TypeError: 'tuple' object does not support item assignment
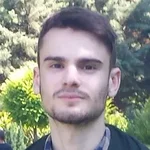
Last updated: Feb 1, 2023 Reading time · 4 min
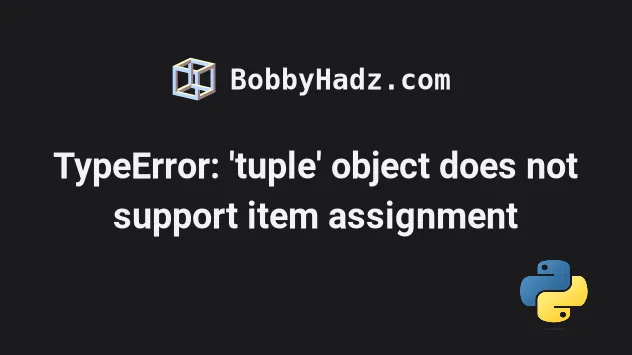
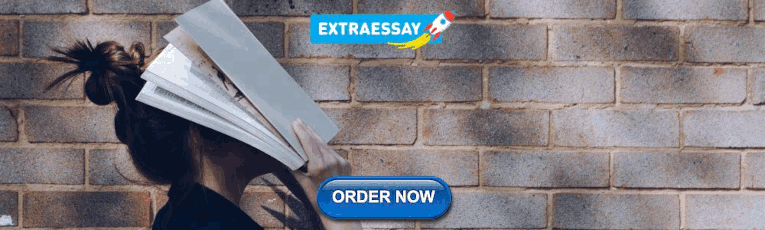
# TypeError: 'tuple' object does not support item assignment
The Python "TypeError: 'tuple' object does not support item assignment" occurs when we try to change the value of an item in a tuple.
To solve the error, convert the tuple to a list, change the item at the specific index and convert the list back to a tuple.

Here is an example of how the error occurs.
We tried to update an element in a tuple, but tuple objects are immutable which caused the error.
# Convert the tuple to a list to solve the error
We cannot assign a value to an individual item of a tuple.
Instead, we have to convert the tuple to a list.
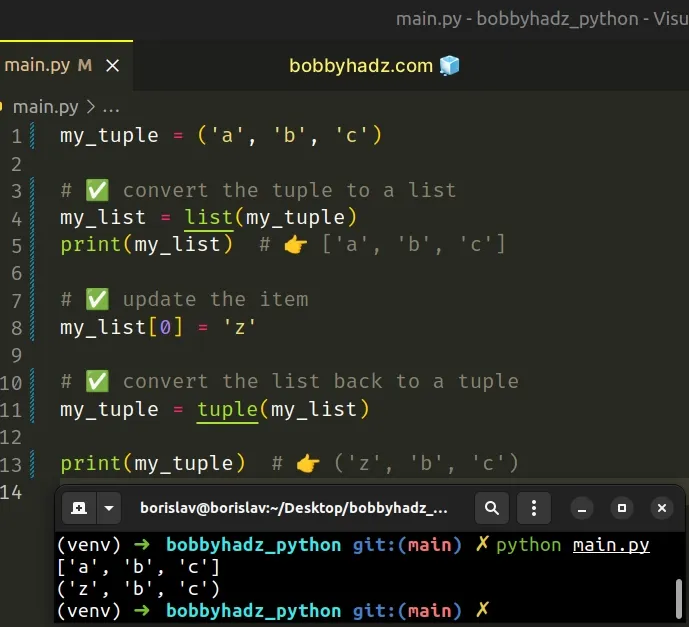
This is a three-step process:
- Use the list() class to convert the tuple to a list.
- Update the item at the specified index.
- Use the tuple() class to convert the list back to a tuple.
Once we have a list, we can update the item at the specified index and optionally convert the result back to a tuple.
Python indexes are zero-based, so the first item in a tuple has an index of 0 , and the last item has an index of -1 or len(my_tuple) - 1 .
# Constructing a new tuple with the updated element
Alternatively, you can construct a new tuple that contains the updated element at the specified index.
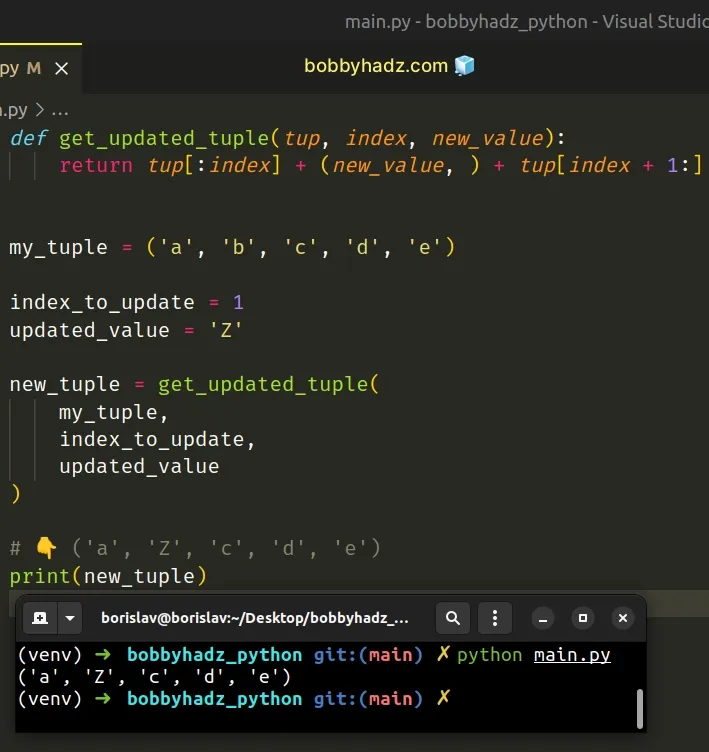
The get_updated_tuple function takes a tuple, an index and a new value and returns a new tuple with the updated value at the specified index.
The original tuple remains unchanged because tuples are immutable.
We updated the tuple element at index 1 , setting it to Z .
If you only have to do this once, you don't have to define a function.
The code sample achieves the same result without using a reusable function.
The values on the left and right-hand sides of the addition (+) operator have to all be tuples.
The syntax for tuple slicing is my_tuple[start:stop:step] .
The start index is inclusive and the stop index is exclusive (up to, but not including).
If the start index is omitted, it is considered to be 0 , if the stop index is omitted, the slice goes to the end of the tuple.
# Using a list instead of a tuple
Alternatively, you can declare a list from the beginning by wrapping the elements in square brackets (not parentheses).
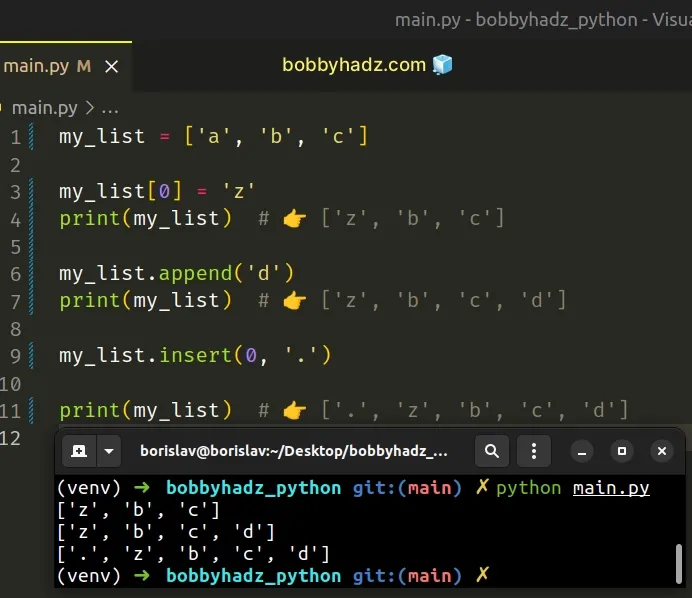
Declaring a list from the beginning is much more efficient if you have to change the values in the collection often.
Tuples are intended to store values that never change.
# How tuples are constructed in Python
In case you declared a tuple by mistake, tuples are constructed in multiple ways:
- Using a pair of parentheses () creates an empty tuple
- Using a trailing comma - a, or (a,)
- Separating items with commas - a, b or (a, b)
- Using the tuple() constructor
# Checking if the value is a tuple
You can also handle the error by checking if the value is a tuple before the assignment.
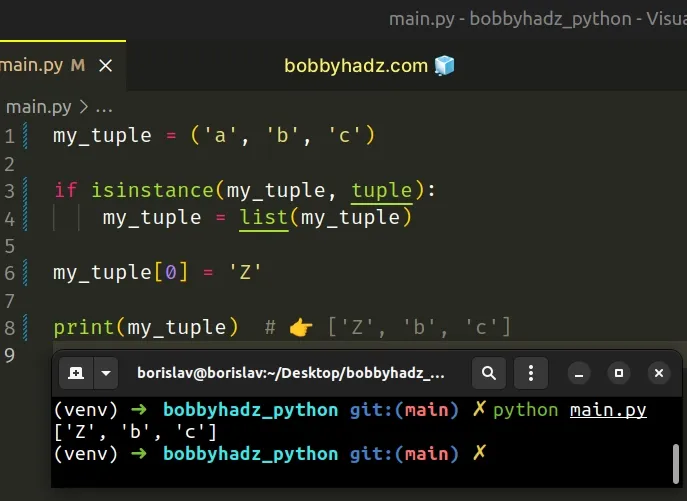
If the variable stores a tuple, we set it to a list to be able to update the value at the specified index.
The isinstance function returns True if the passed-in object is an instance or a subclass of the passed-in class.
If you aren't sure what type a variable stores, use the built-in type() class.
The type class returns the type of an object.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- How to convert a Tuple to an Integer in Python
- How to convert a Tuple to JSON in Python
- Find Min and Max values in Tuple or List of Tuples in Python
- Get the Nth element of a Tuple or List of Tuples in Python
- Creating a Tuple or a Set from user Input in Python
- How to Iterate through a List of Tuples in Python
- Write a List of Tuples to a File in Python
- AttributeError: 'tuple' object has no attribute X in Python
- TypeError: 'tuple' object is not callable in Python [Fixed]
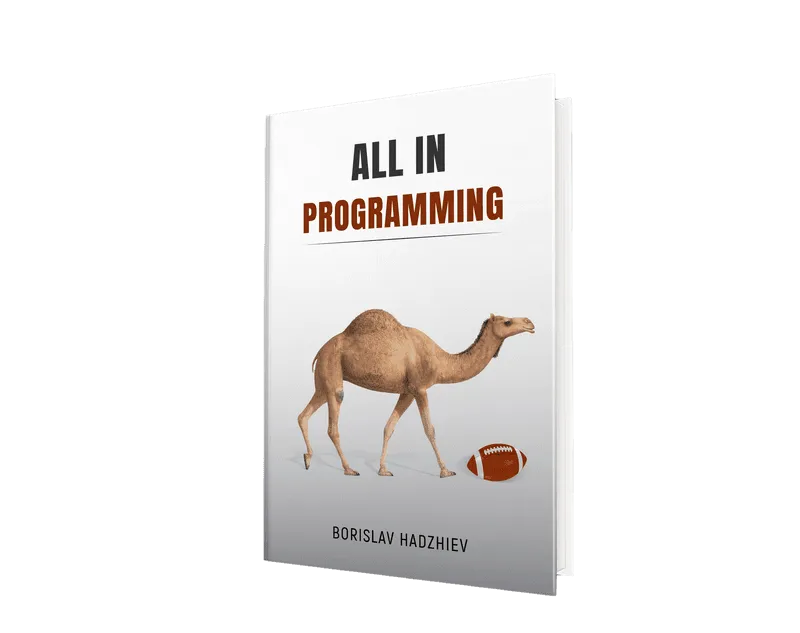
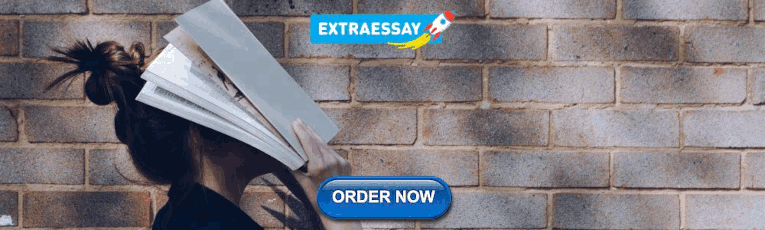
Borislav Hadzhiev
Web Developer
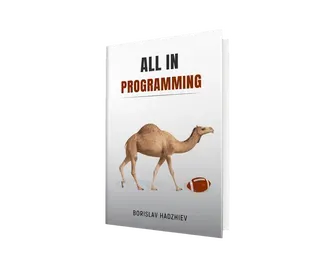
Copyright © 2024 Borislav Hadzhiev
Explore your training options in 10 minutes Get Started
- Graduate Stories
- Partner Spotlights
- Bootcamp Prep
- Bootcamp Admissions
- University Bootcamps
- Coding Tools
- Software Engineering
- Web Development
- Data Science
- Tech Guides
- Tech Resources
- Career Advice
- Online Learning
- Internships
- Apprenticeships
- Tech Salaries
- Associate Degree
- Bachelor's Degree
- Master's Degree
- University Admissions
- Best Schools
- Certifications
- Bootcamp Financing
- Higher Ed Financing
- Scholarships
- Financial Aid
- Best Coding Bootcamps
- Best Online Bootcamps
- Best Web Design Bootcamps
- Best Data Science Bootcamps
- Best Technology Sales Bootcamps
- Best Data Analytics Bootcamps
- Best Cybersecurity Bootcamps
- Best Digital Marketing Bootcamps
- Los Angeles
- San Francisco
- Browse All Locations
- Digital Marketing
- Machine Learning
- See All Subjects
- Bootcamps 101
- Full-Stack Development
- Career Changes
- View all Career Discussions
- Mobile App Development
- Cybersecurity
- Product Management
- UX/UI Design
- What is a Coding Bootcamp?
- Are Coding Bootcamps Worth It?
- How to Choose a Coding Bootcamp
- Best Online Coding Bootcamps and Courses
- Best Free Bootcamps and Coding Training
- Coding Bootcamp vs. Community College
- Coding Bootcamp vs. Self-Learning
- Bootcamps vs. Certifications: Compared
- What Is a Coding Bootcamp Job Guarantee?
- How to Pay for Coding Bootcamp
- Ultimate Guide to Coding Bootcamp Loans
- Best Coding Bootcamp Scholarships and Grants
- Education Stipends for Coding Bootcamps
- Get Your Coding Bootcamp Sponsored by Your Employer
- GI Bill and Coding Bootcamps
- Tech Intevriews
- Our Enterprise Solution
- Connect With Us
- Publication
- Reskill America
- Partner With Us
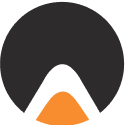
- Resource Center
- Bachelor’s Degree
- Master’s Degree
Python typeerror: ‘tuple’ object does not support item assignment Solution
Tuples are immutable objects . “Immutable” means you cannot change the values inside a tuple. You can only remove them. If you try to assign a new value to an item in a variable, you’ll encounter the “typeerror: ‘tuple’ object does not support item assignment” error.
In this guide, we discuss what this error means and why you may experience it. We’ll walk through an example of this error so you can learn how to solve it in your code.
Find your bootcamp match
Typeerror: ‘tuple’ object does not support item assignment.
While tuples and lists both store sequences of data, they have a few distinctions. Whereas you can change the values in a list, the values inside a tuple cannot be changed. Also, tuples are stored within parenthesis whereas lists are declared between square brackets.
Because you cannot change values in a tuple, item assignment does not work.
Consider the following code snippet:
This code snippet lets us change the first value in the “honor_roll” list to Holly. This works because lists are mutable. You can change their values. The same code does not work with data that is stored in a tuple.
An Example Scenario
Let’s build a program that tracks the courses offered by a high school. Students in their senior year are allowed to choose from a class but a few classes are being replaced.
Start by creating a collection of class names:
We’ve created a tuple that stores the names of each class being offered.
The science department has notified the school that psychology is no longer being offered due to a lack of numbers in the class. We’re going to replace psychology with philosophy as the philosophy class has just opened up a few spaces.
To do this, we use the assignment operator:
This code will replace the value at the index position 3 in our list of classes with “Philosophy”. Next, we print our list of classes to the console so that the user can see what classes are being actively offered:
Use a for loop to print out each class in our tuple to the console. Let’s run our code and see what happens:
Our code returns an error.
The Solution
We’ve tried to use the assignment operator to change a subject in our list. Tuples are immutable so we cannot change their values. This is why our code returns an error.
To solve this problem, we convert our “classes” tuple into a list . This will let us change the values in our sequence of class names.
Do this using the list() method:
We use the list() method to convert the value of “classes” to a list. We assign this new list to the variable “as_list”. Now that we have our list of classes stored as a list, we can change existing classes in the list.
Let’s run our code:
Our code successfully changes the “Psychology” class to “Philosophy”. Our code then prints out the list of classes to the console.
If we need to store our data as a tuple, we can always convert our list back to a tuple once we have changed the values we want to change. We can do this using the tuple() method:
This code converts “as_list” to a tuple and prints the value of our tuple to the console:
We could use this tuple later in our code if we needed our class names stored as a tuple.
The “typeerror: ‘tuple’ object does not support item assignment” error is raised when you try to change a value in a tuple using item assignment.
To solve this error, convert a tuple to a list before you change the values in a sequence. Optionally, you can then convert the list back to a tuple.
Now you’re ready to fix this error in your code like a pro !
About us: Career Karma is a platform designed to help job seekers find, research, and connect with job training programs to advance their careers. Learn about the CK publication .

"Career Karma entered my life when I needed it most and quickly helped me match with a bootcamp. Two months after graduating, I found my dream job that aligned with my values and goals in life!"
Venus, Software Engineer at Rockbot
What's Next?

Get matched with top bootcamps
Ask a question to our community, take our careers quiz.
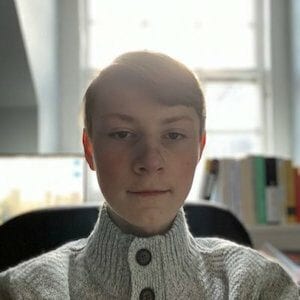
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
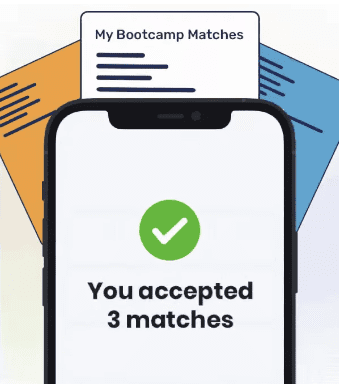
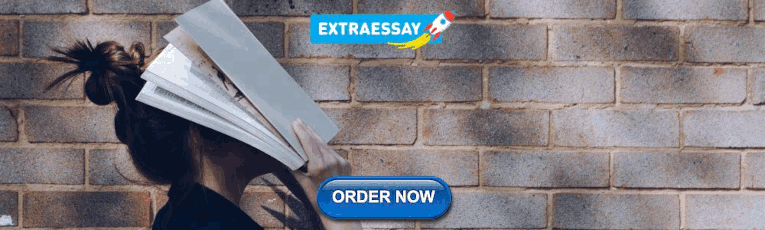
IMAGES
VIDEO
COMMENTS
The Python "TypeError: NoneType object does not support item assignment" occurs when we try to perform an item assignment on a None value. To solve the error, figure out where the variable got assigned a None value and correct the assignment.
The Python "TypeError: 'tuple' object does not support item assignment" occurs when we try to change the value of an item in a tuple. To solve the error, convert the tuple to a list, change the item at the specific index and convert the list back to a tuple.
typeerror: ‘tuple’ object does not support item assignment. While tuples and lists both store sequences of data, they have a few distinctions. Whereas you can change the values in a list, the values inside a tuple cannot be changed. Also, tuples are stored within parenthesis whereas lists are declared between square brackets.