- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
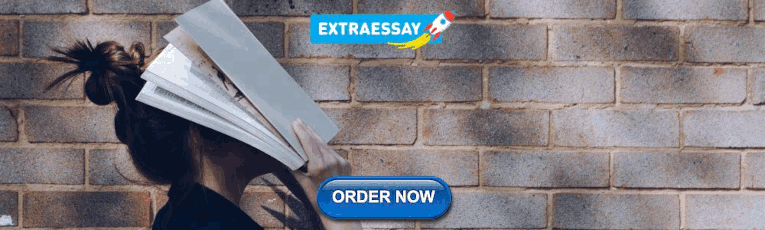
Collections unmodifiableList() method in Java with Examples
- Collections unmodifiableSet() method in Java with Examples
- Collections unmodifiableMap() method in Java with Examples
- Collections unmodifiableCollection() method in Java with Examples
- Java Collections unmodifiableSortedSet() Method with Examples
- Java Collections unmodifiableNavigableSet() Method with Examples
- Collections singletonList() method in Java with Examples
- Java Collections unmodifiableNavigableMap() Method with Examples
- Collections synchronizedList() method in Java with Examples
- Collections list() method in Java with Examples
- AbstractCollection retainAll() method in Java with Examples
- Collection addAll() method in Java with Examples
- Collections checkedList() method in Java with Examples
- ArrayList removeAll() method in Java with Examples
- Java Collections emptyList() Method with Examples
- AbstractSet removeAll() Method in Java with Examples
- Iterate Over Unmodifiable Collection in Java
- AbstractSequentialList removeAll() method in Java with Example
- AbstractCollection in Java with Examples
- Java Collection containsAll() Method with Examples
- Reverse a string in Java
- Initializing a List in Java
- Convert a String to Character Array in Java
- Java Programs - Java Programming Examples
- Convert Double to Integer in Java
- Implementing a Linked List in Java using Class
- Traverse Through a HashMap in Java
- Java Program to find largest element in an array
- Iterate through List in Java
- Factory method design pattern in Java
The unmodifiableList() method of java.util.Collections class is used to return an unmodifiable view of the specified list. This method allows modules to provide users with “read-only” access to internal lists. Query operations on the returned list “read through” to the specified list, and attempts to modify the returned list, whether direct or via its iterator, result in an UnsupportedOperationException.
The returned list will be serializable if the specified list is serializable. Similarly, the returned list will implement RandomAccess if the specified list does.
Parameters: This method takes the list as a parameter for which an unmodifiable view is to be returned.
Return Value: This method returns an unmodifiable view of the specified list.
Below are the examples to illustrate the unmodifiableList() method
Example 2: For UnsupportedOperationException
Please Login to comment...
Similar reads.
- Java - util package
- Java-Collections
- Java-Functions
- Java Programs
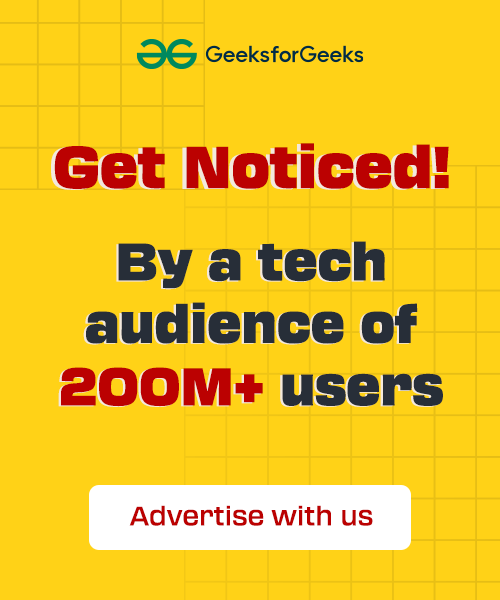
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Generics unchecked assignment

SCJP 1.4 - SCJP 6 - SCWCD 5 - OCEEJBD 6 - OCEJPAD 6 How To Ask Questions How To Answer Questions
How to use checkedList method in java.util.Collections
Best java code snippets using java.util . collections . checkedlist (showing top 20 results out of 432).
- Send Message
- Core Java Tutorials
- Java EE Tutorials
- Java Swing Tutorials
- Spring Framework Tutorials
- Unit Testing
- Build Tools
- Misc Tutorials
Since the return collection/map is unmodifiable, attempting to modify it (adding/removing new elements) throws UnsupportedOperationException .
These methods create shallow copy of the provided collection. That means the collection elements (or key/values in case of map) are not copied/cloned, so if the original elements (if mutable) are changed they will reflect the changes in the new collection and vice-versa.
These methods throw NullPointerException , if the provided collection has null elements.
List.copyOf(collection)
Set.copyof(collection), map.copyof(map), when to use copyof() methods.
Let's see the List.CopyOf() code snippet:
As seen above, this method is just a helper around List.of() method. Note that the List.of() also return a type AbstractImmutableList , so if the provided collection is created by List.of() methods then it is return as it is.
Also the copyOf() methods allow us to assign the provided collection to a collection having elements of super type ( covariant assignment ). In above snippet, there are also 'unchecked' castings, but since the returned collection is immutable this cannot lead to heap pollution situation.
Consider following example without CopyOf method:
Above ClassCastException will be a surprise for a developer who is not aware of aLibOperation() code.
Now let's use CopyOf() method:
As seen in above output, the UnsupportedOperationException is thrown at the right place (aLibOperation) where a wrong operation is performed, instead of very late unexpected ClassCastException .
Set.CopyOf() and Map.copyOf() have the similar behavior as we saw above.
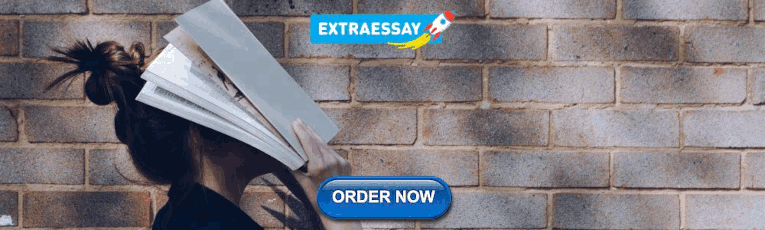
List.CopyOf() vs Collections.unmodifiableList()
Collections.unmodifiableList() (and other similar methods) returns a unmodifiable view of the source collection, so changes made to the source collection reflects in it. Whereas, in case of List.copyOf() method, if the source collection is subsequently modified, the returned List will not reflect such modifications.
Example Project
Dependencies and Technologies Used:
- ListCopyOfExample.java
- ListExample1.java
- ListExample2.java
- MapCopyOfExample.java
- SetCopyOfExample.java
- WithCollectionsUtilExample.java
Unchecked assignment: ‘java.util.List’ to ‘java.util.Collection’
I am having an adapter where i have two lists one list is for InvestorsList where it comes with the list of investors and the other list is called investorListFull which is used to filter results when searching.
Below is how i have declared the lists
Below is how the lists are assigned in my recyclerview adapter constructor
Below is how i am filtering results in investors list
I am getting Unchecked assignment error in publish results investorList.addAll((List) filterResults.values);
Advertisement
I am getting Unchecked cast error in publish results investorList.addAll((List) filterResults.values);
That’s because you’re doing an unchecked cast. Actually, you’re doing both a checked and an unchecked cast there.
(List) filterResults.values is a checked cast. This means that the Java compiler will insert a checkcast instruction into the bytecode to ensure that filterResults.values (an Object ) really is an instance of List .
However, investorList.addAll expects a List<Investor> , not a List . List is a raw type . You can pass a raw-typed List to a method expecting a List<Something> ; but this is flagged as unsafe because the compiler can’t guarantee that it really is a List<Something> – there is nothing about the List that makes it a “list of Something “, because of type erasure. The compiler can insert a checkcast instruction to ensure it’s a List ; but not one to ensure it’s a List<Something> : this fact is unchecked .
What it’s saying with the warning is “there may be something wrong here; I just can’t prove it’s wrong”. If you know for sure – by construction – that filterResults.values really is a List<Investor> , casting it to List<Investor> is safe.
You should write the line as:
Note that this will still give you an unchecked cast warning, because it’s still an unchecked cast – you just avoid the use of raw types as well.
If you feel confident in suppressing the warning, declare a variable, so you can suppress the warning specifically on that variable, rather than having to add the suppression to the method or class; and document why it’s safe to suppress there:
CollectionUtils.java
- All Classes
- Summary:
- Nested |
- Field |
- Constr |
- Detail:
Class Collections
- java.lang.Object
- java.util.Collections
Field Summary
Method summary, methods inherited from class java.lang. object, field detail, method detail, binarysearch, indexofsublist, lastindexofsublist, unmodifiablecollection, unmodifiableset, unmodifiablesortedset, unmodifiablelist, unmodifiablemap, unmodifiablesortedmap, synchronizedcollection, synchronizedset, synchronizedsortedset, synchronizedlist, synchronizedmap, synchronizedsortedmap, checkedcollection, checkedsortedset, checkedlist, checkedsortedmap, emptyiterator, emptylistiterator, emptyenumeration, singletonlist, singletonmap, reverseorder, enumeration, newsetfrommap, aslifoqueue.
Submit a bug or feature For further API reference and developer documentation, see Java SE Documentation . That documentation contains more detailed, developer-targeted descriptions, with conceptual overviews, definitions of terms, workarounds, and working code examples. Copyright © 1993, 2020, Oracle and/or its affiliates. All rights reserved. Use is subject to license terms . Also see the documentation redistribution policy .
Scripting on this page tracks web page traffic, but does not change the content in any way.
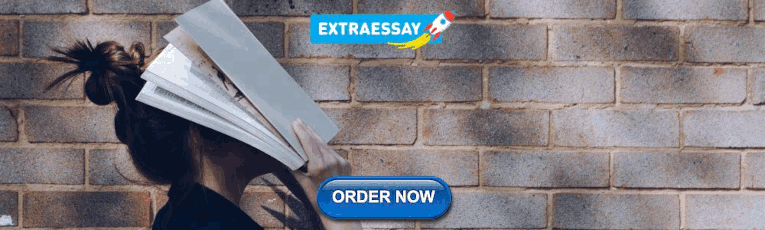
IMAGES
VIDEO
COMMENTS
Map<Integer, String> map = a.getMap(); gets you a warning now: "Unchecked assignment: 'java.util.Map to java.util.Map<java.lang.Integer, java.lang.String>'. Even though the signature of getMap is totally independent of T, and the code is unambiguous regarding the types the Map contains. I know that I can get rid of the warning by reimplementing ...
That's because you're doing an unchecked cast. Actually, you're doing both a checked and an unchecked cast there. (List) filterResults.values is a checked cast. This means that the Java compiler will insert a checkcast instruction into the bytecode to ensure that filterResults.values (an Object) really is an instance of List.
5.2. Checking Type Conversion Before Using the Raw Type Collection. The warning message " unchecked conversion " implies that we should check the conversion before the assignment. To check the type conversion, we can go through the raw type collection and cast every element to our parameterized type.
The "unchecked cast" is a compile-time warning . Simply put, we'll see this warning when casting a raw type to a parameterized type without type checking. An example can explain it straightforwardly. Let's say we have a simple method to return a raw type Map: public class UncheckedCast {. public static Map getRawMap() {.
When you cast an object to ArrayList<Vehicle>, the Java runtime environment can only ensure that the cast to ArrayList<something> succeeds, but due to backwards compatibility (Java 1.4 from 2002), the cast will succeed, no matter if the arraylist contains Integer or String or Vehicle objects. Therefore, in this situation, the compiler warning ...
The unmodifiableCollection() method of java.util.Collections class is used to return an unmodifiable view of the specified collection. This method allows modules to provide users with "read-only" access to internal collections. Query operations on the returned collection "read through" to the specified collection, and attempts to modify the returned collection, whether direct or via ...
The unmodifiableList () method of java.util.Collections class is used to return an unmodifiable view of the specified list. This method allows modules to provide users with "read-only" access to internal lists. Query operations on the returned list "read through" to the specified list, and attempts to modify the returned list, whether ...
This causes "Unchecked assignment: 'java.util.Set' to 'java.util.Collection <? extends java.lang.Object>'". Can this warning be resolved without modifying the classroom class? Rob Spoor
Best Java code snippets using java.util. Collections.checkedList (Showing top 20 results out of 432) java.util Collections checkedList. Collections.addAll (innerList, elements); return Collections.checkedList (innerList, String.class);
Uses of Interface java.util.Collection. This package defines JDI events and event processing. This package contains the JDK's extension to the standard implementation of the java.lang.management API and also defines the management interface for some other components of the platform. Provides a simple high-level Http server API, which can be ...
The root interface in the collection hierarchy. A collection represents a group of objects, known as its elements. Some collections allow duplicate elements and others do not. Some are ordered and others unordered. The JDK does not provide any direct implementations of this interface: it provides implementations of more specific subinterfaces ...
In Java 10, following new static methods to create unmodifiable collections have been added. Since the return collection/map is unmodifiable, attempting to modify it (adding/removing new elements) throws UnsupportedOperationException. These methods create shallow copy of the provided collection. That means the collection elements (or key/values ...
Actually, you're doing both a checked and an unchecked cast there. (List) filterResults.values is a checked cast. This means that the Java compiler will insert a checkcast instruction into the bytecode to ensure that filterResults.values (an Object) really is an instance of List. However, investorList.addAll expects a List<Investor>, not a ...
java.lang.Object. java.util.Collections. public class Collections extends Object. This class consists exclusively of static methods that operate on or return collections. It contains polymorphic algorithms that operate on collections, "wrappers", which return a new collection backed by a specified collection, and a few other odds and ends.
IterableUtils.find (collection, predicate) : null; } /** * Executes the given closure on each but the last element in the collection. * <p> * If the input collection or closure is null, there is no change made. * </p> * * @param <T> the type of object the {@link Iterable} contains * @param <C> the closure type * @param collection the collection ...
Uses of Interface java.util.Collection. Packages that use Collection. Package. Description. java.awt. Contains all of the classes for creating user interfaces and for painting graphics and images. java.beans.beancontext. Provides classes and interfaces relating to bean context. java.security.
•For example, java.util.Arrays.sort -Searching arrays •For example, java.util.Arrays.binarySearch (a sorted in ascending order array) -Check whether two arrays are strictly equal •java.util.Arrays.equals -Fill all or part of an array •java.util.Arrays.fill -Return a string that represents all elements in an array
I'm using the following code to find a one-dimensional list of unique objects in an n-dimensional list (credits to someone on StackOverflow a while ago for the approach): public static <T> List<T> getUniqueObjectsInArray(List<T> array) {. Integer dimension = getDimensions(array); return getUniqueObjectsInArray(array, dimension);
This method acts as a bridge between array-based and collection-based APIs. It allows creation of an array of a particular runtime type. Use toArray () to create an array whose runtime type is Object [] , or use toArray (T []) to reuse an existing array. Suppose x is a collection known to contain only strings.
Class Collections. This class consists exclusively of static methods that operate on or return collections. It contains polymorphic algorithms that operate on collections, "wrappers", which return a new collection backed by a specified collection, and a few other odds and ends. The methods of this class all throw a NullPointerException if the ...