- Python »
- 3.12.3 Documentation »
- The Python Language Reference »
- 7. Simple statements
- Theme Auto Light Dark |
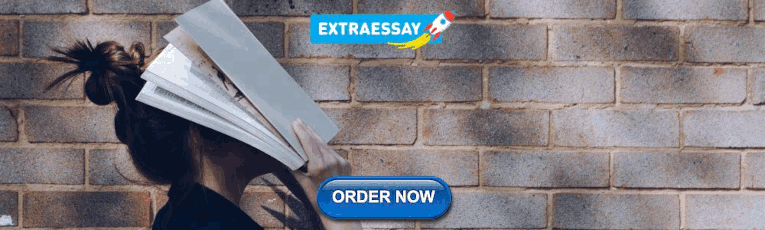
7. Simple statements ¶
A simple statement is comprised within a single logical line. Several simple statements may occur on a single line separated by semicolons. The syntax for simple statements is:
7.1. Expression statements ¶
Expression statements are used (mostly interactively) to compute and write a value, or (usually) to call a procedure (a function that returns no meaningful result; in Python, procedures return the value None ). Other uses of expression statements are allowed and occasionally useful. The syntax for an expression statement is:
An expression statement evaluates the expression list (which may be a single expression).
In interactive mode, if the value is not None , it is converted to a string using the built-in repr() function and the resulting string is written to standard output on a line by itself (except if the result is None , so that procedure calls do not cause any output.)
7.2. Assignment statements ¶
Assignment statements are used to (re)bind names to values and to modify attributes or items of mutable objects:
(See section Primaries for the syntax definitions for attributeref , subscription , and slicing .)
An assignment statement evaluates the expression list (remember that this can be a single expression or a comma-separated list, the latter yielding a tuple) and assigns the single resulting object to each of the target lists, from left to right.
Assignment is defined recursively depending on the form of the target (list). When a target is part of a mutable object (an attribute reference, subscription or slicing), the mutable object must ultimately perform the assignment and decide about its validity, and may raise an exception if the assignment is unacceptable. The rules observed by various types and the exceptions raised are given with the definition of the object types (see section The standard type hierarchy ).
Assignment of an object to a target list, optionally enclosed in parentheses or square brackets, is recursively defined as follows.
If the target list is a single target with no trailing comma, optionally in parentheses, the object is assigned to that target.
If the target list contains one target prefixed with an asterisk, called a “starred” target: The object must be an iterable with at least as many items as there are targets in the target list, minus one. The first items of the iterable are assigned, from left to right, to the targets before the starred target. The final items of the iterable are assigned to the targets after the starred target. A list of the remaining items in the iterable is then assigned to the starred target (the list can be empty).
Else: The object must be an iterable with the same number of items as there are targets in the target list, and the items are assigned, from left to right, to the corresponding targets.
Assignment of an object to a single target is recursively defined as follows.
If the target is an identifier (name):
If the name does not occur in a global or nonlocal statement in the current code block: the name is bound to the object in the current local namespace.
Otherwise: the name is bound to the object in the global namespace or the outer namespace determined by nonlocal , respectively.
The name is rebound if it was already bound. This may cause the reference count for the object previously bound to the name to reach zero, causing the object to be deallocated and its destructor (if it has one) to be called.
If the target is an attribute reference: The primary expression in the reference is evaluated. It should yield an object with assignable attributes; if this is not the case, TypeError is raised. That object is then asked to assign the assigned object to the given attribute; if it cannot perform the assignment, it raises an exception (usually but not necessarily AttributeError ).
Note: If the object is a class instance and the attribute reference occurs on both sides of the assignment operator, the right-hand side expression, a.x can access either an instance attribute or (if no instance attribute exists) a class attribute. The left-hand side target a.x is always set as an instance attribute, creating it if necessary. Thus, the two occurrences of a.x do not necessarily refer to the same attribute: if the right-hand side expression refers to a class attribute, the left-hand side creates a new instance attribute as the target of the assignment:
This description does not necessarily apply to descriptor attributes, such as properties created with property() .
If the target is a subscription: The primary expression in the reference is evaluated. It should yield either a mutable sequence object (such as a list) or a mapping object (such as a dictionary). Next, the subscript expression is evaluated.
If the primary is a mutable sequence object (such as a list), the subscript must yield an integer. If it is negative, the sequence’s length is added to it. The resulting value must be a nonnegative integer less than the sequence’s length, and the sequence is asked to assign the assigned object to its item with that index. If the index is out of range, IndexError is raised (assignment to a subscripted sequence cannot add new items to a list).
If the primary is a mapping object (such as a dictionary), the subscript must have a type compatible with the mapping’s key type, and the mapping is then asked to create a key/value pair which maps the subscript to the assigned object. This can either replace an existing key/value pair with the same key value, or insert a new key/value pair (if no key with the same value existed).
For user-defined objects, the __setitem__() method is called with appropriate arguments.
If the target is a slicing: The primary expression in the reference is evaluated. It should yield a mutable sequence object (such as a list). The assigned object should be a sequence object of the same type. Next, the lower and upper bound expressions are evaluated, insofar they are present; defaults are zero and the sequence’s length. The bounds should evaluate to integers. If either bound is negative, the sequence’s length is added to it. The resulting bounds are clipped to lie between zero and the sequence’s length, inclusive. Finally, the sequence object is asked to replace the slice with the items of the assigned sequence. The length of the slice may be different from the length of the assigned sequence, thus changing the length of the target sequence, if the target sequence allows it.
CPython implementation detail: In the current implementation, the syntax for targets is taken to be the same as for expressions, and invalid syntax is rejected during the code generation phase, causing less detailed error messages.
Although the definition of assignment implies that overlaps between the left-hand side and the right-hand side are ‘simultaneous’ (for example a, b = b, a swaps two variables), overlaps within the collection of assigned-to variables occur left-to-right, sometimes resulting in confusion. For instance, the following program prints [0, 2] :
The specification for the *target feature.
7.2.1. Augmented assignment statements ¶
Augmented assignment is the combination, in a single statement, of a binary operation and an assignment statement:
(See section Primaries for the syntax definitions of the last three symbols.)
An augmented assignment evaluates the target (which, unlike normal assignment statements, cannot be an unpacking) and the expression list, performs the binary operation specific to the type of assignment on the two operands, and assigns the result to the original target. The target is only evaluated once.
An augmented assignment expression like x += 1 can be rewritten as x = x + 1 to achieve a similar, but not exactly equal effect. In the augmented version, x is only evaluated once. Also, when possible, the actual operation is performed in-place , meaning that rather than creating a new object and assigning that to the target, the old object is modified instead.
Unlike normal assignments, augmented assignments evaluate the left-hand side before evaluating the right-hand side. For example, a[i] += f(x) first looks-up a[i] , then it evaluates f(x) and performs the addition, and lastly, it writes the result back to a[i] .
With the exception of assigning to tuples and multiple targets in a single statement, the assignment done by augmented assignment statements is handled the same way as normal assignments. Similarly, with the exception of the possible in-place behavior, the binary operation performed by augmented assignment is the same as the normal binary operations.
For targets which are attribute references, the same caveat about class and instance attributes applies as for regular assignments.
7.2.2. Annotated assignment statements ¶
Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement:
The difference from normal Assignment statements is that only a single target is allowed.
For simple names as assignment targets, if in class or module scope, the annotations are evaluated and stored in a special class or module attribute __annotations__ that is a dictionary mapping from variable names (mangled if private) to evaluated annotations. This attribute is writable and is automatically created at the start of class or module body execution, if annotations are found statically.
For expressions as assignment targets, the annotations are evaluated if in class or module scope, but not stored.
If a name is annotated in a function scope, then this name is local for that scope. Annotations are never evaluated and stored in function scopes.
If the right hand side is present, an annotated assignment performs the actual assignment before evaluating annotations (where applicable). If the right hand side is not present for an expression target, then the interpreter evaluates the target except for the last __setitem__() or __setattr__() call.
The proposal that added syntax for annotating the types of variables (including class variables and instance variables), instead of expressing them through comments.
The proposal that added the typing module to provide a standard syntax for type annotations that can be used in static analysis tools and IDEs.
Changed in version 3.8: Now annotated assignments allow the same expressions in the right hand side as regular assignments. Previously, some expressions (like un-parenthesized tuple expressions) caused a syntax error.
7.3. The assert statement ¶
Assert statements are a convenient way to insert debugging assertions into a program:
The simple form, assert expression , is equivalent to
The extended form, assert expression1, expression2 , is equivalent to
These equivalences assume that __debug__ and AssertionError refer to the built-in variables with those names. In the current implementation, the built-in variable __debug__ is True under normal circumstances, False when optimization is requested (command line option -O ). The current code generator emits no code for an assert statement when optimization is requested at compile time. Note that it is unnecessary to include the source code for the expression that failed in the error message; it will be displayed as part of the stack trace.
Assignments to __debug__ are illegal. The value for the built-in variable is determined when the interpreter starts.
7.4. The pass statement ¶
pass is a null operation — when it is executed, nothing happens. It is useful as a placeholder when a statement is required syntactically, but no code needs to be executed, for example:
7.5. The del statement ¶
Deletion is recursively defined very similar to the way assignment is defined. Rather than spelling it out in full details, here are some hints.
Deletion of a target list recursively deletes each target, from left to right.
Deletion of a name removes the binding of that name from the local or global namespace, depending on whether the name occurs in a global statement in the same code block. If the name is unbound, a NameError exception will be raised.
Deletion of attribute references, subscriptions and slicings is passed to the primary object involved; deletion of a slicing is in general equivalent to assignment of an empty slice of the right type (but even this is determined by the sliced object).
Changed in version 3.2: Previously it was illegal to delete a name from the local namespace if it occurs as a free variable in a nested block.
7.6. The return statement ¶
return may only occur syntactically nested in a function definition, not within a nested class definition.
If an expression list is present, it is evaluated, else None is substituted.
return leaves the current function call with the expression list (or None ) as return value.
When return passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the function.
In a generator function, the return statement indicates that the generator is done and will cause StopIteration to be raised. The returned value (if any) is used as an argument to construct StopIteration and becomes the StopIteration.value attribute.
In an asynchronous generator function, an empty return statement indicates that the asynchronous generator is done and will cause StopAsyncIteration to be raised. A non-empty return statement is a syntax error in an asynchronous generator function.
7.7. The yield statement ¶
A yield statement is semantically equivalent to a yield expression . The yield statement can be used to omit the parentheses that would otherwise be required in the equivalent yield expression statement. For example, the yield statements
are equivalent to the yield expression statements
Yield expressions and statements are only used when defining a generator function, and are only used in the body of the generator function. Using yield in a function definition is sufficient to cause that definition to create a generator function instead of a normal function.
For full details of yield semantics, refer to the Yield expressions section.
7.8. The raise statement ¶
If no expressions are present, raise re-raises the exception that is currently being handled, which is also known as the active exception . If there isn’t currently an active exception, a RuntimeError exception is raised indicating that this is an error.
Otherwise, raise evaluates the first expression as the exception object. It must be either a subclass or an instance of BaseException . If it is a class, the exception instance will be obtained when needed by instantiating the class with no arguments.
The type of the exception is the exception instance’s class, the value is the instance itself.
A traceback object is normally created automatically when an exception is raised and attached to it as the __traceback__ attribute. You can create an exception and set your own traceback in one step using the with_traceback() exception method (which returns the same exception instance, with its traceback set to its argument), like so:
The from clause is used for exception chaining: if given, the second expression must be another exception class or instance. If the second expression is an exception instance, it will be attached to the raised exception as the __cause__ attribute (which is writable). If the expression is an exception class, the class will be instantiated and the resulting exception instance will be attached to the raised exception as the __cause__ attribute. If the raised exception is not handled, both exceptions will be printed:
A similar mechanism works implicitly if a new exception is raised when an exception is already being handled. An exception may be handled when an except or finally clause, or a with statement, is used. The previous exception is then attached as the new exception’s __context__ attribute:
Exception chaining can be explicitly suppressed by specifying None in the from clause:
Additional information on exceptions can be found in section Exceptions , and information about handling exceptions is in section The try statement .
Changed in version 3.3: None is now permitted as Y in raise X from Y .
Added the __suppress_context__ attribute to suppress automatic display of the exception context.
Changed in version 3.11: If the traceback of the active exception is modified in an except clause, a subsequent raise statement re-raises the exception with the modified traceback. Previously, the exception was re-raised with the traceback it had when it was caught.
7.9. The break statement ¶
break may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop.
It terminates the nearest enclosing loop, skipping the optional else clause if the loop has one.
If a for loop is terminated by break , the loop control target keeps its current value.
When break passes control out of a try statement with a finally clause, that finally clause is executed before really leaving the loop.
7.10. The continue statement ¶
continue may only occur syntactically nested in a for or while loop, but not nested in a function or class definition within that loop. It continues with the next cycle of the nearest enclosing loop.
When continue passes control out of a try statement with a finally clause, that finally clause is executed before really starting the next loop cycle.
7.11. The import statement ¶
The basic import statement (no from clause) is executed in two steps:
find a module, loading and initializing it if necessary
define a name or names in the local namespace for the scope where the import statement occurs.
When the statement contains multiple clauses (separated by commas) the two steps are carried out separately for each clause, just as though the clauses had been separated out into individual import statements.
The details of the first step, finding and loading modules, are described in greater detail in the section on the import system , which also describes the various types of packages and modules that can be imported, as well as all the hooks that can be used to customize the import system. Note that failures in this step may indicate either that the module could not be located, or that an error occurred while initializing the module, which includes execution of the module’s code.
If the requested module is retrieved successfully, it will be made available in the local namespace in one of three ways:
If the module name is followed by as , then the name following as is bound directly to the imported module.
If no other name is specified, and the module being imported is a top level module, the module’s name is bound in the local namespace as a reference to the imported module
If the module being imported is not a top level module, then the name of the top level package that contains the module is bound in the local namespace as a reference to the top level package. The imported module must be accessed using its full qualified name rather than directly
The from form uses a slightly more complex process:
find the module specified in the from clause, loading and initializing it if necessary;
for each of the identifiers specified in the import clauses:
check if the imported module has an attribute by that name
if not, attempt to import a submodule with that name and then check the imported module again for that attribute
if the attribute is not found, ImportError is raised.
otherwise, a reference to that value is stored in the local namespace, using the name in the as clause if it is present, otherwise using the attribute name
If the list of identifiers is replaced by a star ( '*' ), all public names defined in the module are bound in the local namespace for the scope where the import statement occurs.
The public names defined by a module are determined by checking the module’s namespace for a variable named __all__ ; if defined, it must be a sequence of strings which are names defined or imported by that module. The names given in __all__ are all considered public and are required to exist. If __all__ is not defined, the set of public names includes all names found in the module’s namespace which do not begin with an underscore character ( '_' ). __all__ should contain the entire public API. It is intended to avoid accidentally exporting items that are not part of the API (such as library modules which were imported and used within the module).
The wild card form of import — from module import * — is only allowed at the module level. Attempting to use it in class or function definitions will raise a SyntaxError .
When specifying what module to import you do not have to specify the absolute name of the module. When a module or package is contained within another package it is possible to make a relative import within the same top package without having to mention the package name. By using leading dots in the specified module or package after from you can specify how high to traverse up the current package hierarchy without specifying exact names. One leading dot means the current package where the module making the import exists. Two dots means up one package level. Three dots is up two levels, etc. So if you execute from . import mod from a module in the pkg package then you will end up importing pkg.mod . If you execute from ..subpkg2 import mod from within pkg.subpkg1 you will import pkg.subpkg2.mod . The specification for relative imports is contained in the Package Relative Imports section.
importlib.import_module() is provided to support applications that determine dynamically the modules to be loaded.
Raises an auditing event import with arguments module , filename , sys.path , sys.meta_path , sys.path_hooks .
7.11.1. Future statements ¶
A future statement is a directive to the compiler that a particular module should be compiled using syntax or semantics that will be available in a specified future release of Python where the feature becomes standard.
The future statement is intended to ease migration to future versions of Python that introduce incompatible changes to the language. It allows use of the new features on a per-module basis before the release in which the feature becomes standard.
A future statement must appear near the top of the module. The only lines that can appear before a future statement are:
the module docstring (if any),
blank lines, and
other future statements.
The only feature that requires using the future statement is annotations (see PEP 563 ).
All historical features enabled by the future statement are still recognized by Python 3. The list includes absolute_import , division , generators , generator_stop , unicode_literals , print_function , nested_scopes and with_statement . They are all redundant because they are always enabled, and only kept for backwards compatibility.
A future statement is recognized and treated specially at compile time: Changes to the semantics of core constructs are often implemented by generating different code. It may even be the case that a new feature introduces new incompatible syntax (such as a new reserved word), in which case the compiler may need to parse the module differently. Such decisions cannot be pushed off until runtime.
For any given release, the compiler knows which feature names have been defined, and raises a compile-time error if a future statement contains a feature not known to it.
The direct runtime semantics are the same as for any import statement: there is a standard module __future__ , described later, and it will be imported in the usual way at the time the future statement is executed.
The interesting runtime semantics depend on the specific feature enabled by the future statement.
Note that there is nothing special about the statement:
That is not a future statement; it’s an ordinary import statement with no special semantics or syntax restrictions.
Code compiled by calls to the built-in functions exec() and compile() that occur in a module M containing a future statement will, by default, use the new syntax or semantics associated with the future statement. This can be controlled by optional arguments to compile() — see the documentation of that function for details.
A future statement typed at an interactive interpreter prompt will take effect for the rest of the interpreter session. If an interpreter is started with the -i option, is passed a script name to execute, and the script includes a future statement, it will be in effect in the interactive session started after the script is executed.
The original proposal for the __future__ mechanism.
7.12. The global statement ¶
The global statement is a declaration which holds for the entire current code block. It means that the listed identifiers are to be interpreted as globals. It would be impossible to assign to a global variable without global , although free variables may refer to globals without being declared global.
Names listed in a global statement must not be used in the same code block textually preceding that global statement.
Names listed in a global statement must not be defined as formal parameters, or as targets in with statements or except clauses, or in a for target list, class definition, function definition, import statement, or variable annotation.
CPython implementation detail: The current implementation does not enforce some of these restrictions, but programs should not abuse this freedom, as future implementations may enforce them or silently change the meaning of the program.
Programmer’s note: global is a directive to the parser. It applies only to code parsed at the same time as the global statement. In particular, a global statement contained in a string or code object supplied to the built-in exec() function does not affect the code block containing the function call, and code contained in such a string is unaffected by global statements in the code containing the function call. The same applies to the eval() and compile() functions.
7.13. The nonlocal statement ¶
When the definition of a function or class is nested (enclosed) within the definitions of other functions, its nonlocal scopes are the local scopes of the enclosing functions. The nonlocal statement causes the listed identifiers to refer to names previously bound in nonlocal scopes. It allows encapsulated code to rebind such nonlocal identifiers. If a name is bound in more than one nonlocal scope, the nearest binding is used. If a name is not bound in any nonlocal scope, or if there is no nonlocal scope, a SyntaxError is raised.
The nonlocal statement applies to the entire scope of a function or class body. A SyntaxError is raised if a variable is used or assigned to prior to its nonlocal declaration in the scope.
The specification for the nonlocal statement.
Programmer’s note: nonlocal is a directive to the parser and applies only to code parsed along with it. See the note for the global statement.
7.14. The type statement ¶
The type statement declares a type alias, which is an instance of typing.TypeAliasType .
For example, the following statement creates a type alias:
This code is roughly equivalent to:
annotation-def indicates an annotation scope , which behaves mostly like a function, but with several small differences.
The value of the type alias is evaluated in the annotation scope. It is not evaluated when the type alias is created, but only when the value is accessed through the type alias’s __value__ attribute (see Lazy evaluation ). This allows the type alias to refer to names that are not yet defined.
Type aliases may be made generic by adding a type parameter list after the name. See Generic type aliases for more.
type is a soft keyword .
New in version 3.12.
Introduced the type statement and syntax for generic classes and functions.
Table of Contents
- 7.1. Expression statements
- 7.2.1. Augmented assignment statements
- 7.2.2. Annotated assignment statements
- 7.3. The assert statement
- 7.4. The pass statement
- 7.5. The del statement
- 7.6. The return statement
- 7.7. The yield statement
- 7.8. The raise statement
- 7.9. The break statement
- 7.10. The continue statement
- 7.11.1. Future statements
- 7.12. The global statement
- 7.13. The nonlocal statement
- 7.14. The type statement
Previous topic
6. Expressions
8. Compound statements
- Report a Bug
- Show Source
- Assignment Statement
An Assignment statement is a statement that is used to set a value to the variable name in a program .
Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted by a variable name.

The symbol used in an assignment statement is called as an operator . The symbol is ‘=’ .
Note: The Assignment Operator should never be used for Equality purpose which is double equal sign ‘==’.
The Basic Syntax of Assignment Statement in a programming language is :
variable = expression ;
variable = variable name
expression = it could be either a direct value or a math expression/formula or a function call
Few programming languages such as Java, C, C++ require data type to be specified for the variable, so that it is easy to allocate memory space and store those values during program execution.
data_type variable_name = value ;
In the above-given examples, Variable ‘a’ is assigned a value in the same statement as per its defined data type. A data type is only declared for Variable ‘b’. In the 3 rd line of code, Variable ‘a’ is reassigned the value 25. The 4 th line of code assigns the value for Variable ‘b’.
Assignment Statement Forms
This is one of the most common forms of Assignment Statements. Here the Variable name is defined, initialized, and assigned a value in the same statement. This form is generally used when we want to use the Variable quite a few times and we do not want to change its value very frequently.
Tuple Assignment
Generally, we use this form when we want to define and assign values for more than 1 variable at the same time. This saves time and is an easy method. Note that here every individual variable has a different value assigned to it.
(Code In Python)
Sequence Assignment
(Code in Python)
Multiple-target Assignment or Chain Assignment
In this format, a single value is assigned to two or more variables.
Augmented Assignment
In this format, we use the combination of mathematical expressions and values for the Variable. Other augmented Assignment forms are: &=, -=, **=, etc.
Browse more Topics Under Data Types, Variables and Constants
- Concept of Data types
- Built-in Data Types
- Constants in Programing Language
- Access Modifier
- Variables of Built-in-Datatypes
- Declaration/Initialization of Variables
- Type Modifier
Few Rules for Assignment Statement
Few Rules to be followed while writing the Assignment Statements are:
- Variable names must begin with a letter, underscore, non-number character. Each language has its own conventions.
- The Data type defined and the variable value must match.
- A variable name once defined can only be used once in the program. You cannot define it again to store other types of value.
- If you assign a new value to an existing variable, it will overwrite the previous value and assign the new value.
FAQs on Assignment Statement
Q1. Which of the following shows the syntax of an assignment statement ?
- variablename = expression ;
- expression = variable ;
- datatype = variablename ;
- expression = datatype variable ;
Answer – Option A.
Q2. What is an expression ?
- Same as statement
- List of statements that make up a program
- Combination of literals, operators, variables, math formulas used to calculate a value
- Numbers expressed in digits
Answer – Option C.
Q3. What are the two steps that take place when an assignment statement is executed?
- Evaluate the expression, store the value in the variable
- Reserve memory, fill it with value
- Evaluate variable, store the result
- Store the value in the variable, evaluate the expression.
Customize your course in 30 seconds
Which class are you in.

Data Types, Variables and Constants
- Variables in Programming Language
- Concept of Data Types
- Declaration of Variables
- Type Modifiers
- Access Modifiers
- Constants in Programming Language
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Download the App

- Free Python 3 Tutorial
- Control Flow
- Exception Handling
- Python Programs
- Python Projects
- Python Interview Questions
- Python Database
- Data Science With Python
- Machine Learning with Python
- Memory Management in Python
- Ways to increment Iterator from inside the For loop in Python
- How to Break out of multiple loops in Python ?
- Scope Resolution in Python | LEGB Rule
- How to add Python to Windows PATH?
- Benefits of Double Division Operator over Single Division Operator in Python
- Specifying the increment in for-loops in Python
- How to use Variables in Python3?
- Check multiple conditions in if statement - Python
- Difference between dir() and vars() in Python
- Variables under the hood in Python
- Understanding the Execution of Python Program
- Viewing all defined variables in Python
- Convert Python String to Float datatype
- Python Main Function
- What is the difference between Python's Module, Package and Library?
- How to convert Float to Int in Python?
- Context Variables in Python
- Why does Python automatically exit a script when it’s done?
Different Forms of Assignment Statements in Python
We use Python assignment statements to assign objects to names. The target of an assignment statement is written on the left side of the equal sign (=), and the object on the right can be an arbitrary expression that computes an object.
There are some important properties of assignment in Python :-
- Assignment creates object references instead of copying the objects.
- Python creates a variable name the first time when they are assigned a value.
- Names must be assigned before being referenced.
- There are some operations that perform assignments implicitly.
Assignment statement forms :-
1. Basic form:
This form is the most common form.
2. Tuple assignment:
When we code a tuple on the left side of the =, Python pairs objects on the right side with targets on the left by position and assigns them from left to right. Therefore, the values of x and y are 50 and 100 respectively.
3. List assignment:
This works in the same way as the tuple assignment.
4. Sequence assignment:
In recent version of Python, tuple and list assignment have been generalized into instances of what we now call sequence assignment – any sequence of names can be assigned to any sequence of values, and Python assigns the items one at a time by position.
5. Extended Sequence unpacking:
It allows us to be more flexible in how we select portions of a sequence to assign.
Here, p is matched with the first character in the string on the right and q with the rest. The starred name (*q) is assigned a list, which collects all items in the sequence not assigned to other names.
This is especially handy for a common coding pattern such as splitting a sequence and accessing its front and rest part.
6. Multiple- target assignment:
In this form, Python assigns a reference to the same object (the object which is rightmost) to all the target on the left.
7. Augmented assignment :
The augmented assignment is a shorthand assignment that combines an expression and an assignment.
There are several other augmented assignment forms:
Please Login to comment...
Similar reads.
- python-basics
- How to Use ChatGPT with Bing for Free?
- 7 Best Movavi Video Editor Alternatives in 2024
- How to Edit Comment on Instagram
- 10 Best AI Grammar Checkers and Rewording Tools
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
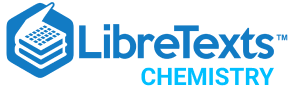
- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
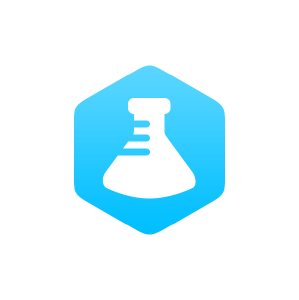
2.3: Arithmetic Operations and Assignment Statements
- Last updated
- Save as PDF
- Page ID 206261
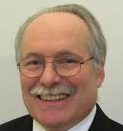
- Robert Belford
- University of Arkansas at Little Rock
hypothes.is tag: s20iostpy03ualr Download Assignment: S2020py03
Learning Objectives
Students will be able to:
- Explain each Python arithmetic operator
- Explain the meaning and use of an assignment statement
- Explain the use of "+" and "*" with strings and numbers
- Use the int() and float() functions to convert string input to numbers for computation
- Incorporate numeric formatting into print statements
- Recognize the four main operations of a computer within a simple Python program
- Create input statements in Python
- Create Python code that performs mathematical and string operations
- Create Python code that uses assignment statements
- Create Python code that formats numeric output
Prior Knowledge
- Understanding of Python print and input statements
- Understanding of mathematical operations
- Understanding of flowchart input symbols
Further Reading
- https://en.wikibooks.org/wiki/Non-Programmer%27s_Tutorial_for_Python_3/Hello,_World
- https://en.wikibooks.org/wiki/Non-Programmer%27s_Tutorial_for_Python_3/Who_Goes_There%3F
Model 1: Arithmetic Operators in Python
Python includes several arithmetic operators: addition, subtraction, multiplication, two types of division, exponentiation and mod .
Critical Thinking Questions:
1. Draw a line between each flowchart symbol and its corresponding line of Python code. Make note of any problems.
2. Execute the print statements in the previous Python program
a. Next to each print statement above, write the output. b. What is the value of the following line of code?
c. Predict the values of 17%3 and 18%3 without using your computer.
3. Explain the purpose of each arithmetic operation:
a. + ____________________________
b. - ____________________________
c. * ____________________________
d. ** ____________________________
e. / ____________________________
f. // ____________________________
g. % ____________________________
An assignment statement is a line of code that uses a "=" sign. The statement stores the result of an operation performed on the right-hand side of the sign into the variable memory location on the left-hand side.
4. Enter and execute the following lines of Python code in the editor window of your IDE (e.g. Thonny):
a. What are the variables in the above python program? b. What does the assignment statement : MethaneMolMs = 16 do? c. What happens if you replace the comma (,) in the print statements with a plus sign (+) and execute the code again? Why does this happen?
5. What is stored in memory after each assignment statement is executed?
Note: Concatenating Strings in python
The "+" concatenates the two strings stored in the variables into one string. "+" can only be used when both operators are strings.
6. Run the following program in the editor window of your IDE (e.g. Thonny) to see what happens if you try to use the "+" with strings instead of numbers?
a. The third line of code contains an assignment statement. What is stored in fullName when the line is executed? b. What is the difference between the two output lines? c. How could you alter your assignment statements so that print(fullName) gives the same output as print(firstName,lastName) d. Only one of the following programs will work. Which one will work, and why doesn’t the other work? Try doing this without running the programs!
e. Run the programs above and see if you were correct. f. The program that worked above results in no space between the number and the street name. How can you alter the code so that it prints properly while using a concatenation operator?
7. Before entering the following code into the Python interpreter (Thonny IDE editor window), predict the output of this program.
Now execute it. What is the actual output? Is this what you thought it would do? Explain.
8. Let’s take a look at a python program that prompts the user for two numbers and subtracts them.
Execute the following code by entering it in the editor window of Thonny.
a. What output do you expect? b. What is the actual output c. Revise the program in the following manner:
- Between lines two and three add the following lines of code: num1 = int(firstNumber) num2 = int(secondNumber)
- Next, replace the statement: difference = firstNumber – secondNumber with the statement: difference = num1 – num2
- Execute the program again. What output did you get?
d. Explain the purpose of the function int(). e. Explain how the changes in the program produced the desired output.
Model 3: Formatting Output in Python
There are multiple ways to format output in python. The old way is to use the string modulo %, and the new way is with a format method function.
9. Look closely at the output for python program 7.
a. How do you indicate the number of decimals to display using
the string modulo (%) ______________________________________________________
the format function ________________________________________________________
b. What happens to the number if you tell it to display less decimals than are in the number, regardless of formatting method used?
c. What type of code allows you to right justify your numbers?
10. Execute the following code by entering it in the editor window of Thonny.
a. Does the output look like standard output for something that has dollars and cents associated with it?
b. Replace the last line of code with the following:
print("Total cost of laptops: $%.2f" % price)
print("Total cost of laptops:" ,format(price, '.2f.))
Discuss the change in the output.
c. Replace the last line of code with the following:
print("Total cost of laptops: $", format(price,'.2f') print("Total cost of laptops: $" ,format(price, '.2f.))
Discuss the change in the output.
d. Experiment with the number ".2" in the ‘0.2f’ of the print above statement by substituting the following numbers and explain the results.
.4 ___________________________________________________
.0 ___________________________________________________
.1 ___________________________________________________
.8 ___________________________________________________
e. Now try the following numbers in the same print statement. These numbers contain a whole number and a decimal. Explain the output for each number.
02.5 ___________________________________________________
08.2 ___________________________________________________
03.1 ___________________________________________________
f. Explain what each part of the format function: format(variable, "%n.nf") does in a print statement where n.n represents a number.
variable ____________________________ First n _________________________
Second n_______________________ f _________________________
g. Revise the print statement by changing the "f" to "d" and laptopCost = 600 . Execute the statements and explain the output format.
print("Total cost of laptops: %2d" % price) print("Total cost of laptops: %10d" % price)
h. Explain how the function format(var,'10d') formats numeric data. var represents a whole number.
11. Use the following program and output to answer the questions below.
a. From the code and comments in the previous program, explain how the four main operations are implemented in this program. b. There is one new function in this sample program. What is it? From the corresponding output, determine what it does.
Application Questions: Use the Python Interpreter to check your work
- 8 to the 4 th power
- The sum of 5 and 6 multiplied by the quotient of 34 and 7 using floating point arithmetic
- Write an assignment statement that stores the remainder obtained from dividing 87 and 8 in the variable leftover
- Assume:
courseLabel = "CHEM" courseNumber = "3350"
Write a line of Python code that concatenates the label with the number and stores the result in the variable courseName . Be sure that there is a space between the course label and the course number when they are concatenated.
- Write one line of Python code that will print the word "Happy!" one hundred times.
- Write one line of code that calculates the cost of 15 items and stores the result in the variable totalCost
- Write one line of code that prints the total cost with a label, a dollar sign, and exactly two decimal places. Sample output: Total cost: $22.5
- Assume:
height1 = 67850 height2 = 456
Use Python formatting to write two print statements that will produce the following output exactly at it appears below:

Homework Assignment: s2020py03
Download the assignment from the website, fill out the word document, and upload to your Google Drive folder the completed assignment along with the two python files.
1. (5 pts) Write a Python program that prompts the user for two numbers, and then gives the sum and product of those two numbers. Your sample output should look like this:
Enter your first number:10 Enter your second number:2 The sum of these numbers is: 12 The product of these two numbers is: 20
- Your program must contain documentation lines that include your name, the date, a line that states "Py03 Homework question 1" and a description line that indicates what the program is supposed to do.
- Paste the code this word document and upload to your Google drive when the assignment is completed, with file name [your last name]_py03_HWQ1
- Save the program as a python file (ends with .py), with file name [your last name]_py03Q1_program and upload that to the Google Drive.
2. (10 pts) Write a program that calculates the molarity of a solution. Molarity is defined as numbers of moles per liter solvent. Your program will calculate molarity and must ask for the substance name, its molecular weight, how many grams of substance you are putting in solution, and the total volume of the solution. Report your calculated value of molarity to 3 decimal places. Your output should also be separated from the input with a line containing 80 asterixis.
Assuming you are using sodium chloride, your input and output should look like:
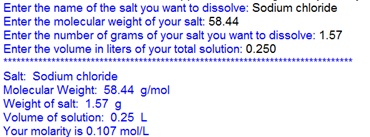
- Your program must contain documentation lines that include your name, the date, a line that states "Py03 Homework question 2" and a description line that indicates what the program is supposed to do.
- Paste the code to question two below
- Save the program as a python file (ends with .py), with file name [your last name]_py03Q2_program and upload that to the Google Drive.
3. (4 pts) Make two hypothes.is annotations dealing with external open access resources on formatting with the format function method of formatting. These need the tag of s20iostpy03ualr .
Copyright Statement

Assignment Statement
The assignment statement is an instruction that stores a value in a variable . You use this instruction any time you want to update the value of a variable.
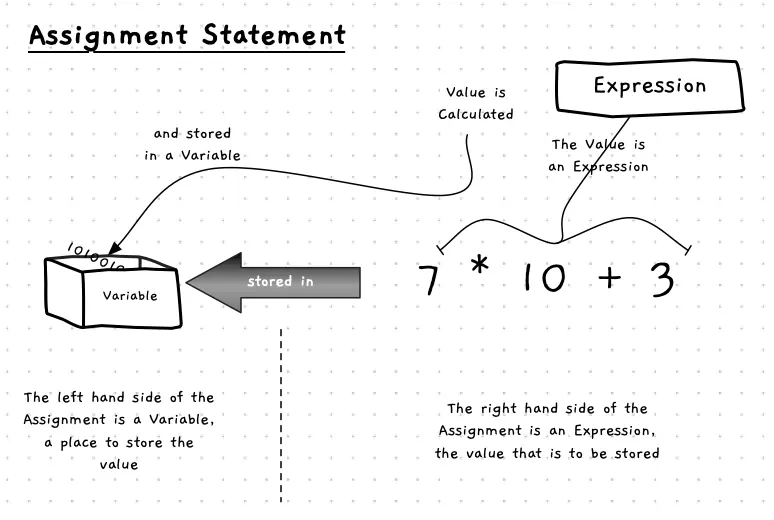
The assignment statement performs two actions. First, it calculates the value of the expression (calculation) on the right-hand side of the assignment operator (the = ). Once it has the value, it stores the value (assigns it) to the variable on the left-hand side of the assignment operator.
Assignment Statement — when, why, and how
When you create a variable, you have identified a piece of information that you want to be able to change as your program runs. Whenever you need to give a variable an initial or new value, you use an assignment statement .
The assignment statement uses the assignment operator = . Whatever is on the right-hand side of = represents the value to be assigned. This could be a literal , a method call , or any other expression . On the left-hand side you write the identifier of the variable you want to store this value in.
For example, you might decide to ask the user for their name. First, you need a variable to store the value. You might decide to call this variable name . Then, the assignment statement lets you read a response from the user and store it in that variable. In this case, the right-hand side of the assignment would be a call to ReadLine , which reads input from standard in and returns it to you. The left-hand side would be the identifier of our variable, name .
It is important to remember that every assignment statement has 2 actions :
- Calculate the value on the right-hand side
- Store it in the variable on the left-hand side.
The ordering of these actions allow you to update the value of a variable using an expression involving the variable being updated. This can be very useful. For example, you might want to update the value of a variable storing the number of steps you have taken today.
In C# the assignment operator is = . Most assignment statements are written using = , with an identifier on the left-hand side and an expression on the right-hand side. The assignment operator can optionally be modified with + , - , * , or / , which are shorthands for adding to, subtracting from, multiplying, and dividing the variable identified on the left-hand side of the statement.
Some assignment statements are written without = . These are assignment statements using increment ( ++ ) or decrement ( -- ), which allow you to add or remove one from a variable’s current value.
For example, x = x - 1 , x -= 1 , and x-- are all assignment statements which do the same thing — assign the variable x a new value that is one lower than its current value.
Basic assignment statement
In this example we use ReadLine to get input from the user and store it in a name variable.
Shorthand assignment statements
The following code shows an example of how to use some of the shorthand assignment statements.
If you ran the above code and entered 17 as the start count you should get this output:
You do not always need to store values in variables. Sometimes you can just use the value and then forget it. For example, in the above code, we read the initial count from the user. This requires us to read it as text, and then convert that text to a number. Given that we do not ever use the details in line again, we do not need to create this variable in the first place. Instead, we could pass the value to the convert function directly as shown below.
Assignment statement up close
The following sliders show how the assignment statement works in detail. These are both relatively simple programs, but notice how much is going on behind the scenes!
Assigning an int division result to an int variable
Assigning an int division result to a double variable.
Assignment Statements
Assignment statements in a program come in two forms – with and without mutations. Assignments without mutation are those that give a value to a variable without using the old value of that variable. Assignments with mutation are variable assignments that use the old value of a variable to calculate a value for the variable.
For example, an increment statement like x = x + 1 MUTATES the value of x by updating its value to be one bigger than it was before. In order to make sense of such a statement, we need to know the previous value of x .
In contrast, a statement like y = x + 1 assigns to y one more than the value in x . We do not need to know the previous value of y , as we are not using it in the assignment statement. (We do need to know the value of x ).
Assignments without mutation
We have already seen the steps necessary to process assignment statements that do not involve variable mutation. Recall that we can declare as a premise any assignment statement or claim from a previous logic block involving variables that have not since changed.
For example, suppose we want to verify the following program so the assert statement at the end will hold:
Since none of the statements involve variable mutation, we can do the verification in a single logic block:
Note that we did need to do ∧i so that the last claim was y == z ∧ y == 6 , even though we had previously established the claims y == z and y == 6 . In order for an assert to hold (at least until we switch Logika modes in chapter 10), we need to have established EXACTLY the claim in the assert in a previous logic block.
Assignments with mutation
Assignments with mutation are trickier – we need to know the old value of a variable in order to reason about its new value. For example, if we have the following program:
Then we might try to add the following logic blocks:
…but then we get stuck in the second logic block. There, x is supposed to refer to the CURRENT value of x (after being incremented), but both our attempted claims are untrue. The current value of x is not one more than itself (this makes no sense!), and we can tell from reading the code that x is now 5, not 4.
To help reason about changing variables, Logika has a special name_old value that refers to the OLD value of a variable called name , just before the latest update. In the example above, we can use x_old in the second logic block to refer to x ’s value just before it was incremented. We can now change our premises and finish the verification as follows:
By the end of the logic block following a variable mutation, we need to express everything we know about the variable’s current value WITHOUT using the _old terminology, as its scope will end when the logic block ends. Moreover, we only ever have one _old value available in a logic block – the variable that was most recently changed. This means we will need logic blocks after each variable mutation to process the changes to any related facts.
Variable swap example
Suppose we have the following Logika program:
We can see that this program gets two user input values, x and y , and then swaps their values. So if x was originally 4 and y was originally 6, then at the end of the program x would be 6 and y would be 4.
We would like to be able to assert what we did – that x now has the original value from y , and that y now has the original value from x . To do this, we might invent dummy constants called xOrig and yOrig that represent the original values of those variables. Then we can add our assert:
We can complete the verification by adding logic blocks after assignment statements, being careful to update all we know (without using the _old value) by the end of each block:
Notice that in each logic block, we express as much as we can about all variables/values in the program. In the first logic block, even though xOrig and yOrig were not used in the previous assignment statement, we still expressed how the current values our other variables compared to xOrig and yOrig . It helps to think about what you are trying to claim in the final assert – since our assert involved xOrig and yOrig , we needed to relate the current values of our variables to those values as we progressed through the program.
Last modified by: Julie Thornton Nov 15, 2023

1.7 Java | Assignment Statements & Expressions
An assignment statement designates a value for a variable. An assignment statement can be used as an expression in Java.
After a variable is declared, you can assign a value to it by using an assignment statement . In Java, the equal sign = is used as the assignment operator . The syntax for assignment statements is as follows:
An expression represents a computation involving values, variables, and operators that, when taking them together, evaluates to a value. For example, consider the following code:
You can use a variable in an expression. A variable can also be used on both sides of the = operator. For example:
In the above assignment statement, the result of x + 1 is assigned to the variable x . Let’s say that x is 1 before the statement is executed, and so becomes 2 after the statement execution.
To assign a value to a variable, you must place the variable name to the left of the assignment operator. Thus the following statement is wrong:
Note that the math equation x = 2 * x + 1 ≠ the Java expression x = 2 * x + 1
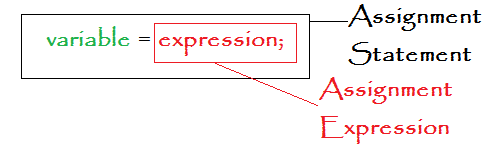
Which is equivalent to:
And this statement
is equivalent to:
Note: The data type of a variable on the left must be compatible with the data type of a value on the right. For example, int x = 1.0 would be illegal, because the data type of x is int (integer) and does not accept the double value 1.0 without Type Casting .
◄◄◄BACK | NEXT►►►
What's Your Opinion? Cancel reply
Enhance your Brain
Subscribe to Receive Free Bio Hacking, Nootropic, and Health Information
HTML for Simple Website Customization My Personal Web Customization Personal Insights
DISCLAIMER | Sitemap | ◘
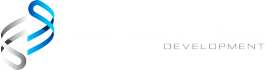
HTML for Simple Website Customization My Personal Web Customization Personal Insights SEO Checklist Publishing Checklist My Tools
Top Posts & Pages

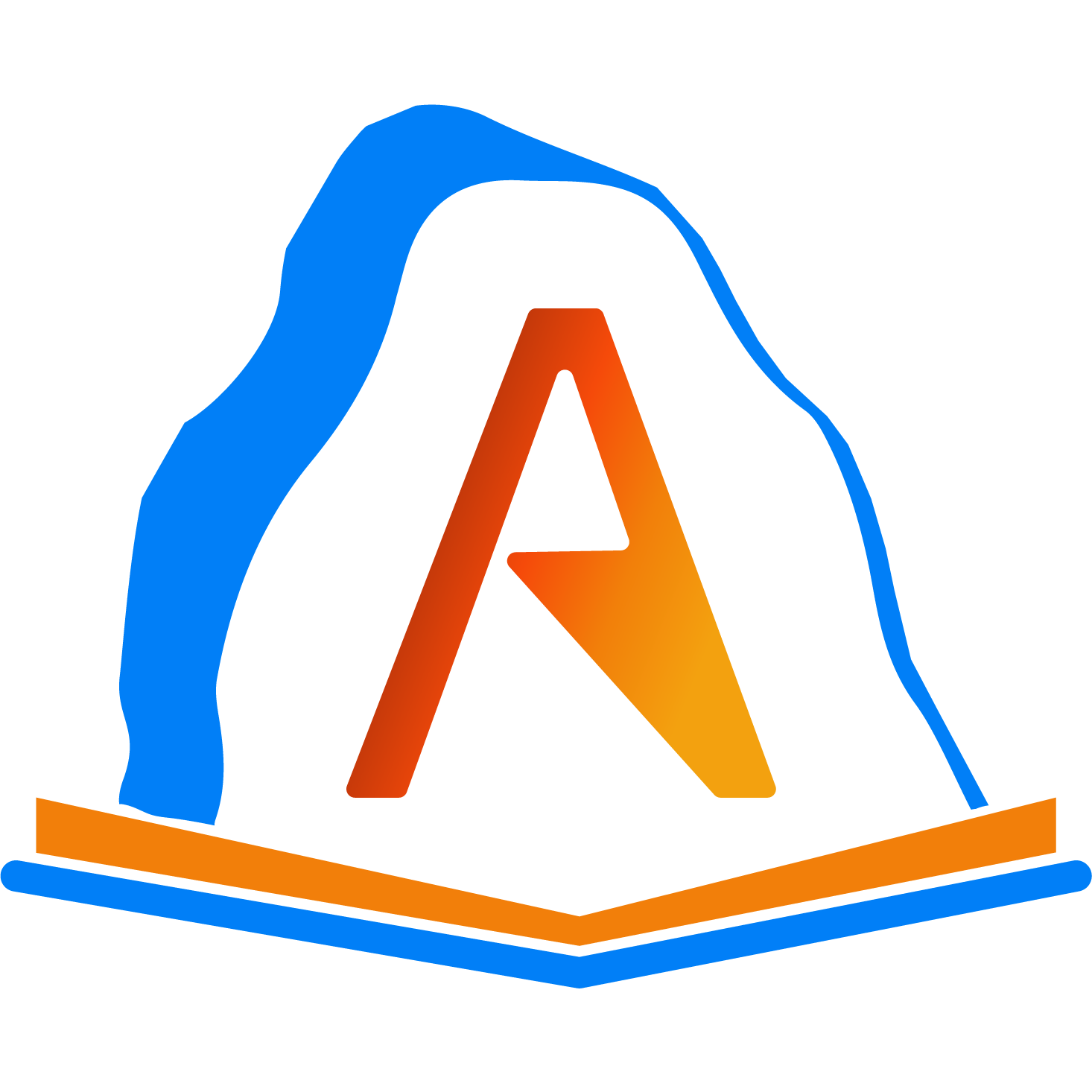
- Table of Contents
- Course Home
- Assignments
- Peer Instruction (Instructor)
- Peer Instruction (Student)
- Change Course
- Instructor's Page
- Progress Page
- Edit Profile
- Change Password
- Scratch ActiveCode
- Scratch Activecode
- Instructors Guide
- About Runestone
- Report A Problem
- 1.1 Preface
- 1.2 Why Programming? Why Java?
- 1.3 Variables and Data Types
- 1.4 Expressions and Assignment Statements
- 1.5 Compound Assignment Operators
- 1.6 Casting and Ranges of Variables
- 1.7 Java Development Environments (optional)
- 1.8 Unit 1 Summary
- 1.9 Unit 1 Mixed Up Code Practice
- 1.10 Unit 1 Coding Practice
- 1.11 Multiple Choice Exercises
- 1.12 Lesson Workspace
- 1.3. Variables and Data Types" data-toggle="tooltip">
- 1.5. Compound Assignment Operators' data-toggle="tooltip" >
1.4. Expressions and Assignment Statements ¶
In this lesson, you will learn about assignment statements and expressions that contain math operators and variables.
1.4.1. Assignment Statements ¶
Remember that a variable holds a value that can change or vary. Assignment statements initialize or change the value stored in a variable using the assignment operator = . An assignment statement always has a single variable on the left hand side of the = sign. The value of the expression on the right hand side of the = sign (which can contain math operators and other variables) is copied into the memory location of the variable on the left hand side.
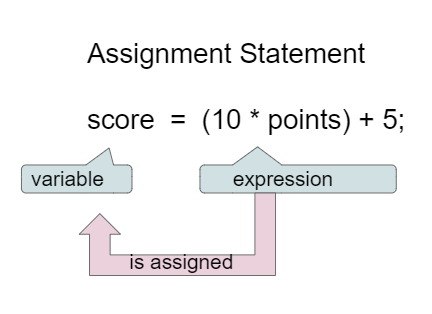
Figure 1: Assignment Statement (variable = expression) ¶
Instead of saying equals for the = operator in an assignment statement, say “gets” or “is assigned” to remember that the variable on the left hand side gets or is assigned the value on the right. In the figure above, score is assigned the value of 10 times points (which is another variable) plus 5.
The following video by Dr. Colleen Lewis shows how variables can change values in memory using assignment statements.
As we saw in the video, we can set one variable to a copy of the value of another variable like y = x;. This won’t change the value of the variable that you are copying from.

Click on the Show CodeLens button to step through the code and see how the values of the variables change.
The program is supposed to figure out the total money value given the number of dimes, quarters and nickels. There is an error in the calculation of the total. Fix the error to compute the correct amount.
Calculate and print the total pay given the weekly salary and the number of weeks worked. Use string concatenation with the totalPay variable to produce the output Total Pay = $3000 . Don’t hardcode the number 3000 in your print statement.

Assume you have a package with a given height 3 inches and width 5 inches. If the package is rotated 90 degrees, you should swap the values for the height and width. The code below makes an attempt to swap the values stored in two variables h and w, which represent height and width. Variable h should end up with w’s initial value of 5 and w should get h’s initial value of 3. Unfortunately this code has an error and does not work. Use the CodeLens to step through the code to understand why it fails to swap the values in h and w.
1-4-7: Explain in your own words why the ErrorSwap program code does not swap the values stored in h and w.
Swapping two variables requires a third variable. Before assigning h = w , you need to store the original value of h in the temporary variable. In the mixed up programs below, drag the blocks to the right to put them in the right order.
The following has the correct code that uses a third variable named “temp” to swap the values in h and w.
The code is mixed up and contains one extra block which is not needed in a correct solution. Drag the needed blocks from the left into the correct order on the right, then check your solution. You will be told if any of the blocks are in the wrong order or if you need to remove one or more blocks.
After three incorrect attempts you will be able to use the Help Me button to make the problem easier.
Fix the code below to perform a correct swap of h and w. You need to add a new variable named temp to use for the swap.
1.4.2. Incrementing the value of a variable ¶
If you use a variable to keep score you would probably increment it (add one to the current value) whenever score should go up. You can do this by setting the variable to the current value of the variable plus one (score = score + 1) as shown below. The formula looks a little crazy in math class, but it makes sense in coding because the variable on the left is set to the value of the arithmetic expression on the right. So, the score variable is set to the previous value of score + 1.
Click on the Show CodeLens button to step through the code and see how the score value changes.
1-4-11: What is the value of b after the following code executes?
- It sets the value for the variable on the left to the value from evaluating the right side. What is 5 * 2?
- Correct. 5 * 2 is 10.
1-4-12: What are the values of x, y, and z after the following code executes?
- x = 0, y = 1, z = 2
- These are the initial values in the variable, but the values are changed.
- x = 1, y = 2, z = 3
- x changes to y's initial value, y's value is doubled, and z is set to 3
- x = 2, y = 2, z = 3
- Remember that the equal sign doesn't mean that the two sides are equal. It sets the value for the variable on the left to the value from evaluating the right side.
- x = 1, y = 0, z = 3
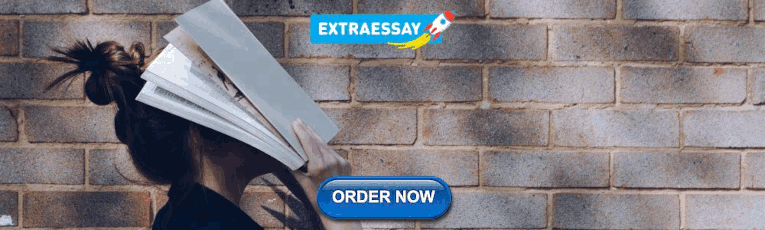
1.4.3. Operators ¶
Java uses the standard mathematical operators for addition ( + ), subtraction ( - ), multiplication ( * ), and division ( / ). Arithmetic expressions can be of type int or double. An arithmetic operation that uses two int values will evaluate to an int value. An arithmetic operation that uses at least one double value will evaluate to a double value. (You may have noticed that + was also used to put text together in the input program above – more on this when we talk about strings.)
Java uses the operator == to test if the value on the left is equal to the value on the right and != to test if two items are not equal. Don’t get one equal sign = confused with two equal signs == ! They mean different things in Java. One equal sign is used to assign a value to a variable. Two equal signs are used to test a variable to see if it is a certain value and that returns true or false as you’ll see below. Use == and != only with int values and not doubles because double values are an approximation and 3.3333 will not equal 3.3334 even though they are very close.
Run the code below to see all the operators in action. Do all of those operators do what you expected? What about 2 / 3 ? Isn’t surprising that it prints 0 ? See the note below.
When Java sees you doing integer division (or any operation with integers) it assumes you want an integer result so it throws away anything after the decimal point in the answer, essentially rounding down the answer to a whole number. If you need a double answer, you should make at least one of the values in the expression a double like 2.0.
With division, another thing to watch out for is dividing by 0. An attempt to divide an integer by zero will result in an ArithmeticException error message. Try it in one of the active code windows above.
Operators can be used to create compound expressions with more than one operator. You can either use a literal value which is a fixed value like 2, or variables in them. When compound expressions are evaluated, operator precedence rules are used, so that *, /, and % are done before + and -. However, anything in parentheses is done first. It doesn’t hurt to put in extra parentheses if you are unsure as to what will be done first.
In the example below, try to guess what it will print out and then run it to see if you are right. Remember to consider operator precedence .
1-4-15: Consider the following code segment. Be careful about integer division.
What is printed when the code segment is executed?
- 0.666666666666667
- Don't forget that division and multiplication will be done first due to operator precedence.
- Yes, this is equivalent to (5 + ((a/b)*c) - 1).
- Don't forget that division and multiplication will be done first due to operator precedence, and that an int/int gives an int result where it is rounded down to the nearest int.
1-4-16: Consider the following code segment.
What is the value of the expression?
- Dividing an integer by an integer results in an integer
- Correct. Dividing an integer by an integer results in an integer
- The value 5.5 will be rounded down to 5
1-4-17: Consider the following code segment.
- Correct. Dividing a double by an integer results in a double
- Dividing a double by an integer results in a double
1-4-18: Consider the following code segment.
- Correct. Dividing an integer by an double results in a double
- Dividing an integer by an double results in a double
1.4.4. The Modulo Operator ¶
The percent sign operator ( % ) is the mod (modulo) or remainder operator. The mod operator ( x % y ) returns the remainder after you divide x (first number) by y (second number) so 5 % 2 will return 1 since 2 goes into 5 two times with a remainder of 1. Remember long division when you had to specify how many times one number went into another evenly and the remainder? That remainder is what is returned by the modulo operator.
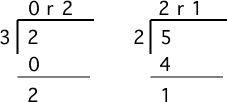
Figure 2: Long division showing the whole number result and the remainder ¶
In the example below, try to guess what it will print out and then run it to see if you are right.
The result of x % y when x is smaller than y is always x . The value y can’t go into x at all (goes in 0 times), since x is smaller than y , so the result is just x . So if you see 2 % 3 the result is 2 .
1-4-21: What is the result of 158 % 10?
- This would be the result of 158 divided by 10. modulo gives you the remainder.
- modulo gives you the remainder after the division.
- When you divide 158 by 10 you get a remainder of 8.
1-4-22: What is the result of 3 % 8?
- 8 goes into 3 no times so the remainder is 3. The remainder of a smaller number divided by a larger number is always the smaller number!
- This would be the remainder if the question was 8 % 3 but here we are asking for the reminder after we divide 3 by 8.
- What is the remainder after you divide 3 by 8?
1.4.5. FlowCharting ¶
Assume you have 16 pieces of pizza and 5 people. If everyone gets the same number of slices, how many slices does each person get? Are there any leftover pieces?
In industry, a flowchart is used to describe a process through symbols and text. A flowchart usually does not show variable declarations, but it can show assignment statements (drawn as rectangle) and output statements (drawn as rhomboid).
The flowchart in figure 3 shows a process to compute the fair distribution of pizza slices among a number of people. The process relies on integer division to determine slices per person, and the mod operator to determine remaining slices.
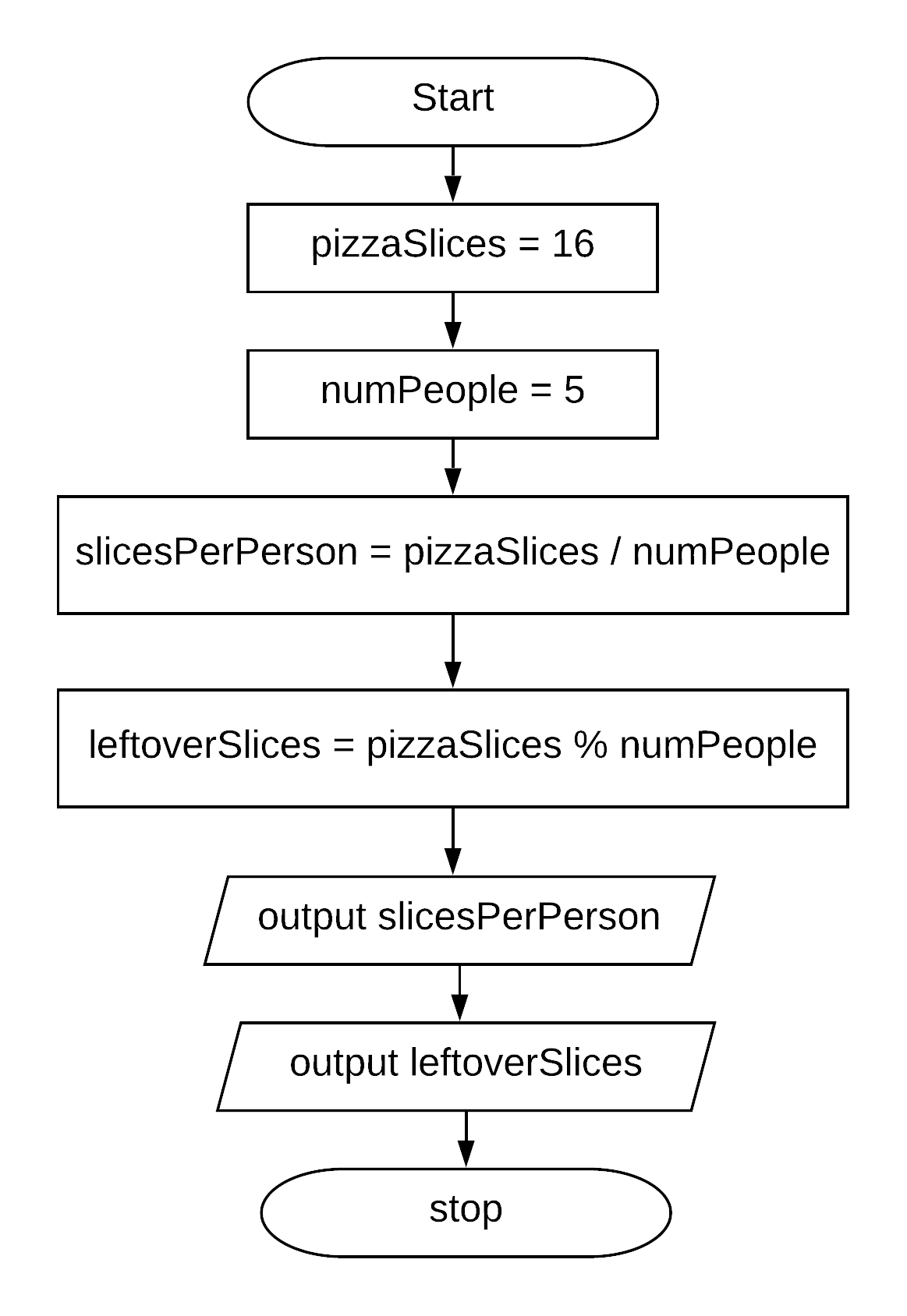
Figure 3: Example Flow Chart ¶
A flowchart shows pseudo-code, which is like Java but not exactly the same. Syntactic details like semi-colons are omitted, and input and output is described in abstract terms.
Complete the program based on the process shown in the Figure 3 flowchart. Note the first line of code declares all 4 variables as type int. Add assignment statements and print statements to compute and print the slices per person and leftover slices. Use System.out.println for output.
1.4.6. Storing User Input in Variables ¶
Variables are a powerful abstraction in programming because the same algorithm can be used with different input values saved in variables.

Figure 4: Program input and output ¶
A Java program can ask the user to type in one or more values. The Java class Scanner is used to read from the keyboard input stream, which is referenced by System.in . Normally the keyboard input is typed into a console window, but since this is running in a browser you will type in a small textbox window displayed below the code. The code below shows an example of prompting the user to enter a name and then printing a greeting. The code String name = scan.nextLine() gets the string value you enter as program input and then stores the value in a variable.
Run the program a few times, typing in a different name. The code works for any name: behold, the power of variables!
Run this program to read in a name from the input stream. You can type a different name in the input window shown below the code.
Try stepping through the code with the CodeLens tool to see how the name variable is assigned to the value read by the scanner. You will have to click “Hide CodeLens” and then “Show in CodeLens” to enter a different name for input.
The Scanner class has several useful methods for reading user input. A token is a sequence of characters separated by white space.
Run this program to read in an integer from the input stream. You can type a different integer value in the input window shown below the code.
A rhomboid (slanted rectangle) is used in a flowchart to depict data flowing into and out of a program. The previous flowchart in Figure 3 used a rhomboid to indicate program output. A rhomboid is also used to denote reading a value from the input stream.
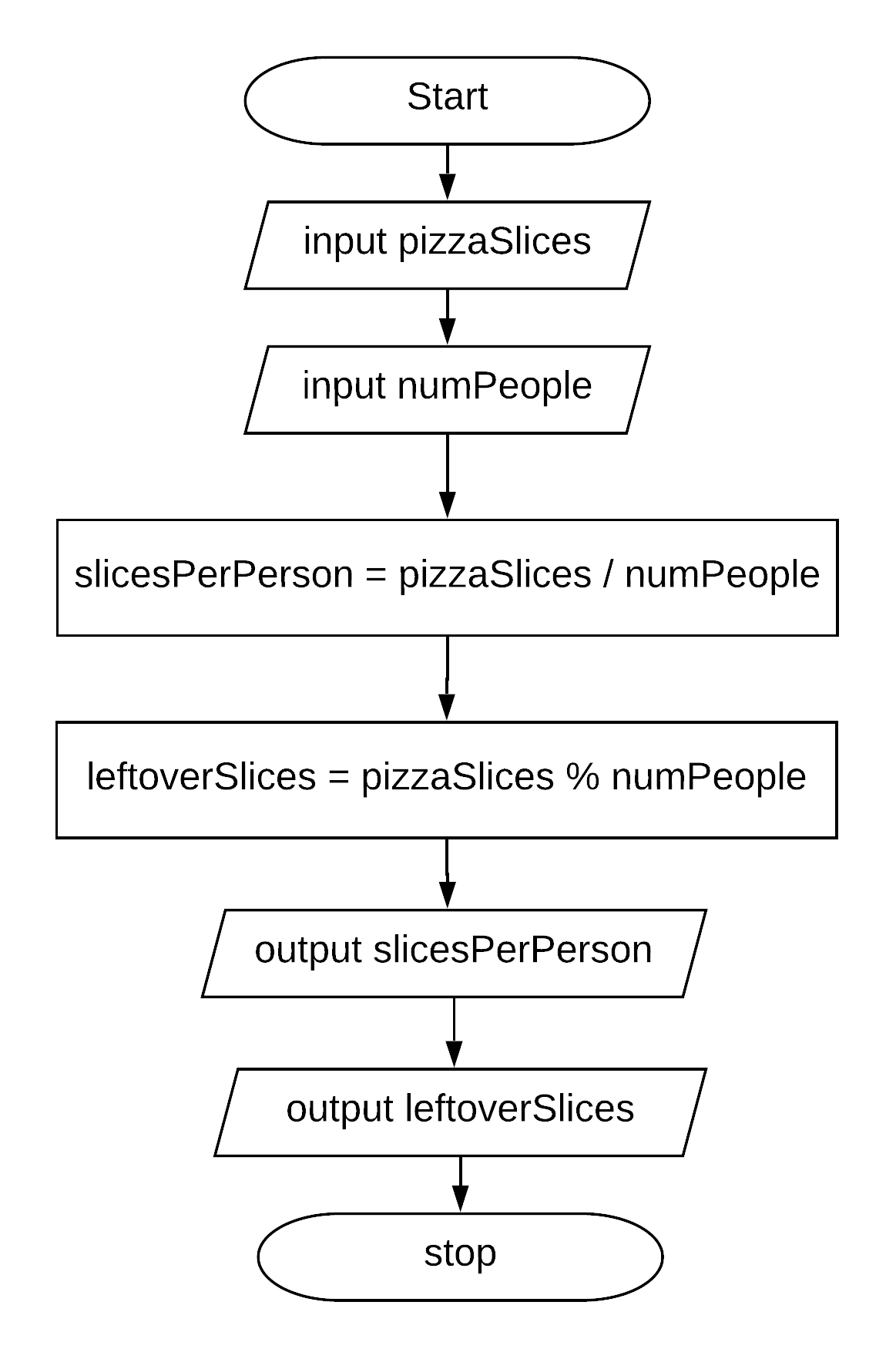
Figure 5: Flow Chart Reading User Input ¶
Figure 5 contains an updated version of the pizza calculator process. The first two steps have been altered to initialize the pizzaSlices and numPeople variables by reading two values from the input stream. In Java this will be done using a Scanner object and reading from System.in.
Complete the program based on the process shown in the Figure 5 flowchart. The program should scan two integer values to initialize pizzaSlices and numPeople. Run the program a few times to experiment with different values for input. What happens if you enter 0 for the number of people? The program will bomb due to division by zero! We will see how to prevent this in a later lesson.
The program below reads two integer values from the input stream and attempts to print the sum. Unfortunately there is a problem with the last line of code that prints the sum.
Run the program and look at the result. When the input is 5 and 7 , the output is Sum is 57 . Both of the + operators in the print statement are performing string concatenation. While the first + operator should perform string concatenation, the second + operator should perform addition. You can force the second + operator to perform addition by putting the arithmetic expression in parentheses ( num1 + num2 ) .
More information on using the Scanner class can be found here https://www.w3schools.com/java/java_user_input.asp
1.4.7. Programming Challenge : Dog Years ¶
In this programming challenge, you will calculate your age, and your pet’s age from your birthdates, and your pet’s age in dog years. In the code below, type in the current year, the year you were born, the year your dog or cat was born (if you don’t have one, make one up!) in the variables below. Then write formulas in assignment statements to calculate how old you are, how old your dog or cat is, and how old they are in dog years which is 7 times a human year. Finally, print it all out.
Calculate your age and your pet’s age from the birthdates, and then your pet’s age in dog years. If you want an extra challenge, try reading the values using a Scanner.
1.4.8. Summary ¶
Arithmetic expressions include expressions of type int and double.
The arithmetic operators consist of +, -, * , /, and % (modulo for the remainder in division).
An arithmetic operation that uses two int values will evaluate to an int value. With integer division, any decimal part in the result will be thrown away, essentially rounding down the answer to a whole number.
An arithmetic operation that uses at least one double value will evaluate to a double value.
Operators can be used to construct compound expressions.
During evaluation, operands are associated with operators according to operator precedence to determine how they are grouped. (*, /, % have precedence over + and -, unless parentheses are used to group those.)
An attempt to divide an integer by zero will result in an ArithmeticException to occur.
The assignment operator (=) allows a program to initialize or change the value stored in a variable. The value of the expression on the right is stored in the variable on the left.
During execution, expressions are evaluated to produce a single value.
The value of an expression has a type based on the evaluation of the expression.

Understanding Assignments
What this handout is about.
The first step in any successful college writing venture is reading the assignment. While this sounds like a simple task, it can be a tough one. This handout will help you unravel your assignment and begin to craft an effective response. Much of the following advice will involve translating typical assignment terms and practices into meaningful clues to the type of writing your instructor expects. See our short video for more tips.
Basic beginnings
Regardless of the assignment, department, or instructor, adopting these two habits will serve you well :
- Read the assignment carefully as soon as you receive it. Do not put this task off—reading the assignment at the beginning will save you time, stress, and problems later. An assignment can look pretty straightforward at first, particularly if the instructor has provided lots of information. That does not mean it will not take time and effort to complete; you may even have to learn a new skill to complete the assignment.
- Ask the instructor about anything you do not understand. Do not hesitate to approach your instructor. Instructors would prefer to set you straight before you hand the paper in. That’s also when you will find their feedback most useful.
Assignment formats
Many assignments follow a basic format. Assignments often begin with an overview of the topic, include a central verb or verbs that describe the task, and offer some additional suggestions, questions, or prompts to get you started.
An Overview of Some Kind
The instructor might set the stage with some general discussion of the subject of the assignment, introduce the topic, or remind you of something pertinent that you have discussed in class. For example:
“Throughout history, gerbils have played a key role in politics,” or “In the last few weeks of class, we have focused on the evening wear of the housefly …”
The Task of the Assignment
Pay attention; this part tells you what to do when you write the paper. Look for the key verb or verbs in the sentence. Words like analyze, summarize, or compare direct you to think about your topic in a certain way. Also pay attention to words such as how, what, when, where, and why; these words guide your attention toward specific information. (See the section in this handout titled “Key Terms” for more information.)
“Analyze the effect that gerbils had on the Russian Revolution”, or “Suggest an interpretation of housefly undergarments that differs from Darwin’s.”
Additional Material to Think about
Here you will find some questions to use as springboards as you begin to think about the topic. Instructors usually include these questions as suggestions rather than requirements. Do not feel compelled to answer every question unless the instructor asks you to do so. Pay attention to the order of the questions. Sometimes they suggest the thinking process your instructor imagines you will need to follow to begin thinking about the topic.
“You may wish to consider the differing views held by Communist gerbils vs. Monarchist gerbils, or Can there be such a thing as ‘the housefly garment industry’ or is it just a home-based craft?”
These are the instructor’s comments about writing expectations:
“Be concise”, “Write effectively”, or “Argue furiously.”
Technical Details
These instructions usually indicate format rules or guidelines.
“Your paper must be typed in Palatino font on gray paper and must not exceed 600 pages. It is due on the anniversary of Mao Tse-tung’s death.”
The assignment’s parts may not appear in exactly this order, and each part may be very long or really short. Nonetheless, being aware of this standard pattern can help you understand what your instructor wants you to do.
Interpreting the assignment
Ask yourself a few basic questions as you read and jot down the answers on the assignment sheet:
Why did your instructor ask you to do this particular task?
Who is your audience.
- What kind of evidence do you need to support your ideas?
What kind of writing style is acceptable?
- What are the absolute rules of the paper?
Try to look at the question from the point of view of the instructor. Recognize that your instructor has a reason for giving you this assignment and for giving it to you at a particular point in the semester. In every assignment, the instructor has a challenge for you. This challenge could be anything from demonstrating an ability to think clearly to demonstrating an ability to use the library. See the assignment not as a vague suggestion of what to do but as an opportunity to show that you can handle the course material as directed. Paper assignments give you more than a topic to discuss—they ask you to do something with the topic. Keep reminding yourself of that. Be careful to avoid the other extreme as well: do not read more into the assignment than what is there.
Of course, your instructor has given you an assignment so that he or she will be able to assess your understanding of the course material and give you an appropriate grade. But there is more to it than that. Your instructor has tried to design a learning experience of some kind. Your instructor wants you to think about something in a particular way for a particular reason. If you read the course description at the beginning of your syllabus, review the assigned readings, and consider the assignment itself, you may begin to see the plan, purpose, or approach to the subject matter that your instructor has created for you. If you still aren’t sure of the assignment’s goals, try asking the instructor. For help with this, see our handout on getting feedback .
Given your instructor’s efforts, it helps to answer the question: What is my purpose in completing this assignment? Is it to gather research from a variety of outside sources and present a coherent picture? Is it to take material I have been learning in class and apply it to a new situation? Is it to prove a point one way or another? Key words from the assignment can help you figure this out. Look for key terms in the form of active verbs that tell you what to do.
Key Terms: Finding Those Active Verbs
Here are some common key words and definitions to help you think about assignment terms:
Information words Ask you to demonstrate what you know about the subject, such as who, what, when, where, how, and why.
- define —give the subject’s meaning (according to someone or something). Sometimes you have to give more than one view on the subject’s meaning
- describe —provide details about the subject by answering question words (such as who, what, when, where, how, and why); you might also give details related to the five senses (what you see, hear, feel, taste, and smell)
- explain —give reasons why or examples of how something happened
- illustrate —give descriptive examples of the subject and show how each is connected with the subject
- summarize —briefly list the important ideas you learned about the subject
- trace —outline how something has changed or developed from an earlier time to its current form
- research —gather material from outside sources about the subject, often with the implication or requirement that you will analyze what you have found
Relation words Ask you to demonstrate how things are connected.
- compare —show how two or more things are similar (and, sometimes, different)
- contrast —show how two or more things are dissimilar
- apply—use details that you’ve been given to demonstrate how an idea, theory, or concept works in a particular situation
- cause —show how one event or series of events made something else happen
- relate —show or describe the connections between things
Interpretation words Ask you to defend ideas of your own about the subject. Do not see these words as requesting opinion alone (unless the assignment specifically says so), but as requiring opinion that is supported by concrete evidence. Remember examples, principles, definitions, or concepts from class or research and use them in your interpretation.
- assess —summarize your opinion of the subject and measure it against something
- prove, justify —give reasons or examples to demonstrate how or why something is the truth
- evaluate, respond —state your opinion of the subject as good, bad, or some combination of the two, with examples and reasons
- support —give reasons or evidence for something you believe (be sure to state clearly what it is that you believe)
- synthesize —put two or more things together that have not been put together in class or in your readings before; do not just summarize one and then the other and say that they are similar or different—you must provide a reason for putting them together that runs all the way through the paper
- analyze —determine how individual parts create or relate to the whole, figure out how something works, what it might mean, or why it is important
- argue —take a side and defend it with evidence against the other side
More Clues to Your Purpose As you read the assignment, think about what the teacher does in class:
- What kinds of textbooks or coursepack did your instructor choose for the course—ones that provide background information, explain theories or perspectives, or argue a point of view?
- In lecture, does your instructor ask your opinion, try to prove her point of view, or use keywords that show up again in the assignment?
- What kinds of assignments are typical in this discipline? Social science classes often expect more research. Humanities classes thrive on interpretation and analysis.
- How do the assignments, readings, and lectures work together in the course? Instructors spend time designing courses, sometimes even arguing with their peers about the most effective course materials. Figuring out the overall design to the course will help you understand what each assignment is meant to achieve.
Now, what about your reader? Most undergraduates think of their audience as the instructor. True, your instructor is a good person to keep in mind as you write. But for the purposes of a good paper, think of your audience as someone like your roommate: smart enough to understand a clear, logical argument, but not someone who already knows exactly what is going on in your particular paper. Remember, even if the instructor knows everything there is to know about your paper topic, he or she still has to read your paper and assess your understanding. In other words, teach the material to your reader.
Aiming a paper at your audience happens in two ways: you make decisions about the tone and the level of information you want to convey.
- Tone means the “voice” of your paper. Should you be chatty, formal, or objective? Usually you will find some happy medium—you do not want to alienate your reader by sounding condescending or superior, but you do not want to, um, like, totally wig on the man, you know? Eschew ostentatious erudition: some students think the way to sound academic is to use big words. Be careful—you can sound ridiculous, especially if you use the wrong big words.
- The level of information you use depends on who you think your audience is. If you imagine your audience as your instructor and she already knows everything you have to say, you may find yourself leaving out key information that can cause your argument to be unconvincing and illogical. But you do not have to explain every single word or issue. If you are telling your roommate what happened on your favorite science fiction TV show last night, you do not say, “First a dark-haired white man of average height, wearing a suit and carrying a flashlight, walked into the room. Then a purple alien with fifteen arms and at least three eyes turned around. Then the man smiled slightly. In the background, you could hear a clock ticking. The room was fairly dark and had at least two windows that I saw.” You also do not say, “This guy found some aliens. The end.” Find some balance of useful details that support your main point.
You’ll find a much more detailed discussion of these concepts in our handout on audience .
The Grim Truth
With a few exceptions (including some lab and ethnography reports), you are probably being asked to make an argument. You must convince your audience. It is easy to forget this aim when you are researching and writing; as you become involved in your subject matter, you may become enmeshed in the details and focus on learning or simply telling the information you have found. You need to do more than just repeat what you have read. Your writing should have a point, and you should be able to say it in a sentence. Sometimes instructors call this sentence a “thesis” or a “claim.”
So, if your instructor tells you to write about some aspect of oral hygiene, you do not want to just list: “First, you brush your teeth with a soft brush and some peanut butter. Then, you floss with unwaxed, bologna-flavored string. Finally, gargle with bourbon.” Instead, you could say, “Of all the oral cleaning methods, sandblasting removes the most plaque. Therefore it should be recommended by the American Dental Association.” Or, “From an aesthetic perspective, moldy teeth can be quite charming. However, their joys are short-lived.”
Convincing the reader of your argument is the goal of academic writing. It doesn’t have to say “argument” anywhere in the assignment for you to need one. Look at the assignment and think about what kind of argument you could make about it instead of just seeing it as a checklist of information you have to present. For help with understanding the role of argument in academic writing, see our handout on argument .
What kind of evidence do you need?
There are many kinds of evidence, and what type of evidence will work for your assignment can depend on several factors–the discipline, the parameters of the assignment, and your instructor’s preference. Should you use statistics? Historical examples? Do you need to conduct your own experiment? Can you rely on personal experience? See our handout on evidence for suggestions on how to use evidence appropriately.
Make sure you are clear about this part of the assignment, because your use of evidence will be crucial in writing a successful paper. You are not just learning how to argue; you are learning how to argue with specific types of materials and ideas. Ask your instructor what counts as acceptable evidence. You can also ask a librarian for help. No matter what kind of evidence you use, be sure to cite it correctly—see the UNC Libraries citation tutorial .
You cannot always tell from the assignment just what sort of writing style your instructor expects. The instructor may be really laid back in class but still expect you to sound formal in writing. Or the instructor may be fairly formal in class and ask you to write a reflection paper where you need to use “I” and speak from your own experience.
Try to avoid false associations of a particular field with a style (“art historians like wacky creativity,” or “political scientists are boring and just give facts”) and look instead to the types of readings you have been given in class. No one expects you to write like Plato—just use the readings as a guide for what is standard or preferable to your instructor. When in doubt, ask your instructor about the level of formality she or he expects.
No matter what field you are writing for or what facts you are including, if you do not write so that your reader can understand your main idea, you have wasted your time. So make clarity your main goal. For specific help with style, see our handout on style .
Technical details about the assignment
The technical information you are given in an assignment always seems like the easy part. This section can actually give you lots of little hints about approaching the task. Find out if elements such as page length and citation format (see the UNC Libraries citation tutorial ) are negotiable. Some professors do not have strong preferences as long as you are consistent and fully answer the assignment. Some professors are very specific and will deduct big points for deviations.
Usually, the page length tells you something important: The instructor thinks the size of the paper is appropriate to the assignment’s parameters. In plain English, your instructor is telling you how many pages it should take for you to answer the question as fully as you are expected to. So if an assignment is two pages long, you cannot pad your paper with examples or reword your main idea several times. Hit your one point early, defend it with the clearest example, and finish quickly. If an assignment is ten pages long, you can be more complex in your main points and examples—and if you can only produce five pages for that assignment, you need to see someone for help—as soon as possible.
Tricks that don’t work
Your instructors are not fooled when you:
- spend more time on the cover page than the essay —graphics, cool binders, and cute titles are no replacement for a well-written paper.
- use huge fonts, wide margins, or extra spacing to pad the page length —these tricks are immediately obvious to the eye. Most instructors use the same word processor you do. They know what’s possible. Such tactics are especially damning when the instructor has a stack of 60 papers to grade and yours is the only one that low-flying airplane pilots could read.
- use a paper from another class that covered “sort of similar” material . Again, the instructor has a particular task for you to fulfill in the assignment that usually relates to course material and lectures. Your other paper may not cover this material, and turning in the same paper for more than one course may constitute an Honor Code violation . Ask the instructor—it can’t hurt.
- get all wacky and “creative” before you answer the question . Showing that you are able to think beyond the boundaries of a simple assignment can be good, but you must do what the assignment calls for first. Again, check with your instructor. A humorous tone can be refreshing for someone grading a stack of papers, but it will not get you a good grade if you have not fulfilled the task.
Critical reading of assignments leads to skills in other types of reading and writing. If you get good at figuring out what the real goals of assignments are, you are going to be better at understanding the goals of all of your classes and fields of study.
You may reproduce it for non-commercial use if you use the entire handout and attribute the source: The Writing Center, University of North Carolina at Chapel Hill
Make a Gift
CS105: Introduction to Python
Variables and assignment statements.
Computers must be able to remember and store data. This can be accomplished by creating a variable to house a given value. The assignment operator = is used to associate a variable name with a given value. For example, type the command:
in the command line window. This command assigns the value 3.45 to the variable named a . Next, type the command:
in the command window and hit the enter key. You should see the value contained in the variable a echoed to the screen. This variable will remember the value 3.45 until it is assigned a different value. To see this, type these two commands:
You should see the new value contained in the variable a echoed to the screen. The new value has "overwritten" the old value. We must be careful since once an old value has been overwritten, it is no longer remembered. The new value is now what is being remembered.
Although we will not discuss arithmetic operations in detail until the next unit, you can at least be equipped with the syntax for basic operations: + (addition), - (subtraction), * (multiplication), / (division)
For example, entering these command sequentially into the command line window:
would result in 12.32 being echoed to the screen (just as you would expect from a calculator). The syntax for multiplication works similarly. For example:
would result in 35 being echoed to the screen because the variable b has been assigned the value a * 5 where, at the time of execution, the variable a contains a value of 7.
After you read, you should be able to execute simple assignment commands using integer and float values in the command window of the Repl.it IDE. Try typing some more of the examples from this web page to convince yourself that a variable has been assigned a specific value.
In programming, we associate names with values so that we can remember and use them later. Recall Example 1. The repeated computation in that algorithm relied on remembering the intermediate sum and the integer to be added to that sum to get the new sum. In expressing the algorithm, we used th e names current and sum .
In programming, a name that refers to a value in this fashion is called a variable . When we think of values as data stored somewhere i n the computer, we can have a mental image such as the one below for the value 10 stored in the computer and the variable x , which is the name we give to 10. What is most important is to see that there is a binding between x and 10.
The term variable comes from the fact that values that are bound to variables can change throughout computation. Bindings as shown above are created, and changed by assignment statements . An assignment statement associates the name to the left of the symbol = with the value denoted by the expression on the right of =. The binding in the picture is created using an assignment statemen t of the form x = 10 . We usually read such an assignment statement as "10 is assigned to x" or "x is set to 10".
If we want to change the value that x refers to, we can use another assignment statement to do that. Suppose we execute x = 25 in the state where x is bound to 10.Then our image becomes as follows:
Choosing variable names
Suppose that we u sed the variables x and y in place of the variables side and area in the examples above. Now, if we were to compute some other value for the square that depends on the length of the side , such as the perimeter or length of the diagonal, we would have to remember which of x and y , referred to the length of the side because x and y are not as descriptive as side and area . In choosing variable names, we have to keep in mind that programs are read and maintained by human beings, not only executed by machines.
Note about syntax
In Python, variable identifiers can contain uppercase and lowercase letters, digits (provided they don't start with a digit) and the special character _ (underscore). Although it is legal to use uppercase letters in variable identifiers, we typically do not use them by convention. Variable identifiers are also case-sensitive. For example, side and Side are two different variable identifiers.
There is a collection of words, called reserved words (also known as keywords), in Python that have built-in meanings and therefore cannot be used as variable names. For the list of Python's keywords See 2.3.1 of the Python Language Reference.
Syntax and Sema ntic Errors
Now that we know how to write arithmetic expressions and assignment statements in Python, we can pause and think about what Python does if we write something that the Python interpreter cannot interpret. Python informs us about such problems by giving an error message. Broadly speaking there are two categories for Python errors:
- Syntax errors: These occur when we write Python expressions or statements that are not well-formed according to Python's syntax. For example, if we attempt to write an assignment statement such as 13 = age , Python gives a syntax error. This is because Python syntax says that for an assignment statement to be well-formed it must contain a variable on the left hand side (LHS) of the assignment operator "=" and a well-formed expression on the right hand side (RHS), and 13 is not a variable.
- Semantic errors: These occur when the Python interpreter cannot evaluate expressions or execute statements because they cannot be associated with a "meaning" that the interpreter can use. For example, the expression age + 1 is well-formed but it has a meaning only when age is already bound to a value. If we attempt to evaluate this expression before age is bound to some value by a prior assignment then Python gives a semantic error.
Even though we have used numerical expressions in all of our examples so far, assignments are not confined to numerical types. They could involve expressions built from any defined type. Recall the table that summarizes the basic types in Python.
The following video shows execution of assignment statements involving strings. It also introduces some commonly used operators on strings. For more information see the online documentation. In the video below, you see the Python shell displaying "=> None" after the assignment statements. This is unique to the Python shell presented in the video. In most Python programming environments, nothing is displayed after an assignment statement. The difference in behavior stems from version differences between the programming environment used in the video and in the activities, and can be safely ignored.
Distinguishing Expressions and Assignments
So far in the module, we have been careful to keep the distinction between the terms expression and statement because there is a conceptual difference between them, which is sometimes overlooked. Expressions denote values; they are evaluated to yield a value. On the other hand, statements are commands (instructions) that change the state of the computer. You can think of state here as some representation of computer memory and the binding of variables and values in the memory. In a state where the variable side is bound to the integer 3, and the variable area is yet unbound, the value of the expression side + 2 is 5. The assignment statement side = side + 2 , changes the state so that value 5 is bound to side in the new state. Note that when you type an expression in the Python shell, Python evaluates the expression and you get a value in return. On the other hand, if you type an assignment statement nothing is returned. Assignment statements do not return a value. Try, for example, typing x = 100 + 50 . Python adds 100 to 50, gets the value 150, and binds x to 150. However, we only see the prompt >>> after Python does the assignment. We don't see the change in the state until we inspect the value of x , by invoking x .
What we have learned so far can be summarized as using the Python interpreter to manipulate values of some primitive data types such as integers, real numbers, and character strings by evaluating expressions that involve built-in operators on these types. Assignments statements let us name the values that appear in expressions. While what we have learned so far allows us to do some computations conveniently, they are limited in their generality and reusability. Next, we introduce functions as a means to make computations more general and reusable.

Developing a Thesis Statement
Many papers you write require developing a thesis statement. In this section you’ll learn what a thesis statement is and how to write one.
Keep in mind that not all papers require thesis statements . If in doubt, please consult your instructor for assistance.
What is a thesis statement?
A thesis statement . . .
- Makes an argumentative assertion about a topic; it states the conclusions that you have reached about your topic.
- Makes a promise to the reader about the scope, purpose, and direction of your paper.
- Is focused and specific enough to be “proven” within the boundaries of your paper.
- Is generally located near the end of the introduction ; sometimes, in a long paper, the thesis will be expressed in several sentences or in an entire paragraph.
- Identifies the relationships between the pieces of evidence that you are using to support your argument.
Not all papers require thesis statements! Ask your instructor if you’re in doubt whether you need one.
Identify a topic
Your topic is the subject about which you will write. Your assignment may suggest several ways of looking at a topic; or it may name a fairly general concept that you will explore or analyze in your paper.
Consider what your assignment asks you to do
Inform yourself about your topic, focus on one aspect of your topic, ask yourself whether your topic is worthy of your efforts, generate a topic from an assignment.
Below are some possible topics based on sample assignments.
Sample assignment 1
Analyze Spain’s neutrality in World War II.
Identified topic
Franco’s role in the diplomatic relationships between the Allies and the Axis
This topic avoids generalities such as “Spain” and “World War II,” addressing instead on Franco’s role (a specific aspect of “Spain”) and the diplomatic relations between the Allies and Axis (a specific aspect of World War II).
Sample assignment 2
Analyze one of Homer’s epic similes in the Iliad.
The relationship between the portrayal of warfare and the epic simile about Simoisius at 4.547-64.
This topic focuses on a single simile and relates it to a single aspect of the Iliad ( warfare being a major theme in that work).
Developing a Thesis Statement–Additional information
Your assignment may suggest several ways of looking at a topic, or it may name a fairly general concept that you will explore or analyze in your paper. You’ll want to read your assignment carefully, looking for key terms that you can use to focus your topic.
Sample assignment: Analyze Spain’s neutrality in World War II Key terms: analyze, Spain’s neutrality, World War II
After you’ve identified the key words in your topic, the next step is to read about them in several sources, or generate as much information as possible through an analysis of your topic. Obviously, the more material or knowledge you have, the more possibilities will be available for a strong argument. For the sample assignment above, you’ll want to look at books and articles on World War II in general, and Spain’s neutrality in particular.
As you consider your options, you must decide to focus on one aspect of your topic. This means that you cannot include everything you’ve learned about your topic, nor should you go off in several directions. If you end up covering too many different aspects of a topic, your paper will sprawl and be unconvincing in its argument, and it most likely will not fulfull the assignment requirements.
For the sample assignment above, both Spain’s neutrality and World War II are topics far too broad to explore in a paper. You may instead decide to focus on Franco’s role in the diplomatic relationships between the Allies and the Axis , which narrows down what aspects of Spain’s neutrality and World War II you want to discuss, as well as establishes a specific link between those two aspects.
Before you go too far, however, ask yourself whether your topic is worthy of your efforts. Try to avoid topics that already have too much written about them (i.e., “eating disorders and body image among adolescent women”) or that simply are not important (i.e. “why I like ice cream”). These topics may lead to a thesis that is either dry fact or a weird claim that cannot be supported. A good thesis falls somewhere between the two extremes. To arrive at this point, ask yourself what is new, interesting, contestable, or controversial about your topic.
As you work on your thesis, remember to keep the rest of your paper in mind at all times . Sometimes your thesis needs to evolve as you develop new insights, find new evidence, or take a different approach to your topic.
Derive a main point from topic
Once you have a topic, you will have to decide what the main point of your paper will be. This point, the “controlling idea,” becomes the core of your argument (thesis statement) and it is the unifying idea to which you will relate all your sub-theses. You can then turn this “controlling idea” into a purpose statement about what you intend to do in your paper.
Look for patterns in your evidence
Compose a purpose statement.
Consult the examples below for suggestions on how to look for patterns in your evidence and construct a purpose statement.
- Franco first tried to negotiate with the Axis
- Franco turned to the Allies when he couldn’t get some concessions that he wanted from the Axis
Possible conclusion:
Spain’s neutrality in WWII occurred for an entirely personal reason: Franco’s desire to preserve his own (and Spain’s) power.
Purpose statement
This paper will analyze Franco’s diplomacy during World War II to see how it contributed to Spain’s neutrality.
- The simile compares Simoisius to a tree, which is a peaceful, natural image.
- The tree in the simile is chopped down to make wheels for a chariot, which is an object used in warfare.
At first, the simile seems to take the reader away from the world of warfare, but we end up back in that world by the end.
This paper will analyze the way the simile about Simoisius at 4.547-64 moves in and out of the world of warfare.
Derive purpose statement from topic
To find out what your “controlling idea” is, you have to examine and evaluate your evidence . As you consider your evidence, you may notice patterns emerging, data repeated in more than one source, or facts that favor one view more than another. These patterns or data may then lead you to some conclusions about your topic and suggest that you can successfully argue for one idea better than another.
For instance, you might find out that Franco first tried to negotiate with the Axis, but when he couldn’t get some concessions that he wanted from them, he turned to the Allies. As you read more about Franco’s decisions, you may conclude that Spain’s neutrality in WWII occurred for an entirely personal reason: his desire to preserve his own (and Spain’s) power. Based on this conclusion, you can then write a trial thesis statement to help you decide what material belongs in your paper.
Sometimes you won’t be able to find a focus or identify your “spin” or specific argument immediately. Like some writers, you might begin with a purpose statement just to get yourself going. A purpose statement is one or more sentences that announce your topic and indicate the structure of the paper but do not state the conclusions you have drawn . Thus, you might begin with something like this:
- This paper will look at modern language to see if it reflects male dominance or female oppression.
- I plan to analyze anger and derision in offensive language to see if they represent a challenge of society’s authority.
At some point, you can turn a purpose statement into a thesis statement. As you think and write about your topic, you can restrict, clarify, and refine your argument, crafting your thesis statement to reflect your thinking.
As you work on your thesis, remember to keep the rest of your paper in mind at all times. Sometimes your thesis needs to evolve as you develop new insights, find new evidence, or take a different approach to your topic.
Compose a draft thesis statement
If you are writing a paper that will have an argumentative thesis and are having trouble getting started, the techniques in the table below may help you develop a temporary or “working” thesis statement.
Begin with a purpose statement that you will later turn into a thesis statement.
Assignment: Discuss the history of the Reform Party and explain its influence on the 1990 presidential and Congressional election.
Purpose Statement: This paper briefly sketches the history of the grassroots, conservative, Perot-led Reform Party and analyzes how it influenced the economic and social ideologies of the two mainstream parties.
Question-to-Assertion
If your assignment asks a specific question(s), turn the question(s) into an assertion and give reasons why it is true or reasons for your opinion.
Assignment : What do Aylmer and Rappaccini have to be proud of? Why aren’t they satisfied with these things? How does pride, as demonstrated in “The Birthmark” and “Rappaccini’s Daughter,” lead to unexpected problems?
Beginning thesis statement: Alymer and Rappaccinni are proud of their great knowledge; however, they are also very greedy and are driven to use their knowledge to alter some aspect of nature as a test of their ability. Evil results when they try to “play God.”
Write a sentence that summarizes the main idea of the essay you plan to write.
Main idea: The reason some toys succeed in the market is that they appeal to the consumers’ sense of the ridiculous and their basic desire to laugh at themselves.
Make a list of the ideas that you want to include; consider the ideas and try to group them.
- nature = peaceful
- war matériel = violent (competes with 1?)
- need for time and space to mourn the dead
- war is inescapable (competes with 3?)
Use a formula to arrive at a working thesis statement (you will revise this later).
- although most readers of _______ have argued that _______, closer examination shows that _______.
- _______ uses _______ and _____ to prove that ________.
- phenomenon x is a result of the combination of __________, __________, and _________.
What to keep in mind as you draft an initial thesis statement
Beginning statements obtained through the methods illustrated above can serve as a framework for planning or drafting your paper, but remember they’re not yet the specific, argumentative thesis you want for the final version of your paper. In fact, in its first stages, a thesis statement usually is ill-formed or rough and serves only as a planning tool.
As you write, you may discover evidence that does not fit your temporary or “working” thesis. Or you may reach deeper insights about your topic as you do more research, and you will find that your thesis statement has to be more complicated to match the evidence that you want to use.
You must be willing to reject or omit some evidence in order to keep your paper cohesive and your reader focused. Or you may have to revise your thesis to match the evidence and insights that you want to discuss. Read your draft carefully, noting the conclusions you have drawn and the major ideas which support or prove those conclusions. These will be the elements of your final thesis statement.
Sometimes you will not be able to identify these elements in your early drafts, but as you consider how your argument is developing and how your evidence supports your main idea, ask yourself, “ What is the main point that I want to prove/discuss? ” and “ How will I convince the reader that this is true? ” When you can answer these questions, then you can begin to refine the thesis statement.
Refine and polish the thesis statement
To get to your final thesis, you’ll need to refine your draft thesis so that it’s specific and arguable.
- Ask if your draft thesis addresses the assignment
- Question each part of your draft thesis
- Clarify vague phrases and assertions
- Investigate alternatives to your draft thesis
Consult the example below for suggestions on how to refine your draft thesis statement.
Sample Assignment
Choose an activity and define it as a symbol of American culture. Your essay should cause the reader to think critically about the society which produces and enjoys that activity.
- Ask The phenomenon of drive-in facilities is an interesting symbol of american culture, and these facilities demonstrate significant characteristics of our society.This statement does not fulfill the assignment because it does not require the reader to think critically about society.
Drive-ins are an interesting symbol of American culture because they represent Americans’ significant creativity and business ingenuity.
Among the types of drive-in facilities familiar during the twentieth century, drive-in movie theaters best represent American creativity, not merely because they were the forerunner of later drive-ins and drive-throughs, but because of their impact on our culture: they changed our relationship to the automobile, changed the way people experienced movies, and changed movie-going into a family activity.
While drive-in facilities such as those at fast-food establishments, banks, pharmacies, and dry cleaners symbolize America’s economic ingenuity, they also have affected our personal standards.
While drive-in facilities such as those at fast- food restaurants, banks, pharmacies, and dry cleaners symbolize (1) Americans’ business ingenuity, they also have contributed (2) to an increasing homogenization of our culture, (3) a willingness to depersonalize relationships with others, and (4) a tendency to sacrifice quality for convenience.
This statement is now specific and fulfills all parts of the assignment. This version, like any good thesis, is not self-evident; its points, 1-4, will have to be proven with evidence in the body of the paper. The numbers in this statement indicate the order in which the points will be presented. Depending on the length of the paper, there could be one paragraph for each numbered item or there could be blocks of paragraph for even pages for each one.
Complete the final thesis statement
The bottom line.
As you move through the process of crafting a thesis, you’ll need to remember four things:
- Context matters! Think about your course materials and lectures. Try to relate your thesis to the ideas your instructor is discussing.
- As you go through the process described in this section, always keep your assignment in mind . You will be more successful when your thesis (and paper) responds to the assignment than if it argues a semi-related idea.
- Your thesis statement should be precise, focused, and contestable ; it should predict the sub-theses or blocks of information that you will use to prove your argument.
- Make sure that you keep the rest of your paper in mind at all times. Change your thesis as your paper evolves, because you do not want your thesis to promise more than your paper actually delivers.
In the beginning, the thesis statement was a tool to help you sharpen your focus, limit material and establish the paper’s purpose. When your paper is finished, however, the thesis statement becomes a tool for your reader. It tells the reader what you have learned about your topic and what evidence led you to your conclusion. It keeps the reader on track–well able to understand and appreciate your argument.

Writing Process and Structure
This is an accordion element with a series of buttons that open and close related content panels.
Getting Started with Your Paper
Interpreting Writing Assignments from Your Courses
Generating Ideas for
Creating an Argument
Thesis vs. Purpose Statements
Architecture of Arguments
Working with Sources
Quoting and Paraphrasing Sources
Using Literary Quotations
Citing Sources in Your Paper
Drafting Your Paper
Generating Ideas for Your Paper
Introductions
Paragraphing
Developing Strategic Transitions
Conclusions
Revising Your Paper
Peer Reviews
Reverse Outlines
Revising an Argumentative Paper
Revision Strategies for Longer Projects
Finishing Your Paper
Twelve Common Errors: An Editing Checklist
How to Proofread your Paper
Writing Collaboratively
Collaborative and Group Writing
Have a language expert improve your writing
Run a free plagiarism check in 10 minutes, generate accurate citations for free.
- Knowledge Base
- How to Write a Thesis Statement | 4 Steps & Examples
How to Write a Thesis Statement | 4 Steps & Examples
Published on January 11, 2019 by Shona McCombes . Revised on August 15, 2023 by Eoghan Ryan.
A thesis statement is a sentence that sums up the central point of your paper or essay . It usually comes near the end of your introduction .
Your thesis will look a bit different depending on the type of essay you’re writing. But the thesis statement should always clearly state the main idea you want to get across. Everything else in your essay should relate back to this idea.
You can write your thesis statement by following four simple steps:
- Start with a question
- Write your initial answer
- Develop your answer
- Refine your thesis statement
Instantly correct all language mistakes in your text
Upload your document to correct all your mistakes in minutes
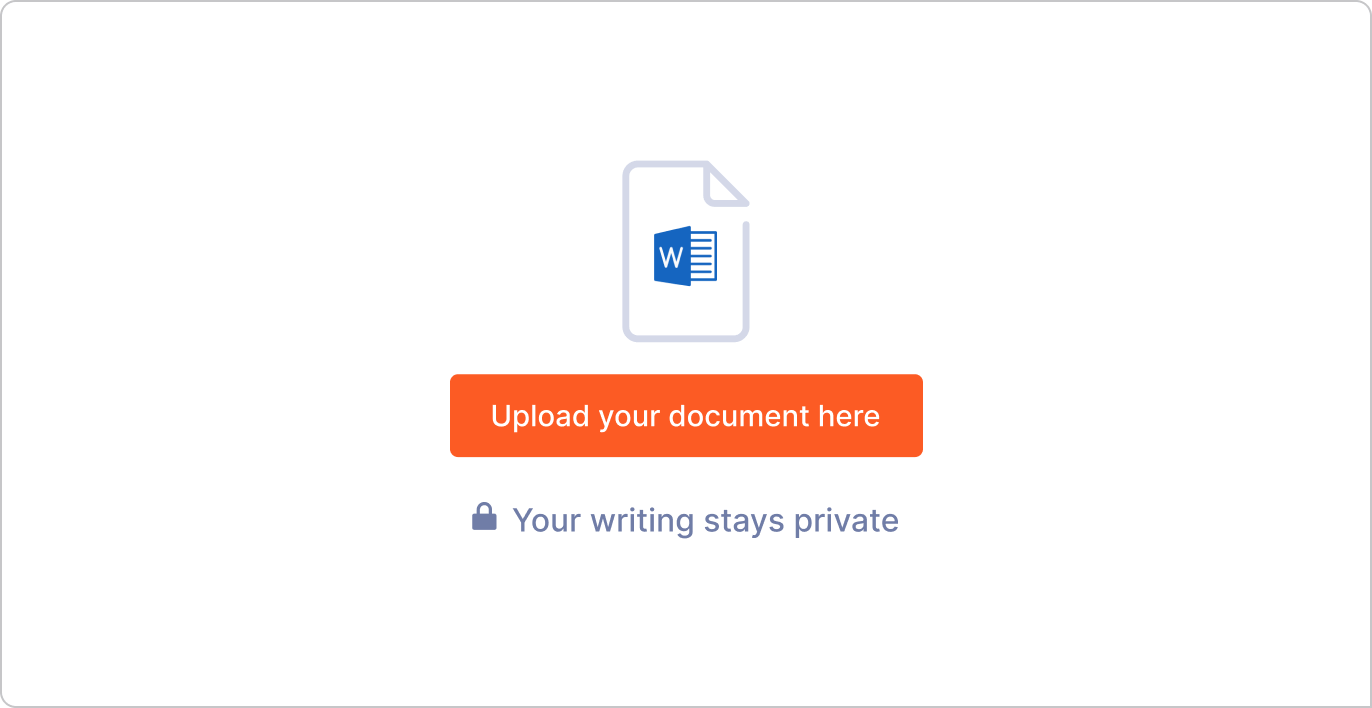
Table of contents
What is a thesis statement, placement of the thesis statement, step 1: start with a question, step 2: write your initial answer, step 3: develop your answer, step 4: refine your thesis statement, types of thesis statements, other interesting articles, frequently asked questions about thesis statements.
A thesis statement summarizes the central points of your essay. It is a signpost telling the reader what the essay will argue and why.
The best thesis statements are:
- Concise: A good thesis statement is short and sweet—don’t use more words than necessary. State your point clearly and directly in one or two sentences.
- Contentious: Your thesis shouldn’t be a simple statement of fact that everyone already knows. A good thesis statement is a claim that requires further evidence or analysis to back it up.
- Coherent: Everything mentioned in your thesis statement must be supported and explained in the rest of your paper.
Prevent plagiarism. Run a free check.
The thesis statement generally appears at the end of your essay introduction or research paper introduction .
The spread of the internet has had a world-changing effect, not least on the world of education. The use of the internet in academic contexts and among young people more generally is hotly debated. For many who did not grow up with this technology, its effects seem alarming and potentially harmful. This concern, while understandable, is misguided. The negatives of internet use are outweighed by its many benefits for education: the internet facilitates easier access to information, exposure to different perspectives, and a flexible learning environment for both students and teachers.
You should come up with an initial thesis, sometimes called a working thesis , early in the writing process . As soon as you’ve decided on your essay topic , you need to work out what you want to say about it—a clear thesis will give your essay direction and structure.
You might already have a question in your assignment, but if not, try to come up with your own. What would you like to find out or decide about your topic?
For example, you might ask:
After some initial research, you can formulate a tentative answer to this question. At this stage it can be simple, and it should guide the research process and writing process .
Here's why students love Scribbr's proofreading services
Discover proofreading & editing
Now you need to consider why this is your answer and how you will convince your reader to agree with you. As you read more about your topic and begin writing, your answer should get more detailed.
In your essay about the internet and education, the thesis states your position and sketches out the key arguments you’ll use to support it.
The negatives of internet use are outweighed by its many benefits for education because it facilitates easier access to information.
In your essay about braille, the thesis statement summarizes the key historical development that you’ll explain.
The invention of braille in the 19th century transformed the lives of blind people, allowing them to participate more actively in public life.
A strong thesis statement should tell the reader:
- Why you hold this position
- What they’ll learn from your essay
- The key points of your argument or narrative
The final thesis statement doesn’t just state your position, but summarizes your overall argument or the entire topic you’re going to explain. To strengthen a weak thesis statement, it can help to consider the broader context of your topic.
These examples are more specific and show that you’ll explore your topic in depth.
Your thesis statement should match the goals of your essay, which vary depending on the type of essay you’re writing:
- In an argumentative essay , your thesis statement should take a strong position. Your aim in the essay is to convince your reader of this thesis based on evidence and logical reasoning.
- In an expository essay , you’ll aim to explain the facts of a topic or process. Your thesis statement doesn’t have to include a strong opinion in this case, but it should clearly state the central point you want to make, and mention the key elements you’ll explain.
If you want to know more about AI tools , college essays , or fallacies make sure to check out some of our other articles with explanations and examples or go directly to our tools!
- Ad hominem fallacy
- Post hoc fallacy
- Appeal to authority fallacy
- False cause fallacy
- Sunk cost fallacy
College essays
- Choosing Essay Topic
- Write a College Essay
- Write a Diversity Essay
- College Essay Format & Structure
- Comparing and Contrasting in an Essay
(AI) Tools
- Grammar Checker
- Paraphrasing Tool
- Text Summarizer
- AI Detector
- Plagiarism Checker
- Citation Generator
A thesis statement is a sentence that sums up the central point of your paper or essay . Everything else you write should relate to this key idea.
The thesis statement is essential in any academic essay or research paper for two main reasons:
- It gives your writing direction and focus.
- It gives the reader a concise summary of your main point.
Without a clear thesis statement, an essay can end up rambling and unfocused, leaving your reader unsure of exactly what you want to say.
Follow these four steps to come up with a thesis statement :
- Ask a question about your topic .
- Write your initial answer.
- Develop your answer by including reasons.
- Refine your answer, adding more detail and nuance.
The thesis statement should be placed at the end of your essay introduction .
Cite this Scribbr article
If you want to cite this source, you can copy and paste the citation or click the “Cite this Scribbr article” button to automatically add the citation to our free Citation Generator.
McCombes, S. (2023, August 15). How to Write a Thesis Statement | 4 Steps & Examples. Scribbr. Retrieved April 11, 2024, from https://www.scribbr.com/academic-essay/thesis-statement/
Is this article helpful?
Shona McCombes
Other students also liked, how to write an essay introduction | 4 steps & examples, how to write topic sentences | 4 steps, examples & purpose, academic paragraph structure | step-by-step guide & examples, what is your plagiarism score.
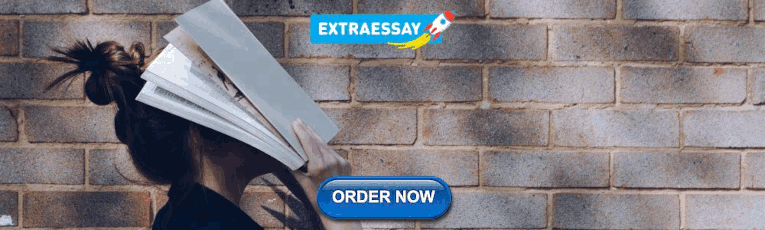
IMAGES
VIDEO
COMMENTS
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Annotated assignment statements¶ Annotation assignment is the combination, in a single statement, of a variable or attribute annotation and an optional assignment statement: annotated_assignment_stmt::= augtarget ":" expression ["=" (starred_expression | yield_expression)] The difference from normal Assignment statements is that only a single ...
Assignment Statement. An Assignment statement is a statement that is used to set a value to the variable name in a program. Assignment statement allows a variable to hold different types of values during its program lifespan. Another way of understanding an assignment statement is, it stores a value in the memory location which is denoted.
Assignment creates object references instead of copying the objects. Python creates a variable name the first time when they are assigned a value. Names must be assigned before being referenced. There are some operations that perform assignments implicitly. Assignment statement forms :-. 1. Basic form: Unmute.
An assignment statement is a line of code that uses a "=" sign. The statement stores the result of an operation performed on the right-hand side of the sign into the variable memory location on the left-hand side. 4. Enter and execute the following lines of Python code in the editor window of your IDE (e.g. Thonny):
Assignment (computer science) In computer programming, an assignment statement sets and/or re-sets the value stored in the storage location (s) denoted by a variable name; in other words, it copies a value into the variable. In most imperative programming languages, the assignment statement (or expression) is a fundamental construct.
The assignment statement is used to store a value in a variable. As in most programming languages these days, the assignment statement has the form: <variable>= <expression>; For example, once we have an int variable j, we can assign it the value of expression 4 + 6: int j; j= 4+6; As a convention, we always place a blank after the = sign but ...
Assignment Statements ¶. Assignment statements initialize or change the value stored in a variable using the assignment operator =. An assignment statement always has a single variable on the left hand side. The value of the expression (which can contain math operators and other variables) on the right of the = sign is stored in the variable ...
The Assignment Statement and Types Topics: Python's Interactive Mode Variables Expressions Assignment Strings, Ints, and Floats . The Python Interactive Shell Python can be used in a way that reminds you of a calculator. In the ``command shell of your system simply type python
The assignment statement is an instruction that stores a value in a variable. You use this instruction any time you want to update the value of a variable. The assignment statement performs two actions. First, it calculates the value of the expression (calculation) on the right-hand side of the assignment operator (the = ).
Assignment Statements. Assignment statements in a program come in two forms - with and without mutations. Assignments without mutation are those that give a value to a variable without using the old value of that variable. Assignments with mutation are variable assignments that use the old value of a variable to calculate a value for the ...
4. Assignment of variables Assignment of statements. It is essential that every variable in a program is given a value explicitly before any attempt is made to use it. It is also very important that the value assigned is of the correct type. The most common form of statement in a program uses the assignment operator, =, and either an expression or a constant to assign a value to a variable:
The meaning of the first assignment is computing the sum of the value in Counter and 1, and saves it back to Counter. Since Counter 's current value is zero, Counter + 1 is 1+0 = 1 and hence 1 is saved into Counter. Therefore, the new value of Counter becomes 1 and its original value 0 disappears. The second assignment statement computes the ...
Assignment To make a variable vary, we assign a new value to it. This is done, unsurprisingly, in an assignment statement such as b = r; // Set b to have the value r has which says, the contents of r (recall, it's a container) are assigned to or replace the contents of b.
In Java, an assignment statement is an expression that evaluates a value, which is assigned to the variable on the left side of the assignment operator. Whereas an assignment expression is the same, except it does not take into account the variable. That's why the following statements are legal: System.out.println(x = 1); Which is equivalent to:
Assignment statements initialize or change the value stored in a variable using the assignment operator =. An assignment statement always has a single variable on the left hand side of the = sign. The value of the expression on the right hand side of the = sign (which can contain math operators and other variables) is copied into the memory ...
ASSIGNMENT STATEMENTS Expressions can be part of a condition statement ((num+num2/5)>num3) or part of an output statement (cout num + num2). But the most common use of expressions is to be the right hand side of an assignment statement. BNF syntax for an assignment statement: <target_var> <assign_operator> <expression>
What this handout is about. The first step in any successful college writing venture is reading the assignment. While this sounds like a simple task, it can be a tough one. This handout will help you unravel your assignment and begin to craft an effective response. Much of the following advice will involve translating typical assignment terms ...
An assignment statement associates the name to the left of the symbol = with the value denoted by the expression on the right of =. The binding in the picture is created using an assignment statemen t of the form x = 10. We usually read such an assignment statement as "10 is assigned to x" or "x is set to 10". If we want to change the value ...
A thesis statement . . . Makes an argumentative assertion about a topic; it states the conclusions that you have reached about your topic. Makes a promise to the reader about the scope, purpose, and direction of your paper. Is focused and specific enough to be "proven" within the boundaries of your paper. Is generally located near the end ...
Watch out for cliches like "making a difference," "broadening my horizons," or "the best thing that ever happened to me." 3. Stay focused. Try to avoid getting off-track or including tangents in your personal statement. Stay focused by writing a first draft and then re-reading what you've written.
5.1. Assignment Statement Basics. The fundamental method of modifying the data in a data set is by way of a basic assignment statement. Such a statement always takes the form: variable = expression; where variable is any valid SAS name and expression is the calculation that is necessary to give the variable its values.
Step 2: Write your initial answer. After some initial research, you can formulate a tentative answer to this question. At this stage it can be simple, and it should guide the research process and writing process. The internet has had more of a positive than a negative effect on education.
SUPREME COURT OF NEW JERSEY . It is ORDERED that effective July 1, 2024, Superior Court Judge Avion M. Benjamin is hereby assigned to the Civil Division of Superior Court in Essex County (Vicinage 5) and is designated as the Civil Presiding Judge for the vicinage. This will amend the 2023-2024 General Assignment Order dated July 31, 2023.