JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
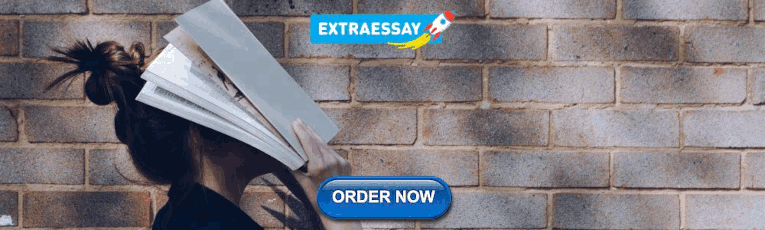
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Logical operators
There are four logical operators in JavaScript: || (OR), && (AND), ! (NOT), ?? (Nullish Coalescing). Here we cover the first three, the ?? operator is in the next article.
Although they are called “logical”, they can be applied to values of any type, not only boolean. Their result can also be of any type.
Let’s see the details.
The “OR” operator is represented with two vertical line symbols:
In classical programming, the logical OR is meant to manipulate boolean values only. If any of its arguments are true , it returns true , otherwise it returns false .
In JavaScript, the operator is a little bit trickier and more powerful. But first, let’s see what happens with boolean values.
There are four possible logical combinations:
As we can see, the result is always true except for the case when both operands are false .
If an operand is not a boolean, it’s converted to a boolean for the evaluation.
For instance, the number 1 is treated as true , the number 0 as false :
Most of the time, OR || is used in an if statement to test if any of the given conditions is true .
For example:
We can pass more conditions:
OR "||" finds the first truthy value
The logic described above is somewhat classical. Now, let’s bring in the “extra” features of JavaScript.
The extended algorithm works as follows.
Given multiple OR’ed values:
The OR || operator does the following:
- Evaluates operands from left to right.
- For each operand, converts it to boolean. If the result is true , stops and returns the original value of that operand.
- If all operands have been evaluated (i.e. all were false ), returns the last operand.
A value is returned in its original form, without the conversion.
In other words, a chain of OR || returns the first truthy value or the last one if no truthy value is found.
For instance:
This leads to some interesting usage compared to a “pure, classical, boolean-only OR”.
Getting the first truthy value from a list of variables or expressions.
For instance, we have firstName , lastName and nickName variables, all optional (i.e. can be undefined or have falsy values).
Let’s use OR || to choose the one that has the data and show it (or "Anonymous" if nothing set):
If all variables were falsy, "Anonymous" would show up.
Short-circuit evaluation.
Another feature of OR || operator is the so-called “short-circuit” evaluation.
It means that || processes its arguments until the first truthy value is reached, and then the value is returned immediately, without even touching the other argument.
The importance of this feature becomes obvious if an operand isn’t just a value, but an expression with a side effect, such as a variable assignment or a function call.
In the example below, only the second message is printed:
In the first line, the OR || operator stops the evaluation immediately upon seeing true , so the alert isn’t run.
Sometimes, people use this feature to execute commands only if the condition on the left part is falsy.
&& (AND)
The AND operator is represented with two ampersands && :
In classical programming, AND returns true if both operands are truthy and false otherwise:
An example with if :
Just as with OR, any value is allowed as an operand of AND:
AND “&&” finds the first falsy value
Given multiple AND’ed values:
The AND && operator does the following:
- For each operand, converts it to a boolean. If the result is false , stops and returns the original value of that operand.
- If all operands have been evaluated (i.e. all were truthy), returns the last operand.
In other words, AND returns the first falsy value or the last value if none were found.
The rules above are similar to OR. The difference is that AND returns the first falsy value while OR returns the first truthy one.
We can also pass several values in a row. See how the first falsy one is returned:
When all values are truthy, the last value is returned:
The precedence of AND && operator is higher than OR || .
So the code a && b || c && d is essentially the same as if the && expressions were in parentheses: (a && b) || (c && d) .
Sometimes, people use the AND && operator as a "shorter way to write if ".
The action in the right part of && would execute only if the evaluation reaches it. That is, only if (x > 0) is true.
So we basically have an analogue for:
Although, the variant with && appears shorter, if is more obvious and tends to be a little bit more readable. So we recommend using every construct for its purpose: use if if we want if and use && if we want AND.
The boolean NOT operator is represented with an exclamation sign ! .
The syntax is pretty simple:
The operator accepts a single argument and does the following:
- Converts the operand to boolean type: true/false .
- Returns the inverse value.
A double NOT !! is sometimes used for converting a value to boolean type:
That is, the first NOT converts the value to boolean and returns the inverse, and the second NOT inverses it again. In the end, we have a plain value-to-boolean conversion.
There’s a little more verbose way to do the same thing – a built-in Boolean function:
The precedence of NOT ! is the highest of all logical operators, so it always executes first, before && or || .
What's the result of OR?
What is the code below going to output?
The answer is 2 , that’s the first truthy value.
What's the result of OR'ed alerts?
What will the code below output?
The answer: first 1 , then 2 .
The call to alert does not return a value. Or, in other words, it returns undefined .
- The first OR || evaluates its left operand alert(1) . That shows the first message with 1 .
- The alert returns undefined , so OR goes on to the second operand searching for a truthy value.
- The second operand 2 is truthy, so the execution is halted, 2 is returned and then shown by the outer alert.
There will be no 3 , because the evaluation does not reach alert(3) .
What is the result of AND?
What is this code going to show?
The answer: null , because it’s the first falsy value from the list.
What is the result of AND'ed alerts?
What will this code show?
The answer: 1 , and then undefined .
The call to alert returns undefined (it just shows a message, so there’s no meaningful return).
Because of that, && evaluates the left operand (outputs 1 ), and immediately stops, because undefined is a falsy value. And && looks for a falsy value and returns it, so it’s done.
The result of OR AND OR
What will the result be?
The answer: 3 .
The precedence of AND && is higher than || , so it executes first.
The result of 2 && 3 = 3 , so the expression becomes:
Now the result is the first truthy value: 3 .
Check the range between
Write an if condition to check that age is between 14 and 90 inclusively.
“Inclusively” means that age can reach the edges 14 or 90 .
Check the range outside
Write an if condition to check that age is NOT between 14 and 90 inclusively.
Create two variants: the first one using NOT ! , the second one – without it.
The first variant:
The second variant:
A question about "if"
Which of these alert s are going to execute?
What will the results of the expressions be inside if(...) ?
The answer: the first and the third will execute.
Check the login
Write the code which asks for a login with prompt .
If the visitor enters "Admin" , then prompt for a password, if the input is an empty line or Esc – show “Canceled”, if it’s another string – then show “I don’t know you”.
The password is checked as follows:
- If it equals “TheMaster”, then show “Welcome!”,
- Another string – show “Wrong password”,
- For an empty string or cancelled input, show “Canceled”
The schema:
Please use nested if blocks. Mind the overall readability of the code.
Hint: passing an empty input to a prompt returns an empty string '' . Pressing ESC during a prompt returns null .
Run the demo
Note the vertical indents inside the if blocks. They are technically not required, but make the code more readable.
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Home » JavaScript Tutorial » An Introduction to JavaScript Logical Operators
An Introduction to JavaScript Logical Operators
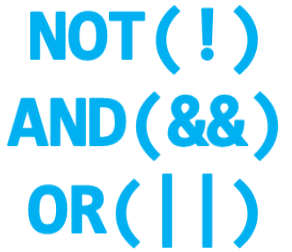
Summary : in this tutorial, you will learn how to use the JavaScript logical operators including the logical NOT operator( ! ), the logical AND operator ( && ) and the logical OR operator ( || ).
The logical operators are important in JavaScript because they allow you to compare variables and do something based on the result of that comparison.
For example, if the result of the comparison is true , you can run a block of code; if it’s false , you can execute another code block.
JavaScript provides three logical operators:
- ! (Logical NOT)
- || (Logical OR)
- && (Logical AND)
1) The Logical NOT operator (!)
JavaScript uses an exclamation point ! to represent the logical NOT operator. The ! operator can be applied to a single value of any type, not just a Boolean value.
When you apply the ! operator to a boolean value, the ! returns true if the value is false and vice versa. For example:
In this example, the eligible is true so !eligible returns false . And since the required is true , the !required returns false .
When you apply the ! operator to a non-Boolean value. The ! operator first converts the value to a boolean value and then negates it.
The following example shows how to use the ! operator:
The logical ! operator works based on the following rules:
- If a is undefined , the result is true .
- If a is null , the result is true .
- If a is a number other than 0 , the result is false .
- If a is NaN , the result is true .
- If a is an object, the result is false.
- If a is an empty string, the result is true. In the case a is a non-empty string, the result is false
The following demonstrates the results of the logical ! operator when applying to a non-boolean value:
Double-negation ( !! )
Sometimes, you may see the double negation ( !! ) in the code. The !! uses the logical NOT operator ( ! ) twice to convert a value to its real boolean value.
The result is the same as using the Boolean() function. For example:
The first ! operator negates the Boolean value of the counter variable. If the counter is true , then the ! operator makes it false and vice versa.
The second ! operator negates that result of the first ! operator and returns the real boolean value of the counter variable.
2) The Logical AND operator ( && )
JavaScript uses the double ampersand ( && ) to represent the logical AND operator. The following expression uses the && operator:
If a can be converted to true , the && operator returns the b ; otherwise, it returns the a . In fact, this rule is applied to all boolean values.
The following truth table illustrates the result of the && operator when it is applied to two Boolean values:
The result of the && operator is true only if both values are true , otherwise, it is false . For example:
In this example, the eligible is false , therefore, the value of the expression eligible && required is false .
See the following example:
In this example, both eligible and required are true , therefore, the value of the expression eligible && required is true .
Short-circuit evaluation
The && operator is short-circuited. It means that the && operator evaluates the second value only if the first one doesn’t suffice to determine the value of an expression. For example:
In this example, b is true therefore the && operator could not determine the result without further evaluating the second expression ( 1/0 ).
The result is Infinity which is the result of the expression ( 1/0 ). However:
In this case, b is false , the && operator doesn’t need to evaluate the second expression because it can determine the final result as false based value of the first value.
The chain of && operators
The following expression uses multiple && operators:
The && operator carries the following:
- Evaluates values from left to right.
- For each value, convert it to a boolean. If the result is false , stops and returns the original value.
- If all values are truthy values, return the last value.
In other words, The && operator returns the first falsy value or the last value if none were found.
If a value can be converted to true , it is so-called a truthy value. If a value can be converted to false , it is a so-called falsy value.
3) The Logical OR operator ( || )
JavaScript uses the double pipe || to represent the logical OR operator. You can apply the || operator to two values of any type:
If a can be converted to true , returns a ; else, returns b . This rule is also applied to boolean values.
The following truth table illustrates the result of the || operator based on the value of the operands:
The || operator returns false if both values evaluate to false . In case either value is true , the || operator returns true . For example:
See another example:
In this example, the expression eligible || required returns false because both values are false .
The || operator is also short-circuited
Similar to the && operator, the || operator is short-circuited. It means that if the first value evaluates to true , the && operator doesn’t evaluate the second one.
The chain of || operators
The following example shows how to use multiple || operators in an expression:
The || operator does the following:
- For each value, converts it to a boolean value. If the result of the conversion is true , stops and returns the value.
- If all values have been evaluated to false , returns the last value.
In other words, the chain of the || operators returns the first truthy value or the last one if no truthy value was found.
Logical operator precedence
When you mix logical operators in an expression, the JavaScript engine evaluates the operators based on a specified order. And this order is called the operator precedence .
In other words, the operator precedence is the order of evaluation of logical operators in an expression.
The precedence of the logical operator is in the following order from the highest to the lowest:
- Logical NOT (!)
- Logical AND (&&)
- Logical OR (||)
- The NOT operator ( ! ) negates a boolean value. The ( !! ) converts a value into its real boolean value.
- The AND operator ( && ) is applied to two Boolean values and returns true if both values are true.
- The OR operator ( || ) is applied to two Boolean values and returns true if one of the operands is true .
- Both && and || operator are short-circuited. They can be also applied to non-Boolean values.
- The logical operator precedence from the highest to the lowest is ! , && and || .
How to Use Logic in JavaScript – Operators, Conditions, Truthy/Falsy, and More

JavaScript is a versatile programming language that empowers developers to create dynamic and interactive web pages.
One of the foundational elements of JavaScript programming is the application of logical operations to make decisions and control program flow.
In this guide, we'll delve into the basics of logical operations in JavaScript. I'll provide simple explanations and ample code snippets to make these concepts easier to understand.
Understanding Logical Operators
Logical operators in JavaScript let developers perform operations on values or expressions, playing a crucial role in effective decision-making within code.
The primary logical operators are && (AND), || (OR), and ! (NOT). Let's look at each one now.
1. AND ( && ) Operator
The AND ( && ) operator in JavaScript is a logical operator that combines two or more conditions. It returns true only if all the conditions being evaluated are true . If any of the conditions is false , the entire expression evaluates to false .
In this example, the isSunny && isWarm condition must be true for the message about perfect weather to be displayed. If either isSunny or isWarm is false , the else block is executed.
Let's look at some scenarios when the AND operator is particularly useful.
When combining conditions: Use && when you want an action to be taken only when multiple conditions are met simultaneously.
In guard clauses: Use && in guard clauses to ensure that certain conditions are met before proceeding with further code execution.
For form validation: In scenarios like form validation, you might use && to check multiple conditions before allowing form submission.
The AND operator is useful when you want to ensure that all specified conditions are true before proceeding with a particular action or decision in your code. It's a fundamental tool for creating more nuanced and context-specific logic in your JavaScript programs.
2. OR ( || ) Operator
The OR ( || ) operator in JavaScript is a logical operator that returns true if at least one of the conditions it connects is true . It is often used when you want an action to occur if any one of multiple conditions is met.
Here's a basic example to illustrate the OR operator:
In this example, the hasCoffee || hasTea condition is true because hasCoffee is true . As a result, the message "You can enjoy a hot beverage!" will be logged.
Here are some scenarios where you might want to use the OR operator:
Fallback Values:
In this case, if the user didn't provide a username ( userInput is an empty string), the OR operator assigns a default value of "Guest" to username . This is a common pattern for providing fallback or default values.
Checking for Multiple Conditions:
This example uses the OR operator to check if it's either the weekend or a holiday, indicating that it's time for a break.
Form Validation:
Here, the OR operator can be used to check if either the username or password is missing. If either condition is true , it prompts the user to fill in both fields.
The OR operator is useful when you want an action to occur if at least one of the specified conditions is true. It's commonly employed in scenarios involving fallback values, checking multiple conditions, or form validation where any of several fields need to be filled.
3. NOT ( ! ) Operator
The NOT ( ! ) operator in JavaScript is a unary operator that negates the truthiness of a value. It's used to invert a boolean value or a truthy/falsy expression. In simpler terms, it turns true into false and false into true . Here's how it works:
Now, let's discuss when you might want to use the NOT operator:
Checking for Negation: The most straightforward use of the NOT operator is when you want to check for the negation of a condition. For example:
In this case, the !isRaining condition is true when it's not raining. It provides a concise way of expressing the idea that it's a good day when it's not raining.
Checking for Falsy Values: The NOT operator is often used to check if a value is falsy. Remember that in JavaScript, certain values are considered falsy, such as false , 0 , null , undefined , NaN , and an empty string "" . The NOT operator can be handy for checking whether a variable holds a falsy value:
In this example, if userRole is null (a falsy value), the condition !userRole evaluates to true , and a default role is assigned.
Creating Clearer Conditions: The NOT operator can also be used to make conditions more explicit or readable. For instance:
This condition checks if the user is not logged in and takes action accordingly.
The NOT operator is useful when you need to negate a boolean value or check for falsy values, providing a concise and readable way to express conditions in your JavaScript code.
How to Combine Logical Operators
You can combine logical operators to create more complex conditions, introducing parentheses to control the order of evaluation.
Let's consider an example where we want to determine if a person is eligible to enter a club based on their age and whether they have a valid ID. The conditions for entry are as follows:
- The person must be at least 18 years old.
- If the person is between 16 and 18 years old, they can enter only if they have a valid ID.
- If the person is under 16, entry is not allowed.
Here's the JavaScript code for this scenario:
In this code:
- age is set to 17 , indicating that the person is 17 years old.
- hasValidID is set to false , indicating that the person does not have a valid ID.
Now, let's evaluate the condition within the if statement:
- (age >= 18) evaluates to false because the person is not 18 or older.
- (age >= 16 && hasValidID) evaluates to true && false , which is false . This is because the person is 17, which satisfies the first part of the condition, but they don't have a valid ID.
Since both parts of the condition are false , the code block inside the else statement is executed, resulting in the output:
This example demonstrates how logical operators can be combined to create complex conditions, allowing you to control the flow of your program based on various factors.
Conditional Statements
Logical operators are frequently employed in conditional statements ( if , else if , and else ) to dictate program flow based on specific conditions.
1. if Statement:
The if statement in JavaScript is used to execute a block of code if a specified condition is true. Logical operators often play a crucial role in defining these conditions.
In this example, the && (AND) operator combines two conditions ( isHungry and hasFood ). The block of code inside the if statement will execute only if both conditions are true. If either isHungry or hasFood is false, the code inside the else block will run.
2. else Statement:
The else statement is paired with the if statement to execute a block of code when the specified condition is false.
Here, the if statement checks if isNight is true. If it is, the corresponding message is printed. If isNight is false, the else block is executed, providing an alternative message for daytime.
3. else if Statement:
The else if statement accommodates multiple conditions, allowing for more complex decision-making.
In this case, the code greets users differently based on the value of timeOfDay . The === operator is used for strict equality comparison, and logical operators like && or || can be incorporated to form more intricate conditions.
These examples illustrate how logical operators are employed within if , else , and else if statements to control the flow of a JavaScript program based on specific conditions.
Ternary Operator
The ternary operator, often denoted by ? : , provides a concise way to express conditional statements. It's a shorthand version of an if-else statement. The basic syntax is:
Here's a breakdown of the components:
- condition : a boolean expression that is evaluated. If it is true, the expression before the : is executed – otherwise, the expression after the : is executed.
- expression_if_true : the value or expression returned if the condition is true.
- expression_if_false : the value or expression returned if the condition is false.
Now, let's take a closer look at the example provided:
In this example:
- isSunny is the condition being checked. If isSunny is true, the value of the entire expression becomes "Enjoy the sunshine!". If isSunny is false, the value becomes "Grab an umbrella!".
- The ? is like asking a question: "Is it sunny?" If the answer is yes, then "Enjoy the sunshine!" is the response (before the : ). If the answer is no, then "Grab an umbrella!" is the response (after the : ).
This can be seen as a shorthand way of writing an if-else statement. The equivalent if-else statement for the example would be:
Both the ternary operator and the if-else statement achieve the same result, but the ternary operator is more concise and is often used for simple conditional assignments.
It's important to note that using the ternary operator excessively or in complex scenarios can reduce code readability, so it's best used for straightforward conditions.
Switch Statement
The switch statement handles multiple conditions efficiently, particularly when there are several possible values for a variable. Extending our day-of-week example:
Here, the switch statement triggers the appropriate message based on the day of the week.
Short-Circuit Evaluation
JavaScript leverages short-circuit evaluation with logical operators, optimizing performance by halting evaluation once the result is determined.
Example 1: Short-Circuit with && Operator
In this example, someFunction() is only called if isTrue is true, showcasing the efficiency of short-circuit evaluation.
Example 2: Short-Circuit with || Operator
Here, username is assigned the default value "Guest" only if the user is not logged in, thanks to short-circuit evaluation.
Truthy and Falsy Values
In JavaScript, logical operators can be used with non-boolean values. Understanding truthy and falsy values is crucial in such scenarios.
Truthy and Falsy Values Overview
Every value in JavaScript has inherent truthiness or falsiness. Falsy values include false , 0 , null , undefined , NaN , and an empty string ( "" ). Truthy values encompass all values not explicitly falsy.
Example: Truthy and Falsy Values
Here, the default value "User" is assigned to roleMessage only if userRole is falsy.
Summary Table
Let's provide a quick reference for the different logical operators:
Practical Applications
Logical operators play a crucial role in real-world JavaScript applications. Here are some practical examples:
Form Validation
In this scenario, the form submission is validated by ensuring both the username and password are provided.
Responsive UI
Logical operators are often used to determine the layout based on the screen width, creating a responsive user interface.
Access Control
Logical operators help control access by verifying both the user role and login status.
Mastering logical operators is integral to writing effective and meaningful JavaScript code. Whether you're creating conditions, making decisions, or controlling program flow, logical operators are essential tools.
By exploring these concepts through numerous examples, you're well-equipped to apply them in your projects. Additionally, understanding truthy and falsy values enhances your ability to work with non-boolean contexts.
Use this guide as a foundation for writing clear and concise JavaScript, and you'll be on your way to building robust and responsive web applications. Happy coding!
frontend developer || technical writer
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
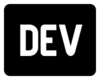
DEV Community

Posted on Feb 6, 2022
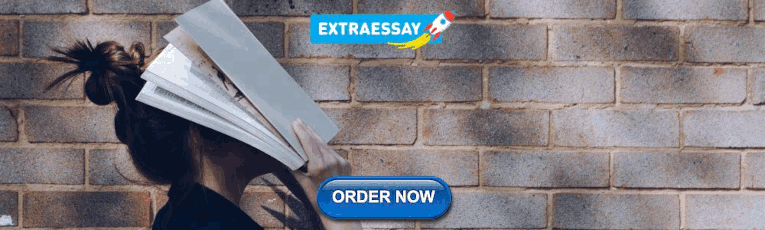
What you should know about the Logical Assignment Operators in JavaScript
Hello World, every year, new features get added to the JavaScript language.This enables developers write better code which translates into awesome products.
In 2021, the Logical assignment operators were added to the language. Its main objective is to assign values to variables. In this post, we will learn how to effectively utilize the logical assignment operators. Let's get started.
The Logical assignment operators
ES2021 introduced three logical assignment operators:
- Logical OR assignment operator ( ||= )
- Logical AND assignment operator ( &&= )
- Nullish coalesing assignment operator ( ??= )
The Logical assignment operators combine the logical operators and assignment expression. If you have forgotten what Logical operators are, here is my post on Logical Operators to help refresh your mind.
Alright, now let's discuss the Logical OR assignment operator ( ||= )
What is the logical OR assignment operator
The logical OR assignment operator (||=) accepts two operands and assigns the right operand to the left operand if the left operand is falsy
The syntax is as below
In the syntax, the ||= will only assign the value of y to x if x is falsy.
Let's take a look at the || operator first. The Logical OR operator || returns the * first * truthy value in an expression.
Consider an example below
The output will be Guest .
- In the example above, the firstName variable is an empty string "" , and the noName variable is a string ( a string stores a sequence of characters).
- Empty strings "" are considered falsy values while non-empty strings(eg. "emma" ) are truthy values.
- Because the || operator returns the first truthy value , the value stored in the noName variable (ie. Guest ) will logged to the console.
Note that : 0 , null , undefined , NaN and "" are classified as falsy values.
Assigning a value to a variable using the ||= operator.
Consider a situation where you want to assign a truthy value to a variable storing a falsy value
Let's see how you can achieve that using the logical OR assignment operator ( ||= )
You can do this (long method)
Let's understand the code above
- The expression on the right : firstName || noName is evaluated first.
- Since the || operator returns the first truthy value, it will return the value Guest
- Using the = (assignment operator), the value Guest is then assigned to the firstName variable which has a falsy value
- Now, anytime we console.log(firstName) , we get the value Guest
The example above can be simplified using the logical OR assignment operator ( ||= ).
The output will be
-The truthy value of 28 will be assigned to the age variable which has a falsy value
- The age now has a value of 28
You can also assign a truthy value to a property in an object if the property is falsy . Take a look at the code below
In the example above
- The || operator evaluates the expression and returns the firsty truthy value ( "emma" )
- The truthy value is now assigned to the userDetails.username property since userDetails.username is falsy
If the first operand is a truthy value, the logical OR assignment operator ( ||= ) will** not assign the value of the second operand to the first. **
Consider the code below
- Because the userDetails.userName property is truthy , the second operand was not evaluated
In summary, the x ||= y will assign the value of y to x if x is falsy .
Using the Logical AND assignment operator ( &&= )
Sometimes you may want to assign a value to a variable even if the initial variable has a value. This is where the logical AND assignment operator ( &&= ) comes in.
What is the logical AND assignment operator ?
The logical AND assignment operator only assigns y to x if x is truthy
* The syntax is as below *
- if the operand on the left side is truthy , the value of y is then assigned to x
Let's see how this was done previously
The output will be efk .
- The if evaluates the condition in the parenthesis ()
- If the condition is true then the expression inside the curly braces {} gets evaluated
- Because the firstName is truthy , we assign the value of userName to firstName .
Using &&= in the same example as above
- Because firstName is a truthy value, the value of userName is now assigned to firstName
The &&= operator is very useful for changing values. Consider the example below
- userDetails.lastName is a truthy value hence the right operand Fordjour is assigned to it.
In the code below, we given an object, and our task is to change the id to a random number between 1 and 10.
Let's see how that can be done using the &&=
The output will vary depending on the random number returned, here is an example.
In summary, the &&= operator assigns the value of the right operand to the left operand if the left operand is truthy
The nullish coalescing assignment operator ( ??= )
The nullish coalescing assignment operator only assigns y to x if x is null or undefined .
Let's see how to use the nullish coalescing assignment operator
- The firstName variable is undefined
- We now assign the value of the right operand to firstName
- firstName now has the value of Emmanuel .
Adding a missing property to an object
- The userDetails.userName is undefined hence nullish
- The nullish coalescing assignment operator ??= then assigns the string Guest to the userDetails.userName
- Now the userDetails object has property userName .
- The logical OR assignment (x ||= y) operator only assigns y to x if x is falsy .
- The logical AND assignment (x &&=y) operator will assign y to x if x is truthy
- The nullish coalescing assignment operator will assign y to x if x is undefined or null .
I trust you have learnt something valuable to add to your coding repository. Is there anything that wasn't clear to you ? I would love to read your feedback on the article.
Writing with love from Ghana. Me daa se (Thank you)
Top comments (0)
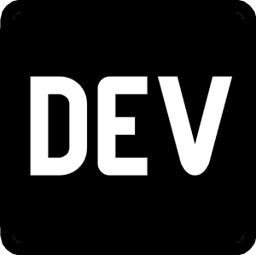
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
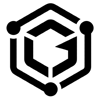
GraphQL Request Cancellation in JavaScript
TheGuildBot - Apr 29

Fixed No Firebase App '[DEFAULT]' has been created - call firebase.initializeApp() In React Native
Bug Blitz - Apr 29
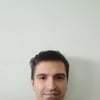
Console Ninja - Your logs on steroids
Giuliano1993 - Apr 29

🎨 Logo Hunter - Netlify Dynamic Site Challenge
Neo Tang - May 12
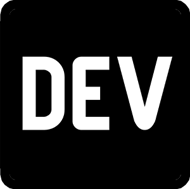
We're a place where coders share, stay up-to-date and grow their careers.
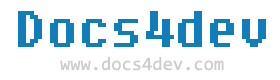
- Array.@@iterator
- Array.@@species
- array.@@unscopables
- Array.array
- array.concat
- Array.copyWithin
- array.entries
- array.every
- array.filter
- Array.findIndex
- Array.flatMap
- Array.forEach
- Array.groupBy
- Array.groupByToMap
- array.includes
- Array.indexOf
- Array.isArray
- Array.lastIndexOf
- array.length
- Array.reduce
- Array.reduceRight
- array.reverse
- array.shift
- array.slice
- array.splice
- Array.toLocaleString
- Array.toSource
- Array.toString
- array.unshift
- array.values
- ArrayBuffer
- ArrayBuffer.@@species
- ArrayBuffer.arrayBuffer
- ArrayBuffer.byteLength
- ArrayBuffer.isView
- arrayBuffer.slice
- Atomics.add
- Atomics.and
- Atomics.compareExchange
- Atomics.exchange
- Atomics.isLockFree
- Atomics.load
- Atomics.notify
- Atomics.store
- Atomics.sub
- Atomics.wait
- Atomics.xor
- BigInt.asIntN
- BigInt.asUintN
- BigInt.bigInt
- BigInt.toLocaleString
- BigInt.toString
- BigInt.valueOf
- BigInt64Array.bigInt64Array
- BigUint64Array.bigUint64Array
- Boolean.boolean
- Boolean.toSource
- Boolean.toString
- Boolean.valueOf
- Class static initialization blocks
- constructor
- Private class fields
- Public class fields
- dataView.buffer
- DataView.byteLength
- DataView.byteOffset
- DataView.dataView
- DataView.getBigInt64
- DataView.getBigUint64
- DataView.getFloat32
- DataView.getFloat64
- DataView.getInt16
- DataView.getInt32
- DataView.getInt8
- DataView.getUint16
- DataView.getUint32
- DataView.getUint8
- DataView.setBigInt64
- DataView.setBigUint64
- DataView.setFloat32
- DataView.setFloat64
- DataView.setInt16
- DataView.setInt32
- DataView.setInt8
- DataView.setUint16
- DataView.setUint32
- DataView.setUint8
- Date.@@toPrimitive
- Date.getDate
- Date.getDay
- Date.getFullYear
- Date.getHours
- Date.getMilliseconds
- Date.getMinutes
- Date.getMonth
- Date.getSeconds
- Date.getTime
- Date.getTimezoneOffset
- Date.getUTCDate
- Date.getUTCDay
- Date.getUTCFullYear
- Date.getUTCHours
- Date.getUTCMilliseconds
- Date.getUTCMinutes
- Date.getUTCMonth
- Date.getUTCSeconds
- Date.getYear
- Date.setDate
- Date.setFullYear
- Date.setHours
- Date.setMilliseconds
- Date.setMinutes
- Date.setMonth
- Date.setSeconds
- Date.setTime
- Date.setUTCDate
- Date.setUTCFullYear
- Date.setUTCHours
- Date.setUTCMilliseconds
- Date.setUTCMinutes
- Date.setUTCMonth
- Date.setUTCSeconds
- Date.setYear
- Date.toDateString
- Date.toGMTString
- Date.toISOString
- Date.toJSON
- Date.toLocaleDateString
- Date.toLocaleString
- Date.toLocaleTimeString
- Date.toSource
- Date.toString
- Date.toTimeString
- Date.toUTCString
- Date.valueOf
- AggregateError
- AggregateError.aggregateError
- Error.columnNumber
- Error.error
- Error.fileName
- Error.lineNumber
- error.message
- Error.stack
- Error.toSource
- Error.toString
- Errors: Already has pragma
- Errors: Array sort argument
- Errors: Bad octal
- Errors: Bad radix
- Errors: Bad regexp flag
- Errors: Bad return or yield
- Errors: Called on incompatible type
- Errors: Cant access lexical declaration before init
- Errors: Cant access property
- Errors: Cant assign to property
- Errors: Cant define property object not extensible
- Errors: Cant delete
- Errors: Cant redefine property
- Errors: Cyclic object value
- Errors: Dead object
- Errors: Delete in strict mode
- Errors: Deprecated caller or arguments usage
- Errors: Deprecated expression closures
- Errors: Deprecated octal
- Errors: Deprecated source map pragma
- Errors: Deprecated String generics
- Errors: Deprecated toLocaleFormat
- Errors: Equal as assign
- Errors: Getter only
- Errors: Hash outside class
- Errors: Identifier after number
- Errors: Illegal character
- Errors: in operator no object
- Errors: Invalid array length
- Errors: Invalid assignment left-hand side
- Errors: Invalid const assignment
- Errors: Invalid date
- Errors: Invalid for-in initializer
- Errors: Invalid for-of initializer
- Errors: invalid right hand side instanceof operand
- Errors: is not iterable
- Errors: JSON bad parse
- Errors: Malformed formal parameter
- Errors: Malformed URI
- Errors: Missing bracket after list
- Errors: Missing colon after property id
- Errors: Missing curly after function body
- Errors: Missing curly after property list
- Errors: Missing formal parameter
- Errors: Missing initializer in const
- Errors: Missing name after dot operator
- Errors: Missing parenthesis after argument list
- Errors: Missing parenthesis after condition
- Errors: Missing semicolon before statement
- Errors: More arguments needed
- Errors: Negative repetition count
- Errors: No non-null object
- Errors: No properties
- Errors: No variable name
- Errors: Non configurable array element
- Errors: Not a codepoint
- Errors: Not a constructor
- Errors: Not a function
- Errors: Not defined
- Errors: Precision range
- Errors: Property access denied
- Errors: Read-only
- Errors: Redeclared parameter
- Errors: Reduce of empty array with no initial value
- Errors: Reserved identifier
- Errors: Resulting string too large
- Errors: Stmt after return
- Errors: Strict Non Simple Params
- Errors: Too much recursion
- Errors: Undeclared var
- Errors: Undefined prop
- Errors: Unexpected token
- Errors: Unexpected type
- Errors: Unnamed function statement
- Errors: Unterminated string literal
- EvalError.evalError
- InternalError
- InternalError.internalError
- RangeError.rangeError
- ReferenceError
- ReferenceError.referenceError
- SyntaxError
- SyntaxError.syntaxError
- TypeError.typeError
- URIError.uRIError
- FinalizationRegistry.finalizationRegistry
- finalizationRegistry.register
- finalizationRegistry.unregister
- Float32Array.float32Array
- Float64Array.float64Array
- arguments.@@iterator
- arguments.callee
- arguments.length
- Arrow functions
- AsyncFunction
- Default parameters
- function.apply
- function.arguments
- function.bind
- function.call
- function.caller
- function.displayName
- Function.function
- Function.length
- Function.name
- Function.toSource
- function.toString
- GeneratorFunction
- Method definitions
- rest parameters
- generator.next
- generator.return
- generator.throw
- BigInt64Array
- BigUint64Array
- decodeURIComponent
- encodeURIComponent
- FinalizationRegistry
- Float32Array
- Float64Array
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- Int16Array.int16Array
- Int32Array.int32Array
- Int8Array.int8Array
- Intl.collator
- Intl.collator.Collator
- Intl.collator.compare
- Intl.collator.resolvedOptions
- Intl.collator.supportedLocalesOf
- Intl.dateTimeFormat
- Intl.dateTimeFormat.DateTimeFormat
- Intl.dateTimeFormat.format
- Intl.dateTimeFormat.formatRange
- Intl.dateTimeFormat.formatRangeToParts
- Intl.dateTimeFormat.formatToParts
- Intl.dateTimeFormat.resolvedOptions
- Intl.dateTimeFormat.supportedLocalesOf
- Intl.displayNames
- Intl.displayNames.DisplayNames
- Intl.displayNames.of
- Intl.displayNames.resolvedOptions
- Intl.displayNames.supportedLocalesOf
- Intl.getCanonicalLocales
- Intl.listFormat
- Intl.listFormat.format
- Intl.listFormat.formatToParts
- Intl.listFormat.ListFormat
- Intl.listFormat.supportedLocalesOf
- Intl.locale
- Intl.locale.baseName
- Intl.locale.calendar
- Intl.locale.calendars
- Intl.locale.caseFirst
- Intl.locale.collation
- Intl.locale.hourCycle
- Intl.locale.hourCycles
- Intl.locale.language
- Intl.locale.Locale
- Intl.locale.maximize
- Intl.locale.minimize
- Intl.locale.numberingSystem
- Intl.locale.numberingSystems
- Intl.locale.numeric
- Intl.locale.region
- Intl.locale.script
- Intl.locale.textInfo
- Intl.locale.timeZones
- Intl.locale.toString
- Intl.locale.weekInfo
- Intl.numberFormat
- Intl.numberFormat.format
- Intl.numberFormat.formatRange
- Intl.numberFormat.formatRangeToParts
- Intl.numberFormat.formatToParts
- Intl.numberFormat.NumberFormat
- Intl.numberFormat.resolvedOptions
- Intl.numberFormat.supportedLocalesOf
- Intl.pluralRules
- Intl.pluralRules.PluralRules
- Intl.pluralRules.resolvedOptions
- Intl.pluralRules.select
- Intl.pluralRules.selectRange
- Intl.pluralRules.supportedLocalesOf
- Intl.relativeTimeFormat
- Intl.relativeTimeFormat.format
- Intl.relativeTimeFormat.formatToParts
- Intl.relativeTimeFormat.RelativeTimeFormat
- Intl.relativeTimeFormat.resolvedOptions
- Intl.relativeTimeFormat.supportedLocalesOf
- Intl.segmenter
- Intl.segmenter.resolvedOptions
- Intl.segmenter.segment
- Intl.segmenter.Segmenter
- Intl.segmenter.supportedLocalesOf
- Intl.segments
- Intl.segments.@@iterator
- Intl.segments.containing
- Intl.supportedValuesOf
- JSON.stringify
- Map.@@iterator
- Map.@@species
- map.entries
- Map.forEach
- Math.fround
- Math.LOG10E
- Math.random
- Math.SQRT1_2
- Iteration protocols
- Lexical grammar
- Strict mode
- Strict mode: Transitioning to strict mode
- Template literals
- Trailing commas
- Number.EPSILON
- Number.isFinite
- Number.isInteger
- Number.isNaN
- Number.isSafeInteger
- Number.MAX_SAFE_INTEGER
- Number.MAX_VALUE
- Number.MIN_SAFE_INTEGER
- Number.MIN_VALUE
- Number.NEGATIVE_INFINITY
- Number.number
- Number.parseFloat
- Number.parseInt
- Number.POSITIVE_INFINITY
- Number.toExponential
- Number.toFixed
- Number.toLocaleString
- Number.toPrecision
- Number.toSource
- Number.toString
- Number.valueOf
- object.__defineGetter__
- object.__defineSetter__
- object.__lookupGetter__
- object.__lookupSetter__
- Object.assign
- object.constructor
- Object.create
- Object.defineProperties
- Object.defineProperty
- Object.entries
- Object.freeze
- Object.fromEntries
- Object.getOwnPropertyDescriptor
- Object.getOwnPropertyDescriptors
- Object.getOwnPropertyNames
- Object.getOwnPropertySymbols
- Object.getPrototypeOf
- Object.hasOwn
- Object.hasOwnProperty
- Object.isExtensible
- Object.isFrozen
- Object.isPrototypeOf
- Object.isSealed
- Object.keys
- Object.object
- Object.preventExtensions
- Object.propertyIsEnumerable
- Object.proto
- Object.seal
- Object.setPrototypeOf
- Object.toLocaleString
- Object.toSource
- Object.toString
- Object.valueOf
- Object.values
- Addition assignment
- async function
- Bitwise AND
- Bitwise AND assignment
- Bitwise NOT
- Bitwise OR assignment
- Bitwise XOR
- Bitwise XOR assignment
- Comma Operator
- Conditional Operator
- Destructuring assignment
- Division assignment
- Exponentiation
- Exponentiation assignment
- Greater than
- Greater than or equal
- Left shift assignment
- Less than or equal
- Logical AND
- Logical AND assignment
- Logical NOT
- Logical nullish assignment
- Logical OR assignment
- Multiplication
- Multiplication assignment
- Nullish coalescing operator
- Object initializer
- Operator Precedence
- Optional chaining
- Property Accessors
- Remainder assignment
- Right shift
- Right shift assignment
- Spread syntax
- Strict equality
- Strict inequality
- Subtraction
- Subtraction assignment
- Unary negation
- Unsigned right shift
- Unsigned right shift assignment
- Promise.all
- Promise.allSettled
- Promise.any
- promise.catch
- promise.finally
- Promise.promise
- Promise.race
- Promise.reject
- Promise.resolve
- promise.then
- Proxy.proxy
- Proxy.proxy.apply
- Proxy.proxy.construct
- Proxy.proxy.defineProperty
- Proxy.proxy.deleteProperty
- Proxy.proxy.get
- Proxy.proxy.getOwnPropertyDescriptor
- Proxy.proxy.getPrototypeOf
- Proxy.proxy.has
- Proxy.proxy.isExtensible
- Proxy.proxy.ownKeys
- Proxy.proxy.preventExtensions
- Proxy.proxy.set
- Proxy.proxy.setPrototypeOf
- Proxy.revocable
- Reflect.apply
- Reflect.comparing_Reflect_and_Object_methods
- Reflect.construct
- Reflect.defineProperty
- Reflect.deleteProperty
- Reflect.get
- Reflect.getOwnPropertyDescriptor
- Reflect.getPrototypeOf
- Reflect.has
- Reflect.isExtensible
- Reflect.ownKeys
- Reflect.preventExtensions
- Reflect.set
- Reflect.setPrototypeOf
- RegExp.@@match
- RegExp.@@matchAll
- RegExp.@@replace
- RegExp.@@search
- RegExp.@@species
- RegExp.@@split
- regExp.compile
- regExp.dotAll
- RegExp.exec
- regExp.flags
- regExp.global
- regExp.hasIndices
- regExp.ignoreCase
- RegExp.lastIndex
- regExp.multiline
- RegExp.regExp
- regExp.source
- regExp.sticky
- regExp.test
- RegExp.toSource
- RegExp.toString
- regExp.unicode
- Set.@@iterator
- Set.@@species
- set.entries
- Set.forEach
- SharedArrayBuffer
- SharedArrayBuffer.byteLength
- SharedArrayBuffer.planned_changes
- SharedArrayBuffer.sharedArrayBuffer
- sharedArrayBuffer.slice
- for-await...of
- import.meta
- try...catch
- String.@@iterator
- string.anchor
- string.blink
- string.bold
- String.charAt
- String.charCodeAt
- String.codePointAt
- string.concat
- String.endsWith
- string.fixed
- string.fontcolor
- string.fontsize
- String.fromCharCode
- String.fromCodePoint
- string.includes
- String.indexOf
- string.italics
- String.lastIndexOf
- String.length
- string.link
- string.localeCompare
- string.match
- String.matchAll
- string.normalize
- String.padEnd
- String.padStart
- string.repeat
- string.replace
- String.replaceAll
- string.search
- string.slice
- string.small
- string.split
- String.startsWith
- string.strike
- String.string
- string.substr
- string.substring
- String.toLocaleLowerCase
- String.toLocaleUpperCase
- String.toLowerCase
- String.toSource
- String.toString
- String.toUpperCase
- string.trim
- String.trimEnd
- String.trimStart
- String.valueOf
- Symbol.@@toPrimitive
- Symbol.asyncIterator
- symbol.description
- Symbol.hasInstance
- Symbol.isConcatSpreadable
- Symbol.iterator
- Symbol.keyFor
- Symbol.match
- Symbol.matchAll
- Symbol.replace
- Symbol.search
- Symbol.species
- Symbol.split
- Symbol.symbol
- Symbol.toPrimitive
- Symbol.toSource
- Symbol.toString
- Symbol.toStringTag
- Symbol.unscopables
- Symbol.valueOf
- TypedArray.@@iterator
- TypedArray.@@species
- typedArray.at
- typedArray.buffer
- TypedArray.byteLength
- TypedArray.byteOffset
- TypedArray.BYTES_PER_ELEMENT
- TypedArray.copyWithin
- typedArray.entries
- typedArray.every
- typedArray.fill
- typedArray.filter
- typedArray.find
- TypedArray.findIndex
- TypedArray.forEach
- TypedArray.from
- typedArray.includes
- TypedArray.indexOf
- typedArray.join
- typedArray.keys
- TypedArray.lastIndexOf
- typedArray.length
- typedArray.map
- TypedArray.name
- TypedArray.of
- typedArray.reduce
- TypedArray.reduceRight
- typedArray.reverse
- typedArray.set
- typedArray.slice
- typedArray.some
- typedArray.sort
- typedArray.subarray
- TypedArray.toLocaleString
- TypedArray.toString
- typedArray.values
- Uint16Array.uint16Array
- Uint32Array.uint32Array
- Uint8Array.uint8Array
- Uint8ClampedArray.uint8ClampedArray
- weakMap.delete
- weakMap.get
- weakMap.has
- weakMap.set
- WeakMap.weakMap
- weakRef.deref
- WeakRef.weakRef
- weakSet.add
- weakSet.delete
- weakSet.has
- WeakSet.weakSet
- WebAssembly.compile
- WebAssembly.compileError
- WebAssembly.compileError.CompileError
- WebAssembly.compileStreaming
- WebAssembly.global.Global
- WebAssembly.instance
- WebAssembly.instance.exports
- WebAssembly.instance.Instance
- WebAssembly.instantiate
- WebAssembly.instantiateStreaming
- WebAssembly.linkError
- WebAssembly.linkError.LinkError
- WebAssembly.memory
- WebAssembly.memory.buffer
- WebAssembly.memory.grow
- WebAssembly.memory.Memory
- WebAssembly.module
- WebAssembly.module.customSections
- WebAssembly.module.exports
- WebAssembly.module.imports
- WebAssembly.module.Module
- WebAssembly.runtimeError
- WebAssembly.runtimeError.RuntimeError
- WebAssembly.table
- WebAssembly.table.get
- WebAssembly.table.grow
- WebAssembly.table.length
- WebAssembly.table.set
- WebAssembly.table.Table
- WebAssembly.validate
Logical AND assignment (&&=)
The logical AND assignment ( x &&= y ) operator only assigns if x is truthy .
Description
Short-circuit evaluation.
The logical AND operator is evaluated left to right, it is tested for possible short-circuit evaluation using the following rule:
(some falsy expression) && expr is short-circuit evaluated to the falsy expression;
Short circuit means that the expr part above is not evaluated , hence any side effects of doing so do not take effect (e.g., if expr is a function call, the calling never takes place).
Logical AND assignment short-circuits as well meaning that x &&= y is equivalent to:
And not equivalent to the following which would always perform an assignment:
Using logical AND assignment
Specifications, browser compatibility.
- Logical AND (&&)
- The nullish coalescing operator ( ?? )
- Bitwise AND assignment ( &= )
© 2005–2022 MDN contributors. Licensed under the Creative Commons Attribution-ShareAlike License v2.5 or later. https://developer.mozilla.org/en-US/docs/Web/JavaScript/Reference/Operators/Logical_AND_assignment
Logical assignment
Logical assignment operators #, short-circuit semantics #, logical assignment support #.
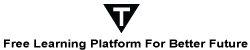
JavaScript Tutorial
Javascript basics, javascript objects, javascript bom, javascript dom, javascript validation, javascript oops, javascript cookies, javascript events, exception handling, javascript misc, javascript advance, differences.
Interview Questions
Logical AND assignment operator (&&=)
The &&= symbol is a "Logical AND assignment operator" and connects two variables. If the initial value is correct, the second value is used. It is graded from left to right.
The following syntax shows the Logical AND assignment operator with the two values.
The examples work with multiple values using the "Logical AND assignment operator" in the javascript.
The following example shows basic values for the "AND assignment operator" in javascript.
The image shows the "logical AND assignment operator's data" as an output.
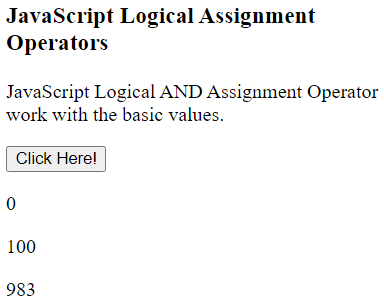
The following example shows the "AND assignment operator" hash in javascript.
The image shows the "logical and assignment operator's data" as an output.
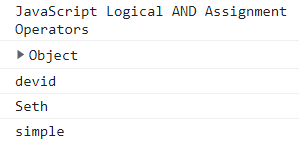
The following example shows an array for the "AND assignment operator" in javascript.
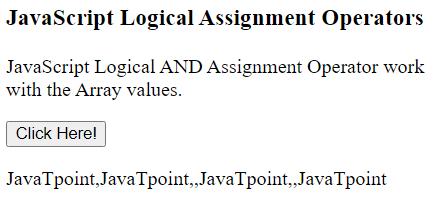
Logical OR assignment operator (||=)
The ||= is a "logical OR assignment operator" between two values. If the initial value is incorrect, the second value is used. It is graded from left to right.
The following syntax shows the Logical OR assignment operator with the two values.
The examples work with multiple values using the " Logical OR assignment operator" in the javascript.
The following example shows basic values for the "Logical OR assignment" in javascript. Here, We can use simple numeric values.
The image shows the "logical OR assignment operator's data" as an output.
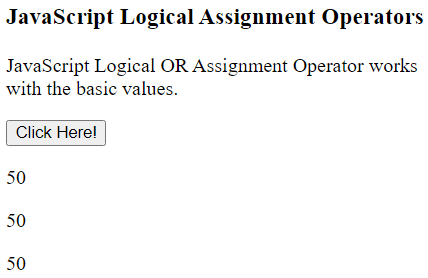
The following example shows the "OR assignment operator" hash in javascript.
The image shows the " Logical OR assignment operator's data" as an output.
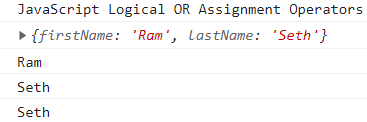
The following example shows arrays for the "OR assignment operator" in javascript.
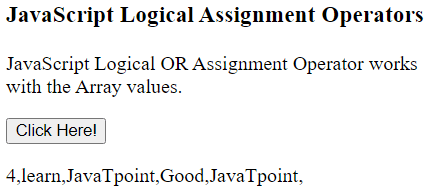
Nullish coalescing assignment operator (??=)
The ??= is a "Nullish coalescing assignment operator" between two values. The second value is assigned if the first value is undefined or null. It is graded from left to right.
The following syntax shows the Logical nullish assignment operator with the two values.
The examples work with multiple values using the " Logical nullish assignment operator" in the javascript.
The following example shows basic values for the "Logical nullish assignment" in javascript. Here, We can use simple numeric values.
The image shows the "logical nullish assignment operator's data" as an output.
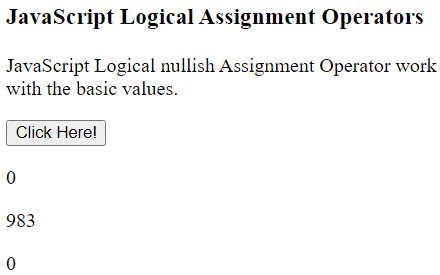
The following example shows hash values for the "Logical nullish assignment" in javascript.
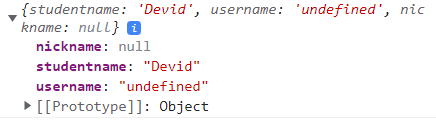
The following example shows array values for the "Logical nullish assignment" in javascript. The nullish operator uses the null and the "jtp" value to show functionality.
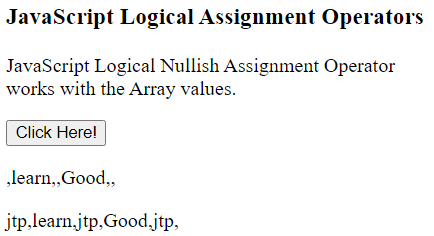
The javascript logical assignment operators help to operate logical conditions between two inputs in all data formats. The "and", "or", and nullish logical operation works by these operators in the javascript language.

- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Skip to main content
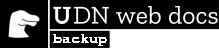
- Logical OR assignment (||=)
The logical OR assignment ( x ||= y ) operator only assigns if x is falsy .
Description
Short-circuit evaluation.
The logical OR operator works like this:
The logical OR operator short-circuits: the second operand is only evaluated if the first operand doesn’t already determine the result.
Logical OR assignment short-circuits as well, meaning it only performs an assignment if the logical operation would evaluate the right-hand side. In other words, x ||= y is equivalent to:
And not equivalent to the following which would always perform an assignment:
Note that this behavior is different to mathematical and bitwise assignment operators.
Setting default content
If the "lyrics" element is empty, set the innerHTML to a default value:
Here the short-circuit is especially beneficial, since the element will not be updated unnecessarily and won't cause unwanted side-effects such as additional parsing or rendering work, or loss of focus, etc.
Note: Pay attention to the value returned by the API you're checking against. If an empty string is returned (a falsy value), ||= must be used, otherwise you want to use the ??= operator (for null or undefined return values).
Specifications
Browser compatibility.
- Logical OR (||)
- The nullish coalescing operator ( ?? )
- Bitwise OR assignment ( |= )
- JavaScript basics
- JavaScript first steps
- JavaScript building blocks
- Introducing JavaScript objects
- Introduction
- Grammar and types
- Control flow and error handling
- Loops and iteration
- Expressions and operators
- Numbers and dates
- Text formatting
- Regular expressions
- Indexed collections
- Keyed collections
- Working with objects
- Details of the object model
- Using promises
- Iterators and generators
- Meta programming
- JavaScript modules
- Client-side JavaScript frameworks
- Client-side web APIs
- A re-introduction to JavaScript
- JavaScript data structures
- Equality comparisons and sameness
- Inheritance and the prototype chain
- Strict mode
- JavaScript typed arrays
- Memory Management
- Concurrency model and Event Loop
- References:
- AggregateError
- ArrayBuffer
- AsyncFunction
- BigInt64Array
- BigUint64Array
- FinalizationRegistry
- Float32Array
- Float64Array
- GeneratorFunction
- InternalError
- ReferenceError
- SharedArrayBuffer
- SyntaxError
- Uint16Array
- Uint32Array
- Uint8ClampedArray
- WebAssembly
- decodeURI()
- decodeURIComponent()
- encodeURI()
- encodeURIComponent()
- parseFloat()
- Addition (+)
- Addition assignment (+=)
- Assignment (=)
- Bitwise AND (&)
- Bitwise AND assignment (&=)
- Bitwise NOT (~)
- Bitwise OR (|)
- Bitwise OR assignment (|=)
- Bitwise XOR (^)
- Bitwise XOR assignment (^=)
- Comma operator (,)
- Conditional (ternary) operator
- Decrement (--)
- Destructuring assignment
- Division (/)
- Division assignment (/=)
- Equality (==)
- Exponentiation (**)
- Exponentiation assignment (**=)
- Function expression
- Greater than (>)
- Greater than or equal (>=)
- Grouping operator ( )
- Increment (++)
- Inequality (!=)
- Left shift (<<)
- Left shift assignment (<<=)
- Less than (<)
- Less than or equal (<=)
- Logical AND (&&)
- Logical AND assignment (&&=)
- Logical NOT (!)
- Logical nullish assignment (??=)
- Multiplication (*)
- Multiplication assignment (*=)
- Nullish coalescing operator (??)
- Object initializer
- Operator precedence
- Optional chaining (?.)
- Pipeline operator (|>)
- Property accessors
- Remainder (%)
- Remainder assignment (%=)
- Right shift (>>)
- Right shift assignment (>>=)
- Spread syntax (...)
- Strict equality (===)
- Strict inequality (!==)
- Subtraction (-)
- Subtraction assignment (-=)
- Unary negation (-)
- Unary plus (+)
- Unsigned right shift (>>>)
- Unsigned right shift assignment (>>>=)
- async function expression
- class expression
- delete operator
- function* expression
- in operator
- new operator
- void operator
- async function
- for await...of
- function declaration
- import.meta
- try...catch
- Arrow function expressions
- Default parameters
- Method definitions
- Rest parameters
- The arguments object
- Private class fields
- Public class fields
- constructor
- Error: Permission denied to access property "x"
- InternalError: too much recursion
- RangeError: argument is not a valid code point
- RangeError: invalid array length
- RangeError: invalid date
- RangeError: precision is out of range
- RangeError: radix must be an integer
- RangeError: repeat count must be less than infinity
- RangeError: repeat count must be non-negative
- ReferenceError: "x" is not defined
- ReferenceError: assignment to undeclared variable "x"
- ReferenceError: can't access lexical declaration "x" before initialization
- ReferenceError: deprecated caller or arguments usage
- ReferenceError: invalid assignment left-hand side
- ReferenceError: reference to undefined property "x"
- SyntaxError: "0"-prefixed octal literals and octal escape seq. are deprecated
- SyntaxError: "use strict" not allowed in function with non-simple parameters
- SyntaxError: "x" is a reserved identifier
- SyntaxError: JSON.parse: bad parsing
- SyntaxError: Malformed formal parameter
- SyntaxError: Unexpected token
- SyntaxError: Using //@ to indicate sourceURL pragmas is deprecated. Use //# instead
- SyntaxError: a declaration in the head of a for-of loop can't have an initializer
- SyntaxError: applying the "delete" operator to an unqualified name is deprecated
- SyntaxError: for-in loop head declarations may not have initializers
- SyntaxError: function statement requires a name
- SyntaxError: identifier starts immediately after numeric literal
- SyntaxError: illegal character
- SyntaxError: invalid regular expression flag "x"
- SyntaxError: missing ) after argument list
- SyntaxError: missing ) after condition
- SyntaxError: missing : after property id
- SyntaxError: missing ; before statement
- SyntaxError: missing = in const declaration
- SyntaxError: missing ] after element list
- SyntaxError: missing formal parameter
- SyntaxError: missing name after . operator
- SyntaxError: missing variable name
- SyntaxError: missing } after function body
- SyntaxError: missing } after property list
- SyntaxError: redeclaration of formal parameter "x"
- SyntaxError: return not in function
- SyntaxError: test for equality (==) mistyped as assignment (=)?
- SyntaxError: unterminated string literal
- TypeError: "x" has no properties
- TypeError: "x" is (not) "y"
- TypeError: "x" is not a constructor
- TypeError: "x" is not a function
- TypeError: "x" is not a non-null object
- TypeError: "x" is read-only
- TypeError: "x" is not iterable
- TypeError: More arguments needed
- TypeError: Reduce of empty array with no initial value
- TypeError: X.prototype.y called on incompatible type
- TypeError: can't access dead object
- TypeError: can't access property "x" of "y"
- TypeError: can't assign to property "x" on "y": not an object
- TypeError: can't define property "x": "obj" is not extensible
- TypeError: can't delete non-configurable array element
- TypeError: can't redefine non-configurable property "x"
- TypeError: cannot use "in" operator to search for "x" in "y"
- TypeError: cyclic object value
- TypeError: invalid "instanceof" operand "x"
- TypeError: invalid Array.prototype.sort argument
- TypeError: invalid arguments
- TypeError: invalid assignment to const "x"
- TypeError: property "x" is non-configurable and can't be deleted
- TypeError: setting getter-only property "x"
- TypeError: variable "x" redeclares argument
- URIError: malformed URI sequence
- Warning: -file- is being assigned a //# sourceMappingURL, but already has one
- Warning: 08/09 is not a legal ECMA-262 octal constant
- Warning: Date.prototype.toLocaleFormat is deprecated
- Warning: JavaScript 1.6's for-each-in loops are deprecated
- Warning: String.x is deprecated; use String.prototype.x instead
- Warning: expression closures are deprecated
- Warning: unreachable code after return statement
- JavaScript technologies overview
- Lexical grammar
- Enumerability and ownership of properties
- Iteration protocols
- Transitioning to strict mode
- Template literals
- Deprecated features
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
- JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
- JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
- JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
JavaScript Comparison and Logical Operators
JavaScript Ternary Operator
JavaScript Booleans
JavaScript Bitwise Operators
- JavaScript Object.is()
- JavaScript typeof Operator
JavaScript operators are special symbols that perform operations on one or more operands (values). For example,
Here, we used the + operator to add the operands 2 and 3 .
JavaScript Operator Types
Here is a list of different JavaScript operators you will learn in this tutorial:
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- String Operators
- Miscellaneous Operators
1. JavaScript Arithmetic Operators
We use arithmetic operators to perform arithmetic calculations like addition, subtraction, etc. For example,
Here, we used the - operator to subtract 3 from 5 .
Commonly Used Arithmetic Operators
Example 1: arithmetic operators in javascript.
Note: The increment operator ++ adds 1 to the operand. And, the decrement operator -- decreases the value of the operand by 1 .
To learn more, visit Increment ++ and Decrement -- Operators .
2. JavaScript Assignment Operators
We use assignment operators to assign values to variables. For example,
Here, we used the = operator to assign the value 5 to the variable x .
Commonly Used Assignment Operators
Example 2: assignment operators in javascript, 3. javascript comparison operators.
We use comparison operators to compare two values and return a boolean value ( true or false ). For example,
Here, we have used the > comparison operator to check whether a (whose value is 3 ) is greater than b (whose value is 2 ).
Since 3 is greater than 2 , we get true as output.
Note: In the above example, a > b is called a boolean expression since evaluating it results in a boolean value.
Commonly Used Comparison Operators
Example 3: comparison operators in javascript.
The equality operators ( == and != ) convert both operands to the same type before comparing their values. For example,
Here, we used the == operator to compare the number 3 and the string 3 .
By default, JavaScript converts string 3 to number 3 and compares the values.
However, the strict equality operators ( === and !== ) do not convert operand types before comparing their values. For example,
Here, JavaScript didn't convert string 4 to number 4 before comparing their values.
Thus, the result is false , as number 4 isn't equal to string 4 .
4. JavaScript Logical Operators
We use logical operators to perform logical operations on boolean expressions. For example,
Here, && is the logical operator AND . Since both x < 6 and y < 5 are true , the combined result is true .
Commonly Used Logical Operators
Example 4: logical operators in javascript.
Note: We use comparison and logical operators in decision-making and loops. You will learn about them in detail in later tutorials.
More on JavaScript Operators
We use bitwise operators to perform binary operations on integers.
Note: We rarely use bitwise operators in everyday programming. If you are interested, visit JavaScript Bitwise Operators to learn more.
In JavaScript, you can also use the + operator to concatenate (join) two strings. For example,
Here, we used the + operator to concatenate str1 and str2 .
JavaScript has many more operators besides the ones we listed above. You will learn about them in detail in later tutorials.
Table of Contents
- Introduction
- JavaScript Arithmetic Operators
- JavaScript Assignment Operators
- JavaScript Comparison Operators
- JavaScript Logical Operators
Video: JavaScript Operators
Sorry about that.
Related Tutorials
JavaScript Tutorial
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
Interview Preparation
- Interview Preparation For Software Developers
- Must Coding Questions - Company-wise
- Must Do Coding Questions - Topic-wise
- Company-wise Practice Problems
- Company Preparation
- Competitive Programming
- Software Design-Patterns
- Company-wise Interview Experience
- Experienced - Interview Experiences
- Internship - Interview Experiences
Practice @Geeksforgeeks
- Problem of the Day
- Topic-wise Practice
- Difficulty Level - School
- Difficulty Level - Basic
- Difficulty Level - Easy
- Difficulty Level - Medium
- Difficulty Level - Hard
- Leaderboard !!
- Explore More...
Data Structures
- Linked List
- Binary Tree
- Binary Search Tree
- Advance Data Structures
- All Data Structures
- Analysis of Algorithms
- Searching Algorithms
- Sorting Algorithms
- Pattern Searching
- Geometric Algorithms
- Mathematical Algorithms
- Randomized Algorithms
- Greedy Algorithms
- Dynamic Programming
- Divide & Conquer
- Backtracking
- Branch & Bound
- All Algorithms
Programming Languages
- Web Technologies
- Tailwind CSS
- Web Browser
- File Formats
Computer Science Subjects
- Operating Systems
- Computer Network
- Computer Organization & Architecture
- Compiler Design
- Digital Elec. & Logic Design
- Software Engineering
- Engineering Mathematics
Data Science & ML
- Complete Data Science Course
- Data Science Tutorial
- Machine Learning Tutorial
- Deep Learning Tutorial
- NLP Tutorial
- Machine Learning Projects
- Data Analysis Tutorial
Tutorial Library
- Python Tutorial
- Django Tutorial
- Pandas Tutorial
- Kivy Tutorial
- Tkinter Tutorial
- OpenCV Tutorial
- Selenium Tutorial
- GATE CS Notes
- Gate Corner
- Previous Year GATE Papers
- Last Minute Notes (LMNs)
- Important Topic For GATE CS
- GATE Course
- Previous Year Paper: CS exams
- Git Tutorial
- AWS Tutorial
- Docker Tutorial
- Kubernetes Tutorial
- Microsoft Azure Tutorial
QUIZ Section
- Python Quiz
- JavaScript Quiz
- Data Structures Quiz
- Algorithms Quiz
- Topic-wise MCQs
School Content
- CBSE Notes 2023-24
- CBSE Class 8 Notes
- CBSE Class 9 Notes
- CBSE Class 10 Notes
- CBSE Class 11 Notes
- CBSE Class 12 Notes
- School Programming
- English Grammar
- Accountancy
- Business Studies
- Human Resource Management
- Top 100 Puzzles
- Mathematical Riddles
Does JavaScript Operators like or ( || ), and ( && ), null coalescing ( ?? ) makes Weak Comparison?
JavaScript operators like logical OR (||), logical AND (&&) , and null coalescing (??) are used for making conditional evaluations and performing logical operations in JavaScript.
While these operators are commonly used for controlling the flow of the code execution and handling values in the different scenarios it’s essential to understand how they behave in terms of comparison and evaluation.
We will discuss different approaches of JavaScript operators like or ( || ), and ( && ), null coalescing ( ?? ) makes a weak comparison:
Table of Content
Weak Comparison with Logical Operators (|| and &&)
Null coalescing operator ().
The logical OR (||) and logical AND (&&) operators in JavaScript perform the weak comparison. The Weak comparison means that the operands are coerced to the boolean values before being evaluated. In the case of the logical OR (||) if any of the operands evaluates to true the result is true. Similarly in the case of the logical AND (&&) if all operands evaluate to the true the result is true.
Example : Below is an of JavaScript operators Weak comparison with Logical Operators (|| and &&):
The null coalescing operator (??) is used to the provide a default value for the nullable values. It returns the right-hand operand when the left-hand operand is null or undefined; otherwise, it returns the left-hand operand.
Example : Below is an example of JavaScript Operators of Null Coalescing Operator (??):
Please Login to comment...
Similar reads.
- JavaScript-Questions
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Regular expressions
Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp , and with the match() , matchAll() , replace() , replaceAll() , search() , and split() methods of String . This chapter describes JavaScript regular expressions.
Creating a regular expression
You construct a regular expression in one of two ways:
- Using a regular expression literal, which consists of a pattern enclosed between slashes, as follows: js const re = / ab + c / ; Regular expression literals provide compilation of the regular expression when the script is loaded. If the regular expression remains constant, using this can improve performance.
- Or calling the constructor function of the RegExp object, as follows: js const re = new RegExp ( "ab+c" ) ; Using the constructor function provides runtime compilation of the regular expression. Use the constructor function when you know the regular expression pattern will be changing, or you don't know the pattern and are getting it from another source, such as user input.
Writing a regular expression pattern
A regular expression pattern is composed of simple characters, such as /abc/ , or a combination of simple and special characters, such as /ab*c/ or /Chapter (\d+)\.\d*/ . The last example includes parentheses, which are used as a memory device. The match made with this part of the pattern is remembered for later use, as described in Using groups .
Note: If you are already familiar with the forms of a regular expression, you may also read the cheat sheet for a quick lookup for a specific pattern/construct.
Using simple patterns
Simple patterns are constructed of characters for which you want to find a direct match. For example, the pattern /abc/ matches character combinations in strings only when the exact sequence "abc" occurs (all characters together and in that order). Such a match would succeed in the strings "Hi, do you know your abc's?" and "The latest airplane designs evolved from slabcraft." . In both cases the match is with the substring "abc" . There is no match in the string "Grab crab" because while it contains the substring "ab c" , it does not contain the exact substring "abc" .
Using special characters
When the search for a match requires something more than a direct match, such as finding one or more b's, or finding white space, you can include special characters in the pattern. For example, to match a single "a" followed by zero or more "b" s followed by "c" , you'd use the pattern /ab*c/ : the * after "b" means "0 or more occurrences of the preceding item." In the string "cbbabbbbcdebc" , this pattern will match the substring "abbbbc" .
The following pages provide lists of the different special characters that fit into each category, along with descriptions and examples.
Assertions include boundaries, which indicate the beginnings and endings of lines and words, and other patterns indicating in some way that a match is possible (including look-ahead, look-behind, and conditional expressions).
Distinguish different types of characters. For example, distinguishing between letters and digits.
Groups group multiple patterns as a whole, and capturing groups provide extra submatch information when using a regular expression pattern to match against a string. Backreferences refer to a previously captured group in the same regular expression.
Indicate numbers of characters or expressions to match.
If you want to look at all the special characters that can be used in regular expressions in a single table, see the following:
Note: A larger cheat sheet is also available (only aggregating parts of those individual articles).
If you need to use any of the special characters literally (actually searching for a "*" , for instance), you must escape it by putting a backslash in front of it. For instance, to search for "a" followed by "*" followed by "b" , you'd use /a\*b/ — the backslash "escapes" the "*" , making it literal instead of special.
Similarly, if you're writing a regular expression literal and need to match a slash ("/"), you need to escape that (otherwise, it terminates the pattern). For instance, to search for the string "/example/" followed by one or more alphabetic characters, you'd use /\/example\/[a-z]+/i —the backslashes before each slash make them literal.
To match a literal backslash, you need to escape the backslash. For instance, to match the string "C:\" where "C" can be any letter, you'd use /[A-Z]:\\/ — the first backslash escapes the one after it, so the expression searches for a single literal backslash.
If using the RegExp constructor with a string literal, remember that the backslash is an escape in string literals, so to use it in the regular expression, you need to escape it at the string literal level. /a\*b/ and new RegExp("a\\*b") create the same expression, which searches for "a" followed by a literal "*" followed by "b".
If escape strings are not already part of your pattern you can add them using String.prototype.replace() :
The "g" after the regular expression is an option or flag that performs a global search, looking in the whole string and returning all matches. It is explained in detail below in Advanced Searching With Flags .
Why isn't this built into JavaScript? There is a proposal to add such a function to RegExp.
Using parentheses
Parentheses around any part of the regular expression pattern causes that part of the matched substring to be remembered. Once remembered, the substring can be recalled for other use. See Groups and backreferences for more details.
Using regular expressions in JavaScript
Regular expressions are used with the RegExp methods test() and exec() and with the String methods match() , matchAll() , replace() , replaceAll() , search() , and split() .
When you want to know whether a pattern is found in a string, use the test() or search() methods; for more information (but slower execution) use the exec() or match() methods. If you use exec() or match() and if the match succeeds, these methods return an array and update properties of the associated regular expression object and also of the predefined regular expression object, RegExp . If the match fails, the exec() method returns null (which coerces to false ).
In the following example, the script uses the exec() method to find a match in a string.
If you do not need to access the properties of the regular expression, an alternative way of creating myArray is with this script:
(See Using the global search flag with exec() for further info about the different behaviors.)
If you want to construct the regular expression from a string, yet another alternative is this script:
With these scripts, the match succeeds and returns the array and updates the properties shown in the following table.
As shown in the second form of this example, you can use a regular expression created with an object initializer without assigning it to a variable. If you do, however, every occurrence is a new regular expression. For this reason, if you use this form without assigning it to a variable, you cannot subsequently access the properties of that regular expression. For example, assume you have this script:
However, if you have this script:
The occurrences of /d(b+)d/g in the two statements are different regular expression objects and hence have different values for their lastIndex property. If you need to access the properties of a regular expression created with an object initializer, you should first assign it to a variable.
Advanced searching with flags
Regular expressions have optional flags that allow for functionality like global searching and case-insensitive searching. These flags can be used separately or together in any order, and are included as part of the regular expression.
To include a flag with the regular expression, use this syntax:
Note that the flags are an integral part of a regular expression. They cannot be added or removed later.
For example, re = /\w+\s/g creates a regular expression that looks for one or more characters followed by a space, and it looks for this combination throughout the string.
You could replace the line:
and get the same result.
The m flag is used to specify that a multiline input string should be treated as multiple lines. If the m flag is used, ^ and $ match at the start or end of any line within the input string instead of the start or end of the entire string.
Using the global search flag with exec()
RegExp.prototype.exec() method with the g flag returns each match and its position iteratively.
In contrast, String.prototype.match() method returns all matches at once, but without their position.
Using unicode regular expressions
The u flag is used to create "unicode" regular expressions; that is, regular expressions which support matching against unicode text. An important feature that's enabled in unicode mode is Unicode property escapes . For example, the following regular expression might be used to match against an arbitrary unicode "word":
Unicode regular expressions have different execution behavior as well. RegExp.prototype.unicode contains more explanation about this.
Note: Several examples are also available in:
- The reference pages for exec() , test() , match() , matchAll() , search() , replace() , split()
- The guide articles: character classes , assertions , groups and backreferences , quantifiers
Using special characters to verify input
In the following example, the user is expected to enter a phone number. When the user presses the "Check" button, the script checks the validity of the number. If the number is valid (matches the character sequence specified by the regular expression), the script shows a message thanking the user and confirming the number. If the number is invalid, the script informs the user that the phone number is not valid.
The regular expression looks for:
- the beginning of the line of data: ^
- followed by three numeric characters \d{3} OR | a left parenthesis \( , followed by three digits \d{3} , followed by a close parenthesis \) , in a non-capturing group (?:)
- followed by one dash, forward slash, or decimal point in a capturing group ()
- followed by three digits \d{3}
- followed by the match remembered in the (first) captured group \1
- followed by four digits \d{4}
- followed by the end of the line of data: $
An online tool to learn, build, & test Regular Expressions.
An online regex builder/debugger
An online interactive tutorials, Cheat sheet, & Playground.
An online visual regex tester.
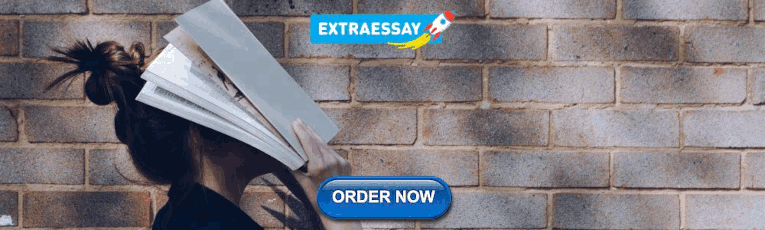
IMAGES
VIDEO
COMMENTS
Description. Logical OR assignment short-circuits, meaning that x ||= y is equivalent to x || (x = y), except that the expression x is only evaluated once. No assignment is performed if the left-hand side is not falsy, due to short-circuiting of the logical OR operator. For example, the following does not throw an error, despite x being const: js.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
The following example uses the logical assignment operator to display a default message if the search result element is empty: document.querySelector('.search-result').textContent ||= 'Sorry! No result found'; Code language: JavaScript (javascript) The Logical AND assignment operator. The logical AND assignment operator only assigns y to x if x ...
This is made to assign a default value, in this case the value of y, if the x variable is falsy. The boolean operators in JavaScript can return an operand, and not always a boolean result as in other languages. The Logical OR operator ( ||) returns the value of its second operand, if the first one is falsy, otherwise the value of the first ...
In classical programming, the logical OR is meant to manipulate boolean values only. If any of its arguments are true, it returns true, otherwise it returns false. In JavaScript, the operator is a little bit trickier and more powerful. But first, let's see what happens with boolean values.
The following example shows how to use the ! operator: !a. The logical ! operator works based on the following rules: If a is undefined, the result is true. If a is null, the result is true. If a is a number other than 0, the result is false. If a is NaN, the result is true. If a is an object, the result is false.
Logical operators are frequently employed in conditional statements (if, else if, and else) to dictate program flow based on specific conditions. 1. if Statement: The if statement in JavaScript is used to execute a block of code if a specified condition is true. Logical operators often play a crucial role in defining these conditions.
The logical OR assignment operator (||=) accepts two operands and assigns the right operand to the left operand if the left operand is falsy. The syntax is as below. x ||= y. In the syntax, the ||= will only assign the value of y to x if x is falsy. Let's take a look at the || operator first.
JavaScript Logical AND assignment (&&=) Operator. This operator is represented by x &&= y, and it is called the logical AND assignment operator. It assigns the value of y into x only if x is a truthy value. We use this operator x &&= y like this. Now break this expression into two parts, x && (x = y). If the value of x is true, then the ...
Short circuit means that the expr part above is not evaluated, hence any side effects of doing so do not take effect (e.g., if expr is a function call, the calling never takes place). Logical AND assignment short-circuits as well meaning that x &&= y is equivalent to: And not equivalent to the following which would always perform an assignment:
Logical assignment. Published 07 May 2020 · Tagged with ECMAScript ES2021. JavaScript supports a range of compound assignment operators that let programmers succinctly express a binary operation together with assignment. Currently, only mathematical or bitwise operations are supported. What has been missing is the ability to combine logical ...
In 2021, with the latest version of javascript, a new type of operator alled "Logical Assignment Operators" was introduced for programming. That is, they have introduced a new type of operator ...
The &&= symbol is a "Logical AND assignment operator" and connects two variables. If the initial value is correct, the second value is used. It is graded from left to right. Syntax. The following syntax shows the Logical AND assignment operator with the two values. Value1 &&= Value2. Value1 &&= Value2. Examples.
The logical OR operator short-circuits: the second operand is only evaluated if the first operand doesn't already determine the result. Logical OR assignment short-circuits as well, meaning it only performs an assignment if the logical operation would evaluate the right-hand side. In other words, x ||= y is equivalent to: x || (x = y); And ...
JavaScript Assignment Operators. We use assignment operators to assign values to variables. For example, let x = 5; Here, we used the = operator to assign the value 5 to the variable x. Commonly Used Assignment Operators. Operator ... Example 4: Logical Operators in JavaScript
JavaScript Logical OR assignment (||=) Operator. This operator is represented by x ||= y and it is called a logical OR assignment operator. If the value of x is falsy then the value of y will be assigned to x. When we divide it into two parts it becomes x || ( x = y ). It checks if x is true or false, if the value of x is falsy then it runs the ...
Basic keywords and general expressions in JavaScript. These expressions have the highest precedence (higher than operators ). The this keyword refers to a special property of an execution context. Basic null, boolean, number, and string literals. Array initializer/literal syntax. Object initializer/literal syntax.
Weak Comparison with Logical Operators (|| and &&) The logical OR (||) and logical AND (&&) operators in JavaScript perform the weak comparison. The Weak comparison means that the operands are coerced to the boolean values before being evaluated. In the case of the logical OR (||) if any of the operands evaluates to true the result is true.
JavaScript Logical OR invalid assignment. 12. Ternary operators returning "true : false". Why? 1. How am I mis-using the || logical operator in this piece of code? 2. Javascript logical operators: multiple || syntax dilemma. 1. Or operator not working in IF statement Node.js. 0.
Regular expressions are patterns used to match character combinations in strings. In JavaScript, regular expressions are also objects. These patterns are used with the exec() and test() methods of RegExp, and with the match(), matchAll(), replace(), replaceAll(), search(), and split() methods of String. This chapter describes JavaScript regular expressions.