
- PHP All Exercises & Assignments
Practice your PHP skills using PHP Exercises & Assignments. Tutorials Class provides you exercises on PHP basics, variables, operators, loops, forms, and database.
Once you learn PHP, it is important to practice to understand PHP concepts. This will also help you with preparing for PHP Interview Questions.
Here, you will find a list of PHP programs, along with problem description and solution. These programs can also be used as assignments for PHP students.
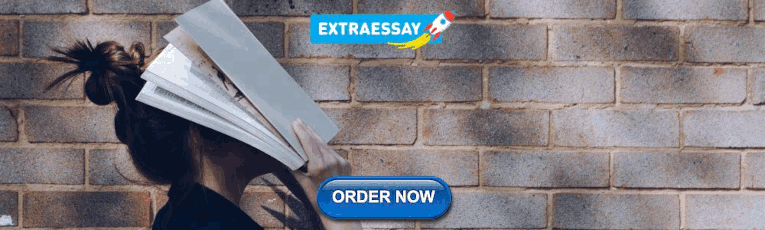
Write a program to count 5 to 15 using PHP loop
Description: Write a Program to display count, from 5 to 15 using PHP loop as given below.
Rules & Hint
- You can use “for” or “while” loop
- You can use variable to initialize count
- You can use html tag for line break
View Solution/Program
5 6 7 8 9 10 11 12 13 14 15
Write a program to print “Hello World” using echo
Description: Write a program to print “Hello World” using echo only?
Conditions:
- You can not use any variable.
View Solution /Program
Hello World
Write a program to print “Hello PHP” using variable
Description: Write a program to print “Hello PHP” using php variable?
- You can not use text directly in echo but can use variable.
Write a program to print a string using echo+variable.
Description: Write a program to print “Welcome to the PHP World” using some part of the text in variable & some part directly in echo.
- You have to use a variable that contains string “PHP World”.
Welcome to the PHP World
Write a program to print two variables in single echo
Description: Write a program to print 2 php variables using single echo statement.
- First variable have text “Good Morning.”
- Second variable have text “Have a nice day!”
- Your output should be “Good morning. Have a nice day!”
- You are allowed to use only one echo statement in this program.
Good Morning. Have a nice day!
Write a program to check student grade based on marks
Description:.
Write a program to check student grade based on the marks using if-else statement.
- If marks are 60% or more, grade will be First Division.
- If marks between 45% to 59%, grade will be Second Division.
- If marks between 33% to 44%, grade will be Third Division.
- If marks are less than 33%, student will be Fail.
Click to View Solution/Program
Third Division
Write a program to show day of the week using switch
Write a program to show day of the week (for example: Monday) based on numbers using switch/case statements.
- You can pass 1 to 7 number in switch
- Day 1 will be considered as Monday
- If number is not between 1 to 7, show invalid number in default
It is Friday!
Write a factorial program using for loop in php
Write a program to calculate factorial of a number using for loop in php.
The factorial of 3 is 6
Factorial program in PHP using recursive function
Exercise Description: Write a PHP program to find factorial of a number using recursive function .
What is Recursive Function?
- A recursive function is a function that calls itself.
Write a program to create Chess board in PHP using for loop
Write a PHP program using nested for loop that creates a chess board.
- You can use html table having width=”400px” and take “30px” as cell height and width for check boxes.

Write a Program to create given pattern with * using for loop
Description: Write a Program to create following pattern using for loops:
- You can use for or while loop
- You can use multiple (nested) loop to draw above pattern
View Solution/Program using two for loops
* ** *** **** ***** ****** ******* ********
Simple Tips for PHP Beginners
When a beginner start PHP programming, he often gets some syntax errors. Sometimes these are small errors but takes a lot of time to fix. This happens when we are not familiar with the basic syntax and do small mistakes in programs. These mistakes can be avoided if you practice more and taking care of small things.
I would like to say that it is never a good idea to become smart and start copying. This will save your time but you would not be able to understand PHP syntax. Rather, Type your program and get friendly with PHP code.
Follow Simple Tips for PHP Beginners to avoid errors in Programming
- Start with simple & small programs.
- Type your PHP program code manually. Do not just Copy Paste.
- Always create a new file for new code and keep backup of old files. This will make it easy to find old programs when needed.
- Keep your PHP files in organized folders rather than keeping all files in same folder.
- Use meaningful names for PHP files or folders. Some examples are: “ variable-test.php “, “ loops.php ” etc. Do not just use “ abc.php “, “ 123.php ” or “ sample.php “
- Avoid space between file or folder names. Use hyphens (-) instead.
- Use lower case letters for file or folder names. This will help you make a consistent code
These points are not mandatory but they help you to make consistent and understandable code. Once you practice this for 20 to 30 PHP programs, you can go further with more standards.
The PHP Standard Recommendation (PSR) is a PHP specification published by the PHP Framework Interop Group.
Experiment with Basic PHP Syntax Errors
When you start PHP Programming, you may face some programming errors. These errors stops your program execution. Sometimes you quickly find your solutions while sometimes it may take long time even if there is small mistake. It is important to get familiar with Basic PHP Syntax Errors
Basic Syntax errors occurs when we do not write PHP Code correctly. We cannot avoid all those errors but we can learn from them.
Here is a working PHP Code example to output a simple line.
Output: Hello World!
It is better to experiment with PHP Basic code and see what errors happens.
- Remove semicolon from the end of second line and see what error occurs
- Remove double quote from “Hello World!” what error occurs
- Remove PHP ending statement “?>” error occurs
- Use “
- Try some space between “
Try above changes one at a time and see error. Observe What you did and what error happens.
Take care of the line number mentioned in error message. It will give you hint about the place where there is some mistake in the code.
Read Carefully Error message. Once you will understand the meaning of these basic error messages, you will be able to fix them later on easily.
Note: Most of the time error can be found in previous line instead of actual mentioned line. For example: If your program miss semicolon in line number 6, it will show error in line number 7.
Using phpinfo() – Display PHP Configuration & Modules
phpinfo() is a PHP built-in function used to display information about PHP’s configuration settings and modules.
When we install PHP, there are many additional modules also get installed. Most of them are enabled and some are disabled. These modules or extensions enhance PHP functionality. For example, the date-time extension provides some ready-made function related to date and time formatting. MySQL modules are integrated to deal with PHP Connections.
It is good to take a look on those extensions. Simply use
phpinfo() function as given below.
Example Using phpinfo() function

Write a PHP program to add two numbers
Write a program to perform sum or addition of two numbers in PHP programming. You can use PHP Variables and Operators
PHP Program to add two numbers:
Write a program to calculate electricity bill in php.
You need to write a PHP program to calculate electricity bill using if-else conditions.
- For first 50 units – Rs. 3.50/unit
- For next 100 units – Rs. 4.00/unit
- For next 100 units – Rs. 5.20/unit
- For units above 250 – Rs. 6.50/unit
- You can use conditional statements .
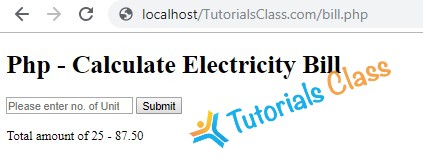
Write a simple calculator program in PHP using switch case
You need to write a simple calculator program in PHP using switch case.
Operations:
- Subtraction
- Multiplication

Remove specific element by value from an array in PHP?
You need to write a program in PHP to remove specific element by value from an array using PHP program.
Instructions:
- Take an array with list of month names.
- Take a variable with the name of value to be deleted.
- You can use PHP array functions or foreach loop.
Solution 1: Using array_search()
With the help of array_search() function, we can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 2: Using foreach()
By using foreach() loop, we can also remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [3]=> string(5) “april” [4]=> string(3) “may” }
Solution 3: Using array_diff()
With the help of array_diff() function, we also can remove specific elements from an array.
array(4) { [0]=> string(3) “jan” [1]=> string(3) “feb” [2]=> string(5) “march” [4]=> string(3) “may” }
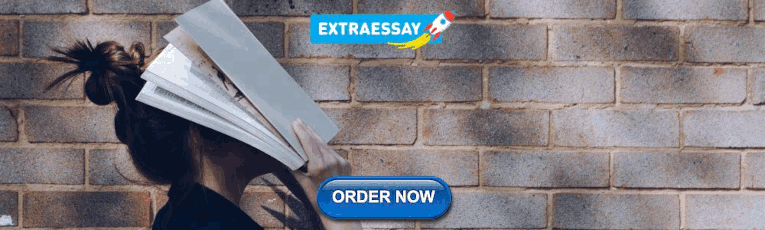
Write a PHP program to check if a person is eligible to vote
Write a PHP program to check if a person is eligible to vote or not.
- Minimum age required for vote is 18.
- You can use PHP Functions .
- You can use Decision Making Statements .
Click to View Solution/Program.
You Are Eligible For Vote
Write a PHP program to calculate area of rectangle
Write a PHP program to calculate area of rectangle by using PHP Function.
- You must use a PHP Function .
- There should be two arguments i.e. length & width.
View Solution/Program.
Area Of Rectangle with length 2 & width 4 is 8 .
- Next »
- PHP Exercises Categories
- PHP Top Exercises
- PHP Variables
- PHP Decision Making
- PHP Functions
- PHP Operators
- Sat. Jul 13th, 2024
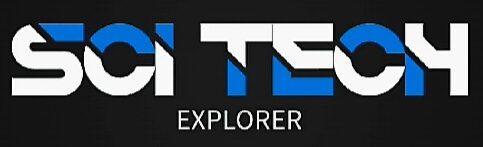
Sci Tech Explorer

Internet Programming 2

Internet programming semester 2 lab book all programs with solution, Php TYBCS Computer Science Lab book or Workbook .
Internet Programming 2: Assignment 6
Internet programming 2: assignment 7, internet programming 2: assignment 8, internet programming 2: assignment 9, internet programming 2: assignment 10, internet programming 2: assignment 11, china to build world’s largest underwater ‘ghost particle’ detector, israel conflict with palestine 2023, q2.write the html code which generates the following output., q3. write the html code for generating the form as shown below….
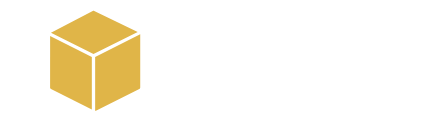
Space for google add
- _Practical's
- __JavaScript
- __Visual Basic
LAB COURSE II TYBCS- Solved Assignments | JAVA - csprogramx

👉 Assignment 6
👉 assignment 2 , set a, 1. create a student table with fields roll number, name, percentage. insert values in the table. display all the details of the student table in a tabular format on the screen (using swing). 2. write a program to display information about the database and list all the tables in the database. (use databasemetadata). 3 . write a program to display information about all columns in the student table. (use resultsetmetadata)., 1. write a menu driven program (command line interface) to perform the following operations on student table. 1. insert 2. modify 3. delete 4. search 5. view all 6. exit , 1. write a program that create 2 threads – each displaying a message (pass the message as a parameter to the constructor). the threads should display the messages continuously till the user presses ctrl-c. also display the thread information as it is running., 2. write a java program to calculate the sum and average of an array of 1000 integers (generated randomly) using 10 threads. each thread calculates the sum of 100 integers. use these values to calculate average. [use join method ].
👉 Assignment 6
Post a Comment

Please send me solutions for java sem 2
TYBCS JAVA SEM II assignment are not visible
If anyone has Doubts or suggestions please let me know
Search This Blog
- January 2023 3
- July 2022 4
- June 2022 5
- January 2022 13
- June 2021 1
- December 2020 26
- November 2020 21
- October 2020 53
Report Abuse
- Privacy Policy
Popular Posts

Define a class Employee .Create a subclass called “Manager” . display the details of the manager having the max total salary.

LAB COURSE III TYBCS Assignments | PHP - csprogramx

Create a form to accept student information using cookies.
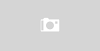
System Programming & Operating System Lab I
Menu footer widget.
- Privacy Policy
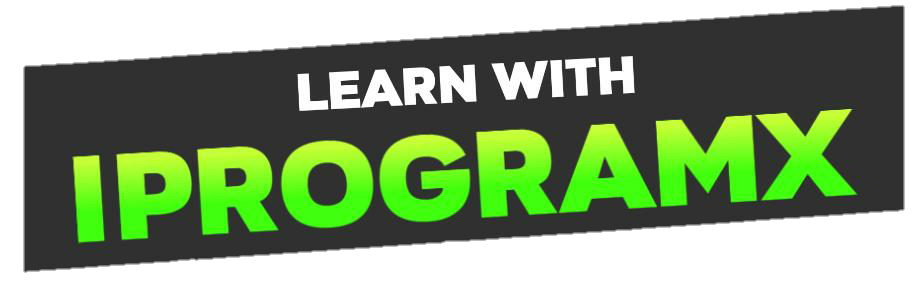
- _HTML & CSS
- _PHP Solve Slips
- _PHP Project Topics
- _OS/SysPro Slips
- Download Apk
TYBCS PHP Assignment 3
Post a comment.

what about setc???
I need setc??????
I want set c answers??
This comment has been removed by the author.
i have done all of them. specially i enjoyed with Set c Q2.
Can u pls send me that solution!
how to run set b q1 plz tell
Social Plugin
Popular posts.
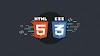
Create an html5 page of My College | HTML & CSS - IProgramX
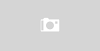
TYBcs OS-Syspro Slip 2-2 | IProgramX
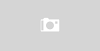
TYBcs OS-Syspro Slip 1-1 | IProgramX
Total pageviews, contact form.
- html & css 11
- php solve slips 32
Menu Footer Widget
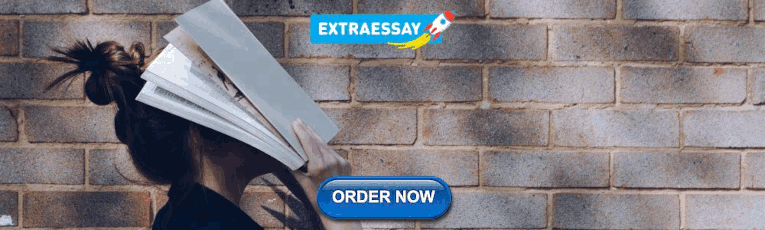
IMAGES
VIDEO
COMMENTS
Q. 2 Write a PHP script for the following: Design a form to accept two strings from the user. Find the first occurrence and the last occurrence of the small ...
Slip16: Write a PHP script for the following: Design a form to accept the marks of 5 different subjects of a student, having serial number, subject name & marks out of 100. Display theresult in the tabular format which will have total, percentage and grade.
TYBCS PHP Assignment 1. Q: 1) Write a PHP script for the following: Design a form to accept a string. Write a function to count the total number of vowels (a,e,i,o,u) from the string. Show the occurrences of each vowel from the string. Check whether the given string is a palindrome or not, without using built-in function.
TYBCS PHP Assignment 2. a) Display the elements of an array along with the keys. c) Delete an element from an array from the given key/index. e) Traverse the elements in an array in random order [ [Hint: use shuffle ()]. Q:2) Accept a string from the user and check whether it is a palindrome or not (Implement stack operations using array built ...
TYBCS PHP Assignment 1 Assignment 2 Assignment 3 Assignment 4 Assignment 5. NEW UPDATE. Post a Comment. 14 Comments. Karan February 5, 2019 at 8:38 AM. what about assignment no 6-11? Reply Delete. Replies. Reply. masonry stone work January 5, 2020 at 7:03 PM. Please i want assignment no 6 to 11.
Find and fix vulnerabilities Codespaces. Instant dev environments
Accept a string to be searched. Search for the string in all text files in the current folder. Use a separate thread for each file. The result should display the filename, line number where the string is found. for executing this program 1.you have create one folder and give name according to you (folder name is thread) 2.
Q) Write a PHP script to accept 2 strings from the user, the first string should be a sentence and second can be a word. a. Delete a small part from first string after accepting position and number of characters to remove. b. Insert the given small string in the given big string at specified position without removing any characters from the big ...
Write a program to print 2 php variables using single echo statement. Conditions: First variable have text "Good Morning." Second variable have text "Have a nice day!" Your output should be "Good morning. Have a nice day!" You are allowed to use only one echo statement in this program. View Solution/Program
Semester-IV. CS-341:Paper-I: Systems Programming and Operating System - II CS-342:Paper-II: Theoretical Computer Science and Compiler Construction-II CS-343:Paper-III: Computer Networks-II CS-344:Paper-IV: Web Development and PHP programming-II CS-345:Paper-V: Programming in Java-II CS-346:Paper-VI: Business Applications.
Q: 2) Write a menu driven program to perform the following operations on. associative arrays: 1. Sort the array by values (changing the keys) in ascending, descending order. 2. Also sort the array by values without changing the keys. 3. Filter the odd elements from an array. 4. Sort the different arrays at a glance using single function. 5.
Internet programming semester 2 lab book all programs with solution, Php TYBCS Computer Science Lab book or Workbook. Internet Programming 2: Assignment 6 Internet Programming 2: Assignment 7
👉 Assignment 2 SET A 1. Create a student table with fields roll number, name, percentage. Insert values in the table. ... LAB COURSE III TYBCS Assignments | PHP - csprogramx . October 31, 2020. Create a form to accept student information using cookies. November 02, 2020.
Assignment Related Programs are Here. Contribute to shubhamdsk/java-TYBCS-practical-Assignments-with-Solution development by creating an account on GitHub.
TYBCS IP SLIPS 10 Marks. 1) Design HTML form, accept student Name, Age, and Mobile No. from user. Using java Script validate for following. a) Student Name should not be empty. b) Student Age must be between 1 to 20. 2) . Design a HTML form to accept a string. Write a PHP script for the following.
Q.1. Define a Student class (roll number, name, percentage). Define a default and parameterized constructor. Keep a count of objects created. Create objects...
GitHub - Prasad-RP/Java-Practical-Assignment-TYBCS: All Programs with Solution. This repository has been archived by the owner on Jan 9, 2024. It is now read-only. Prasad-RP / Java-Practical-Assignment-TYBCS Public archive. Notifications.
TYBCS Java Assignment 2 - Sem I. 1. Define a Student class (roll number, name, percentage). Define a default and parameterized constructor. Keep a count of objects created. Create objects using parameterized constructor and display the object count after each object is created. (Use static member and method).
TYBCS PHP Assignment 3. Q: 1) Write a program to read two file names from user and append contents of first file into second file. Q: 2) Write program to read directory name from user and display content of the directory. Q: 1) Write a program to read a flat file "student.dat", calculate the percentage and display the data from file in ...
Assignment Related Programs are Here. Contribute to shubhamdsk/java-TYBCS-practical-Assignments-with-Solution development by creating an account on GitHub.
2]And then you have to create second package as name TY (i.e you have to create one folder named as TY) in this folder save the program TYMarks.java and then compile this program in same directory as javac TYMarks.java and then java TYMarks.(Use program TYMarks.java).