- Entrepreneurship
- 3D & Animation
- Deep Learning
- Machine Learning
- Game Development
- Computer Vision
- Data Analysis
- Software Testing
- Spring Framework
- Visual Studio
- Web Development
- Ethical Hacking
- IT Certification
- Network & Security
- Content Marketing
- Digital Marketing
- Social Media Marketing
- Memory & Study Skills
- Photography
- Engineering
Free Educational Tutorials
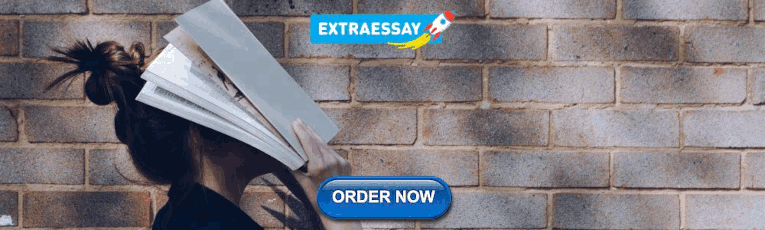
Algorithms in Java :Live problem solving & Design Techniques
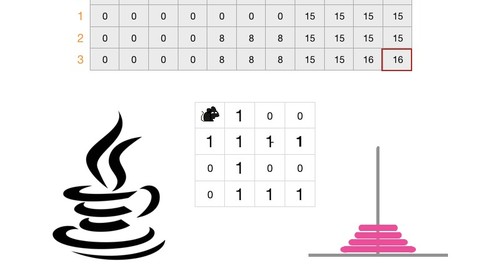
Description
Algorithm Design Techniques: Live problem-solving in Java
Algorithms are everywhere. One great algorithm applied sensibly can result in a System like GOOGLE!
Completer scientists have worked from 100s of years and derived some of the techniques that can be applied to write and design algorithms.
So Why to reinvent the wheel ??
Let’s go through some of the most famous algorithm design techniques in this course.
Once you will come to know these design techniques It will become very easy for you to approach a problem by identifying which technique to apply to solve that correctly and efficiently.
0. Complexity analysis
1. Recursion is the base of any algorithm design
2. Backtracking
3. Divide and Conquer
4. Greedy algorithms
5. Dynamic programming
And WE WILL WRITE THE CODE LINE BY LINE IN JAVA !!
By the end of this course –
1. You will understand how to design algorithms
2. A lot of coding practice and design live problems in Java
3. Algorithm Complexity analysis
If you are preparing for your coding Interview or doing competitive programming This course will be a big help for you.
THRILLED? I welcome you to the course and I am sure this will be fun!!
If it does not – It comes with a 30 Days money-back guarantee so don’t think twice to give it a shot.
Happy Learning
Basics>Strong;
Who this course is for:
- Looking to learn to design algorithms
- Want to develop how to think solve algo problems
- Code Famous Algorithms in Java
Requirements
- Basic Java – Loops and Conditions
Last Updated 1/2021
Download Links
Direct Download
Algorithms in Java :Live problem solving & Design Techniques.zip (4.4 GB) | Mirror
Torrent Download
Algorithms in Java :Live problem solving & Design Techniques.torrent (153 KB) | Mirror
Source: https://www.udemy.com/course/algorithm-design-techniques-live-problem-solving-in-java/
You might also like
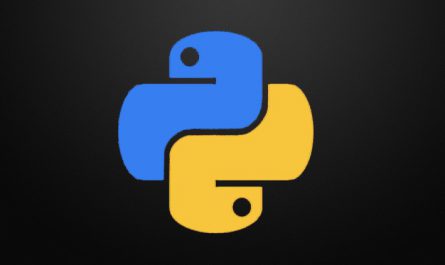
Python GUI Development with PyQt6 & Qt Designer
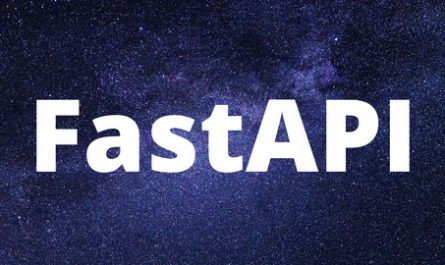
FastAPI Full Stack Web Development (API + Webapp)
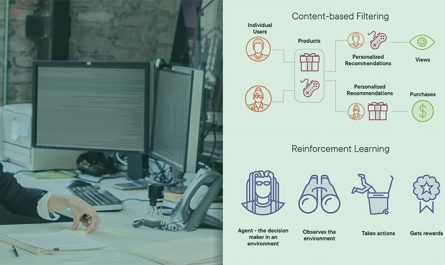
Machine Learning Literacy – Practical Application
Leave a reply cancel reply.
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
Notify me of follow-up comments by email.
Notify me of new posts by email.

Algorithms in Java - Tutorial
1.1. motivation, 1.2. what is an algorithm, 1.3. complexity, 2.1. quicksort, 2.2. mergesort, 2.3. standard java array sorting, 2.4. testing the sort algorithm, 3.2. typical graph problems.
Algorithms in Java. This article describes some very common algorithm in Java.
1. Algorithm
It could be argued that for most problems someone else has already written an implementation of an algorithm which solves this problem. So rather by copying the implementation and using it you can also solve the problem. While this argumentation is true and while code reuse is important, I believe that having a sound understanding of the basis algorithms for standard problems helps to solve other analytical problems. So please see this article as a kind of brain teaser. Understanding algorithms can be very rewarding.
In addition everybody has to sort Arrays or Lists, so I believe it is important to at least understand the complexity for this problem and the available implementations of these.
An algorithm describes a way of solving a specific problem.
The complexity of an algorithm is dependent on the problem and on the algorithm itself. It can be described using the Big-O notation. An algorithm is said to have a complexity of O(n) if the runtime of solving a problem with this algorithm, depends on the number of elements (n) of this problem. For example a sort algorithm for a list with n elements is said to have the complexity of O(n^2) if the runtime of this algorithm increases exponentially with the number of elements.
A problem is said to be NP-hard (nondeterministic polynomial-time hard) if no algorithm exists which solves the problem in polynomial time.
2. Sort in Java
Sort algorithms are ordering the elements of a list according to a certain order. For the Java examples I will assume that we are sorting an array of integers. The examples for this chapter will be created in a Java project "de.vogella.algorithms.sort". The sorting algorithm will implement the following interface.
Quicksort is a fast, recursive, non-stable sort algorithm which works by the divide and conquer principle. It has in average O(n log (n)) and in the worst case O(n2) complexity. Quicksort selects first a pivot elements. It then divides the elements of the list into two lists based on this pivot element. Every element which is smaller than the pivot element is placed in the left list and every element which is larger is placed in the right list. If an element is equal to the pivot element then it can go in any list. After this sorting the algorithm is then called recursively for the left and right list. If a list contains only one (or zero) elements then it sorted. Quicksort does not copy any data it just exchanges the values in the array therefore it does not consume a lot of memory (only on the call stack for the recursive call of the function. The disadvantages of Quicksort is that it does not work well on already sorted lists or an lists with lots of similar values.
Mergesort is a fast, recursive, stable sort algorithm which works by the divide and conquer principle. The algorithm has a complexity of O(n log (n)). Similar to Quicksort the list of elements which should be sorted is divided into two lists. These lists are sorted independently and then combined. During the combination of the lists the elements are inserted (or merged) on the correct place in the list. Merge can be implemented in several ways.
The simplest way of merge is the following :
Assume the size of the left array is k, the size of the right array is m and the size of the total array is n (=k+m).
Create a helper array with the size n
Copy the elements of the left array into the left part of the helper array. This is position 0 until k-1.
Copy the elements of the right array into the right part of the helper array. This is position k until m-1.
Create an index variable i=0; and j=k+1
Loop over the left and the right part of the array and copy always the smallest value back into the original array. Once i=k all values have been copied back the original array. The values of the right array are already in place.
Compared to quicksort the mergesort algorithm puts less effort in dividing the list but more into the merging of the solution.
Standard mergesort does require a copy of the array although there are (complex) implementations of mergesort which allow to avoid this copying.
Java offers a standard way of sorting Arrays with Arrays.sort(). This sort algorithm is a modified quicksort which shows more frequently a complexity of O(n log(n)). See the Javadoc for details.
Here is a small unit test which you can use to test the sort algorithms.
A graph is made out of nodes and directed edges which define a connection from one node to another node. A node (or vertex) is a discrete position in a graph. Edges can be directed or undirected. Edges have an associated distance (also called costs or weight). The distance between two nodes a and b is labeled as [a,b]. The mathematical description for graphs is G= {V,E}, meaning that a graph is defined by a set of vertexes (V) and a collection of edges. The "order" of a graph is the number of nodes. The "size" of a graph is the number of edges.
Typical graph problems are:
finding the shortest path from a specific node to another node
finding the maximum possible flow through a network where each edge has a pre-defined maximum capacity (maximum-flow minimum-cut problem)
4. Links and Literature
Excellent Java Book with a very good part on Algorithms and Data Structures
German Page over Algorithms with an implementation in Java
Sort Algorithms described
If you need more assistance we offer Online Training and Onsite training as well as consulting
See License for license information .
Algorithms in Java :Live problem solving & Design Techniques
Course comparison
See Better Alternatives
Skills related
People often say.
Simple Learning
Applied fundamentals and worthy instructor
Good and logic Introduction
High Quality and easy Material
Recommended, Engaging and Informative
This course provides a comprehensive overview of algorithms, with a focus on complex problem solving in Java algorithms. Additionally, various algorithm design techniques are discussed, making the course a wealth of information for coder/developers. The purpose of this course is to give the coder/developer the opportunity to learn these design techniques and make the process easier. It will help to get ahead of the game and solve problems before they arise.
Course Introduction
Introduction to Algorithms
Complexity Analysis
AI review summary
Most favorable.
Students appreciate the course as it provides practical knowledge on solving various problems. They find the topics on time and space complexity solutions helpful. The course content is considered good when attached to the product.
Related content for you
No items found.
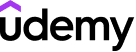
This course includes
SkillMapper rating :
Start date :
Amount of students :
Downloadable resources :
Certificate of completion :
© SkillMapper SAS
Privacy Policy
Terms and use

Data Structures and Algorithms with Java

This course, “Data Structures and Algorithms with Java” is designed to provide an in-depth understanding of data structures and algorithms and how to implement them using Java. Whether you’re a beginner or an experienced programmer, this course will provide you with the knowledge and skills you need to become proficient in data structures and algorithms.
Throughout the course, you’ll learn about a wide range of data structures including arrays, stacks, queues, linked lists, skip lists, hash tables, binary search trees, Cartesian trees, B-trees, red-black trees, splay trees, AVL trees, and KD trees. Additionally, you’ll learn about a wide range of algorithms such as Quicksort, Mergesort, Timsort, Heapsort, bubble sort, insertion sort, selection sort, tree sort, shell sort, bucket sort, radix sort, counting sort, and cubesort.
As you progress through the course, you’ll also learn about algorithm design techniques such as greedy algorithms, dynamic programming, divide and conquer, backtracking, and randomized algorithms. To help you apply and practice the concepts you learn, the course includes hands-on exercises and examples. The course will also cover the Time and Space Complexity of the algorithm and Data Structures, so that you can understand the trade-offs of choosing one over the other.
By the end of the course, you’ll have a solid understanding of data structures and algorithms and how to use them effectively in Java. This course is perfect for anyone who wants to improve their skills as a developer or prepare for a career in computer science or data science.
If you’re ready to begin your journey towards mastering data structures and algorithms with Java, this course is perfect for you, Sign up now and start your journey towards mastering data structures and algorithms with Java.
Course Content
About instructor.

Yasin Cakal

Course Includes
- Course Certificate

Email Address
Remember Me
Registration confirmation will be emailed to you.
Introduction to Algorithm Design Techniques
Understand different algorithm design techniques with the help of relevant examples.
Exhaustive search algorithms
- Branch-and-bound algorithms
- Greedy algorithms
- Dynamic programming algorithms
- Recursive algorithms
- Divide-and-conquer algorithms
- Randomized algorithms
Over the last half a century, computer scientists have discovered that many algorithms share similar ideas, even though they solve very different problems. For now, we’ll mention the most common algorithm design techniques so that future examples can be categorized in terms of the algorithm’s design methodology.
To illustrate the design techniques, we’ll consider a very simple problem that plagued nearly everyone before the era of mobile phones, when people used cordless phones. Suppose your cordless phone rings, but you’ve misplaced the handset somewhere in your home. How do you find it? To complicate matters, you’ve just walked into your home with an armful of groceries, and it’s dark out, so you cannot rely solely on eyesight.
In an exhaustive search, or brute force, the algorithm examines every possible alternative to find one particular solution.
Level up your interview prep. Join Educative to access 70+ hands-on prep courses.
Algorithms in Java :Live problem solving & Design Techniques

Share this page
Rating 4.0 out of 5 (90 ratings in Udemy)
- Algorithm Design in Computer Systems
- Algorithm Design Techniques
- How to Code a Algo in Java
- Calculate time and space complexity
- Dynamic Programming, Greedy, D&C and Much more
Algorithm Design Techniques: Live problem-solving in Java
Algorithms are everywhere. One great algorithm applied sensibly can result in a System like GOOGLE!
Completer scientists have worked from 100s of years and derived some of the …
Completer scientists have worked from 100s of years and derived some of the techniques that can be applied to write and design algorithms.
So Why to reinvent the wheel ??
Let’s go through some of the most famous algorithm design techniques in this course.
Once you will come to know these design techniques It will become very easy for you to approach a problem by identifying which technique to apply to solve that correctly and efficiently.
0. Complexity analysis
1. Recursion is the base of any algorithm design
2. Backtracking
3. Divide and Conquer
4. Greedy algorithms
5. Dynamic programming
And WE WILL WRITE THE CODE LINE BY LINE IN JAVA !!
By the end of this course -
1. You will understand how to design algorithms
2. A lot of coding practice and design live problems in Java
3. Algorithm Complexity analysis
If you are preparing for your coding Interview or doing competitive programming This course will be a big help for you.
THRILLED? I welcome you to the course and I am sure this will be fun!!
If it does not - It comes with a 30 Days money-back guarantee so don’t think twice to give it a shot.
Happy Learning
Basics>Strong;
Learn Java practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
- Java Expressions, Statements and Blocks
Java Flow Control
- Java if...else Statement
- Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
- Java break Statement
- Java continue Statement
- Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
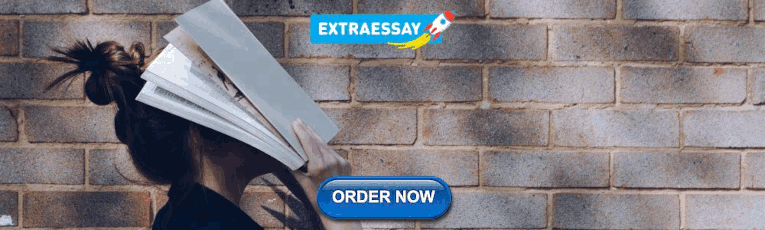
Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
- Java sort()
- Java binarySearch()
- Java ArrayList sort()
- Java ArrayList retainAll()
The Java collections framework provides various algorithms that can be used to manipulate elements stored in data structures.
Algorithms in Java are static methods that can be used to perform various operations on collections.
Since algorithms can be used on various collections, these are also known as generic algorithms .
Let's see the implementation of different methods available in the collections framework.
1. Sorting Using sort()
The sort() method provided by the collections framework is used to sort elements. For example,
Here the sorting occurs in natural order (ascending order). However, we can customize the sorting order of the sort() method using the Comparator interface .
To learn more, visit Java Sorting .
2. Shuffling Using shuffle()
The shuffle() method of the Java collections framework is used to destroy any kind of order present in the data structure. It does just the opposite of the sorting. For example,
When we run the program, the shuffle() method will return a random output.
The shuffling algorithm is mainly used in games where we want random output.
3. Routine Data Manipulation
In Java, the collections framework provides different methods that can be used to manipulate data.
- reverse() - reverses the order of elements
- fill() - replace every element in a collection with the specified value
- copy() - creates a copy of elements from the specified source to destination
- swap() - swaps the position of two elements in a collection
- addAll() - adds all the elements of a collection to other collection
For example,
Note : While performing the copy() method both the lists should be of the same size.
4. Searching Using binarySearch()
The binarySearch() method of the Java collections framework searches for the specified element. It returns the position of the element in the specified collections. For example,
Note : The collection should be sorted before performing the binarySearch() method.
To know more, visit Java Binary Search .
5. Composition
- frequency() - returns the count of the number of times an element is present in the collection
- disjoint() - checks if two collections contain some common element
6. Finding Extreme Values
The min() and max() methods of the Java collections framework are used to find the minimum and the maximum elements, respectively. For example,
Table of Contents
- Sorting Using sort()
- Shuffling Using shuffle()
- Routine Data Manipulation
- Searching Using binarySearch()
- Composition
- Finding Extreme Values
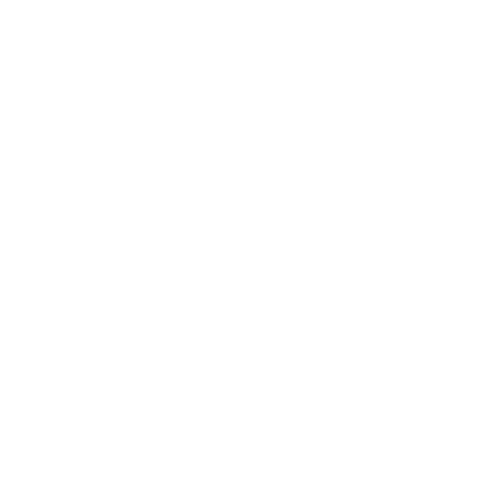
Solving Real-World Problems Using Appropriate Algorithm Design Techniques
Algorithms are at the heart of problem-solving in computer science. They act as step-by-step instructions for solving a specific problem efficiently. From searching and sorting algorithms to graph algorithms and machine learning algorithms, they are used to solve a wide range of real-world problems. In this article, we will explore how appropriate algorithm design techniques can be employed to tackle real-world problems effectively.
Understanding Real-World Problems
Real-world problems are complex and often involve large data sets. It is crucial to deeply understand the problem at hand before attempting to solve it with an algorithm. This involves analyzing the problem's requirements, identifying the input and output constraints, and considering any specific requirements or limitations.
Selecting Appropriate Algorithm Design Techniques
Once we have a clear understanding of the problem, we can start selecting appropriate algorithm design techniques. There are several commonly used algorithm design techniques, including:
Brute Force
The brute force technique involves trying out all possible solutions and selecting the one that meets the problem's requirements. Although this approach can be time-consuming, it is often a good starting point for solving real-world problems.
Greedy Algorithms
Greedy algorithms make locally optimal choices at each step with the hope of reaching a global optimum. They are useful for solving optimization problems where making the best decision at each step leads to the overall best solution. For example, the Dijkstra's algorithm for finding the shortest path in a graph is based on the greedy approach.
Divide and Conquer
The divide and conquer technique breaks down a complex problem into smaller, more manageable subproblems and solves them individually. The solutions to the smaller subproblems are then combined to obtain the solution to the original problem. The merge sort and quicksort algorithms are classic examples of divide and conquer techniques.
Dynamic Programming
Dynamic programming is used to solve problems by breaking them down into overlapping subproblems and solving each subproblem only once. The solutions to the subproblems are stored in a table and can be reused to solve larger problems. The famous Fibonacci sequence calculation using dynamic programming is one such example.
Optimizing Algorithms
Efficiency is a crucial aspect of solving real-world problems using algorithms. Optimizing algorithms can significantly improve their performance. There are various ways to optimize an algorithm, including:
- Analyzing the time complexity and space complexity to identify bottlenecks and areas for improvement.
- Employing data structures that are best suited for the problem at hand, such as hash tables, trees, or graphs.
- Utilizing algorithmic techniques like memoization, pruning, or parallelization to enhance execution speed.
Implementing Algorithms Using Java
Java is a widely used programming language for implementing algorithms due to its robustness and extensive libraries. When implementing algorithms in Java, it is essential to leverage the language's built-in features and libraries effectively.
Building real-world problem-solving algorithms using Java involves writing clear, modular, and well-documented code. It is recommended to follow best practices, such as employing object-oriented programming principles, using design patterns, and conducting thorough testing.
Algorithms are powerful tools for solving real-world problems efficiently. By employing suitable algorithm design techniques, understanding the problem domain deeply, and optimizing the algorithms, we can tackle even the most complex problems effectively. With Java as a programming language, we can implement these algorithms accurately and efficiently. So, embrace the algorithm design techniques and start solving real-world problems with confidence using Java.
noob to master © copyleft
Enhancing Problem-Solving Skills in Java Programming: A Comprehensive Guide
Avinash bidkar, introduction.
Problem-solving skills lie at the heart of successful Java programming. In the realm of software development, the ability to dissect complex challenges, design efficient solutions, and implement them through code is paramount. This article delves into the art of honing problem-solving skills within the context of Java programming. By combining logical thinking, algorithmic strategies, and effective coding practice, developers can elevate their problem-solving skills in Java programming and create elegant, robust solutions.
Understanding Problem-Solving in Java Programming
Problem-solving in the realm of Java programming refers to the systematic approach of identifying, analyzing, and solving coding challenges. In a software developer course, you’ll learn that it involves breaking down intricate problems into manageable components and devising logical strategies to tackle them. Logical thinking and algorithmic understanding play a pivotal role in this process, allowing developers to construct well-structured and optimized solutions. Such skills are indispensable for crafting software that is not only functional but also efficient.
Guidelines for Enhancing Problem-Solving Skills
Here’s a rundown of the guidelines to enhance your problem-Solving skills.
- Breaking down complex problems: Start by breaking down a complex problem into smaller sub-problems. This approach simplifies the overall challenge and makes it easier to develop individual solutions for each component.
- Developing a systematic approach: Establish a systematic approach to problem-solving. Define the problem, identify the required inputs and desired outputs, and outline the steps needed to bridge the gap.
- Understanding before coding: Resist the urge to immediately dive into coding. Spend ample time understanding the problem statement, its nuances, and potential edge cases. A solid understanding is the foundation of an effective solution.
- Utilizing pseudocode and flowcharts: Before writing actual code, create pseudocode or flowcharts to outline the logical flow of your solution. This helps in visualizing the process and identifying potential flaws early on.
- Incremental development and testing: Build your solution incrementally, testing each component as you progress. This approach allows you to catch errors early and refine your solution iteratively.
Effective Coding Practice for Problem-Solving
Regular coding practice is essential for honing problem-solving skills. Engage in a variety of coding challenges that span different difficulty levels and problem domains. Online coding platforms, such as LeetCode, HackerRank, and CodeSignal, offer a wealth of practice problems and allow you to benchmark your skills against a global community of programmers. Mastering problem-solving skills in Java programming involves a combination of understanding the language's features, algorithms, and data structures, as well as practicing systematic approaches to tackling challenges.
Applying Algorithms for Efficient Solutions
Algorithms are step-by-step instructions for solving a specific problem. Learning and implementing various algorithms can greatly improve your problem-solving capabilities. Start by understanding the fundamental algorithms such as sorting, searching, and graph traversal. As you become more comfortable, move on to more advanced algorithms like dynamic programming, greedy algorithms, and divide-and-conquer techniques. Regularly practicing these algorithms through coding exercises will help you develop a toolkit of strategies to approach different types of problems effectively.
Strengthening Logical Thinking Abilities
Logical thinking is the foundation of problem-solving. It involves breaking down complex problems into smaller, manageable parts and understanding the relationships between different elements. To enhance your logical thinking skills, practice solving puzzles, brain teasers, and mathematical problems. Additionally, try to analyze the structure of various programming challenges and identify patterns and dependencies. Cultivating this skill will enable you to devise creative and efficient solutions to intricate programming problems.
Learning from Real-world Examples
Real-world examples provide practical insights into applying problem-solving skills in Java programming. Let's explore a few examples:
Example 1: Finding the factorial of a number: Break down the problem into smaller steps, creating a loop to multiply consecutive integers.
Example 2: Implementing binary search: Utilize the divide and conquer strategy to narrow down the search space and efficiently find the target element.
Example 3: Solving the Fibonacci sequence: Employ dynamic programming to optimize recursive calculations and generate Fibonacci numbers efficiently.
Overcoming Common Challenges
When solving programming problems, you're likely to encounter common challenges such as bugs, runtime errors, and inefficiencies. Embrace these challenges as opportunities to learn. Debugging is a crucial aspect of problem-solving. Cultivate a systematic approach to identifying and fixing errors by using tools like debugging environments and print statements. Additionally, optimize your code by analyzing time and space complexity, identifying bottlenecks, and making informed decisions to improve efficiency.
Putting Problem-Solving Skills to the Test
Practicing problem-solving skills is essential for improvement. Utilize coding platforms like LeetCode, HackerRank, and Codeforces to access a wide range of programming challenges. These platforms often categorize problems by difficulty, allowing you to gradually progress from easy to more complex tasks. Set aside dedicated time for consistent practice, and challenge yourself by attempting problems that initially seem daunting.
Collaborate with fellow programmers, engage in coding competitions, and participate in online forums to gain exposure to different problem-solving approaches.
An essential talent for Java programmers is the ability to solve problems. Developers can become skilled problem solvers by following logical rules, practicing code often, learning algorithms, and cultivating logical thought. The secret to maximizing the potential of problem-solving abilities is constant learning combined with practical experience. As you set out on this path, keep in mind that every obstacle you overcome will help you get closer to mastering Java programming and being able to create unique, effective solutions.
How can I improve my problem-solving skills in Java programming?
Enhance your problem-solving skills in Java by practicing algorithmic challenges, breaking down complex problems into smaller tasks, and leveraging data structures effectively. Regular coding practice and participating in coding competitions can also contribute to your skill development.
What strategies can I employ to tackle challenging Java programming problems?
Approach difficult Java programming problems step by step, analyze the problem requirements thoroughly, devise a clear plan, and implement it incrementally. Utilize debugging tools and collaborate with peers to gain different perspectives on problem-solving techniques.
Are there any specific resources for practicing Java problem-solving?
Numerous online platforms offer Java programming challenges and exercises, such as LeetCode, HackerRank, and Codeforces. These resources provide a range of problems to solve, helping you refine your problem-solving skills and Java proficiency.
How do I optimize my Java code for better problem-solving outcomes?
Optimize Java code by choosing efficient data structures, minimizing unnecessary iterations, and employing dynamic programming or memoization techniques when appropriate. Regularly review and refactor your code to improve its clarity and performance.
Q. Can problem-solving in Java programming benefit my overall programming proficiency?
Enhancing your problem-solving skills in Java translates to improved programming proficiency in general. The analytical mindset and structured problem-solving techniques you develop will prove valuable across various programming languages and domains.
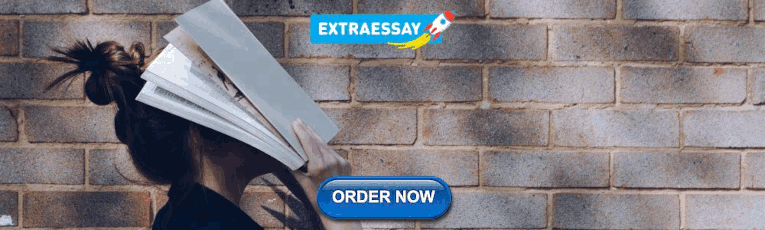
IMAGES
VIDEO
COMMENTS
Description. Algorithm Design Techniques: Live problem-solving in Java. Algorithms are everywhere. One great algorithm applied sensibly can result in a System like GOOGLE! Completer scientists have worked from 100s of years and derived some of the techniques that can be applied to write and design algorithms.
Once you will come to know these design techniques It will become very easy for you to approach a problem by identifying which technique to apply to solve that correctly and efficiently. 0. Complexity analysis. 1. Recursion is the base of any algorithm design. 2. Backtracking. 3. Divide and Conquer.
Let's go through some of the most famous algorithm design techniques in this course. Once you will come to know these design techniques It will become very easy for you to approach a problem by identifying which technique to apply to solve that correctly and efficiently.
1. You will understand how to design algorithms. 2. A lot of coding practice and design live problems in Java. 3. Algorithm Complexity analysis. AND. If you are preparing for your coding Interview or doing competitive programming This course will be a big help for you.
This course is all about algorithms! We'll start by looking into the concept of recursion — what does it mean for a method to call itself? Once we wrap our minds around this tricky concept, we'll look at how to use recursion to solve some problems. Next, we'll start to think about how we can evaluate the effectiveness of our algorithms.
Course Overview. As a developer, mastering the concepts of algorithms and being proficient in implementing them is essential to improving problem-solving skills. This course aims to equip you with an in-depth understanding of algorithms and how they can be utilized for problem solving in Java. Starting with the basics, you'll gain a ...
2. Sort in Java. Sort algorithms are ordering the elements of a list according to a certain order. For the Java examples I will assume that we are sorting an array of integers. The examples for this chapter will be created in a Java project "de.vogella.algorithms.sort". The sorting algorithm will implement the following interface.
Additionally, various algorithm design techniques are discussed, making the course a wealth of information for coder/developers. The purpose of this course is to give the coder/developer the opportunity to learn these design techniques and make the process easier. It will help to get ahead of the game and solve problems before they arise.
This course provides a comprehensive overview of algorithms, with a focus on complex problem solving in Java algorithms. Additionally, various algorithm de ... Algorithms in Java :Live problem solving & Design Techniques by Basics Strong (Udemy) Recursion,BackTracking,Divide & Conquer,Dynamic Programming,Greedy Algorithms via Data Structures ...
This LiveLessons course describes how to apply patterns and frameworks to alleviate the complexity of developing software via the use of object-oriented design techniques and Java programming language features. We'll use a case study based on many patterns in the book Design Patterns: Elements of Reusable Object-Oriented Software (the so-called ...
Yasin Cakal. This course, "Data Structures and Algorithms with Java" is designed to provide an in-depth understanding of data structures and algorithms and how to implement them using Java. Whether you're a beginner or an experienced programmer, this course will provide you with the knowledge and skills you need to become proficient in ...
Additionally, it explores advanced techniques like computational geometry, parallel algorithms, and online algorithms, offering a comprehensive exploration of the diverse field of algorithmic problem-solving. Intended audience. This course is designed to cater to a broad range of audiences interested in algorithms and data structures.
Over the last half a century, computer scientists have discovered that many algorithms share similar ideas, even though they solve very different problems. For now, we'll mention the most common algorithm design techniques so that future examples can be categorized in terms of the algorithm's design methodology.
Problem Solving: Different problems require different algorithms, and by having a classification, it can help identify the best algorithm for a particular problem. Performance Comparison: By classifying algorithms, it is possible to compare their performance in terms of time and space complexity, making it easier to choose the best algorithm for a particular use case.
Algorithm Design Techniques: Live problem-solving in Java. Algorithms are everywhere. One great algorithm applied sensibly can result in a System like GOOGLE! Completer scientists have worked from 100s of years and derived some of the …
Algorithms in Java are static methods that can be used to perform various operations on collections. Since algorithms can be used on various collections, these are also known as generic algorithms. Let's see the implementation of different methods available in the collections framework. 1. Sorting Using sort () The sort() method provided by the ...
Algorithms are powerful tools for solving real-world problems efficiently. By employing suitable algorithm design techniques, understanding the problem domain deeply, and optimizing the algorithms, we can tackle even the most complex problems effectively. With Java as a programming language, we can implement these algorithms accurately and ...
Description. Welcome to "Mastering Data Structures and Algorithms with Java" - the ultimate course to learn and understand the core concepts of data structures and algorithms using Java programming language. This course is designed for anyone who wants to improve their coding skills and become a proficient Java developer.
Introduction Problem-solving skills lie at the heart of successful Java programming. In the realm of software development, the ability to dissect complex challenges, design efficient solutions, and implement them through code is paramount. This article delves into the art of honing problem-solving skills within the context of Java programming.
Algorithm is a step-by-step procedure for solving a problem or accomplishing a task. In the context of data structures and algorithms, it is a set of well-defined instructions for performing a specific computational task. Algorithms are fundamental to computer science and play a very important role in designing efficient solutions for various ...
GeeksforGeeks is a computer science portal for geeks who want to learn and practice programming, data structures, algorithms, and more. You can find well-written articles, quizzes, interview questions, and problem of the day challenges on various topics and levels of difficulty. You can also use the online C compiler to test your code and get instant feedback.
"Problem Solving in Data Structures & Algorithms" is a series of books about the usage of Data Structures and Algorithms in computer programming. The book is easy to follow and is written for interview preparation point of view. In these books, the examples are solved in various languages like Go, C, C++, Java, C#, Python, VB, JavaScript and PHP.
The Bellman Ford Algorithm Visualized. 11min video. Learn how to use Java algorithms for data analysis and coding from top-rated instructors. Whether you're interested in learning about Java, or preparing for a Java algorithms interview, Udemy has a course to help you achieve your goals.
Some alert ... ...
Problem Overview: Gain a clear understanding of the problem statement and its significance in various applications such as hashing, modular arithmetic, and combinatorial analysis. Key Concepts : Familiarize yourself with essential concepts such as array manipulation, modular arithmetic, and hashing techniques, laying the foundation for ...