- Trending Categories

- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
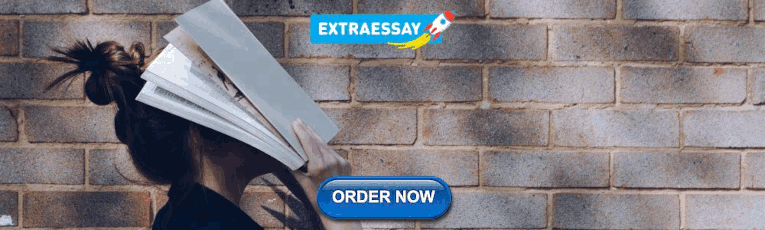
NULL pointer in C
A null pointer is a pointer which points nothing.
Some uses of the null pointer are:
a) To initialize a pointer variable when that pointer variable isn’t assigned any valid memory address yet.
b) To pass a null pointer to a function argument when we don’t want to pass any valid memory address.
c) To check for null pointer before accessing any pointer variable. So that, we can perform error handling in pointer related code e.g. dereference pointer variable only if it’s not NULL.
Live Demo

Learning faster. Every day.
Related Articles
- Null Pointer Exception in C#
- Differentiate the NULL pointer with Void pointer in C language
- Calling class method through NULL class pointer in C++
- Null Pointer Exception in Java Programming
- Why is address zero used for the null pointer in C/C++?
- Calling a member function on a NULL object pointer in C++
- Why do we check for a NULL pointer before deleting in C/C++?
- Double Pointer (Pointer to Pointer) in C
- What should we assign to a C++ pointer: A Null or 0?
- Pointer Arithmetic in C/C++
- void pointer in C
- Function Pointer in C
- Explain the concept of pointer to pointer and void pointer in C language?
- How to define pointer to pointer in C language?
- Explain the concept of Array of Pointer and Pointer to Pointer in C programming
Kickstart Your Career
Get certified by completing the course
Next: Invalid Dereference , Previous: Pointer Dereference , Up: Pointers [ Contents ][ Index ]
14.6 Null Pointers
A pointer value can be null , which means it does not point to any object. The cleanest way to get a null pointer is by writing NULL , a standard macro defined in stddef.h . You can also do it by casting 0 to the desired pointer type, as in (char *) 0 . (The cast operator performs explicit type conversion; See Explicit Type Conversion .)
You can store a null pointer in any lvalue whose data type is a pointer type:
These two, if consecutive, can be combined into a declaration with initializer,
You can also explicitly cast NULL to the specific pointer type you want—it makes no difference.
To test whether a pointer is null, compare it with zero or NULL , as shown here:
Since testing a pointer for not being null is basic and frequent, all but beginners in C will understand the conditional without need for != NULL :

NULL Pointer In C [Explained With Examples] – CsTutorialpoint
Hello friends, in today’s article we are going to talk about NULL Pointer In C Language
Today we will learn in detail about, what is NULL Pointer In C and why and how they are used in C language.
So without wasting time let’s first understand what is NULL Pointer In C
![NULL Pointer In C [Explained With Examples] - CsTutorialpoint NULL Pointer In C](https://cstutorialpoint.com/wp-content/uploads/2022/08/NULL-Pointer-In-C.webp)
What is NULL Pointer In C
In C language, when we do not have any address to assign to a pointer variable, then we assign that pointer variable with NULL.
NULL is a keyword which means that now the pointer is not pointing to anything and when the pointer is not pointing to anything then such pointer is called NULL Pointer .
We can also say that “ a NULL pointer is a pointer that is not pointing to nothing .” NULL is a constant whose value is zero (0). We can create a NULL Pointer by assigning NULL or zero (0) to the pointer variable.
- data_type -: any data type can come here like int, char, float, etc.
- pointer_name -: Pointer name you can keep anything according to you.
- NULL -: Here NULL is a keyword which we assign to pointer variable to make NULL Pointer.
Here ptr is a NULL pointer.
Let’s understand NULL Pointer better through a program.
Example Program of Null pointer
Check out this program, In this program, we have declared four pointer variables, out of which we have assigned the first pointer (ptr1) to the address of one variable and we have left the second pointer (ptr2) as declared without assigning anything.
We have assigned the third pointer (ptr3) with zero (0) and assigned the fourth pointer with NULL. And as we know, assigning any pointer to zero or NULL becomes a NULL pointer, so ptr3 and ptr4 is a NULL pointer and ptr1 and pt2 are not a NULL pointer.
Some important points of the NULL pointer
- If we compare a null pointer to a pointer that is pointing to an object or function, then this comparison will be unequal.
- In C language, we can compare two null pointers of any type because they are both equal.
- In C language, NULL pointers cannot be dereferenced. If you try to do this then there will be a segmentation fault.
- According to the C standard, 0 is a null pointer constant. example -: “int *ptr = 0;” Here “ptr” is a null pointer.
- NULL vs Void Pointer -: NULL is a value in a null pointer and Void is a type in a void pointer.
Use of null pointer in C
- When a pointer does not point to any valid address, then such pointer becomes a dangling pointer. By assigning NULL to such pointer, we can prevent it from becoming a dangling pointer.
- The null pointer is used in error handling.
Friends, I hope you have found the answer to your question and you will not have to search about what is NULL Pointer In C and why and how they are used in C language.
However, if you want any information related to this post or related to programming language, or computer science, then comment below I will clear your all doubts
If you want a complete tutorial on C language, then see here C Language Tutorial . Here you will get all the topics of C Programming Tutorial step by step.
Friends, if you liked this post, then definitely share this post with your friends so that they can get information about Null Pointer In C
To get the information related to Programming Language, Coding, C, C ++, subscribe to our website newsletter. So that you will get information about our upcoming new posts soon.
Jeetu Sahu is A Web Developer | Computer Engineer | Passionate about Coding, Competitive Programming, and Blogging
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
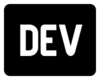
DEV Community

Posted on Oct 29, 2023
How C-Pointers Works: A Step-by-Step Beginner's Tutorial
In this comprehensive C Pointers tutorial, my primary goal is to guide you through the fundamentals of C pointers from the ground up. By the end of this tutorial, you will have gained an in-depth understanding of the following fundamental topics:
- What is a Pointer?
- How Data is Stored in Memory?
- Storing Memory Addresses using Pointers
Accessing Data through Pointers
- Pointer Arithmetic
- Pointer to Pointer (Double Pointers)
- Passing Pointers as Function Arguments
Arrays of Pointers
Null pointers, prerequisite:.
To grasp pointers effectively, you should be comfortable with basic C programming concepts, including variables, data types, functions, loops, and conditional statements. This familiarity with C programming forms the foundation for understanding how pointers work within the language. Once you have a solid grasp of these fundamental concepts, you can confidently delve into the intricacies of C pointers.
What is a pointer?
A pointer serves as a reference that holds the memory location of another variable. This memory address allows us to access the value stored at that location in the memory. You can think of a pointer as a way to reference or point to the location where data is stored in your computer's memory
Pointers can be a challenging concept for beginners to grasp, but in this tutorial, I'll explain them using real-life analogies to make the concept clearer. However, Before delving into pointers and their workings, it's important to understand the concept of a memory address.
A memory address is a unique identifier that points to a specific location in a computer's memory. Think of it like a street address for data stored in your computer's RAM (Random Access Memory). Just as a street address tells you where a particular house is located in the physical world, a memory address tells the computer where a specific piece of information or data is stored in its memory.
Take a look at the image below for a better understanding:

In this illustration, each block represents one byte of memory. It's important to note that every byte of memory has a unique address. To make it easier to understand, I've represented the addresses in decimal notation, but computers actually store these addresses using hexadecimal values. Hexadecimal is a base-16 numbering system commonly used in computing to represent memory addresses and other low-level data. It's essential to be aware of this representation when working with memory-related concepts in computer programming
How data is stored in the memory:
Every piece of data in your computer, whether it's a number, a character, or a program instruction, is stored at a specific memory address. The amount of space reserved for each data type can vary, and it is typically measured in bytes (where 1 byte equals 8 bits, with each bit representing either 0 or 1). The specific sizes of data types also depend on the computer architecture you are using. For instance, on most 64-bit Linux machines, you'll find the following typical sizes for common data types: char = 1 byte int = 4 bytes float = 4 bytes double = 8 bytes These sizes define how much memory each data type occupies and are crucial for memory management and efficient data representation in computer systems.
You can use the sizeof operator to determine the size of data types on your computer. example:
In this example: sizeof(char) returns the size of the char data type in bytes. sizeof(int) returns the size of the int data type in bytes. sizeof(float) returns the size of the float data type in bytes. sizeof(double) returns the size of the double data type in bytes. When you run this code, it will print the sizes of these data types on your specific computer, allowing you to see the actual sizes used by your system.
When you declare a variable, the computer allocates a specific amount of memory space corresponding to the chosen data type. For instance, when you declare a variable of type char, the computer reserves 1 byte of memory because the size of the 'char' data type is conventionally 1 byte.

In this example, we declare a variable n of type char without assigning it a specific value. The memory address allocated for the n variable is 106 . This address, 106 , is where the computer will store the char variable n, but since we haven't assigned it a value yet, the content of this memory location may initially contain an unpredictable or uninitialized value.
When we assign the value 'C' to the variable n, the character 'C' is stored in the memory location associated with the variable n. When we assign the value 'C' to the variable n, the character 'C' is stored in the memory location associated with the variable n.

As mentioned earlier, a byte can only store numerical values. When we store the letter 'C' in a byte, the byte actually holds the ASCII code for 'C,' which is 67. In computer memory, characters are represented using their corresponding ASCII codes. So, in memory, the character 'C' is stored as the numerical value 67. Here's how it looks in memory

Since integers are typically stored within four bytes of memory, let's consider the same example with an int variable. In this scenario, the memory structure would appear as follows:

In this example, the memory address where the variable t is stored is 121. An int variable like “t” typically uses four consecutive memory addresses, such as 121, 122, 123, and 124. The starting address, in this case, 121, represents the location of the first byte of the int, and the subsequent addresses sequentially represent the following bytes that collectively store the complete int value.
If you want to know the memory address of a variable in a program, you can use the 'address of' unary operator, often denoted as the '&' operator. This operator allows you to access the specific memory location where a variable is stored.
When you run the following program on your computer: It will provide you with specific memory addresses for the variables c and n. However, each time you rerun the program, it might allocate new memory addresses for these variables. It's important to understand that while you can determine the memory address of a variable using the & operator, the exact memory location where a variable is stored is typically managed by the system and the compiler. As a programmer, you cannot directly control or assign a specific memory location for a variable. Instead, memory allocation and management are tasks handled by the system and the compiler.
Storing memory address using pointers
As mentioned earlier, a pointer is a variable that stores the memory address of another variable. This memory address allows us to access the value stored at that location in memory. You can think of a pointer as a way to reference or point to the location where data is stored in your computer's memory.
Now, let's begin by declaring and initializing pointers. This step is essential because it sets up the pointer to hold a specific memory address, enabling us to interact with the data stored at that location.
Declaring Pointers: To declare a pointer, you specify the data type it points to, followed by an asterisk (*), and then the pointer's name. For example:
Here, we've declared a pointer named ptr that can point to integers.

The size of pointers on 64-bit systems is usually 8 bytes (64 bits). To determine the pointer size on your system, you can use the sizeof operator:
Initializing Pointers: Once you've declared a pointer, you typically initialize it with the memory address it should point to. Once again, To obtain the memory address of a variable, you can employ the address-of operator (&). For instance:
In this program:
We declare an integer variable x and initialize it with the value 10. This line creates a variable x in memory and assigns the value 10 to it.

We declare an integer pointer ptr using the int *ptr syntax. This line tells the compiler that ptr will be used to store the memory address of an integer variable.

We initialize the pointer ptr with the memory address of the variable x . This is achieved with the line ptr = &x; . The & operator retrieves the memory address of x, and this address is stored in the pointer ptr .

Dereferencing Pointers: To access the data that a pointer is pointing to, you need to dereference the pointer. Dereferencing a pointer means accessing the value stored at the memory address that the pointer points to. In C, you can think of pointers as variables that store memory addresses rather than actual values. To get the actual value (data) stored at that memory address, you need to dereference the pointer.
Dereferencing is done using the asterisk (*) operator. Here's an example:
It looks like this in the memory: int x = 10; variable 'x' stores the value 10:

int *ptr = &x; Now, the pointer 'ptr' point to the address of 'x':

int value = *ptr; Dereference 'ptr' to get the value stored at the address it points to:

Reading and Modifying Data: Pointers allow you to not only read but also modify data indirectly:
Note: The asterisk is a versatile symbol with different meanings depending on where it's used in your C program, for example: Declaration: When used during variable declaration, the asterisk (*) indicates that a variable is a pointer to a specific data type. For example: int *ptr; declares 'ptr' as a pointer to an integer.
Dereferencing: Inside your code, the asterisk (*) in front of a pointer variable is used to access the value stored at the memory address pointed to by the pointer. For example: int value = *ptr; retrieves the value at the address 'ptr' points to.
Pointer Arithmetic:
Pointer arithmetic is the practice of performing mathematical operations on pointers in C. This allows you to navigate through arrays, structures, and dynamically allocated memory. You can increment or decrement pointers, add or subtract integers from them, and compare them. It's a powerful tool for efficient data manipulation, but it should be used carefully to avoid memory-related issues.
Incrementing a Pointer:
Now, this program is how it looks in the memory: int arr[4] = {10, 20, 30, 40};

This behavior is a key aspect of pointer arithmetic. When you add an integer to a pointer, it moves to the memory location of the element at the specified index, allowing you to efficiently access and manipulate elements within the array. It's worth noting that you can use pointer arithmetic to access elements in any position within the array, making it a powerful technique for working with arrays of data. Now, let's print the memory addresses of the elements in the array from our previous program.
If you observe the last two digits of the first address is 40, and the second one is 44. You might be wondering why it's not 40 and 41. This is because we're working with an integer array, and in most systems, the size of an int data type is 4 bytes. Therefore, the addresses are incremented in steps of 4. The first address shows 40, the second 44, and the third one 48
Decrementing a Pointer Decrement (--) a pointer variable, which makes it point to the previous element in an array. For example, ptr-- moves it to the previous one. For example:
Explanation:
We have an integer array arr with 5 elements, and we initialize a pointer ptr to point to the fourth element (value 40) using &arr[3].
Then, we decrement the pointer ptr by one with the statement ptr--. This moves the pointer to the previous memory location, which now points to the third element (value 30).
Finally, we print the value pointed to by the decremented pointer using *ptr, which gives us the value 30.
In this program, we demonstrate how decrementing a pointer moves it to the previous memory location in the array, allowing you to access and manipulate the previous element.
Pointer to pointer
Pointers to pointers, or double pointers, are variables that store the address of another pointer. In essence, they add another level of indirection. These are commonly used when you need to modify the pointer itself or work with multi-dimensional arrays.
To declare and initialize a pointer to a pointer, you need to add an extra asterisk (*) compared to a regular pointer. Let's go through an example:
In this example, ptr2 is a pointer to a pointer. It points to the memory location where the address of x is stored (which is ptr1 ).

The below program will show you how to print the value of x through pointer to pointer
In this program, we first explain that it prints the value of x using a regular variable, a pointer, and a pointer to a pointer. We then print the memory addresses of x , ptr1 , and ptr2 .
Passing Pointers as Function Arguments:
In C, you can pass pointers as function arguments. This allows you to manipulate the original data directly, as opposed to working with a copy of the data, as you would with regular variables. Here's how it works:
How to Declare and Define Functions that Take Pointer Arguments: In your function declaration and definition, you specify that you're passing a pointer by using the * operator after the data type. For example:
In the above function, we declare ptr as a pointer to an integer. This means it can store the memory address of an integer variable.
Why Would You Pass Pointers to Functions?
Passing pointers to functions allows you to:
- Modify the original data directly within the function.
- Avoid making a copy of the data, which can be more memory-efficient.
- Share data between different parts of your program efficiently.
This concept is especially important when working with large data structures or when you need to return multiple values from a function.
Call by Value vs. Call by Reference:
Understanding how data is passed to functions is crucial when working with pointers. there are two common ways that data can be passed to functions: call by value and call by reference.
Call by Value:
When you pass data by value, a copy of the original data is created inside the function. Any modifications to this copy do not affect the original data outside of the function. This is the default behavior for most data types when you don't use pointers.
Call by Reference (Using Pointers):
When you pass data by reference, you're actually passing a pointer to the original data's memory location. This means any changes made within the function will directly affect the original data outside the function. This is achieved by passing pointers as function arguments, making it call by reference. Using pointers as function arguments allows you to achieve call by reference behavior, which is particularly useful when you want to modify the original data inside a function and have those changes reflected outside the function.
Let's dive into some code examples to illustrate how pointers work as function arguments. We'll start with a simple example to demonstrate passing a pointer to a function and modifying the original data.
Consider this example:
In this code, we define a function modifyValue that takes a pointer to an integer. We pass the address of the variable num to this function, and it doubles the value stored in num directly.
This is a simple demonstration of passing a pointer to modify a variable's value. Pointers allow you to work with the original data efficiently.
An array of pointers is essentially an array where each element is a pointer. These pointers can point to different data types (int, char, etc.), providing flexibility and efficiency in managing memory.
How to Declare an Array of Pointers? To declare an array of pointers, you specify the type of data the pointers will point to, followed by square brackets to indicate it's an array, and then the variable name. For example:
Initializing an Array of Pointers You can initialize an array of pointers to each element to point to a specific value, For example:
How to Access Elements Through an Array of Pointers? To access elements through an array of pointers, you can use the pointer notation. For example:
This program demonstrates how to access and print the values pointed to by the pointers in the array.
A NULL pointer is a pointer that lacks a reference to a valid memory location. It's typically used to indicate that a pointer doesn't have a specific memory address assigned, often serving as a placeholder or default value for pointers.
Here's a code example that demonstrates the use of a NULL pointer:
In this example, we declare a pointer ptr and explicitly initialize it with the value NULL. We then use an if statement to check if the pointer is NULL. Since it is, the program will print "The pointer is NULL." This illustrates how NULL pointers are commonly used to check if a pointer has been initialized or assigned a valid memory address.
conclusion:
You've embarked on a comprehensive journey through the intricacies of C pointers. You've learned how pointers store memory addresses, enable data access, facilitate pointer arithmetic, and how they can be used with arrays and functions. Additionally, you've explored the significance of NULL pointers.
By completing this tutorial, you've equipped yourself with a robust understanding of pointers in C. You can now confidently navigate memory, manipulate data efficiently, and harness the power of pointers in your programming projects. These skills will be invaluable as you advance in your coding endeavors. Congratulations on your accomplishment, and keep coding with confidence!
Reference: C - Pointers - Tutorials Point
Pointers in C: A One-Stop Solution for Using C Pointers - simplilearn
Top comments (3)
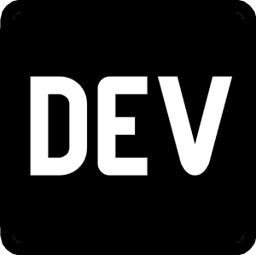
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Jan 7, 2024
Love your way to write articles, could you add an article for, .o files, .h files, lists and makefile? Thank you in advance!

- Joined Nov 4, 2023
Great post. Thank you so much for this.

- Email [email protected]
- Joined Jul 7, 2023
Thank you for your kind words! I'm thrilled to hear that you enjoyed the article. Your feedback means a lot to me. If you have any questions or if there's a specific topic you'd like to see in future posts, feel free to let me know. Thanks again for your support
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
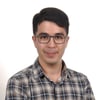
How to full screen a browser in Playwright?
Alperen Coşkun - Feb 25
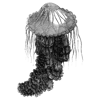
Docker for beginners: Creating Database Containers
João Vitor - Mar 28
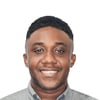
How to install Bootstrap 5 in Angular 17... Standalone components Including css,js & icons.
Okoro chimezie bright - Mar 10
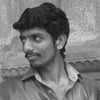
let,const & old var revisited
yogeshwaran - Mar 29
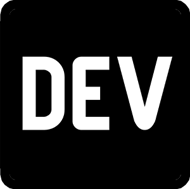
We're a place where coders share, stay up-to-date and grow their careers.
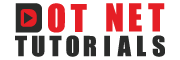
Null Pointer in C
Back to: C Tutorials For Beginners and Professionals
Null Pointer in C Language with Examples
In this article, I will discuss Null Pointer in C Language with Examples. Please read our previous articles discussing Pointer to Constant in C Language with Examples.
What is a Null Pointer?
In C programming, a null pointer is a pointer that does not point to any valid memory location. It’s a special type of pointer used to indicate that it is not intended to point to an accessible memory location. Using a null pointer is essential for error handling and to avoid undefined behavior caused by uninitialized or dangling pointers. A null pointer is a special reserved value defined in a stddef header file.
If we do not have any address which is to be assigned to the pointer, then it is known as a null pointer. When a NULL value is assigned to the pointer, it is considered a Null pointer. So, A null pointer is a pointer that points to nothing. Some uses of the null pointer are as follows:
- Used to initialize a pointer variable when that pointer variable isn’t assigned any valid memory address yet.
- Used to pass a null pointer to a function argument when we don’t want to pass any valid memory address.
- Used to check for a null pointer before accessing any pointer variable. So that we can perform error handling in pointer-related code, e.g., dereference pointer variable only if it’s not NULL.
Characteristics of a Null Pointer in C Language:
- Initialization: A null pointer is typically initialized using the macro NULL, defined in several standard libraries, like <stddef.h>, <stdio.h>, <stdlib.h>, and others.
- Comparison: A null pointer can be compared against other pointers. It is often used in conditions to check whether a pointer is valid.
- Assignment: Any pointer can be assigned NULL.
- Dereferencing: Dereferencing a null pointer leads to undefined behavior, often resulting in a runtime error or a program crash.
Null Pointer in C Language:
The pointer variable, initialized with the null value, is called the Null Pointer. Null Pointer doesn’t point to any memory location until we are not assigning the address. The size of the Null pointer is also 2 bytes, according to the DOS Compiler.
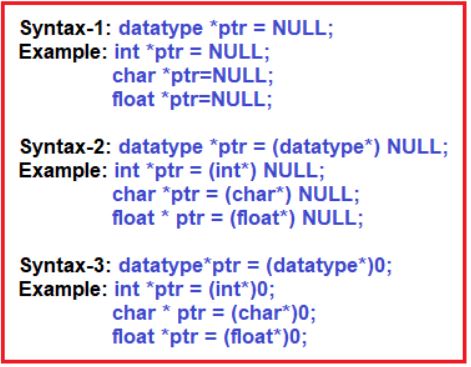
Example Usage of Null Pointers
Here’s a simple example demonstrating the use of null pointers:
When to Use Null Pointers in C Language?
- Initialization: Initialize pointers to NULL when they are declared but not yet assigned to a specific memory address. This prevents them from becoming dangling pointers.
- After free: Set pointers to NULL after freeing dynamically allocated memory to prevent dangling pointers.
- Error Handling: Return NULL from functions to indicate an error when the function is supposed to return a pointer.
- End of Data Structures: In linked lists, trees, and similar data structures, null pointers often signify the end of the structure or an empty node.
- Conditional Checks: Check whether a pointer is NULL before dereferencing it to ensure that it points to valid memory.
Example: Initializing and Checking a Null Pointer
In this example, ptr is initialized to NULL and then checked. The program will print “The pointer is null.”
Example: Using Null Pointers in Function Return
A common use case for null pointers is in functions that return pointers. A null pointer can signal an error or a special condition.
Here, allocateMemory returns NULL if memory allocation fails. The main function checks the returned pointer and prints a message accordingly.
Example: Null Pointers in Linked Lists
Null pointers are frequently used in data structures like linked lists to mark the end of the list.
In this example, head is a null pointer indicating that the linked list is initially empty.
Key Points:
- A null pointer does not point to any valid memory location.
- It’s a good practice to initialize pointers to NULL until they are assigned a valid address.
- Always check if a pointer is null before dereferencing it to avoid runtime errors.
- Null pointers are a key part of many data structures and algorithms in C.
Null Pointer use Cases in C Language:
When we do not assign any memory address to the pointer variable..
In the below example, we declare the pointer variable *ptr, but it does not contain the address of any variable. The dereferencing of the uninitialized pointer variable will show the compile-time error as it does not point to any variable. The following C program shows some unpredictable results and causes the program to crash. Therefore, we can say that keeping an uninitialized pointer in a program can cause the program to crash.
How do we avoid the above problem?
We can avoid the problem in C Programming Language by using a Null pointer. A null pointer points to the 0th memory location, a reserved memory that cannot be dereferenced. In the below example, we create a pointer *ptr and assign a NULL value to the pointer, which means that it does not point to any variable. After creating a pointer variable, we add the condition in which we check whether the value of a pointer is null or not.
When we use the malloc() function?
In the below example, we use the built-in malloc() function to allocate the memory. If the malloc() function is unable to allocate the memory, then it returns a NULL pointer. Therefore, it is necessary to add the condition to check whether the value of a pointer is null. If the value of a pointer is not null, it means that the memory is allocated.
Note: It is always a good programming practice to assign a Null value to the pointer when we do not know the exact address of memory.
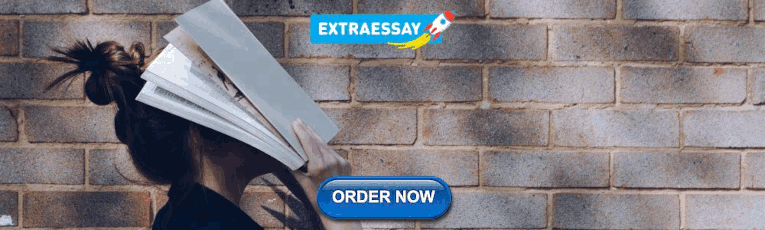
Applications of Null Pointer
Following are the applications of a Null pointer:
- It is used to initialize the pointer variable when the pointer does not point to a valid memory address.
- It is used to perform error handling with pointers before dereferencing the pointers.
- It is passed as a function argument and returned from a function when we do not want to pass the actual memory address.
In the next article, I will discuss Void Pointer in C Language with Examples. In this article, I try to explain Null Pointers in C Language with Examples . I hope you enjoy this Null Pointer in C Language with Examples article. I would like to have your feedback. Please post your feedback, questions, or comments about this article.
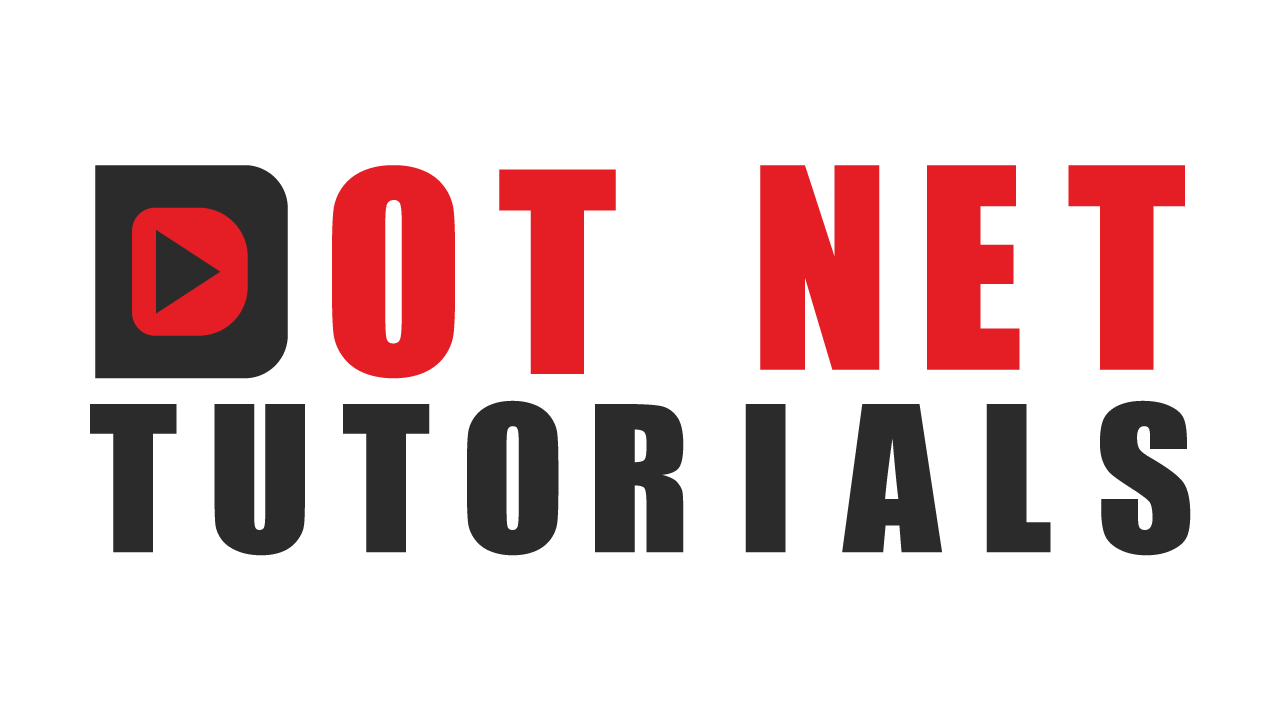
About the Author: Pranaya Rout
Pranaya Rout has published more than 3,000 articles in his 11-year career. Pranaya Rout has very good experience with Microsoft Technologies, Including C#, VB, ASP.NET MVC, ASP.NET Web API, EF, EF Core, ADO.NET, LINQ, SQL Server, MYSQL, Oracle, ASP.NET Core, Cloud Computing, Microservices, Design Patterns and still learning new technologies.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *

12.8 — Null pointers
In the previous lesson ( 12.7 -- Introduction to pointers ), we covered the basics of pointers, which are objects that hold the address of another object. This address can be dereferenced using the dereference operator (*) to get the object at that address:
The above example prints:
In the prior lesson, we also noted that pointers do not need to point to anything. In this lesson, we’ll explore such pointers (and the various implications of pointing to nothing) further.
Null pointers
Besides a memory address, there is one additional value that a pointer can hold: a null value. A null value (often shortened to null ) is a special value that means something has no value. When a pointer is holding a null value, it means the pointer is not pointing at anything. Such a pointer is called a null pointer .
The easiest way to create a null pointer is to use value initialization:
Best practice
Value initialize your pointers (to be null pointers) if you are not initializing them with the address of a valid object.
Because we can use assignment to change what a pointer is pointing at, a pointer that is initially set to null can later be changed to point at a valid object:
The nullptr keyword
Much like the keywords true and false represent Boolean literal values, the nullptr keyword represents a null pointer literal. We can use nullptr to explicitly initialize or assign a pointer a null value.
In the above example, we use assignment to set the value of ptr2 to nullptr , making ptr2 a null pointer.
Use nullptr when you need a null pointer literal for initialization, assignment, or passing a null pointer to a function.
Dereferencing a null pointer results in undefined behavior
Much like dereferencing a dangling (or wild) pointer leads to undefined behavior, dereferencing a null pointer also leads to undefined behavior. In most cases, it will crash your application.
The following program illustrates this, and will probably crash or terminate your application abnormally when you run it (go ahead, try it, you won’t harm your machine):
Conceptually, this makes sense. Dereferencing a pointer means “go to the address the pointer is pointing at and access the value there”. A null pointer holds a null value, which semantically means the pointer is not pointing at anything. So what value would it access?
Accidentally dereferencing null and dangling pointers is one of the most common mistakes C++ programmers make, and is probably the most common reason that C++ programs crash in practice.
Whenever you are using pointers, you’ll need to be extra careful that your code isn’t dereferencing null or dangling pointers, as this will cause undefined behavior (probably an application crash).
Checking for null pointers
Much like we can use a conditional to test Boolean values for true or false , we can use a conditional to test whether a pointer has value nullptr or not:
The above program prints:
In lesson 4.9 -- Boolean values , we noted that integral values will implicitly convert into Boolean values: an integral value of 0 converts to Boolean value false , and any other integral value converts to Boolean value true .
Similarly, pointers will also implicitly convert to Boolean values: a null pointer converts to Boolean value false , and a non-null pointer converts to Boolean value true . This allows us to skip explicitly testing for nullptr and just use the implicit conversion to Boolean to test whether a pointer is a null pointer. The following program is equivalent to the prior one:
Conditionals can only be used to differentiate null pointers from non-null pointers. There is no convenient way to determine whether a non-null pointer is pointing to a valid object or dangling (pointing to an invalid object).
Use nullptr to avoid dangling pointers
Above, we mentioned that dereferencing a pointer that is either null or dangling will result in undefined behavior. Therefore, we need to ensure our code does not do either of these things.
We can easily avoid dereferencing a null pointer by using a conditional to ensure a pointer is non-null before trying to dereference it:
But what about dangling pointers? Because there is no way to detect whether a pointer is dangling, we need to avoid having any dangling pointers in our program in the first place. We do that by ensuring that any pointer that is not pointing at a valid object is set to nullptr .
That way, before dereferencing a pointer, we only need to test whether it is null -- if it is non-null, we assume the pointer is not dangling.
A pointer should either hold the address of a valid object, or be set to nullptr. That way we only need to test pointers for null, and can assume any non-null pointer is valid.
Unfortunately, avoiding dangling pointers isn’t always easy: when an object is destroyed, any pointers to that object will be left dangling. Such pointers are not nulled automatically! It is the programmer’s responsibility to ensure that all pointers to an object that has just been destroyed are properly set to nullptr .
When an object is destroyed, any pointers to the destroyed object will be left dangling (they will not be automatically set to nullptr ). It is your responsibility to detect these cases and ensure those pointers are subsequently set to nullptr .
Legacy null pointer literals: 0 and NULL
In older code, you may see two other literal values used instead of nullptr .
The first is the literal 0 . In the context of a pointer, the literal 0 is specially defined to mean a null value, and is the only time you can assign an integral literal to a pointer.
As an aside…
On modern architectures, the address 0 is typically used to represent a null pointer. However, this value is not guaranteed by the C++ standard, and some architectures use other values. The literal 0 , when used in the context of a null pointer, will be translated into whatever address the architecture uses to represent a null pointer.
Additionally, there is a preprocessor macro named NULL (defined in the <cstddef> header). This macro is inherited from C, where it is commonly used to indicate a null pointer.
Both 0 and NULL should be avoided in modern C++ (use nullptr instead). We discuss why in lesson 12.11 -- Pass by address (part 2) .
Favor references over pointers whenever possible
Pointers and references both give us the ability to access some other object indirectly.
Pointers have the additional abilities of being able to change what they are pointing at, and to be pointed at null. However, these pointer abilities are also inherently dangerous: A null pointer runs the risk of being dereferenced, and the ability to change what a pointer is pointing at can make creating dangling pointers easier:
Since references can’t be bound to null, we don’t have to worry about null references. And because references must be bound to a valid object upon creation and then can not be reseated, dangling references are harder to create.
Because they are safer, references should be favored over pointers, unless the additional capabilities provided by pointers are required.
Favor references over pointers unless the additional capabilities provided by pointers are needed.
Question #1
1a) Can we determine whether a pointer is a null pointer or not? If so, how?
Show Solution
Yes, we can use a conditional (if statement or conditional operator) on the pointer. A pointer will convert to Boolean false if it is a null pointer, and true otherwise.
1b) Can we determine whether a non-null pointer is valid or dangling? If so, how?
There is no easy way to determine this.
Question #2
For each subitem, answer whether the action described will result in behavior that is: predictable, undefined, or possibly undefined. If the answer is “possibly undefined”, clarify when.
2a) Assigning a new address to a non-const pointer
Predictable.
2b) Assigning nullptr to a pointer
2c) Dereferencing a pointer to a valid object
2d) Dereferencing a dangling pointer
2e) Dereferencing a null pointer
2f) Dereferencing a non-null pointer
Possibly undefined, if the pointer is dangling.
Question #3
Why should we set pointers that aren’t pointing to a valid object to ‘nullptr’?
We can not determine whether a non-null pointer is valid or dangling, and accessing a dangling pointer will result in undefined behavior. Therefore, we need to ensure that we do not have any dangling pointers in our program.
If we ensure all pointers are either pointing to valid objects or set to nullptr , then we can use a conditional to test for null to ensure we don’t dereference a null pointer, and assume all non-null pointers are pointing to valid objects.
Pointers in C / C++ [Full Course] – FREE
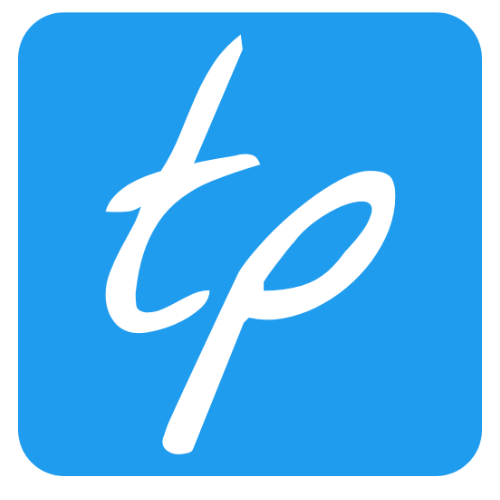
Null Pointer in C/C++
In this Lecture, we will discuss about Null Pointer in C++.
You can allocate memory on the heap using the new operator like this.
It will allocate the memory on the heap and returns a pointer pointing to that memory location. We can initialise our pointer with this value. Now, our pointer ptr points to a memory location on the heap, which contains the integer value. Afterward, we can access that value using the pointer, or we can also change the content of that value using the pointer like this.
After usage, we must delete the allocated memory on the heap. For that, we will use the delete operator to delete the memory like this.
After this line, the memory allocated in the heap will be deleted. However, the pointer ptr will still be pointing to that memory location, which is now an invalid memory location because the memory is already deallocated. So we need to make sure that after the delete operator, the pointer ptr must point to NULL. Like this,
Now pointer ptr is a NULL Pointer. If we don’t assign NULL t pointer, the pointer will be called a dangling pointer ptr. Now, if we try to access any value using this pointer, it can result in an undefined behavior, because pointer ptr is pointing to a memory location that is already deleted. Therefore, we must initialise the pointer to NULL after deleting the memory. The value of NULL is 0.
Also, while accessing the value using a pointer, we should always first check if the memory is valid or not. For that, we can pass the pointer in an if condition. Like this,
If the pointer is pointing to a NULL value, it means it’s not a valid value, and we can’t access the value. However, if the pointer contains the NULL, which is also equivalent to zero, this if condition will fail and will not go to this if block.
When we declare a pointer and don’t initialise it, the default value will be a garbage value. It means the pointer is pointing to some invalid memory location.
Now, if someone tried to access the value through this pointer, it can result in undefined behavior. Therefore, we should always initialise a pointer while declaring it. Either we need to initialise it with a valid address either on its stack or in heap, or if we plan to assign a valid point at a later stage, then we must initialise the pointer with NULL.
While accessing the value, we must check if the given pointer is valid or not like this, and then only should we access the value at that memory location pointed by this pointer.
If you try to delete a null pointer, then it can also result in undefined behaviour, if data type of Object is a User defained data type. Therefore, before deleting a pointer, we should always check if the pointer is valid or not using the if operator. If the pointer is valid or contains a valid memory location, it will return true, and we can delete the memory. However, if the pointer is a null pointer, then the if statement will return false, and we won’t delete the value.
The complete example is as follows,
In this lecture, we learned about Null Pointer in C and C++.
Basic Programs
- Hello World
- Taking Input from User
- Find ASCII Value of Character
- Using gets() function
- Switch Case
- Checking for Vowel
- Reversing Case of Character
- Swapping Two Numbers
- Largest and Smallest using Global Declaration
- Basic for Loop
- Basic while Loop
- Basic do-while Loop
- Nested for Loops
- Program to find Factorial of number
- Fibonacci Series Program
- Palindrome Program
- Program to find Sum of Digits
- Program to reverse a String
- Program to find Average of n Numbers
- Armstrong Number
- Checking input number for Odd or Even
- Print Factors of a Number
- Find sum of n Numbers
- Print first n Prime Numbers
- Find Largest among n Numbers
- Exponential without pow() method
- Find whether number is int or float
- Print Multiplication Table of input Number
- Reverse an Array
- Insert Element to Array
- Delete Element from Array
- Largest and Smallest Element in Array
- Sum of N Numbers using Arrays
- Sort Array Elements
- Remove Duplicate Elements
- Sparse Matrix
- Square Matrix
- Determinant of 2x2 matrix
- Normal and Trace of Square Matrix
- Addition and Subtraction of Matrices
- Matrix Mulitplication
- Simple Program
- Memory Management
- Array of Pointers
- Pointer Increment and Decrement
- Pointer Comparison
- Pointer to a Pointer
- Concatenate Strings using Pointer
- Reverse a String using Pointer
- Pointer to a Function
- Null Pointer
- isgraph() and isprint()
- Removing Whitespaces
- gets() and strlen()
- strlen() and sizeof()
- Frequency of characters in string
- Count Number of Vowels
- Adding Two Numbers
- Fibonacci Series
- Sum of First N Numbers
- Sum of Digits
- Largest Array Element
- Prime or Composite
- LCM of Two Numbers
- GCD of Two Numbers
- Reverse a String
Files and Streams
- List Files in Directory
- Size of File
- Write in File
- Reverse Content of File
- Copy File to Another File
Important Concepts
- Largest of three numbers
- Second largest among three numbers
- Adding two numbers using pointers
- Sum of first and last digit
- Area and Circumference of Circle
- Area of Triangle
- Basic Arithmetic Operations
- Conversion between Number System
- Celsius to Fahrenheit
- Simple Interest
- Greatest Common Divisor(GCD)
- Roots of Quadratic Roots
- Identifying a Perfect Square
- Calculate nPr and nCr
Miscellaneous
- Windows Shutdown
- Without Main Function
- Menu Driven Program
- Changing Text Background Color
- Current Date and Time
Using Null Pointer Program
NULL is a macro in C, defined in the <stdio.h> header file, and it represent a null pointer constant. Conceptually, when a pointer has that Null value it is not pointing anywhere.
If you declare a pointer in C, and don't assign it a value, it will be assigned a garbage value by the C compiler, and that can lead to errors.
Void pointer is a specific pointer type. void * which is a pointer that points to some data location in storage, which doesn't have any specific type.
Don't confuse the void * pointer with a NULL pointer.
NULL pointer is a value whereas, Void pointer is a type.
Below is a program to define a NULL pointer.
Program Output:

Use Null Pointer to mark end of Pointer Array in C
Now let's see a program in which we will use the NULL pointer in a practical usecase.
We will create an array with string values ( char * ), and we will keep the last value of the array as NULL. We will also define a search() function to search for name in the array.
Inside the search() function, while searching for a value in the array, we will use NULL pointer to identify the end of the array.
So let's see the code,
Peter is in the list. Scarlett not found.
This is a simple program to give you an idea of how you can use the NULL pointer. But there is so much more that you can do. You can ask the user to input the names for the array. And then the user can also search for names. So you just have to customize the program a little to make it support user input.
- ← Prev
- Next →
C Tutorial
c mcq tests.

- Design Pattern
- Interview Q
C Control Statements
C functions, c dynamic memory, c structure union, c file handling, c preprocessor, c command line, c programming test, c interview.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

What is Null Pointer in C?
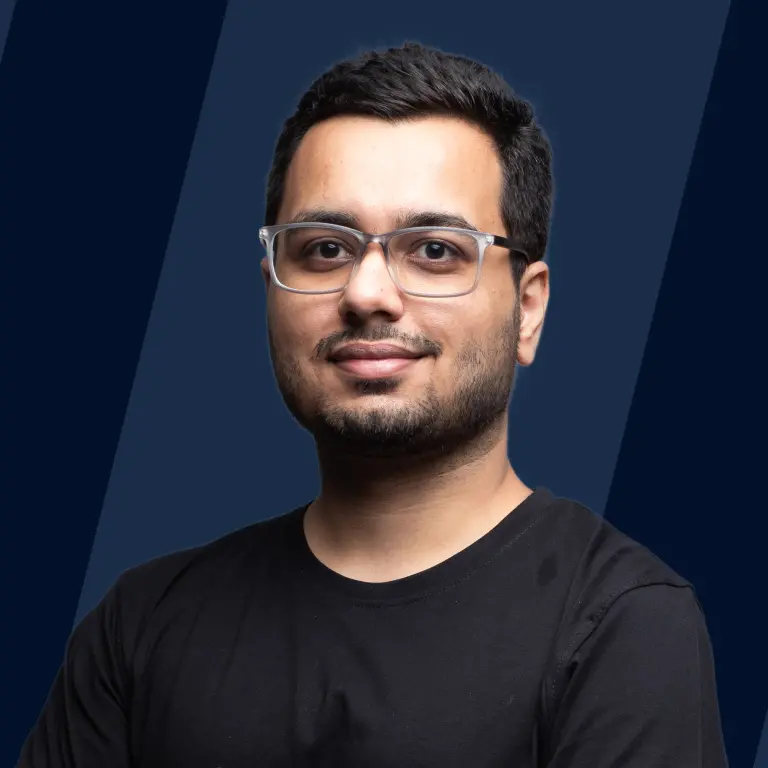
In the C programming language, a null pointer is a pointer that does not point to any memory location and hence does not hold the address of any variables. It just stores the segment's base address. That is, the null pointer in C holds the value Null, but the type of the pointer is void. A null pointer is a special reserved value declared in a header file called stddef . Null indicates that the pointer is pointing to the first memory location. meaning that 0th location. At a high level, NULL is a null pointer that is implemented in C for a multitude of scenarios.
- To initialize a pointer variable when it hasn't been assigned a proper memory address yet.
- Before accessing any pointer variable, check for a null pointer. We can handle errors in pointer-related code this way, for example, only dereference a pointer variable if it is not NULL.
- When we don't want to pass a valid memory address to a function argument, we can pass a null pointer.
generally, we can say that a null pointer is a pointer that does not point to any object.
The syntax of a null pointer can be defined in two ways.
Applications of Null Pointer in C
A Null pointer can be used in the following ways:
- When the pointer variable does not point to a valid memory address, it is used to initialize it.
- Before dereferencing pointers , it is utilized to execute error handling.
- When we don't want to pass the memory location directly, we pass it as a function argument, which is then utilized to return from a function.
Example of Null Pointer in C
We can assign 0 directly to the pointer so that it will not point to any of the memory locations.
Explanation: In the above example, we initialized three pointers as *ptr1 , *ptr2 , and *ptr3 , and we assigned a value to the num variable in *ptr1 and compared it by 0 because *ptr1 is not equal to null, therefore it would output the result as NOT NULL, and we did not assign any value to *ptr2 and As a result, the output will be printed as NOT NULL. We assigned value 0 to *ptr3 , which is equal to null, hence the output will be NULL.
Some More Examples
Example 1. this example illustrates inappropriate use of the null constant for overloaded functions.
Assume you want func(int* i) to be called from the main function. Because the constant NULL is equal to the integer 0, the main function calls func(int i) instead of func(int* i) Only when func(int i) do not not exist is constant 0 implicitly turned to (void*)0 .
Example 2. This Example Illustrates How nullptr is Used in Overloading Functions
Example 3. the following expressions illustrate the correct and incorrect use of the nullptr constant, example 4. this example illustrates that a non-type template parameter or argument can have the std::nullptr_t type, example 5. this example illustrates how to use nullptr in exception handling, how does null pointer work in c.
In C, a null pointer is a variable that has no valid address and is allocated to zero or NULL. Normally, the null pointer does not point to anything. NULL is a macro constant defined in several header files in the C programming language, including stdio.h , alloc.h , mem.h , stddef.h , and stdlib.h . Also, take note that NULL should only be used when working with pointers.
Best Practices for NULL Pointer Usage
To avoid programming errors, use a NULL pointer as a best practice.
- Before using a pointer, make it a practice to assign it a value. Don't utilize the pointer before it's been initialized.
- If you don't have a valid memory location to store in a pointer variable, set it to NULL instead.
- Check for a NULL value before utilizing a pointer in any of your function code.
What are the Uses of NULL Pointer in C?
Avoid crashing a program.
Our program may crash if we pass any garbage value in our code or to a certain method. We can avoid this by using a NULL pointer.
To avoid crasing in a program we can write the code like this:
Explanation: We define function fun1() in the following code and pass a pointer ptrvarA to it. When the function fun1() is called, it checks whether the passed pointer is a null pointer or not. So we must verify if the pointer's passed value is null or not , because if it is not set to any value, it will take the garbage value and terminate your program, resulting in the program crashing.
While Freeing (de-allocating) Memory
Assume you have a pointer pointing to a memory location where data is stored. If you don't need the data any longer, you should remove it and free up some memory .
However, even after the data has been freed, the pointer still points to the same memory location. A dangling pointer is a name given to this type of pointer. Set the pointer to NULL to get rid of the dangling pointer .
NULL Pointer Uses in Linked-List
The Data and Link fields are contained in every node. The data field holds the actual data that we intend to save in the linked list. The link connects each node to the other nodes. The head of the Linked List has a pointer link to the first node. Because it does not point to another node in the Linked List, the last node-link has a null pointer. This null variable is updated with the pointer of the new node when we add a new node at the end of the Linked List.
In Linked List, a NULL pointer is also significant. We know that in a Linked List, we use a pointer to connect one node to its successor node.
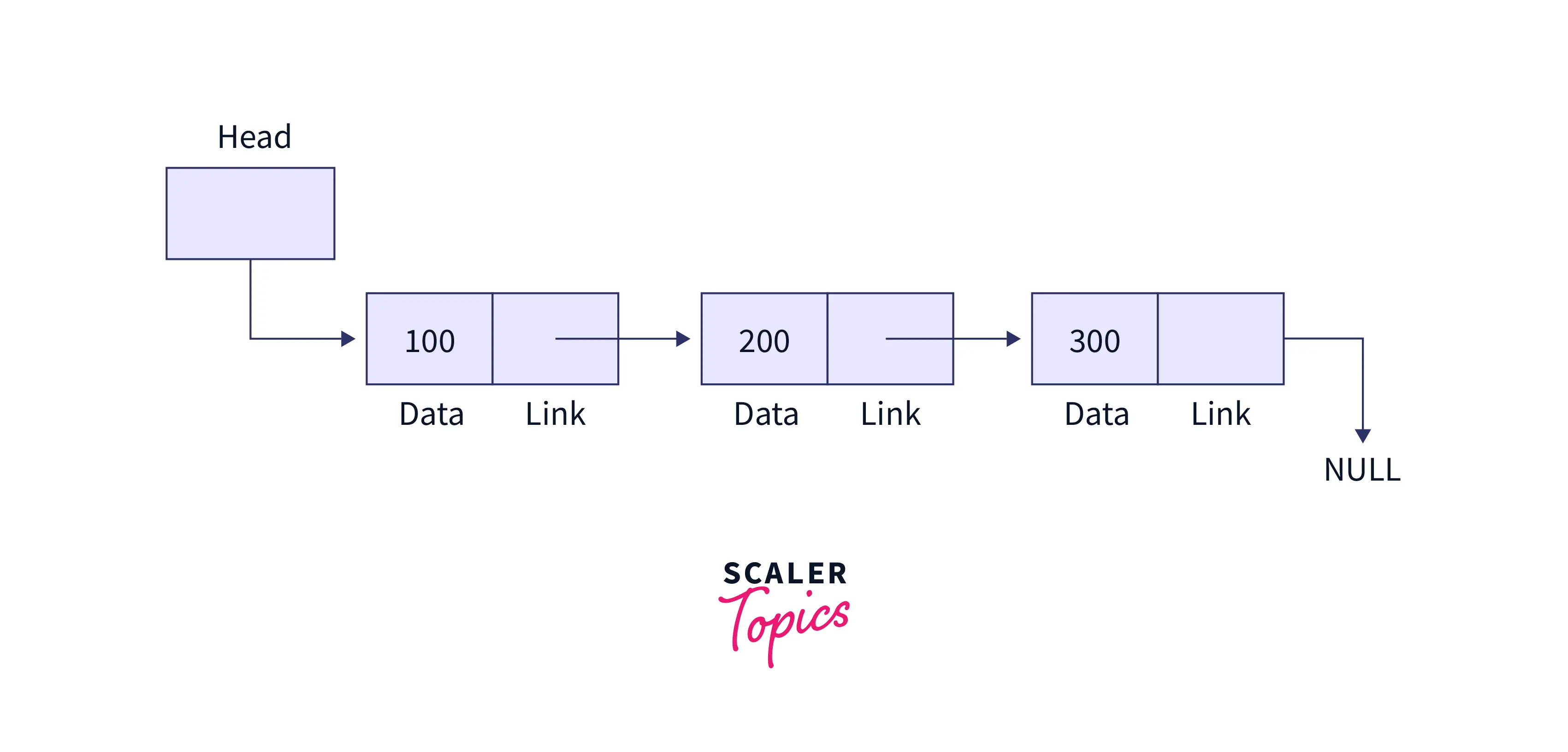
Because there is no successor node for the last node, the link of the last node must be set to NULL.
Examples to Implement Null Pointer in C
Example - 1:
Example - 2:
Example - 3:
Example - 4:
Null Pointer Use Cases in C Language
When we do not assign any memory address to the pointer variable.
In the example below, we declared the pointer variable *ptr , but it has no variable address. Dereferencing the uninitialized pointer variable will result in a compile-time error because it does not point to any variable. The C program that follows creates a variety of spectacular results before ultimately crashing. As an outcome, we can deduce that a program can crash if an uninitialized pointer is left in it.
How to Avoid the Above Problem?
We can overcome the above problem in the C programming language by utilizing a Null pointer. A null pointer refers to the 0th memory location , which is a shared memory area that can't be dereferenced. In the example below, We create a pointer *ptr and assign it the value NULL to indicate that it doesn't point to any variables. We add a condition after creating a pointer variable that checks if the value of the pointer is null.
When We Use the malloc() Function
The built-in malloc() method is used to allocate memory in the example below. If the malloc() function fails to allocate memory, the result is a NULL pointer . As an outcome, a condition to check whether the value of a pointer is null or not must be included; if the value of a pointer is not null, memory must be allocated.
It's usually good programming practice to set a Null value to the pointer when we don't know the actual memory address.
Difference between NULL and Void Pointer
Learn more about Pointers in C.
- Pointers in C
- When we don't know the actual address of memory, it's always useful to set a Null value to the pointer.
- A Null pointer is a variable in the C programming language that has a value of zero or has an address that points to nothing.
- In C, we use the keyword NULL to make a variable a null pointer, which is a predefined macro.
- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
- Application of Pointer in C++
- weak_ptr in C++
- void Pointer in C++
- Pointer to an Array in C++
- String Functions In C++
- Using Range in C++ Switch Case
- User-Defined Data Types In C
- Nested Try Blocks in C++
- C++23 <print> Header
- Macros In C++
- std::future in C++
- How to Define Constants in C++?
- Decision Making in C++
- Polymorphic Exceptions In C++
- String Tokenization in C
- auto_ptr in C++
- C++ Global Variables
- Unique_ptr in C++
- Partial Template Specialization in C++
NULL Pointer in C++
A NULL Pointer in C++ indicates the absence of a valid memory address in C++. It tells that the pointer is not pointing to any valid memory location In other words, it has the value “NULL” (or ‘ nullpt r’ since C++11). This is generally done at the time of variable declaration to check whether the pointer points to some valid memory address or not. It is also returned by several inbuilt functions as a failure response.
Trying to dereference a NULL pointer i.e. trying to access the memory it points to leads to some undefined behavior leading to the program crash.
Syntax of Null Pointer in C++
We can create a NULL pointer of any type by simply assigning the value NULL to the pointer as shown:
A null pointer is represented by the value 0 or by using the keyword NULL. With the new versions of C++ like C++11 and later, we can use “nullptr” to indicate a null pointer.
Checking NULL Pointer
We can check whether a pointer is a NULL pointer by using the equality comparison operator.
The above expression will return true if the pointer is a NULL pointer. False otherwise.
Applications of Null Pointer in C++
Null Pointer finds its applications in the following scenarios:
- Initialization: It is a good practice to Initialize pointers to a null value as it helps avoid undefined behavior by explicitly indicating they are not pointing to valid memory locations.
- Default Values: Null pointers act as default or initial values for pointers when no valid address is assigned to the pointers.
- Error Handling: They are useful in error conditions or to signify the absence of data that enables better handling of exceptional cases.
- Resource Release: To release the resources, like the destructor of a class, or to set pointers to NULL after deletion we can use a null pointer to avoid accidentally using or accessing the released memory.
- Sentinel Values : A null pointer can be used to indicate the end of a data structure or a list like in the linked list last node has a null pointer as the next field.
Example of NULL Pointer in C++
The below example demonstrates the dereferencing and assignment of a null pointer to another value.
Explanation : In the example given above first the pointer is pointing to a null value. First, we check whether the pointer is pointing to a null value or not before dereferencing it to avoid any kind of runtime error. Then we assign the pointer a valid memory address and then check it before dereferencing it. As the pointer is not pointing to a null value, the else part is executed.
Disadvantages of NULL Pointers in C++
NULL pointer makes it possible to check for pointer errors but it also has its limitations:
- Dereferencing a NULL pointer causes undefined behavior that may lead to runtime errors like segmentation faults.
- We need to check explicitly for NULL pointers before dereferencing it to avoid undefined behavior.
It is important to understand null pointers in C++ to handle pointers safely and prevent unexpected runtime errors. They signify the absence of valid memory addresses and help in error handling and pointer initialization. Proper usage and precautions regarding null pointers are essential in writing error-free C++ code.
Please Login to comment...
Similar reads.
- cpp-pointer
- Geeks Premier League 2023
- Geeks Premier League
- 10 Best Free Note-Taking Apps for Android - 2024
- 10 Best VLC Media Player Alternatives in 2024 (Free)
- 10 Best Free Time Management and Productivity Apps for Android - 2024
- 10 Best Adobe Illustrator Alternatives in 2024
- 30 OOPs Interview Questions and Answers (2024)
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
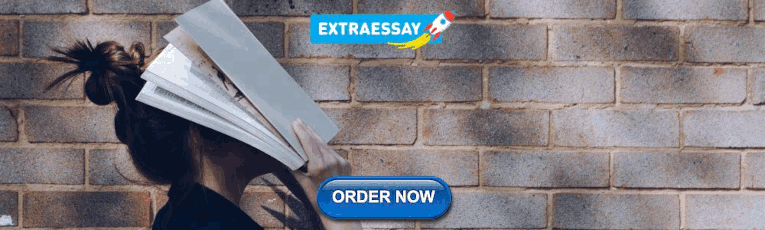
IMAGES
VIDEO
COMMENTS
If you want to change the pointer inside the function you need to pass the actual pointer as a pointer, i.e. a pointer to a pointer: void my_function(char **a) {. *a = NULL; } Use the address-of operator & when you call the function to get the address of the pointer: my_function(&ptr); answered Apr 30, 2013 at 12:01. Some programmer dude.
Syntax of Null Pointer Declaration in C type pointer_name = NULL; type pointer_name = 0;. We just have to assign the NULL value. Strictly speaking, NULL expands to an implementation-defined null pointer constant which is defined in many header files such as "stdio.h", "stddef.h", "stdlib.h" etc. Uses of NULL Pointer in C
After using free(ptr), it's always advisable to nullify the pointer variable by declaring again to NULL . e.g.: free(ptr); ptr = NULL; If not re-declared to NULL, the pointer variable still keeps on pointing to the same address ( 0x1000 ), this pointer variable is called a dangling pointer .
They can be created by assigning a NULL value to the pointer. A pointer of any type can be assigned the NULL value. Syntax. data_type * pointer_name = NULL; or pointer_name = NULL. It is said to be good practice to assign NULL to the pointers currently not in use. 7. Void Pointer. The Void pointers in C are the pointers of type void. It means ...
C++ Server Side Programming Programming C. A null pointer is a pointer which points nothing. Some uses of the null pointer are: a) To initialize a pointer variable when that pointer variable isn't assigned any valid memory address yet. b) To pass a null pointer to a function argument when we don't want to pass any valid memory address.
A pointer value can be null, which means it does not point to any object. The cleanest way to get a null pointer is by writing NULL, a standard macro defined in stddef.h. You can also do it by casting 0 to the desired pointer type, as in (char *) 0. (The cast operator performs explicit type conversion; See Explicit Type Conversion .)
NULL is a constant whose value is zero (0). We can create a NULL Pointer by assigning NULL or zero (0) to the pointer variable. Syntax -: pointer_name -: Pointer name you can keep anything according to you. NULL -: Here NULL is a keyword which we assign to pointer variable to make NULL Pointer. Example -:
Pointer to a null pointer is valid. Explanation: What happens here is that when a Null pointer is created, it points to null, without any doubt. But the variable of Null pointer takes some memory. Hence when a pointer to a null pointer is created, it points to an actual memory space, which in turn points to null.
NULL Pointers. A NULL pointer is a pointer that lacks a reference to a valid memory location. It's typically used to indicate that a pointer doesn't have a specific memory address assigned, often serving as a placeholder or default value for pointers. Here's a code example that demonstrates the use of a NULL pointer:
A null pointer points to the 0th memory location, a reserved memory that cannot be dereferenced. In the below example, we create a pointer *ptr and assign a NULL value to the pointer, which means that it does not point to any variable. After creating a pointer variable, we add the condition in which we check whether the value of a pointer is ...
Explanation: In the above-modified code, we assign a pointer_var to the "NULL" value and we check with the condition if the value of the pointer is null or not. In most of the operating system, codes or programs are not allowed to access any memory which has its address as 0 because the memory with address zero 0is only reserved by the operating system as it has special importance, which ...
A null value (often shortened to null) is a special value that means something has no value. When a pointer is holding a null value, it means the pointer is not pointing at anything. Such a pointer is called a null pointer. The easiest way to create a null pointer is to use value initialization:
Now pointer ptr is a NULL Pointer. If we don't assign NULL t pointer, the pointer will be called a dangling pointer ptr. Now, if we try to access any value using this pointer, it can result in an undefined behavior, because pointer ptr is pointing to a memory location that is already deleted. Therefore, we must initialise the pointer to NULL ...
The macro NULL is a null-pointer constant and has either an integer type or a pointer type. Using NULL to assign or initialize a non-pointer variable will lead to question marks from other programmers at the least and it might result in compiler failures. A line like. int a = NULL; is not considered good code and it will make the code less ...
Using Null Pointer Program. NULL is a macro in C, defined in the <stdio.h> header file, and it represent a null pointer constant. Conceptually, when a pointer has that Null value it is not pointing anywhere. If you declare a pointer in C, and don't assign it a value, it will be assigned a garbage value by the C compiler, and that can lead to ...
In C. C is a different beast. In C NULL can be defined as 0 or as ((void *)0), C99 allows for implementation defined null pointer constants. So it actually comes down to the implementation's definition of NULL and you will have to inspect it in your standard library.
To prevent dangling pointers, assign NULL to a pointer after releasing the memory it refers to. Dereference attempts are safe operations that guard against potential issues brought on by the use of dangling pointers. Interoperability with Libraries: C libraries and APIs frequently employ NULL Pointers.
C implementations can define NULL either as ((void*)0) the outer parentheses are necessary) or as 0, or in any of several other forms. C++, which is a different language, has different requirements for null pointer constants, and C++ implementations commonly define NULL as 0. The point of having NULL define by the implementation is that you don ...
In C, a null pointer is a variable that has no valid address and is allocated to zero or NULL. Normally, the null pointer does not point to anything. NULL is a macro constant defined in several header files in the C programming language, including stdio.h, alloc.h, mem.h, stddef.h, and stdlib.h. Also, take note that NULL should only be used ...
We can create a NULL pointer of any type by simply assigning the value NULL to the pointer as shown: int* ptrName = NULL; // before C++11. int* ptrName = nullptr. int* ptrName = 0; // by assigning the value 0. A null pointer is represented by the value 0 or by using the keyword NULL. With the new versions of C++ like C++11 and later, we can use ...
a is type of XY and s is a pointer of this class that points to null. How can I program the assignment-operator so that if s is a null pointer, that a.p=0? c++; Share. Improve this question. Follow asked Sep 1, 2023 at 13:51. Stefan ... References cannot be null in C++, only pointers can be.