+123-456-7890
[email protected]
Mon - Fri: 7AM - 7PM
Conference on optimizing Ruby code execution
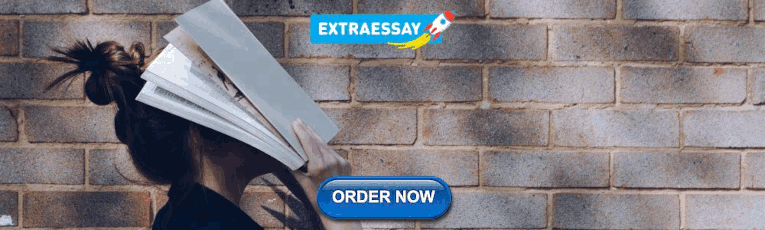
An In-depth Guide to Master the Ruby Case Statement
In Ruby, case statements serve as a robust substitute for a series of if/elsif conditions, streamlining the decision-making process within your code. This article elucidates various scenarios where case statements prove particularly beneficial and delves into the mechanics of their operation.
Structure of a Ruby Case Statement
A Ruby case statement is structured around several key components:
- case : Initiates the case statement, evaluating the variable in question;
- when : Represents individual conditions to be matched;
- else : Executes an alternative action if no conditions match, though this is optional.
Utilizing Case Statements with Ranges
Case statements exhibit remarkable flexibility, as demonstrated in scenarios requiring action based on a variable’s range. For instance, assessing a ‘capacity’ value to determine fuel status can be elegantly handled with a case statement:
Implementing Regex Conditions
Case statements also accommodate regular expressions for conditions, allowing for sophisticated pattern matching. For example, evaluating a ‘serial_code’ to gauge product risk levels:
When to Opt for Alternatives to Case Statements
For straightforward 1:1 mappings, such as assigning URLs based on a ‘country’ variable, utilizing a hash map might offer a more efficient solution than a case statement:
Insight into the === Method
The essence of case statement functionality in Ruby is the === method, which is invoked on the left-side object, enabling flexible and diverse condition checks, including range inclusion.
The Efficacy of Procs in Case Statements
The Proc class, implementing the === method, extends the versatility of case statements. This enables dynamic condition evaluations, such as distinguishing between even and odd numbers:
Comparative Table
Through exploring the capabilities and applications of Ruby case statements, it’s clear they offer a powerful tool for enhancing code readability and efficiency. Embracing case statements in your Ruby projects can simplify complex conditional logic, paving the way for clearer and more maintainable code.
Recommended Articles
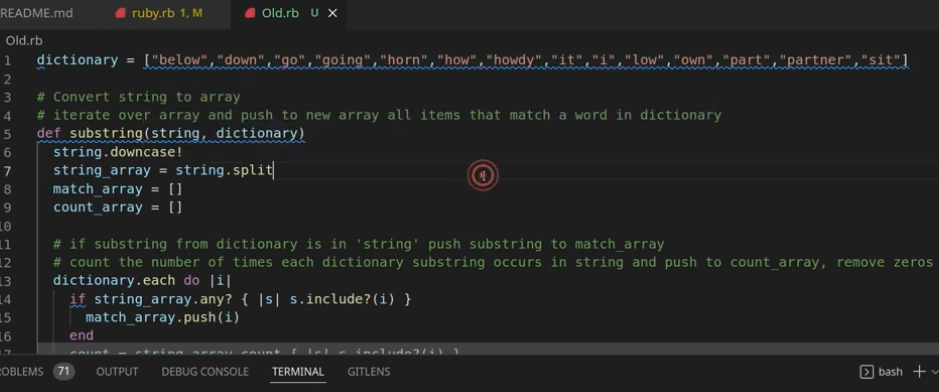
Ruby’s Substring Extraction: A Comprehensive Guide
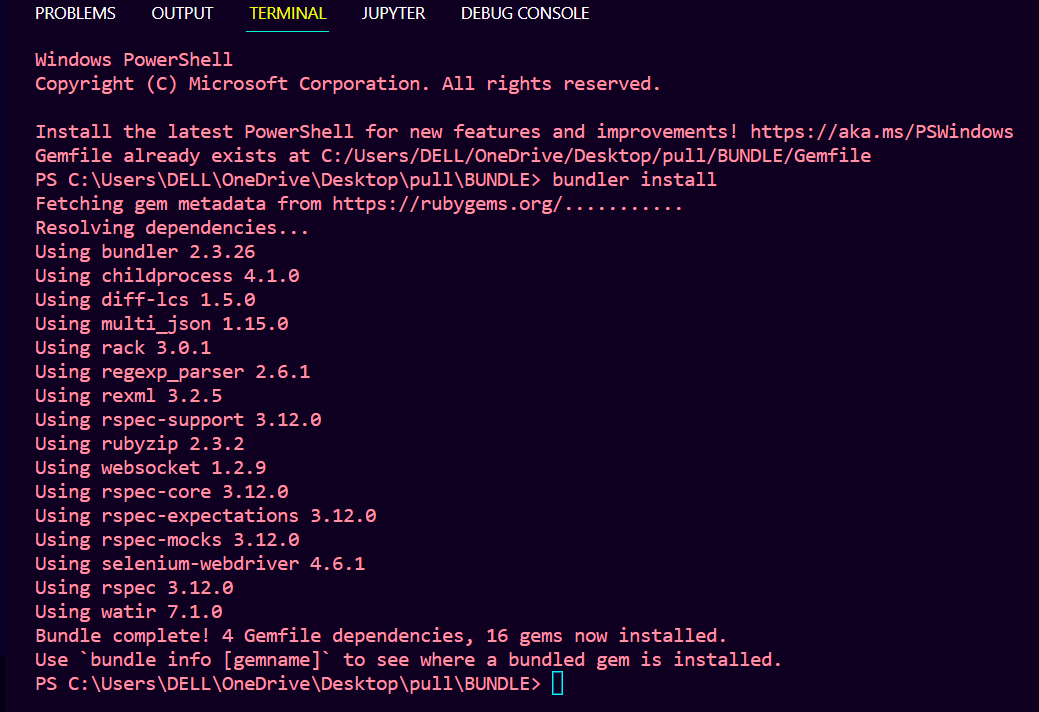
Ruby Gems & Bundler: Code Mastery
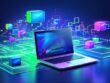
Ruby Sets: An Efficient Approach to Data Management
Leave a comment cancel reply.
You must be logged in to post a comment.
TheDeveloperBlog.com
Home | Contact Us
C-Sharp | Java | Python | Swift | GO | WPF | Ruby | Scala | F# | JavaScript | SQL | PHP | Angular | HTML
- Ruby Case Examples: Ranges, Strings and Regexp
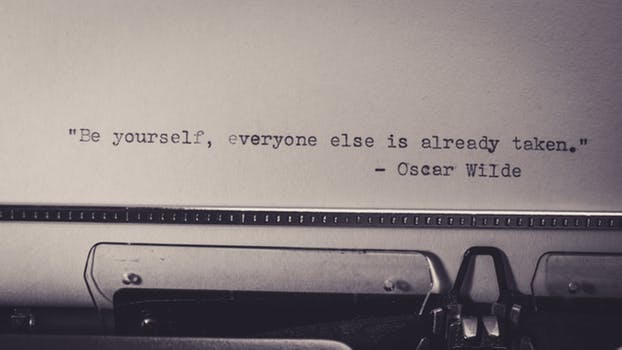
Related Links:
- Ruby Convert Types: Arrays and Strings
- Ruby File Handling: File and IO Classes
- Ruby Regexp Match Method
- Ruby String Array Examples
- Ruby include: Array Contains Method
- Ruby Class Examples: Self, Super and Module
- Ruby DateTime Examples: require date
- Ruby 2D Array Examples
- Ruby Number Examples: Integer, Float, zero and eql
- Ruby Exception Examples: Begin and Rescue
- Top 65 Ruby Interview Questions (2021)
- Ruby on Rails Interview Questions (2021)
- Ruby String Examples (each char, each line)
- Ruby if Examples: elsif, else and unless
- Ruby Math Examples: floor, ceil, round and truncate
- Ruby Sub, gsub: Replace String
- Ruby Substring Examples
- Ruby Console: Puts, Print and stdin
- Ruby Remove Duplicates From Array
- Ruby Random Number Generator: rand, srand
- Ruby Recursion Example
- Ruby ROT13 Method
- Ruby Iterator: times, step Loops
- Ruby String Length, For Loop Over Chars
- Ruby Join Example (Convert Array to String)
- Ruby Format String Examples
- Ruby Copy Array Example
- Ruby Keywords
- Ruby Nil Value Examples: NoMethodError
- Learn Ruby Tutorial
- Ruby Method: Def, Arguments and Return Values
- Ruby Fibonacci Sequence Example
- Ruby Hash Examples
- Ruby While, Until and For Loop Examples
- Learn Ruby on Rails Tutorial
- Ruby Word Count: Split Method
- Ruby Sort Arrays (Use Block Syntax)
- Ruby Array Examples
- Ruby Split String Examples
Related Links
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Ruby Programming Language
- Ruby For Beginners
- Ruby Programming Language (Introduction)
- Comparison of Java with other programming languages
- Similarities and Differences between Ruby and C language
- Similarities and Differences between Ruby and C++
- Environment Setup in Ruby
- How to install Ruby on Linux?
- How to install Ruby on Windows?
- Interesting facts about Ruby Programming Language
- Ruby | Keywords
- Ruby | Data Types
- Ruby Basic Syntax
- Hello World in Ruby
- Ruby | Types of Variables
- Global Variable in Ruby
- Comments in Ruby
- Ruby | Ranges
- Ruby Literals
- Ruby Directories
- Ruby | Operators
- Operator Precedence in Ruby
- Operator Overloading in Ruby
- Ruby | Pre-define Variables & Constants
- Ruby | unless Statement and unless Modifier
Control Statements
- Ruby | Decision Making (if, if-else, if-else-if, ternary) | Set - 1
- Ruby | Loops (for, while, do..while, until)
Ruby | Case Statement
- Ruby | Control Flow Alteration
- Ruby Break and Next Statement
- Ruby redo and retry Statement
- BEGIN and END Blocks In Ruby
- File Handling in Ruby
- Ruby | Methods
- Method Visibility in Ruby
- Recursion in Ruby
- Ruby Hook Methods
- Ruby | Range Class Methods
- The Initialize Method in Ruby
- Ruby | Method overriding
- Ruby Date and Time
OOP Concepts
- Object-Oriented Programming in Ruby | Set 1
- Object Oriented Programming in Ruby | Set-2
- Ruby | Class & Object
- Private Classes in Ruby
- Freezing Objects | Ruby
- Ruby | Inheritance
- Polymorphism in Ruby
- Ruby | Constructors
- Ruby | Access Control
- Ruby | Encapsulation
- Ruby Mixins
- Instance Variables in Ruby
- Data Abstraction in Ruby
- Ruby Static Members
- Ruby | Exceptions
- Ruby | Exception handling
- Catch and Throw Exception In Ruby
- Raising Exceptions in Ruby
- Ruby | Exception Handling in Threads | Set - 1
- Ruby | Exception Class and its Methods
- Ruby | Regular Expressions
- Ruby Search and Replace
Ruby Classes
- Ruby | Float Class
- Ruby | Integer Class
- Ruby | Symbol Class
- Ruby | Struct Class
- Ruby | Dir Class and its methods
- Ruby | MatchData Class
Ruby Module
- Ruby | Module
- Ruby | Comparable Module
- Ruby | Math Module
- Include v/s Extend in Ruby
Collections
- Ruby | Arrays
- Ruby | String Basics
- Ruby | String Interpolation
- Ruby | Hashes Basics
- Ruby | Hash Class
- Ruby | Blocks
Ruby Threading
- Ruby | Introduction to Multi-threading
- Ruby | Thread Class-Public Class Methods
- Ruby | Thread Life Cycle & Its States
Miscellaneous
- Ruby | Types of Iterators
- Ruby getters and setters Method
The case statement is a multiway branch statement just like a switch statement in other languages. It provides an easy way to forward execution to different parts of code based on the value of the expression.
There are 3 important keywords which are used in the case statement:
- case : It is similar to the switch keyword in another programming languages. It takes the variables that will be used by when keyword.
- when : It is similar to the case keyword in another programming languages. It is used to match a single condition. There can be multiple when statements into a single case statement.
- else : It is similar to the default keyword in another programming languages. It is optional and will execute when nothing matches.
Flow Chart:
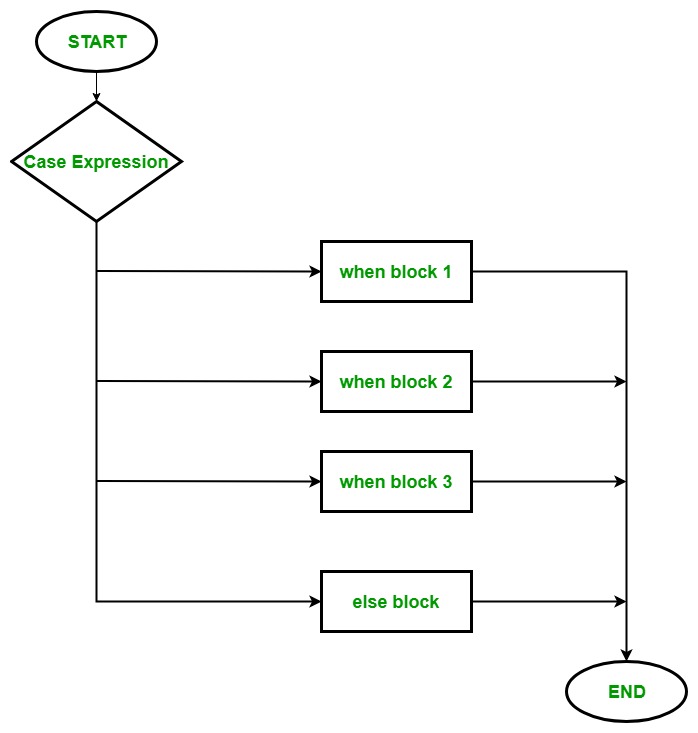
Important Points:
Please Login to comment...
Similar reads.
- Ruby-Basics
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- PyQt5 ebook
- Tkinter ebook
- SQLite Python
- wxPython ebook
- Windows API ebook
- Java Swing ebook
- Java games ebook
- MySQL Java ebook
Ruby variables
last modified October 18, 2023
In this part of the Ruby tutorial, we examine variables in more detail.
A variable is a place to store data. Each variable is given a unique name. There are some naming conventions which apply to variable names. Variables hold objects. More precisely, they refer to a specific object located in computer memory. Each object is of certain data type. There are built-in data types and there are custom-built data types.
Ruby belongs to the family of dynamic languages. Unlike strongly typed languages like Java, C or Pascal, dynamic languages do not declare a variable to be of certain data type. Instead of that, the interpreter determines the data type at the moment of the assignment. Variables in Ruby can contain different values and different types of values over time.
The term variable comes from the fact that variables, unlike constants, can take different values over time. In the example, there is a variable called i . First it is assigned a value 5, later a different value 7.
Ruby variable naming conventions
Ruby, like any other programming language, has some naming conventions for variable identifiers.
Ruby is a case sensitive language. It means that age and Age are two different variable names. Most languages are case sensitive. BASIC is an exception; it is a case insensitive language. While we can create different names by changing the case of the characters, this practice is not recommended.
The code example defines two variables: I and i . They hold different values.
Variable names in Ruby can be created from alphanumeric characters and the underscore _ character. A variable cannot begin with a number. This makes it easier for the interpreter to distinguish a literal number from a variable. Variable names cannot begin with a capital letter. If an identifier begins with a capital letter, it is considered to be a constant in Ruby.
In this script, we show a few valid variable names.
Variable names should be meaningful . It is a good programming practice to choose descriptive names for variables. The programs are more readable then.
The script shows three descriptive variable names. The place_of_birth is more descriptive to a programmer than e.g. pob. It is generally considered OK to choose simple variable names in loops.
Ruby sigils
Variable identifiers can start with special characters also called sigils . A sigil is a symbol attached to an identifier. Variable sigils in Ruby denote variable scope. This is in contrast to Perl, where sigils denote data type. The Ruby variable sigils are $ and @ .
We have four variables with different scopes. A scope is the range in which a variable can be referenced. We use special built-in methods to determine the scope of the variables.
A variable without a sigil is a local variable. A local variable is valid only locally: e.g., inside a method, block or a module.
Global variables start with $ character. They are valid everywhere. The use of global variables should be limited in programs.
A variable name starting with a @ sigil is an instance variable. This variable is valid inside an object.
Finally we have a class variable. This variable is valid for all instances of a specific class.
The local_variables gives an array of all local variables defined in a specific context. Our context is Ruby toplevel.
Similarly, the global_variables produces an array of globals. We do not print all globals to the terminal, because there are many of them. Each Ruby script starts with a bunch of predefined variables. Instead of that, we call the include? method of the array to check if our global is defined in the array. Also note that we are referencing variables with their symbols. (Symbols start with a colon character.)
The self pseudo variable points to the receiver of the instance_variables method. The receiver in our case is the main , the Ruby toplevel execution area.
Finally we have an array of class variables. The main is an instance of the Animal class.
We see symbolic names of the variables.
Ruby local variables
Local variables are variables that are valid within a local area of a Ruby source code. This area is also referred to as local scope . Local variables exist within the definition of a Ruby module, method, class.
We have a method called method1 , which has one variable. The variable is local. This means that it is valid only within the method definition. We can refer to the x variable only between the method name and the end keyword.
This is the definition of the method1 method. Inside the method, we create a local x variable. We print the value of the variable to the terminal.
The method is called.
We try to refer to a local variable outside the definition of the method. This leads to a NameError . The Ruby interpreter cannot find such identifier.
Running the example gives the above output.
The following example is a slight modification of a previous example.
We have two x variables. One is defined inside the method1 and the other one is defined outside. They are two distinct local variables. They do not clash with each other.
We have created a local x variable, which holds value 5. The variable is valid in the local scope of the main execution area. It is not valid inside the method1 .
Inside the definition of the method1 a new local variable x is defined. It has value 10. It exists in the body of the method1 method. After the end keyword it ceases to exist.
If a method takes parameters, a local variable is created for each of these parameters.
We have a method definition, which takes two values. The method returns the area of a rectangle.
The rectangle_area method takes two parameters. They are the sides of a rectangle, for which we calculate the area. Two local variables are automatically created for identifiers a and b. We call the local_variables method to see what local variables we have in the method.
Here we pass two values to the method rectangle_area . The values will be assigned to two local variables, created inside the method.
The output shows three things. The first two are the names of the local variables within the rectangle_area method. The third is the calculated area of the given rectangle.
A method may be defined inside another method. The inner methods have their own local variables.
In this Ruby script, we create three methods. The method2 and method3 are inner methods. The method2 is defined inside the method1 and the method3 is defined inside method2 . Each method's local variables are only accessible in the method in which they were defined.
From the output we can see that method1 has two local variables, m1 and m2 . The inner method2 has local variables m3 and m4 . The method3 , the innermost method, has local variables m5 and m6 .
The last example of this section will present several demonstrations of a local scope.
In the code example, we create local variables inside a module, method, class and toplevel. The local_variables is a method of the Kernel module that returns all current local variables.
A module is a collection of methods and constants. We create two local variables m1 and m2 .
Two local variables, v and w , are created in method1 .
The x and y local variables are created inside the definition of the Some class.
Finally, two local variables that belong to the Ruby toplevel's local scope are created.
The output shows local variables for each local scope.
Ruby global variables
Global variables are valid everywhere in the script. They start with a $ sigil in Ruby.
The use of global variables is discouraged. Global variables easily lead to many programming errors. Global variables should be used only when there is a reason to do so. Instead of global variables, programmers are advised to use local variables whenever possible.
In the example we have a global variable $gb . We show that the variable can be referenced in a module, method, class and a toplevel. The global variable $gb is valid in all these entities.
A global variable $gb is created; it has value 6.
Inside a module's definition we print the global variable's value.
Inside the definition of a method we print the value of the global variable.
Inside the definition of a class we print the value of the global variable.
Finally, in the toplevel execution area we print the global variable's value and whether the variable is in the array produced by the global_variables method.
The output of the example confirms that the global variable is accessible everywhere.
When a Ruby script starts, it has access to multiple predefined global variables. These globals are not considered harmful and help solve common programming jobs.
The script shows a $LOAD_PATH global variable. The variable lists directories which are searched by load and require methods. The $: is a short synonym for the $LOAD_PATH name.
More global variables will be presented in the Predefined variables section of this chapter.
Ruby instance, class variables
In this section we briefly cover instance and class variables. They will be described in Object-oriented programming chapter in more detail.
Instance variables are variables that belong to a particular object instance. Each object has its own object variables. Instance variables start with a @ sigil. Class variables belong to a specific class. All objects created from a particular class share class variables. Class variables start with @@ characters.
We create a custom Being class. The Being class has one class and one instance variable.
The @@is is an class variable. This variable is shared by all instances of the Being class. The logic of this example is that Being is and NotBeing is not.
The initialize method is a constructor. The method is called when the object is created. A @name instance variable is created. This variable is specific to a concrete object.
The to_s method is called, when the object is a parameter of a printing method, like p or puts . In our case, the method gives a short human readable description of the object.
The does_exist? method returns the class variable.
Three objects from the Being class are created. Each of the objects has a different name. The name of the object will be stored in the instance method, which is unique to each object instance. This will be used in the to_s method, which give a short description of the object.
The puts method takes the created objects as three parameters. It calls the to_s method on each of these objects.
Finally, we call the does_exist? method of each of the instances and print their return values. The output of these three methods is the same, because each method returns the class variable.
Output of the example. The first three messages are unique. The strings are stored in the instance variables of the objects. The true value is the value of the class variable, which is called three times.
Ruby environment & command-line variables
The ENV constant gives access to environment variables. It is a Ruby hash. Each environment variable is a key to the ENV hash.
The ARGV constant holds command-line argument values. They are passed by the programmer when the script is launched. The ARGV is an array that stores the arguments as strings. The $* is an alias to the ARGV .
Both ENV and ARGV are global constants.
In the script we loop through the ARGV array and print each of its values.
We have given three command-line arguments. They are printed to the console, each on a separate line.
The following example will deal with environment variables.
The script will print values of three environment variables to the terminal. The values depend on the OS settings of our operating system.
This is a sample output.
Ruby pseudo variables
Ruby has a few variables which are called pseudo variables . They are different from regular variables. We cannot assign values to pseudo variables.
The self is the receiver of the current method. The nil is the sole instance of the NilClass . It represents the absense of a value. The true is the sole instance of the TrueClass . It represents boolean true. The false is a sole instance of FalseClass . It represents boolean false.
The true and false are values of a boolean datatype. From another point of view, they are instances of specific classes. This is because everything in Ruby is an object. This looks like unnecessarily complicated. But it is the consequence of the aforementioned Ruby idiom.
This is an example of pseudo variables. We print all four pseudo variables with the p method. Then we find out the class name for all of them.
In this context, the self pseudo variable returns the main execution context.
Example output.
In the second example of this section, we further look at the self .
As we have said, the self references the receiver of the current method. The above example shows three examples of different receivers.
The receiver is the class called Some .
Here is another receiver: a class named Other .
And the third receiver is the Ruby toplevel.
The last example of the section will present other three pseudo variables.
The above example shows true , false and nil pseudo variables at work.
The true is used in boolean expression. The message is always printed.
This message is never printed. The condition is not met. In the boolean expression we always get a negative value.
If global values are referenced and have not been initialized, they contain the nil pseudo variable. It stands for the absence of a value.
Ruby predefined variables
Ruby has plenty of predefined global variables. This is a heritage of Perl language. Ruby was influenced strongly by Perl. They are accessible when the Ruby script starts. We have a few examples for the predefined Ruby variables.
Three predefined variables have been used: $0 , $* and $$ . The $0 stores the current script name. The $* variable stores command-line arguments. And the $$ stores the PID (process id) of the script.
The $? global variable stores the exit status of the last executed child process.
We run two external child processes and check their exit status with the $? variable.
With the use of the system method we start a child process. It is an echo bash command, which prints a message to the terminal.
In the second case we execute the bash exit command with status 1. This time we use the %x operator which executes a command between two selected delimiters. We have chosen [] characters.
The first child process terminates with status 0, the second with exit status 1.
In the final example, we show three global predefined variables that are used with regular expressions.
When we apply the =~ operator on a string, Ruby sets some variables. The $& variable has a string that matched the last last regular expression match. The $` has a string preceding $& and the $’ has a string following the $& .
In this part of the Ruby tutorial, we looked more deeply at the Ruby variables.

Using the Case (Switch) Ruby Statement
GrapchicStock / Getty Images
- Ruby Programming
- PHP Programming
- Java Programming
- Javascript Programming
- Delphi Programming
- C & C++ Programming
- Visual Basic
In most computer languages , the case or conditional (also known as switch ) statement compares the value of a variable with that of several constants or literals and executes the first path with a matching case. In Ruby , it's a bit more flexible (and powerful).
Instead of a simple equality test being performed, the case equality operator is used, opening the door to many new uses.
There are some differences from other languages though. In C , a switch statement is a kind of replacement for a series of if and goto statements. The cases are technically labels, and the switch statement will go to the matching label. This exhibits a behavior called "fallthrough," as the execution doesn't stop when it reaches another label.
This is usually avoided using a break statement, but fallthrough is sometimes intentional. The case statement in Ruby, on the other hand, can be seen as a shorthand for a series of if statements. There is no fallthrough, only the first matching case will be executed.
The Basic Form of a Case Statement
The basic form of a case statement is as follows.
As you can see, this is structured something like an if/else if/else conditional statement. The name (which we'll call the value ), in this case inputted from the keyboard, is compared to each of the cases from the when clauses (i.e. cases ), and the first when block with a matching case will be executed. If none of them match, the else block will be executed.
What's interesting here is how the value is compared to each of the cases. As mentioned above, in C++ , and other C-like languages, a simple value comparison is used. In Ruby, the case equality operator is used.
Remember that the type of the left-hand side of a case equality operator is important, and the cases are always the left-hand side. So, for each when clause, Ruby will evaluate case === value until it finds a match.
If we were to input Bob , Ruby would first evaluate "Alice" === "Bob" , which would be false since String#=== is defined as the comparison of the strings. Next, /[qrz].+/i === "Bob" would be executed, which is false since Bob doesn't begin with Q, R or Z.
Since none of the cases matched, Ruby will then execute the else clause.
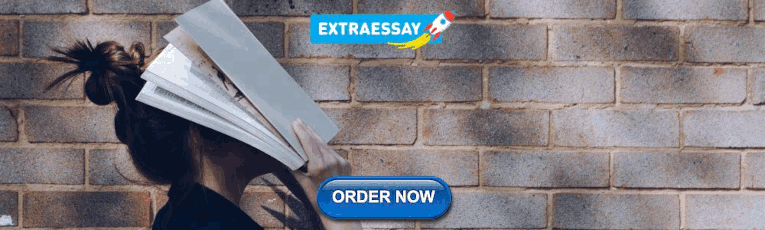
How the Type Comes Into Play
A common use of the case statement is to determine the type of value and do something different depending on its type. Though this breaks Ruby's customary duck typing, it's sometimes necessary to get things done.
This works by using the Class#=== (technically, the Module#=== ) operator, which tests if the right-hand side is_a? left-hand side.
The syntax is simple and elegant:
Another Possible Form
If the value is omitted, the case statement works a bit differently: it works almost exactly like an if/else if/else statement. The advantages of using the case statement over an if statement, in this case, are merely cosmetic.
A More Compact Syntax
There are times when there are a large number of small when clauses. Such a case statement easily grows too large to fit on the screen. When this is the case (no pun intended), you can use the then keyword to put the body of the when clause on the same line.
While this makes for some very dense code, as long as each when clause is very similar, it actually becomes more readable.
When you should use single-line and multi-line when clauses are up to you, it's a matter of style. However, mixing the two is not recommended - a case statement should follow a pattern to be as readable as possible.
Case Assignment
Like if statements, case statements evaluate to the last statement in the when clause. In other words, they can be used in assignments to provide a kind of table. However, don't forget that case statements are much more powerful than simple array or hash lookups. Such a table doesn't necessarily need to use literals in the when clauses.
If there is no matching when clause and no else clause, then the case statement will evaluate to nil .
- Using the Switch Statement for Multiple Choices in Java
- The 7 Best Programming Languages to Learn for Beginners
- Conditional Statements in Java
- String Literals
- What Are Ternary (Conditional) Operators in Ruby?
- If-Then and If-Then-Else Conditional Statements in Java
- The JavaScript Ternary Operator as a Shortcut for If/Else Statements
- How to Use Loops in Ruby
- Control Statements in C++
- How to Use String Substitution in Ruby
- Basic Guide to Creating Arrays in Ruby
- Using the Command Line to Run Ruby Scripts
- Java Expressions Introduced
- Using the Each Method in Ruby
- Global Variables in Ruby
- Using the "Split" Method

Variables and Constants in Ruby
Introduction.
Variables and constants are fundamental concepts in programming languages, including Ruby. They allow us to store and manipulate data, making our programs adaptable and dynamic. In this tutorial, we will dive into the basics of variables and constants in Ruby, understanding their usage and exploring some practical examples.
Declaration and Assignment
In Ruby, variables are declared by simply assigning a value to them. There is no need to specify a datatype explicitly. Let's consider the following code snippet:
In the above example, we have declared a variable called message and assigned it the value "Hello, Ruby!" . Ruby dynamically determines the datatype based on the assigned value.
Naming Conventions and Scope
When naming variables, it is important to follow certain conventions to ensure code readability. Variable names in Ruby are case-sensitive and must start with a lowercase letter or an underscore. It is common to use snake_case for variable names.
Another aspect to consider is variable scope. Ruby employs different types of variable scope, such as local, global, and instance variables, which are beyond the scope of this tutorial. However, it is crucial to understand the scope of a variable to avoid potential issues and ensure code functionality.
Similar to variables, constants in Ruby are declared by assigning a value to them. However, constant names start with an uppercase letter. Let's see an example:
In this case, we have declared a constant PI and assigned it the value 3.14 . It is important to note that constants should not be reassigned once they are declared, although a warning, rather than an error, will be raised.
Usage and Advantages
Constants are useful when we have values that will remain unchanged throughout our program, such as mathematical constants or configuration details. By using constants, we can improve code readability and maintainability, as it clearly indicates the intention of non-changing values.
Practical Examples
To gain a better understanding, let's explore a couple of practical examples.
Example 1: Simple Addition
In this example, we declare two variables num1 and num2 and assign them the values 10 and 5 , respectively. We then add these two numbers and store the result in the variable sum . Finally, we display the sum using puts .
Example 2: Calculation of Area
Here, we calculate the area of a rectangle by multiplying the length and width. Similar to the previous example, we declare length and width variables, assign them respective values, calculate the area, and display the result using puts .
Variables and constants are crucial elements in the Ruby programming language, allowing us to store and manipulate data effectively. In this tutorial, we covered the basics of variables and constants, exploring their declaration, naming conventions, scope, and practical usage through code examples. It is recommended to practice and experiment with different scenarios to strengthen your understanding of these concepts.
Remember, mastering variables and constants is a pivotal step towards becoming a proficient Ruby programmer. Keep coding and discovering the infinite possibilities!

Hi, I'm Ada, your personal AI tutor. I can help you with any coding tutorial. Go ahead and ask me anything.
I have a question about this topic
Give more examples
Ruby Style Guide
Table of contents, guiding principles, a note about consistency, translations, source encoding, tabs or spaces, indentation, maximum line length, no trailing whitespace, line endings, should i terminate files with a newline, should i terminate expressions with ; , one expression per line, operator method call, spaces and operators, safe navigation, spaces and braces, no space after bang, no space inside range literals, indent when to case, indent conditional assignment, empty lines between methods, two or more empty lines, empty lines around attribute accessor, empty lines around access modifier, empty lines around bodies, trailing comma in method arguments, spaces around equals, line continuation in expressions, multi-line method chains, method arguments alignment, implicit options hash, dsl method calls, space in method calls, space in brackets access, multi-line arrays alignment, english for identifiers, snake case for symbols, methods and variables, identifiers with a numeric suffix, capitalcase for classes and modules, snake case for files, snake case for directories, one class per file, screaming snake case for constants, predicate methods suffix, predicate methods prefix, dangerous method suffix, relationship between safe and dangerous methods, unused variables prefix, other parameter, then in multi-line expression, condition placement, ternary operator vs if, nested ternary operators, semicolon in if, case vs if-else, returning result from if / case, one-line cases, semicolon in when, semicolon in in, double negation, multi-line ternary operator, if as a modifier, multi-line if modifiers, nested modifiers, if vs unless, using else with unless, parentheses around condition, multi-line while do, while as a modifier, while vs until, infinite loop, loop with break, explicit return, explicit self, shadowing methods, safe assignment in condition, begin blocks, nested conditionals, raise vs fail, raising explicit runtimeerror, exception class messages, return from ensure, implicit begin, contingency methods, suppressing exceptions, using rescue as a modifier, using exceptions for flow of control, blind rescues, exception rescuing ordering, reading from a file, writing to a file, release external resources, auto-release external resources, atomic file operations, standard exceptions, parallel assignment, values swapping, dealing with trailing underscore variables in destructuring assignment, self-assignment, conditional variable initialization shorthand, existence check shorthand, identity comparison, explicit use of the case equality operator, is_a vs kind_of, is_a vs instance_of, instance_of vs class comparison, proc application shorthand, single-line blocks delimiters, single-line do … end block, explicit block argument, trailing comma in block parameters, nested method definitions, multi-line lambda definition, stabby lambda definition with parameters, stabby lambda definition without parameters, proc vs proc.new, short methods, top-level methods, no single-line methods, endless methods, double colons, colon method definition, method definition parentheses, method call parentheses, too many params, optional arguments, keyword arguments order, boolean keyword arguments, keyword arguments vs optional arguments, keyword arguments vs option hashes, arguments forwarding, block forwarding, private global methods, consistent classes, mixin grouping, single-line classes, file classes, namespace definition, modules vs classes, module_function, solid design, define to_s, attr family, accessor/mutator method names, don’t extend struct.new, don’t extend data.define, duck typing, no class vars, leverage access modifiers (e.g. private and protected ), access modifiers indentation, defining class methods, alias method lexically, alias_method, class and self, defining constants within a block, factory methods, disjunctive assignment in constructor, no comments, rationale comments, english comments, english syntax, no superfluous comments, comment upkeep, refactor, don’t comment, annotations placement, annotations keyword format, multi-line annotations indentation, inline annotations, document annotations, magic comments first, below shebang, one magic comment per line, separate magic comments from code, literal array and hash, no trailing array commas, no gappy arrays, first and last, set vs array, symbols as keys, no mutable keys, hash literals, hash literal values, hash literal as last array item, no mixed hash syntaxes, avoid hash[] constructor, hash#fetch defaults, use hash blocks, hash#values_at and hash#fetch_values, hash#transform_keys and hash#transform_values, ordered hashes, no modifying collections, accessing elements directly, provide alternate accessor to collections, map / find / select / reduce / include / size, count vs size, reverse_each, object#yield_self vs object#then, slicing with ranges, underscores in numerics, numeric literal prefixes, integer type checking, random numbers, float division, float comparison, exponential notation, string interpolation, consistent string literals, no character literals, curlies interpolate, string concatenation, don’t abuse gsub, string#chars, named format tokens, long strings, squiggly heredocs, heredoc delimiters, heredoc method calls, heredoc argument closing parentheses, no datetime, plain text search, using regular expressions as string indexes, prefer non-capturing groups, do not mix named and numbered captures, refer named regexp captures by name, avoid perl-style last regular expression group matchers, avoid numbered groups, limit escapes, caret and dollar regexp, multi-line regular expressions, comment complex regular expressions, use gsub with a block or a hash for complex replacements, %q shorthand, percent literal braces, no needless metaprogramming, no monkey patching, block class_eval, eval comment docs, no method_missing, prefer public_send, prefer __send__, rd (block) comments, no ruby_version in the gemspec, no flip-flops, no non- nil checks, global input/output streams, array coercion, ranges or between, predicate methods, no cryptic perlisms, use require_relative whenever possible, always warn, no optional hash params, instance vars, optionparser, no param mutations, three is the number thou shalt count, functional code, no explicit .rb to require, sources of inspiration, how to contribute, spread the word, introduction.
Role models are important.
This Ruby style guide recommends best practices so that real-world Ruby programmers can write code that can be maintained by other real-world Ruby programmers. A style guide that reflects real-world usage gets used, while a style guide that holds to an ideal that has been rejected by the people it is supposed to help risks not getting used at all - no matter how good it is.
The guide is separated into several sections of related guidelines. We’ve tried to add the rationale behind the guidelines (if it’s omitted we’ve assumed it’s pretty obvious).
We didn’t come up with all the guidelines out of nowhere - they are mostly based on the professional experience of the editors, feedback and suggestions from members of the Ruby community and various highly regarded Ruby programming resources, such as "Programming Ruby" and "The Ruby Programming Language" .
This style guide evolves over time as additional conventions are identified and past conventions are rendered obsolete by changes in Ruby itself.
Programs must be written for people to read, and only incidentally for machines to execute.
It’s common knowledge that code is read much more often than it is written. The guidelines provided here are intended to improve the readability of code and make it consistent across the wide spectrum of Ruby code. They are also meant to reflect real-world usage of Ruby instead of a random ideal. When we had to choose between a very established practice and a subjectively better alternative we’ve opted to recommend the established practice. [ 1 ]
There are some areas in which there is no clear consensus in the Ruby community regarding a particular style (like string literal quoting, spacing inside hash literals, dot position in multi-line method chaining, etc.). In such scenarios all popular styles are acknowledged and it’s up to you to pick one and apply it consistently.
Ruby had existed for over 15 years by the time the guide was created, and the language’s flexibility and lack of common standards have contributed to the creation of numerous styles for just about everything. Rallying people around the cause of community standards took a lot of time and energy, and we still have a lot of ground to cover.
Ruby is famously optimized for programmer happiness. We’d like to believe that this guide is going to help you optimize for maximum programmer happiness.
A foolish consistency is the hobgoblin of little minds, adored by little statesmen and philosophers and divines.
A style guide is about consistency. Consistency with this style guide is important. Consistency within a project is more important. Consistency within one class or method is the most important.
However, know when to be inconsistent — sometimes style guide recommendations just aren’t applicable. When in doubt, use your best judgment. Look at other examples and decide what looks best. And don’t hesitate to ask!
In particular: do not break backwards compatibility just to comply with this guide!
Some other good reasons to ignore a particular guideline:
When applying the guideline would make the code less readable, even for someone who is used to reading code that follows this style guide.
To be consistent with surrounding code that also breaks it (maybe for historic reasons) — although this is also an opportunity to clean up someone else’s mess (in true XP style).
Because the code in question predates the introduction of the guideline and there is no other reason to be modifying that code.
When the code needs to remain compatible with older versions of Ruby that don’t support the feature recommended by the style guide.
Translations of the guide are available in the following languages:
Chinese Simplified
Chinese Traditional
Egyptian Arabic
Portuguese (pt-BR)
Source Code Layout
Nearly everybody is convinced that every style but their own is ugly and unreadable. Leave out the "but their own" and they’re probably right…
Use UTF-8 as the source file encoding.
Use only spaces for indentation. No hard tabs.
Use two spaces per indentation level (aka soft tabs).
Limit lines to 80 characters.
A lot of people these days feel that a maximum line length of 80 characters is just a remnant of the past and makes little sense today. After all - modern displays can easily fit 200+ characters on a single line. Still, there are some important benefits to be gained from sticking to shorter lines of code.
First, and foremost - numerous studies have shown that humans read much faster vertically and very long lines of text impede the reading process. As noted earlier, one of the guiding principles of this style guide is to optimize the code we write for human consumption.
Additionally, limiting the required editor window width makes it possible to have several files open side-by-side, and works well when using code review tools that present the two versions in adjacent columns.
The default wrapping in most tools disrupts the visual structure of the code, making it more difficult to understand. The limits are chosen to avoid wrapping in editors with the window width set to 80, even if the tool places a marker glyph in the final column when wrapping lines. Some web based tools may not offer dynamic line wrapping at all.
Some teams strongly prefer a longer line length. For code maintained exclusively or primarily by a team that can reach agreement on this issue, it is okay to increase the line length limit up to 100 characters, or all the way up to 120 characters. Please, restrain the urge to go beyond 120 characters.
Avoid trailing whitespace.
Use Unix-style line endings. [ 2 ]
End each file with a newline.
Don’t use ; to terminate statements and expressions.
Use one expression per line.
Avoid dot where not required for operator method calls.
Use spaces around operators, after commas, colons and semicolons. Whitespace might be (mostly) irrelevant to the Ruby interpreter, but its proper use is the key to writing easily readable code.
There are a few exceptions:
Exponent operator:
Slash in rational literals:
Safe navigation operator:
Avoid chaining of &. . Replace with . and an explicit check. E.g. if users are guaranteed to have an address and addresses are guaranteed to have a zip code:
If such a change introduces excessive conditional logic, consider other approaches, such as delegation:
No spaces after ( , [ or before ] , ) . Use spaces around { and before } .
{ and } deserve a bit of clarification, since they are used for block and hash literals, as well as string interpolation.
For hash literals two styles are considered acceptable. The first variant is slightly more readable (and arguably more popular in the Ruby community in general). The second variant has the advantage of adding visual difference between block and hash literals. Whichever one you pick - apply it consistently.
With interpolated expressions, there should be no padded-spacing inside the braces.
No space after ! .
No space inside range literals.
Indent when as deep as case .
This is the style established in both "The Ruby Programming Language" and "Programming Ruby". Historically it is derived from the fact that case and switch statements are not blocks, hence should not be indented, and the when and else keywords are labels (compiled in the C language, they are literally labels for JMP calls).
When assigning the result of a conditional expression to a variable, preserve the usual alignment of its branches.
Use empty lines between method definitions and also to break up methods into logical paragraphs internally.
Don’t use several empty lines in a row.
Use empty lines around attribute accessor.
Use empty lines around access modifier.
Don’t use empty lines around method, class, module, block bodies.
Avoid comma after the last parameter in a method call, especially when the parameters are not on separate lines.
Use spaces around the = operator when assigning default values to method parameters:
While several Ruby books suggest the first style, the second is much more prominent in practice (and arguably a bit more readable).
Avoid line continuation with \ where not required. In practice, avoid using line continuations for anything but string concatenation.
Adopt a consistent multi-line method chaining style. There are two popular styles in the Ruby community, both of which are considered good - leading . and trailing . .
When continuing a chained method call on another line, keep the . on the second line.
When continuing a chained method call on another line, include the . on the first line to indicate that the expression continues.
A discussion on the merits of both alternative styles can be found here .
Align the arguments of a method call if they span more than one line. When aligning arguments is not appropriate due to line-length constraints, single indent for the lines after the first is also acceptable.
Omit the outer braces around an implicit options hash.
Omit both the outer braces and parentheses for methods that are part of an internal DSL (e.g., Rake, Rails, RSpec).
Do not put a space between a method name and the opening parenthesis.
Do not put a space between a receiver name and the opening brackets.
Align the elements of array literals spanning multiple lines.
Naming Conventions
The only real difficulties in programming are cache invalidation and naming things.
Name identifiers in English.
Use snake_case for symbols, methods and variables.
Do not separate numbers from letters on symbols, methods and variables.
Use CapitalCase for classes and modules. (Keep acronyms like HTTP, RFC, XML uppercase).
Use snake_case for naming files, e.g. hello_world.rb .
Use snake_case for naming directories, e.g. lib/hello_world/hello_world.rb .
Aim to have just a single class/module per source file. Name the file name as the class/module, but replacing CapitalCase with snake_case .
Use SCREAMING_SNAKE_CASE for other constants (those that don’t refer to classes and modules).
The names of predicate methods (methods that return a boolean value) should end in a question mark (i.e. Array#empty? ). Methods that don’t return a boolean, shouldn’t end in a question mark.
Avoid prefixing predicate methods with the auxiliary verbs such as is , does , or can . These words are redundant and inconsistent with the style of boolean methods in the Ruby core library, such as empty? and include? .
The names of potentially dangerous methods (i.e. methods that modify self or the arguments, exit! (doesn’t run the finalizers like exit does), etc) should end with an exclamation mark if there exists a safe version of that dangerous method.
Define the non-bang (safe) method in terms of the bang (dangerous) one if possible.
Prefix with _ unused block parameters and local variables. It’s also acceptable to use just _ (although it’s a bit less descriptive). This convention is recognized by the Ruby interpreter and tools like RuboCop will suppress their unused variable warnings.
When defining binary operators and operator-alike methods, name the parameter other for operators with "symmetrical" semantics of operands. Symmetrical semantics means both sides of the operator are typically of the same or coercible types.
Operators and operator-alike methods with symmetrical semantics (the parameter should be named other ): `, `-`, ` + , / , % , * , == , > , < , | , & , ^ , eql? , equal? .
Operators with non-symmetrical semantics (the parameter should not be named other ): << , [] (collection/item relations between operands), === (pattern/matchable relations).
Note that the rule should be followed only if both sides of the operator have the same semantics. Prominent exception in Ruby core is, for example, Array#*(int) .
Flow of Control
Do not use for , unless you know exactly why. Most of the time iterators should be used instead. for is implemented in terms of each (so you’re adding a level of indirection), but with a twist - for doesn’t introduce a new scope (unlike each ) and variables defined in its block will be visible outside it.
Do not use then for multi-line if / unless / when / in .
Always put the condition on the same line as the if / unless in a multi-line conditional.
Prefer the ternary operator( ?: ) over if/then/else/end constructs. It’s more common and obviously more concise.
Use one expression per branch in a ternary operator. This also means that ternary operators must not be nested. Prefer if/else constructs in these cases.
Do not use if x; … . Use the ternary operator instead.
Prefer case over if-elsif when compared value is the same in each clause.
Leverage the fact that if and case are expressions which return a result.
Use when x then … for one-line cases.
Do not use when x; … . See the previous rule.
Do not use in pattern; … . Use in pattern then … for one-line in pattern branches.
Use ! instead of not .
Avoid unnecessary uses of !!
!! converts a value to boolean, but you don’t need this explicit conversion in the condition of a control expression; using it only obscures your intention.
Consider using it only when there is a valid reason to restrict the result true or false . Examples include outputting to a particular format or API like JSON, or as the return value of a predicate? method. In these cases, also consider doing a nil check instead: !something.nil? .
Do not use and and or in boolean context - and and or are control flow operators and should be used as such. They have very low precedence, and can be used as a short form of specifying flow sequences like "evaluate expression 1, and only if it is not successful (returned nil ), evaluate expression 2". This is especially useful for raising errors or early return without breaking the reading flow.
Avoid several control flow operators in one expression, as that quickly becomes confusing:
Simply put - because they add some cognitive overhead, as they don’t behave like similarly named logical operators in other languages.
First of all, and and or operators have lower precedence than the = operator, whereas the && and || operators have higher precedence than the = operator, based on order of operations.
Also && has higher precedence than || , where as and and or have the same one. Funny enough, even though and and or were inspired by Perl, they don’t have different precedence in Perl.
Avoid multi-line ?: (the ternary operator); use if / unless instead.
Prefer modifier if / unless usage when you have a single-line body. Another good alternative is the usage of control flow and / or .
Avoid modifier if / unless usage at the end of a non-trivial multi-line block.
Avoid nested modifier if / unless / while / until usage. Prefer && / || if appropriate.
Prefer unless over if for negative conditions (or control flow || ).
Do not use unless with else . Rewrite these with the positive case first.
Don’t use parentheses around the condition of a control expression.
Do not use while/until condition do for multi-line while/until .
Prefer modifier while/until usage when you have a single-line body.
Prefer until over while for negative conditions.
Use Kernel#loop instead of while / until when you need an infinite loop.
Use Kernel#loop with break rather than begin/end/until or begin/end/while for post-loop tests.
Avoid return where not required for flow of control.
Avoid self where not required. (It is only required when calling a self write accessor, methods named after reserved words, or overloadable operators.)
As a corollary, avoid shadowing methods with local variables unless they are both equivalent.
Don’t use the return value of = (an assignment) in conditional expressions unless the assignment is wrapped in parentheses. This is a fairly popular idiom among Rubyists that’s sometimes referred to as safe assignment in condition .
Avoid the use of BEGIN blocks.
Do not use END blocks. Use Kernel#at_exit instead.
Avoid use of nested conditionals for flow of control.
Prefer a guard clause when you can assert invalid data. A guard clause is a conditional statement at the top of a function that bails out as soon as it can.
Prefer next in loops instead of conditional blocks.
Prefer raise over fail for exceptions.
Don’t specify RuntimeError explicitly in the two argument version of raise .
Prefer supplying an exception class and a message as two separate arguments to raise , instead of an exception instance.
Do not return from an ensure block. If you explicitly return from a method inside an ensure block, the return will take precedence over any exception being raised, and the method will return as if no exception had been raised at all. In effect, the exception will be silently thrown away.
Use implicit begin blocks where possible.
Mitigate the proliferation of begin blocks by using contingency methods (a term coined by Avdi Grimm).
Don’t suppress exceptions.
Avoid using rescue in its modifier form.
Don’t use exceptions for flow of control.
Avoid rescuing the Exception class. This will trap signals and calls to exit , requiring you to kill -9 the process.
Put more specific exceptions higher up the rescue chain, otherwise they’ll never be rescued from.
Use the convenience methods File.read or File.binread when only reading a file start to finish in a single operation.
Use the convenience methods File.write or File.binwrite when only opening a file to create / replace its content in a single operation.
Release external resources obtained by your program in an ensure block.
Use versions of resource obtaining methods that do automatic resource cleanup when possible.
When doing file operations after confirming the existence check of a file, frequent parallel file operations may cause problems that are difficult to reproduce. Therefore, it is preferable to use atomic file operations.
Prefer the use of exceptions from the standard library over introducing new exception classes.
Assignment & Comparison
Avoid the use of parallel assignment for defining variables. Parallel assignment is allowed when it is the return of a method call (e.g. Hash#values_at ), used with the splat operator, or when used to swap variable assignment. Parallel assignment is less readable than separate assignment.
Use parallel assignment when swapping 2 values.
Avoid the use of unnecessary trailing underscore variables during parallel assignment. Named underscore variables are to be preferred over underscore variables because of the context that they provide. Trailing underscore variables are necessary when there is a splat variable defined on the left side of the assignment, and the splat variable is not an underscore.
Use shorthand self assignment operators whenever applicable.
Use ||= to initialize variables only if they’re not already initialized.
Use &&= to preprocess variables that may or may not exist. Using &&= will change the value only if it exists, removing the need to check its existence with if .
Prefer equal? over == when comparing object_id . Object#equal? is provided to compare objects for identity, and in contrast Object#== is provided for the purpose of doing value comparison.
Similarly, prefer using Hash#compare_by_identity than using object_id for keys:
Note that Set also has Set#compare_by_identity available.
Avoid explicit use of the case equality operator === . As its name implies it is meant to be used implicitly by case expressions and outside of them it yields some pretty confusing code.
Prefer is_a? over kind_of? . The two methods are synonyms, but is_a? is the more commonly used name in the wild.
Prefer is_a? over instance_of? .
While the two methods are similar, is_a? will consider the whole inheritance chain (superclasses and included modules), which is what you normally would want to do. instance_of? , on the other hand, only returns true if an object is an instance of that exact class you’re checking for, not a subclass.
Use Object#instance_of? instead of class comparison for equality.
Do not use eql? when using == will do. The stricter comparison semantics provided by eql? are rarely needed in practice.
Blocks, Procs & Lambdas
Use the Proc call shorthand when the called method is the only operation of a block.
Prefer {…} over do…end for single-line blocks. Avoid using {…} for multi-line blocks (multi-line chaining is always ugly). Always use do…end for "control flow" and "method definitions" (e.g. in Rakefiles and certain DSLs). Avoid do…end when chaining.
Some will argue that multi-line chaining would look OK with the use of {…}, but they should ask themselves - is this code really readable and can the blocks' contents be extracted into nifty methods?
Use multi-line do … end block instead of single-line do … end block.
Consider using explicit block argument to avoid writing block literal that just passes its arguments to another block.
Avoid comma after the last parameter in a block, except in cases where only a single argument is present and its removal would affect functionality (for instance, array destructuring).
Do not use nested method definitions, use lambda instead. Nested method definitions actually produce methods in the same scope (e.g. class) as the outer method. Furthermore, the "nested method" will be redefined every time the method containing its definition is called.
Use the new lambda literal syntax for single-line body blocks. Use the lambda method for multi-line blocks.
Don’t omit the parameter parentheses when defining a stabby lambda with parameters.
Omit the parameter parentheses when defining a stabby lambda with no parameters.
Prefer proc over Proc.new .
Prefer proc.call() over proc[] or proc.() for both lambdas and procs.
Avoid methods longer than 10 LOC (lines of code). Ideally, most methods will be shorter than 5 LOC. Empty lines do not contribute to the relevant LOC.
Avoid top-level method definitions. Organize them in modules, classes or structs instead.
Avoid single-line methods. Although they are somewhat popular in the wild, there are a few peculiarities about their definition syntax that make their use undesirable. At any rate - there should be no more than one expression in a single-line method.
One exception to the rule are empty-body methods.
Only use Ruby 3.0’s endless method definitions with a single line body. Ideally, such method definitions should be both simple (a single expression) and free of side effects.
Use :: only to reference constants (this includes classes and modules) and constructors (like Array() or Nokogiri::HTML() ). Do not use :: for regular method calls.
Do not use :: to define class methods.
Use def with parentheses when there are parameters. Omit the parentheses when the method doesn’t accept any parameters.
Use parentheses around the arguments of method calls, especially if the first argument begins with an open parenthesis ( , as in f((3 + 2) + 1) .
Method Call with No Arguments
Always omit parentheses for method calls with no arguments.
Methods That Have "keyword" Status in Ruby
Always omit parentheses for methods that have "keyword" status in Ruby.
Non-Declarative Methods That Have "keyword" Status in Ruby
For non-declarative methods with "keyword" status (e.g., various Kernel instance methods), two styles are considered acceptable. By far the most popular style is to omit parentheses. Rationale: The code reads better, and method calls look more like keywords. A less-popular style, but still acceptable, is to include parentheses. Rationale: The methods have ordinary semantics, so why treat them differently, and it’s easier to achieve a uniform style by not worrying about which methods have "keyword" status. Whichever one you pick, apply it consistently.
Using super with Arguments
Always use parentheses when calling super with arguments:
Avoid parameter lists longer than three or four parameters.
Define optional arguments at the end of the list of arguments. Ruby has some unexpected results when calling methods that have optional arguments at the front of the list.
Put required keyword arguments before optional keyword arguments. Otherwise, it’s much harder to spot optional keyword arguments there, if they’re hidden somewhere in the middle.
Use keyword arguments when passing a boolean argument to a method.
Prefer keyword arguments over optional arguments.
Use keyword arguments instead of option hashes.
Use Ruby 2.7’s arguments forwarding.
Use Ruby 3.1’s anonymous block forwarding.
In most cases, block argument is given name similar to &block or &proc . Their names have no information and & will be sufficient for syntactic meaning.
If you really need "global" methods, add them to Kernel and make them private.
Classes & Modules
Use a consistent structure in your class definitions.
Split multiple mixins into separate statements.
Prefer a two-line format for class definitions with no body. It is easiest to read, understand, and modify.
Don’t nest multi-line classes within classes. Try to have such nested classes each in their own file in a folder named like the containing class.
Define (and reopen) namespaced classes and modules using explicit nesting. Using the scope resolution operator can lead to surprising constant lookups due to Ruby’s lexical scoping , which depends on the module nesting at the point of definition.
Prefer modules to classes with only class methods. Classes should be used only when it makes sense to create instances out of them.
Prefer the use of module_function over extend self when you want to turn a module’s instance methods into class methods.
When designing class hierarchies make sure that they conform to the Liskov Substitution Principle .
Try to make your classes as SOLID as possible.
Always supply a proper to_s method for classes that represent domain objects.
Use the attr family of functions to define trivial accessors or mutators.
For accessors and mutators, avoid prefixing method names with get_ and set_ . It is a Ruby convention to use attribute names for accessors (readers) and attr_name= for mutators (writers).
Avoid the use of attr . Use attr_reader and attr_accessor instead.
Consider using Struct.new , which defines the trivial accessors, constructor and comparison operators for you.
Don’t extend an instance initialized by Struct.new . Extending it introduces a superfluous class level and may also introduce weird errors if the file is required multiple times.
Don’t extend an instance initialized by Data.define . Extending it introduces a superfluous class level.
Prefer duck-typing over inheritance.
Avoid the usage of class ( @@ ) variables due to their "nasty" behavior in inheritance.
As you can see all the classes in a class hierarchy actually share one class variable. Class instance variables should usually be preferred over class variables.
Assign proper visibility levels to methods ( private , protected ) in accordance with their intended usage. Don’t go off leaving everything public (which is the default).
Indent the public , protected , and private methods as much as the method definitions they apply to. Leave one blank line above the visibility modifier and one blank line below in order to emphasize that it applies to all methods below it.
Use def self.method to define class methods. This makes the code easier to refactor since the class name is not repeated.
Prefer alias when aliasing methods in lexical class scope as the resolution of self in this context is also lexical, and it communicates clearly to the user that the indirection of your alias will not be altered at runtime or by any subclass unless made explicit.
Since alias , like def , is a keyword, prefer bareword arguments over symbols or strings. In other words, do alias foo bar , not alias :foo :bar .
Also be aware of how Ruby handles aliases and inheritance: an alias references the method that was resolved at the time the alias was defined; it is not dispatched dynamically.
In this example, Fugitive#given_name would still call the original Westerner#first_name method, not Fugitive#first_name . To override the behavior of Fugitive#given_name as well, you’d have to redefine it in the derived class.
Always use alias_method when aliasing methods of modules, classes, or singleton classes at runtime, as the lexical scope of alias leads to unpredictability in these cases.
When class (or module) methods call other such methods, omit the use of a leading self or own name followed by a . when calling other such methods. This is often seen in "service classes" or other similar concepts where a class is treated as though it were a function. This convention tends to reduce repetitive boilerplate in such classes.
Do not define constants within a block, since the block’s scope does not isolate or namespace the constant in any way.
Define the constant outside of the block instead, or use a variable or method if defining the constant in the outer scope would be problematic.
Classes: Constructors
Consider adding factory methods to provide additional sensible ways to create instances of a particular class.
In constructors, avoid unnecessary disjunctive assignment ( ||= ) of instance variables. Prefer plain assignment. In ruby, instance variables (beginning with an @ ) are nil until assigned a value, so in most cases the disjunction is unnecessary.
Good code is its own best documentation. As you’re about to add a comment, ask yourself, "How can I improve the code so that this comment isn’t needed?". Improve the code and then document it to make it even clearer.
Write self-documenting code and ignore the rest of this section. Seriously!
If the how can be made self-documenting, but not the why (e.g. the code works around non-obvious library behavior, or implements an algorithm from an academic paper), add a comment explaining the rationale behind the code.
Write comments in English.
Use one space between the leading # character of the comment and the text of the comment.
Comments longer than a word are capitalized and use punctuation. Use one space after periods.
Avoid superfluous comments.
Keep existing comments up-to-date. An outdated comment is worse than no comment at all.
Good code is like a good joke: it needs no explanation.
Avoid writing comments to explain bad code. Refactor the code to make it self-explanatory. ("Do or do not - there is no try." Yoda)
Comment Annotations
Annotations should usually be written on the line immediately above the relevant code.
The annotation keyword is followed by a colon and a space, then a note describing the problem.
If multiple lines are required to describe the problem, subsequent lines should be indented three spaces after the # (one general plus two for indentation purposes).
In cases where the problem is so obvious that any documentation would be redundant, annotations may be left at the end of the offending line with no note. This usage should be the exception and not the rule.
Use TODO to note missing features or functionality that should be added at a later date.
Use FIXME to note broken code that needs to be fixed.
Use OPTIMIZE to note slow or inefficient code that may cause performance problems.
Use HACK to note code smells where questionable coding practices were used and should be refactored away.
Use REVIEW to note anything that should be looked at to confirm it is working as intended. For example: REVIEW: Are we sure this is how the client does X currently?
Use other custom annotation keywords if it feels appropriate, but be sure to document them in your project’s README or similar.
Magic Comments
Place magic comments above all code and documentation in a file (except shebangs, which are discussed next).
Place magic comments below shebangs when they are present in a file.
Use one magic comment per line if you need multiple.
Separate magic comments from code and documentation with a blank line.
Collections
Prefer literal array and hash creation notation (unless you need to pass parameters to their constructors, that is).
Prefer %w to the literal array syntax when you need an array of words (non-empty strings without spaces and special characters in them). Apply this rule only to arrays with two or more elements.
Prefer %i to the literal array syntax when you need an array of symbols (and you don’t need to maintain Ruby 1.9 compatibility). Apply this rule only to arrays with two or more elements.
Avoid comma after the last item of an Array or Hash literal, especially when the items are not on separate lines.
Avoid the creation of huge gaps in arrays.
When accessing the first or last element from an array, prefer first or last over [0] or [-1] . first and last take less effort to understand, especially for a less experienced Ruby programmer or someone from a language with different indexing semantics.
Use Set instead of Array when dealing with unique elements. Set implements a collection of unordered values with no duplicates. This is a hybrid of Array 's intuitive inter-operation facilities and Hash 's fast lookup.
Prefer symbols instead of strings as hash keys.
Avoid the use of mutable objects as hash keys.
Use the Ruby 1.9 hash literal syntax when your hash keys are symbols.
Use the Ruby 3.1 hash literal value syntax when your hash key and value are the same.
Wrap hash literal in braces if it is a last array item.
Don’t mix the Ruby 1.9 hash syntax with hash rockets in the same hash literal. When you’ve got keys that are not symbols stick to the hash rockets syntax.
Hash::[] was a pre-Ruby 2.1 way of constructing hashes from arrays of key-value pairs, or from a flat list of keys and values. It has an obscure semantic and looks cryptic in code. Since Ruby 2.1, Enumerable#to_h can be used to construct a hash from a list of key-value pairs, and it should be preferred. Instead of Hash[] with a list of literal keys and values, just a hash literal should be preferred.
Use Hash#key? instead of Hash#has_key? and Hash#value? instead of Hash#has_value? .
Use Hash#each_key instead of Hash#keys.each and Hash#each_value instead of Hash#values.each .
Use Hash#fetch when dealing with hash keys that should be present.
Introduce default values for hash keys via Hash#fetch as opposed to using custom logic.
Prefer the use of the block instead of the default value in Hash#fetch if the code that has to be evaluated may have side effects or be expensive.
Use Hash#values_at or Hash#fetch_values when you need to retrieve several values consecutively from a hash.
Prefer transform_keys or transform_values over each_with_object or map when transforming just the keys or just the values of a hash.
Rely on the fact that as of Ruby 1.9 hashes are ordered.
Do not modify a collection while traversing it.
When accessing elements of a collection, avoid direct access via [n] by using an alternate form of the reader method if it is supplied. This guards you from calling [] on nil .
When providing an accessor for a collection, provide an alternate form to save users from checking for nil before accessing an element in the collection.
Prefer map over collect , find over detect , select over find_all , reduce over inject , include? over member? and size over length . This is not a hard requirement; if the use of the alias enhances readability, it’s ok to use it. The rhyming methods are inherited from Smalltalk and are not common in other programming languages. The reason the use of select is encouraged over find_all is that it goes together nicely with reject and its name is pretty self-explanatory.
Don’t use count as a substitute for size . For Enumerable objects other than Array it will iterate the entire collection in order to determine its size.
Use flat_map instead of map + flatten . This does not apply for arrays with a depth greater than 2, i.e. if users.first.songs == ['a', ['b','c']] , then use map + flatten rather than flat_map . flat_map flattens the array by 1, whereas flatten flattens it all the way.
Prefer reverse_each to reverse.each because some classes that include Enumerable will provide an efficient implementation. Even in the worst case where a class does not provide a specialized implementation, the general implementation inherited from Enumerable will be at least as efficient as using reverse.each .
The method Object#then is preferred over Object#yield_self , since the name then states the intention, not the behavior. This makes the resulting code easier to read.
Slicing arrays with ranges to extract some of their elements (e.g ary[2..5] ) is a popular technique. Below you’ll find a few small considerations to keep in mind when using it.
[0..-1] in ary[0..-1] is redundant and simply synonymous with ary .
Ruby 2.6 introduced endless ranges, which provide an easier way to describe a slice going all the way to the end of an array.
Ruby 2.7 introduced beginless ranges, which are also handy in slicing. However, unlike the somewhat obscure -1 in ary[1..-1] , the 0 in ary[0..42] is clear as a starting point. In fact, changing it to ary[..42] could potentially make it less readable. Therefore, using code like ary[0..42] is fine. On the other hand, ary[nil..42] should be replaced with ary[..42] or arr[0..42] .
Add underscores to large numeric literals to improve their readability.
Prefer lowercase letters for numeric literal prefixes. 0o for octal, 0x for hexadecimal and 0b for binary. Do not use 0d prefix for decimal literals.
Use Integer to check the type of an integer number. Since Fixnum is platform-dependent, checking against it will return different results on 32-bit and 64-bit machines.
Prefer to use ranges when generating random numbers instead of integers with offsets, since it clearly states your intentions. Imagine simulating a roll of a dice:
When performing float-division on two integers, either use fdiv or convert one-side integer to float.
Avoid (in)equality comparisons of floats as they are unreliable.
Floating point values are inherently inaccurate, and comparing them for exact equality is almost never the desired semantics. Comparison via the ==/!= operators checks floating-point value representation to be exactly the same, which is very unlikely if you perform any arithmetic operations involving precision loss.
When using exponential notation for numbers, prefer using the normalized scientific notation, which uses a mantissa between 1 (inclusive) and 10 (exclusive). Omit the exponent altogether if it is zero.
The goal is to avoid confusion between powers of ten and exponential notation, as one quickly reading 10e7 could think it’s 10 to the power of 7 (one then 7 zeroes) when it’s actually 10 to the power of 8 (one then 8 zeroes). If you want 10 to the power of 7, you should do 1e7 .
One could favor the alternative engineering notation, in which the exponent must always be a multiple of 3 for easy conversion to the thousand / million / … system.
Alternative : engineering notation:
Prefer string interpolation and string formatting to string concatenation:
Adopt a consistent string literal quoting style. There are two popular styles in the Ruby community, both of which are considered good - single quotes by default and double quotes by default.
Single Quote
Prefer single-quoted strings when you don’t need string interpolation or special symbols such as \t , \n , ' , etc.
Double Quote
Prefer double-quotes unless your string literal contains " or escape characters you want to suppress.
Don’t use the character literal syntax ?x . Since Ruby 1.9 it’s basically redundant - ?x would be interpreted as 'x' (a string with a single character in it).
Don’t leave out {} around instance and global variables being interpolated into a string.
Don’t use Object#to_s on interpolated objects. It’s called on them automatically.
Avoid using String#` when you need to construct large data chunks. Instead, use `String#<<`. Concatenation mutates the string instance in-place and is always faster than `String# , which creates a bunch of new string objects.
Don’t use String#gsub in scenarios in which you can use a faster and more specialized alternative.
Prefer the use of String#chars over String#split with empty string or regexp literal argument.
Prefer the use of sprintf and its alias format over the fairly cryptic String#% method.
When using named format string tokens, favor %<name>s over %{name} because it encodes information about the type of the value.
Break long strings into multiple lines but don’t concatenate them with + . If you want to add newlines, use heredoc. Otherwise use \ :
Use Ruby 2.3’s squiggly heredocs for nicely indented multi-line strings.
Use descriptive delimiters for heredocs. Delimiters add valuable information about the heredoc content, and as an added bonus some editors can highlight code within heredocs if the correct delimiter is used.
Place method calls with heredoc receivers on the first line of the heredoc definition. The bad form has significant potential for error if a new line is added or removed.
Place the closing parenthesis for method calls with heredoc arguments on the first line of the heredoc definition. The bad form has potential for error if the new line before the closing parenthesis is removed.
Date & Time
Prefer Time.now over Time.new when retrieving the current system time.
Don’t use DateTime unless you need to account for historical calendar reform - and if you do, explicitly specify the start argument to clearly state your intentions.
Regular Expressions
Some people, when confronted with a problem, think "I know, I’ll use regular expressions." Now they have two problems.
Don’t use regular expressions if you just need plain text search in string.
For simple constructions you can use regexp directly through string index.
Use non-capturing groups when you don’t use the captured result.
Do not mix named captures and numbered captures in a Regexp literal. Because numbered capture is ignored if they’re mixed.
Prefer using names to refer named regexp captures instead of numbers.
Don’t use the cryptic Perl-legacy variables denoting last regexp group matches ( $1 , $2 , etc). Use Regexp.last_match(n) instead.
Avoid using numbered groups as it can be hard to track what they contain. Named groups can be used instead.
Character classes have only a few special characters you should care about: ^ , - , \ , ] , so don’t escape . or brackets in [] .
Be careful with ^ and $ as they match start/end of line, not string endings. If you want to match the whole string use: \A and \z (not to be confused with \Z which is the equivalent of /\n?\z/ ).
Use x (free-spacing) modifier for multi-line regexps.
Use x modifier for complex regexps. This makes them more readable and you can add some useful comments.
For complex replacements sub / gsub can be used with a block or a hash.
Percent Literals
Use %() (it’s a shorthand for %Q ) for single-line strings which require both interpolation and embedded double-quotes. For multi-line strings, prefer heredocs.
Avoid %() or the equivalent %q() unless you have a string with both ' and " in it. Regular string literals are more readable and should be preferred unless a lot of characters would have to be escaped in them.
Use %r only for regular expressions matching at least one / character.
Avoid the use of %x unless you’re going to execute a command with backquotes in it (which is rather unlikely).
Avoid the use of %s . It seems that the community has decided :"some string" is the preferred way to create a symbol with spaces in it.
Use the braces that are the most appropriate for the various kinds of percent literals.
() for string literals ( %q , %Q ).
[] for array literals ( %w , %i , %W , %I ) as it is aligned with the standard array literals.
{} for regexp literals ( %r ) since parentheses often appear inside regular expressions. That’s why a less common character with { is usually the best delimiter for %r literals.
() for all other literals (e.g. %s , %x )
Metaprogramming
Avoid needless metaprogramming.
Do not mess around in core classes when writing libraries (do not monkey-patch them).
The block form of class_eval is preferable to the string-interpolated form.
Supply Location
When you use the string-interpolated form, always supply __FILE__ and __LINE__ , so that your backtraces make sense:
define_method
define_method is preferable to class_eval { def … }
When using class_eval (or other eval ) with string interpolation, add a comment block showing its appearance if interpolated (a practice used in Rails code):
Avoid using method_missing for metaprogramming because backtraces become messy, the behavior is not listed in #methods , and misspelled method calls might silently work, e.g. nukes.luanch_state = false . Consider using delegation, proxy, or define_method instead. If you must use method_missing :
Be sure to also define respond_to_missing?
Only catch methods with a well-defined prefix, such as find_by_* --make your code as assertive as possible.
Call super at the end of your statement
Delegate to assertive, non-magical methods:
Prefer public_send over send so as not to circumvent private / protected visibility.
Prefer __send__ over send , as send may overlap with existing methods.
API Documentation
Use YARD and its conventions for API documentation.
Don’t use block comments. They cannot be preceded by whitespace and are not as easy to spot as regular comments.
This is not really a block comment syntax, but more of an attempt to emulate Perl’s POD documentation system.
There’s an rdtool for Ruby that’s pretty similar to POD. Basically rdtool scans a file for =begin and =end pairs, and extracts the text between them all. This text is assumed to be documentation in RD format . You can read more about it here .
RD predated the rise of RDoc and YARD and was effectively obsoleted by them. [ 3 ]
Gemfile and Gemspec
The gemspec should not contain RUBY_VERSION as a condition to switch dependencies. RUBY_VERSION is determined by rake release , so users may end up with wrong dependency.
Fix by either:
Post-install messages.
Add both gems as dependency (if permissible).
If development dependencies, move to Gemfile.
Avoid the use of flip-flop operators .
Don’t do explicit non- nil checks unless you’re dealing with boolean values.
Use $stdout/$stderr/$stdin instead of STDOUT/STDERR/STDIN . STDOUT/STDERR/STDIN are constants, and while you can actually reassign (possibly to redirect some stream) constants in Ruby, you’ll get an interpreter warning if you do so.
Use warn instead of $stderr.puts . Apart from being more concise and clear, warn allows you to suppress warnings if you need to (by setting the warn level to 0 via -W0 ).
Prefer the use of Array#join over the fairly cryptic Array#* with a string argument.
Use Array() instead of explicit Array check or [*var] , when dealing with a variable you want to treat as an Array, but you’re not certain it’s an array.
Use ranges or Comparable#between? instead of complex comparison logic when possible.
Prefer the use of predicate methods to explicit comparisons with == . Numeric comparisons are OK.
Avoid using Perl-style special variables (like $: , $; , etc). They are quite cryptic and their use in anything but one-liner scripts is discouraged.
Use the human-friendly aliases provided by the English library if required.
For all your internal dependencies, you should use require_relative . Use of require should be reserved for external dependencies
This way is more expressive (making clear which dependency is internal or not) and more efficient (as require_relative doesn’t have to try all of $LOAD_PATH contrary to require ).
Write ruby -w safe code.
Avoid hashes as optional parameters. Does the method do too much? (Object initializers are exceptions for this rule).
Use module instance variables instead of global variables.
Use OptionParser for parsing complex command line options and ruby -s for trivial command line options.
Do not mutate parameters unless that is the purpose of the method.
Avoid more than three levels of block nesting.
Code in a functional way, avoiding mutation when that makes sense.
Omit the .rb extension for filename passed to require and require_relative .
The method tap can be helpful for debugging purposes but should not be left in production code.
This is simpler and more efficient.
Here are some tools to help you automatically check Ruby code against this guide.
RuboCop is a Ruby static code analyzer and formatter, based on this style guide. RuboCop already covers a significant portion of the guide and has plugins for most popular Ruby editors and IDEs.
RubyMine 's code inspections are partially based on this guide.
This guide started its life in 2011 as an internal company Ruby coding guidelines (written by Bozhidar Batsov ). Bozhidar had always been bothered as a Ruby developer about one thing - Python developers had a great programming style reference ( PEP-8 ) and Rubyists never got an official guide, documenting Ruby coding style and best practices. Bozhidar firmly believed that style matters. He also believed that a great hacker community, such as Ruby has, should be quite capable of producing this coveted document. The rest is history…
At some point Bozhidar decided that the work he was doing might be interesting to members of the Ruby community in general and that the world had little need for another internal company guideline. But the world could certainly benefit from a community-driven and community-sanctioned set of practices, idioms and style prescriptions for Ruby programming.
Bozhidar served as the guide’s only editor for a few years, before a team of editors was formed once the project transitioned to RuboCop HQ.
Since the inception of the guide we’ve received a lot of feedback from members of the exceptional Ruby community around the world. Thanks for all the suggestions and the support! Together we can make a resource beneficial to each and every Ruby developer out there.
Many people, books, presentations, articles and other style guides influenced the community Ruby style guide. Here are some of them:
"The Elements of Style"
"The Elements of Programming Style"
The Python Style Guide (PEP-8)
"Programming Ruby"
"The Ruby Programming Language"
Contributing
The guide is still a work in progress - some guidelines are lacking examples, some guidelines don’t have examples that illustrate them clearly enough. Improving such guidelines is a great (and simple way) to help the Ruby community!
In due time these issues will (hopefully) be addressed - just keep them in mind for now.
Nothing written in this guide is set in stone. It’s our desire to work together with everyone interested in Ruby coding style, so that we could ultimately create a resource that will be beneficial to the entire Ruby community.
Feel free to open tickets or send pull requests with improvements. Thanks in advance for your help!
You can also support the project (and RuboCop) with financial contributions via one of the following platforms:
GitHub Sponsors
It’s easy, just follow the contribution guidelines below:
Fork rubocop/ruby-style-guide on GitHub
Make your feature addition or bug fix in a feature branch.
Include a good description of your changes
Push your feature branch to GitHub
Send a Pull Request
This guide is written in AsciiDoc and is published as HTML using AsciiDoctor . The HTML version of the guide is hosted on GitHub Pages.
Originally the guide was written in Markdown, but was converted to AsciiDoc in 2019.

A community-driven style guide is of little use to a community that doesn’t know about its existence. Tweet about the guide, share it with your friends and colleagues. Every comment, suggestion or opinion we get makes the guide just a little bit better. And we want to have the best possible guide, don’t we?
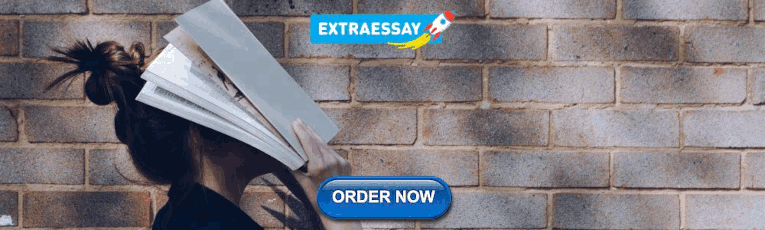
IMAGES
VIDEO
COMMENTS
Ruby Case & Ranges. The case statement is more flexible than it might appear at first sight. Let's see an example where we want to print some message depending on what range a value falls in. case capacity. when 0. "You ran out of gas." when 1..20. "The tank is almost empty. Quickly, find a gas station!"
Yes, there is a parallel assignment in your first sample ( limit,pattern = q[0],q[1] ). But when you try to involve a case expression, it stops being one. By the way, you could simply write limit, pattern = q. indeed, but it would be more the ruby way if the intent (on the left hand side of the =) would be reflected in the the syntax of writing ...
Structure of a Ruby Case Statement. A Ruby case statement is structured around several key components: case: Initiates the case statement, evaluating the variable in question; when: Represents individual conditions to be matched; else: Executes an alternative action if no conditions match, though this is optional. Utilizing Case Statements with ...
Ruby program that times case-statement count = 100000 n1 = Time.now.usec x = 0 v = 5 # Version 1: assign a variable with a case-statement. count.times do x = case v when 0..1 then 1 when 2..3 then 2 when 4..6 then 3 else 0 end end puts x n2 = Time.now.usec # Version 2: assign a variable with an if-statement.
a = 2 case when a == 1, a == 2 puts "a is one or two" when a == 3 puts "a is three" else puts "I don't know what a is" end. Again, the then and else are optional. The result value of a case expression is the last value executed in the expression. Since Ruby 2.7, case expressions also provide a more powerful experimental pattern matching feature ...
Ruby | Case Statement. The case statement is a multiway branch statement just like a switch statement in other languages. It provides an easy way to forward execution to different parts of code based on the value of the expression. There are 3 important keywords which are used in the case statement: case: It is similar to the switch keyword in ...
Let's start with the most basic of basics, the assigning of variables to barewords: a = "Ruby". In the above code, we have assigned a value of "Ruby" to the bareword a, which tells our ...
Assignment. In Ruby, assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: v = 5. Assignment creates a local variable if the variable was not previously referenced. An assignment expression result is always the assigned value, including assignment methods.
Pattern matching. Pattern matching is a feature allowing deep matching of structured values: checking the structure and binding the matched parts to local variables. Pattern matching in Ruby is implemented with the case / in expression: case <expression>. in <pattern1>.
Ruby, like any other programming language, has some naming conventions for variable identifiers. Ruby is a case sensitive language. It means that age and Age are two different variable names. ... We cannot assign values to pseudo variables. The self is the receiver of the current method. The nil is the sole instance of the NilClass. It ...
Using the Case (Switch) Ruby Statement. In most computer languages, the case or conditional (also known as switch) statement compares the value of a variable with that of several constants or literals and executes the first path with a matching case. In Ruby, it's a bit more flexible (and powerful). Instead of a simple equality test being ...
a = 1. method `a' called. a = 99. During the parse, Ruby sees the use of a in the first puts statement and, as it hasn't yet seen any assignment to a, assumes that it is a method call. By the time it gets to the second puts statement, though, it has seen an assignment, and so treats a as a variable.
Case. In Ruby a case-statement tests values. The statement matches the value of a variable against ranges and other values. And it yields a specified value. ... assign a variable with a case-statement. count.times do x = case v when 0..1 then 1 when 2..3 then 2 when 4..6 then 3 else 0 end end puts x n2 = Time.now.usec # Version 2: ...
Variables Declaration and Assignment. In Ruby, variables are declared by simply assigning a value to them. There is no need to specify a datatype explicitly. ... Variable names in Ruby are case-sensitive and must start with a lowercase letter or an underscore. It is common to use snake_case for variable names.
Ruby has a fairly powerful case..when..else construct for when you need to match criteria against a single variable. What is the "canonical" way to match criteria against multiple variables without simply nesting case statements?. Wrapping multiple variables in an array (like [x, y]) and matching against it isn't equivalent, because Ruby won't apply the magical case === operator to the ...
Assignment ¶ ↑. In Ruby assignment uses the = (equals sign) character. This example assigns the number five to the local variable v: v = 5. Assignment creates a local variable if the variable was not previously referenced. Local Variable Names ¶ ↑. A local variable name must start with a lowercase US-ASCII letter or a character with the ...
Ruby had existed for over 15 years by the time the guide was created, and the language's flexibility and lack of common standards have contributed to the creation of numerous styles for just about everything. ... swapping variable assignment # Swapping variable assignment is a special case because it will allow you to # swap the values that ...
You cannot compare variables, you can only compare objects, and variables aren't objects. Ruby doesn't have a case statement, only a case expression. (In fact, Ruby doesn't have statements at all .) (This may sound overly pedantic, but understanding the difference between variables and objects is fundamental not only in Ruby, but in programming ...