- Graphics and multimedia
- Language Features
- Unix/Linux programming
- Source Code
- Standard Library
- Tips and Tricks
- Tools and Libraries
- Windows API
- Copy constructors, assignment operators,
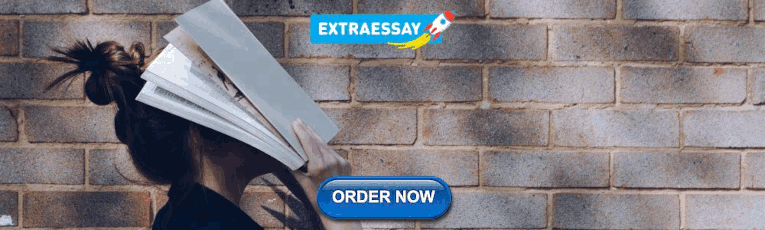
Copy constructors, assignment operators, and exception safe assignment

Learn C++ practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c++ interactively, introduction to c++.
- Getting Started With C++
- Your First C++ Program
- C++ Comments
C++ Fundamentals
- C++ Keywords and Identifiers
- C++ Variables, Literals and Constants
- C++ Data Types
- C++ Type Modifiers
- C++ Constants
- C++ Basic Input/Output
- C++ Operators
Flow Control
- C++ Relational and Logical Operators
- C++ if, if...else and Nested if...else
- C++ for Loop
- C++ while and do...while Loop
- C++ break Statement
- C++ continue Statement
- C++ goto Statement
- C++ switch..case Statement
- C++ Ternary Operator
- C++ Functions
- C++ Programming Default Arguments
- C++ Function Overloading
- C++ Inline Functions
- C++ Recursion
Arrays and Strings
- C++ Array to Function
- C++ Multidimensional Arrays
- C++ String Class
Pointers and References
- C++ Pointers
- C++ Pointers and Arrays
- C++ References: Using Pointers
- C++ Call by Reference: Using pointers
- C++ Memory Management: new and delete
Structures and Enumerations
- C++ Structures
- C++ Structure and Function
- C++ Pointers to Structure
- C++ Enumeration
Object Oriented Programming
C++ Classes and Objects
C++ Constructors
C++ Constructor Overloading
C++ Destructors
- C++ Access Modifiers
- C++ Encapsulation
- C++ friend Function and friend Classes
Inheritance & Polymorphism
- C++ Inheritance
- C++ Public, Protected and Private Inheritance
- C++ Multiple, Multilevel and Hierarchical Inheritance
- C++ Function Overriding
- C++ Virtual Functions
- C++ Abstract Class and Pure Virtual Function
STL - Vector, Queue & Stack
- C++ Standard Template Library
- C++ STL Containers
- C++ std::array
- C++ Vectors
- C++ Forward List
- C++ Priority Queue
STL - Map & Set
- C++ Multimap
- C++ Multiset
- C++ Unordered Map
- C++ Unordered Set
- C++ Unordered Multiset
- C++ Unordered Multimap
STL - Iterators & Algorithms
- C++ Iterators
- C++ Algorithm
- C++ Functor
Additional Topics
- C++ Exceptions Handling
- C++ File Handling
- C++ Ranged for Loop
- C++ Nested Loop
- C++ Function Template
C++ Class Templates
- C++ Type Conversion
- C++ Type Conversion Operators
- C++ Operator Overloading
Advanced Topics
- C++ Namespaces
- C++ Preprocessors and Macros
- C++ Storage Class
- C++ Bitwise Operators
- C++ Buffers
- C++ istream
- C++ ostream
C++ Tutorials
- C++ clock()
- How to pass and return object from C++ Functions?
A constructor is a special member function that is called automatically when an object is created.
In C++, a constructor has the same name as that of the class , and it does not have a return type. For example,
Here, the function Wall() is a constructor of the class Wall . Notice that the constructor
- has the same name as the class,
- does not have a return type, and
- C++ Default Constructor
A constructor with no parameters is known as a default constructor . For example,
Here, when the wall1 object is created, the Wall() constructor is called. length{5.5} is invoked when the constructor is called, and sets the length variable of the object to 5.5 .
Note: If we have not defined any constructor, copy constructor, or move constructor in our class, then the C++ compiler will automatically create a default constructor with no parameters and empty body.
Defaulted Constructor
When we have to rely on default constructor to initialize the member variables of a class, we should explicitly mark the constructor as default in the following way:
If we want to set a default value, then we should use value initialization. That is, we include the default value inside braces in the declaration of member variables in the follwing way.
Let's see an example:
- C++ Parameterized Constructor
In C++, a constructor with parameters is known as a parameterized constructor. This is the preferred method to initialize member data. For example,
Here, we have defined a parameterized constructor Wall() that has two parameters: double len and double hgt . The values contained in these parameters are used to initialize the member variables length and height .
: length{len}, height{hgt} is the member initializer list.
- length{len} initializes the member variable length with the value of the parameter len
- height{hgt} initializes the member variable height with the value of the parameter hgt .
When we create an object of the Wall class, we pass the values for the member variables as arguments. The code for this is:
With the member variables thus initialized, we can now calculate the area of the wall with the calculateArea() method.
Note : A constructor is primarily used to initialize objects. They are also used to run a default code when an object is created.
- C++ Member Initializer List
Consider the constructor:
is member initializer list.
The member initializer list is used to initialize the member variables of a class.
The order or initialization of the member variables is according to the order of their declaration in the class rather than their declaration in the member initializer list.
Since the member variables are declared in the class in the following order:
The length variable will be initialized first even if we define our constructor as following:
- C++ Copy Constructor
The copy constructor in C++ is used to copy data from one object to another. For example,
In this program, we have used a copy constructor to copy the contents of one object of the Wall class to another. The code of the copy constructor is:
Notice that the parameter of this constructor has the address of an object of the Wall class.
We then assign the values of the variables of the obj object to the corresponding variables of the object, calling the copy constructor. This is how the contents of the object are copied.
In main() , we then create two objects wall1 and wall2 and then copy the contents of wall1 to wall2 :
Here, the wall2 object calls its copy constructor by passing the reference of the wall1 object as its argument.
- C++ Default Copy Constructor
If we don't define any copy constructor, move constructor, or move assignment in our class, then the C++ compiler will automatically create a default copy constructor that does memberwise copy assignment. It suffices in most cases. For example,
In this program, we have not defined a copy constructor. The compiler used the default copy constructor to copy the contents of one object of the Wall class to another.
- C++ Friend Function and Classes
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
C++ Tutorial
cppreference.com
Copy assignment operator.
A copy assignment operator is a non-template non-static member function with the name operator = that can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
For the formal copy assignment operator syntax, see function declaration . The syntax list below only demonstrates a subset of all valid copy assignment operator syntaxes.
[ edit ] Explanation
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type, the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M & or const volatile M & .
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) .
Due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument.
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
The implicitly-declared (or defaulted on its first declaration) copy assignment operator has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is neither deleted nor trivial, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++14) . For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types, the operator performs member-wise copy assignment of the object's direct bases and non-static data members, in their initialization order, using built-in assignment for the scalars, memberwise copy-assignment for arrays, and copy assignment operator for class types (called non-virtually).
[ edit ] Deleted copy assignment operator
An implicitly-declared or explicitly-defaulted (since C++11) copy assignment operator for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a non-static data member of a const-qualified non-class type (or possibly multi-dimensional array thereof).
- T has a non-static data member of a reference type.
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that the overload resolution as applied to find M 's copy assignment operator
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- it is not user-provided (meaning, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy assignment operator selected for every direct base of T is trivial;
- the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial.
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Eligible copy assignment operator
Triviality of eligible copy assignment operators determines whether the class is a trivially copyable type .
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either a prvalue such as a nameless temporary or an xvalue such as the result of std::move ), and selects the copy assignment if the argument is an lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
It is unspecified whether virtual base class subobjects that are accessible through more than one path in the inheritance lattice, are assigned more than once by the implicitly-defined copy assignment operator (same applies to move assignment ).
See assignment operator overloading for additional detail on the expected behavior of a user-defined copy-assignment operator.
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy constructor
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 16:13.
- This page has been accessed 1,374,856 times.
- Privacy policy
- About cppreference.com
- Disclaimers


22.3 — Move constructors and move assignment
In lesson 22.1 -- Introduction to smart pointers and move semantics , we took a look at std::auto_ptr, discussed the desire for move semantics, and took a look at some of the downsides that occur when functions designed for copy semantics (copy constructors and copy assignment operators) are redefined to implement move semantics.
In this lesson, we’ll take a deeper look at how C++11 resolves these problems via move constructors and move assignment.
Recapping copy constructors and copy assignment
First, let’s take a moment to recap copy semantics.
Copy constructors are used to initialize a class by making a copy of an object of the same class. Copy assignment is used to copy one class object to another existing class object. By default, C++ will provide a copy constructor and copy assignment operator if one is not explicitly provided. These compiler-provided functions do shallow copies, which may cause problems for classes that allocate dynamic memory. So classes that deal with dynamic memory should override these functions to do deep copies.
Returning back to our Auto_ptr smart pointer class example from the first lesson in this chapter, let’s look at a version that implements a copy constructor and copy assignment operator that do deep copies, and a sample program that exercises them:
In this program, we’re using a function named generateResource() to create a smart pointer encapsulated resource, which is then passed back to function main(). Function main() then assigns that to an existing Auto_ptr3 object.
When this program is run, it prints:
(Note: You may only get 4 outputs if your compiler elides the return value from function generateResource())
That’s a lot of resource creation and destruction going on for such a simple program! What’s going on here?
Let’s take a closer look. There are 6 key steps that happen in this program (one for each printed message):
- Inside generateResource(), local variable res is created and initialized with a dynamically allocated Resource, which causes the first “Resource acquired”.
- Res is returned back to main() by value. We return by value here because res is a local variable -- it can’t be returned by address or reference because res will be destroyed when generateResource() ends. So res is copy constructed into a temporary object. Since our copy constructor does a deep copy, a new Resource is allocated here, which causes the second “Resource acquired”.
- Res goes out of scope, destroying the originally created Resource, which causes the first “Resource destroyed”.
- The temporary object is assigned to mainres by copy assignment. Since our copy assignment also does a deep copy, a new Resource is allocated, causing yet another “Resource acquired”.
- The assignment expression ends, and the temporary object goes out of expression scope and is destroyed, causing a “Resource destroyed”.
- At the end of main(), mainres goes out of scope, and our final “Resource destroyed” is displayed.
So, in short, because we call the copy constructor once to copy construct res to a temporary, and copy assignment once to copy the temporary into mainres, we end up allocating and destroying 3 separate objects in total.
Inefficient, but at least it doesn’t crash!
However, with move semantics, we can do better.
Move constructors and move assignment
C++11 defines two new functions in service of move semantics: a move constructor, and a move assignment operator. Whereas the goal of the copy constructor and copy assignment is to make a copy of one object to another, the goal of the move constructor and move assignment is to move ownership of the resources from one object to another (which is typically much less expensive than making a copy).
Defining a move constructor and move assignment work analogously to their copy counterparts. However, whereas the copy flavors of these functions take a const l-value reference parameter (which will bind to just about anything), the move flavors of these functions use non-const rvalue reference parameters (which only bind to rvalues).
Here’s the same Auto_ptr3 class as above, with a move constructor and move assignment operator added. We’ve left in the deep-copying copy constructor and copy assignment operator for comparison purposes.
The move constructor and move assignment operator are simple. Instead of deep copying the source object (a) into the implicit object, we simply move (steal) the source object’s resources. This involves shallow copying the source pointer into the implicit object, then setting the source pointer to null.
When run, this program prints:
That’s much better!
The flow of the program is exactly the same as before. However, instead of calling the copy constructor and copy assignment operators, this program calls the move constructor and move assignment operators. Looking a little more deeply:
- Res is returned back to main() by value. Res is move constructed into a temporary object, transferring the dynamically created object stored in res to the temporary object. We’ll talk about why this happens below.
- Res goes out of scope. Because res no longer manages a pointer (it was moved to the temporary), nothing interesting happens here.
- The temporary object is move assigned to mainres. This transfers the dynamically created object stored in the temporary to mainres.
- The assignment expression ends, and the temporary object goes out of expression scope and is destroyed. However, because the temporary no longer manages a pointer (it was moved to mainres), nothing interesting happens here either.
So instead of copying our Resource twice (once for the copy constructor and once for the copy assignment), we transfer it twice. This is more efficient, as Resource is only constructed and destroyed once instead of three times.
Related content
Move constructors and move assignment should be marked as noexcept . This tells the compiler that these functions will not throw exceptions.
We introduce noexcept in lesson 27.9 -- Exception specifications and noexcept and discuss why move constructors and move assignment are marked as noexcept in lesson 27.10 -- std::move_if_noexcept .
When are the move constructor and move assignment called?
The move constructor and move assignment are called when those functions have been defined, and the argument for construction or assignment is an rvalue. Most typically, this rvalue will be a literal or temporary value.
The copy constructor and copy assignment are used otherwise (when the argument is an lvalue, or when the argument is an rvalue and the move constructor or move assignment functions aren’t defined).
Implicit move constructor and move assignment operator
The compiler will create an implicit move constructor and move assignment operator if all of the following are true:
- There are no user-declared copy constructors or copy assignment operators.
- There are no user-declared move constructors or move assignment operators.
- There is no user-declared destructor.
The implicit move constructor and implicit move assignment operator both do a memberwise move. That is, each member of the moved-from object is moved to the moved-to object.
The key insight behind move semantics
You now have enough context to understand the key insight behind move semantics.
If we construct an object or do an assignment where the argument is an l-value, the only thing we can reasonably do is copy the l-value. We can’t assume it’s safe to alter the l-value, because it may be used again later in the program. If we have an expression “a = b” (where b is an lvalue), we wouldn’t reasonably expect b to be changed in any way.
However, if we construct an object or do an assignment where the argument is an r-value, then we know that r-value is just a temporary object of some kind. Instead of copying it (which can be expensive), we can simply transfer its resources (which is cheap) to the object we’re constructing or assigning. This is safe to do because the temporary will be destroyed at the end of the expression anyway, so we know it will never be used again!
C++11, through r-value references, gives us the ability to provide different behaviors when the argument is an r-value vs an l-value, enabling us to make smarter and more efficient decisions about how our objects should behave.
Key insight
Move semantics is an optimization opportunity.
Move functions should always leave both objects in a valid state
In the above examples, both the move constructor and move assignment functions set a.m_ptr to nullptr. This may seem extraneous -- after all, if a is a temporary r-value, why bother doing “cleanup” if parameter a is going to be destroyed anyway?
The answer is simple: When a goes out of scope, the destructor for a will be called, and a.m_ptr will be deleted. If at that point, a.m_ptr is still pointing to the same object as m_ptr , then m_ptr will be left as a dangling pointer. When the object containing m_ptr eventually gets used (or destroyed), we’ll get undefined behavior.
When implementing move semantics, it is important to ensure the moved-from object is left in a valid state, so that it will destruct properly (without creating undefined behavior).
Automatic l-values returned by value may be moved instead of copied
In the generateResource() function of the Auto_ptr4 example above, when variable res is returned by value, it is moved instead of copied, even though res is an l-value. The C++ specification has a special rule that says automatic objects returned from a function by value can be moved even if they are l-values. This makes sense, since res was going to be destroyed at the end of the function anyway! We might as well steal its resources instead of making an expensive and unnecessary copy.
Although the compiler can move l-value return values, in some cases it may be able to do even better by simply eliding the copy altogether (which avoids the need to make a copy or do a move at all). In such a case, neither the copy constructor nor move constructor would be called.
Disabling copying
In the Auto_ptr4 class above, we left in the copy constructor and assignment operator for comparison purposes. But in move-enabled classes, it is sometimes desirable to delete the copy constructor and copy assignment functions to ensure copies aren’t made. In the case of our Auto_ptr class, we don’t want to copy our templated object T -- both because it’s expensive, and whatever class T is may not even support copying!
Here’s a version of Auto_ptr that supports move semantics but not copy semantics:
If you were to try to pass an Auto_ptr5 l-value to a function by value, the compiler would complain that the copy constructor required to initialize the function parameter has been deleted. This is good, because we should probably be passing Auto_ptr5 by const l-value reference anyway!
Auto_ptr5 is (finally) a good smart pointer class. And, in fact the standard library contains a class very much like this one (that you should use instead), named std::unique_ptr. We’ll talk more about std::unique_ptr later in this chapter.
Another example
Let’s take a look at another class that uses dynamic memory: a simple dynamic templated array. This class contains a deep-copying copy constructor and copy assignment operator.
Now let’s use this class in a program. To show you how this class performs when we allocate a million integers on the heap, we’re going to leverage the Timer class we developed in lesson 18.4 -- Timing your code . We’ll use the Timer class to time how fast our code runs, and show you the performance difference between copying and moving.
On one of the author’s machines, in release mode, this program executed in 0.00825559 seconds.
Now let’s run the same program again, replacing the copy constructor and copy assignment with a move constructor and move assignment.
On the same machine, this program executed in 0.0056 seconds.
Comparing the runtime of the two programs, (0.00825559 - 0.0056) / 0.00825559 * 100 = 32.1% faster!
Do not implement move semantics using std::swap
Since the goal of move semantics is to move a resource from a source object to a destination object, you might think about implementing the move constructor and move assignment operator using std::swap() . However, this is a bad idea, as std::swap() calls both the move constructor and move assignment on move-capable objects, which would result in an infinite recursion. You can see this happen in the following example:
This prints:
And so on… until the stack overflows.
You can implement the move constructor and move assignment using your own swap function, as long as your swap member function does not call the move constructor or move assignment. Here’s an example of how that can be done:
This works as expected, and prints:
Deleting the move constructor and move assignment
You can delete the move constructor and move assignment using the = delete syntax in the exact same way you can delete the copy constructor and copy assignment.
If you delete the copy constructor, the compiler will not generate an implicit move constructor (making your objects neither copyable nor movable). Therefore, when deleting the copy constructor, it is useful to be explicit about what behavior you want from your move constructors. Either explicitly delete them (making it clear this is the desired behavior), or default them (making the class move-only).
The rule of five says that if the copy constructor, copy assignment, move constructor, move assignment, or destructor are defined or deleted, then each of those functions should be defined or deleted.
While deleting only the move constructor and move assignment may seem like a good idea if you want a copyable but not movable object, this has the unfortunate consequence of making the class not returnable by value in cases where mandatory copy elision does not apply. This happens because a deleted move constructor is still declared, and thus is eligible for overload resolution. And return by value will favor a deleted move constructor over a non-deleted copy constructor. This is illustrated by the following program:
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Assignment Operators In C++
- Move Assignment Operator in C++ 11
- JavaScript Assignment Operators
- Assignment Operators in Programming
- Is assignment operator inherited?
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- bitset operator[] in C++ STL
- C++ Assignment Operator Overloading
- Self assignment check in assignment operator
- Copy Constructor vs Assignment Operator in C++
- Operators in C++
- C++ Arithmetic Operators
- Bitwise Operators in C++
- Casting Operators in C++
- Assignment Operators in C
- Assignment Operators in Python
- Compound assignment operators in Java
- Arithmetic Operators in C
- Operators in C
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other words, it is used to store some kind of information.
The right-hand side value will be assigned to the variable on the left-hand side. The variable and the value should be of the same data type.
The value can be a literal or another variable of the same data type.
Compound Assignment Operators
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++:
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
- Bitwise AND Assignment Operator ( &= )
- Bitwise OR Assignment Operator ( |= )
- Bitwise XOR Assignment Operator ( ^= )
- Left Shift Assignment Operator ( <<= )
- Right Shift Assignment Operator ( >>= )
Lets see each of them in detail.
1. Addition Assignment Operator (+=)
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way.
This above expression is equivalent to the expression:
2. Subtraction Assignment Operator (-=)
The subtraction assignment operator (-=) in C++ enables you to update the value of the variable by subtracting another value from it. This operator is especially useful when you need to perform subtraction and store the result back in the same variable.
3. Multiplication Assignment Operator (*=)
In C++, the multiplication assignment operator (*=) is used to update the value of the variable by multiplying it with another value.
4. Division Assignment Operator (/=)
The division assignment operator divides the variable on the left by the value on the right and assigns the result to the variable on the left.
5. Modulus Assignment Operator (%=)
The modulus assignment operator calculates the remainder when the variable on the left is divided by the value or variable on the right and assigns the result to the variable on the left.
6. Bitwise AND Assignment Operator (&=)
This operator performs a bitwise AND between the variable on the left and the value on the right and assigns the result to the variable on the left.
7. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator performs a bitwise OR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
8. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator performs a bitwise XOR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
9. Left Shift Assignment Operator (<<=)
The left shift assignment operator shifts the bits of the variable on the left to left by the number of positions specified on the right and assigns the result to the variable on the left.
10. Right Shift Assignment Operator (>>=)
The right shift assignment operator shifts the bits of the variable on the left to the right by a number of positions specified on the right and assigns the result to the variable on the left.
Also, it is important to note that all of the above operators can be overloaded for custom operations with user-defined data types to perform the operations we want.
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- Geeks Premier League
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Move Constructors and Move Assignment Operators (C++)
- 9 contributors
This topic describes how to write a move constructor and a move assignment operator for a C++ class. A move constructor enables the resources owned by an rvalue object to be moved into an lvalue without copying. For more information about move semantics, see Rvalue Reference Declarator: && .
This topic builds upon the following C++ class, MemoryBlock , which manages a memory buffer.
The following procedures describe how to write a move constructor and a move assignment operator for the example C++ class.
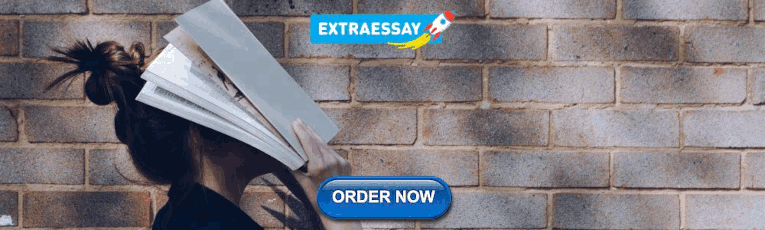
To create a move constructor for a C++ class
Define an empty constructor method that takes an rvalue reference to the class type as its parameter, as demonstrated in the following example:
In the move constructor, assign the class data members from the source object to the object that is being constructed:
Assign the data members of the source object to default values. This prevents the destructor from freeing resources (such as memory) multiple times:
To create a move assignment operator for a C++ class
Define an empty assignment operator that takes an rvalue reference to the class type as its parameter and returns a reference to the class type, as demonstrated in the following example:
In the move assignment operator, add a conditional statement that performs no operation if you try to assign the object to itself.
In the conditional statement, free any resources (such as memory) from the object that is being assigned to.
The following example frees the _data member from the object that is being assigned to:
Follow steps 2 and 3 in the first procedure to transfer the data members from the source object to the object that is being constructed:
Return a reference to the current object, as shown in the following example:
Example: Complete move constructor and assignment operator
The following example shows the complete move constructor and move assignment operator for the MemoryBlock class:
Example Use move semantics to improve performance
The following example shows how move semantics can improve the performance of your applications. The example adds two elements to a vector object and then inserts a new element between the two existing elements. The vector class uses move semantics to perform the insertion operation efficiently by moving the elements of the vector instead of copying them.
This example produces the following output:
Before Visual Studio 2010, this example produced the following output:
The version of this example that uses move semantics is more efficient than the version that does not use move semantics because it performs fewer copy, memory allocation, and memory deallocation operations.
Robust Programming
To prevent resource leaks, always free resources (such as memory, file handles, and sockets) in the move assignment operator.
To prevent the unrecoverable destruction of resources, properly handle self-assignment in the move assignment operator.
If you provide both a move constructor and a move assignment operator for your class, you can eliminate redundant code by writing the move constructor to call the move assignment operator. The following example shows a revised version of the move constructor that calls the move assignment operator:
The std::move function converts the lvalue other to an rvalue.
Rvalue Reference Declarator: && std::move
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources

Andrew Lock | .NET Escapades Andrew Lock
Thoughts about primary constructors: 3 pros and 5 cons.
In my previous post I provided an introduction to primary constructors in C#12. In this post I describe some of the ways I like to use primary constructor and also some of the things I don't like about them.
The main approaches to primary constructors
Basic field initialization, initialization in test code, dependency injection in mvc controllers, duplicate capture (minor), implicit fields can't be readonly, implicit fields change the struct layout, naming convention confusion, record confusion.
As I described in my previous post , there are two main ways to use primary constructors:
- Using the parameters to initialize fields and properties.
- Using the parameters directly in members, implicitly capturing the parameters as fields.
In the first case, you use the constructor parameters in initialization code for fields and properties:
and the compiler synthesizes a constructor that does the assignment for you:
The other approach is to reference the parameters directly in members:
In this case, the compiler also adds mutable fields to the class to capture the variables:
I go into more detail in my previous post , so I'll leave it there for now. For the remainder of the post I describe what I consider to be the sweet spot for primary constructors, a well as some things to watch out for and that I don't like!
The best use cases for primary constructors
In this section I provide an opinionated list of cases where I think primary constructors are a good fit. I don't think there's anything revolutionary or controversial in this section, but you never know!
One of the primary use cases for primary constructors is initialization of fields and properties, and this is where it really shines in my opinion. Primary constructors strictly reduce the amount of code you have to write, and instead the compiler generates that code for you. What's not to like!?
The example I showed at the start of this post, in which we're simply assigning to a couple of fields, is a prime example of a good use case:
Where things start to get more complex is if you want to add validation. Adding a throw expression for null isn't too bad:
But we've already partially obscured the simple fields. Once we start needing to use ternaries, things get very borderline in my opinion:
Whether you choose the firstName or lastName formatting shown above (they're equivalent), it's all a bit much in my opinion. You also can't use the handy guard methods introduced in .NET 7+ when initializing fields like this, unless you call out to a separate function. All of that feels like bending over backwards to use primary constructors, where a standard constructor would actually be less code and easier to read:
Obviously you could create your own helpers to work around these limitations and reduce the verbosity while sticking to primary constructors. Or, you could just use standard constructors in those cases instead of reinventing the wheel.
So, to reiterate, I think primary constructors are great when you just need to do simple initialization of fields or properties. But if you need validation of the parameters, I have a very low threshold for converting to a standard constructor.
One prime example of code that doesn't need to do any validation of the constructor parameters is your test classes. Take this very simple example of an xunit test for the Person type:
Ignore the question of whether you think this test adds value, or if really need to use the ITestOutputHelper in the method. The point of interest here is that adding ITestOutputHelper as a primary constructor parameter simplifies the test class in general. Compare it to the version without a primary constructor:
This practically doubles the length of the test code, without adding any extra value. It's completely irrelevant to the test whether or not ITestOutputHelper is obviously stored in a field and it doesn't matter whether it's mutable or not. Using primary constructors here is unequivocally a net win here.
If you're using ASP.NET Core, you're presumably also using dependency injection. Most of the time, you're probably not doing much or any validation of the dependencies that are injected into your classes. As per my original suggestion, that makes them a good candidate for primary constructors. For example:
I think it's safe to say a "regular" constructor doesn't add anything over a primary constructor in this case; it would simply be extra duplication. In fact, this is a case where you don't gain much from initializing fields over using the values directly:
This obviously looks quite a lot nicer, but just be aware that you're now creating the dependencies as mutable fields behind-the-scenes. That means you can assign to them, even though you probably don't ever want to do that.
If your class controller class really looks this simple, you could also just inject the dependencies directly into the Get() method instead of using a constructor.
That said, MVC controllers are probably a good candidate for this style of primary constructor: they likely won't have any validation requirements for the injected dependencies; they shouldn't have any complex logic in them which would mean we want to make sure the fields are readonly ; and you could even name the parameters using "field" conventions to make the source more apparent (more on that later).
In fact, you'll probably find that a lot of day-to-day code you write in your application could use primary constructors without any significant impact other than reducing the lines of code.
Nevertheless there are things to watch out for, over-and-above the "overly complex initializer" that I've already flagged. In many ways I'm actually not a fan of some aspects of primary constructors, particularly when they're used with implicit capture. In the next section I describe some of the things I don't like.
Gotchas, or, "things I don't like"
Before I start, I'll just point out that none of these complaints are deal-breakers, or mean that you shouldn't necessarily use them. They're just things that I don't like about some usages of primary constructors, or issues I've run into.
Also bear in mind my current perspective as a library-author (the Datadog .NET client library); I may well be concerned about different things to you! For what it's worth, while writing this post, I found a post on NDepend's blog from a year ago, and they highlighted many of the same gripes!
This complaint is a relatively obvious and minor one: if you initialize a field with a primary constructor parameter and you implicitly capture the value in a member, then you'll have two fields storing the same value:
This issue is obvious enough that the compiler automatically adds warnings if you do this by accident:

Given there's warnings about this case, this generally isn't something you'll need to worry about too much normally, but it's definitely something to be aware of (i.e. don't ignore the warnings!), and makes sense when you think about how the capture is implemented.
I showed previously that if you implicitly capture a primary constructor parameter, the compiler generates a field to store it:
An important feature of those fields is that they're mutable , i.e. they aren't marked readonly . That means you're free to set these fields outside of the constructor body, as I showed in my previous post .
If that's what you need then no problem. But if a field shouldn't be changed, I would normally religiously mark it as readonly . Marking the field as readonly makes it easier to reason about the intent of the field and it removes a whole class of bugs related to accidental modification of the field.
This again is a relatively minor point, and it's also something that I wouldn't be surprised to see addressed in a future version of C#, but right now, it irks me a bit.
The "answer" here is to not use implicit capture if you want readonly fields. Instead, create the fields manually, mark them readonly , and use the primary constructor to initialize the fields instead.
This is a relatively niche issue, but if you're working with struct s, high-performance code, and/or native interop, then it's sometimes important to know exactly how big a type is, and how its contents are laid out. That involves knowing which fields exist, as well as the order they're declared.
If I have a struct like this:
Then the size of the struct is the sum of the size of the fields. How many fields are there? Based on what we've seen already, there's 3:
- string <firstName>P
- string <lastName>P
Which gives a size of 8 × 3 = 24 bytes. Ok, all well and good. But what if in a later refactoring we realise we don't want to split firstName and lastName, and instead just use name :
Suddenly we've gone from 3 fields to 2, but without obviously changing the fields (unless you know how primary constructors are implemented!) This could have very bad consequences if we're relying on the size of Person anywhere!
Similarly, if we care about the order of the fields, we're a bit stuck. How are the implicit fields created relative to the "real" fields? We can check the decompiled code, but fundamentally we're at the whim of the compiler here, and the details could change in the future.
This is obviously a niche use case for most people and the simple answer is just don't use primary constructors in this situation. Or, again, use primary constructors to initialize existing fields, and don't rely on the implicit capture. Are you noticing a pattern here 😉
The next point is again a minor one, but it's one that you inevitably have to face early on: what conventions should you use for the primary constructor parameters?
Whether or not this is a problem for you may depend on the naming conventions you use. In this post I'm assuming you're using the default Visual Studio-style conventions used by the .NET runtime team . In these conventions: classes, properties, and methods use PascalCase; parameters use camelCase: and private instance fields use camelCase with an _ prefix.
Now, lets consider an example you've seen previously:
In this example, the primary constructor parameters are acting much more like fields than like parameters, so does it really make sense to use camalCase for the parameters here? 🤔 Maybe the following would make more sense:
Internal to the type, this feels much better. You get better glanceability that the variables being set in SetName are effectively fields.
However, now the constructor parameter names themselves are a bit weird…
This isn't really an issue if you're never going to directly create an instance of the type, because it's an MVC controller or an xunit test class for example. These are created by the framework directly, so you never have the weird code above. But it would also seems strange to have different conventions for these special cases.
The problem obviously goes away if you only use primary constructors for initialization, as now they really are only parameters and there aren't any implicit fields:
Now, even though I said I'm focusing on the current .NET runtime conventions, I'll point out that using camelCase for private fields and referencing them with this. doesn't solve the problem for you: your code won't even compile if you try to use this. :

For what it's worth, Microsoft suggests using the "parameter" naming convention. This is probably because they say :
It's important to view primary constructor parameters as parameters
Which I kind of disagree with. I think it's important to understand how they're implemented (as fields if you use implicit capture), but to each their own.
OK, this final one is definitely overly pedantic, but it caught me the other day and made me grumble. I was working on some code that was using a record initially, something a bit like this:
At some point, I realised I didn't want the structural equality record brings for Person , so I changed the Person definition to be a class instead of record :
And then, my code stopped compiling.

And it took me far too long to realise that despite the essentially identical syntax, it was because record converts the primary constructor parameters into properties, whereas for class it doesn't. And I obviously know that, but when syntax that is identical has fundamentaly different behaviour in slightly different circumstances, and you're in the flow, sometimes it catches you out. Grumble grumble.
You probably noticed a recurrent theme throughout my griping: using primary constructors for initialization is fine; all the sharp edges are around implicit capture. This made me wonder: is there a way to use only the initialization features of primary constructors. And it turns out, yes! In the next post I'll show how you can use a Roslyn analyzer to enforce that behaviour!
In my previous post I provided an introduction to primary constructors and how they work behind-the-scenes. In this post I expanded on that post to describe some of the cases I think primary constructors work well for. I then discussed some of the various gripes I have with primary constructors, mostly stemming from the implicit capture usage. In the next post I'll show an analyzer you can use to ensure primary constructors are only used for initialization.
Popular Tags
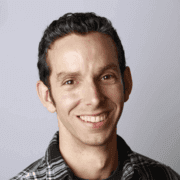
Stay up to the date with the latest posts!
Building new functionality, writing unit tests, and learning new technologies has never been easier or more fun.
Mastering Slash Commands with GitHub Copilot in Visual Studio

Cynthia Zanoni

Laurent Bugnion
May 14th, 2024 1
GitHub Copilot, the AI-powered coding assistant, revolutionizes coding in Visual Studio with its advanced features. In this series, we delve into the efficiency and convenience offered by Slash Commands, elevating your coding workflow.
Introducing Slash Commands
Slash Commands are predefined actions within GitHub Copilot, accessible through the prompt interface. Bruno Capuano, in his latest video, elucidates these commands, accessible via the Slash button in the Copilot Chat window or by typing a forward slash in the message box.
Alternatively, you can also access the Slash Commands by typing a forward slash in the message box.
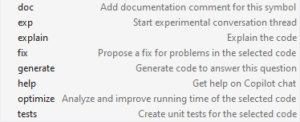
Key commands include:
- doc : Insert a documentation comment in the current cursor position.
- exp : Start a new conversation thread with a fresh context.
- explain : Provide an explanation for the selected code.
- fix : Suggest fixes for code errors and typos.
- generate : Generate new code snippets based on your input.
- optimize : Recommend code optimizations to improve performance.
- tests : Create a unit test for the current code selection.
- help : Access help and support for GitHub Copilot.
A Practical Example with /fix
Demonstrating the power of Slash Commands, the /fix command automatically suggests corrections for typos and errors, enhancing code quality and efficiency.
In the video, Bruno demonstrates how GitHub Copilot can automatically suggest corrections for typos and other issues. This command can be used in the main chat window, and it’s also accessible in the inline chat by pressing Alt-Slash (Alt-/) or through the right-click context menu.
As you can see, these Slash Commands can significantly improve your productivity in Visual Studio. Learn how to Install GitHub Copilot in Visual Studio .
Additional Resources
To learn more about GitHub Copilot and Slash Commands, check out our resource collection here. You can watch the full video here . For ongoing updates, stay tuned to this blog and consider subscribing to our YouTube channel for more insights and tutorials.
- Code Faster and Better with GitHub Copilot’s New Features: Slash Commands and Context Variables
- Announcing the GitHub Extension for Visual Studio

Cynthia Zanoni Cloud Advocate, Developer Relations

Laurent Bugnion Principal Cloud Advocate, Developer Relations
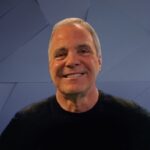
- Share full article
Advertisement
Supported by
wordplay, the crossword column
This Is the Life!
Wind your way through Adam Wagner’s puzzle.

By Deb Amlen
Jump to: Today’s Theme | Tricky Clues
THURSDAY PUZZLE — When does a crossword puzzle need a visual hint at the theme? We’ve seen circled squares, shaded squares, bolded lines and even black square s used as aids to help solvers organize their thoughts. These elements are usually added to the grids during the editing process.
This devilishly clever puzzle from Adam Wagner includes shaded squares, and thank goodness they’re there. Not having some sort of visual element might have led to cross words (sorry).
Adding a visual element is only one of many jobs of the puzzle editors.
“We usually talk about visual elements like this during the meetings when we’re accepting the puzzle,” Christina Iverson, a puzzle editor, said. “In this case, we just thought the visual would be too hard to see without any shading, and if testers find something confusing, we’ll change the presentation,” she added.
Joel Fagliano, the digital puzzle editor, agreed with Ms. Iverson and said that other ideas for clarifying the theme were discussed. But, he added: “Ultimately, we settled on what you see now. Even if it makes the puzzle a little easier for a Thursday, it seems worth it to make the theme clear to all solvers.”
Today’s Theme
First, allow me to give kudos to Mr. Wagner for filling this grid. Making a puzzle where the true answers are basically hidden but what we write in the squares are unrelated words or phrases is next-level constructing as far as I’m concerned. You’ll see what I mean.
In this grid, we’re making BOX BRAIDS as a nod to the “hairstyle worn by Janet Jackson in the 1993 film ‘ Poetic Justice .’” Solving it may make you feel as if you have a lot on your plait. (Sorry, I couldn’t resist.)
BOX BRAIDS — the revealer at 32A — involve separating sections of hair into a sort of grid pattern and braiding each section. Sometimes synthetic hair is braided in to help lengthen and smooth out the braids. The square sections, or boxes, give this hairstyle its name.
In each quadrant of Mr. Wagner’s puzzle, a series of seven shaded squares winds its way down, but what could that mean?
It turns out that these are braids that exist in the puzzle’s boxes. Note that they are two-strand braids, not three. I’m not even sure filling a puzzle with three interwoven entries is possible, at least not with good fill.
We need to solve the clues in two columns — 2D and 3D, for example — in order to make sense of the entries.
For example, at 2D, the answer to the clue “Academic achievements” seems to be DOG BEDS, which makes no sense. Similarly, the answer to “Doesn’t allow,” at 3D, seems to be FERRIES. Now what do we do?
We braid the entries, of course. If we follow the shaded squares in that sector starting at 2D, the answer becomes DEGREES. And if we follow the white squares that are interwoven with 2D, the answer becomes FORBIDS.
Tricky Clues
7A. You can say a lot of things about William TAFT , the 27th president, but you can’t say that he didn’t have a sense of humor. He was the person “who said of himself, in 1912, ‘No one candidate was ever elected ex-president by such a large majority.’”
18A. The king in the clue “What a king might sit on?” is a mattress, and the answer is SLATS.
20A. MR. T, who was born Laurence Tureaud, is the “’80s celeb who aptly appeared on Letterman?”
29A. The “One hawing but not hemming?” is an ASS, which makes a “hee haw” sound.
41A. A clue such as the blissful comment “‘This is the life!’” capitalizes on the common phrase in order to misdirect the solver. The clue is really hinting at a description of life, as in flora and fauna. The answer is BIOTA.
19D. “Light entertainment,” or plays, TV shows and movies that don’t require a lot of mental effort, is a Britishism that is sometimes used in the States as well. In Mr. Wagner’s puzzle, however, we are supposed to take the word “light” literally: The answer is LASER SHOW.
24D. We’ve seen this type of heteronym clue before: The word “row” can mean a line or it can mean a quarrel. In this puzzle it’s the latter, and “Two in a row?” are FOES.
40D. The “Platform whose exploitation is called ‘jailbreaking,’ for short” is IOS. In the past, if you were the owner of an Apple device and wanted to download an app that was not compatible with the closed operating system, you had to jailbreak it. This meant that you had to install an app that allowed users to customize their system beyond the strict Apple settings. The downside to jailbreaking was platform instability and horrible battery life. With the proliferation of apps that work on multiple platforms, jailbreaking may not be as widespread as it used to be.
53D. FIT is a “Fashionable look, in lingo.” According to Dictionary.com, a “ FIT check ” is a request for comment on said FIT.
Constructor Notes
Look, I know I’ve gone on record about preferring themes that feel “human.” But you have to understand — that was before I knew how to code! Now that I’ve learned Python’s deep magic, I’m happy to be a hypocrite. And for those curious, here are a few other good theme pairs that didn’t make the cut: CORN OIL HARPS ON CAR POOL HORNS IN LA SPARK POUTERS LOST ARK PAUPERS NECTARS TOELESS NO CLASS TEETERS MONROES BETTERS MENTORS BO TREES Turns out there really aren’t any viable pairs longer than seven letters, which ended up being a happy bit of serendipity in terms of being able to pull this off in a 15x15 grid. Hope you enjoyed!
Join Our Other Game Discussions
Want to be part of the conversation about New York Times Games, or maybe get some help with a particularly thorny puzzle? Here are the:
Spelling Bee Forum
Wordle Review
Connections Companion
Improve Your Crossword Solving
Work your way through our guide, “ How to Solve the New York Times Crossword .” It contains an explanation of most of the types of clues you will see in the puzzles and a practice Mini at the end of each section.
Want to Submit Crosswords to The New York Times?
The New York Times Crossword has an open submission system, and you can submit your puzzles online . For tips on how to get started, read our series “ How to Make a Crossword Puzzle .”
The Tipping Point
Almost finished solving but need a bit more help? We’ve got you covered.
Spoiler alert: Subscribers can take a peek at the answer key .
Trying to get back to the main Gameplay page? You can find it here .
Deb Amlen is a games columnist for The Times. She helps readers learn to solve the Times Crossword, and writes about games, puzzles and language. More about Deb Amlen
It’s Game Time!
Take your puzzling skills in new directions..
WordleBot , our daily Wordle companion that tells you how skillful or lucky you are, is getting an upgrade. Here’s what to know .
The editor of Connections , our new game about finding common threads between words, talks about how she makes this daily puzzle feel fun .
We asked some of the best Sudoku solvers in the world for their tips and tricks. Try them to tackle even the most challenging puzzles.
Read today’s Wordle Review , and get insights on the game from our columnists.
We asked Times readers how they play Spelling Bee. The hive mind weighed in with their favorite tips and tricks .
Ready to play? Try Wordle , Spelling Bee or The Crossword .
Off the Grid: Sally breaks down USA TODAY's daily crossword puzzle, The Strokes

There are spoilers ahead. You might want to solve today's puzzle before reading further! The Strokes
Constructor: Jeanne D. Breen
Editor: Amanda Rafkin
Comments from Today’s Crossword Constructor
Jeanne: After many years as a regular solver, I started constructing crosswords last spring. An earlier version of this puzzle – with THE STROKES [clued to the band] as the revealer, rather than the title – was one of the first ones I created. The theme, swimming, is one of my passions. I started swimming laps in medical school and have been doing so ever since (though I won’t say how many years that entails 😊). I currently swim about 15,000 yards (~8.5 miles) per week at my local YMCA. With warmer weather approaching, I’ll enjoy some open water swims in Long Island Sound. Both the physical and mental health benefits are considerable!
Random Thoughts & Interesting Things
- BACK TO THE FUTURE (17A: Franchise with a DeLorean) The DeLorean, manufactured from 1981-1982, was the only car produced by the DeLorean Motor Company. The car had stainless-steel outer body panels and gull-wing doors. Although it had a short run, the DeLorean achieved fame for being the car retrofitted as a time machine in the BACK TO THE FUTURE franchise. The first BACK TO THE FUTURE movie was released in 1985. This was followed by BACK TO THE FUTURE Part II in 1989, and BACK TO THE FUTURE Part III in 1990. I'm quite excited about the fact that BACK TO THE FUTURE: The Musical premiered on Broadway last year. My husband and I have tickets to see a touring production of the musical this fall.
- EEL (22A: Unagi, on a sushi menu) Unagi is the Japanese word for freshwater EEL.
- CREEK (27A: People also known as the Muscogee) The Muscogee are a group of indigenous peoples whose historic homelands are in the southeastern United States. The Muskogee are also known as the Mvskoke, Muscogee CREEK, or just CREEK. Former U.S. Poet Laureate Joy Harjo is a member of the Muscogee CREEK Nation.
- GAMMA (35A: Third Greek letter) Whenever the Greek alphabet is referenced in the puzzle, I try to take that opportunity to review my knowledge of its 24 letters. The Greek alphabet begins with alpha, beta, GAMMA, delta, epsilon, zeta, and eta.
- OPERA (42A: Performance at La Scala) La Scala is an OPERA house in Milan, Italy. It first opened in 1778.
- RBG (45A: SCOTUS justice who wore dissent collars) Ruth Bader Ginsburg (1933-2020), known as RBG, was a member of the Supreme Court of the United States (SCOTUS) from 1993-2020. During her time on the Supreme Court, RBG wore a number of different collars, an accessory she added to the robe, which was designed for men. She had a particular collar she wore on days she dissented. After Ruth Bader Ginsburg's death, Time magazine was granted access to photograph some of her favorite collars , including her dissent collar.
- ATLAS (48A: He had the weight of the world on his shoulders) In Greek mythology, ATLAS is a Titan (a pre-Olympian God). Following a 10-year war between the Titans and the Olympians, which the Olympians ultimately won, ATLAS was condemned to stand at the western edge of the earth and hold up the sky.
- ALLAN (51A: "Barbie" character with a colorful striped shirt) Part of Mattel's Barbie brand, the ALLAN doll was introduced in 1964 as Ken's friend. (Side note: In 1991, the spelling of the doll's name was changed to Alan.) In the 2023 movie Barbie, the character of ALLAN, who wore a colorful striped shirt, was played by Michael Cera.
- BUTTERFLY EFFECT (61A: Concept that trivial events might have big consequences) The BUTTERFLY EFFECT concept originated in weather science. Mathematician and meteorologist Edward Norton Lorenz was studying weather models of tornados, and noticed that a very small change in initial conditions could result in a significantly different outcome. The idea of the BUTTERFLY EFFECT has been adapted to contexts beyond weather science.
- SOUR (70A: One of the five basic tastes) The five basic tastes detected by taste buds are salty, sweet, bitter, SOUR, and umami (savory).
- CATNAP (5D: Refreshing bit of shut-eye) My cat, Willow, highly recommends CATNAPs.
- ODE (7D: "___ to the Bear Hug" (Clint Smith poem)) Clint Smith is a poet and a staff writer for The Atlantic . "ODE to the Bear Hug" is from his 2023 collection of poetry, Above Ground.
- LARA (29D: Fictional adventurer Croft) LARA Croft is the protagonist of the video game franchise Tomb Raider . Angelina Jolie played LARA Croft in the movies based on the video game, LARA Croft: Tomb Raider (2001) and LARA Croft: Tomb Raider - The Cradle of Life (2003).
- EMU (32D: Bird that lays green eggs) EMU eggs are a dark green color, and are an average of 5 inches by 3.5 inches, weighing over a pound.
- APPA (36D: Dad, in Korean) I learned the word APPA from the March 18, 2021 puzzle . More importantly, I remembered it when solving this puzzle!
- SELA (39D: "CSI: NY" actress Ward) CSI:NY , part of the CSI franchise, originally aired from 2004-2013. SELA Ward portrayed Detective First Grade Jo Danville.
- GLUTEN (47D: Celiac disease trigger) GLUTEN is a protein naturally present in some grains, such as wheat, barley, and rye. Celiac disease is an auto-immune condition that causes an intolerance to GLUTEN to develop.
- SAWYER (49D: Broadcast journalist Diane) Among Diane SAWYER's many achievements, in 1984 she became the first female correspondent for 60 Minutes . She has also been an anchor on ABC World News Tonight , Good Morning America , and 20/20 . Since 2014, she has been a special contributor for ABC News.
- ERAS (57D: "The ___ Tour (Taylor's Version)") The ERAS Tour (Taylor's Version) is an extended cut version of Taylor Swift's concert movie – Taylor Swift | The ERAS Tour – that is available on Disney+.
- FLO (62D: Progressive's saleswoman) Since 2008, ads for Progressive insurance have featured Stephanie Courtney playing the role of a saleswoman named Flo.
- LOU (63D: Mary ___ Retton) Mary LOU Retton was the first American woman to win the Olympics all-around gold medal for gymnastics. That was at the 1984 Summer Olympics. She was inducted into the International Gymnastics Hall of Fame in 1997. More recently, in 2018 Mary LOU Retton was a contestant on the 27th season of Dancing with the Stars .
- SEND HELP (40D: "We're in a pickle!")
- CAR (64D: A Mustang is one, but a Clydesdale isn't)
Crossword Puzzle Theme Synopsis
- BACK TO THE FUTURE (17A: Franchise with a DeLorean)
- BREAST PUMPS (37A: Lactation devices)
- BUTTERFLY EFFECT (61A: Concept that trivial events might have big consequences)
The first word of each theme answer is a swimming STROKE: BACKSTROKE, BREASTSTROKE, and BUTTERFLY STROKE.
THE STROKES, who Jeanne mentioned in her constructor notes, are an indie rock band. Their 2020 album, The New Abnormal , won a Grammy Award for Best Rock Album. Today, the band's name makes a perfect title for this theme highlighting swimming STROKES. Congratulations to Jeanne D. Breen making a USA TODAY debut! Thank you, Jeanne, for this enjoyable puzzle.
For more on USA TODAY’s Crossword Puzzles
- USA TODAY’s Daily Crossword Puzzles
- Sudoku & Crossword Puzzle Answers
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Have a question about this project? Sign up for a free GitHub account to open an issue and contact its maintainers and the community.
By clicking “Sign up for GitHub”, you agree to our terms of service and privacy statement . We’ll occasionally send you account related emails.
Already on GitHub? Sign in to your account
[Proposal]: Auto-default structs #5737
RikkiGibson commented Feb 9, 2022 • edited by jcouv
- 👍 10 reactions
- 👎 1 reaction
- 🎉 2 reactions
- ❤️ 2 reactions
TahirAhmadov commented Feb 14, 2022
Sorry, something went wrong.
RikkiGibson commented Feb 14, 2022
Rikkigibson commented feb 18, 2022.
This comment was marked as off-topic.
RikkiGibson commented Mar 17, 2022
- 👍 3 reactions
KathleenDollard commented Mar 17, 2022
Rikkigibson commented apr 4, 2022.
- 🎉 3 reactions
HopefulFrog commented Apr 13, 2022
Youssef1313 commented Apr 13, 2022 • edited
- 👍 2 reactions
- 😕 1 reaction
RikkiGibson commented Apr 14, 2022
Hopefulfrog commented apr 14, 2022.
paulozemek commented Apr 21, 2022
PathogenDavid commented Apr 21, 2022
IS4Code commented Mar 3, 2023
jamesford42 commented Jan 26, 2024
- 👍 1 reaction
No branches or pull requests
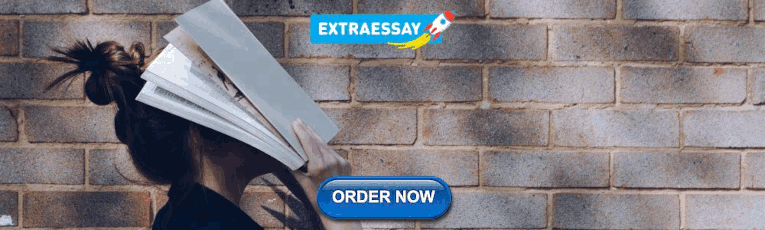
IMAGES
VIDEO
COMMENTS
Copy constructor is called when a new object is created from an existing object, as a copy of the existing object. And assignment operator is called when an already initialized object is assigned a new value from another existing object. Example-. t2 = t1; // calls assignment operator, same as "t2.operator=(t1);"
But, there are some basic differences between them: Copy constructor. Assignment operator. It is called when a new object is created from an existing object, as a copy of the existing object. This operator is called when an already initialized object is assigned a new value from another existing object. It creates a separate memory block for ...
The copy constructor is for creating a new object. It copies an existing object to a newly constructed object.The copy constructor is used to initialize a new instance from an old instance. It is not necessarily called when passing variables by value into functions or as return values out of functions. The assignment operator is to deal with an ...
Copy constructors, assignment operators, and exception safe assignment. Score: 4.3/5 (3169 votes) What is a copy constructor? A copy constructor is a special constructor for a class/struct that is used to make a copy of an existing instance. According to the C++
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
Copy Assignment Not really a constructor Assign an instance of a type to be a copy of another instance. Copy Constructors A copy constructor is called when an instance of a type is constructed from another instance Two ways it can be called. Copy Constructors
If a class doesn't define a move constructor, the compiler generates an implicit one if there's no user-declared copy constructor, copy assignment operator, move assignment operator, or destructor. If no explicit or implicit move constructor is defined, operations that would otherwise use a move constructor use the copy constructor instead. ...
A copy constructor is a constructor which can be called with an argument of the same class type and copies the content of the argument without mutating the argument. Contents. ... of the implicitly-defined copy constructor is deprecated if T has a user-defined destructor or user-defined copy assignment operator. (since C++11)
The difference between the copy constructor and the copy assignment operator causes a lot of confusion for new programmers, but it's really not all that difficult. Summarizing: If a new object has to be created before the copying can occur, the copy constructor is used (note: this includes passing or returning objects by value). ...
Constructor in C++ is a special method that is invoked automatically at the time of object creation. It is used to initialize the data members of new objects generally. ... Move Constructors and Move Assignment Operators. Prerequisites: lvalue referencervalue referenceCopy Semantics (Copy Constructor) References: In C++ there are two types of ...
A constructor is a special member function that is called automatically when an object is created. In this tutorial, we will learn about the C++ constructors with the help of examples. ... If we don't define any copy constructor, move constructor, or move assignment in our class, then the C++ compiler will automatically create a default copy ...
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
C++11 defines two new functions in service of move semantics: a move constructor, and a move assignment operator. Whereas the goal of the copy constructor and copy assignment is to make a copy of one object to another, the goal of the move constructor and move assignment is to move ownership of the resources from one object to another (which is typically much less expensive than making a copy).
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
This topic describes how to write a move constructor and a move assignment operator for a C++ class. A move constructor enables the resources owned by an rvalue object to be moved into an lvalue without copying. For more information about move semantics, see Rvalue Reference Declarator: &&. This topic builds upon the following C++ class ...
In the first case, you use the constructor parameters in initialization code for fields and properties: public class Person (string firstName, string lastName) {private readonly string _firstName = firstName; // 👈 initialized private readonly string _lastName = lastName;} and the compiler synthesizes a constructor that does the assignment ...
Normally copy constructors chain so that they are copy constructed from the base up. Here because you are calling the assignment operator the copy constructor must call the default constructor to default initialize the object from the bottom up first. Then you go down again using the assignment operator. This seems rather inefficient.
As you can see, these Slash Commands can significantly improve your productivity in Visual Studio. Learn how to Install GitHub Copilot in Visual Studio.. Additional Resources. To learn more about GitHub Copilot and Slash Commands, check out our resource collection here. You can watch the full video here.For ongoing updates, stay tuned to this blog and consider subscribing to our YouTube ...
The design statement approach will also help the institution adjust to the ever-changing capabilities of AI tools. The most challenging, and arguably most important, next step goes beyond logistics and new practices and focuses on shifting from a product-oriented assignment paradigm to a process-oriented one.
A clue such as the blissful comment "'This is the life!'" capitalizes on the common phrase in order to misdirect the solver. The clue is really hinting at a description of life, as in ...
Comments from Today's Crossword Constructor. Jeanne: After many years as a regular solver, I started constructing crosswords last spring. An earlier version of this puzzle - with THE STROKES ...
6. Both the constructor and assignment operator are called. You can check this by printing something in operator =. This happens because operator = is defined to take a const Holder &, but b is of type A *. So first the Holder(T *) constructor is called to create a temporary object, then this object is assigned to holder through operator =.
Now, with this proposal implemented, the compiler will add F = 0 in the constructor for you (unless you already have F = something in the constructor in an always-reachable code path). I'm not sure I understand your example. Are you saying that with structs, a new call somehow reuses the variable on the left side of the assignment? My assumption would have been that the second call of new S ...
34. Oh my. Initialization and assignment. Well, that's confusion for sure! To initialize is to make ready for use. And when we're talking about a variable, that means giving the variable a first, useful value. And one way to do that is by using an assignment. So it's pretty subtle: assignment is one way to do initialization.
Copy Constructor (If no copy constructor is defined) Calls the base class copy constructor passing the src object. Then calls the copy constructor of each member using the src objects members as the value to be copied. Assignment Operator. Calls the base class assignment operator passing the src object.
There's copy constructor and there's assignment operator. Since A != B, the copy assignment operator will be called. Short answer: operator = from class A, since you're assigning to class A. Long answer: A=B will not work, since A and B are class types. You probably mean: A a; B b; a = b; In which case, operator = for class A will be called ...