If you're seeing this message, it means we're having trouble loading external resources on our website.
If you're behind a web filter, please make sure that the domains *.kastatic.org and *.kasandbox.org are unblocked.
To log in and use all the features of Khan Academy, please enable JavaScript in your browser.
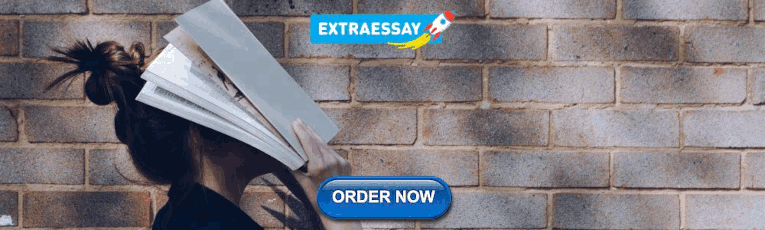
Unit 1: Algorithms
About this unit.
We've partnered with Dartmouth college professors Tom Cormen and Devin Balkcom to teach introductory computer science algorithms, including searching, sorting, recursion, and graph theory. Learn with a combination of articles, visualizations, quizzes, and coding challenges.
Intro to algorithms
- What is an algorithm and why should you care? (Opens a modal)
- A guessing game (Opens a modal)
- Route-finding (Opens a modal)
- Discuss: Algorithms in your life (Opens a modal)
Binary search
- Binary search (Opens a modal)
- Implementing binary search of an array (Opens a modal)
- Challenge: Binary search (Opens a modal)
- Running time of binary search (Opens a modal)
- Running time of binary search 5 questions Practice
Asymptotic notation
- Asymptotic notation (Opens a modal)
- Big-θ (Big-Theta) notation (Opens a modal)
- Functions in asymptotic notation (Opens a modal)
- Big-O notation (Opens a modal)
- Big-Ω (Big-Omega) notation (Opens a modal)
- Comparing function growth 4 questions Practice
- Asymptotic notation 5 questions Practice
Selection sort
- Sorting (Opens a modal)
- Challenge: implement swap (Opens a modal)
- Selection sort pseudocode (Opens a modal)
- Challenge: Find minimum in subarray (Opens a modal)
- Challenge: implement selection sort (Opens a modal)
- Analysis of selection sort (Opens a modal)
- Project: Selection sort visualizer (Opens a modal)
Insertion sort
- Insertion sort (Opens a modal)
- Challenge: implement insert (Opens a modal)
- Insertion sort pseudocode (Opens a modal)
- Challenge: Implement insertion sort (Opens a modal)
- Analysis of insertion sort (Opens a modal)
Recursive algorithms
- Recursion (Opens a modal)
- The factorial function (Opens a modal)
- Challenge: Iterative factorial (Opens a modal)
- Recursive factorial (Opens a modal)
- Challenge: Recursive factorial (Opens a modal)
- Properties of recursive algorithms (Opens a modal)
- Using recursion to determine whether a word is a palindrome (Opens a modal)
- Challenge: is a string a palindrome? (Opens a modal)
- Computing powers of a number (Opens a modal)
- Challenge: Recursive powers (Opens a modal)
- Multiple recursion with the Sierpinski gasket (Opens a modal)
- Improving efficiency of recursive functions (Opens a modal)
- Project: Recursive art (Opens a modal)
Towers of Hanoi
- Towers of Hanoi (Opens a modal)
- Towers of Hanoi, continued (Opens a modal)
- Challenge: Solve Hanoi recursively (Opens a modal)
- Move three disks in Towers of Hanoi 3 questions Practice
- Divide and conquer algorithms (Opens a modal)
- Overview of merge sort (Opens a modal)
- Challenge: Implement merge sort (Opens a modal)
- Linear-time merging (Opens a modal)
- Challenge: Implement merge (Opens a modal)
- Analysis of merge sort (Opens a modal)
- Overview of quicksort (Opens a modal)
- Challenge: Implement quicksort (Opens a modal)
- Linear-time partitioning (Opens a modal)
- Challenge: Implement partition (Opens a modal)
- Analysis of quicksort (Opens a modal)
Graph representation
- Describing graphs (Opens a modal)
- Representing graphs (Opens a modal)
- Challenge: Store a graph (Opens a modal)
- Describing graphs 6 questions Practice
- Representing graphs 5 questions Practice
Breadth-first search
- Breadth-first search and its uses (Opens a modal)
- The breadth-first search algorithm (Opens a modal)
- Challenge: Implement breadth-first search (Opens a modal)
- Analysis of breadth-first search (Opens a modal)
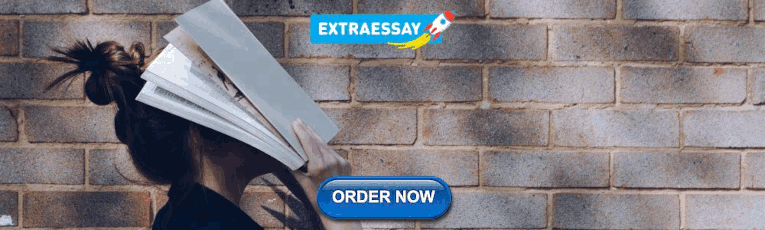
Further learning
- Where to go from here (Opens a modal)
Browse Course Material
Course info.
- Prof. John Guttag
Departments
- Electrical Engineering and Computer Science
As Taught In
- Computer Science
Introduction to Computer Science and Programming
Lecture 3: problem solving.
- Download video
- Download transcript

You are leaving MIT OpenCourseWare

Problem Solving

« Previous | Next »
Session Overview
Session activities, lecture videos.
Flash and JavaScript are required for this feature.
Lecture 3: Problem Solving
> Download from iTunes U (MP4 - 105MB)
> Download from Internet Archive (MP4 - 105MB)
> Download English-US transcript (PDF)
> Download English-US caption (SRT)
About this Video Topics covered: Termination, decrementing functions, exhaustive enumeration, brute force, while loop, for loop, approximation, specifications, bisection search. Resources Lecture code handout (PDF) Lecture code (PY)
Check Yourself
What does it mean for a program to terminate?
› View/hide answer
Either the program will return a value, or throw an exception. A program that does not terminate runs indefinitely, typically because it's gotten stuck in a loop.
What is a for loop?
A for loop takes some sort of iterable object (a list, tuple, or string) and performs its function once for each item in that object. Any function that depends on the input can have a different result at each step, since the input is the current item.
Further Study
These optional resources are provided for students that wish to explore this topic more fully.
- Loops . An Introduction to Python.
CMPU 101 - Computer Science I: Problem-Solving and Abstraction
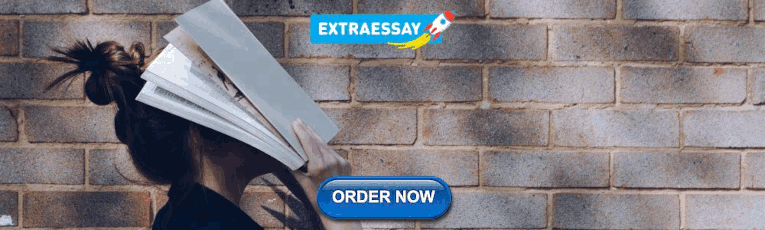
IMAGES
VIDEO
COMMENTS
About this unit. Learn to define algorithms, express them in flow chart and pseudocode, and assess their correctness and efficiency. See how algorithms can be used as shortcuts to solve problems that cannot be solved in a reasonable amount of time, and how this applies to undecidable problems and parallel and distributed computing.
Unit 1 - Problem Solving and Computing. Flashcards. Learn. Test. Match. Flashcards. Learn. Test. Match. Created by. Numb3rwiz Teacher. Terms in this set (17) Input. A device or component that allows information to be given to a computer. Output. Any device or component that receives information from a computer. Algorithm.
3. Calculation: by moving beads on the abacus's spindles, the user can perform addition, subtraction, multiplication and division. • Modern computers contain powerful central processing units that perform calculations at astonishing speeds. 4. User Interface: the beads and spindles on the abacus.
Vocabulary Terms & Concepts Unit 1 Problem Solving & Computing Learn with flashcards, games, and more — for free.
We've partnered with Dartmouth college professors Tom Cormen and Devin Balkcom to teach introductory computer science algorithms, including searching, sorting, recursion, and graph theory. Learn with a combination of articles, visualizations, quizzes, and coding challenges.
How can we use a structured problem-solving process and apply it to address various problems? Enduring Understandings with Unit Goals . EU 1: There are strategies and processes that can be used to become a more effective problem-solver. Utilize a structured problem-solving process and apply it to address various problems.
This two-part course builds upon the programming skills that you learned in our Introduction to Interactive Programming in Python course. We will augment those skills with both important programming practices and critical mathematical problem solving skills. These skills underlie larger scale computational problem solving and programming.
MIT OpenCourseWare is a web based publication of virtually all MIT course content. OCW is open and available to the world and is a permanent MIT activity
Computer Science Discoveries Unit 1 Chapter 1 Lesson 3: Exploring Problem Solving Subject Area. Digital Literacy and Computer Science. Grade(s) 6, 7, 8. Overview. In this lesson, the class applies the problem-solving process to three different problems: a word search, a seating arrangement for a birthday party, and planning a trip. ...
X Exclude words from your search Put - in front of a word you want to leave out. For example, jaguar speed -car Search for an exact match Put a word or phrase inside quotes.
playlist:GE3151Problem solving and Python Programming:https://youtube.com/playlist?list=PLsSVwg9fgFKkOu8uQWjk8W6dpm6PeilLVnotes:https://manojkumardheeran.blo...
Unit 1: Principles of Computer Science Level: 3 Unit type: External Guided learning hours: 120 Unit in brief This unit covers the principles that underpin all areas of computer science. It will develop your computational-thinking skills and you will apply those skills to solve problems. Unit introduction Problem solving is an essential skill in ...
00:37 - components of computer04:54 - various types of memory(RAM & ROM)07:06 - COMPUTER LANGUAGES09:33 - operating systems and types of OS13:14 - types of n...
CMPU 101 - Computer Science I: Problem-Solving and Abstraction. Semester Offered: Fall and Spring. 1 unit (s) Introduces the fundamentals of computer science by describing the functional styles of programming, examining basic sequential and recursive algorithms, and studying linear data structures including arrays and linear collection classes ...
Number of steps in the Problem Solving Process, To gather materials, research, and make a plan, To figure out the problem you are solving, To test out a plan. Problem Solving Process. IOSP. Computers computing. Solving Real World Problems. Parts of an App. 100. ... Information the computer gives to users.
CSD Unit 1 - Problem Solving and Computing*** 5 months ago by . Stephanie Vincent. 68% average accuracy. 17 plays. 9th - 11th grade . Computers. 0. Save. Share. Copy and Edit. Edit. Super resource. With Super, get unlimited access to this resource and over 100,000 other Super resources. Thank you for being Super. Get unlimited access to this ...
Problem Solving and Computing - Lesson 6 Name(s)_____ Period _____ Date _____ Activity Guide - Apps with Processing Key Vocabulary: Processing - The thinking work computers do to turn input into output. Apps and Processing. For each app, choose one type of processing it uses and explain how it helps turn the input into output. ...
Copy of 6.05 Infections and Health Activity Worksheet. Lab05b - Lab Assignment. Activity Guide - Unit 5 Lesson 2 23-24. Apcsa U6 Coding Bat - Screenshots of solutions for some of Array-1 problem sets. Activity Guide - Flippy Do Pt 1 - Unit 1 Lesson 4. Hexadecimal Conversion.
GE3151 PROBLEM SOLVING AND PYTHON PROGRAMMING SYLLABUS UNIT I COMPUTATIONAL THINKING AND PROBLEM SOLVING 9. Fundamentals of Computing - Identification of Computational Problems -Algorithms, building blocks of algorithms (statements, state, control flow, functions), notation (pseudo code, flow chart, programming language), algorithmic problem solving, simple strategies for developing ...
Figure 1 shows a black and white bitmapped image. Figure 2 shows the memory locations where the image is stored. The first byte used for the pixel data is at location 187. The pixel data are stored row-by-row, starting with row 1: • black pixels are encoded with the bit set to 1 • white pixels are encoded with the bit set to 0. Figure 1
Preamble. Algorithmic Thinkingis a fundamental skill in this 21st Century. This course provides the foundations of Computational Problem Solving. It focuses on principles and methods rather than on systems and tools thus providing transferable skills to any other domain. It also provides foundation for developing computational perspectives of ...
UNIT I COMPUTATIONAL THINKING AND PROBLEM SOLVING 9. Fundamentals of Computing - Identification of Computational Problems -Algorithms, building blocks of algorithms (statements, state, control flow, functions), notation (pseudo code, flow chart, programming language), algorithmic problem solving, simple strategies for developing algorithms (iteration, recursion).
Problem Solving and Computing - Lesson 6 Name(s)_victoria minor_____ Period __mod 1____ Date 9/27/23 Activity Guide - Apps with Processing Key Vocabulary: Processing - The thinking work computers do to turn input into output. Apps and Processing For each app, choose one type of processing it uses and explain how it helps turn the input into output.