- Scipy lecture notes »
- 1. Getting started with Python for science »
- 1.4. NumPy: creating and manipulating numerical data »

- Edit Improve this page: Edit it on Github.
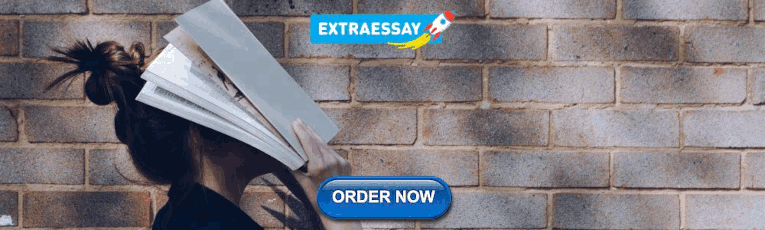
1.4.1. The NumPy array object ¶
Section contents
- What are NumPy and NumPy arrays?
- Creating arrays
- Basic data types
- Basic visualization
- Indexing and slicing
- Copies and views
- Fancy indexing
1.4.1.1. What are NumPy and NumPy arrays? ¶
Numpy arrays ¶.
For example, An array containing:
- values of an experiment/simulation at discrete time steps
- signal recorded by a measurement device, e.g. sound wave
- pixels of an image, grey-level or colour
- 3-D data measured at different X-Y-Z positions, e.g. MRI scan
Why it is useful: Memory-efficient container that provides fast numerical operations.
NumPy Reference documentation ¶
On the web: https://numpy.org/doc/
Interactive help:
Looking for something:
Import conventions ¶
The recommended convention to import numpy is:
1.4.1.2. Creating arrays ¶
Manual construction of arrays ¶.
2-D, 3-D, … :
Exercise: Simple arrays
- Create a simple two dimensional array. First, redo the examples from above. And then create your own: how about odd numbers counting backwards on the first row, and even numbers on the second?
- Use the functions len() , numpy.shape() on these arrays. How do they relate to each other? And to the ndim attribute of the arrays?
Functions for creating arrays ¶
In practice, we rarely enter items one by one…
Evenly spaced:
or by number of points:
Common arrays:
np.random : random numbers (Mersenne Twister PRNG):
Exercise: Creating arrays using functions
- Experiment with arange , linspace , ones , zeros , eye and diag .
- Create different kinds of arrays with random numbers.
- Try setting the seed before creating an array with random values.
- Look at the function np.empty . What does it do? When might this be useful?
1.4.1.3. Basic data types ¶
You may have noticed that, in some instances, array elements are displayed with a trailing dot (e.g. 2. vs 2 ). This is due to a difference in the data-type used:
Different data-types allow us to store data more compactly in memory, but most of the time we simply work with floating point numbers. Note that, in the example above, NumPy auto-detects the data-type from the input.
You can explicitly specify which data-type you want:
The default data type is floating point:
There are also other types:
1.4.1.4. Basic visualization ¶
Now that we have our first data arrays, we are going to visualize them.
Start by launching IPython:
Or the notebook:
Once IPython has started, enable interactive plots:
Or, from the notebook, enable plots in the notebook:
The inline is important for the notebook, so that plots are displayed in the notebook and not in a new window.
Matplotlib is a 2D plotting package. We can import its functions as below:
And then use (note that you have to use show explicitly if you have not enabled interactive plots with %matplotlib ):
Or, if you have enabled interactive plots with %matplotlib :
- 1D plotting :
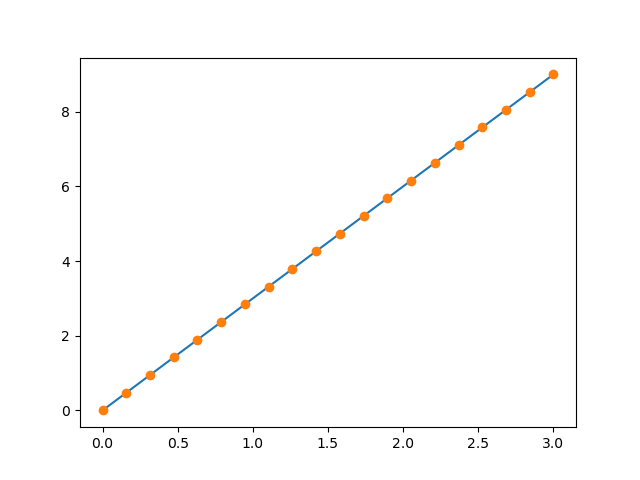
- 2D arrays (such as images):
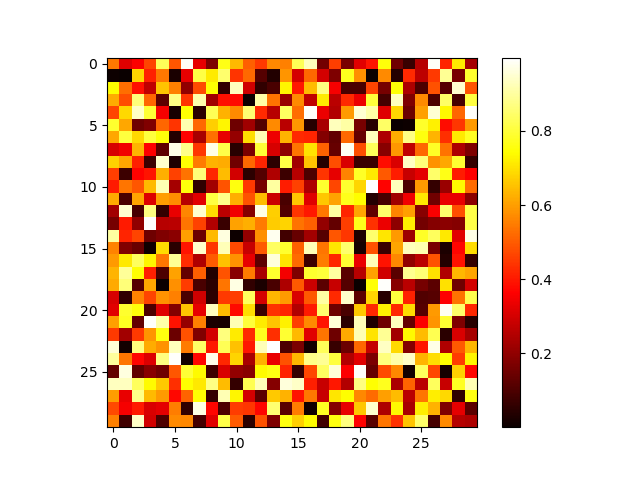
More in the: matplotlib chapter
Exercise: Simple visualizations
- Plot some simple arrays: a cosine as a function of time and a 2D matrix.
- Try using the gray colormap on the 2D matrix.
1.4.1.5. Indexing and slicing ¶
The items of an array can be accessed and assigned to the same way as other Python sequences (e.g. lists):
Indices begin at 0, like other Python sequences (and C/C++). In contrast, in Fortran or Matlab, indices begin at 1.
The usual python idiom for reversing a sequence is supported:
For multidimensional arrays, indices are tuples of integers:
- In 2D, the first dimension corresponds to rows , the second to columns .
- for multidimensional a , a[0] is interpreted by taking all elements in the unspecified dimensions.
Slicing : Arrays, like other Python sequences can also be sliced:
Note that the last index is not included! :
All three slice components are not required: by default, start is 0, end is the last and step is 1:
A small illustrated summary of NumPy indexing and slicing…
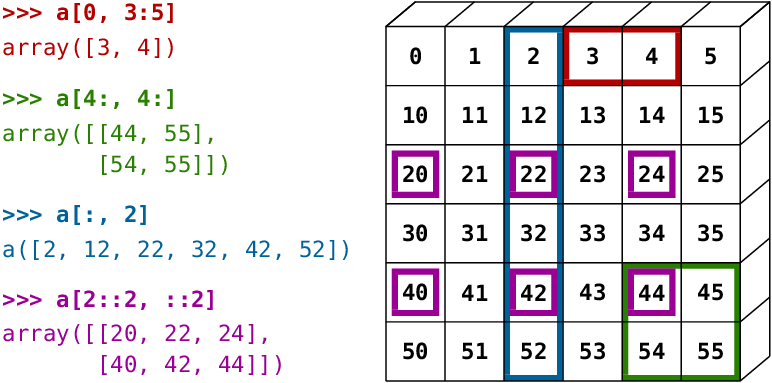
You can also combine assignment and slicing:
Exercise: Indexing and slicing
Try the different flavours of slicing, using start , end and step : starting from a linspace, try to obtain odd numbers counting backwards, and even numbers counting forwards.
Reproduce the slices in the diagram above. You may use the following expression to create the array:
Exercise: Array creation
Create the following arrays (with correct data types):
Par on course: 3 statements for each
Hint : Individual array elements can be accessed similarly to a list, e.g. a[1] or a[1, 2] .
Hint : Examine the docstring for diag .
Exercise: Tiling for array creation
Skim through the documentation for np.tile , and use this function to construct the array:
1.4.1.6. Copies and views ¶
A slicing operation creates a view on the original array, which is just a way of accessing array data. Thus the original array is not copied in memory. You can use np.may_share_memory() to check if two arrays share the same memory block. Note however, that this uses heuristics and may give you false positives.
When modifying the view, the original array is modified as well :
This behavior can be surprising at first sight… but it allows to save both memory and time.
Worked example: Prime number sieve
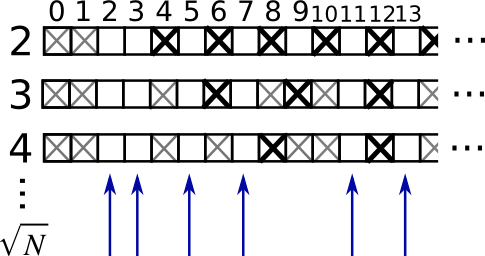
Compute prime numbers in 0–99, with a sieve
- Construct a shape (100,) boolean array is_prime , filled with True in the beginning:
- Cross out 0 and 1 which are not primes:
- For each integer j starting from 2, cross out its higher multiples:
Skim through help(np.nonzero) , and print the prime numbers
- Move the above code into a script file named prime_sieve.py
- Run it to check it works
- Use the optimization suggested in the sieve of Eratosthenes :
Skip j which are already known to not be primes The first number to cross out is
1.4.1.7. Fancy indexing ¶
NumPy arrays can be indexed with slices, but also with boolean or integer arrays ( masks ). This method is called fancy indexing . It creates copies not views .
Using boolean masks ¶
Indexing with a mask can be very useful to assign a new value to a sub-array:
Indexing with an array of integers ¶
Indexing can be done with an array of integers, where the same index is repeated several time:
New values can be assigned with this kind of indexing:
When a new array is created by indexing with an array of integers, the new array has the same shape as the array of integers:
The image below illustrates various fancy indexing applications
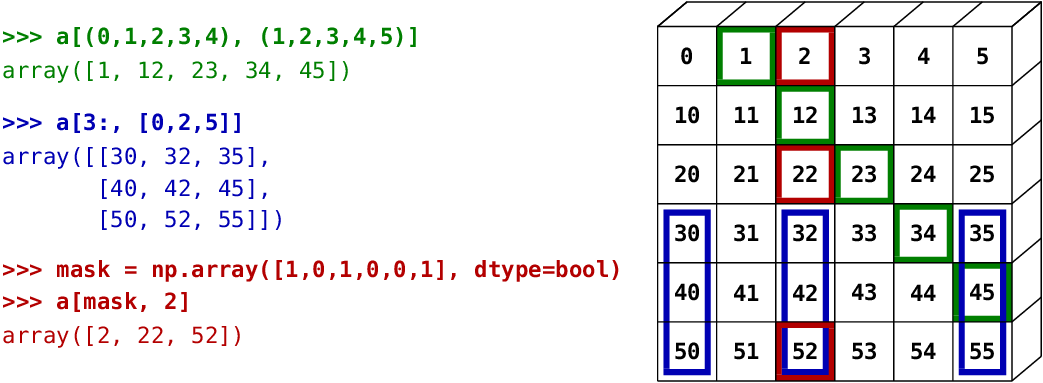
Exercise: Fancy indexing
- Again, reproduce the fancy indexing shown in the diagram above.
- Use fancy indexing on the left and array creation on the right to assign values into an array, for instance by setting parts of the array in the diagram above to zero.
Table Of Contents
- NumPy arrays
- NumPy Reference documentation
- Import conventions
- Manual construction of arrays
- Functions for creating arrays
- 1.4.1.3. Basic data types
- 1.4.1.4. Basic visualization
- 1.4.1.5. Indexing and slicing
- 1.4.1.6. Copies and views
- Using boolean masks
- Indexing with an array of integers
Previous topic
1.4. NumPy: creating and manipulating numerical data
1.4.2. Numerical operations on arrays
- Show Source
Quick search
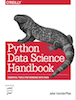
The text is released under the CC-BY-NC-ND license , and code is released under the MIT license . If you find this content useful, please consider supporting the work by buying the book !
The Basics of NumPy Arrays
< Understanding Data Types in Python | Contents | Computation on NumPy Arrays: Universal Functions >
Data manipulation in Python is nearly synonymous with NumPy array manipulation: even newer tools like Pandas ( Chapter 3 ) are built around the NumPy array. This section will present several examples of using NumPy array manipulation to access data and subarrays, and to split, reshape, and join the arrays. While the types of operations shown here may seem a bit dry and pedantic, they comprise the building blocks of many other examples used throughout the book. Get to know them well!
We'll cover a few categories of basic array manipulations here:
- Attributes of arrays : Determining the size, shape, memory consumption, and data types of arrays
- Indexing of arrays : Getting and setting the value of individual array elements
- Slicing of arrays : Getting and setting smaller subarrays within a larger array
- Reshaping of arrays : Changing the shape of a given array
- Joining and splitting of arrays : Combining multiple arrays into one, and splitting one array into many
NumPy Array Attributes ¶
First let's discuss some useful array attributes. We'll start by defining three random arrays, a one-dimensional, two-dimensional, and three-dimensional array. We'll use NumPy's random number generator, which we will seed with a set value in order to ensure that the same random arrays are generated each time this code is run:
Each array has attributes ndim (the number of dimensions), shape (the size of each dimension), and size (the total size of the array):
Another useful attribute is the dtype , the data type of the array (which we discussed previously in Understanding Data Types in Python ):
Other attributes include itemsize , which lists the size (in bytes) of each array element, and nbytes , which lists the total size (in bytes) of the array:
In general, we expect that nbytes is equal to itemsize times size .
Array Indexing: Accessing Single Elements ¶
If you are familiar with Python's standard list indexing, indexing in NumPy will feel quite familiar. In a one-dimensional array, the $i^{th}$ value (counting from zero) can be accessed by specifying the desired index in square brackets, just as with Python lists:
To index from the end of the array, you can use negative indices:
In a multi-dimensional array, items can be accessed using a comma-separated tuple of indices:
Values can also be modified using any of the above index notation:
Keep in mind that, unlike Python lists, NumPy arrays have a fixed type. This means, for example, that if you attempt to insert a floating-point value to an integer array, the value will be silently truncated. Don't be caught unaware by this behavior!
Array Slicing: Accessing Subarrays ¶
Just as we can use square brackets to access individual array elements, we can also use them to access subarrays with the slice notation, marked by the colon ( : ) character. The NumPy slicing syntax follows that of the standard Python list; to access a slice of an array x , use this:
If any of these are unspecified, they default to the values start=0 , stop= size of dimension , step=1 . We'll take a look at accessing sub-arrays in one dimension and in multiple dimensions.
One-dimensional subarrays ¶
A potentially confusing case is when the step value is negative. In this case, the defaults for start and stop are swapped. This becomes a convenient way to reverse an array:
Multi-dimensional subarrays ¶
Multi-dimensional slices work in the same way, with multiple slices separated by commas. For example:
Finally, subarray dimensions can even be reversed together:
Accessing array rows and columns ¶
One commonly needed routine is accessing of single rows or columns of an array. This can be done by combining indexing and slicing, using an empty slice marked by a single colon ( : ):
In the case of row access, the empty slice can be omitted for a more compact syntax:
Subarrays as no-copy views ¶
One important–and extremely useful–thing to know about array slices is that they return views rather than copies of the array data. This is one area in which NumPy array slicing differs from Python list slicing: in lists, slices will be copies. Consider our two-dimensional array from before:
Let's extract a $2 \times 2$ subarray from this:
Now if we modify this subarray, we'll see that the original array is changed! Observe:
This default behavior is actually quite useful: it means that when we work with large datasets, we can access and process pieces of these datasets without the need to copy the underlying data buffer.
Creating copies of arrays ¶
Despite the nice features of array views, it is sometimes useful to instead explicitly copy the data within an array or a subarray. This can be most easily done with the copy() method:
If we now modify this subarray, the original array is not touched:
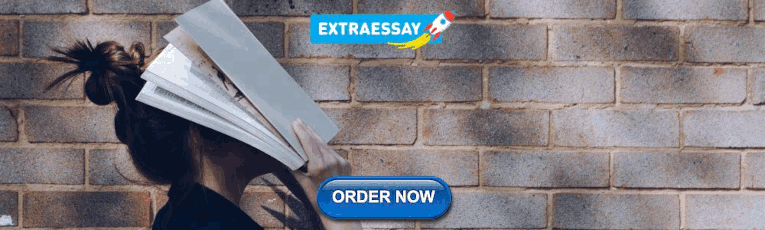
Reshaping of Arrays ¶
Another useful type of operation is reshaping of arrays. The most flexible way of doing this is with the reshape method. For example, if you want to put the numbers 1 through 9 in a $3 \times 3$ grid, you can do the following:
Note that for this to work, the size of the initial array must match the size of the reshaped array. Where possible, the reshape method will use a no-copy view of the initial array, but with non-contiguous memory buffers this is not always the case.
Another common reshaping pattern is the conversion of a one-dimensional array into a two-dimensional row or column matrix. This can be done with the reshape method, or more easily done by making use of the newaxis keyword within a slice operation:
We will see this type of transformation often throughout the remainder of the book.
Array Concatenation and Splitting ¶
All of the preceding routines worked on single arrays. It's also possible to combine multiple arrays into one, and to conversely split a single array into multiple arrays. We'll take a look at those operations here.
Concatenation of arrays ¶
Concatenation, or joining of two arrays in NumPy, is primarily accomplished using the routines np.concatenate , np.vstack , and np.hstack . np.concatenate takes a tuple or list of arrays as its first argument, as we can see here:
You can also concatenate more than two arrays at once:
It can also be used for two-dimensional arrays:
For working with arrays of mixed dimensions, it can be clearer to use the np.vstack (vertical stack) and np.hstack (horizontal stack) functions:
Similary, np.dstack will stack arrays along the third axis.
Splitting of arrays ¶
The opposite of concatenation is splitting, which is implemented by the functions np.split , np.hsplit , and np.vsplit . For each of these, we can pass a list of indices giving the split points:
Notice that N split-points, leads to N + 1 subarrays. The related functions np.hsplit and np.vsplit are similar:
Similarly, np.dsplit will split arrays along the third axis.
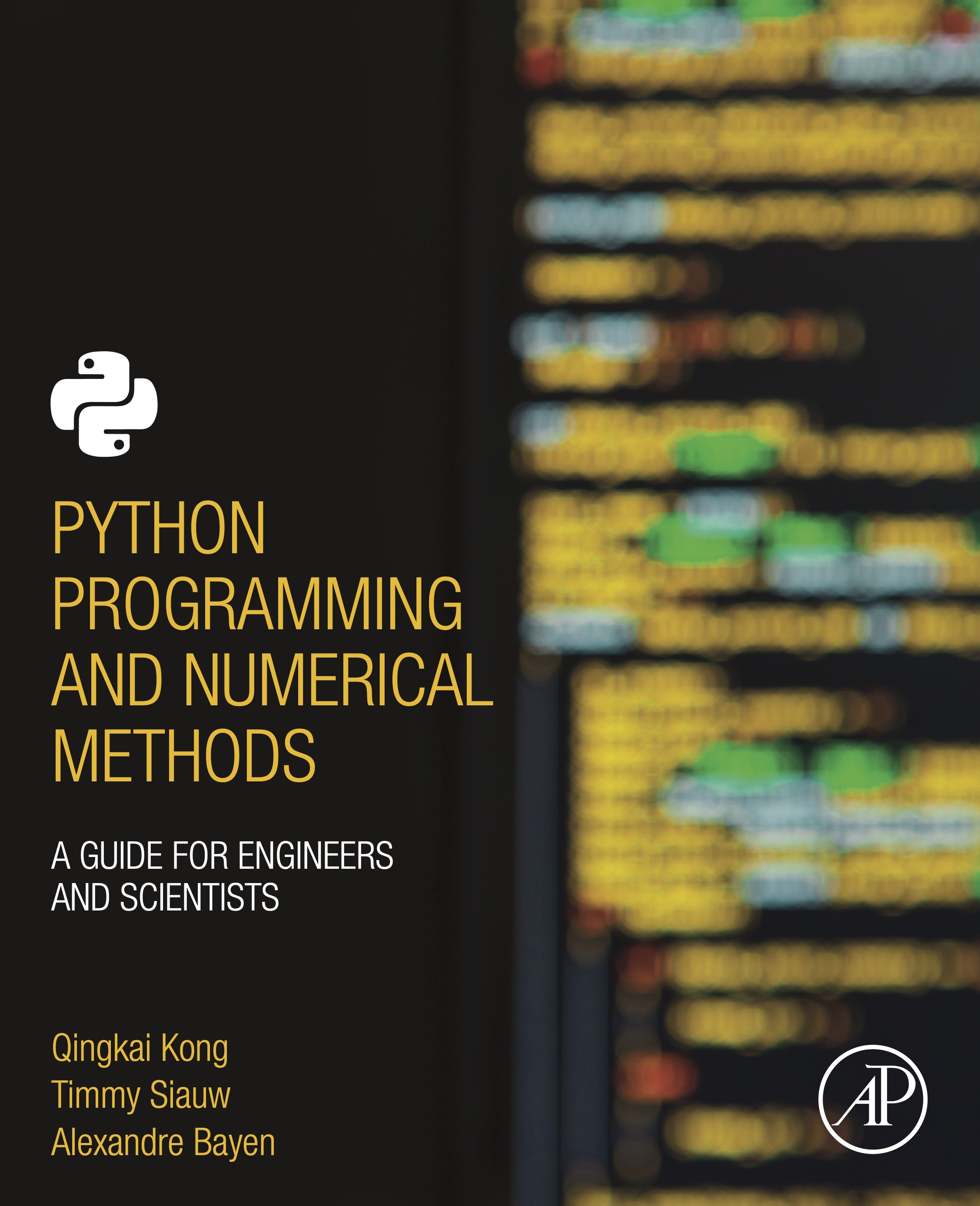
Python Numerical Methods

This notebook contains an excerpt from the Python Programming and Numerical Methods - A Guide for Engineers and Scientists , the content is also available at Berkeley Python Numerical Methods .
The copyright of the book belongs to Elsevier. We also have this interactive book online for a better learning experience. The code is released under the MIT license . If you find this content useful, please consider supporting the work on Elsevier or Amazon !
< 2.6 Data Structure - Dictionaries | Contents | 2.8 Summary and Problems >
Introducing Numpy Arrays ¶
In the 2nd part of this book, we will study the numerical methods by using Python. We will use array/matrix a lot later in the book. Therefore, here we are going to introduce the most common way to handle arrays in Python using the Numpy module . Numpy is probably the most fundamental numerical computing module in Python.
NumPy is important in scientific computing, it is coded both in Python and C (for speed). On its website, a few important features for Numpy is listed:
a powerful N-dimensional array object
sophisticated (broadcasting) functions
tools for integrating C/C++ and Fortran code
useful linear algebra, Fourier transform, and random number capabilities
Here we will only introduce you the Numpy array which is related to the data structure, but we will gradually touch on other aspects of Numpy in the following chapters.
In order to use Numpy module, we need to import it first. A conventional way to import it is to use “np” as a shortened name.
WARNING! Of course, you could call it any name, but conventionally, “np” is accepted by the whole community and it is a good practice to use it for obvious purposes.
To define an array in Python, you could use the np.array function to convert a list.
TRY IT! Create the following arrays:
\(x = \begin{pmatrix} 1 & 4 & 3 \\ \end{pmatrix}\)
\(y = \begin{pmatrix} 1 & 4 & 3 \\ 9 & 2 & 7 \\ \end{pmatrix}\)
NOTE! A 2-D array could use a nested lists to represent, with the inner list represent each row.
Many times we would like to know the size or length of an array. The array shape attribute is called on an array M and returns a 2 × 3 array where the first element is the number of rows in the matrix M and the second element is the number of columns in M. Note that the output of the shape attribute is a tuple. The size attribute is called on an array M and returns the total number of elements in matrix M.
TRY IT! Find the rows, columns and the total size for array y.
NOTE! You may notice the difference that we only use y.shape instead of y.shape() , this is because shape is an attribute rather than a method in this array object. We will introduce more of the object-oriented programming in a later chapter. For now, you need to remember that when we call a method in an object, we need to use the parentheses, while the attribute don’t.
Very often we would like to generate arrays that have a structure or pattern. For instance, we may wish to create the array z = [1 2 3 … 2000]. It would be very cumbersome to type the entire description of z into Python. For generating arrays that are in order and evenly spaced, it is useful to use the arange function in Numpy.
TRY IT! Create an array z from 1 to 2000 with an increment 1.
Using the np.arange , we could create z easily. The first two numbers are the start and end of the sequence, and the last one is the increment. Since it is very common to have an increment of 1, if an increment is not specified, Python will use a default value of 1. Therefore np.arange(1, 2000) will have the same result as np.arange(1, 2000, 1) . Negative or noninteger increments can also be used. If the increment “misses” the last value, it will only extend until the value just before the ending value. For example, x = np.arange(1,8,2) would be [1, 3, 5, 7].
TRY IT! Generate an array with [0.5, 1, 1.5, 2, 2.5].
Sometimes we want to guarantee a start and end point for an array but still have evenly spaced elements. For instance, we may want an array that starts at 1, ends at 8, and has exactly 10 elements. For this purpose you can use the function np.linspace . linspace takes three input values separated by commas. So A = linspace(a,b,n) generates an array of n equally spaced elements starting from a and ending at b.
TRY IT! Use linspace to generate an array starting at 3, ending at 9, and containing 10 elements.
Getting access to the 1D numpy array is similar to what we described for lists or tuples, it has an index to indicate the location. For example:
For 2D arrays, it is slightly different, since we have rows and columns. To get access to the data in a 2D array M, we need to use M[r, c], that the row r and column c are separated by comma. This is referred to as array indexing. The r and c could be single number, a list and so on. If you only think about the row index or the column index, than it is similar to the 1D array. Let’s use the \(y = \begin{pmatrix} 1 & 4 & 3 \\ 9 & 2 & 7 \\ \end{pmatrix}\) as an example.
TRY IT! Get the element at first row and 2nd column of array y .
TRY IT! Get the first row of array y .
TRY IT! Get the last column of array y .
TRY IT! Get the first and third column of array y .
There are some predefined arrays that are really useful. For example, the np.zeros , np.ones , and np.empty are 3 useful functions. Let’s see the examples.
TRY IT! Generate a 3 by 5 array with all the as 0.
TRY IT! Generate a 5 by 3 array with all the element as 1.
NOTE! The shape of the array is defined in a tuple with row as the first item, and column as the second. If you only need a 1D array, then it could be only one number as the input: np.ones(5) .
TRY IT! Generate a 1D empty array with 3 elements.
NOTE! The empty array is not really empty, it is filled with random very small numbers.
You can reassign a value of an array by using array indexing and the assignment operator. You can reassign multiple elements to a single number using array indexing on the left side. You can also reassign multiple elements of an array as long as both the number of elements being assigned and the number of elements assigned is the same. You can create an array using array indexing.
TRY IT! Let a = [1, 2, 3, 4, 5, 6]. Reassign the fourth element of A to 7. Reassign the first, second, and thrid elements to 1. Reassign the second, third, and fourth elements to 9, 8, and 7.
TRY IT! Create a zero array b with shape 2 by 2, and set \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) using array indexing.
WARNING! Although you can create an array from scratch using indexing, we do not advise it. It can confuse you and errors will be harder to find in your code later. For example, b[1, 1] = 1 will give the result \(b = \begin{pmatrix} 0 & 0 \\ 0 & 1 \\ \end{pmatrix}\) , which is strange because b[0, 0], b[0, 1], and b[1, 0] were never specified.
Basic arithmetic is defined for arrays. However, there are operations between a scalar (a single number) and an array and operations between two arrays. We will start with operations between a scalar and an array. To illustrate, let c be a scalar, and b be a matrix.
b + c , b − c , b * c and b / c adds a to every element of b, subtracts c from every element of b, multiplies every element of b by c, and divides every element of b by c, respectively.
TRY IT! Let \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) . Add and substract 2 from b. Multiply and divide b by 2. Square every element of b. Let c be a scalar. On your own, verify the reflexivity of scalar addition and multiplication: b + c = c + b and cb = bc.
Describing operations between two matrices is more complicated. Let b and d be two matrices of the same size. b − d takes every element of b and subtracts the corresponding element of d. Similarly, b + d adds every element of d to the corresponding element of b.
TRY IT! Let \(b = \begin{pmatrix} 1 & 2 \\ 3 & 4 \\ \end{pmatrix}\) and \(d = \begin{pmatrix} 3 & 4 \\ 5 & 6 \\ \end{pmatrix}\) . Compute b + d and b - d.
There are two different kinds of matrix multiplication (and division). There is element-by-element matrix multiplication and standard matrix multiplication. For this section, we will only show how element-by-element matrix multiplication and division work. Standard matrix multiplication will be described in later chapter on Linear Algebra. Python takes the * symbol to mean element-by-element multiplication. For matrices b and d of the same size, b * d takes every element of b and multiplies it by the corresponding element of d. The same is true for / and **.
TRY IT! Compute b * d, b / d, and b**d.
The transpose of an array, b, is an array, d, where b[i, j] = d[j, i]. In other words, the transpose switches the rows and the columns of b. You can transpose an array in Python using the array method T .
TRY IT! Compute the transpose of array b.
Numpy has many arithmetic functions, such as sin, cos, etc., can take arrays as input arguments. The output is the function evaluated for every element of the input array. A function that takes an array as input and performs the function on it is said to be vectorized .
TRY IT! Compute np.sqrt for x = [1, 4, 9, 16].
Logical operations are only defined between a scalar and an array and between two arrays of the same size. Between a scalar and an array, the logical operation is conducted between the scalar and each element of the array. Between two arrays, the logical operation is conducted element-by-element.
TRY IT! Check which elements of the array x = [1, 2, 4, 5, 9, 3] are larger than 3. Check which elements in x are larger than the corresponding element in y = [0, 2, 3, 1, 2, 3].
Python can index elements of an array that satisfy a logical expression.
TRY IT! Let x be the same array as in the previous example. Create a variable y that contains all the elements of x that are strictly bigger than 3. Assign all the values of x that are bigger than 3, the value 0.
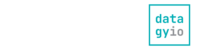
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Indexing and Slicing NumPy Arrays: A Complete Guide
- September 16, 2022 December 30, 2022
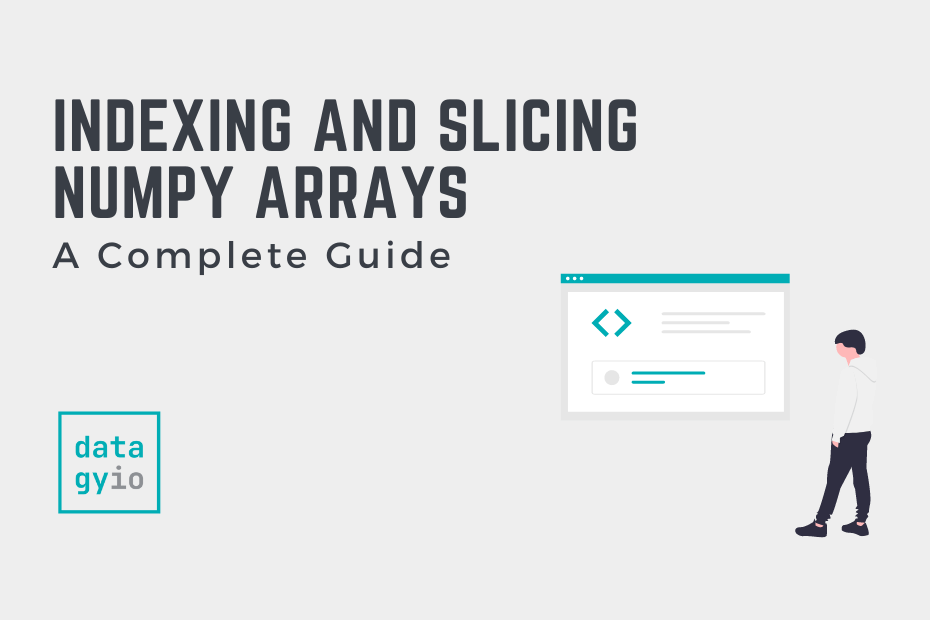
This comprehensive guide will teach you all the different ways to index and slice NumPy arrays. NumPy is an essential library for any data analyst or data scientist using Python. Effectively indexing and slicing NumPy arrays can make you a stronger programmer.
By the end of this tutorial, you’ll have learned:
- How NumPy array indexing and slicing works
- How to index one-dimensional, two-dimensional, and three-dimensional arrays
- How to slice NumPy arrays using ranges, conditions, and more
Table of Contents
Understanding NumPy Array Indexing
Much like working with Python lists , NumPy arrays are based on a 0 index . This means that the index starts at position 0 and continues through to the length of the list minus 1. Similarly, NumPy arrays can be negatively indexed, meaning that their last item can be accessed using the value of -1.
NumPy arrays go beyond basic Python lists by having a number of tricks up their sleeve. However, much of the functionality that exists for Python lists (such as indexing and slicing) will carry forward to NumPy arrays.
How to Access One-Dimensional NumPy Array Elements with Indexing
Let’s see how we can access the first item in a NumPy array by using standard Python x[obj] syntax, where x is the array and obj is the selection:
In the code above, we were able to access the first item by indexing the 0 th index. Similarly, we can access the last item in a NumPy array by using the index of -1, as shown below:
Now that you’ve learned how to index one-dimensional arrays, let’s take a look at how you can access items via indexing in two-dimensional arrays.
How to Access Two-Dimensional NumPy Array Elements with Indexing
Accessing items in two dimensional NumPy arrays can be done in a number of helpful ways. Let’s first look at how to access items using traditional Python indexing by loading a two-dimensional array and double-indexing an array:
We can see that by accessing the 0th index and then the 1st index, we can access the second item of the first array. This approach works in the same way as accessing items in nested lists of lists.
Let’s see a different, NumPy specific way of accessing items in a 2-dimensional NumPy array:
We can see that this simplifies the code significantly. By passing in a single index element containing two items, we’re able to drill into multiple dimensions. This method is also more efficient – the method works without first creating a new, temporary array .
What’s more, is that you can even combine this with negative indexing. This can be particularly helpful when you don’t know how many items an array has! Let’s see how we can access the last item of the first array:
This allows you to combine both positive and negative indices when indexing an array.
Now that you know how to work with two-dimensional arrays, let’s take a look at indexing three-dimensional arrays.
How to Access Three-Dimensional NumPy Array Elements with Indexing
Accessing items in three-dimensional NumPy arrays works in much the same way as working with two-dimensional arrays. Since we know that accessing items works more efficiently by using a single square-bracket, let’s see how we can work three-dimensional arrays:
In the example above, we create an array of shape (2, 2, 2) . The way that the indexing works is that it access data from the outside in . In this case, we are accessing the last array, then the first array, and finally the first value – returning the value 5.
Slicing and Striding NumPy Arrays
Similar to Python lists, you can slice and stride over NumPy arrays. This allows you to access multiple values in array from a starting position to a stop position, at a specific interval. Let’s take a look at a simpler example first, where we access items from the second to the second last item:
By using slicing, the array values from the start up to (but not including) the stop value are returned .
The value before or after the colon : is optional: if a number is omited, then the array is sliced from the first and to the last items respectively.
We can also stride over the array at a particular interval using a third, optional argument in the slice . This follows the convention of [start : stop : stride] , where stride defaults to 1. Let’s see how we can stride over a NumPy array from the first to the last at an interval of 2:
In the following section, you’ll learn how to use integer array indexing in NumPy to access multiple elements.
Integer Array Indexing in NumPy to Access Multiple Elements
You can easily access multiple items via their index in a NumPy array by indexing using a list of items. This allows you to easily get multiple items without needing to index the array multiple times.
Let’s take a look at what this looks like by accessing the first and third item in an array:
What’s important to note here is that by indexing you’re passing in a list of values (make note of the double square brackets).
This method also works with negative indices, as shown below:
Similarly, the order of the indices doesn’t need to be sequential! You can access items in whatever order you want. In the example below, we access first the third item, then the first:
In the section below, you’ll learn how to use boolean indexing in NumPy arrays for conditional slicing.
Boolean Indexing in NumPy Arrays for Conditional Slicing
Using boolean indexing with NumPy arrays makes it very easy to index only items meeting a certain condition. This process is significantly simpler and more readable than normal ways of filtering lists . Let’s see how we can use boolean indexing to select only values under 3:
Let’s break down what we’re doing in the code above:
- We create a boolean array by evaluating arr < 3 which returns an array of boolean values of items meeting the condition
- We index using this boolean array, returning only values less than 3
Similarly, we can use this method to filter in more complex ways. For example, if we only wanted to return even items, we could use the modulo operator to filter our array:
In this tutorial, you learned how to index NumPy arrays. You first learned simple indexing, allowing you to access a single value. Then, you learned how to work with two-dimensional and three-dimensional arrays. From there, you learned how to slice and stride over NumPy arrays, similar to working with Python lists. Finally, you learned powerful boolean indexing in order to index arrays based on a condition.
Additional Resources
To learn more about related topics, check out the tutorials below:
- Flatten an Array with NumPy flatten
- NumPy where: Process Array Elements Conditionally
- NumPy linspace: Creating Evenly Spaced Arrays with np.linspace
- Official Documentation: NumPy Indexing
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
How to Use Slice Assignment in NumPy?
NumPy slice assignment allows you to use slicing on the left-hand side of an assignment operation to overwrite a specific subsequence of a NumPy array at once. The right side of the slice assignment operation provides the exact number of elements to replace the selected slice. For example, a[::2] = [...] would overwrite every other value of NumPy array a .
Here’s a minimal example of slice assignment:
The NumPy slice assignment operation doesn’t need the same shape on the left and right side because NumPy will use broadcasting to bring the array-like data structure providing the replacement data values into the same shape as the array to be overwritten.
The next example shows how to replace every other value of a 1D array with the same value. The left and right operands don’t have the same array shape—but NumPy figures it out through broadcasting .
For 2D arrays, you can use the advanced slice notation —selection comma-separated by axis—to replace whole columns like so:
Let’s dive into a practical example about NumPy slice assignments from my Python One-Liners book next. Take your time to study it and watch the explainer video to polish your NumPy slicing skills once and for all.
Practical Example Slice Assignment NumPy

Real-world data is seldomly clean: It may contain errors because of faulty sensor, or it may contain missing data because of damaged sensors. In this one-liner example, you learn about how to quickly handle smaller cleaning tasks in a single line of code .
Say, you have installed a temperature sensor in your garden to measure temperature data over a period of many weeks. Every Sunday, you uninstall the temperature sensor from the garden and take it in your house to digitize the sensor values. Now, you realize that the Sunday sensor values are faulty because they partially measured the temperature at your home and not at the outside location.
In this mini code project, you want to “clean” your data by replacing every Sunday sensor value with the average sensor value of the last seven days. But before we dive into the code, let’s explore the most important concepts you need as a basic understanding.
Examples Slice Assignment NumPy
In NumPy’s slice assignment feature, you specify the values to be replaced on the left-hand side of the equation and the values that replace them on the right-hand side of the equation.
Here is an example:
The code snippet creates an array containing 16 times the value 4. Then we use slice assignment to replace the 15 trailing sequence values with the value 16. Recall that the notation a[start:stop:step] selects the sequence starting at index “start”, ending in index “stop” (exclusive), and considering only every “step”-th sequence element. Thus, the notation a[1::] replaces all sequence elements but the first one.
This example shows how to use slice assignment with all parameters specified. An interesting twist is that we specify only a single value “16” to replace the selected elements. Do you already know the name of this feature?
Correct, broadcasting is the name of the game! The right-hand side of the equation is automatically transformed into a NumPy array. The shape of this array is equal to the left-hand array.
Before we investigate how to solve the problem with a new one-liner, let me quickly explain the shape property of NumPy arrays. Every array has an associated shape attribute (a tuple ). The i-th tuple value specifies the number of elements of the i-th axis. Hence, the number of tuple values is the dimensionality of the NumPy array.
Read over the following examples about the shapes of different arrays:
We create three arrays a , b , and c .
- Array a is 1D, so the shape tuple has only a single element.
- Array b is 2D, so the shape tuple has two elements.
- Array c is 3D, so the shape tuple has three elements.
Problem Formulation
This is everything you need to know to solve the following problem:
Given an array of temperature values, replace every seventh temperature value with the average of the last seven days.
Take a guess : what’s the output of this code snippet?
First, the puzzle creates the data matrix “ tmp ” with a one-dimensional sequence of sensor values. In every line, we define all seven sensor values for seven days of the week (Monday, Tuesday, Wednesday, Thursday, Friday, Saturday, and Sunday).
Second, we use slice assignment to replace all the Sunday values of this array. As Sunday is the seventh day of the week, the expression “ tmp[6::7] ” selects the respective Sunday values starting from the seventh element in the sequence (again: the Sunday sensor value).
Third, we reshape the one-dimensional sensor array into a two-dimensional array with seven columns. This makes it easier for us to calculate the weekly average temperature value to replace the Sunday data. Note that the dummy shape tuple value -1 (in “ tmp.reshape((-1,7)) ”) means that the number of rows ( axis 0 ) should be selected automatically. In our case, it results in the following array after reshaping :
It’s one row per week and one column per weekday.
Now we calculate the 7-day average by collapsing every row into a single average value using the np.average() function with the axis argument: axis=1 means that the second axis is collapsed into a single average value. This is the result of the right-hand side of the equation:
After replacing all Sunday sensor values, we get the following final result of the one-liner:
This example is drawn from my Python One-Liners book:
Python One-Liners Book: Master the Single Line First!
Python programmers will improve their computer science skills with these useful one-liners.
Python One-Liners will teach you how to read and write “one-liners”: concise statements of useful functionality packed into a single line of code. You’ll learn how to systematically unpack and understand any line of Python code, and write eloquent, powerfully compressed Python like an expert.
The book’s five chapters cover (1) tips and tricks, (2) regular expressions, (3) machine learning, (4) core data science topics, and (5) useful algorithms.
Detailed explanations of one-liners introduce key computer science concepts and boost your coding and analytical skills . You’ll learn about advanced Python features such as list comprehension , slicing , lambda functions , regular expressions , map and reduce functions, and slice assignments .
You’ll also learn how to:
- Leverage data structures to solve real-world problems , like using Boolean indexing to find cities with above-average pollution
- Use NumPy basics such as array , shape , axis , type , broadcasting , advanced indexing , slicing , sorting , searching , aggregating , and statistics
- Calculate basic statistics of multidimensional data arrays and the K-Means algorithms for unsupervised learning
- Create more advanced regular expressions using grouping and named groups , negative lookaheads , escaped characters , whitespaces, character sets (and negative characters sets ), and greedy/nongreedy operators
- Understand a wide range of computer science topics , including anagrams , palindromes , supersets , permutations , factorials , prime numbers , Fibonacci numbers, obfuscation , searching , and algorithmic sorting
By the end of the book, you’ll know how to write Python at its most refined , and create concise, beautiful pieces of “Python art” in merely a single line.
Get your Python One-Liners on Amazon!!
Where to Go From Here?
Do you love data science? But you struggle to get everything together and develop a good intuition about the NumPy library?
To help you improving your code understanding speed in NumPy, I co-authored a brand-new NumPy book based on puzzle-based learning. Puzzle-based learning is a new, very practical approach to learning to code — based on my experience of teaching more than 60,000 ambitious Python coders online.
Get your “ Coffee Break NumPy ” now!
While working as a researcher in distributed systems, Dr. Christian Mayer found his love for teaching computer science students.
To help students reach higher levels of Python success, he founded the programming education website Finxter.com that has taught exponential skills to millions of coders worldwide. He’s the author of the best-selling programming books Python One-Liners (NoStarch 2020), The Art of Clean Code (NoStarch 2022), and The Book of Dash (NoStarch 2022). Chris also coauthored the Coffee Break Python series of self-published books. He’s a computer science enthusiast, freelancer , and owner of one of the top 10 largest Python blogs worldwide.
His passions are writing, reading, and coding. But his greatest passion is to serve aspiring coders through Finxter and help them to boost their skills. You can join his free email academy here.
scipy.optimize.linear_sum_assignment #
Solve the linear sum assignment problem.
The cost matrix of the bipartite graph.
Calculates a maximum weight matching if true.
An array of row indices and one of corresponding column indices giving the optimal assignment. The cost of the assignment can be computed as cost_matrix[row_ind, col_ind].sum() . The row indices will be sorted; in the case of a square cost matrix they will be equal to numpy.arange(cost_matrix.shape[0]) .
for sparse inputs
The linear sum assignment problem [1] is also known as minimum weight matching in bipartite graphs. A problem instance is described by a matrix C, where each C[i,j] is the cost of matching vertex i of the first partite set (a ‘worker’) and vertex j of the second set (a ‘job’). The goal is to find a complete assignment of workers to jobs of minimal cost.
Formally, let X be a boolean matrix where \(X[i,j] = 1\) iff row i is assigned to column j. Then the optimal assignment has cost
where, in the case where the matrix X is square, each row is assigned to exactly one column, and each column to exactly one row.
This function can also solve a generalization of the classic assignment problem where the cost matrix is rectangular. If it has more rows than columns, then not every row needs to be assigned to a column, and vice versa.
This implementation is a modified Jonker-Volgenant algorithm with no initialization, described in ref. [2] .
New in version 0.17.0.
https://en.wikipedia.org/wiki/Assignment_problem
DF Crouse. On implementing 2D rectangular assignment algorithms. IEEE Transactions on Aerospace and Electronic Systems , 52(4):1679-1696, August 2016, DOI:10.1109/TAES.2016.140952
numpy.where #
Return elements chosen from x or y depending on condition .
When only condition is provided, this function is a shorthand for np.asarray(condition).nonzero() . Using nonzero directly should be preferred, as it behaves correctly for subclasses. The rest of this documentation covers only the case where all three arguments are provided.
Where True, yield x , otherwise yield y .
Values from which to choose. x , y and condition need to be broadcastable to some shape.
An array with elements from x where condition is True, and elements from y elsewhere.
The function that is called when x and y are omitted
If all the arrays are 1-D, where is equivalent to:
This can be used on multidimensional arrays too:
The shapes of x, y, and the condition are broadcast together:
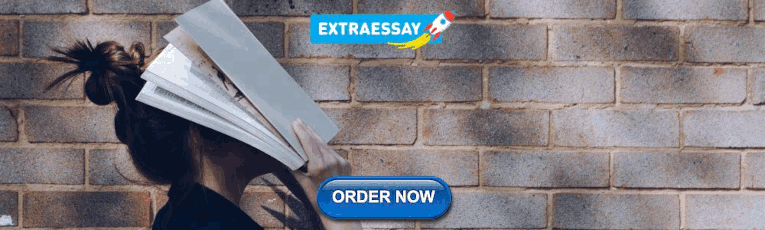
IMAGES
VIDEO
COMMENTS
Element Assignment in NumPy Arrays. We can assign new values to an element of a NumPy array using the = operator, just like regular python lists. A few examples are below (note that this is all one code block, which means that the element assignments are carried forward from step to step). array([0.12, 0.94, 0.66, 0.73, 0.83])
Use numpy.meshgrid () to make arrays of indexes that you can use to index into both your original array and the array of values for the third dimension. import numpy as np. import scipy as sp. import scipy.stats.distributions. a = np.zeros((2,3,4)) z = sp.stats.distributions.randint.rvs(0, 4, size=(2,3))
ndarrays. #. ndarrays can be indexed using the standard Python x[obj] syntax, where x is the array and obj the selection. There are different kinds of indexing available depending on obj : basic indexing, advanced indexing and field access. Most of the following examples show the use of indexing when referencing data in an array.
There are 6 general mechanisms for creating arrays: Conversion from other Python structures (i.e. lists and tuples) Intrinsic NumPy array creation functions (e.g. arange, ones, zeros, etc.) Replicating, joining, or mutating existing arrays. Reading arrays from disk, either from standard or custom formats. Creating arrays from raw bytes through ...
This function instead copies "by field name", such that fields in the dst are assigned from the identically named field in the src. This applies recursively for nested structures. This is how structure assignment worked in numpy >= 1.6 to <= 1.13. Parameters: dst ndarray src ndarray. The source and destination arrays during assignment.
Numpy arrays have a copy method which you can use for just this purpose. So if you write. b = a.copy() then Python will first actually make a copy of the array - that is, it sets aside a new region of memory, let's say at address 0x123904381, then goes to memory address 0x123674283 and copies all the values of the array from the latter section ...
An instructive first step is to visualize, given the patch size and image shape, what a higher-dimensional array of patches would look like. We have a 2d array img with shape (254, 319) and a (10, 10) 2d patch. This means our output shape (before taking the mean of each "inner" 10x10 array) would be: Python.
The NumPy array object ... Use fancy indexing on the left and array creation on the right to assign values into an array, for instance by setting parts of the array in the diagram above to zero. Table Of Contents. 1.4.1. The NumPy array object. 1.4.1.1. What are NumPy and NumPy arrays?
Data manipulation in Python is nearly synonymous with NumPy array manipulation: even newer tools like Pandas are built around the NumPy array. This section will present several examples of using NumPy array manipulation to access data and subarrays, and to split, reshape, and join the arrays. While the types of operations shown here may seem a ...
One can index and assign to a structured array with a multi-field index, where the index is a list of field names. The behavior of multi-field indexes will change from Numpy 1.15 to Numpy 1.16. In Numpy 1.16, the result of indexing with a multi-field index will be a view into the original array, as follows:
When copy=False and a copy is made for other reasons, the result is the same as if copy=True, with some exceptions for 'A', see the Notes section.The default order is 'K'. subok bool, optional. If True, then sub-classes will be passed-through, otherwise the returned array will be forced to be a base-class array (default).
Introducing Numpy Arrays. In the 2nd part of this book, we will study the numerical methods by using Python. We will use array/matrix a lot later in the book. Therefore, here we are going to introduce the most common way to handle arrays in Python using the Numpy module. Numpy is probably the most fundamental numerical computing module in Python.
delete (arr, obj [, axis]) Return a new array with sub-arrays along an axis deleted. insert (arr, obj, values [, axis]) Insert values along the given axis before the given indices. append (arr, values [, axis]) Append values to the end of an array. resize (a, new_shape) Return a new array with the specified shape.
September 16, 2022. This comprehensive guide will teach you all the different ways to index and slice NumPy arrays. NumPy is an essential library for any data analyst or data scientist using Python. Effectively indexing and slicing NumPy arrays can make you a stronger programmer. By the end of this tutorial, you'll have learned: How NumPy ...
In NumPy's slice assignment feature, you specify the values to be replaced on the left-hand side of the equation and the values that replace them on the right-hand side of the equation. Here is an example: import numpy as np. a = np.array( [4] * 16) print(a) # [4 4 4 4 4 4 4 4 4 4 4 4 4 4 4 4] a[1::] = [16] * 15.
Python has a dynamic approach when it comes to types: every element in a list can have a different type. But numpy works with matrices where all elements have the same type. Therefore assigning a float to an int matrix, will convert the row first to int s. This will construct an array: >>> arr = np.array([[3, -4, 4], [1, -2, 2]],dtype=np.float)
The NumPy array is a data structure consisting of two parts: ... It must be noted here that during the assignment of x[[1, 2]] no view or copy is created as the assignment happens in-place. Other operations# The numpy.reshape function creates a view where possible or a copy otherwise. In most cases, the strides can be modified to reshape the ...
scipy.optimize.linear_sum_assignment. #. Solve the linear sum assignment problem. The cost matrix of the bipartite graph. Calculates a maximum weight matching if true. An array of row indices and one of corresponding column indices giving the optimal assignment. The cost of the assignment can be computed as cost_matrix[row_ind, col_ind].sum().
Row assignment to numpy array. 8. Assign values to a numpy array for each row with specified columns. 1. Python, Numpy: Cannot assign the values of a numpy array to a column of a matrix. 1. Assign values to multiple columns of numpy matrix without looping. 0.
numpy.where(condition, [x, y, ]/) #. Return elements chosen from x or y depending on condition. Note. When only condition is provided, this function is a shorthand for np.asarray(condition).nonzero(). Using nonzero directly should be preferred, as it behaves correctly for subclasses. The rest of this documentation covers only the case where all ...
OK, my mistake, unlike Pytorch, numpy.array () ONLY creates 1D arrays. The correct behaviour would be to do something like total_array = np.zeros () or np.empty () np.array can create 2d arrsys - if you give a nested list. total_array[0,0]=1 is the more idiomatic way of indexing a 2d array.