
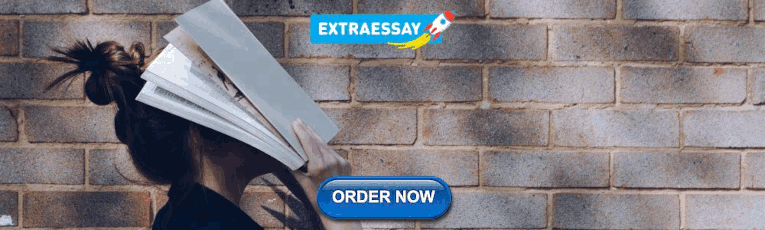
Java Program Case Study on Public Static Void Main
A case study on public static void main (250 words)
Case study:
The program structure of a simple java program is given below with different steps
- Step-1: Click start+run and then type notepad in run dialog box and click OK. It displays Notepad.
- Step-2: In run dialogbox type cmd and click OK. It displays command prompt.
- Step-3: Type the following program in the Notepad and save the program as “example.java” in a
- c:\xxxx >javac example.java
- c:\xxxx>java example
Explanation:
- Exception in thread “main” java. lang.NoClassDefFoundError: ex
- public is an access specifier. If a class is visible to all classes then the public is used
- main() must be declared as public since it must be called by outside of its class.
- The keyword static allows main() to be called without creating an object of the class.
- The keyword void represents that main( ) does not return a value.
- The main method contains one parameter String args[].
- We can send some input values (arguments) at run time to the String args[] of the main method . These arguments are called command-line arguments. These command-line arguments are passed at the command prompt.
- System is a predefined class that provides access to the system.
- out is the output stream.
- println() method display the output in different lines. If we use print() method it display the output in the same line
Leave a Comment Cancel reply
Save my name, email, and website in this browser for the next time I comment.
Please Enable JavaScript in your Browser to Visit this Site.
- Demystifying “public static void main()”: Building a “Hello World” Java Application
By Alejandro Gervasio October 15, 2018 Java Core
The Java language is a neat example of the implementation of the OOP (object-oriented programming) paradigm. While it's fair to admit that mastering the paradigm's foundations is in most cases a difficult process, funny enough, Java itself is fairly easy to learn.
In this introductory article, we'll learn how to develop from scratch a simple Java application that prints out to the console the classic “Hello World” message.
Defining the “HelloWorldApplication” Class
To keep things simple, we'll create our example “Hello World” application without relying on any IDE in particular.
Therefore, let’s launch our preferred text editor, (e.g. NotePad on Windows or Text Edit on Mac), and write the following code:
Now, let's save the code snippet in a file called HelloWorldApplication.java , in the location of our choice in the file system.
While at first sight the example looks pretty naive, it actually covers a lot of background. Thus, let's break it down in several parts, so we can understand more clearly how it works:
- The HelloWorldApplication class : we defined it with the public access modifier preceding the class keyword and the class name. This means that the class is public, hence we can access it from anywhere within the application, with no restrictions whatsoever.
- The main() method is actually the sample application’s entry point. The public access modifier declares the method public, while that the static keyword implies that there’s no need to instantiate the class to call it. Lastly, the void keyword signals that the method returns no value(s).
- The main() method arguments, String[] args: an array of strings , which we can use for passing command-line arguments to the method.
- The System.out.println statement does exactly what we might expect: it prints out to the console the “Hello World!” text.
Compiling the “HelloWorldApplication” Class
At this point, HelloWorldApplication.java is just a plain text file with a fancy extension. To make it an executable file, though, first we need to compile it.
Compiling a Java file means basically converting the source code to bytecode, a format which can be interpreted and executed by the JVM (Java Virtual Machine).
Therefore, to compile our source file, let’s open up a command prompt. Next, let’s navigate to the location in the file system where we previously saved the HelloWorldApplication.java file, and type the javac command, followed by the name of the source file (the .java extension is required):
The javac command reads Java classes and interface definitions, and compiles them into bytecode class files. In addition, it can also process annotations in Java source files and classes.
Once we have run the javac command, a new executable HelloWorldApplication.class file should have been created in the same location of HelloWorldApplication.java .
Running the Executable File
The java command runs a Java program from a command prompt. When we invoke the java command, the JRE (Java Runtime Environment) is loaded, along with the supplied class. Finally, the class' main() method is executed.
In this case, the HelloWorldApplication class' main() method will be called. As a result, we should see the “Hello World!” text printed out in the console:
Further Improvements
Of course, there's plenty of room to improve the existing functionality of the HelloWorldApplication class.
We could refactor, for instance, the main() method, so it can take one or more command-line arguments and print them out to the console, along with the “Hello World!” message:
Likewise, we should first recompile the HelloWorldApplication.java file by using the javac command, and then rerun the class file by passing the command-line arguments to the java command:
As expected, the refactored example will generate the following output:
In this tutorial, we learned how to build from scratch a basic Java application. We deliberately kept all the examples easy to understand, as we put strong emphasis on learning a few key concepts of the Java language.
Here's a quick summary of them:
- Class: the de-facto unit of data and behavior in Java, and the blueprint for objects. Objects or class instances can maintain internal state through one or more fields, and implement behavior via one or multiple methods.
- The public access modifier: it allows us to declare the public visibility of class fields and methods.
- Method or function: a code block container, which we can use to encapsulate behavior within a certain class.
- Array: a dynamically-created container object that holds a fixed number of elements of the same type.
Featured Posts
- An Introductory Look at Java Strings
Support this Site
As the old saying goes, content is king . Of course, delivering high-quality Java content in a reasonable timeline takes time and a lot of effort.
Do you like the current content and want to see many other Java topics covered in the near future? Then feel free to hire me , or please consider making a donation:
In either case, thank you for your support!
- Java Core OOP
- Design Patterns Spring
- Hibernate Vavr
- Bean Validation Java EE
public static void main (string args[]) Explanation
In Java, JVM (Java Virtual Machine) will always look for a specific method signature to start running an application, and that would be the public static void main (String args[]). The main() method represents the entry point of Java programs, and knowing how to use it correctly is very important.
In the above application example, we are using the public static void main. Each word has a different meaning and purpose. Keep learning to know more about each and every keyword.
It is an Access Modifier , which defines who can access this Method. Public means that this Method will be accessible by any Class(If other Classes can access this Class.).
Static is a keyword that identifies the class-related thing. It means the given Method or variable is not instance-related but Class related. It can be accessed without creating the instance of a Class.
It is used to define the Return Type of the Method. It defines what the method can return. Void means the Method will not return any value.
Main is the name of the Method . This Method name is searched by JVM as a starting point for an application with a particular signature only.
String args[] / String… args
Here args is an argument of the type String array. Which is used to pass the command-line argument to the main method. Though the type of the argument (String array) is fixed, you can still change the name from args to anything. Also with the introduction of java args, instead of writing String args[], String… args can be used. You can either use String array (String args[]) or var args(String… args) variable of String type. Both will work the same way.
Given all the options, the syntaxes of the main methods are the same and all will work fine.
78 Comments → public static void main (string args[]) Explanation
Hi, Good explanation! If I have a Main class (Main.java) and two more classes, Schoolmember and Staff (Schoolmember.java and Staff.java), do I have to have “public static void main(String[] args)” in all of these or only in Main? Thank You
Sorry for the late reply. It depends on what you want to achieve. Main is supposed to work as starting point of the application. If you are using other classes in Main.java and trying to invoke those methods from Main.java then one main method in Main.java is enough.
But if these classes are independent and you want to execute them individually then they need to have main method too.
i found it nice and easy to understand the topic of the public static void main . It would be better to explain it using video . Than it gates to our mind and if we want to tack it out also it will not come .
Thank you, Kaushal. Creating a video for this short topic is not easy but will see if it is possible.
Well explained. Thank you
hi public is not access modifier , it is access specifier in java
What does it mean?
Thanks a lot. Explained in an easy way!!
Hi, I already knew the main method is the entry point of the program and makes it execute the program properly but this explanation is really good for adults and kids. Thank you very much. This helped me a lot for public static void main(String[] args). I appreciated it a lot. I am a seven year old kid and I love programming.
Welcome Sachet
you are wrong in main definition like “ss” there we are use “is”
Static Static ss a keyword….. what is the ss means
That was a typo. I meant to say “Static is a keyword….”. It has been corrected now.
A Java program is a sequence of Java instructions that are executed in a certain order. Since the Java instructions are executed in a certain order, a Java program has a start and an end.
Nice explanation….
What is the meaning of creating an instance of the class ??
What is the meaning of creating an instance of the class ?? Please reply me.
Nice One Explanation !!
thank u for the given content\
Main method is very important in java. Program execution starts from main () method only.
why we are using string[] args in pubic static void main(String[] args) why we are not using character array or int array i need elabrate answer
Sir how to call a method how the whole process goes in a program please explain with example
hello sir if we not use static in with voidmain() how we run void main() function without static keyword
You can write main method without static keyword, there will not be any issue. But it will not work as expected. It will be treated as normal method and in order to execute it you need to call given method explicitly. While static keyword will make this method to be treated as entry point. Hope it clarifies.
thank u for update ur information i have some cofusion in null point exception please send me a link of ur site in null point exception
I dont have any article specifically written on null pointer exception
Can you please explain…how internally works these SYSOUT statement?? System.out.println(“args[” +i+ “]:”+args[i] ); System.out.println(“args[ i ]:”+args[i] );
http://www.mathcs.emory.edu/~cheung/Courses/170/Syllabus/09/command-args.html
1) System -is a class in the java.lang package .
2) out -is a static member of the System class, and is an instance of java.io.PrintStream .
3) println -is a method of java.io.PrintStream. This method is overloaded to print message to output destination, which is typically a console or file.
what is command line argument
Command passed at time of runtime.. On console you pass your input as an argument..
what is array of string
It is a argument passed to Main method.
why we pass only string as the parameter of the main method?
Argument passed in main method is array of string and not string. This is the standard followed.
I want list and description of in build classes and their sub classes…like printsteam is class and println() is its method…
Why we usethis method main(string args())
It works as starting point of application execution.
Thanks I guess this will useful for me
hi can you explain why public static void should write in the same order/sequence as written now.
Public and static keyword position can be changed. There is no such rule of using public before static. But as void is method return type it should come just before method name which is main in this case. Hence this sequence. public static void main static public void main.
Both are same. But as a standard we right as in the first case.
The grammar in this article and in the comments is horrendous.
u write a new english dictionary
It is shortcut English grammar that is enough for developers to understand each other so they can write efficient English with minimal words – like good programming style that is less verbose
I want to know why JVM treats main(String [] args) method as a starting point of program, can anyone tell me the internal functioning of this method, like how it gets execute and what threads are required to execute this method so that JVM can understand this method as the starting point of the program.?
Suppose you are watching a movie and the scene of movie starts from middle of a story and as you continue watching movie, u need to understand the current scene of movie and for that movie makes gives u a starting part of movie in somewhere middle of movie(Gives you information something like” 15years before ” in movie)..by reading this words in movie you get to know the starting of movie… So likewise u place you main method anywhere in program(inside class) the JVM will read full code in its language to find main() method and starts acknowledging(executing) the code from main method… and if you dont provide main() method in code the JVM will not understand the story of your code will give error…
good explanation but give me parameters at the same time explanation. i mean which type of parameters in using java. and please give me different meanings Hello and hello in java
My program still runs if I use other word instead of args in “String[] args”(Parameter to main method). I want to know the reason behind it.
args is just a name of argument. You can use any name you want but type should be array of string, Hope it clarifies.
Very Good Explanation Thank you
great article.thnk uu
Thank you so much! that’s a good article. I appreciated you
i want more explanation. i know this information.
read java book. java complete reference
Good Article thanks
It’s really nice & understandable words.
Why “String[] ags” is passed as parameter of main function and without passing this parameter what will happen?
This is a standard which needs to follow if we want jvm to search it to execute it. There is no harm in removing this parameter from method but then that method will be treated as other java methods and it will not be directly called by JVM.
you will get a compilation error if you dont use that
The main method will compile successfully but will not run. As it will be treated as any other method. Certain standard to be followed for main method
good to know!!!
hi!!! Nice Explanation Thanx
String args[] : Parameter to main method…
Please site how to use the main() with values given to parameters (like what the difference would be with (or without) these values).
If u dont use it shows error in execution….that y we r passing infinite arguments in String type…string is one.type of class ….so,first letter is capital
Hello Sir , Nice Info wanted to ask what are the parameters does main method takes thanku
String args[]
Please fix your grammatical errors. It makes understanding this content difficult.
content is gud…dont alwayz look at grammar
u go for a tuition to learn grammar and become an English professor. you are learning java or checking english errors!
right ,we have developer ,most engineering pass out in first but those student not able to do coding this is different between software developer and english gentleman.
this is very nice info,thank you so much for author.
In this platform not a english debata,it is a programming language platform so does not matter english grammer.(some of the english grammer mistake so we will be adjust……)
You’re a bloody ass, Gaurang.
Hi, Good Explanation… Thanking you….
Leave A Comment Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
This site uses Akismet to reduce spam. Learn how your comment data is processed .
Code With C
The Way to Programming
- C Tutorials
- Java Tutorials
- Python Tutorials
- PHP Tutorials
- Java Projects
Java’s Public Static Void – Why It Matters and What It Means
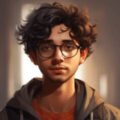
The Main method is a special method that is used to execute a program. When you run a java program in the command prompt then it calls main method. In this article, I am going to tell you why main method is public static void and why it is important to understand main method.
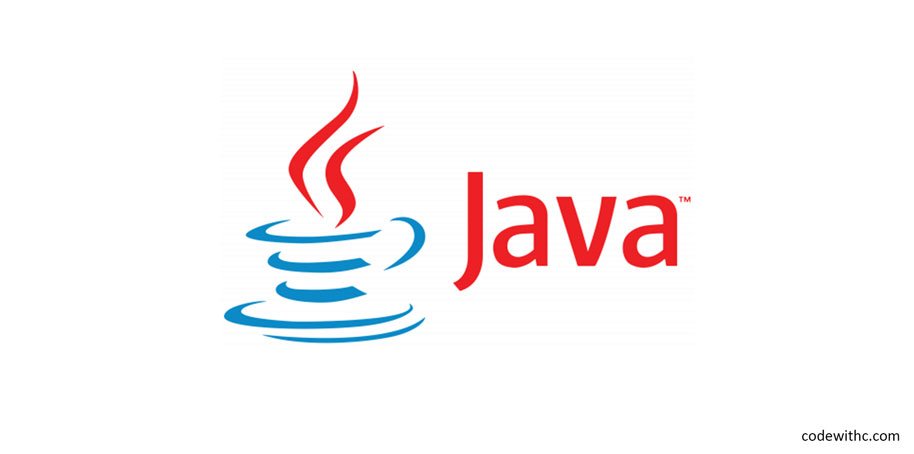
Main method in java is the first entry point of a class. It is where you will start the execution of a class. This main method is public static void and you can’t change the main method. The main method in java is the entry point of the class and it’s always present in the class file. The main method in java is the first method that executes when you run a java program. If you are a beginner in java programming then you may not know the concept of main method in java. If you want to understand the main method in java, then you must read this article. Here are the 5 important reasons why main method in java is public static void.
What is the Main method?
The Main method is a method that is used to execute a program. The method contains the code that is used to run the program. The main method is a method which will be called when the program starts. The main method is required to be declared at the start of the code. This method will be executed when the application starts.
Main Method with Parameters: This is the most common type of the main method. Here you need to specify the arguments or the parameters.
Main Method without Parameters – If you don’t provide any arguments or parameters then it is known as the main method without parameters.
Main Method without Parameter and Argument: If you don’t provide any arguments or parameters then it is known as the main method without parameters and arguments.
Why Main method is public static void?
When you run a program in the command prompt, then it calls main method of the class. So, the main method is the first thing that will be called when you run the program. That means main method is the first thing to be executed. This is the reason that the main method is a static method.
A static method is a method that can be accessed without creating an object. When you create an object, it creates a new memory space, so it is impossible to access the static method without an object. So, main method is a static method and it can be accessed without an object.
Why public, private or protected?
The public method can be accessed from outside, the private method can be accessed only inside the class and the protected method can be accessible both within and outside the class.
Conclusion:
I hope this article will help you to understand the importance of the main method and how it is a static method. If you have any questions regarding this article, then you can leave a comment below.
You Might Also Like
Revolutionary feedback control project for social networking: enhancing information spread, the evolution of web development: past, present, and future, defining the future: an overview of software services, exploring the layers: the multifaceted world of software, deep dive: understanding the ‘case when’ clause in sql.
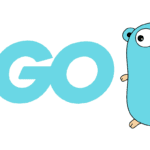
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Latest Posts
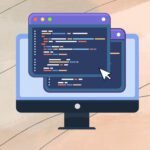
Top Python Project Ideas for Final Year Students – Python Projects
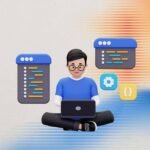
Top Python Projects for Class 12 Students – Class 12 Python Project Ideas to Ace Your Programming Skills
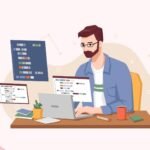
Top Python Project for Boosting Your Resume
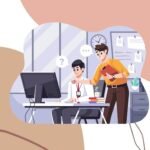
Essential Python Project for Beginners: Complete PDF Guide | Python Projects
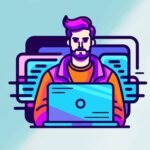
Ultimate Python Package Project Guide
Privacy overview.
Sign in to your account
Username or Email Address
Remember Me
- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
- Top 50 Interesting Unknown Facts about Programming
- Which Data Structure to choose for frequent insert and delete operation?
- Why can templates only be implemented in the header file?
- Top 50 ChatGPT Statistics & Facts
- What is the best way to inject Dependency in Java?
- 25 Interesting DSA Terms/Concepts Every Programmer Should Know
- GFact | Why doesn't C++ have Variable Length Arrays library?
- What are the Risks of using mysql_* Functions in PHP?
- 25 Interesting Facts about Arrays in Java
- How do you avoid a worst case algorithm for a quick sort?
- GFact | Bootstrapped Programming languages
- Hyperloop Technology
- GFact | In C++ a Structure can have Static Members
- GFact | Why setTimeout callback doesn't get called or work?
- GFact | Why Printing Boolean in C++ Display as 0 and 1
- GFact | What is an atomic operation?
- GFact | Most efficient way to Concatenate Strings in Python
- GFact | Enumeration constants
- GFact | const Behaviour in C and C++
Will the program run if we write static public void main in Java?
The entry point of a Java program is traditionally declared using the public static void main(String[] args) method. However, the language allows some flexibility in the order and visibility modifiers when declaring the main method. This has led to the question: What happens if we write static public void main instead of the conventional public static void main?
The Standard Declaration
The standard declaration of the main method in Java is:
This syntax is established by convention and follows the rules specified by the Java language.
The Reordered Declaration
Surprisingly, Java is lenient when it comes to the order of access modifiers and the static keyword in the main method declaration. The following declaration is also valid:
Here, we have switched the positions of static and public without any negative impact on the program’s execution. This flexibility might raise eyebrows among developers accustomed to strictly adhering to conventions, but it remains within the scope of Java’s language specifications.
Yes, the Java program will run successfully if you write static public void main instead of the conventional public static void main. Java is flexible regarding the order of access modifiers, and both declarations are valid for the main method.
The Reasoning Behind It:
Java’s syntax flexibility in the ‘main’ method declaration is a result of the language’s design decisions. The main method is a special method that serves as the entry point for the Java Virtual Machine (JVM) when executing a Java program. The JVM looks specifically for the public static void main signature, but the order of public and static is not a concern.
This flexibility can be attributed to the design principle of making the language user-friendly and avoiding unnecessary constraints on developers. It allows programmers to write the main method in a way that aligns with their preferences while still adhering to the fundamental requirements.
Best Practices and Conventions
While Java allows the flexibility to reorder static and public in the main method declaration, developers are encouraged to follow established conventions. The standard declaration (public static void main) is widely adopted in the Java community, and deviating from it might lead to confusion among other developers who expect the conventional syntax.
Maintaining a consistent coding style across projects and teams fosters readability and collaboration. Adhering to conventions ensures that codebases are easily understandable and maintainable, even by developers unfamiliar with the specific project.
Conclusion:
In the grand scheme of Java programming, the order of static and public in the main method is a matter of personal or team preference. While the language allows flexibility, the best practice is to follow conventions for consistency and to make code more accessible to others. The orderly disorder in this aspect of Java syntax reminds us that, in a well-designed language, there’s often room for flexibility without sacrificing correctness or readability.
Please Login to comment...
Similar reads.
- Otter.ai vs. Fireflies.ai: Which AI Transcribes Meetings More Accurately?
- Google Chrome Will Soon Let You Talk to Gemini In The Address Bar
- AI Interior Designer vs. Virtual Home Decorator: Which AI Can Transform Your Home Into a Pinterest Dream Faster?
- Top 10 Free Webclipper on Chrome Browser in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
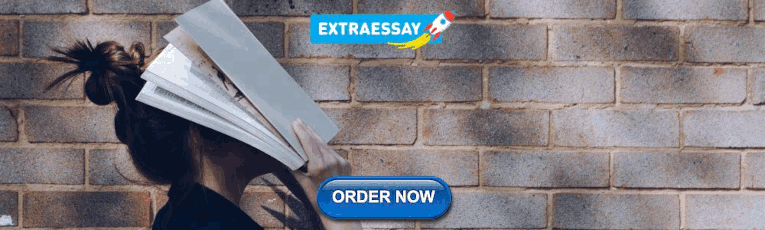
Object-Oriented Programming in Java – A Beginner's Guide

Hi, folks! Today we are going to talk about object-oriented programming in Java.
This article will help give you a thorough understanding of the underlying principles of object-oriented programming and its concepts.
Once you understand these concepts, you should have the confidence and ability to develop basic problem-solving applications using object-oriented programming principles in Java.
What is Object-Oriented Programming?
Object-oriented programming (OOP) is a fundamental programming paradigm based on the concept of “ objects ” . These objects can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods).
The core concept of the object-oriented approach is to break complex problems into smaller objects.
In this article, we will be looking at the following OOP concepts:
What is Java?
- What is a class?
- What is an object?
- What is a Java Virtual Machine (JVM)?
- How access modifiers work in Java.
- How constructors work in Java.
- How methods work in Java.
- Key principles of OOP.
- Interfaces in Java.
Java is a general-purpose, class-based, object-oriented programming language, which works on different operating systems such as Windows, Mac, and Linux.
You can use Java to develop:
- Desktop applications
- Web applications
- Mobile applications (especially Android apps)
- Web and application servers
- Big data processing
- Embedded systems
And much more.
In Java, every application starts with a class name, and this class must match the file name. When saving a file, save it using the class name and add “ .java ” to the end of the file name.
Let's write a Java program that prints the message “ Hello freeCodeCamp community. My name is ... ” .
We are going to start by creating our first Java file called Main.java, which can be done in any text editor. After creating and saving the file, we are going to use the below lines of code to get the expected output.
Don't worry if you don't understand the above code at the moment. We are going to discuss, step by step, each line of code just below.
For now, I want you to start by noting that every line of code that runs in Java must be in a class.
You may also note that Java is case-sensitive. This means that Java has the ability to distinguish between upper and lower case letters. For example, the variable “ myClass ” and the variable “ myclass ” are two totally different things.
Alright, let's see what that code's doing:
Let's first look at the main() method: public static void main(String[] args) .
This method is required in every Java program, and it is the most important one because it is the entry point of any Java program.
Its syntax is always public static void main(String[] args) . The only thing that can be changed is the name of the string array argument. For example, you can change args to myStringArgs .
What is a Class in Java?
A class is defined as a collection of objects. You can also think of a class as a blueprint from which you can create an individual object.
To create a class, we use the keyword class .
Syntax of a class in Java:
In the above syntax, we have fields (also called variables) and methods, which represent the state and behavior of the object, respectively.
Note that in Java, we use fields to store data, while we use methods to perform operations.
Let's take an example:
We are going to create a class named “ Main ” with a variable “ y ” . The variable “ y ” is going to store the value 2.
Note that a class should always start with an uppercase first letter, and the Java file should match the class name.
What is an Object in Java?
An object is an entity in the real world that can be distinctly identified. Objects have states and behaviors. In other words, they consist of methods and properties to make a particular type of data useful.
An object consists of:
- A unique identity: Each object has a unique identity, even if the state is identical to that of another object.
- State/Properties/Attributes: State tells us how the object looks or what properties it has.
- Behavior: Behavior tells us what the object does.
Examples of object states and behaviors in Java:
Let's look at some real-life examples of the states and behaviors that objects can have.
- Object: car.
- State: color, brand, weight, model.
- Behavior: break, accelerate, turn, change gears.
- Object: house.
- State: address, color, location.
- Behavior: open door, close door, open blinds.
Syntax of an object in Java:
What is the java virtual machine (jvm).
The Java virtual machine (JVM) is a virtual machine that enables a computer to run Java programs.
The JVM has two primary functions, which are:
- To allow Java programs to run on any device or operating system (this is also known as the "Write once, run anywhere" principle).
- And, to manage and optimize program memory.
How Access Modifiers Work in Java
In Java, access modifiers are keywords that set the accessibility of classes, methods, and other members.
These keywords determine whether a field or method in a class can be used or invoked by another method in another class or sub-class.
Access modifiers may also be used to restrict access.
In Java, we have four types of access modifiers, which are:
Let's look at each one in more detail now.
Default Access Modifier
The default access modifier is also called package-private. You use it to make all members within the same package visible, but they can be accessed only within the same package.
Note that when no access modifier is specified or declared for a class, method, or data member, it automatically takes the default access modifier.
Here is an example of how you can use the default access modifier:
Now let's see what this code is doing:
void output() : When there is no access modifier, the program automatically takes the default modifier.
SampleClass obj = new SampleClass(); : This line of code allows the program to access the class with the default access modifier.
obj.output(); : This line of code allows the program to access the class method with the default access modifier.
The output is: Hello World! This is an Introduction to OOP - Beginner's guide. .
Public Access Modifier
The public access modifier allows a class, a method, or a data field to be accessible from any class or package in a Java program. The public access modifier is accessible within the package as well as outside the package.
In general, a public access modifier does not restrict the entity at all.
Here is an example of how the public access modifier can be used:
Now let's see what's going on in that code:
In the above example,
- The public class Car is accessed from the Main class.
- The public variable tireCount is accessed from the Main class.
- The public method display() is accessed from the Main class.
Private Access Modifier
The private access modifier is an access modifier that has the lowest accessibility level. This means that the methods and fields declared as private are not accessible outside the class. They are accessible only within the class which has these private entities as its members.
You may also note that the private entities are not visible even to the subclasses of the class.
Here is an example of what would happen if you try accessing variables and methods declared private, outside the class:
Alright, what's going on here?
- private String activity : The private access modifier makes the variable “activity” a private one.
- SampleClass task = new SampleClass(); : We have created an object of SampleClass.
- task.activity = "We are learning the core concepts of OOP."; : On this line of code we are trying to access the private variable and field from another class (which can never be accessible because of the private access modifier).
When we run the above program, we will get the following error:
This is because we are trying to access the private variable and field from another class.
So, the best way to access these private variables is to use the getter and setter methods.
Getters and setters are used to protect your data, particularly when creating classes. When we create a getter method for each instance variable, the method returns its value while a setter method sets its value.
Let's have a look at how we can use the getters and setters method to access the private variable.
When we run the above program, this is the output:
As we have a private variable named task in the above example, we have used the methods getTask() and setTask() in order to access the variable from the outer class. These methods are called getter and setter in Java.
We have used the setter method ( setTask() ) to assign value to the variable and the getter method ( getTask() ) to access the variable.
To learn more about the this keyword, you can read this article here .
Protected Access Modifier
When methods and data members are declared protected , we can access them within the same package as well as from subclasses.
We can also say that the protected access modifier is somehow similar to the default access modifier. It is just that it has one exception, which is its visibility in subclasses.
Note that classes cannot be declared protected. This access modifier is generally used in a parent-child relationship.
Let's have a look at how we can use the protected access modifier:
What's this code doing?
In this example, the class Test which is present in another package is able to call the multiplyTwoNumbers() method, which is declared protected.
The method is able to do so because the Test class extends class Addition and the protected modifier allows the access of protected members in subclasses (in any packages).
What are Constructors in Java?
A constructor in Java is a method that you use to initialize newly created objects.
Syntax of a constructor in Java:
So what's going on in this code?
- We have started by creating the Main class.
- After that, we have created a class attribute, which is the variable a .
- Third, we have created a class constructor for the Main class.
- After that, we have set the initial value for variable a that we have declared. The variable a will have a value of 9. Our program will just take 3 times 3, which is equal to 9. You are free to assign any value to the variable a . (In programming, the symbol “*” means multiplication).
Every Java program starts its execution in the main() method. So, we have used the public static void main(String[] args) , and that is the point from where the program starts its execution. In other words, the main() method is the entry point of every Java program.
Now I'll explain what every keyword in the main() method does.
The public keyword.
The public keyword is an access modifier . Its role is to specify from where the method can be accessed, and who can access it. So, when we make the main() method public, it makes it globally available. In other words, it becomes accessible to all parts of the program.
The static keyword.
When a method is declared with a static keyword, it is known as a static method. So, the Java main() method is always static so that the compiler can call it without or before the creation of an object of the class.
If the main() method is allowed to be non-static, then the Java Virtual Machine will have to instantiate its class while calling the main() method.
The static keyword is also important as it saves unnecessary memory wasting which would have been used by the object declared only for calling the main() method by the Java Virtual Machine.
The Void keyword.
The void keyword is a keyword used to specify that a method doesn’t return anything. Whenever the main() method is not expected to return anything, then its return type is void. So, this means that as soon as the main() method terminates, the Java program terminates too.
Main is the name of the Java main method. It is the identifier that the Java Virtual Machine looks for as the starting point of the java program.
The String[] args .
This is an array of strings that stores Java command line arguments.
The next step is to create an object of the class Main. We have created a function call that calls the class constructor.
The last step is to print the value of a , which is 9.
How Methods Work in Java
A method is a block of code that performs a specific task. In Java, we use the term method, but in some other programming languages such as C++, the same method is commonly known as a function.
In Java, there are two types of methods:
- User-defined Methods : These are methods that we can create based on our requirements.
- Standard Library Methods : These are built-in methods in Java that are available to use.
Let me give you an example of how you can use methods in Java.
Java methods example 1:
In the above example, we have created a method named divideNumbers() . The method takes two parameters x and y, and we have called the method by passing two arguments firstNumber and secondNumber .
Now that you know some Java basics, let's look at object-oriented programming principles in a bit more depth.
Key Principles of Object-Oriented Programming.
There are the four main principles of the Object-Oriented Programming paradigm. These principles are also known as the pillars of Object-Oriented Programming.
The four main principles of Object-Oriented Programming are:
- Encapsulation (I will also touch briefly on Information Hiding)
- Inheritance
- Abstraction
- Polymorphism
Encapsulation and Information Hiding in Java
Encapsulation is when you wrap up your data under a single unit. In simple terms, it is more or less like a protective shield that prevents the data from being accessed by the code outside this shield.
A simple example of encapsulation is a school bag. A school bag can keep all your items safe in one place, such as your books, pens, pencils, ruler, and more.
Information hiding or data hiding in programming is about protecting data or information from any inadvertent change throughout the program. This is a powerful Object-Oriented Programming feature, and it is closely associated with encapsulation.
The idea behind encapsulation is to ensure that " sensitive " data is hidden from users. To achieve this, you must:
- Declare class variables/attributes as private .
- Provide public get and set methods to access and update the value of a private variable.
As you remember, private variables can only be accessed within the same class and an external class cannot access them. However, they can be accessed if we provide public get and set methods.
Let me give you an additional example that demonstrates how the get and set methods work:
Inheritance in Java
Inheritance allows classes to inherit attributes and methods of other classes. This means that parent classes extend attributes and behaviors to child classes. Inheritance supports reusability.
A simple example that explains the term inheritance is that human beings (in general) inherit certain properties from the class "Human" such as the ability to speak, breathe, eat, drink, and so on.
We group the "inheritance concept" into two categories:
- subclass (child) - the class that inherits from another class.
- superclass (parent) - the class being inherited from.
To inherit from a class, we use the extends keyword.
In the below example, the JerryTheMouse class is created by inheriting the methods and fields from the Animal class.
JerryTheMouse is the subclass and Animal is the superclass.
Abstraction in Java
Abstraction is a concept in object-oriented programming that lets you show only essential attributes and hides unnecessary information in your code. The main purpose of abstraction is to hide unnecessary details from your users.
A simple example to explain abstraction is to think about the process that comes into play when you send an email. When you send an email, complex details such as what happens as soon as it is sent and the protocol that the server uses are hidden from you.
When you send an e-mail, you just need to enter the email address of the receiver, the email subject, type the content, and click send.
You can abstract stuff by using abstract classes or interfaces .
The abstract keyword is a non-access modifier, used for classes and methods:
- Abstract class: is a restricted class that cannot be used to create objects. To access it, it must be inherited from another class.
- Abstract method: A method that doesn't have its body is known as an abstract method. We use the same abstract keyword to create abstract methods.
The body of an abstract method is provided by the subclass (inherited from).
Polymorphism in Java
Polymorphism refers to the ability of an object to take on many forms. Polymorphism normally occurs when we have many classes that are related to each other by inheritance.
Polymorphism is similar to how a person can have different characteristics at the same time.
For instance, a man can be a father, a grandfather, a husband, an employee, and so forth – all at the same time. So, the same person possesses different characteristics or behaviors in different situations.
We are going to create objects Cow and Cat, and call the animalSound() method on each of them.
Inheritance and polymorphism are very useful for code reusability. You can reuse the attributes and methods of an existing class when you create a new class.
Interfaces in Java
An interface is a collection of abstract methods. In other words, an interface is a completely " abstract class " used to group related methods with empty bodies.
An interface specifies what a class can do but not how it can do it.
We have looked at some of the main object-oriented programming concepts in this article. Having a good understanding of these concepts is essential if you want to use them well and write good code.
I hope this article was helpful.
My name is Patrick Cyubahiro, I am a software & web developer, UI/UX designer, technical writer, and Community Builder.
Feel free to connect with me on Twitter: @ Pat_Cyubahiro , or to write to: ampatrickcyubahiro[at]gmail.com
Thanks for reading and happy learning!
Community Builder, Software & web developer, UI/IX Designer, Technical Writer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
1(888)499-5521
1(888)814-4206
Amount to be Paid
Please don't hesitate to contact us if you have any questions. Our support team will be more than willing to assist you.
Advocate Educational Integrity
Our service exists to help you grow as a student, and not to cheat your academic institution. We suggest you use our work as a study aid and not as finalized material. Order a personalized assignment to study from.
- Words to pages
- Pages to words

Why is writing essays so hard?
Patterns and boring topics imposed by schools and universities are not very conducive to creativity and human development. Such essays are very difficult to write, because many are not interested in this and do not see the meaning of the text. There are a number of criteria that make it impossible to write essays:
- Boring and incomprehensible topics. Many topics could be more interesting, but teachers formulate them in a way that makes you want to yawn.
- Templates. 90% do not know how to make an essay interesting, how to turn this detailed answer to a question into a living story.
- Fear of not living up to expectations. It seems to many that the essay is stupid and that they simply did not understand the question. There is a fear of getting a bad mark and disappointing the professor, parents and classmates. There is a fear of looking stupid and embarrassing in front of the team.
- Lack of experience. People don't know what and how to write about. In order to make a good essay, you need to have a perfect understanding of the topic and have the skills of a writer.
That is why the company EssaysWriting provides its services. We remove the responsibility for the result from the clients and do everything to ensure that the scientific work is recognized.
Orders of are accepted for more complex assignment types only (e.g. Dissertation, Thesis, Term paper, etc.). Special conditions are applied to such orders. That is why please kindly choose a proper type of your assignment.
Finished Papers
Progressive delivery is highly recommended for your order. This additional service allows tracking the writing process of big orders as the paper will be sent to you for approval in parts/drafts* before the final deadline.
What is more, it guarantees:
- 30 days of free revision;
- A top writer and the best editor;
- A personal order manager.
* You can read more about this service here or please contact our Support team for more details.
It is a special offer that now costs only +15% to your order sum!
Would you like to order Progressive delivery for your paper?
Customer Reviews
Once your essay writing help request has reached our writers, they will place bids. To make the best choice for your particular task, analyze the reviews, bio, and order statistics of our writers. Once you select your writer, put the needed funds on your balance and we'll get started.

5 Signs of a quality essay writer service
Finished Papers
Megan Sharp
Customer Reviews
Can I speak with my essay writer directly?
A professional essay writing service is an instrument for a student who’s pressed for time or who doesn’t speak English as a first language. However, in 2022 native English-speaking students in the U.S. become to use essay help more and more. Why is that so? Mainly, because academic assignments are too boring and time-consuming. Also, because having an essay writer on your team who’s ready to come to homework rescue saves a great deal of trouble. is one of the best new websites where you get help with your essays from dedicated academic writers for a reasonable price.
Free essays categories
Dr.Jeffrey (PhD)
Why do I have to pay upfront for you to write my essay?
Finished Papers

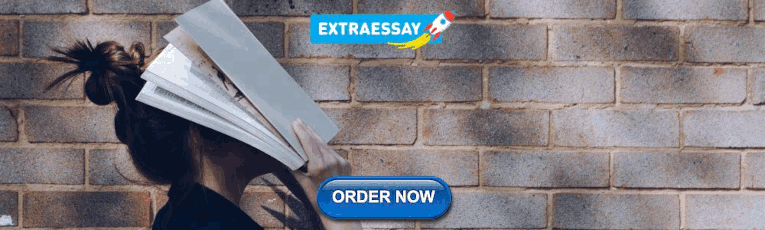
IMAGES
VIDEO
COMMENTS
Case study: The program structure of a simple java program is given below with different steps. Step-1: Click start+run and then type notepad in run dialog box and click OK. It displays Notepad. Step-2: In run dialogbox type cmd and click OK. It displays command prompt. Step-3: Type the following program in the Notepad and save the program as ...
Apart from the above-mentioned signature of main, you could use public static void main (String args []) or public static void main (String… args) to call the main function in Java. The main method is called if its formal parameter matches that of an array of Strings. Note: Main Method is Cumpulosory in Java.
The public access modifier declares the method public, while that the static keyword implies that there's no need to instantiate the class to call it. Lastly, the void keyword signals that the method returns no value(s). The main() method arguments, String[] args: an array of strings, which we can use for passing command-line arguments to the ...
void: It tells the return type of the method. "void" means the main method will not return any value. main: It is the name of the method. String args[]: It is the argument of the main method.
The three words have orthogonal meanings. public means that the method will be visible from classes in other packages. static means that the method is not attached to a specific instance, and it has no " this ". It is more or less a function. void is the return type. It means "this method returns nothing".
Understanding "static" in "public static void main" in Java. main () method: The main () method, in Java, is the entry point for the JVM (Java Virtual Machine) into the java program. JVM launches the java program by invoking the main () method. Static is a keyword. The role of adding static before any entity is to make that entity a ...
Public and static keyword position can be changed. There is no such rule of using public before static. But as void is method return type it should come just before method name which is main in this case. Hence this sequence. public static void main static public void main. Both are same. But as a standard we right as in the first case.
public class Test {public static void main (String [] args) {System. out. println ("Hello, World!". In this article you'll learn what each component of the main method means.. Java Main Method Syntax. The syntax of the main method is always:. public static void main (String [] args) {// some code}. You can change only the name of the String array argument. For example, you can change args to ...
Java's Public Static Void - Why It Matters and What It Means. Main method in java is the first entry point of a class. It is where you will start the execution of a class. This main method is public static void and you can't change the main method. The main method in java is the entry point of the class and it's always present in the ...
The keyword static is used to achieve such purposes. In this case, use of static ensures that the main () method is intended to be invoked in a regular way rather than by objects. As far as main ...
Yes, the Java program will run successfully if you write static public void main instead of the conventional public static void main. Java is flexible regarding the order of access modifiers, and both declarations are valid for the main method. Java's syntax flexibility in the 'main' method declaration is a result of the language's ...
The keyword public static void main is the means by which you create a main method within the Java application. It's the core method of the program and calls all others. It's the core method of ...
d) A case study AIM: A case study on public static void main(250 words) Case study: The SOURCE-CODE structure of a simple java SOURCE-CODE is given below with different steps. Step-1: Click start+run and then type notepad in run dialog box and click OK. It displays Notepad. Step-2: In run dialogbox type cmd and click OK. It displays command prompt.
To pass the quiz, you'll need to: Define the void and public statement keywords in the public static void main statement. Know what the statement signifies in a public statement. Select the code ...
main() must be declared as static because JVM does not know how to create an object of a class, so it needs a standard way to access the main method which is possible by declaring main() as static. If a method is declared as static then we can call that method outside the class without creating an object using the syntax ClassName.methodName(); .
Object-oriented programming (OOP) is a fundamental programming paradigm based on the concept of " objects ". These objects can contain data in the form of fields (often known as attributes or properties) and code in the form of procedures (often known as methods). The core concept of the object-oriented approach is to break complex problems ...
This type of work takes up to fourteen days. We will consider any offers from customers and advise the ideal option, with the help of which we will competently organize the work and get the final result even better than we expected. High Priority Status. 77.
Write A Case Study On Public Static Void Main( 250 Words) - 100% Success rate 1524 Orders prepared. ID 8764. 13 Customer ... REVIEWS HIRE. Benny. Write A Case Study On Public Static Void Main( 250 Words) Connect with the writers. Once paid, the initial draft will be made. For any query r to ask for revision, you can get in touch with the online ...
The method main must be declared public, static, and void. It must specify a formal parameter (§8.4.1) whose declared type is array of String. it must be public otherwise it would not be possible to call it. it must be static since you have no way to instantiate an object before calling it. the list of String arguments is there to allow to ...
Write A Case Study On Public Static Void Main ( 250 Words) Hire a Writer. The shortest time frame in which our writers can complete your order is 6 hours. Length and the complexity of your "write my essay" order are determining factors. If you have a lengthy task, place your order in advance + you get a discount!
Choose the formatting style for your paper (MLA, APA, Chicago/Turabian, or Harvard), and we will make all of your footnotes, running heads, and quotations shine. Our professional essay writer can help you with any type of assignment, whether it is an essay, research paper, term paper, biography, dissertation, review, course work, or any other ...