remain readable:
Imagine the time you'd save if you never had to build another internal tool, write a SQL report, or manage another admin panel again. Basedash is built by internal tool builders, for internal tool builders. Our mission is to change the way developers work, so you can focus on building your product.
Learn more about Basedash
Book a demo

Use one-liners in callbacks
One-liners can be effectively used within callback functions:
Know when to use if vs. ternary operator
The ternary operator is concise but use a regular if when the condition or actions are too complex for a ternary to remain clear.
Consider one-liners for default values
A one-liner if can set a default value if one isn't already assigned:
Be careful with one-liners and scope
Understand the scope of variables used in one-liners to avoid reference errors:
Remember operator precedence
When using logical operators in one-liners, keep operator precedence in mind to avoid unexpected results:
Avoid using one-liners for function declarations
Defining functions within one-liners can lead to readability and hoisting issues:
Use one-liners with template literals
Template literals can make your one-liners more readable when dealing with strings:
Understand limitations with const
Remember that const declarations cannot be used in traditional one-line if statements due to block-scoping:
Click to keep reading
Ship faster, worry less with Basedash
You're busy enough with product work to be weighed down building, maintaining, scoping and developing internal apps and admin panels. Forget all of that, and give your team the admin panel that you don't have to build. Launch in less time than it takes to run a standup.
Sign up for free

Dashboards and charts
Edit data, create records, oversee how your product is running without the need to build or manage custom software.
ADMIN PANEL
Sql composer with ai.

Related posts

How to Remove Characters from a String in JavaScript
Jeremy Sarchet

How to Sort Strings in JavaScript

How to Remove Spaces from a String in JavaScript

Detecting Prime Numbers in JavaScript
Robert Cooper

How to Parse Boolean Values in JavaScript

How to Remove a Substring from a String in JavaScript
All JavaScript guides

JavaScript One-Liner If-Else: The Ternary Operator Unleashed
Ah, the humble if-else statement: the bread and butter of decision-making in programming. But what if I told you that you could shrink that chunky if-else into a sleek one-liner? Enter the ternary operator, JavaScript’s way to make conditionals concise and sometimes even a bit cryptic. Let’s dive into how you can use this in your code to make it cleaner or just show off to your fellow devs.
What’s the Ternary Operator?
The ternary operator is like that one friend who always gets to the point. It’s a one-liner that has three parts: a condition, a result for true, and a result for false. It looks something like this:
Simple, right? It’s an expression, not a statement, which means it returns a value. That’s why you can use it in assignments, return statements, and more. Let’s see it in action:
Here, if isCoffeeTime is true, we’re brewing coffee. Otherwise, we’re coding away. The ternary operator is perfect for these quick decisions.
Ternary in the Wild: Real-World Examples
Assigning classes in react.
In React, you often need to dynamically assign classes. The ternary operator is your ally here. Imagine you have a button that needs to be highlighted when active:
This keeps your JSX clean and readable, without the need for a verbose if-else statement.
Conditional Rendering in Vue.js
Vue.js is all about simplicity, and the ternary operator fits right in. Say you want to display a message based on a user’s subscription status:
In one line, you’ve handled two different states. Vue-licious!
Setting State in Angular
Angular’s template syntax can also benefit from the succinctness of the ternary operator. Consider a scenario where you’re toggling a menu’s visibility:
Here, the ternary operator is used within the *ngIf directive to determine if the menu content should be rendered. Neat and sweet.
Quick Decisions in Svelte
Svelte aims to write less code and the ternary operator is perfectly at home in this framework. Let’s say you’re showing a user status:
The ternary operator makes it a breeze to conditionally render text in Svelte templates.
When Not to Use the Ternary Operator
While ternary operators are cool, they’re not always the best choice. If your logic is complex, using a ternary can make your code harder to read. Always prioritize clarity over cleverness. If you find yourself nesting ternaries, it’s probably time to step back and use a good old if-else statement or even better, a switch statement or a lookup object.
The ternary operator is a powerful tool in your JavaScript arsenal. It can help you write concise and readable code across different frameworks. Remember, though, with great power comes great responsibility. Use it wisely to keep your code clean and maintainable.
Stay tuned for the second half of this article, where we’ll dive deeper into advanced use cases and the nuances of the ternary operator. Until then, happy coding!
Advanced Ternary Operator Techniques
Now that we’ve covered the basics and seen the ternary operator in action with different frameworks, let’s step up our game. Advanced usage of the ternary operator can involve nesting (though, as warned before, tread lightly), using it for more than just assignment, and even some tricks that might just make you the wizard of one-liners.
Nesting Ternary Operators
Nesting ternary operators allows you to handle multiple conditions. However, it’s crucial to keep readability in mind. If you must nest, do it sparingly and format your code for clarity:
Here, each condition is clearly separated onto a new line, which helps maintain readability. But remember, if this starts to look like a tangled mess, consider a different approach.
Ternary as an Argument in Function Calls
You can use a ternary operator directly in function arguments to conditionally pass values without declaring them beforehand:
This can lead to more compact and inline code, especially when you’re dealing with simple conditions.
Immediate-Invoked Function Expressions (IIFEs)
For those times when you need an if-else with side effects, an immediately-invoked function expression (IIFE) can be combined with a ternary operator:
This pattern encapsulates the logic and executes it immediately, which can be handy in certain situations.
Logical AND (&&) and OR (||) with Ternary
Sometimes you might want to execute something only if a condition is true. This is where you can combine logical operators with the ternary operator for short-circuit evaluation:
Here, console.log('Show logout button') is only executed if loggedIn is true. Otherwise, the right side of the OR ( || ) operator is executed.
Ternary Operator for Object Property Access
You can use a ternary operator to conditionally access object properties without running into undefined errors:
This is particularly useful when dealing with objects that might have optional properties.
Best Practices and Pitfalls
When using the ternary operator, keep these best practices in mind:
- Clarity Over Cleverness : Always prefer readability over writing the shortest code possible.
- Avoid Deep Nesting : If you’re nesting more than once, consider refactoring your code.
- Format for Readability : If you use nested ternaries, format them in a way that makes the logic clear.
- Use for Simple Conditions : Ternary operators are best for simple, straightforward conditions.
- Comment as Needed : If the ternary makes a section of code less obvious, don’t hesitate to add a comment.
Wrapping Up
The ternary operator is a fantastic tool in JavaScript, allowing you to write concise and expressive code. Whether you’re working with React, Vue, Angular, or Svelte, it can help you keep your templates and scripts clean and to the point. Just remember to use it judiciously and never at the expense of the understandability of your code. Now go forth and ternary with confidence, but always keep the sage advice of “less is more” in your coder’s toolkit. Happy coding, folks!
You May Also Like
Inline functions in javascript: a deep dive, unleashing the power of helper functions in javascript.

JavaScript One-Liner If-Else Statements

In JavaScript, you can have if-else statements on one line. To write an if-else statement on one line, follow the terna ry conditional operator syntax:
For example:
This is a useful way to compress short and simple if-else statements. It makes the code shorter but preserves the readability.
However, do not overuse the ternary operator. Converting long if-else statements into one-liners makes your code verbose.
The Ternary Operator – One Line If-Else Statements in JavaScript
Writing a one-line if-else statement in JavaScript is possible by using the ternary operator.
Here is the syntax of the one-liner ternary operator:
For instance, let’s say you have an if-else statement that checks if a person is an adult based on their age:
Running this code prints “Adult” into the console.
This code works fine. But you can make it shorter by using the ternary operator.
This way you were able to save 4 lines of code. And the code is still readable and clear.
Nested One-Line If…Else…Else If Statements in JavaScript
Trying to express everything in one line is no good. The ternary operator should only be used when the code can be made shorter but the readability remains.
However, JavaScript allows you to construct nested ternary operators to replace if…else…else if statements.
This corresponds to:
For instance, you can convert an if…else…else if statement like this:
To an expression like this using the nested ternary operator:
However, you already see why this is a bad idea. The nested ternary operator only makes the code hard to read in this example. Using the ternary operator this way is ineffective.
In this situation, you have two options:
- Use a regular if…else…else if statement instead of a nested ternary operator. That is still perfectly valid JavaScript code!
- Break the nested ternary operator into multiple lines.
Let’s next understand how the latter option works.
Multi-Line Ternary Operator in JavaScript
Your motive to use the ternary operator is probably to express if-else statements as one-liners. But it is good to know you can break a ternary operator into multiple lines too.
As you already saw, the one-liner ternary operator syntax in JavaScript is:
But you can break this expression into multiple lines this way:
Breaking the expression to multiple lines works with nested ternary operators too.
Generally, this type of nested ternary expression:
Becomes a multi-line ternary expression:
Let’s see a concrete example of this by going back to the example of checking the age of a person:
This ternary expression is rather verbose. Assuming you do not want to put it back to a regular if…else…else if statement, you can break it down to multiple lines:
However, it is up to debate whether this makes the code any better than the original if…else…else if statement. Anyway, now you know it is possible.
Be Careful with the Ternary Operator
Your goal is to always write readable and concise code.
Even though the ternary operator is a built-in mechanism, you can treat it as a “trick in a book”.
If you want, you may use the ternary operator to replace basic if…else statements with one-liners. However, avoid increasing the code complexity by replacing longer if…else…else if statements with one-liners. It is more than fine to use regular if…else statements in your code!
If you still want to use a lengthy ternary operator, please make sure to at least break it down into multiple lines.
In JavaScript, you can turn your if-else statements into one-liners using the ternary operator. The ternary operator follows this syntax:
For instance:
JavaScript also supports nested ternary operators for if…else…else if statements. This way you can have as long chains of ternary operators as you like.
Usually, a nested ternary operator causes a long one-liner expression. To avoid this, you can break the ternary operator down into multiple lines:
Keep in mind that using a one-liner if-else statement makes sense if it improves the code quality! Applying it on a complex if…else statement does not make sense as it decreases the code quality.
Here is a “good” example of a rather bad usage of the ternary operator:
Thanks for reading. Happy coding!
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Ternary Operator
JavaScript switch...case Statement
- JavaScript try...catch...finally Statement
- JavaScript if...else Statement
The JavaScript if...else statement is used to execute/skip a block of code based on a condition.
Here's a quick example of the if...else statement. You can read the rest of the tutorial if you want to learn about if...else in greater detail.
In the above example, the program displays You passed the examination. if the score variable is equal to 50 . Otherwise, it displays You failed the examination.
In computer programming, the if...else statement is a conditional statement that executes a block of code only when a specific condition is met. For example,
Suppose we need to assign different grades to students based on their scores.
- If a student scores above 90 , assign grade A .
- If a student scores above 75 , assign grade B .
- If a student scores above 65 , assign grade C .
These conditional tasks can be achieved using the if...else statement.
- JavaScript if Statement
We use the if keyword to execute code based on some specific condition.
The syntax of if statement is:
The if keyword checks the condition inside the parentheses () .
- If the condition is evaluated to true , the code inside { } is executed.
- If the condition is evaluated to false , the code inside { } is skipped.
Note: The code inside { } is also called the body of the if statement.
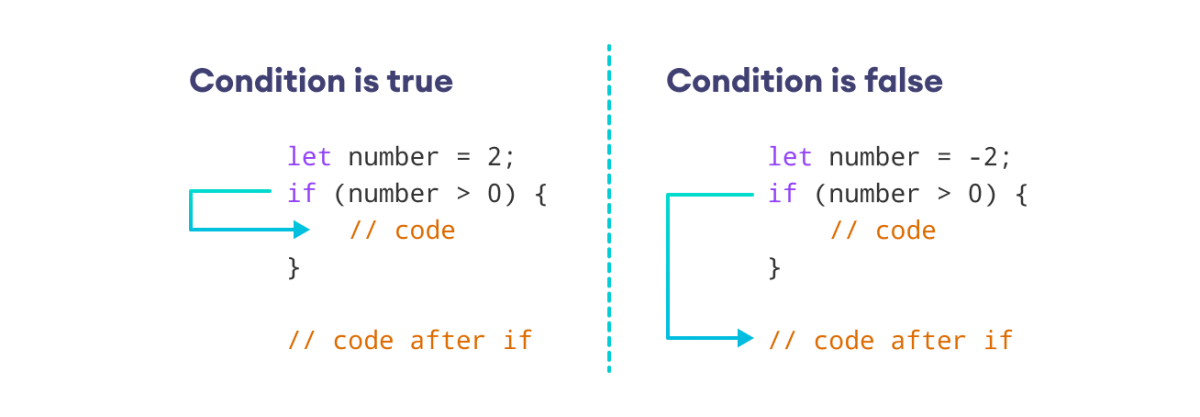
Example 1: JavaScript if Statement
Sample Output 1
In the above program, when we enter 5 , the condition number > 0 evaluates to true . Thus, the body of the if statement is executed.
Sample Output 2
Again, when we enter -1 , the condition number > 0 evaluates to false . Hence, the body of the if statement is skipped.
Since console.log("nice number"); is outside the body of the if statement, it is always executed.
Note: We use comparison and logical operators in our if conditions. To learn more, you can visit JavaScript Comparison and Logical Operators .
- JavaScript else Statement
We use the else keyword to execute code when the condition specified in the preceding if statement evaluates to false .
The syntax of the else statement is:
The if...else statement checks the condition and executes code in two ways:
- If condition is true , the code inside if is executed. And, the code inside else is skipped.
- If condition is false , the code inside if is skipped. Instead, the code inside else is executed.
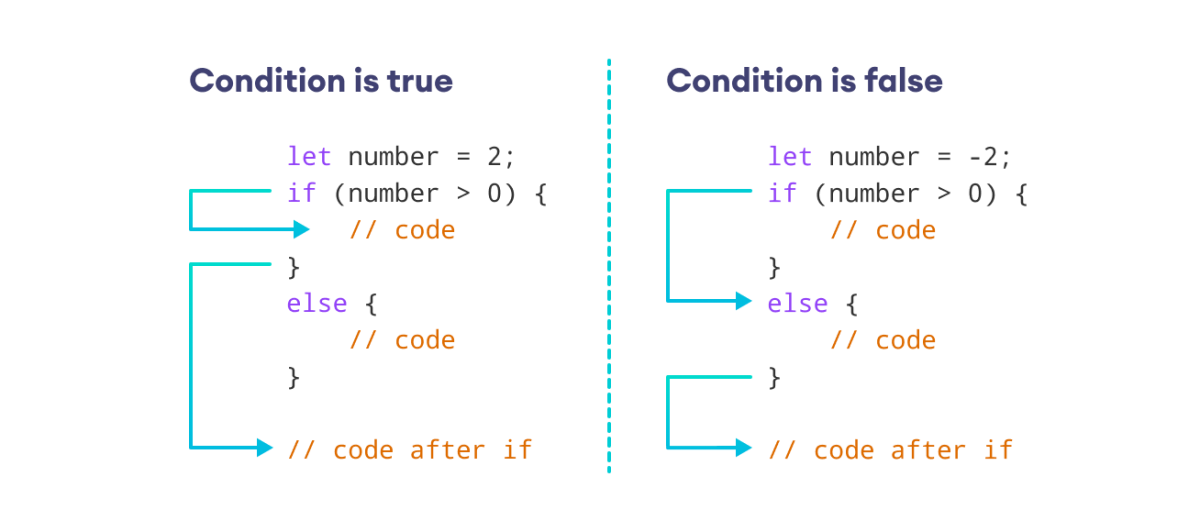
Example 2: JavaScript if…else Statement
In the above example, the if statement checks for the condition age >= 18 .
Since we set the value of age to 17 , the condition evaluates to false .
Thus, the code inside if is skipped. And, code inside else is executed.
We can omit { } in if…else statements when we have a single line of code to execute. For example,
- JavaScript else if Statement
We can use the else if keyword to check for multiple conditions.
The syntax of the else if statement is:
- First, the condition in the if statement is checked. If the condition evaluates to true , the body of if is executed, and the rest is skipped.
- Otherwise, the condition in the else if statement is checked. If true , its body is executed and the rest is skipped.
- Finally, if no condition matches, the block of code in else is executed.
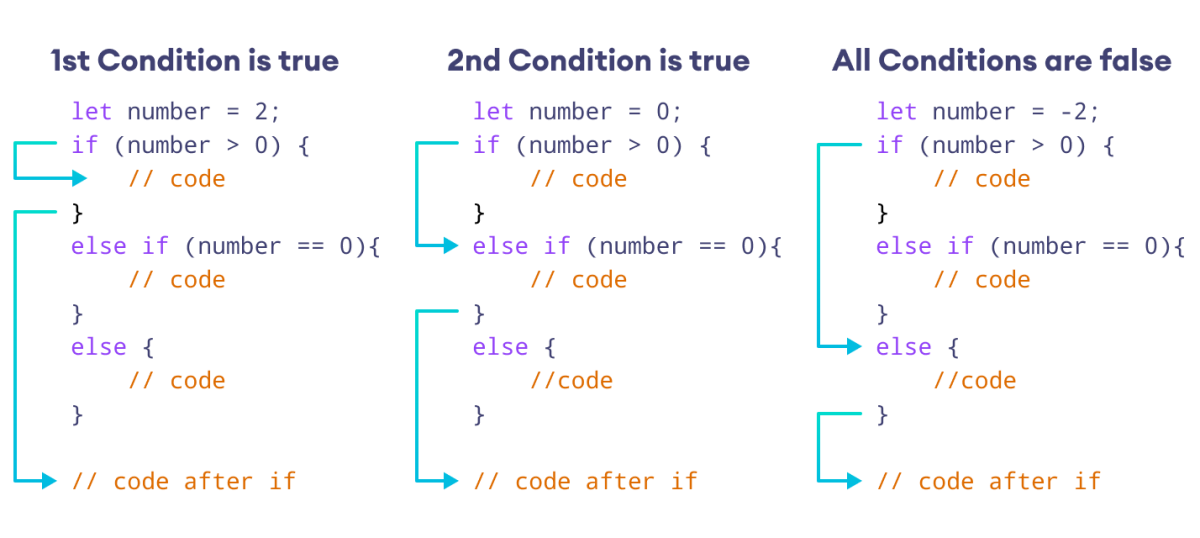
Example 3: JavaScript if...else if Statement
In the above example, we used the if statement to check for the condition rating <= 2 .
Likewise, we used the else if statement to check for another condition, rating >= 4 .
Since the else if condition is satisfied, the code inside it is executed.
We can use the else if keyword as many times as we want. For example,
In the above example, we used two else if statements.
The second else if statement was executed as its condition was satisfied.
- Nested if...else Statement
When we use an if...else statement inside another if...else statement, we create a nested if...else statement. For example,
Outer if...else
In the above example, the outer if condition checks if a student has passed or failed using the condition marks >= 40 . If it evaluates to false , the outer else statement will print Failed .
On the other hand, if marks >= 40 evaluates to true , the program moves to the inner if...else statement.
Inner if...else statement
The inner if condition checks whether the student has passed with distinction using the condition marks >= 80 .
If marks >= 80 evaluates to true , the inner if statement will print Distinction .
Otherwise, the inner else statement will print Passed .
Note: Avoid nesting multiple if…else statements within each other to maintain code readability and simplify debugging.
More on JavaScript if...else Statement
We can use the ternary operator ?: instead of an if...else statement if the operation we're performing is very simple. For example,
can be written as
We can replace our if…else statement with the switch statement when we deal with a large number of conditions.
For example,
In the above example, we used if…else to evaluate five conditions, including the else block.
Now, let's use the switch statement for the same purpose.
As you can see, the switch statement makes our code more readable and maintainable.
In addition, switch is faster than long chains of if…else statements.
We can use logical operators such as && and || within an if statement to add multiple conditions. For example,
Here, we used the logical operator && to add two conditions in the if statement.
Table of Contents
- Introduction
Video: JavaScript if...else
Sorry about that.
Related Tutorials
JavaScript Tutorial

What is Javascript one line if? How To Use It?
In this comprehensive article, we will explore the ins and outs of the JavaScript one line if statement .
We will cover its syntax, use cases, best practices, and how it can contribute to writing cleaner, more readable code.
Whether you’re a beginner or an experienced developer, understanding this powerful technique will elevate your JavaScript skills to the next level.
What is javascript one line if?
The JavaScript one line if statement is a condensed version of the traditional if-else statement. It is often used when you need to perform a simple conditional check and execute a single line of code based on the result.
The basic syntax is as follows:
The condition is the expression that is evaluated for truthiness or falsiness . If the condition evaluates to true, the expression immediately after the ? is executed.
Otherwise, if the condition is false, the expression after the : is executed .
Benefit of Javascript one liner if
One of the primary benefits of using the one line if statement is its ability to simplify code . Instead of writing multiple lines of code for a simple condition, you can achieve the same result in a single line.
Let’s look at an example:
The above code can be rewritten using the one line if statement:
As you can see, the one line if statement reduces the code’s verbosity and makes it more concise.
How to do if statement one line javascript?
The JavaScript one line if statement is a versatile tool that can be used in various scenarios. Let’s explore some common use cases to this:
1. Ternary Operators
The one line if statement is often referred to as the “ternary operator” because it involves three operands: the condition, the expression executed if the condition is true, and the expression executed if the condition is false.
2. Setting Default Values
You can use the one line if statement to set default values for variables if they are undefined or null.
3. Short-Circuiting
The one line if statement can help with short-circuiting, where an expression stops being evaluated as soon as the result is determined.
Best Practices for Using JavaScript One Line If
While the JavaScript one line if statement can be a powerful tool, it’s essential to use it judiciously and follow best practices:
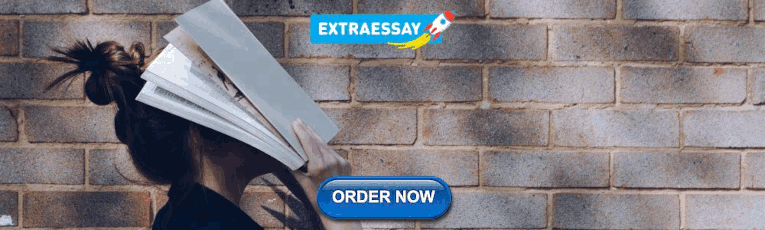
1. Keep It Simple and Readable
The primary advantage of the one line if statement is its simplicity. Avoid using complex expressions that may confuse other developers reading your code.
2. Limit to Single Expressions
As the name suggests, the one line if statement is best suited for executing a single expression based on a condition. Avoid using it for lengthy code blocks.
3. Add Parentheses for Clarity
To enhance code readability, consider using parentheses around the conditional expression.
4. Avoid Nested Ternary Operators
While you can nest ternary operators, doing so can quickly become unreadable. Opt for regular if-else statements if the logic becomes more complex.
5. Use Comments to Explain Complex Conditions
If your condition involves intricate logic, use comments to explain what it does and why.
Anyway here are other Javascript functions that can possibly enhance your JavaScript skills.
- What is Javascript serialization? How It Works?
- String Filter JavaScript: Simplifying Data Processing
To conclude, JavaScript one line if statement is a valuable tool for developers seeking to write cleaner and more efficient code. With its concise syntax, it simplifies conditional checks and reduces code verbosity. By understanding its use cases and best practices, you can leverage the power of the one line if statement to improve your JavaScript coding skills.
So, next time you encounter a straightforward condition that requires an if-else statement, consider using the one line if statement to create elegant and concise code snippets.
Leave a Comment Cancel reply
You must be logged in to post a comment.


Mastering JavaScript Inline If Statements: A Complete 2021 Guide
Conditional logic allows us to control program flow based on dynamic values and user input. It is one of the core building blocks of writing reusable, maintainable code.
If you‘ve written any substantial JavaScript, you‘ve surely used standard if/else statements many times. But did you know there are other more compact ways to write conditionals right inside expressions?
Inline if statements provide a minimized syntax for conditionally executing code or evaluating different expressions. In this comprehensive guide, you‘ll learn:
- What are inline if statements and when should you use them?
- How to write inline if/else logic using ternary operators
- When to use logical && instead for conditionals
- Pros, cons, and best practices for inline if usage
- How performance compares to standard if statements
- Examples and real-world applications for writing better conditional code
By the end, you‘ll master these core techniques to write cleaner conditional logic in your JavaScript code. Let‘s get started!
Why Use Inline If Statements?
Before we look at the syntax, when might you want to use an inline if statement vs a standard multiline if block?
Some common use cases for inline if:
- Assigning variables or passing function arguments conditionally
- Returning different values from a function based on a condition
- Selecting between two expressions based on a boolean test
- Conditionally executing a statement or block of code
Benefits of inline if include:
- More compact "one line" syntax
- Avoid unnecessary code nesting and blocks
- Improved readability in some cases
- Easier to return or assign conditional values directly
However, for more complex conditional logic, standard if statements are usually preferable.
As a rule of thumb, consider using inline if for simpleconditionals, and standard if statements for longer multiline logic.
Now let‘s look at the different options for writing inline if/else in JavaScript…
Inline If with the Ternary Operator
The ternary operator provides us with the most flexibility and options for inline conditional logic.
Ternary Syntax
Here is the syntax for a ternary operator:
It checks the condition , and executes either the exprIfTrue or exprIfFalse based on the result.
Some examples:
The condition can be any valid JavaScript expression that evaluates to true/truthy or false/falsy.
This allows us to assign values or return results inline based on a conditional check.
Ternary for Multiple Conditions
We can also chain multiple ternary operators together to have multiple case conditions:
Here we have nested ternaries to check for different age brackets.
Chaining ternaries works well for a limited number of conditions. Beyond 2-3, it often hurts readability – so consider standard if/else instead for more complex logic flows.
Ternary Use Cases
Some common examples of using ternary operators:
- Assign variable values conditionally
- Conditionally pass function arguments
- Return different function results based on condition
- Choose between two expressions
Ternary vs If…Else
So when should you prefer the ternary operator vs standard if/else conditional statements?
Consider ternary when:
- You need to assign a variable or return value conditionally
- The logic is simple (1-2 conditions)
- You want a compact "one liner" syntax
- Readability is improved by avoiding nesting/blocks
Prefer standard if statement when:
- Multiple complex conditions and logic branches
- Multiline logic that is harder to read in ternary
- Code clarity is improved by blocks and indentation
- Nesting ternaries hurts readability
The ternary operator is ideal for quick inline conditional checks and assignments. For longer conditional flows, standard if statements are often preferable.
Inline If using Logical &&
Along with the ternary operator, we can also use JavaScript‘s logical AND (&&) for inline conditionals.
Here is an example:
The && works by first evaluating the left side. If it is truthy, it also executes the right side. If falsy, it stops without running the right side.
Some other examples:
When to use Logical &&
The && operator is best for:
- Conditionally executing a function or expression
- Defaulting variables if null/undefined
- Short circuiting execution if falsy
It provides a minimal syntax for these cases.
However, the && operator does have limitations:
- Only runs right side, no ability to return else value
- Can‘t use it to return different values like ternary
- Only useful for conditionally executing code, not returning
So in summary, && is a lighter inline syntax that works well for conditionally executing code. The ternary operator is more flexible overall.
Comparing Ternary and && Performance
An important consideration is if these inline conditionals have any performance differences compared to standard if statements.
Good news – JavaScript engines are excellent at optimizing conditionals.
According to benchmarks, there is negligible difference in performance between ternary, &&, and regular if statements.
Some notable findings from performance tests:
- All 3 options optimize to similar assembly output
- AND is fractionally faster in isolated tests
- Performance varies more based on code structure
- Don‘t optimize prematurely based on assumptions
So you can focus on writing clean readable code rather than micro-performance. Only optimize conditionals when you have evidence of real bottlenecks.
Common Mistakes to Avoid
Let‘s now go over some best practices and common mistakes when using inline if statements:
- Avoid long or complex ternary expressions – these hurt readability. Standard if statements are better suited.
- Don‘t nest ternaries too deeply – Chaining beyond 2-3 levels often makes code harder to understand.
- Always wrap conditions in () – This avoids incorrect operator precedence.
- Use good variable names – Well-named variables help document inline conditionals.
- Watch out for assignment vs equality – Using = rather than == can lead to unintended results.
- Be careful when returning object literals – The { } syntax affects statement interpretation.
Following these best practices will help you avoid issues when leveraging inline if/else conditionals.
Putting Into Practice
The best way to get comfortable with inline conditionals is seeing examples of how they can be used in real code.
Here are some practical applications:
There are many cases where compact inline if/else logic can help write cleaner code vs standard if blocks.
Key Takeaways
Here are some key tips to remember:
- Use ternary operators for conditional assignment/returns
- Logical && has a lighter syntax for conditionally executing code
- Standard if statements are better for complex multiline logic
- Neither ternary nor && have significant performance costs
- Balance readability vs brevity when choosing expressions
- Avoid nesting ternaries too deeply
With the right approach, inline if statements allow you to write conditional code that is clear, compact, and efficient.
JavaScript offers flexible options for inline conditionals – from ternary and && operators to regular multiline if. By mastering all these techniques, you can write professional-grade conditional logic.
I hope this guide has helped demystify inline if statements! Let me know if you have any other questions.
You maybe like,
Related posts, "what‘s the fastest way to duplicate an array in javascript".
As a fellow JavaScript developer, you may have asked yourself this same question while building an application. Copying arrays often comes up when juggling data…
1 Method: Properly Include the jQuery Library
As a JavaScript expert and editor at thelinuxcode.com, I know first-hand how frustrating the "jQuery is not defined" error can be. This single error can…
A Beginner‘s Guide to Formatting Decimal Places in JavaScript
As a web developer, you‘ll frequently run into situations where you need to display nice, cleanly formatted numbers in your interfaces. Have you ever had…
A Complete Guide to Dynamic DOM Manipulation with JavaScript‘s appendChild()
As a Linux developer building modern web applications, being able to efficiently interact with the DOM (Document Object Model) using JavaScript is a crucial skill.…
A Complete Guide to Dynamically Adding Properties in JavaScript
As an experienced JavaScript developer, I often get asked about the different ways to add properties to objects in JavaScript. While this may seem like…
A Complete Guide to Dynamically Changing Image Sources with JavaScript
This comprehensive tutorial explains how to change image sources dynamically in JavaScript. We‘ll cover the ins and outs of swapping images on the fly using…
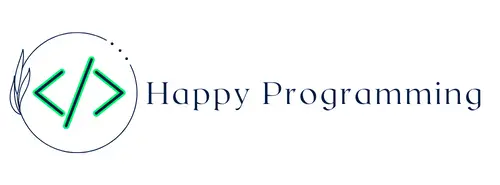
How To Create and Use One-line If statement in JavaScript?

BoYoYo Tough Dog Toys for Aggressive Chewers Large Breed, Indestructible Dog Toys for Large Dogs, Dog Chew Toys for Aggressive Chewers, Durable Dog Toys, Super Chewer Dog Toys for Medium Dog, Heavy…

CELSIUS Assorted Flavors Official Variety Pack, Functional Essential Energy Drinks, 12 Fl Oz (Pack of 12)
One-line if statements allow writing conditional logic concisely in JavaScript . When used properly, they can make code more readable by reducing visual clutter.
Benefits of One-Line If Statements
- Avoid boilerplate code and nested indentation
- Improve readability for simple logic
- Prevent unintended scope changes
- Reduce noise and focus attention
- Concise syntax for frequent binary checks
Drawbacks to Consider
- Can reduce understandability if overused
- Limited to simple expression without blocks
- Lack support for else if and multiple branches
- Easy to introduce subtle bugs and mistakes
Ternary Operator If/Else
- Syntax options and variations
- Conditional (immediate) execution vs assignment
- Single expression vs full statement syntax
- Limitations and good use cases
- Examples including nesting ternaries
Logical AND If Statement
- Short-circuit evaluation behavior
- Truthy/falsy gotchas to watch out for
- Lack of else clause and empty statement
- Examples including default assignment
- Edge cases and cautionary tales
Logical OR If Statement
Multi-line if statement alternatives.
- Traditional if/else statements
- Switch case statements
- Immediately invoked function expressions (IIFEs)
- When to prefer multi-line conditional logic
Readability Best Practices
- Formatting guidelines for one-liners
- Limiting line length
- Comments for clarification
- Appropriate and judicious use
- Refactoring criteria and improving legibility
By the end of this deep dive, you’ll thoroughly understand the ins and outs of writing readable and robust one-line if statements in JavaScript. Let’s get started!
One-line if statements provide several advantages over standard multi-line conditional blocks:
Avoid Boilerplate Code and Nested Indentation
By collapsing if/else logic into a single line, one-liners avoid extraneous braces, indentation, and boilerplate JavaScript syntax.
This cleans up the appearance of simple conditional checks:
The reduction in visual clutter helps focus attention on the logic rather than the syntactic structure.
Improve Readability for Simple Logic
For straightforward true/false checks, one-line conditionals can actually improve readability over multi-line alternatives. The intent becomes more immediately clear.
By skimming for the binary operators like ? :, && and ||, you can quickly grasp simple conditional logic.
Prevent Unintended Scope Changes
Unlike multi-line if statements, one-liners don’t create a new nested lexical scope in JavaScript. This avoids issues when conditionally setting variables:
Reduce Noise and Focus Attention
Removing indentation, braces, and boilerplate focuses attention purely on the conditional logic. This forces you to distill conditionals down to their essence.
Concise Syntax for Frequent Binary Checks
For very common binary true/false checks, the compact operators ? : and && || provide convenient shorthand syntax. This reduces visual repetition.
However, one-liners also come with drawbacks, especially if overused.
While one-line if statements can help clean up simple conditionals, be aware of these potential downsides:
Can Reduce Understandability if Overused
Too many compact one-liners packed together make it harder to linearly scan and understand code. It becomes challenging to parse the program flow when scroll-reading.
Limited to Simple Expression Without Blocks
One-line conditionals only allow an immediate expression, not a full block with arbitrary logic. This forces code fragmentation into separate one-liners.
Lack Support for else if and Multiple Branches
Most one-line formats like ?: and && only offer simple if/else logic. Complex conditional chains require multi-line if/else instead.
Easy to Introduce Subtle Bugs and Mistakes
Omitting braces increases the risk of bugs when adding or modifying logic. It’s also easy to incorrectly assign instead of compare equality.
Not Always More Readable Than Multi-Line
Although one-liners remove visual noise, deeply nested and fragmented one-liners often become less readable than well-formatted multi-line conditional blocks.
Mixing One-Liners and Multi-Line Can Be Jarring
The constant switching between compact one-liners and multi-line blocks creates cognitive dissonance. It interrupts the reading flow when scanning code.
So while one-line if statements can help clean up simple checks, take care not to overuse them or force complex logic into compact syntax.
Now let’s explore specific one-line options starting with the ternary operator.
The ternary operator provides an inline way to evaluate if/else conditional logic:
Let’s break down the syntax:
- condition – Expression evaluating to true or false
- ? – Separator denoting start of if case
- exprIfTrue – Expression to execute if condition is truthy
- : – Separator between if and else cases
- exprIfFalse – Expression to execute if condition is falsy
This allows implementing if/else logic in a single line instead of multiple.
For example:
Ternary statements make great one-line shortcuts but also introduce complexity. Here are some nuances to understand:
Conditional (Immediate) Execution vs Assignment
The ternary operator is commonly used for conditional assignment to a variable:
However, it can also execute code conditionally without an assignment:
This provides a concise one-line if/else statement shorthand specifically for conditional execution, avoiding unnecessary temporary variables.
Single Expression vs Full Statement Syntax
By default, the ternary expects simple expressions in the if and else clauses:
But you can also execute multi-line statements using a comma operator:
This allows more complex logic while keeping the ternary syntax compact.
Limitations and Good Use Cases
The ternary operator only supports an if and else expression. It does not have else if or additional conditional branches.
So the ternary works best for simple binary checks and assignments, not complex multi-way logic. Good use cases:
- Toggle between two possible values like on/off, enabled/disabled etc
- Pick between two code paths conditionally
- Set a default value if a condition fails
- Initializing a variable based on a condition
Examples Including Nesting Ternaries
Here are some examples of using the ternary operator effectively:
Default Value Assignment
Conditional Console Output
Nested Ternary
Nesting ternaries allows implementing cascading if/else logic concisely. However, deeply nested ternaries rapidly become unreadable.
In summary, the ternary operator provides a compact inline syntax for basic conditional assignment and execution. Next, we’ll cover using logical operators && and || for one-line if statements.
The logical AND operator && can be used to execute a statement if a condition is truth:
This greets the user only if getUser() returned a valid user object.
Let’s explore how logical AND works and situational nuances to be aware of when using it in conditionals.
Short-Circuit Evaluation
The && operator uses short-circuit evaluation:
- Evaluates the left condition first
- If falsy, short-circuits and does NOT evaluate the right side
- If truthy, executes the right expression
This avoids needing to explicitly compare truthiness:
Short-circuiting makes logical AND ideal for optional chained calls like:
If user is falsy, the right side will not execute, preventing potential errors.
Truthy/Falsy Gotchas
Due to short-circuiting behavior, subtle bugs can occur based on truthy/falsy assumptions.
For example, this appears to check if cards contains any elements:
However, it will print the message if cards is truthy , even if it’s an empty array!
Instead, compare length explicitly:
So take care to properly check truthiness when using && in conditionals.
Lack of Else Clause
A drawback of logical AND is there is no else clause – it only executes the right side statement if the condition passes.
This limits it to straightforward binary true/false cases with a single expression. For anything more complex, use a full if/else statement.
Examples Including Default Assignment
Here are some examples of using logical AND effectively in one-line conditionals:
Check Empty Array
Default Assignment
Guarding Function Calls
Overall, logical AND provides a convenient compact syntax for executing expressions based on truthy conditions. But take care to properly handle truthy/falsy assumptions and edge cases due to lack of an else clause.
The logical OR operator || can serve as a concise one-line if statement as well:
This logs a message if the getUser() check returned a falsy value.
Logical OR works similarly to AND but with inverted behavior due to short-circuit evaluation:
- If truthy, short-circuits and does NOT evaluate the right side
- If falsy, executes the right expression
Next, we’ll explore nuances and cautionary tales when using || in one-line conditionals.
Due to short-circuiting, pay close attention to truthy/falsy assumptions.
This appears to log if user is null:
But it will execute the right side for any falsy value like 0 or empty string! Instead compare equality:
So double check truthiness assumptions when using || conditionally.
Similar to &&, logical OR does not have an else clause. It only executes the right expression if the condition is falsy .
There is no way to specify a second expression if the condition passes. This limits || for simple binary cases.
Here are some examples of using logical OR well:
Assigning Default Values
Providing Fallback Values
Conditionally Executing Code
In summary, logical OR provides a way to execute code or values based on falsy conditions. But use caution to avoid wrong assumptions due to truthy/falsy evaluations.
Now let’s look at some multi-line conditional alternatives.
For complex conditional logic, one-line statements may not provide enough flexibility. Some common multi-line options include:
Traditional if/else Statements
Regular if/else conditional blocks allow full control flow with else if and else branches:
The ability to check multiple conditions and choose between different code paths helps handle more complex logic.
Switch Case Statements
Switch case is useful when conditionally executing different code blocks based on discrete values:
The orderly cases and ability to fallthrough make switch well-suited for certain types of conditional logic.
Immediately Invoked Function Expressions (IIFE)
Wrapping conditional logic in an IIFE avoids leaking to the surrounding scope:
IIFEs allow using multi-line conditional logic without introducing variable hoisting issues.
When to Prefer Multi-line Conditionals
Consider using a multi-line conditional format if:
- You need else if / else clauses
- The logic is complex or verbose
- Code clarity is more important than brevity
- Scope issues exist with one-liners
- Mixing one-liners and multi-line becomes messy
Now let’s look at some readability best practices when using one-line conditionals.
To keep one-line if statements maintainable, consider these formatting and usage suggestions:
Break Lines on Operators
Consider breaking a long ternary operator over multiple lines:
Or break AND/OR logical statements:
This improves readability while keeping compact syntax.
Limit Line Length
In general, try to keep lines under 80 characters when possible by breaking conditionals across multiple lines.
Use Comments for Clarification
Add occasional comments above one-liners to document their intent if not immediately clear:
Use Braces for Complex Logic
If you need multiple statements in a conditional branch, use braces:
Don’t try to cram complex logic into a single statement.
Use Judiciously and Refactor
Resist overusing one-liners. If you have successive conditionals or deep nesting, consider refactoring to multi-line conditionals for better readability.
Appropriate and Judicious Use
In general, follow these guidelines for using one-liners judiciously:
- Reserve for simple true/false checks and assignments
- Avoid cramming complex logic into terse syntax
- Prefer multi-line conditionals for nested logic
- Only use when logic is immediately clear to the reader
- Refactor to multi-line if any ambiguity arises
- Document unclear one-liners with preceding comments
- Format for line length and readability
Err on the side of clarity rather than brevity.
Refactoring Criteria
Consider refactoring one-liners to multi-line conditionals if:
- They exceed 80 characters per line
- You need to scroll horizontally to read them
- There is nested conditional logic
- You are repetitively toggling between one-liners and multi-line
- Intermixing one-liners and multi-line becomes disjointed
The goal is to use the best syntax for overall code clarity and improve legibility. Ask yourself if expanding a one-liner to multiple clearly formatted lines would help or harm understandability.
The key takeaways:
- One-line if statements using ?:, && and || can improve compactness
- Avoid overusing terse one-liners at the expense of readability
- Stick to simple true/false checks rather than complex logic
- Prefer multi-line conditionals for nested clauses
- Use sensible formatting, line splitting, and comments
- Refactor code when one-liners become unreadable
- Ensure logic remains immediately clear to the reader
One-line if statements have appropriate uses for cleaning up trivial conditionals. But take care not to overuse them or obscure intent.
Prioritize code clarity through judicious use, formatting, and refactoring. The end goal is readable and maintainable software.
I hope this guide provides a thorough understanding of how to effectively leverage one-line if statements in JavaScript . Let me know if you have any other questions!

Outward Hound Nina Ottosson Dog Smart Dog Puzzle Interactive Treat Puzzle Dog Enrichment Dog Toy, Level 1 Beginner, Orange

Shure SM7B Dynamic Vocal Microphone
Leave a comment cancel reply.
Save my name, email, and website in this browser for the next time I comment.
- Skip to primary navigation
- Skip to main content
- Skip to primary sidebar
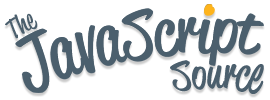
JavaScriptSource
Search 5,000+ Free JavaScript Snippets
One-Liner If-Else Statements
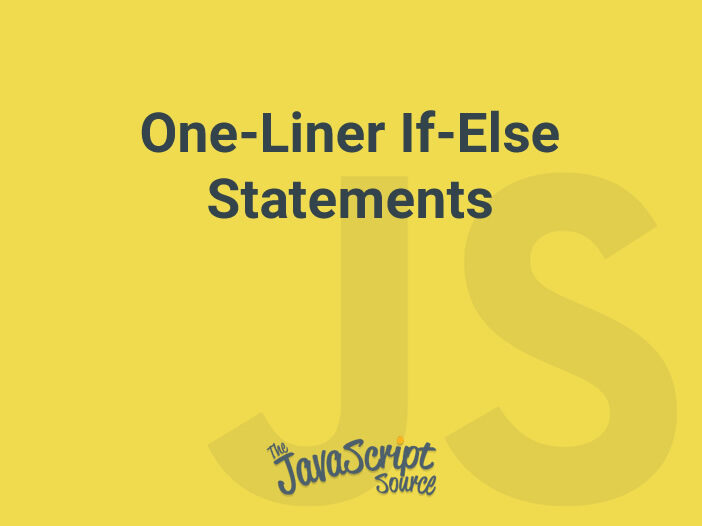
This is a common feature in many programming languages. Instead of writing an if-else on multiple lines, you can use the ternary operator to write the whole statement with one line of code.
For example:
However, do not overuse this. It can quickly make your code more verbose too. It is wise to replace only simple expressions with this to improve readability and reduce lines of code.
https://medium.com/geekculture/20-javascript-snippets-to-code-like-a-pro-86f5fda5598e
Related Snippets:
- JavaScript Variables Explained
- Bitwise Operators in JavaScript
- Arithmetic Operators in JavaScript
- JavaScript Booleans Explained
Proposal: Annotate types in multiple assignment
In the latest version of Python (3.12.3), type annotation for single variable assignment is available:
However, in some scenarios like when we want to annotate the tuple of variables in return, the syntax of type annotation is invalid:
In this case, I propose two new syntaxes to support this feature:
- Annotate directly after each variable:
- Annotate the tuple of return:
In other programming languages, as I know, Julia and Rust support this feature in there approaches:
I’m pretty sure this has already been suggested. Did you go through the mailing list and searched for topics here? Without doing that, there’s nothing to discuss here. (Besides linking to them).
Secondly, try to not edit posts, but post a followup. Some people read these topics in mailing list mode and don’t see your edits.
- https://mail.python.org
- https://mail.python.org/archives

For reference, PEP 526 has a note about this in the “Rejected/Postponed Proposals” section:
Allow type annotations for tuple unpacking: This causes ambiguity: it’s not clear what this statement means: x, y: T Are x and y both of type T , or do we expect T to be a tuple type of two items that are distributed over x and y , or perhaps x has type Any and y has type T ? (The latter is what this would mean if this occurred in a function signature.) Rather than leave the (human) reader guessing, we forbid this, at least for now.
Personally I think the meaning of this is rather clear, especially when combined with an assignment, and I would like to see this.
Thank you for your valuable response, both regarding the discussion convention for Python development and the history of this feature.
I have found a related topic here: https://mail.python.org/archives/list/[email protected]/thread/5NZNHBDWK6EP67HSK4VNDTZNIVUOXMRS/
Here’s the part I find unconvincing:
Under what circumstances will fun() be hard to annotate, but a, b will be easy?
It’s better to annotate function arguments and return values, not variables. The preferred scenario is that fun() has a well-defined return type, and the type of a, b can be inferred (there is no reason to annotate it). This idea is presupposing there are cases where that’s difficult, but I’d like to see some examples where that applies.
Does this not work?
You don’t need from __future__ as of… 3.9, I think?

3.10 if you want A | B too: PEP 604 , although I’m not sure which version the OP is using and 3.9 hasn’t reached end of life yet.
We can’t always infer it, so annotating a variable is sometimes necessary or useful. But if the function’s return type is annotated then a, b = fun() allows type-checkers to infer the types of a and b . This stuff isn’t built in to Python and is evolving as typing changes, so what was inferred in the past might be better in the future.
So my question above was: are there any scenarios where annotating the function is difficult, but annotating the results would be easy? That seems like the motivating use case.
Would it be a solution to put it on the line above? And not allow assigning on the same line? Then it better mirrors function definitions.
It’s a long thread, so it might have been suggested already.
Actually, in cases where the called function differs from the user-defined function, we should declare the types when assignment unpacking.
Here is a simplified MWE:
NOTE: In PyTorch, the __call__ function is internally wrapped from forward .
Can’t you write this? That’s shorter than writing the type annotations.
This is the kind of example I was asking for, thanks. Is the problem that typing tools don’t trace the return type through the call because the wrapping isn’t in python?
I still suggest to read the thread you linked, like I’m doing right now.
The __call__ function is not the same as forward . There might be many other preprocessing and postprocessing steps involved inside it.
Yeah, quite a bit of pre-processing in fact… unless you don’t have hooks by the looks of it:
Related Topics
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Logical OR assignment (||=)
The logical OR assignment ( ||= ) operator only evaluates the right operand and assigns to the left if the left operand is falsy .
Description
Logical OR assignment short-circuits , meaning that x ||= y is equivalent to x || (x = y) , except that the expression x is only evaluated once.
No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const :
Neither would the following trigger the setter:
In fact, if x is not falsy, y is not evaluated at all.
Setting default content
If the "lyrics" element is empty, display a default value:
Here the short-circuit is especially beneficial, since the element will not be updated unnecessarily and won't cause unwanted side-effects such as additional parsing or rendering work, or loss of focus, etc.
Note: Pay attention to the value returned by the API you're checking against. If an empty string is returned (a falsy value), ||= must be used, so that "No lyrics." is displayed instead of a blank space. However, if the API returns null or undefined in case of blank content, ??= should be used instead.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Logical OR ( || )
- Nullish coalescing operator ( ?? )
- Bitwise OR assignment ( |= )
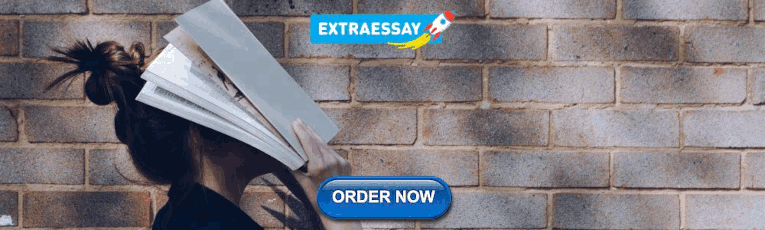
IMAGES
VIDEO
COMMENTS
One line if/else in JavaScript [duplicate] Ask Question Asked 9 years ago. Modified 9 years ago. Viewed 126k times ... Combine all into one line. You don't need to create empty object, it can have properties and if brevity is what you want don't need the isItMuted either .
I know you can set variables with one line if/else statements by doing var variable = (condition) ? (true block) : (else block), but I was wondering if there was a way to put an else if statement in there. Any suggestions would be appreciated, thanks everyone!
Clean One-Line JavaScript Conditionals. August 12, 2021 - 3 minutes read. Writing shorter conditionals in JavaScript, when appropriate, can help keep our code concise yet clean. I will demonstrate three different shorthand JavaScript conditionals: Condensed if...else block. One-line ternary expression. One-line binary expression
What's a One-Line If in JavaScript? In the JS universe, we're often faced with the need to execute a quick conditional check. Sure, we've got the traditional if-else blocks, but sometimes, that's like bringing a bazooka to a knife fight - overkill. Enter the one-line if: a compact way to handle conditions without the fluff.
Of course, if your plan was if/else all along, there may be a better tool: The Ternary Operator. The ternatory operator (so named because it takes three operands), is one of the more intimidating pieces of JavaScript syntax a new coder is likely to encounter. It looks strange and alien, and the way it works is sometimes profoundly unclear.
The conditional (ternary) operator is the only JavaScript operator that takes three operands: a condition followed by a question mark (?), then an expression to execute if the condition is truthy followed by a colon (:), and finally the expression to execute if the condition is falsy. This operator is frequently used as an alternative to an if ...
This guide covers how to create one-line if statements in JavaScript. This guide covers how to create one-line if statements in JavaScript. About. ... The ternary operator is the true one-liner for if-else statements: condition ? exprIfTrue : exprIfFalse; ... Handle assignment within one-line if.
The ternary operator is like that one friend who always gets to the point. It's a one-liner that has three parts: a condition, a result for true, and a result for false. It looks something like this: condition ? exprIfTrue : exprIfFalse; Simple, right? It's an expression, not a statement, which means it returns a value. That's why you can ...
In JavaScript, you can have if-else statements on one line. To write an if-else statement on one line, follow the ternary conditional operator syntax: For example: This is a useful way to compress short and simple if-else statements. It makes the code shorter but preserves the readability. However, do not overuse the ternary operator. Converting […]
statement1. else. statement2. An expression that is considered to be either truthy or falsy. Statement that is executed if condition is truthy. Can be any statement, including further nested if statements. To execute multiple statements, use a block statement ( { /* ... */ }) to group those statements.
The JavaScript if...else statement is used to execute/skip a block of code based on a condition. Here's a quick example of the if...else statement. You can read the rest of the tutorial if you want to learn about if...else in greater detail. Example. let score = 45;
The JavaScript one line if statement is a condensed version of the traditional if-else statement. It is often used when you need to perform a simple conditional check and execute a single line of code based on the result. The basic syntax is as follows:
Conditional logic allows us to control program flow based on dynamic values and user input. It is one of the core building blocks of writing reusable, maintainable code. If you've written any substantial JavaScript, you've surely used standard if/else statements many times. But did you know there are other more compact ways to write conditionals … Mastering JavaScript Inline If ...
Logical AND If Statement. The logical AND operator && can be used to execute a statement if a condition is truth: condition && expressionIfTrue. JavaScript. For example: const user = getUser(); user && greetUser(user); JavaScript. This greets the user only if getUser() returned a valid user object.
The One Liner If Statement ... Apr 16, 2020--Listen. Share. The conditional (ternary) operator is the only JavaScript operator that takes ... (single-line variable assignments and single-line ...
One-Liner If-Else Statements This is a common feature in many programming languages. Instead of writing an if-else on multiple lines, you can use the ternary operator to write the whole statement with one line of code.
The assignment operator is completely different from the equals (=) sign used as syntactic separators in other locations, which include:Initializers of var, let, and const declarations; Default values of destructuring; Default parameters; Initializers of class fields; All these places accept an assignment expression on the right-hand side of the =, so if you have multiple equals signs chained ...
Assignment in a conditional statement is valid in javascript, because your just asking "if assignment is valid, do something which possibly includes the result of the assignment". But indeed, assigning before the conditional is also valid, not too verbose, and more commonly used. - okdewit.
In the latest version of Python (3.12.3), type annotation for single variable assignment is available: a: int = 1 However, in some scenarios like when we want to annotate the tuple of variables in return, the syntax of type annotation is invalid: from typing import Any def fun() -> Any: # when hard to annotate the strict type return 1, True a: int, b: bool = fun() # INVALID In this case, I ...
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.