
- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - Functions
- C - Main Functions
- C - Return Statement
- C - Scope Rules
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointer Arithmetics
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Recursion
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
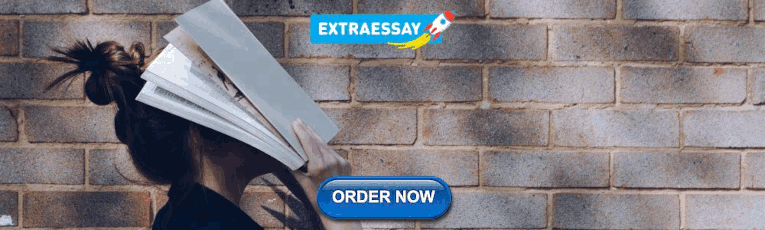
Assignment Operators in C
In C, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable or an expression. The value to be assigned forms the right hand operand, whereas the variable to be assigned should be the operand to the left of = symbol, which is defined as a simple assignment operator in C. In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple assignment operator (=)
The = operator is the most frequently used operator in C. As per ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed. You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented assignment operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression a+=b has the same effect of performing a+b first and then assigning the result back to the variable a.
Similarly, the expression a<<=b has the same effect of performing a<<b first and then assigning the result back to the variable a.
Here is a C program that demonstrates the use of assignment operators in C:
When you compile and execute the above program, it produces the following result −
Home » Learn C Programming from Scratch » C Assignment Operators
C Assignment Operators
Summary : in this tutorial, you’ll learn about the C assignment operators and how to use them effectively.
Introduction to the C assignment operators
An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
After the assignmment, the counter variable holds the number 1.
The following example adds 1 to the counter and assign the result to the counter:
The = assignment operator is called a simple assignment operator. It assigns the value of the left operand to the right operand.
Besides the simple assignment operator, C supports compound assignment operators. A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.
The following example uses a compound-assignment operator (+=):
The expression:
is equivalent to the following expression:
The following table illustrates the compound-assignment operators in C:
- A simple assignment operator assigns the value of the left operand to the right operand.
- A compound assignment operator performs the operation specified by the additional operator and then assigns the result to the left operand.

Assignment Operators In C [ Full Information With Examples ]
![Assignment Operators In C [ Full Information With Examples ] Assignment Operators In C](https://cstutorialpoint.com/wp-content/uploads/2021/04/Assignment-Operators-in-C-.png)
Assignment Operators In C
Assignment operators is a binary operator which is used to assign values in a variable , with its right and left sides being a one-one operand. The operand on the left side is variable in which the value is assigned and the right side operands can contain any of the constant, variable, and expression.
The Assignment operator is a lower priority operator. its priority has much lower than the rest of the other operators. Its priority is more than just the comma operator. The priority of all other operators is more than the assignment operator.
We can assign the same value to multiple variables simultaneously by the assignment operator.
x = y = z = 100
Here x, y, and z are initialized to 100.
In C language, the assignment operator can be divided into two categories.
- Simple assignment operator
- Compound assignment operators
1. Simple Assignment Operator In C
This operator is used to assign left-side values to the right-side operands, simple assignment operators are represented by (=).
2. Compound Assignment Operators In C
Compound Assignment Operators use the old value of a variable to calculate its new value and reassign the value obtained from the calculation to the same variable.
Examples of compound assignment operators are: (Example: + =, – =, * =, / =,% =, & =, ^ =)
Look at these two statements:
Here in this example, adding 5 to the x variable in the second statement is again being assigned to the x variable.
Compound Assignment Operators provide us with the C language to perform such operation even more effecient and in less time.
Syntax of Compound Assignment Operators
Here op can be any arithmetic operators (+, -, *, /,%).
The above statement is equivalent to the following depending on the function:
Let us now know about some important compound assignment operators one by one.
“+ =” -: This operator adds the right operand to the left operand and assigns the output to the left operand.
“- =” -: This operator subtracts the right operand from the left operand and returns the result to the left operand.
“* =” -: This operator multiplies the right operand with the left operand and assigns the result to the left operand.
“/ =” -: This operator splits the left operand with the right operand and assigns the result to the left operand.
“% =” -: This operator takes the modulus using two operands and assigns the result to the left operand.
There are many other assignment operators such as left shift and (<< =) operator, right shift and operator (>> =), bitwise and assignment operator (& =), bitwise OR assignment operator (^ =)
List of Assignment Operators In C
Read More -:
- What is Operators In C
- Relational Operators In C
- Logical Operators In C
- Bitwise Operators In C
- Arithmetic Operators In C
- Conditional Operator in C
- Download C Language Notes Pdf
- C Language Tutorial For Beginners
- C Programming Examples With Output
- 250+ C Programs for Practice PDF Free Download
Friends, I hope you have found the answer of your question and you will not have to search about the Assignment operators in C Language
However, if you want any information related to this post or related to programming language, computer science, then comment below I will clear your all doubts.
If you want a complete tutorial of C language, then see here C Language Tutorial . Here you will get all the topics of C Programming Tutorial step by step.
Friends, if you liked this post, then definitely share this post with your friends so that they can get information about the Assignment operators in C Language
To get the information related to Programming Language, Coding, C, C ++, subscribe to our website newsletter. So that you will get information about our upcoming new posts soon.
Jeetu Sahu is A Web Developer | Computer Engineer | Passionate about Coding, Competitive Programming, and Blogging
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Assignment Operators
- 6 contributors
An assignment operation assigns the value of the right-hand operand to the storage location named by the left-hand operand. Therefore, the left-hand operand of an assignment operation must be a modifiable l-value. After the assignment, an assignment expression has the value of the left operand but isn't an l-value.
assignment-expression : conditional-expression unary-expression assignment-operator assignment-expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators:
In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place. The left operand must not be an array, a function, or a constant. The specific conversion path, which depends on the two types, is outlined in detail in Type Conversions .
- Assignment Operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Assignment Operators in C
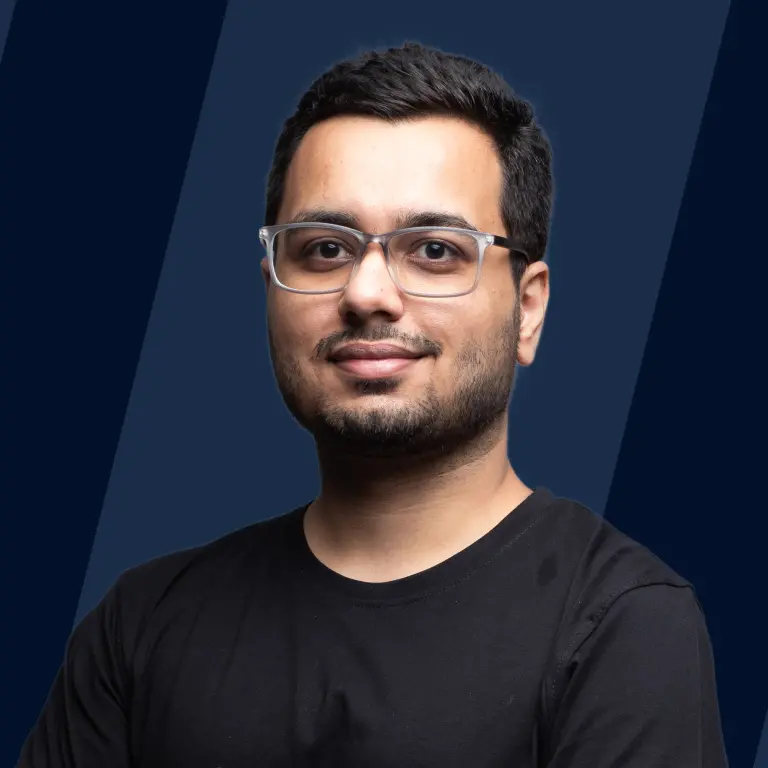
Operators are a fundamental part of all the computations that computers perform. Today we will learn about one of them known as Assignment Operators in C. Assignment Operators are used to assign values to variables. The most common assignment operator is = . Assignment Operators are Binary Operators.
Types of Assignment Operators in C
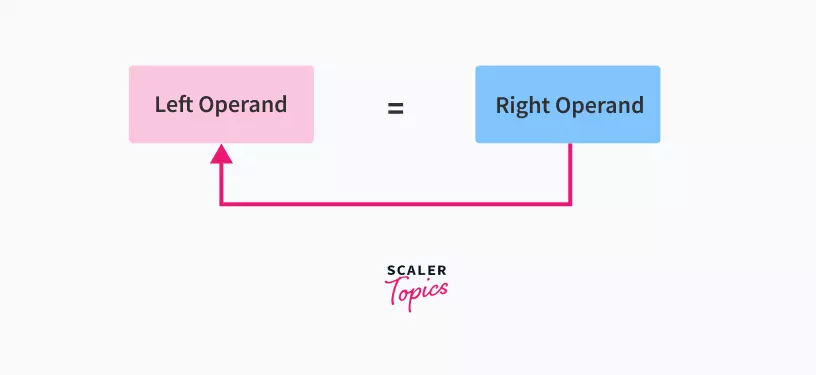
Here is a list of the assignment operators that you can find in the C language:
- basic assignment ( = )
- subtraction assignment ( -= )
- addition assignment ( += )
- division assignment ( /= )
- multiplication assignment ( *= )
- modulo assignment ( %= )
- bitwise XOR assignment ( ^= )
- bitwise OR assignment ( |= )
- bitwise AND assignment ( &= )
- bitwise right shift assignment ( >>= )
- bitwise left shift assignment ( <<= )
Working of Assignment Operators in C
This is the complete list of all assignment operators in C. To read the meaning of operator please keep in mind the above example.
Example for Assignment Operators in C
Basic assignment ( = ) :
Subtraction assignment ( -= ) :
Addition assignment ( += ) :
Division assignment ( /= ) :
Multiplication assignment ( *= ) :
Modulo assignment ( %= ) :
Bitwise XOR assignment ( ^= ) :
Bitwise OR assignment ( |= ) :
Bitwise AND assignment ( &= ) :
Bitwise right shift assignment ( >>= ) :
Bitwise left shift assignment ( <<= ) :
This is the detailed explanation of all the assignment operators in C that we have. Hopefully, This is clear to you.
Practice Problems on Assignment Operators in C
1. what will be the value of a after the following code is executed.
A) 10 B) 11 C) 12 D) 15
Answer – C. 12 Explanation: a starts at 10, increases by 5 to 15, then decreases by 3 to 12. So, a is 12.
2. After executing the following code, what is the value of num ?
A) 4 B) 8 C) 16 D) 32
Answer: C) 16 Explanation: After right-shifting 8 (binary 1000) by one and then left-shifting the result by two, the value becomes 16 (binary 10000).
Q. How does the /= operator function? Is it a combination of two other operators?
A. The /= operator is a compound assignment operator in C++. It divides the left operand by the right operand and assigns the result to the left operand. It is equivalent to using the / operator and then the = operator separately.
Q. What is the most basic operator among all the assignment operators available in the C language?
A. The most basic assignment operator in the C language is the simple = operator, which is used for assigning a value to a variable.
- Assignment operators are used to assign the result of an expression to a variable.
- There are two types of assignment operators in C. Simple assignment operator and compound assignment operator.
- Compound Assignment operators are easy to use and the left operand of expression needs not to write again and again.
- They work the same way in C++ as in C.
C Programming Tutorial
- Assignment Operator in C
Last updated on July 27, 2020
We have already used the assignment operator ( = ) several times before. Let's discuss it here in detail. The assignment operator ( = ) is used to assign a value to the variable. Its general format is as follows:
The operand on the left side of the assignment operator must be a variable and operand on the right-hand side must be a constant, variable or expression. Here are some examples:
The precedence of the assignment operator is lower than all the operators we have discussed so far and it associates from right to left.
We can also assign the same value to multiple variables at once.
here x , y and z are initialized to 100 .
Since the associativity of the assignment operator ( = ) is from right to left. The above expression is equivalent to the following:
Note that expressions like:
are called assignment expression. If we put a semicolon( ; ) at the end of the expression like this:
then the assignment expression becomes assignment statement.
Compound Assignment Operator #
Assignment operations that use the old value of a variable to compute its new value are called Compound Assignment.
Consider the following two statements:
Here the second statement adds 5 to the existing value of x . This value is then assigned back to x . Now, the new value of x is 105 .
To handle such operations more succinctly, C provides a special operator called Compound Assignment operator.
The general format of compound assignment operator is as follows:
where op can be any of the arithmetic operators ( + , - , * , / , % ). The above statement is functionally equivalent to the following:
Note : In addition to arithmetic operators, op can also be >> (right shift), << (left shift), | (Bitwise OR), & (Bitwise AND), ^ (Bitwise XOR). We haven't discussed these operators yet.
After evaluating the expression, the op operator is then applied to the result of the expression and the current value of the variable (on the RHS). The result of this operation is then assigned back to the variable (on the LHS). Let's take some examples: The statement:
is equivalent to x = x + 5; or x = x + (5); .
Similarly, the statement:
is equivalent to x = x * 2; or x = x * (2); .
Since, expression on the right side of op operator is evaluated first, the statement:
is equivalent to x = x * (y + 1) .
The precedence of compound assignment operators are same and they associate from right to left (see the precedence table ).
The following table lists some Compound assignment operators:
The following program demonstrates Compound assignment operators in action:
Expected Output:
Load Comments
- Intro to C Programming
- Installing Code Blocks
- Creating and Running The First C Program
- Basic Elements of a C Program
- Keywords and Identifiers
- Data Types in C
- Constants in C
- Variables in C
- Input and Output in C
- Formatted Input and Output in C
- Arithmetic Operators in C
- Operator Precedence and Associativity in C
- Increment and Decrement Operators in C
- Relational Operators in C
- Logical Operators in C
- Conditional Operator, Comma operator and sizeof() operator in C
- Implicit Type Conversion in C
- Explicit Type Conversion in C
- if-else statements in C
- The while loop in C
- The do while loop in C
- The for loop in C
- The Infinite Loop in C
- The break and continue statement in C
- The Switch statement in C
- Function basics in C
- The return statement in C
- Actual and Formal arguments in C
- Local, Global and Static variables in C
- Recursive Function in C
- One dimensional Array in C
- One Dimensional Array and Function in C
- Two Dimensional Array in C
- Pointer Basics in C
- Pointer Arithmetic in C
- Pointers and 1-D arrays
- Pointers and 2-D arrays
- Call by Value and Call by Reference in C
- Returning more than one value from function in C
- Returning a Pointer from a Function in C
- Passing 1-D Array to a Function in C
- Passing 2-D Array to a Function in C
- Array of Pointers in C
- Void Pointers in C
- The malloc() Function in C
- The calloc() Function in C
- The realloc() Function in C
- String Basics in C
- The strlen() Function in C
- The strcmp() Function in C
- The strcpy() Function in C
- The strcat() Function in C
- Character Array and Character Pointer in C
- Array of Strings in C
- Array of Pointers to Strings in C
- The sprintf() Function in C
- The sscanf() Function in C
- Structure Basics in C
- Array of Structures in C
- Array as Member of Structure in C
- Nested Structures in C
- Pointer to a Structure in C
- Pointers as Structure Member in C
- Structures and Functions in C
- Union Basics in C
- typedef statement in C
- Basics of File Handling in C
- fputc() Function in C
- fgetc() Function in C
- fputs() Function in C
- fgets() Function in C
- fprintf() Function in C
- fscanf() Function in C
- fwrite() Function in C
- fread() Function in C
Recent Posts
- Machine Learning Experts You Should Be Following Online
- 4 Ways to Prepare for the AP Computer Science A Exam
- Finance Assignment Online Help for the Busy and Tired Students: Get Help from Experts
- Top 9 Machine Learning Algorithms for Data Scientists
- Data Science Learning Path or Steps to become a data scientist Final
- Enable Edit Button in Shutter In Linux Mint 19 and Ubuntu 18.04
- Python 3 time module
- Pygments Tutorial
- How to use Virtualenv?
- Installing MySQL (Windows, Linux and Mac)
- What is if __name__ == '__main__' in Python ?
- Installing GoAccess (A Real-time web log analyzer)
- Installing Isso
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
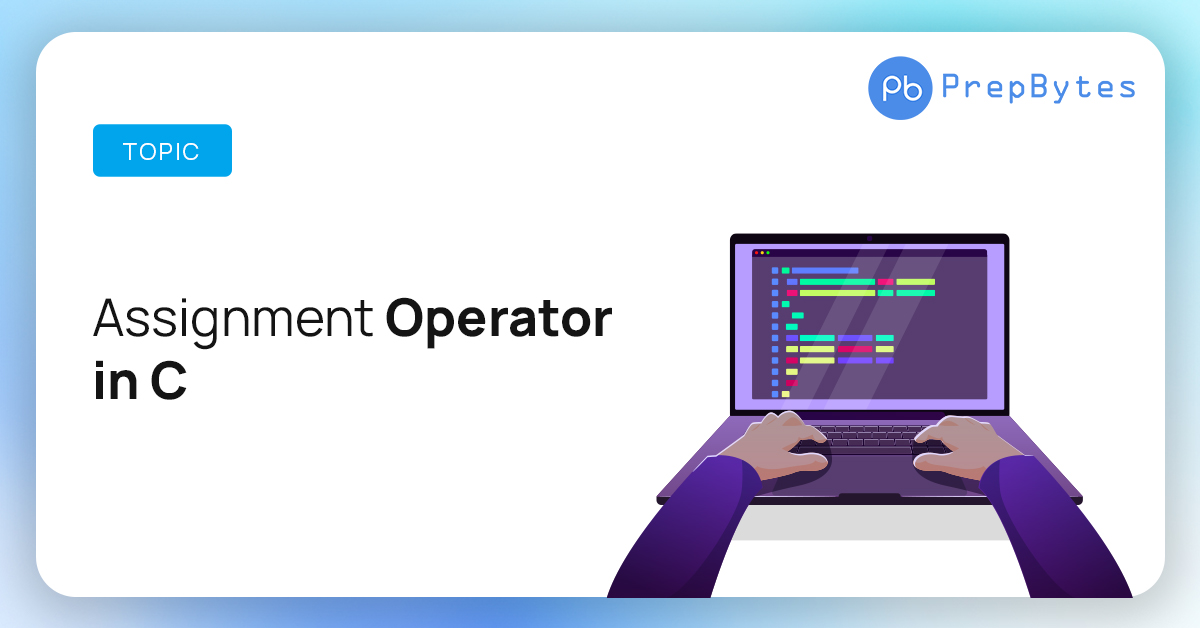
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
C Functions
C structures, c operators.
Operators are used to perform operations on variables and values.
In the example below, we use the + operator to add together two values:
Although the + operator is often used to add together two values, like in the example above, it can also be used to add together a variable and a value, or a variable and another variable:
C divides the operators into the following groups:
- Arithmetic operators
- Assignment operators
- Comparison operators
- Logical operators
- Bitwise operators
Arithmetic Operators
Arithmetic operators are used to perform common mathematical operations.
Assignment Operators
Assignment operators are used to assign values to variables.
In the example below, we use the assignment operator ( = ) to assign the value 10 to a variable called x :
The addition assignment operator ( += ) adds a value to a variable:
A list of all assignment operators:
Comparison Operators
Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions.
The return value of a comparison is either 1 or 0 , which means true ( 1 ) or false ( 0 ). These values are known as Boolean values , and you will learn more about them in the Booleans and If..Else chapter.
Comparison operators are used to compare two values.
Note: The return value of a comparison is either true ( 1 ) or false ( 0 ).
In the following example, we use the greater than operator ( > ) to find out if 5 is greater than 3:
A list of all comparison operators:
Logical Operators
You can also test for true or false values with logical operators.
Logical operators are used to determine the logic between variables or values:
C Exercises
Test yourself with exercises.
Fill in the blanks to multiply 10 with 5 , and print the result:
Start the Exercise

COLOR PICKER

Report Error
If you want to report an error, or if you want to make a suggestion, do not hesitate to send us an e-mail:
Top Tutorials
Top references, top examples, get certified.
- C++ Tutorial
- Java Tutorial
- Python Tutorial
- HTML Tutorial
- CSS Tutorial
- C Introduction
- C Data Types and Modifiers
- C Naming Conventions
- C Integer Variable
- C Float and Double Variables
- C Character Variable
- C Constant Variable
- C Typecasting
- C Operators
- C Assignment Operators
- C Arithmetic Operators
- C Relational Operators
- C Logical Operators
- C Operator Precedence
- C Environment Setup
- C Program Basic Structure
- C printf() Function
- C scanf() Function
- C Decision Making
- C if Statement
- C if else Statement
- C if else if Statement
- C Conditional Operator
- C switch case Statement
- C Mathematical Functions
- C while Loop
- C do while Loop
- C break and continue
- C One Dimensional Array
- C Two Dimensional Array
- C String Methods
- C Bubble Sort
- C Selection Sort
- C Insertion Sort
- C Linear Search
- C Binary Search
- C Dynamic Memory Allocation
- C Structure
- C Recursive Function
- C Circular Queue
- C Double Ended Queue
- C Singly Linked List
- C Doubly Linked List
- C Stack using Linked List
- C Queue using Linked List
- C Text File Handling
- C Binary File Handling
Assignment Operators in C Programming
Operators in c.
In this lesson, we are going to look at what is assignment operator and will learn the different types of assignment operator with the help of examples.
What is Assignment Operator
Assignment Operator in C is used for assigning value to a variable. Using the assignment operator we can assign the value which is on the right side of the operator to the variable on the left side of the operator.
Now let's see the examples for more understanding.
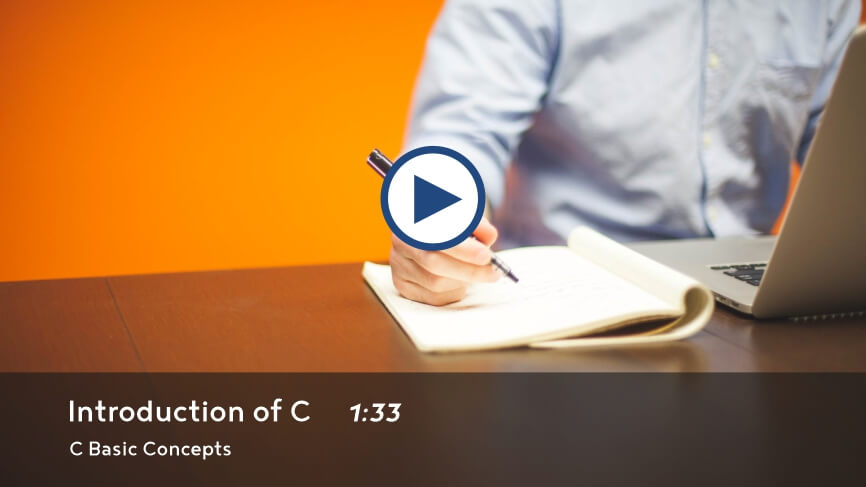
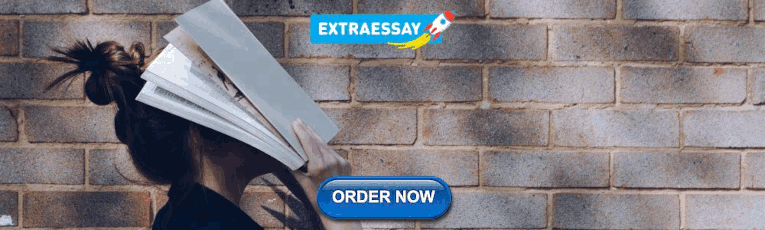
Example 1 (Assign Operator =)
The = operator is used to assign the value from right to left. As you can see the value 5 which is on the right side of the operator = has been assigned to the left in the variable a . In the same way value of the variable a (5) which is on the right side of the operator = has been assigned to the left in the variable b
Note: The lines started with // are called comments which are used to provide information about lines of code. We will learn more about comments in our upcoming lessons.
Example 2 (Add and Assign Operator +=)
The += operator is a combination of + and = operators. This operator first adds the current value of the variable on right to the value on the left and then assigns the result to the variable on the left.
Example 3 (Subtract and Assign Operator -=)
The -= operator is a combination of - and = operators. This operator first subtract the current value of the variable on right from the value on the left and then assigns the result to the variable on the left.
Example 4 (Multiply and Assign Operator *=)
The *= operator is a combination of * and = operators. This operator first multiplies the current value of the variable on left with the value on the right and then assigns the result to the variable on the left.
Example 5 (Divide and Assign Operator /=)
The /= operator is a combination of / and = operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result ( quotient ) to the variable on the left.
Example 6 (Modulus and Assign Operator %=)
The %= operator is a combination of % and = operators. This operator first divides the current value of the variable on left by the value on the right and then assigns the result ( remainder ) to the variable on the left.
Test Your Knowledge
Attempt the multiple choice quiz to check if the lesson is adequately clear to you.
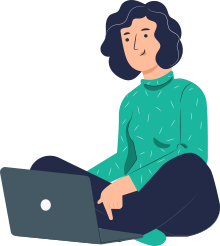
For over a decade, Dremendo has been recognized for providing quality education. We proudly introduce our newly open online learning platform, which offers free access to popular computer courses.
Our Courses
News updates.
- Refund and Cancellation
- Privacy Policy
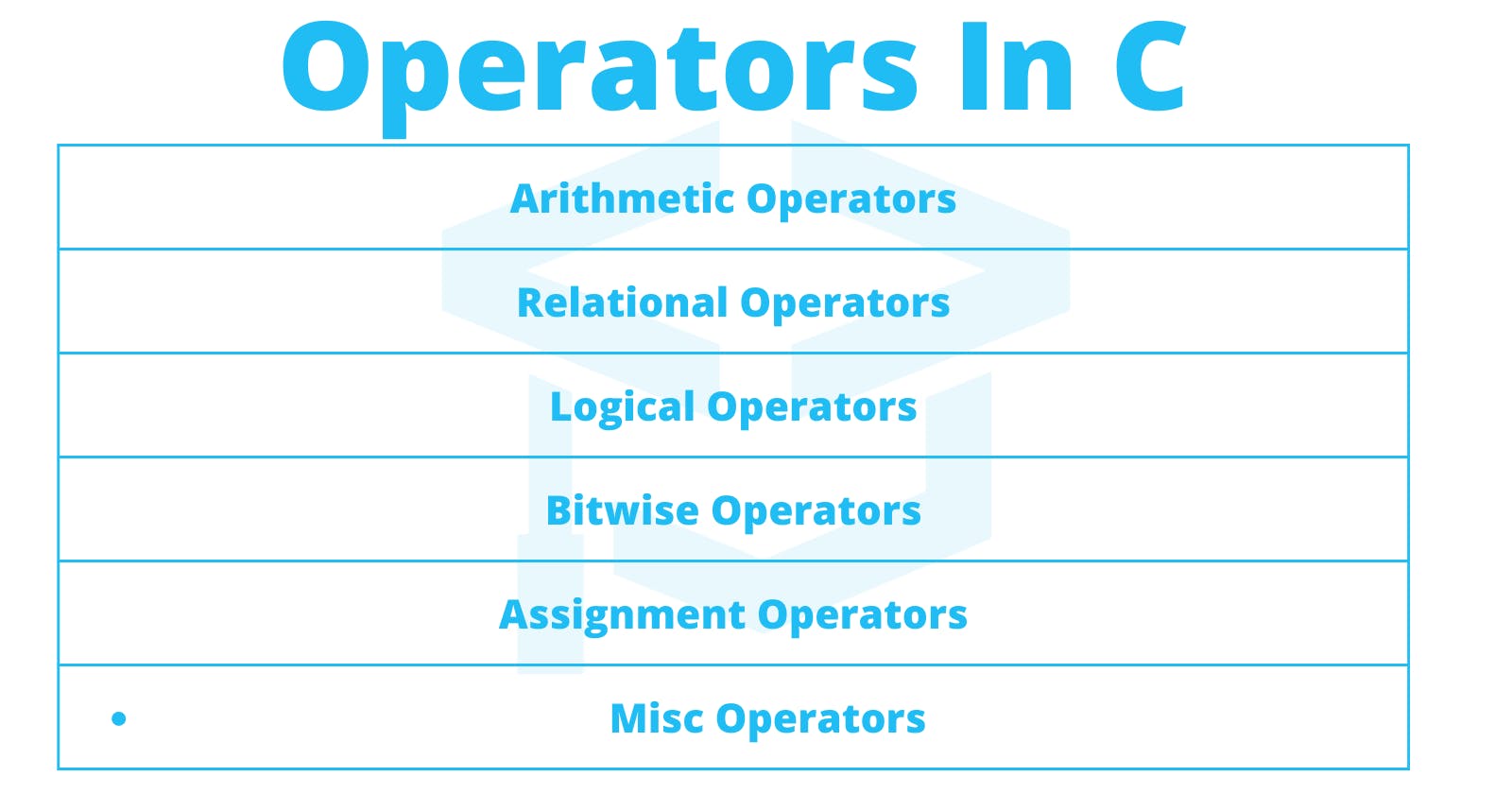
Operators in C Programming
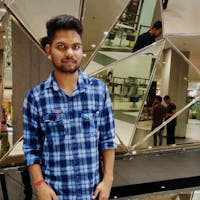
Table of contents
What is operator, sample code, sample code.
An Operator in computer programming is a symbol that helps us to perform mathematical and logical operations. We have 6 types of operators in C
Arithmetic Operators
Relational operators, logical operators, bitwise operators, assignment operators, misc operators, video explaination.
Now we will learn every type of operator in detail. Let's get started :
As the name suggests Arithmetic Operators helps us to perform arithmetic operations in c Programming. Look at the table to get more ideas about Arithmetic Operators.
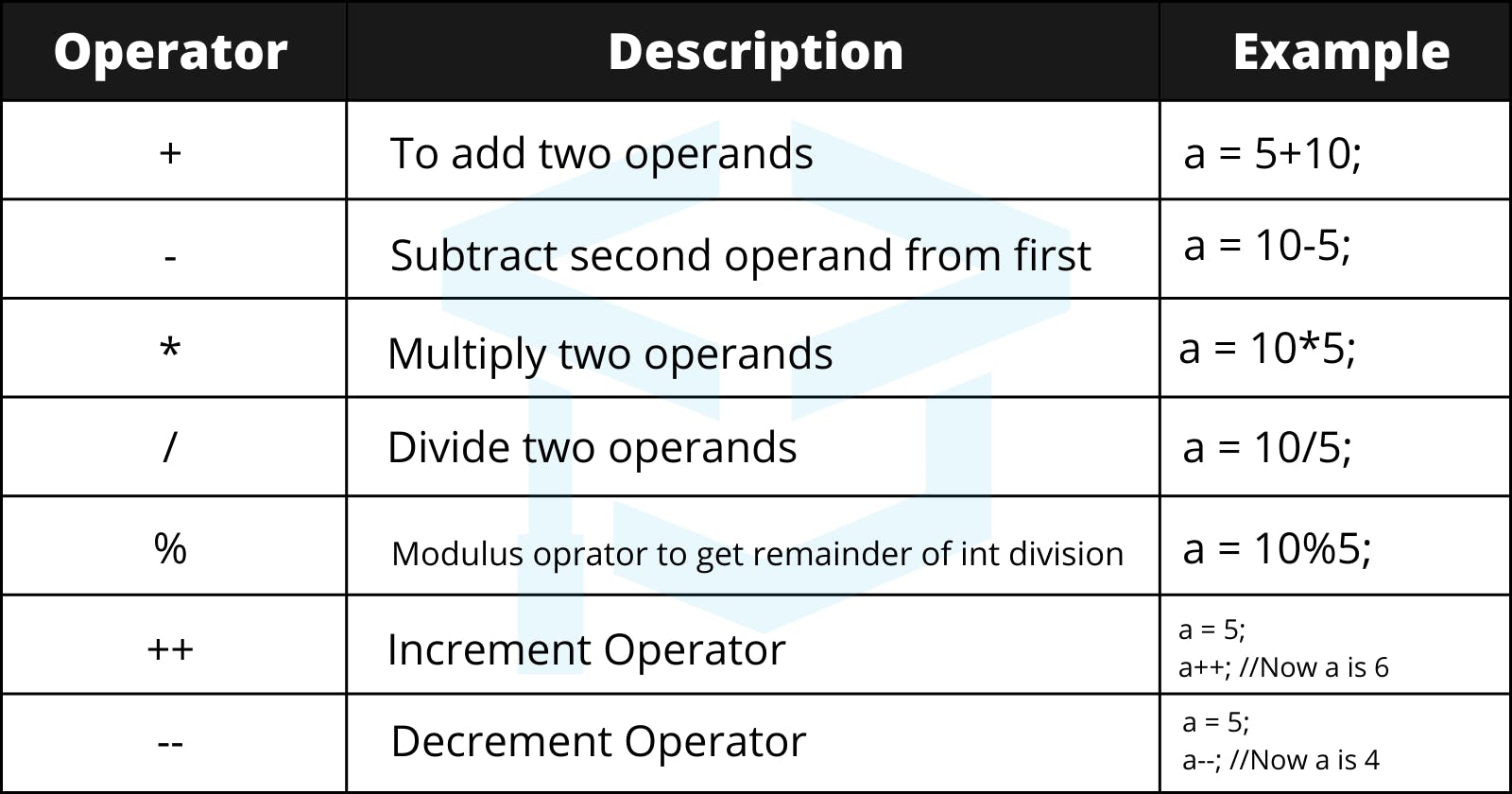
Relational Operators helps us to figure out certain relations between two operands. It returns true or false based on whether the condition is true or false. And in the C language true and false are represented by 1 and 0 respectively. Let's understand how to work with Relational Operators using the table below.
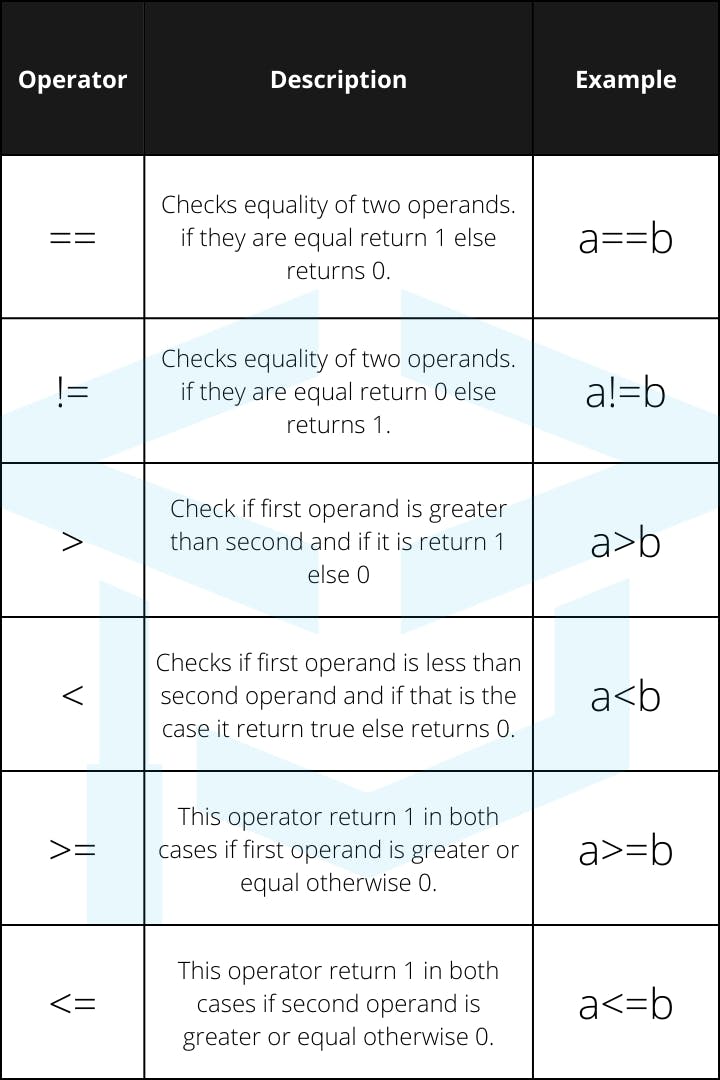
Logical Operators are used to checking the and , or and not conditions between two statements or operands. Let's look at the table.

As the name suggests bitwise operator returns the value by comparing two operands bit by bit after converting it to binary.
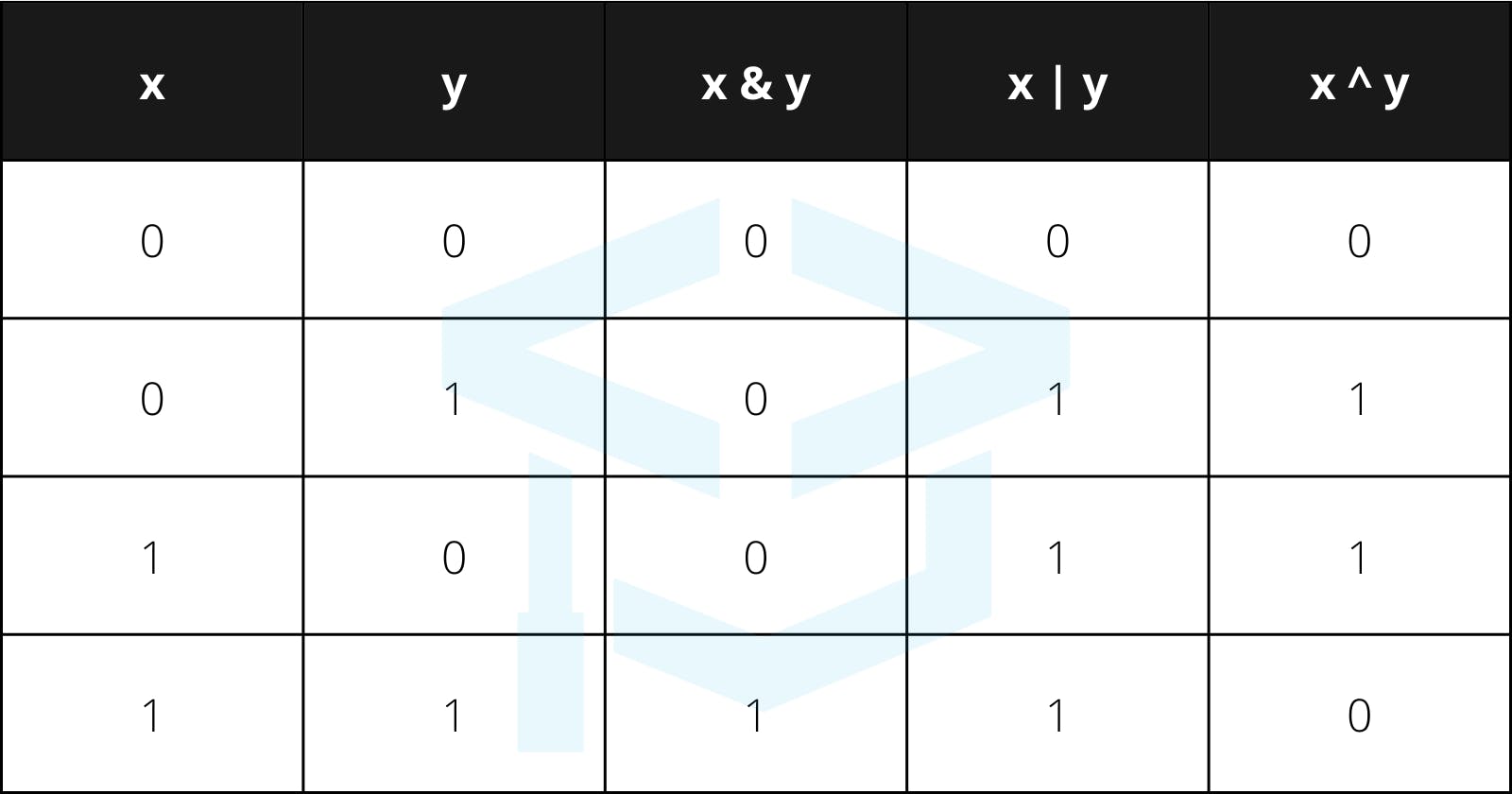
Let's understand with an example suppose a = 5, and b = 7; In binary A = 101 // 5 In binary B = 111 // 7 in binary Let's calculate A & B
Starting from right in A we have 1 and in b we have 1 it will return 1. Second place A it's 0 B it's 1 hence 0. Now third and last place it's 1 in A and 1 in B so the result will be 1. Finally, we have our result as 101 which again in decimal would be 5.
So A & B will result in 5.
There are some more bitwise operators but we don't use them usually. These are One's Complement Operator ~ , Binary Left Shift Operator << and Binary Right Shift Operator >> .
Simple Assignment Operators is = which is used to assign values to variables in a programming language. for example. a = 3 . We are assigning value to 3 to variable a. but assignment variable can also be used with Arithmetic and Bitwise operators let's look at the example.
There are also some miscellaneous operators in C.
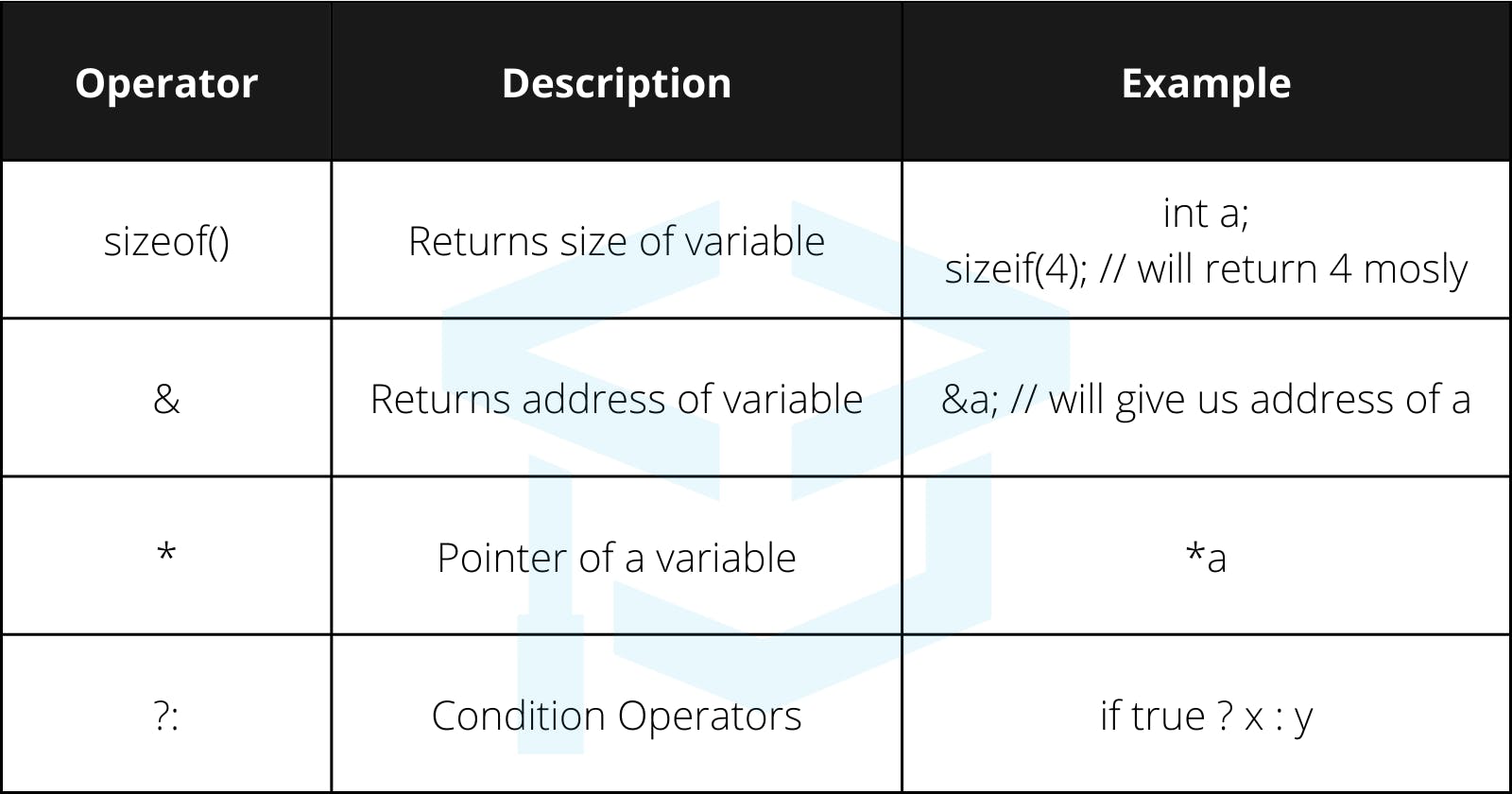
These are all the operators we can use in c to write programs. I hope I have cleared all the doubts related to operators in C. If you still have any doubts regarding operators or this article just comment down. And do checkout my youtube channel.
Did you find this article valuable?
Support Rishabh Kumar by becoming a sponsor. Any amount is appreciated!
- C - Introduction
- C - Comments
- C - Data Types
- C - Type Casting
- C - Operators
- C - Strings
- C - Booleans
- C - If Else
- C - While Loop
- C - For Loop
- C - goto Statement
- C - Continue Statement
- C - Break Statement
- C - Functions
- C - Scope of Variables
- C - Pointers
- C - Typedef
- C - Format Specifiers
- C Standard Library
- C - Data Structures
- C - Examples
- C - Interview Questions
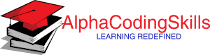
- Programming Languages
- Web Technologies
- Database Technologies
- Microsoft Technologies
- Python Libraries
- Data Structures
- Interview Questions
- PHP & MySQL
- C++ Standard Library
- Java Utility Library
- Java Default Package
- PHP Function Reference
C - Bitwise OR and assignment operator
The Bitwise OR and assignment operator (|=) assigns the first operand a value equal to the result of Bitwise OR operation of two operands.
(x |= y) is equivalent to (x = x | y)
The Bitwise OR operator (|) is a binary operator which takes two bit patterns of equal length and performs the logical OR operation on each pair of corresponding bits. It returns 1 if either or both bits at the same position are 1, else returns 0.
The example below describes how bitwise OR operator works:
The code of using Bitwise OR operator (|) is given below:
The output of the above code will be:
Example: Find largest power of 2 less than or equal to given number
Consider an integer 1000. In the bit-wise format, it can be written as 1111101000. However, all bits are not written here. A complete representation will be 32 bit representation as given below:
Performing N |= (N>>i) operation, where i = 1, 2, 4, 8, 16 will change all right side bit to 1. When applied on 1000, the result in 32 bit representation is given below:
Adding one to this result and then right shifting the result by one place will give largest power of 2 less than or equal to 1000.
The below code will calculate the largest power of 2 less than or equal to given number.
The above code will give the following output:
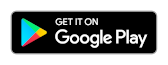
- Data Structures Tutorial
- Algorithms Tutorial
- JavaScript Tutorial
- Python Tutorial
- MySQLi Tutorial
- Java Tutorial
- Scala Tutorial
- C++ Tutorial
- C# Tutorial
- PHP Tutorial
- MySQL Tutorial
- SQL Tutorial
- PHP Function reference
- C++ - Standard Library
- Java.lang Package
- Ruby Tutorial
- Rust Tutorial
- Swift Tutorial
- Perl Tutorial
- HTML Tutorial
- CSS Tutorial
- AJAX Tutorial
- XML Tutorial
- Online Compilers
- QuickTables
- NumPy Tutorial
- Pandas Tutorial
- Matplotlib Tutorial
- SciPy Tutorial
- Seaborn Tutorial
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, c introduction.
- Keywords & Identifier
- Variables & Constants
- C Data Types
- C Input/Output
- C Operators
- C Introduction Examples
C Flow Control
- C if...else
- C while Loop
- C break and continue
- C switch...case
- C Programming goto
- Control Flow Examples
C Functions
- C Programming Functions
- C User-defined Functions
- C Function Types
- C Recursion
- C Storage Class
- C Function Examples
- C Programming Arrays
- C Multi-dimensional Arrays
- C Arrays & Function
- C Programming Pointers
- C Pointers & Arrays
- C Pointers And Functions
- C Memory Allocation
- Array & Pointer Examples
C Programming Strings
- C Programming String
- C String Functions
- C String Examples
Structure And Union
- C Structure
- C Struct & Pointers
- C Struct & Function
- C struct Examples
C Programming Files
- C Files Input/Output
- C Files Examples
Additional Topics
- C Enumeration
- C Preprocessors
- C Standard Library
- C Programming Examples
- Check Whether a Number is Even or Odd
- Make a Simple Calculator Using switch...case
C Programming Operators
C switch Statement
C if...else Statement
- Find LCM of two Numbers
C Ternary Operator
We use the ternary operator in C to run one code when the condition is true and another code when the condition is false. For example,
Here, when the age is greater than or equal to 18 , Can Vote is printed. Otherwise, Cannot Vote is printed.
Syntax of Ternary Operator
The syntax of ternary operator is :
The testCondition is a boolean expression that results in either true or false . If the condition is
- true - expression1 (before the colon) is executed
- false - expression2 (after the colon) is executed
The ternary operator takes 3 operands (condition, expression1 and expression2) . Hence, the name ternary operator .
Example: C Ternary Operator
In the above example, we have used a ternary operator that checks whether a user can vote or not based on the input value. Here,
- age >= 18 - test condition that checks if input value is greater or equal to 18
- printf("You can vote") - expression1 that is executed if condition is true
- printf("You cannot vote") - expression2 that is executed if condition is false
Here, the user inputs 12 , so the condition becomes false . Hence, we get You cannot vote as output.
This time the input value is 24 which is greater than 18 . Hence, we get You can vote as output.
Assign the ternary operator to a variable
In C programming, we can also assign the expression of the ternary operator to a variable. For example,
Here, if the test condition is true , expression1 will be assigned to the variable. Otherwise, expression2 will be assigned.
Let's see an example
In the above example, the test condition (operator == '+') will always be true . So, the first expression before the colon i.e the summation of two integers num1 and num2 is assigned to the result variable.
And, finally the result variable is printed as an output giving out the summation of 8 and 7 . i.e 15 .
Ternary Operator Vs. if...else Statement in C
In some of the cases, we can replace the if...else statement with a ternary operator. This will make our code cleaner and shorter.
Let's see an example:
We can replace this code with the following code using the ternary operator.
Here, both the programs are doing the same task, checking even/odd numbers. However, the code using the ternary operator looks clean and concise.
In such cases, where there is only one statement inside the if...else block, we can replace it with a ternary operator .
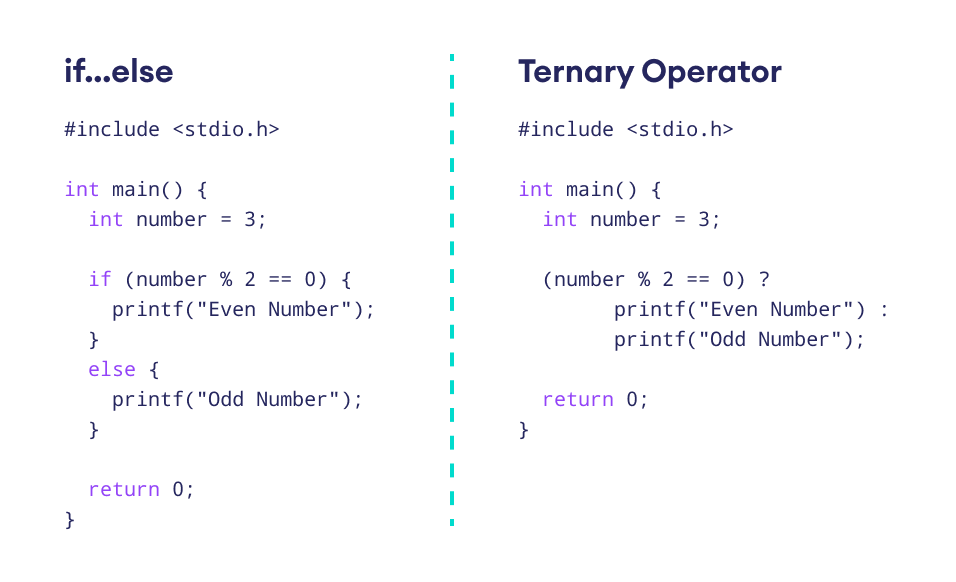
Table of Contents
- Introduction
- Ternary Operator
- Example: Ternary Operator
- Ternary Operator with Variable
- Ternary Operator Vs. if...else
Sorry about that.
Related Tutorials
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Bitmask in C++
- C++ Variable Templates
- vTable And vPtr in C++
- Address Operator & in C
- Macros In C++
- Variable Shadowing in C++
- Unique_ptr in C++
- Pass By Reference In C
- C++ Program For Sentinel Linear Search
- Partial Template Specialization in C++
- Difference Between Constant and Literals
- Concurrency in C++
- String Tokenization in C
- Decision Making in C++
- auto_ptr in C++
- shared_ptr in C++
- Mutex in C++
- C++ 20 - <semaphore> Header
- Compound Statements in C++
Assignment Operators In C++
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other words, it is used to store some kind of information.
The right-hand side value will be assigned to the variable on the left-hand side. The variable and the value should be of the same data type.
The value can be a literal or another variable of the same data type.
Compound Assignment Operators
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++:
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
- Bitwise AND Assignment Operator ( &= )
- Bitwise OR Assignment Operator ( |= )
- Bitwise XOR Assignment Operator ( ^= )
- Left Shift Assignment Operator ( <<= )
- Right Shift Assignment Operator ( >>= )
Lets see each of them in detail.
1. Addition Assignment Operator (+=)
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way.
This above expression is equivalent to the expression:
2. Subtraction Assignment Operator (-=)
The subtraction assignment operator (-=) in C++ enables you to update the value of the variable by subtracting another value from it. This operator is especially useful when you need to perform subtraction and store the result back in the same variable.
3. Multiplication Assignment Operator (*=)
In C++, the multiplication assignment operator (*=) is used to update the value of the variable by multiplying it with another value.
4. Division Assignment Operator (/=)
The division assignment operator divides the variable on the left by the value on the right and assigns the result to the variable on the left.
5. Modulus Assignment Operator (%=)
The modulus assignment operator calculates the remainder when the variable on the left is divided by the value or variable on the right and assigns the result to the variable on the left.
6. Bitwise AND Assignment Operator (&=)
This operator performs a bitwise AND between the variable on the left and the value on the right and assigns the result to the variable on the left.
7. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator performs a bitwise OR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
8. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator performs a bitwise XOR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
9. Left Shift Assignment Operator (<<=)
The left shift assignment operator shifts the bits of the variable on the left to left by the number of positions specified on the right and assigns the result to the variable on the left.
10. Right Shift Assignment Operator (>>=)
The right shift assignment operator shifts the bits of the variable on the left to the right by a number of positions specified on the right and assigns the result to the variable on the left.
Also, it is important to note that all of the above operators can be overloaded for custom operations with user-defined data types to perform the operations we want.
Please Login to comment...
- Geeks Premier League 2023
- Geeks Premier League
- How to Delete Whatsapp Business Account?
- Discord vs Zoom: Select The Efficienct One for Virtual Meetings?
- Otter AI vs Dragon Speech Recognition: Which is the best AI Transcription Tool?
- Google Messages To Let You Send Multiple Photos
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
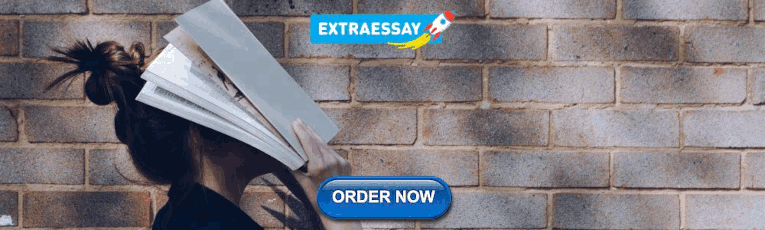
IMAGES
VIDEO
COMMENTS
Different types of assignment operators are shown below: 1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: 2. "+=": This operator is combination of '+' and '=' operators.
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
The Assignment operators in C are some of the Programming operators that are useful for assigning the values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: int i = 10; The below table displays all the assignment operators present in C Programming with an example. C Assignment Operators.
The += assignment operator is a combination of + arithmetic operator and = simple assignment operator. For example, x += y; is equivalent to x = x+y;. It adds the right side value to the value of left side operand and assign the result back to the left-hand side operand. #include <stdio.h> int main () { int x = 100, y = 20, z = 50 ...
An operator is a symbol that operates on a value or a variable. For example: + is an operator to perform addition. In this tutorial, you will learn about different C operators such as arithmetic, increment, assignment, relational, logical, etc. with the help of examples.
Summary: in this tutorial, you'll learn about the C assignment operators and how to use them effectively.. Introduction to the C assignment operators. An assignment operator assigns the vale of the right-hand operand to the left-hand operand. The following example uses the assignment operator (=) to assign 1 to the counter variable:
Assignment Operators In C. Assignment operators is a binary operator which is used to assign values in a variable, with its right and left sides being a one-one operand. The operand on the left side is variable in which the value is assigned and the right side operands can contain any of the constant, variable, and expression. Example -: x = 18 ...
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: | =. In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place.
A. The most basic assignment operator in the C language is the simple = operator, which is used for assigning a value to a variable. Conclusion. Assignment operators are used to assign the result of an expression to a variable. There are two types of assignment operators in C. Simple assignment operator and compound assignment operator.
The assignment operator ( = ) is used to assign a value to the variable. Its general format is as follows: variable = right_side. The operand on the left side of the assignment operator must be a variable and operand on the right-hand side must be a constant, variable or expression. Here are some examples:
Example of Assignment Operator in C. For example, consider the following line of code: int a = 10 Types of Assignment Operators in C. Here is a list of the assignment operators that you can find in the C language: Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the ...
Comparison operators are used to compare two values (or variables). This is important in programming, because it helps us to find answers and make decisions. The return value of a comparison is either 1 or 0, which means true ( 1) or false ( 0 ). These values are known as Boolean values, and you will learn more about them in the Booleans and If ...
Example 4 (Multiply and Assign Operator *=) int a=7, b=10, c=5; a*=2; // can be written as a=a*2 and result is 14. b*=c; // can be written as b=b*c and result is 50. The *= operator is a combination of * and = operators. This operator first multiplies the current value of the variable on left with the value on the right and then assigns the ...
Simple Assignment Operators is = which is used to assign values to variables in a programming language. for example. a = 3. We are assigning value to 3 to variable a. but assignment variable can also be used with Arithmetic and Bitwise operators let's look at the example.
Using assignment operators. - [Instructor] In C programming, a variable can be modified by itself. The format for self-modifying a variable is shown in this code. Variable a is declared and ...
Operators are symbols used for performing some kind of operation in C. There are six types of operators, Arithmetic Operators, Relational Operators, Logical Operators, Bitwise Operators, Assignment Operators, and Miscellaneous Operators. Operators can also be of type unary, binary, and ternary according to the number of operators they are using.
The precedence of operators determines which operator is executed first if there is more than one operator in an expression. Let us consider an example: int x = 5 - 17* 6; In C, the precedence of * is higher than - and =. Hence, 17 * 6 is evaluated first. Then the expression involving - is evaluated as the precedence of - is higher than that of =.
The Bitwise OR and assignment operator (|=) assigns the first operand a value equal to the result of Bitwise OR operation of two operands. The Bitwise OR operator (|) is a binary operator which takes two bit patterns of equal length and performs the logical OR operation on each pair of corresponding bits. It returns 1 if either or both bits at ...
In this video, learn Assignment Operator in C Programming | C Programming Tutorial. Find all the videos of the C Programming Complete Course in this playlis...
Syntax of Ternary Operator. The syntax of ternary operator is : testCondition ? expression1 : expression 2; The testCondition is a boolean expression that results in either true or false.If the condition is . true - expression1 (before the colon) is executed; false - expression2 (after the colon) is executed; The ternary operator takes 3 operands (condition, expression1 and expression2).
You may also use multiple operators in one expression. In these cases, C++ has established operator precedence to determine how things get evaluated. For example, postfix operators work left to right. So do additive, multiplicative, and shift operators. Unary, conditional, and assignment operators have precedence from right to left.
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.