Python Enhancement Proposals
- Python »
- PEP Index »
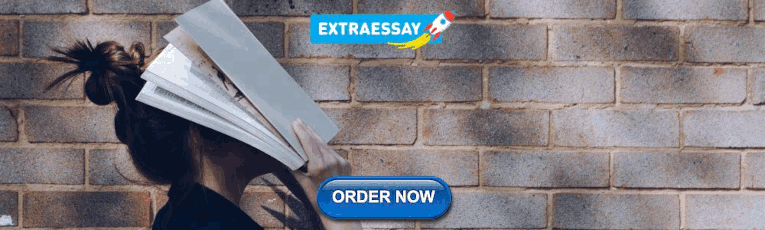
PEP 572 – Assignment Expressions
The importance of real code, exceptional cases, scope of the target, relative precedence of :=, change to evaluation order, differences between assignment expressions and assignment statements, specification changes during implementation, _pydecimal.py, datetime.py, sysconfig.py, simplifying list comprehensions, capturing condition values, changing the scope rules for comprehensions, alternative spellings, special-casing conditional statements, special-casing comprehensions, lowering operator precedence, allowing commas to the right, always requiring parentheses, why not just turn existing assignment into an expression, with assignment expressions, why bother with assignment statements, why not use a sublocal scope and prevent namespace pollution, style guide recommendations, acknowledgements, a numeric example, appendix b: rough code translations for comprehensions, appendix c: no changes to scope semantics.
This is a proposal for creating a way to assign to variables within an expression using the notation NAME := expr .
As part of this change, there is also an update to dictionary comprehension evaluation order to ensure key expressions are executed before value expressions (allowing the key to be bound to a name and then re-used as part of calculating the corresponding value).
During discussion of this PEP, the operator became informally known as “the walrus operator”. The construct’s formal name is “Assignment Expressions” (as per the PEP title), but they may also be referred to as “Named Expressions” (e.g. the CPython reference implementation uses that name internally).
Naming the result of an expression is an important part of programming, allowing a descriptive name to be used in place of a longer expression, and permitting reuse. Currently, this feature is available only in statement form, making it unavailable in list comprehensions and other expression contexts.
Additionally, naming sub-parts of a large expression can assist an interactive debugger, providing useful display hooks and partial results. Without a way to capture sub-expressions inline, this would require refactoring of the original code; with assignment expressions, this merely requires the insertion of a few name := markers. Removing the need to refactor reduces the likelihood that the code be inadvertently changed as part of debugging (a common cause of Heisenbugs), and is easier to dictate to another programmer.
During the development of this PEP many people (supporters and critics both) have had a tendency to focus on toy examples on the one hand, and on overly complex examples on the other.
The danger of toy examples is twofold: they are often too abstract to make anyone go “ooh, that’s compelling”, and they are easily refuted with “I would never write it that way anyway”.
The danger of overly complex examples is that they provide a convenient strawman for critics of the proposal to shoot down (“that’s obfuscated”).
Yet there is some use for both extremely simple and extremely complex examples: they are helpful to clarify the intended semantics. Therefore, there will be some of each below.
However, in order to be compelling , examples should be rooted in real code, i.e. code that was written without any thought of this PEP, as part of a useful application, however large or small. Tim Peters has been extremely helpful by going over his own personal code repository and picking examples of code he had written that (in his view) would have been clearer if rewritten with (sparing) use of assignment expressions. His conclusion: the current proposal would have allowed a modest but clear improvement in quite a few bits of code.
Another use of real code is to observe indirectly how much value programmers place on compactness. Guido van Rossum searched through a Dropbox code base and discovered some evidence that programmers value writing fewer lines over shorter lines.
Case in point: Guido found several examples where a programmer repeated a subexpression, slowing down the program, in order to save one line of code, e.g. instead of writing:
they would write:
Another example illustrates that programmers sometimes do more work to save an extra level of indentation:
This code tries to match pattern2 even if pattern1 has a match (in which case the match on pattern2 is never used). The more efficient rewrite would have been:
Syntax and semantics
In most contexts where arbitrary Python expressions can be used, a named expression can appear. This is of the form NAME := expr where expr is any valid Python expression other than an unparenthesized tuple, and NAME is an identifier.
The value of such a named expression is the same as the incorporated expression, with the additional side-effect that the target is assigned that value:
There are a few places where assignment expressions are not allowed, in order to avoid ambiguities or user confusion:
This rule is included to simplify the choice for the user between an assignment statement and an assignment expression – there is no syntactic position where both are valid.
Again, this rule is included to avoid two visually similar ways of saying the same thing.
This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
This rule is included to discourage side effects in a position whose exact semantics are already confusing to many users (cf. the common style recommendation against mutable default values), and also to echo the similar prohibition in calls (the previous bullet).
The reasoning here is similar to the two previous cases; this ungrouped assortment of symbols and operators composed of : and = is hard to read correctly.
This allows lambda to always bind less tightly than := ; having a name binding at the top level inside a lambda function is unlikely to be of value, as there is no way to make use of it. In cases where the name will be used more than once, the expression is likely to need parenthesizing anyway, so this prohibition will rarely affect code.
This shows that what looks like an assignment operator in an f-string is not always an assignment operator. The f-string parser uses : to indicate formatting options. To preserve backwards compatibility, assignment operator usage inside of f-strings must be parenthesized. As noted above, this usage of the assignment operator is not recommended.
An assignment expression does not introduce a new scope. In most cases the scope in which the target will be bound is self-explanatory: it is the current scope. If this scope contains a nonlocal or global declaration for the target, the assignment expression honors that. A lambda (being an explicit, if anonymous, function definition) counts as a scope for this purpose.
There is one special case: an assignment expression occurring in a list, set or dict comprehension or in a generator expression (below collectively referred to as “comprehensions”) binds the target in the containing scope, honoring a nonlocal or global declaration for the target in that scope, if one exists. For the purpose of this rule the containing scope of a nested comprehension is the scope that contains the outermost comprehension. A lambda counts as a containing scope.
The motivation for this special case is twofold. First, it allows us to conveniently capture a “witness” for an any() expression, or a counterexample for all() , for example:
Second, it allows a compact way of updating mutable state from a comprehension, for example:
However, an assignment expression target name cannot be the same as a for -target name appearing in any comprehension containing the assignment expression. The latter names are local to the comprehension in which they appear, so it would be contradictory for a contained use of the same name to refer to the scope containing the outermost comprehension instead.
For example, [i := i+1 for i in range(5)] is invalid: the for i part establishes that i is local to the comprehension, but the i := part insists that i is not local to the comprehension. The same reason makes these examples invalid too:
While it’s technically possible to assign consistent semantics to these cases, it’s difficult to determine whether those semantics actually make sense in the absence of real use cases. Accordingly, the reference implementation [1] will ensure that such cases raise SyntaxError , rather than executing with implementation defined behaviour.
This restriction applies even if the assignment expression is never executed:
For the comprehension body (the part before the first “for” keyword) and the filter expression (the part after “if” and before any nested “for”), this restriction applies solely to target names that are also used as iteration variables in the comprehension. Lambda expressions appearing in these positions introduce a new explicit function scope, and hence may use assignment expressions with no additional restrictions.
Due to design constraints in the reference implementation (the symbol table analyser cannot easily detect when names are re-used between the leftmost comprehension iterable expression and the rest of the comprehension), named expressions are disallowed entirely as part of comprehension iterable expressions (the part after each “in”, and before any subsequent “if” or “for” keyword):
A further exception applies when an assignment expression occurs in a comprehension whose containing scope is a class scope. If the rules above were to result in the target being assigned in that class’s scope, the assignment expression is expressly invalid. This case also raises SyntaxError :
(The reason for the latter exception is the implicit function scope created for comprehensions – there is currently no runtime mechanism for a function to refer to a variable in the containing class scope, and we do not want to add such a mechanism. If this issue ever gets resolved this special case may be removed from the specification of assignment expressions. Note that the problem already exists for using a variable defined in the class scope from a comprehension.)
See Appendix B for some examples of how the rules for targets in comprehensions translate to equivalent code.
The := operator groups more tightly than a comma in all syntactic positions where it is legal, but less tightly than all other operators, including or , and , not , and conditional expressions ( A if C else B ). As follows from section “Exceptional cases” above, it is never allowed at the same level as = . In case a different grouping is desired, parentheses should be used.
The := operator may be used directly in a positional function call argument; however it is invalid directly in a keyword argument.
Some examples to clarify what’s technically valid or invalid:
Most of the “valid” examples above are not recommended, since human readers of Python source code who are quickly glancing at some code may miss the distinction. But simple cases are not objectionable:
This PEP recommends always putting spaces around := , similar to PEP 8 ’s recommendation for = when used for assignment, whereas the latter disallows spaces around = used for keyword arguments.)
In order to have precisely defined semantics, the proposal requires evaluation order to be well-defined. This is technically not a new requirement, as function calls may already have side effects. Python already has a rule that subexpressions are generally evaluated from left to right. However, assignment expressions make these side effects more visible, and we propose a single change to the current evaluation order:
- In a dict comprehension {X: Y for ...} , Y is currently evaluated before X . We propose to change this so that X is evaluated before Y . (In a dict display like {X: Y} this is already the case, and also in dict((X, Y) for ...) which should clearly be equivalent to the dict comprehension.)
Most importantly, since := is an expression, it can be used in contexts where statements are illegal, including lambda functions and comprehensions.
Conversely, assignment expressions don’t support the advanced features found in assignment statements:
- Multiple targets are not directly supported: x = y = z = 0 # Equivalent: (z := (y := (x := 0)))
- Single assignment targets other than a single NAME are not supported: # No equivalent a [ i ] = x self . rest = []
- Priority around commas is different: x = 1 , 2 # Sets x to (1, 2) ( x := 1 , 2 ) # Sets x to 1
- Iterable packing and unpacking (both regular or extended forms) are not supported: # Equivalent needs extra parentheses loc = x , y # Use (loc := (x, y)) info = name , phone , * rest # Use (info := (name, phone, *rest)) # No equivalent px , py , pz = position name , phone , email , * other_info = contact
- Inline type annotations are not supported: # Closest equivalent is "p: Optional[int]" as a separate declaration p : Optional [ int ] = None
- Augmented assignment is not supported: total += tax # Equivalent: (total := total + tax)
The following changes have been made based on implementation experience and additional review after the PEP was first accepted and before Python 3.8 was released:
- for consistency with other similar exceptions, and to avoid locking in an exception name that is not necessarily going to improve clarity for end users, the originally proposed TargetScopeError subclass of SyntaxError was dropped in favour of just raising SyntaxError directly. [3]
- due to a limitation in CPython’s symbol table analysis process, the reference implementation raises SyntaxError for all uses of named expressions inside comprehension iterable expressions, rather than only raising them when the named expression target conflicts with one of the iteration variables in the comprehension. This could be revisited given sufficiently compelling examples, but the extra complexity needed to implement the more selective restriction doesn’t seem worthwhile for purely hypothetical use cases.
Examples from the Python standard library
env_base is only used on these lines, putting its assignment on the if moves it as the “header” of the block.
- Current: env_base = os . environ . get ( "PYTHONUSERBASE" , None ) if env_base : return env_base
- Improved: if env_base := os . environ . get ( "PYTHONUSERBASE" , None ): return env_base
Avoid nested if and remove one indentation level.
- Current: if self . _is_special : ans = self . _check_nans ( context = context ) if ans : return ans
- Improved: if self . _is_special and ( ans := self . _check_nans ( context = context )): return ans
Code looks more regular and avoid multiple nested if. (See Appendix A for the origin of this example.)
- Current: reductor = dispatch_table . get ( cls ) if reductor : rv = reductor ( x ) else : reductor = getattr ( x , "__reduce_ex__" , None ) if reductor : rv = reductor ( 4 ) else : reductor = getattr ( x , "__reduce__" , None ) if reductor : rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
- Improved: if reductor := dispatch_table . get ( cls ): rv = reductor ( x ) elif reductor := getattr ( x , "__reduce_ex__" , None ): rv = reductor ( 4 ) elif reductor := getattr ( x , "__reduce__" , None ): rv = reductor () else : raise Error ( "un(deep)copyable object of type %s " % cls )
tz is only used for s += tz , moving its assignment inside the if helps to show its scope.
- Current: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) tz = self . _tzstr () if tz : s += tz return s
- Improved: s = _format_time ( self . _hour , self . _minute , self . _second , self . _microsecond , timespec ) if tz := self . _tzstr (): s += tz return s
Calling fp.readline() in the while condition and calling .match() on the if lines make the code more compact without making it harder to understand.
- Current: while True : line = fp . readline () if not line : break m = define_rx . match ( line ) if m : n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v else : m = undef_rx . match ( line ) if m : vars [ m . group ( 1 )] = 0
- Improved: while line := fp . readline (): if m := define_rx . match ( line ): n , v = m . group ( 1 , 2 ) try : v = int ( v ) except ValueError : pass vars [ n ] = v elif m := undef_rx . match ( line ): vars [ m . group ( 1 )] = 0
A list comprehension can map and filter efficiently by capturing the condition:
Similarly, a subexpression can be reused within the main expression, by giving it a name on first use:
Note that in both cases the variable y is bound in the containing scope (i.e. at the same level as results or stuff ).
Assignment expressions can be used to good effect in the header of an if or while statement:
Particularly with the while loop, this can remove the need to have an infinite loop, an assignment, and a condition. It also creates a smooth parallel between a loop which simply uses a function call as its condition, and one which uses that as its condition but also uses the actual value.
An example from the low-level UNIX world:
Rejected alternative proposals
Proposals broadly similar to this one have come up frequently on python-ideas. Below are a number of alternative syntaxes, some of them specific to comprehensions, which have been rejected in favour of the one given above.
A previous version of this PEP proposed subtle changes to the scope rules for comprehensions, to make them more usable in class scope and to unify the scope of the “outermost iterable” and the rest of the comprehension. However, this part of the proposal would have caused backwards incompatibilities, and has been withdrawn so the PEP can focus on assignment expressions.
Broadly the same semantics as the current proposal, but spelled differently.
Since EXPR as NAME already has meaning in import , except and with statements (with different semantics), this would create unnecessary confusion or require special-casing (e.g. to forbid assignment within the headers of these statements).
(Note that with EXPR as VAR does not simply assign the value of EXPR to VAR – it calls EXPR.__enter__() and assigns the result of that to VAR .)
Additional reasons to prefer := over this spelling include:
- In if f(x) as y the assignment target doesn’t jump out at you – it just reads like if f x blah blah and it is too similar visually to if f(x) and y .
- import foo as bar
- except Exc as var
- with ctxmgr() as var
To the contrary, the assignment expression does not belong to the if or while that starts the line, and we intentionally allow assignment expressions in other contexts as well.
- NAME = EXPR
- if NAME := EXPR
reinforces the visual recognition of assignment expressions.
This syntax is inspired by languages such as R and Haskell, and some programmable calculators. (Note that a left-facing arrow y <- f(x) is not possible in Python, as it would be interpreted as less-than and unary minus.) This syntax has a slight advantage over ‘as’ in that it does not conflict with with , except and import , but otherwise is equivalent. But it is entirely unrelated to Python’s other use of -> (function return type annotations), and compared to := (which dates back to Algol-58) it has a much weaker tradition.
This has the advantage that leaked usage can be readily detected, removing some forms of syntactic ambiguity. However, this would be the only place in Python where a variable’s scope is encoded into its name, making refactoring harder.
Execution order is inverted (the indented body is performed first, followed by the “header”). This requires a new keyword, unless an existing keyword is repurposed (most likely with: ). See PEP 3150 for prior discussion on this subject (with the proposed keyword being given: ).
This syntax has fewer conflicts than as does (conflicting only with the raise Exc from Exc notation), but is otherwise comparable to it. Instead of paralleling with expr as target: (which can be useful but can also be confusing), this has no parallels, but is evocative.
One of the most popular use-cases is if and while statements. Instead of a more general solution, this proposal enhances the syntax of these two statements to add a means of capturing the compared value:
This works beautifully if and ONLY if the desired condition is based on the truthiness of the captured value. It is thus effective for specific use-cases (regex matches, socket reads that return '' when done), and completely useless in more complicated cases (e.g. where the condition is f(x) < 0 and you want to capture the value of f(x) ). It also has no benefit to list comprehensions.
Advantages: No syntactic ambiguities. Disadvantages: Answers only a fraction of possible use-cases, even in if / while statements.
Another common use-case is comprehensions (list/set/dict, and genexps). As above, proposals have been made for comprehension-specific solutions.
This brings the subexpression to a location in between the ‘for’ loop and the expression. It introduces an additional language keyword, which creates conflicts. Of the three, where reads the most cleanly, but also has the greatest potential for conflict (e.g. SQLAlchemy and numpy have where methods, as does tkinter.dnd.Icon in the standard library).
As above, but reusing the with keyword. Doesn’t read too badly, and needs no additional language keyword. Is restricted to comprehensions, though, and cannot as easily be transformed into “longhand” for-loop syntax. Has the C problem that an equals sign in an expression can now create a name binding, rather than performing a comparison. Would raise the question of why “with NAME = EXPR:” cannot be used as a statement on its own.
As per option 2, but using as rather than an equals sign. Aligns syntactically with other uses of as for name binding, but a simple transformation to for-loop longhand would create drastically different semantics; the meaning of with inside a comprehension would be completely different from the meaning as a stand-alone statement, while retaining identical syntax.
Regardless of the spelling chosen, this introduces a stark difference between comprehensions and the equivalent unrolled long-hand form of the loop. It is no longer possible to unwrap the loop into statement form without reworking any name bindings. The only keyword that can be repurposed to this task is with , thus giving it sneakily different semantics in a comprehension than in a statement; alternatively, a new keyword is needed, with all the costs therein.
There are two logical precedences for the := operator. Either it should bind as loosely as possible, as does statement-assignment; or it should bind more tightly than comparison operators. Placing its precedence between the comparison and arithmetic operators (to be precise: just lower than bitwise OR) allows most uses inside while and if conditions to be spelled without parentheses, as it is most likely that you wish to capture the value of something, then perform a comparison on it:
Once find() returns -1, the loop terminates. If := binds as loosely as = does, this would capture the result of the comparison (generally either True or False ), which is less useful.
While this behaviour would be convenient in many situations, it is also harder to explain than “the := operator behaves just like the assignment statement”, and as such, the precedence for := has been made as close as possible to that of = (with the exception that it binds tighter than comma).
Some critics have claimed that the assignment expressions should allow unparenthesized tuples on the right, so that these two would be equivalent:
(With the current version of the proposal, the latter would be equivalent to ((point := x), y) .)
However, adopting this stance would logically lead to the conclusion that when used in a function call, assignment expressions also bind less tight than comma, so we’d have the following confusing equivalence:
The less confusing option is to make := bind more tightly than comma.
It’s been proposed to just always require parentheses around an assignment expression. This would resolve many ambiguities, and indeed parentheses will frequently be needed to extract the desired subexpression. But in the following cases the extra parentheses feel redundant:
Frequently Raised Objections
C and its derivatives define the = operator as an expression, rather than a statement as is Python’s way. This allows assignments in more contexts, including contexts where comparisons are more common. The syntactic similarity between if (x == y) and if (x = y) belies their drastically different semantics. Thus this proposal uses := to clarify the distinction.
The two forms have different flexibilities. The := operator can be used inside a larger expression; the = statement can be augmented to += and its friends, can be chained, and can assign to attributes and subscripts.
Previous revisions of this proposal involved sublocal scope (restricted to a single statement), preventing name leakage and namespace pollution. While a definite advantage in a number of situations, this increases complexity in many others, and the costs are not justified by the benefits. In the interests of language simplicity, the name bindings created here are exactly equivalent to any other name bindings, including that usage at class or module scope will create externally-visible names. This is no different from for loops or other constructs, and can be solved the same way: del the name once it is no longer needed, or prefix it with an underscore.
(The author wishes to thank Guido van Rossum and Christoph Groth for their suggestions to move the proposal in this direction. [2] )
As expression assignments can sometimes be used equivalently to statement assignments, the question of which should be preferred will arise. For the benefit of style guides such as PEP 8 , two recommendations are suggested.
- If either assignment statements or assignment expressions can be used, prefer statements; they are a clear declaration of intent.
- If using assignment expressions would lead to ambiguity about execution order, restructure it to use statements instead.
The authors wish to thank Alyssa Coghlan and Steven D’Aprano for their considerable contributions to this proposal, and members of the core-mentorship mailing list for assistance with implementation.
Appendix A: Tim Peters’s findings
Here’s a brief essay Tim Peters wrote on the topic.
I dislike “busy” lines of code, and also dislike putting conceptually unrelated logic on a single line. So, for example, instead of:
instead. So I suspected I’d find few places I’d want to use assignment expressions. I didn’t even consider them for lines already stretching halfway across the screen. In other cases, “unrelated” ruled:
is a vast improvement over the briefer:
The original two statements are doing entirely different conceptual things, and slamming them together is conceptually insane.
In other cases, combining related logic made it harder to understand, such as rewriting:
as the briefer:
The while test there is too subtle, crucially relying on strict left-to-right evaluation in a non-short-circuiting or method-chaining context. My brain isn’t wired that way.
But cases like that were rare. Name binding is very frequent, and “sparse is better than dense” does not mean “almost empty is better than sparse”. For example, I have many functions that return None or 0 to communicate “I have nothing useful to return in this case, but since that’s expected often I’m not going to annoy you with an exception”. This is essentially the same as regular expression search functions returning None when there is no match. So there was lots of code of the form:
I find that clearer, and certainly a bit less typing and pattern-matching reading, as:
It’s also nice to trade away a small amount of horizontal whitespace to get another _line_ of surrounding code on screen. I didn’t give much weight to this at first, but it was so very frequent it added up, and I soon enough became annoyed that I couldn’t actually run the briefer code. That surprised me!
There are other cases where assignment expressions really shine. Rather than pick another from my code, Kirill Balunov gave a lovely example from the standard library’s copy() function in copy.py :
The ever-increasing indentation is semantically misleading: the logic is conceptually flat, “the first test that succeeds wins”:
Using easy assignment expressions allows the visual structure of the code to emphasize the conceptual flatness of the logic; ever-increasing indentation obscured it.
A smaller example from my code delighted me, both allowing to put inherently related logic in a single line, and allowing to remove an annoying “artificial” indentation level:
That if is about as long as I want my lines to get, but remains easy to follow.
So, in all, in most lines binding a name, I wouldn’t use assignment expressions, but because that construct is so very frequent, that leaves many places I would. In most of the latter, I found a small win that adds up due to how often it occurs, and in the rest I found a moderate to major win. I’d certainly use it more often than ternary if , but significantly less often than augmented assignment.
I have another example that quite impressed me at the time.
Where all variables are positive integers, and a is at least as large as the n’th root of x, this algorithm returns the floor of the n’th root of x (and roughly doubling the number of accurate bits per iteration):
It’s not obvious why that works, but is no more obvious in the “loop and a half” form. It’s hard to prove correctness without building on the right insight (the “arithmetic mean - geometric mean inequality”), and knowing some non-trivial things about how nested floor functions behave. That is, the challenges are in the math, not really in the coding.
If you do know all that, then the assignment-expression form is easily read as “while the current guess is too large, get a smaller guess”, where the “too large?” test and the new guess share an expensive sub-expression.
To my eyes, the original form is harder to understand:
This appendix attempts to clarify (though not specify) the rules when a target occurs in a comprehension or in a generator expression. For a number of illustrative examples we show the original code, containing a comprehension, and the translation, where the comprehension has been replaced by an equivalent generator function plus some scaffolding.
Since [x for ...] is equivalent to list(x for ...) these examples all use list comprehensions without loss of generality. And since these examples are meant to clarify edge cases of the rules, they aren’t trying to look like real code.
Note: comprehensions are already implemented via synthesizing nested generator functions like those in this appendix. The new part is adding appropriate declarations to establish the intended scope of assignment expression targets (the same scope they resolve to as if the assignment were performed in the block containing the outermost comprehension). For type inference purposes, these illustrative expansions do not imply that assignment expression targets are always Optional (but they do indicate the target binding scope).
Let’s start with a reminder of what code is generated for a generator expression without assignment expression.
- Original code (EXPR usually references VAR): def f (): a = [ EXPR for VAR in ITERABLE ]
- Translation (let’s not worry about name conflicts): def f (): def genexpr ( iterator ): for VAR in iterator : yield EXPR a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a simple assignment expression.
- Original code: def f (): a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): if False : TARGET = None # Dead code to ensure TARGET is a local variable def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Let’s add a global TARGET declaration in f() .
- Original code: def f (): global TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def f (): global TARGET def genexpr ( iterator ): global TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Or instead let’s add a nonlocal TARGET declaration in f() .
- Original code: def g (): TARGET = ... def f (): nonlocal TARGET a = [ TARGET := EXPR for VAR in ITERABLE ]
- Translation: def g (): TARGET = ... def f (): nonlocal TARGET def genexpr ( iterator ): nonlocal TARGET for VAR in iterator : TARGET = EXPR yield TARGET a = list ( genexpr ( iter ( ITERABLE )))
Finally, let’s nest two comprehensions.
- Original code: def f (): a = [[ TARGET := i for i in range ( 3 )] for j in range ( 2 )] # I.e., a = [[0, 1, 2], [0, 1, 2]] print ( TARGET ) # prints 2
- Translation: def f (): if False : TARGET = None def outer_genexpr ( outer_iterator ): nonlocal TARGET def inner_generator ( inner_iterator ): nonlocal TARGET for i in inner_iterator : TARGET = i yield i for j in outer_iterator : yield list ( inner_generator ( range ( 3 ))) a = list ( outer_genexpr ( range ( 2 ))) print ( TARGET )
Because it has been a point of confusion, note that nothing about Python’s scoping semantics is changed. Function-local scopes continue to be resolved at compile time, and to have indefinite temporal extent at run time (“full closures”). Example:
This document has been placed in the public domain.
Source: https://github.com/python/peps/blob/main/peps/pep-0572.rst
Last modified: 2023-10-11 12:05:51 GMT
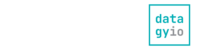
- Learn Python
- Python Lists
- Python Dictionaries
- Python Strings
- Python Functions
- Learn Pandas & NumPy
- Pandas Tutorials
- Numpy Tutorials
- Learn Data Visualization
- Python Seaborn
- Python Matplotlib
Python: Return Multiple Values from a Function
- October 29, 2021 December 19, 2022
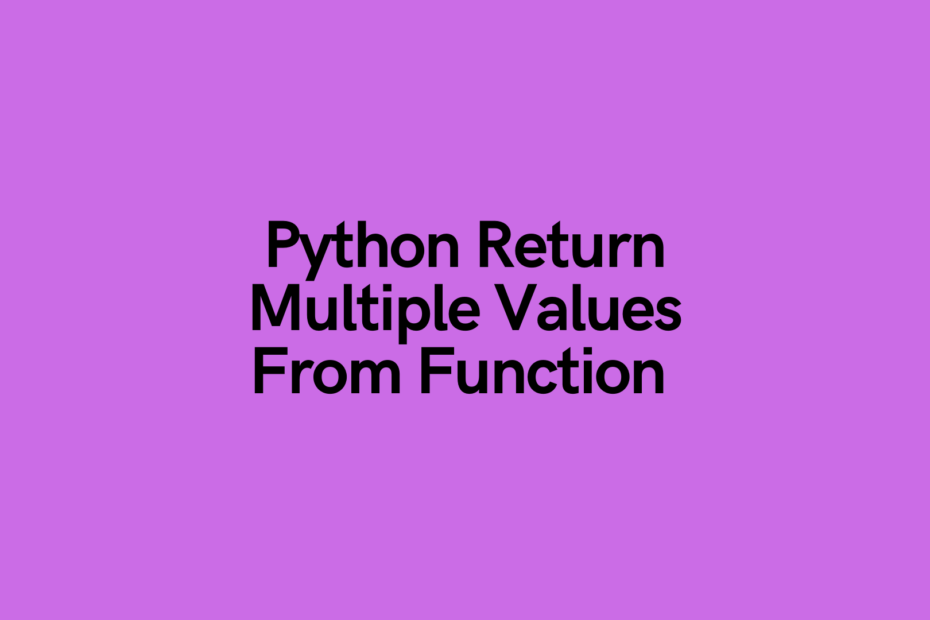
In this tutorial, you’ll learn how to use Python to return multiple values from your functions . This is a task that is often quite difficult in some other languages, but very easy to do in Python.
You’ll learn how to use tuples, implicitly or explicitly, lists, and dictionaries to return multiple values from a function. You’ll also learn how to identify which method is best suited for your use case. You’ll also learn how to unpack items of unequal length, using the unpacking operator ( * ).
Being able to work with functions is an incredibly useful skill that allows you to more readily follow the DRY (don’t repeat yourself) principle. Functions allow your code to be significantly more readable and less repetitive. All of this allows your code to be more maintainable and reduces complexity of the code.
The Quick Answer: Use Tuple Unpacking
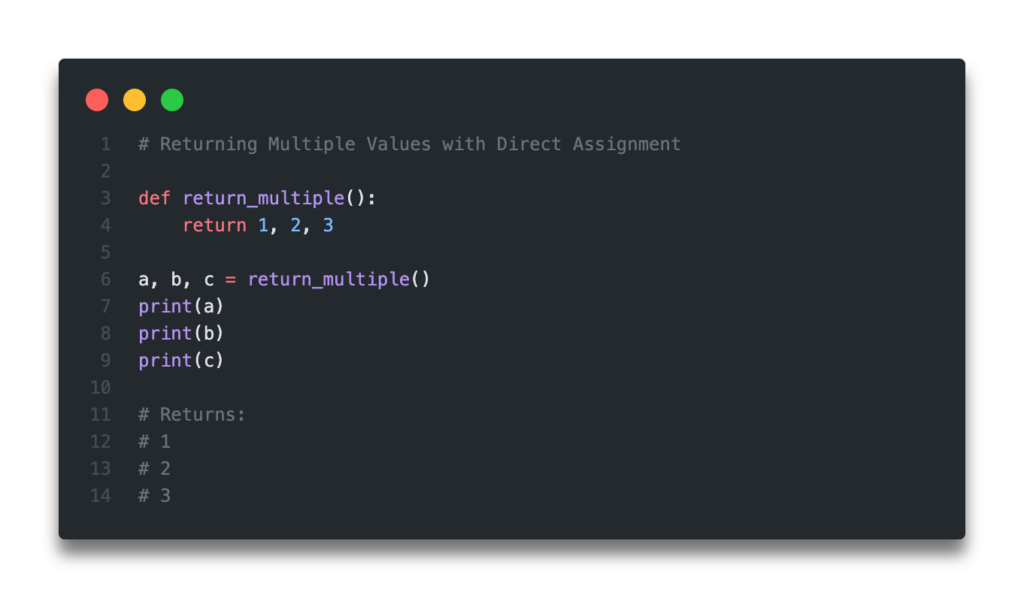
Table of Contents
How do Functions Return Values in Python?
Python functions are easy ways to structure code into dynamic processes that are readable and reusable. While Python functions can accept inputs, in this tutorial, we’ll be looking at function outputs . Specifically, we’ll be look at how functions return values.
Let’s take a look at how a function in Python is designed and how to return one value and how to return two values.
In the example above, we have defined two different functions, return_one() and return_two() . The former of these returns only a single value. Meanwhile, the latter function, return_two() , returns two values. This is done by separating the values by commas.
In the next section, you’ll learn how and why returning multiple values actually works.
Want to learn more about calculating the square root in Python? Check out my tutorial here , which will teach you different ways of calculating the square root, both without Python functions and with the help of functions.
How to Return Multiple Values from a Python Function with Tuples
In the previous section, you learned how to configure a Python function to return more than a single value.
The way that this works, is that Python actually turns the values (separated by commas) into a tuple. We can see how this works by assigning the function to a variable and checking its type.
We can see in the code above that when we assign our function to a variable, that a tuple is generated.
This may surprise you, however, since you don’t actually tell the function to return (1, 2, 3) . Python implicitly handles converting the return values into a tuple. It’s not the parentheses that turn the return value into a tuple, but rather the comma along with the parentheses.
We can verify this by checking the type of the value (1), for example. This returns: int .
Again, this might surprise you. If we changed our value to (1,) , however, we return a different result.
A lot of this may seem like semantics, but it allows you to understand why these things actually work. Now, let’s learn how to assign these multiple variables to different variables.
Let’s look at the same function as before. Instead of assigning the return values to a single tuple, let’s unpack our tuple and return three separate values .
The reason this works is that Python is handling unpacking these values for us. Because we have the same number of assignment variables as we do values in the return statement, Python handles the assignment of these values for us.
In the next section, you’ll learn how to unpack multiple values from a Python to variables with mismatched lengths.
Want to learn more about Python for-loops? Check out my in-depth tutorial that takes your from beginner to advanced for-loops user! Want to watch a video instead? Check out my YouTube tutorial here .
How to Unpack Multiple Values from a Python Function to Unequal Lengths
In the example above, you learned how to return multiple values from a Python function by unpacking values from the return tuple.
There may be many times when your function returns multiple values, but you only care about a few. You don’t really care about the other values, but Python will not let you return only a few of them.
Let’s see what this looks like:
This happens because our assignment needs to match the number of items returned.
However, Python also comes with an unpacking operator , which is denoted by * . Say that we only cared about the first item returned. We still need to assign the remaining values to another variable, but we can easily group them into a single variable, using the unpacking operator.
Let’s see how this works in Python:
Here, we have unpacked the first value to our variable a , and all other variables to the variable b , using the notation of *b .
In the next section, you’ll learn how to return multiple values from a Python function using lists.
Want to learn how to use the Python zip() function to iterate over two lists? This tutorial teaches you exactly what the zip() function does and shows you some creative ways to use the function.
How to Return Multiple Values from a Python Function with Lists
Similar to returning multiple values using tuples, as shown in the previous examples, we can return multiple values from a Python function using lists.
One of the big differences between Python sets and lists is that lists are mutable in Python , meaning that they can be changed. If this is an important characteristic of the values you return, then this is a good way to go.
Let’s see how we can return multiple values from a function, using both assignment to a single variable and to multiple variables.
In the next section, you’ll learn how to use Python dictionaries to better understand return values.
Want to learn more about Python list comprehensions? Check out this in-depth tutorial that covers off everything you need to know, with hands-on examples. More of a visual learner, check out my YouTube tutorial here .
How to Return Multiple Values from a Python Function with Dictionaries
In both examples above, if you’re returning all values to a single variable, it can be difficult to determine what each value represents. For example, while you can access all the items in a tuple or in a list using indexing, it can be difficult to determine what each value represents.
Let’s take a look at a more complicated function that creates variables for speed , time , and distance travelled for a car.
If we returned this as a tuple or as a list, then we would need to know which variable represents what item. However, we can also return these items as a dictionary. When we do this, we can access each item by its key .
Let’s see how we can do this in Python:
Need to check if a key exists in a Python dictionary? Check out this tutorial , which teaches you five different ways of seeing if a key exists in a Python dictionary, including how to return a default value.
In this tutorial, you learned how to return multiple values from Python functions. You learned how and why multiple values can be returned and how to optimize how values are returned for your use cases, by learning how to return tuples, lists, and dictionaries. You also learned how to unpack multiple values to variables of different lengths.
To learn more about Python functions, check out the official documentation here .
Nik Piepenbreier
Nik is the author of datagy.io and has over a decade of experience working with data analytics, data science, and Python. He specializes in teaching developers how to use Python for data science using hands-on tutorials. View Author posts
1 thought on “Python: Return Multiple Values from a Function”
Pingback: Functions in Python • datagy
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Python Return Multiple Values – How to Return a Tuple, List, or Dictionary

You can return multiple values from a function in Python.
To do so, return a data structure that contains multiple values, like a list containing the number of miles to run each week.
Data structures in Python are used to store collections of data, which can be returned from functions. In this article, we’ll explore how to return multiple values from these data structures: tuples, lists, and dictionaries.
A tuple is an ordered, immutable sequence. That means, a tuple can’t change.
Use a tuple, for example, to store information about a person: their name, age, and location.
Here’s how you’d write a function that returns a tuple.
Notice that we didn’t use parentheses in the return statement. That’s because you can return a tuple by separating each item with a comma, as shown in the above example.
“It is actually the comma which makes a tuple, not the parentheses,” the documentation points out. However, parentheses are required with empty tuples or to avoid confusion.
Here’s an example of a function that uses parentheses () to return a tuple.
A list is an ordered, mutable sequence. That means, a list can change.
You can use a list to store cities:
Or test scores:
Take a look at the function below. It returns a list that contains ten numbers.
Here’s another example. This time we pass in several arguments when we call the function.
It’s easy to confuse tuples and lists. After all, both are containers that store objects. However, remember these key differences:
- Tuples can’t change.
- Lists can change.
Dictionaries
A dictionary contains key-value pairs wrapped in curly brackets {} . Each “key” has a related “value.”
Consider the dictionary of employees below. Each employee name is a “key” and their position is the “value.”
Here’s how you’d write a function that returns a dictionary with a key, value pair.
In the above example, “Boston” is the key and “United States” is the value .
We’ve covered a lot of ground. The key point is this: you can return multiple values from a Python function, and there are several ways to do so.
I write about the programming skills you need to develop and the concepts you need to learn, and the best ways to learn them at amymhaddad.com .
Programmer and writer | howtolearneffectively.com | dailyskillplanner.com
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Best practice for returning unknown data type from a dict?
Python 3.12 on Windows 10 Pro. I’m still fairly new to Python and have not seen this covered in my tutorial.
I’m not finding much at all after a Google search.
I have a function which checks to see if a dictionary key exists. And if it doesn’t, it returns a blank string '' . But sometimes the value in the dict can be a string, float, or int. Or the key may not exist at all, which is where I’m having trouble.
Let’s make an example program.
- What are ways for me to deal with this?
- Should I pass the type of variable I want returned into the getdictval() function as parameter 3? I may expect to return a str, float, or int.
My idea to pass the data type I want:
One point I would make is that dict is a reserved word for the dictionary type, so shouldn’t be used as the name of a variable.
Pass the actual type instead of a string. Functions are first-class objects in Python, so you can just pass them around, and then getdictval can call it to create the “default” value instead of needing any conditional logic:
Better yet, use collections.defaultdict , which wraps this for you already.
The only “reserved words” in Python are the keywords that are part of the language grammar (i.e., things that would cause a syntax error if misused). dict is a built-in name, meaning that a value is pre-assigned in the widest possible scope. It is possible to shadow this with proper understanding, although it may still be considered poor style. Everyone should have a proper understanding of this regardless, so:
Thanks I think I fixed all the instances of dict variable.
I’m looking into the mydict.setdefault() function. My issues is a bit more complicated since this is actually a dict of dicts. I will have to be careful to figure out exactly where to set the defaults for what I call the “subdict”.
The data looks a bit like this:
I’m finding Python to be really helpful! I just have to find out more about the functions related to dicts.
Yes, it is a name that denotes the built-in dictionary type - I wrote that “dict is a reserved word for the dictionary type”, not that dict is a reserved word of the language. I could have expressed it better, but I was describing it in the sense you have described.
I think the names of built-ins, like dict , set , list etc. should be treated as if they were reserved words - I have never seen production code where these were used interchangeably to denote user-defined variables.
P. S. I’ve just tried to find - unsuccessfully - prominent examples where built-ins names were used as variables, or people advocating for that. Which is why I think with “proper understanding” shadowing built-ins is never a good idea.
Have you looked at the stdlib collections.defaultdict class? Perhaps not completely what you want, but it’s good to know it’s there:
Can be nested:
Your method looks more like get with an empty string as a default than it does setdefault .
Related Topics
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
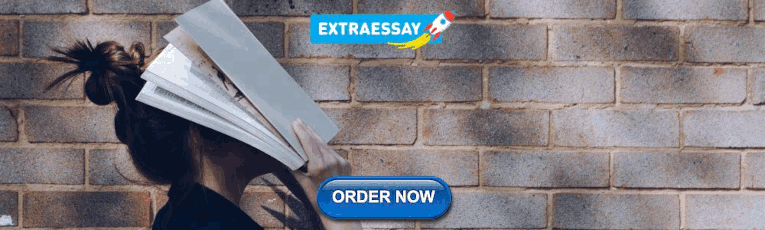
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
In this article, we are going to see how to assign a function to a variable in Python. In Python, we can assign a function to a variable. And using that variable we can call the function as many as times we want. Thereby, increasing code reusability.
Implementation
Simply assign a function to the desired variable but without () i.e. just with the name of the function. If the variable is assigned with function along with the brackets (), None will be returned.
Output:
The following programs will help you understand better:
Example 1:
Example 2: parameterized function
Please Login to comment...
Similar reads.
- Python function-programs
- Python-Functions
- 10 Ways to Use Microsoft OneNote for Note-Taking
- 10 Best Yellow.ai Alternatives & Competitors in 2024
- 10 Best Online Collaboration Tools 2024
- 10 Best Autodesk Maya Alternatives for 3D Animation and Modeling in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
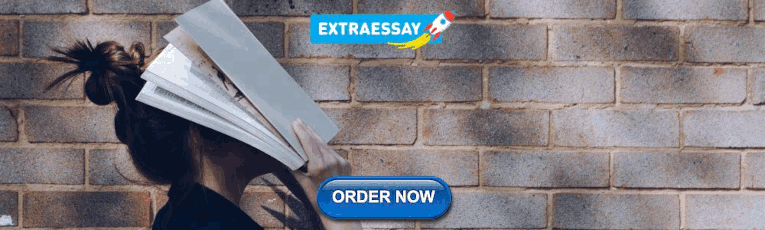
COMMENTS
The Python return statement is a key component of functions and methods.You can use the return statement to make your functions send Python objects back to the caller code. These objects are known as the function's return value.You can use them to perform further computation in your programs. Using the return statement effectively is a core skill if you want to code custom functions that are ...
x() is not the function, it's the call of the function. In python, functions are simply a type of variable, and can generally be used like any other variable. For example: def power_function(power): return lambda x : x**power power_function(3)(2) This returns 8. power_function is a function that
Understanding the Return Statement. The return statement in Python is used to exit a function and return a value to the caller. It allows you to pass back a specific result or data from a function, enabling you to utilize the output for further computation or processing. The return statement is often placed at the end of a function and marks ...
To create a new variable or to update the value of an existing one in Python, you'll use an assignment statement. This statement has the following three components: A left operand, which must be a variable. The assignment operator ( =) A right operand, which can be a concrete value, an object, or an expression.
Python Return Statements Explained: What They Are and Why You Use Them. All functions return a value when called. If a return statement is followed by an expression list, that expression list is evaluated and the value is returned: >>> def greater_than_1(n): ... return n > 1.
In this lesson, you'll learn about the biggest change in Python 3.8: the introduction of assignment expressions.Assignment expression are written with a new notation (:=).This operator is often called the walrus operator as it resembles the eyes and tusks of a walrus on its side.. Assignment expressions allow you to assign and return a value in the same expression.
Unparenthesized assignment expressions are prohibited for the value of a keyword argument in a call. Example: foo(x = y := f(x)) # INVALID foo(x=(y := f(x))) # Valid, though probably confusing. This rule is included to disallow excessively confusing code, and because parsing keyword arguments is complex enough already.
Multiplying 0 * 0 Multiplying 1 * 1 Multiplying 2 * 2 [1, 4] You define a function named slow_calculation that multiplies the given number x with itself. A list comprehension then iterates through 0, 1, and 2 returned by range(3).An assignment expression binds the value result to the return of slow_calculation with i.You add the result to the newly built list as long as it is greater than 0.
Python return statement. Last Updated : 09 Jun, 2022. A return statement is used to end the execution of the function call and "returns" the result (value of the expression following the return keyword) to the caller. The statements after the return statements are not executed. If the return statement is without any expression, then the ...
Exercise 1: Create a function in Python. Exercise 2: Create a function with variable length of arguments. Exercise 3: Return multiple values from a function. Exercise 4: Create a function with a default argument. Exercise 5: Create an inner function to calculate the addition in the following way. Exercise 6: Create a recursive function.
In Python, is it possible to make multiple assignments in the following manner (or, rather, is there a shorthand): import random def random_int(): return random.randint(1, 100) a, b = # for each variable assign the return values from random_int
Let's see how we can return multiple values from a function, using both assignment to a single variable and to multiple variables. # Returning Multiple Values to Lists def return_multiple (): return [ 1, 2, 3 ] # Return all at once. return_all = return_multiple() # Return multiple variables.
Each new version of Python adds new features to the language. For Python 3.8, the biggest change is the addition of assignment expressions.Specifically, the := operator gives you a new syntax for assigning variables in the middle of expressions. This operator is colloquially known as the walrus operator.. This tutorial is an in-depth introduction to the walrus operator.
You can return the function from a function. You can store them in data structures such as hash tables, lists, …. Example 1: Functions without arguments. In this example, the first method is A () and the second method is B (). A () method returns the B () method that is kept as an object with name returned_function and will be used for ...
You can return multiple values from a function in Python. To do so, return a data structure that contains multiple values, like a list containing the number of miles to run each week. def miles_to_run(minimum_miles): week_1 = minimum_miles + 2 week_2 = minimum_miles +
By taking advantage of Python's flexibility in object types, as well as the new assignment expressions in Python 3.8, you can call this simplified function within a conditional if statement and get the return value if the check passes:
Python 3.12 on Windows 10 Pro. I'm still fairly new to Python and have not seen this covered in my tutorial. I'm not finding much at all after a Google search. I have a function which checks to see if a dictionary key exists. And if it doesn't, it returns a blank string ''. But sometimes the value in the dict can be a string, float, or int.
Assignment Expressions. For more information on concepts covered in this lesson, you can check out: Here's a feature introduced in version 3.8 of Python, which can simplify the function we're currently working on. It's called an assignment expression, and it allows you to save the return value of a function to a variable while at the same ...
In these two functions the "print x" lines do different things: one refers to the global variable x, and the other refers to the local variable x. The x in g is local because of the assignment. This could be even more confusing (than it already is) if it was possible to bury the assignment inside some larger expression/statement.
In Python, we can assign a function to a variable. And using that variable we can call the function as many as times we want. Thereby, increasing code reusability. Implementation. Simply assign a function to the desired variable but without () i.e. just with the name of the function. If the variable is assigned with function along with the ...
I think you are using 'global' incorrectly. See Python reference. You should declare variable without global and then inside the function when you want to access global variable you declare it global yourvar. #!/usr/bin/python total def checkTotal(): global total total = 0 See this example:
I am working on a Python project where I need to sort a list of integers. I wrote a custom function to sort the list, but instead of returning the sorted list, it returns None. Here is the function I wrote: