Introduction to JavaScript
Welcome to JavaScript! If you’re brand new to JavaScript or Programming here’s where you can start.
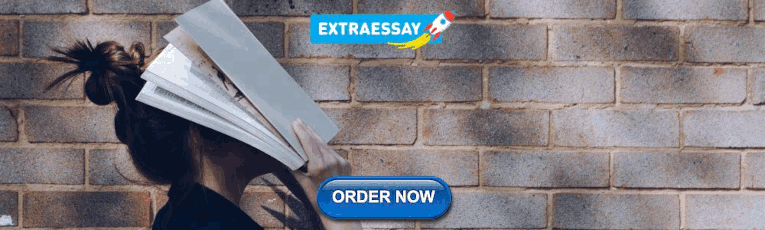
Setting up a Development Environment
Learn the essential steps for setting up a development environment for JavaScript. Follow a simple example to get started and develop your JavaScript projects more efficiently.
What is JavaScript?
Discover the basics of JavaScript, a popular programming language used for creating interactive web pages. Learn through simple examples and gain a better understanding of its versatility and ease of use, perfect for beginners in web development.
Why Should I learn JavaScript?
Learn about the benefits of learning JavaScript and why it’s important. Discover how this versatile and popular programming language can enhance your web development skills and improve your job prospects.
JavaScript Fundamentals
Let’s learn the fundamentals of script building.
- Hello, world!
- Code structure
- The modern mode, "use strict"
- Interaction: alert, prompt, confirm
- Type Conversions
- Basic operators, maths
- Comparisons
- Conditional branching: if, '?'
- Logical operators
- Nullish coalescing operator '??'
- Loops: while and for
- The "switch" statement
- Function expressions
- Arrow functions, the basics
- JavaScript specials
Sibling chapters
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript tutorial.
JavaScript is the world's most popular programming language.
JavaScript is the programming language of the Web.
JavaScript is easy to learn.
This tutorial will teach you JavaScript from basic to advanced.
Examples in Each Chapter
With our "Try it Yourself" editor, you can edit the source code and view the result.
My First JavaScript
Try it Yourself »
Use the Menu
We recommend reading this tutorial, in the sequence listed in the menu.
If you have a large screen, the menu will always be present on the left.
If you have a small screen, open the menu by clicking the top menu sign ☰ .
Learn by Examples
Examples are better than 1000 words. Examples are often easier to understand than text explanations.
This tutorial supplements all explanations with clarifying "Try it Yourself" examples.
If you try all the examples, you will learn a lot about JavaScript, in a very short time!
Why Study JavaScript?
JavaScript is one of the 3 languages all web developers must learn:
1. HTML to define the content of web pages
2. CSS to specify the layout of web pages
3. JavaScript to program the behavior of web pages
This tutorial covers every version of JavaScript:
- The Original JavaScript ES1 ES2 ES3 (1997-1999)
- The First Main Revision ES5 (2009)
- The Second Revision ES6 (2015)
- The Yearly Additions (2016, 2017 ... 2021, 2022)
Advertisement
Learning Speed
In this tutorial, the learning speed is your choice.
Everything is up to you.
If you are struggling, take a break, or re-read the material.
Always make sure you understand all the "Try-it-Yourself" examples.
The only way to become a clever programmer is to: Practice. Practice. Practice. Code. Code. Code !
Test Yourself With Exercises
Create a variable called carName and assign the value Volvo to it.
Start the Exercise
Commonly Asked Questions
- How do I get JavaScript?
- Where can I download JavaScript?
- Is JavaScript Free?
You don't have to get or download JavaScript.
JavaScript is already running in your browser on your computer, on your tablet, and on your smart-phone.
JavaScript is free to use for everyone.
My Learning
Track your progress with the free "My Learning" program here at W3Schools.
Log in to your account, and start earning points!
This is an optional feature. You can study at W3Schools without using My Learning.

JavaScript References
W3Schools maintains a complete JavaScript reference, including all HTML and browser objects.
The reference contains examples for all properties, methods and events, and is continuously updated according to the latest web standards.
JavaScript Quiz Test
Test your JavaScript skills at W3Schools!
Start JavaScript Quiz!
JavaScript Exam - Get Your Diploma!
Kickstart your career.
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Web Programming Step by Step , Lecture 12
Web programming step by step, lecture 12 introduction to javascript, reading: 7.1 - 7.4.
Except where otherwise noted, the contents of this presentation are Copyright 2010 Marty Stepp and Jessica Miller.

- 7.1: Key JavaScript Concepts
- 7.2, 7.3, 7.4: JavaScript Syntax
Client-side scripting (7.1.1)

- often this code manipulates the page or responds to user actions
Why use client-side programming?
PHP already allows us to create dynamic web pages. Why also use client-side scripting?
- usability : can modify a page without having to post back to the server (faster UI)
- efficiency : can make small, quick changes to page without waiting for server
- event-driven : can respond to user actions like clicks and key presses
- security : has access to server's private data; client can't see source code
- compatibility : not subject to browser compatibility issues
- power : can write files, open connections to servers, connect to databases, ...
What is JavaScript? (7.1)
- a lightweight programming language ("scripting language")
- insert dynamic text into HTML (ex: user name)
- react to events (ex: page load user click)
- get information about a user's computer (ex: browser type)
- perform calculations on user's computer (ex: form validation)
- a web standard (but not supported identically by all browsers )
- NOT related to Java other than by name and some syntactic similarities
JavaScript vs. Java

- interpreted , not compiled
- fewer and "looser" data types
- variables don't need to be declared
- errors often silent (few exceptions)
- "first-class" functions are used in many situations
- contained within a web page and integrates with its HTML/CSS content
JavaScript vs. PHP
- both are interpreted , not compiled
- both are relaxed about syntax, rules, and types
- both are case-sensitive
- both have built-in regular expressions for powerful text processing
- JS is more object-oriented: noun.verb() , less procedural: verb(noun)
- JS focuses on UIs and interacting with a document; PHP on HTML output and files/forms
- JS code runs on the client's browser; PHP code runs on the web server
Linking to a JavaScript file: script
- script tag should be placed in HTML page's head
- script code is stored in a separate .js file
- but this is bad style (should separate content, presentation, and behavior)
A JavaScript statement: alert

- a JS command that pops up a dialog box with a message
Variables and types (7.2.1, 7.2.3)
- variables are declared with the var keyword (case sensitive)
- Number , Boolean , String , Array , Object , Function , Null , Undefined
- can find out a variable's type by calling typeof
String type (7.2.7)
- charAt returns a one-letter String (there is no char type)
- length property (not a method as in Java)
- concatenation with + : 1 + 1 is 2 , but "1" + 1 is "11"
Event-driven programming

- JS programs have no main ; they respond to user actions called events
- event-driven programming : writing programs driven by user events
Buttons: <button>
the canonical clickable UI control (inline)
- button's text appears inside tag; can also contain images
- choose the control (e.g. button) and event (e.g. mouse click) of interest
- write a JavaScript function to run when the event occurs
- attach the function to the event on the control
JavaScript functions
- the above could be the contents of example.js linked to our HTML page
- statements placed into functions can be evaluated in response to user events
Event handlers
- when you interact with the element, the function will execute
- onclick is just one of many event HTML attributes we'll use
- A better user experience would be to have the message appear on the page...
Document Object Model ( DOM ) (7.1.4)
a set of JavaScript objects that represent each element on the page

- most JS code manipulates elements on an HTML page
- e.g. see whether a box is checked
- e.g. insert some new text into a div
- e.g. make a paragraph red
DOM element objects (7.2.5)

- every element on the page has a corresponding DOM object
- access/modify the attributes of the DOM object with objectName . attributeName
Accessing elements: document.getElementById
- document.getElementById returns the DOM object for an element with a given id
- can change the text in most form controls by setting the value property
More advanced example
- can change the text inside most elements by setting the innerHTML property
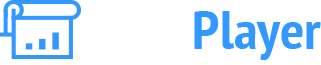
- My presentations
Auth with social network:
Download presentation
We think you have liked this presentation. If you wish to download it, please recommend it to your friends in any social system. Share buttons are a little bit lower. Thank you!
Presentation is loading. Please wait.
INTRODUCTION TO JAVASCRIPT
Published by Owen Carter Modified over 5 years ago
Similar presentations
Presentation on theme: "INTRODUCTION TO JAVASCRIPT"— Presentation transcript:
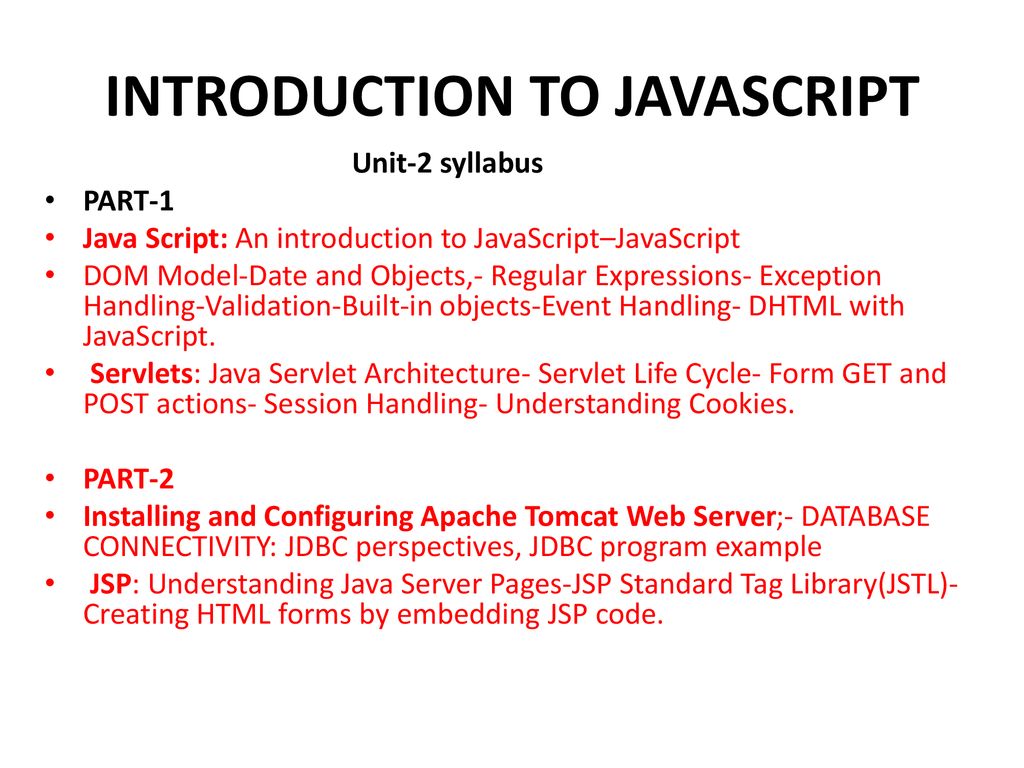
JavaScript I. JavaScript is an object oriented programming language used to add interactivity to web pages. Different from Java, even though bears some.
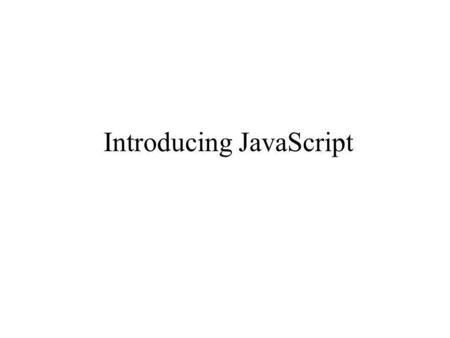
Introducing JavaScript
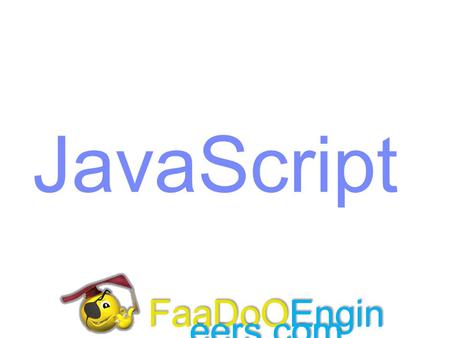
JavaScript FaaDoOEngineers.com FaaDoOEngineers.com.
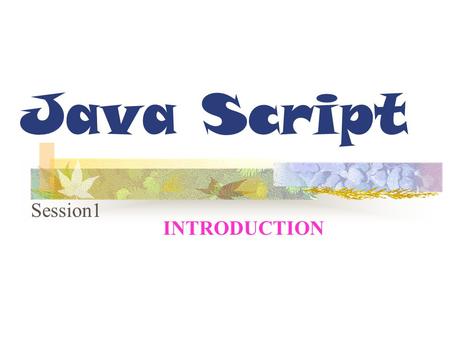
Java Script Session1 INTRODUCTION.
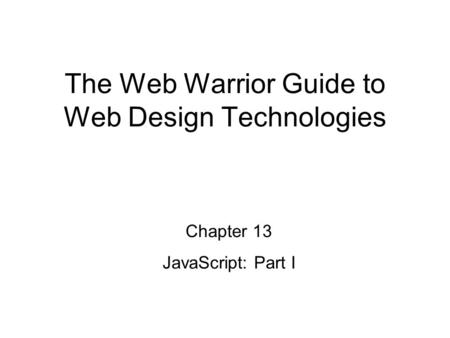
The Web Warrior Guide to Web Design Technologies
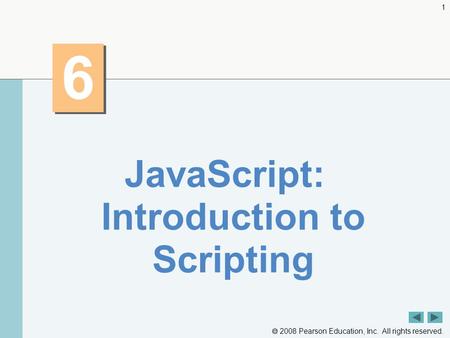
2008 Pearson Education, Inc. All rights reserved JavaScript: Introduction to Scripting.
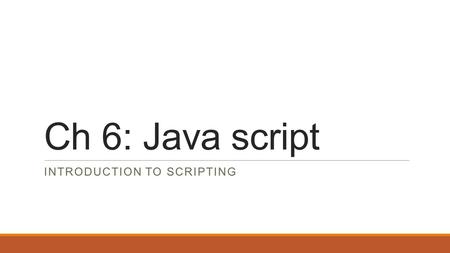
Introduction to scripting
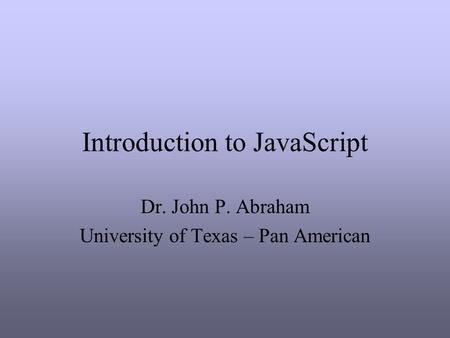
Introduction to JavaScript Dr. John P. Abraham University of Texas – Pan American.
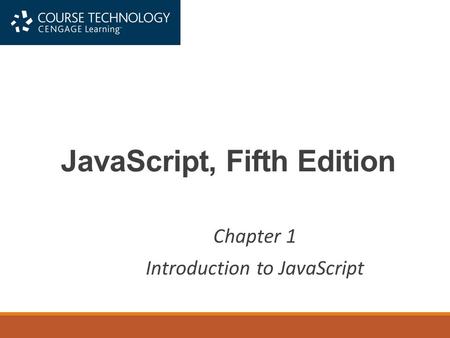
JavaScript, Fifth Edition Chapter 1 Introduction to JavaScript.
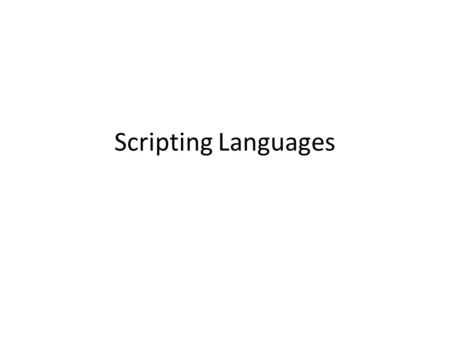
Scripting Languages.
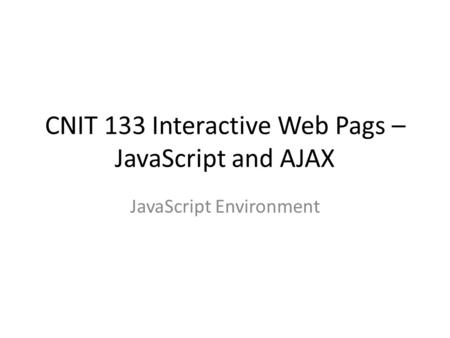
CNIT 133 Interactive Web Pags – JavaScript and AJAX JavaScript Environment.
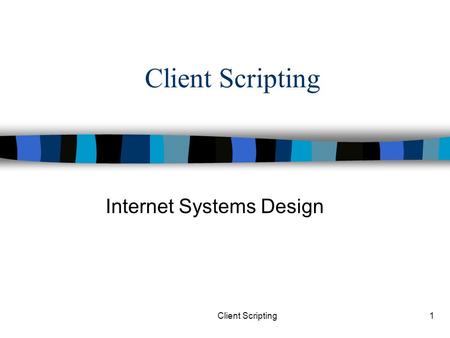
Client Scripting1 Internet Systems Design. Client Scripting2 n “A scripting language is a programming language that is used to manipulate, customize,
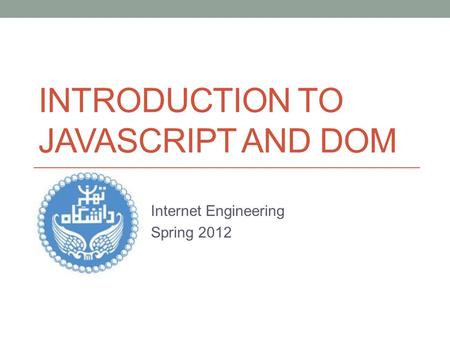
INTRODUCTION TO JAVASCRIPT AND DOM Internet Engineering Spring 2012.
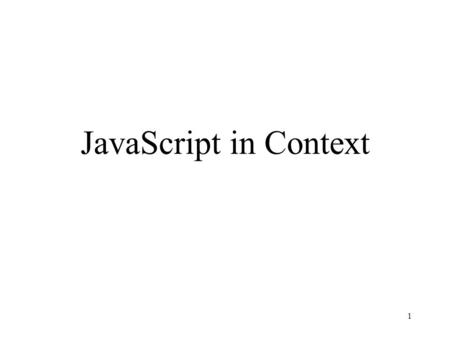
1 JavaScript in Context. Server-Side Programming.
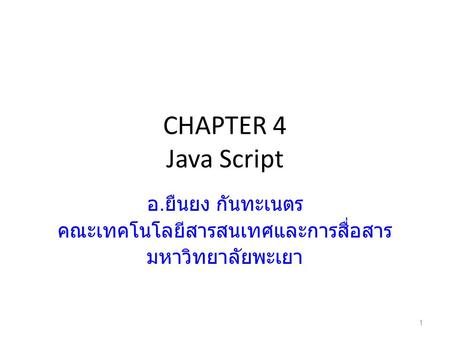
CHAPTER 4 Java Script อ. ยืนยง กันทะเนตร คณะเทคโนโลยีสารสนเทศและการสื่อสาร มหาวิทยาลัยพะเยา 1.
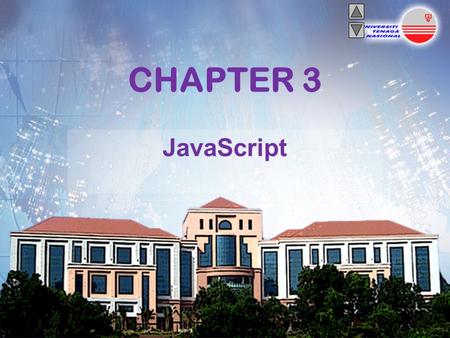
2003 Prentice Hall, Inc. All rights reserved. CHAPTER 3 JavaScript 1.
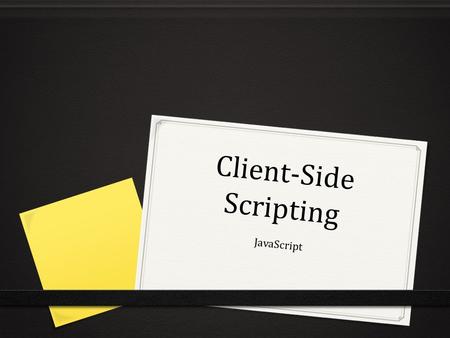
Client-Side Scripting JavaScript. produced by Netscape for use within HTML Web pages. built into all the major modern browsers. properties lightweight,
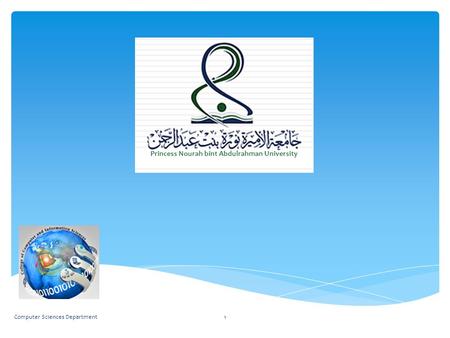
1Computer Sciences Department Princess Nourah bint Abdulrahman University.
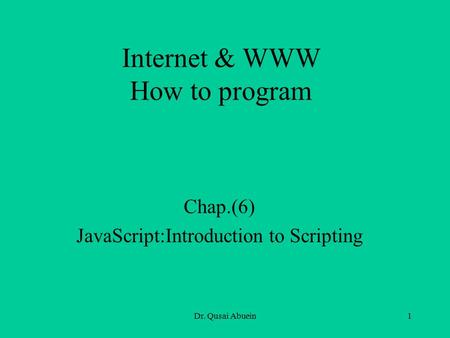
Dr. Qusai Abuein1 Internet & WWW How to program Chap.(6) JavaScript:Introduction to Scripting.
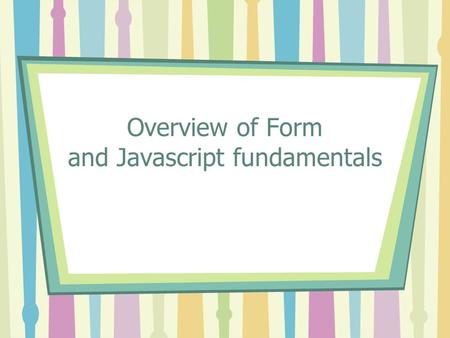
Overview of Form and Javascript fundamentals. Brief matching exercise 1. This is the software that allows a user to access and view HTML documents 2.
About project
© 2024 SlidePlayer.com Inc. All rights reserved.
Top 9 JavaScript frameworks to create beautiful presentation slides
Presentation frameworks are tools or libraries that can help you create presentations using web technologies that you are familiar with, such as HTML, CSS, JavaScript, Markdown, Vue, React, and more. You’ll have full control over the appearance and layout of your slides.
They allow you to export your slides as HTML files that can be viewed in any modern browser. You don’t need to install any software or plugin to view your slides. You can also share your slides online using platforms such as Slides.com, GitHub Pages, Netlify.
Reveal ( 64.2k ⭐) — An open source HTML presentation framework that allows you to create beautiful and interactive presentations using web technologies. You can use HTML, CSS, JavaScript, Markdown, LaTeX, and more to create stunning slides with animations, transitions, code highlighting, and other features.
Impress ( 37.3k ⭐) — Another open source HTML presentation framework that is similar to reveal.js, but with a different approach, inspired by the idea behind prezi.com. It uses CSS3 3D transforms to create dynamic and spatial presentations that can zoom, rotate, and pan across the slides.
Sli dev ( 27.3k ⭐) — A web-based slides maker and presenter that is designed for developers. It allows you to create beautiful and interactive presentations using Markdown, HTML, Vue components, and other web technologies. You can also use features such as live coding, recording, drawing, LaTeX, diagrams, icons, and more to enhance your slides.
MDX Deck ( 11.1k ⭐) — A library based on MDX that allows you to create presentations using Markdown and React components. You can write your slides in a single MDX file and separate them with --- . You can also import and use any React component in your slides, as well as customize the theme and layout of your presentation.
Spectacle ( 9.5k ⭐) — A React-based library for creating sleek presentations using JSX syntax that gives you the ability to live demo your code, created and maintained by Formidable Labs. You can use it to create beautiful and interactive slides with animations, transitions, code highlighting, and other features.
Code Surfer ( 6.2k ⭐) — A library that allows you to create presentations using Markdown and React components. You can write your slides in a single MDX file and separate them with --- , add code highlighting, code zooming, code scrolling, code focusing, code morphing, and fun to MDX Deck slides.
WebSlides ( 6.1k ⭐) — A library that allows you to create beautiful HTML presentations and websites. Just choose a demo and customize it in minutes. 120+ slides ready to use. You can use HTML, CSS, JavaScript, Markdown, LaTeX, and more to create stunning slides with animations, transitions, code highlighting, and other features.
Fusuma ( 5.3k ⭐) — A tool that allows you to create slides with Markdown easily. You can use HTML, CSS, JavaScript, Markdown, Vue components, and other web technologies to create stunning slides with animations, transitions, code highlighting, and other features.
PptxGenJS ( 2.1k ⭐) — A JavaScript library that allows you to create presentations, compatible with PowerPoint, Keynote, and other applications that support the Open Office XML (OOXML) format. You can use it to generate PPTX files with just a few simple JavaScript commands in any modern desktop and mobile browser. You can also integrate PptxGenJS with Node, Angular, React, and Electron.
Common features
Presentation frameworks typically share several common features that aim to enhance the creation and delivery of visually engaging and interactive presentations. Here are some of the common features you can find:
Ease of use : They allow you to use web technologies that you are familiar with, such as HTML, CSS, JavaScript, Markdown, Vue, React, and more. You don’t need to learn a new software or tool to create your slides. You can also use your favorite code editor or IDE to write and edit your slides.
Nested slides : They allow you to create sub-sections or sub-topics within your presentation. You can use nested slides to organize your content, add more details, or create interactive menus.
Markdown support : Markdown is a lightweight markup language that allows you to format text using simple syntax. You can use Markdown to write your slides in a plain text editor and then convert them to HTML. Markdown makes it easy to create headings, lists, links, images, code blocks, and more.
Auto-Animate : A feature that automatically animates the transitions between slides or elements to create smooth and dynamic effects for your presentation, detect the changes between slides and animate them accordingly.
PDF export : You can use PDF export to print your presentation, share it online, or view it offline. PDF export can also preserve the layout, fonts, and images of your presentation.
Speaker notes : You can use speaker notes to prepare your speech, add additional information, or provide references. Speaker notes are usually hidden from the audience but visible to you in a separate window or screen.
LaTeX support : LaTeX is a document preparation system that allows you to create high-quality typesetting for mathematical and scientific expressions. You can use LaTeX to write complex formulas, equations, symbols, and diagrams in your presentation. LaTeX can also handle cross-references, citations, and bibliographies.
Syntax highlighted code : You can use syntax highlighted code to display your source code in your presentation. Syntax highlighted code can make your code more readable, understandable, and attractive.

A Presentation Framework by @marcolago
Press Down Key to continue Or Swipe Up if you prefer.
What is it?
Flowtime.js is a framework for easily building HTML presentations or websites.
It’s built with web standards in mind and on top of a solid full page grid layout.
The animations are managed with native and accelerated CSS3 transitions. Javascript takes care of the navigation behaviour and adds advanced functionalities and configuration options.
Press Down Key or Swipe Up to continue.
Compatibility and Support
Flowtime.js is fully compatible with real moderns browsers:
- Internet Explorer 10
Internet Explorer 9 and some old versions of other browsers lack the transitions and some modern Javascript features but the main navigation and functionalities will work.
In older browsers degrades to a bi-directional scrolling navigation with anchor links.
Touch Devices Support
On touch devices you can navigate through slides swiping in all directions.
By now Flowtime.js was tested and works on Safari Mobile and Chrome Mobile on iOS devices but the support will be wider in the near future.
Mastering the Navigation
You can navigate in many ways. The most common way is using the keyboard.
Navigation Keys and default behaviours:
- Down Key goes to the next page or shows up the next fragment (we’ll see this later).
- Up Key goes to the previous page or hides the fragment.
- Right Key goes to the adjacent page in the next section (if available, it’s like a grid, otherwise it goes to the last page in the next section).
- Left Key goes to the adjacent page in the previous section.
Alternate Navigation Control
Pressing the Shift Key you can alternate the the default navigation keys behaviour; let’s see how:
- Down Key goes to the next page skipping all the fragments.
- Up Key goes to the previous page skipping all the fragments.
- Right Key goes to the first page in the next section.
- Left Key goes to the first page in the previous section.
Some Other Keys
Because: the more, the better.
- Page Up Key goes to the first page of the current section.
- Page Down Key goes to the last page of the current section.
- Home Key goes to the first page of the first section.
- End Key goes to the last page of the last section.
Overview Mode.
You can look at the entire presentation by pressing ESC Key . When in Overview Mode you can go back to the Page Mode by pressing again the ESC Key.
Try it out!
WARNING! Experimental Feature. If you experience problems in webkit browser you can use the alternate Overview Mode; see the documentation for more info.
Navigating the Overview
In Overview Mode the arrow keys works in the same way as in Page Mode, highlighting the future destination. To navigate to the highlighted page just press Return or Enter Key or click on the desired page.
Navigate via Links or Javascript API
You can link every page by simply building the href value using this schema:
Where data-id attribute is an optional attribute you can add to every section or page. I.e.: if you want to go to back to the first page click here (press backspace to come back to this page) .
You can also trigger every navigation behaviour using the Flowtime.js Javascript API . Take a look at the documentation if you want to learn more.
Navigate With History
Flowtime.js offers a full support for the HTML5 History API where available or gracefully degrades on the hashchange event.
This means that you can navigate using the browser’s back and forward buttons and deeplink a page for sharing purposes.
Progress Indicator
You can enable a default progress indicator useful not only to know what’s the current page you are looking, but also as a navigation tool.
Look at the bottom right corner and you can see a miniature of the presentation structure.
Clicking on a page thumb will navigate to that page.
Fragments Support
The built in fragments navigation allows to advance step by step inside a page. Press down to try.
You can discover single elements or even a single part of an element, one at a time.
Fragments navigation is deeply customizable with some configuration options You can learn how in the documentation .
Fragments Unleashed
Thanks to some special classes you can add a couple of useful custom behaviours.
- The .step fragment will partially fades out;
- The .shy fragment will completely hides itself;
- You can use this two special fragment types to easily create special effects.
This is a .shy example!
And this is a .step one!
Default HTML Structures
Flowtime.js comes with a default theme that styles the most common HTML structures, like:
1 st Level Heading
2 nd level heading, 3 rd level heading, 4 th , 5 th and 6 th level heading, unordered lists.
- Another item.
- Just another item.
- Ok, we get it!
Ordered Lists
Definition lists, quotes and citations.
My favourite quotation: The bad craftsman blames his tools .
Sotto un cespo di rose scarlatte dai al rospo the caldo col latte. Sotto un cespo di rose paonazze tocca al rospo lavare le tazze.
Quoting and citing with <blockquote>, <q>, <cite>, and the cite attribute on HTML5 Doctor.
Theme and Styling
If you don’t like the default theme or you want to build your own (or both things), or if you want to build a website on top of Flowtime.js, you can write your own theme and replace the default one.
Theme and core css are in separate files so you can’t break the layout (unless you override some classes).
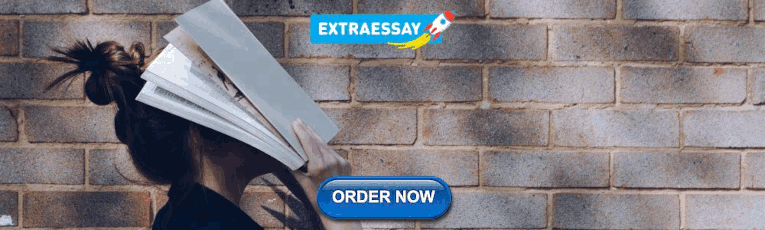
Fluid Layout
Everything can be fluid, just use em , rem and % units if you want to make an element resizable.
Image Management
Images are fluid like all the other content.
You can insert images in the flow or stack images ones on top the others with a minimal markup overhead to create some fancy fragments tricks.
Go to the next pages to see fluid images in action and how stacked images can be managed, both in the flow or centered in the slide. Look at the source code to learn how to write the markup.

Stacked Images

Centered Stacked Images

About the Centered Stack
You can center anything, not only images!
Just like this content. Useful for splash pages and titles.
And you are not limited to stacks, you can center what you want.
Native Parallax Support
By popular demand Flowtime.js includes native parallax support*.
Simply add a parallax class to anything you want to have parallax enabled and configure the amount of distance for all elements or for a single element using data-parallax attribute.
For an example go to the next page ; for more info read the documentation .
* By the way: I’m not a parallax fan; but ehi… this is the "web 3.0".
The Invaders from Audiogalaxy

This Page Is Just for Testing the Parallax
In the previous page, from left to right:
Code Snippets Highlight
Code highlight is a courtesy of Lea Verou’s Prism . Because I really didn’t want to re-invent the wheel. You can use what you want, it’s not a dependency (but it’s very smart and cool).
Events and Custom Implementations
When navigated to a page Flowtime.js fires a custom flowtimenavigation event full of useful properties to customize or build your components or behaviours.
Read the documentation to learn more about this event and its properties.
Some Examples
You can take a look at some examples to explore some of the possibilities that Flowtime.js offers to customize your experience.
- Cross Direction
- Custom Fragment Animations
- Duplicated IDs
- Modal Overlay
- Page Titles in Navigation
- Scroll the Current Section Only
- Sub Pages Demo
- Video Embedding and Controls
Continues …
More Examples
- Default Behavior;
- gridNavigation(false);
- nearestPageToTop(true);
- rememberSectionsLastPage(true);
- rememberSectionsStatus(true);
If you need a specific example or if you have a question about a feature feel free to ask or open an issue .
Use It! It’s Free
This project is open source, feel free to contribute to the development on Github .
Feedbacks, suggestions and bug reports are welcomes.
Use it as you wish and build great things. And when you have done so let me know the URL, I will appreciate it.
Designed and coded by Marco Lago Interaction/Experience/Game/Designer/Developer
You can find me on Twitter as @marcolago
If you like this work spread the word, you know how ;)

- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Presentation API
Secure context: This feature is available only in secure contexts (HTTPS), in some or all supporting browsers .
Experimental: This is an experimental technology Check the Browser compatibility table carefully before using this in production.
The Presentation API lets a user agent (such as a Web browser) effectively display web content through large presentation devices such as projectors and network-connected televisions. Supported types of multimedia devices include both displays which are wired using HDMI, DVI, or the like, or wireless, using DLNA , Chromecast , AirPlay , or Miracast .
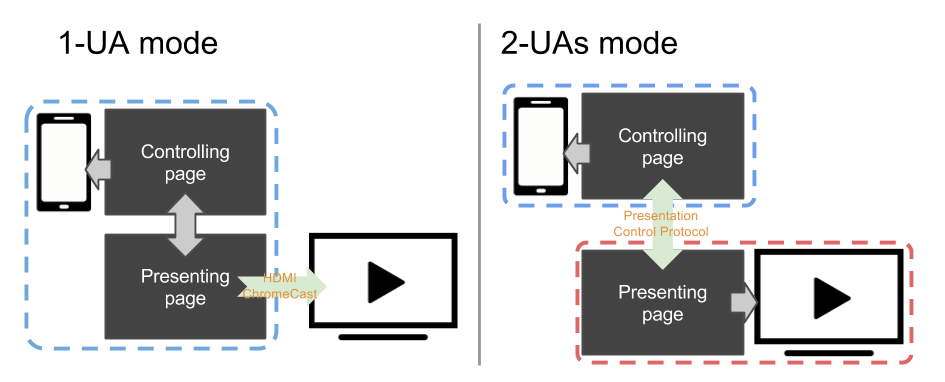
In general, a web page uses the Presentation Controller API to specify the web content to be rendered on presentation device and initiate the presentation session. With Presentation Receiver API, the presenting web content obtains the session status. With providing both the controller page and the receiver one with a messaged-based channel, a Web developer can implement the interaction between these two pages.
Depending on the connection mechanism provided by the presentation device, any controller- and receiver page can be rendered by the same user agent, or by separated user agents.
- For 1-UA mode devices, both pages are loaded by the same user agent. However, rendering result of the receiver page will be sent to the presentation device via supported remote rendering protocol.
- For 2-UAs mode device, the receiver page is loaded directly on the presentation device. Controlling user agent communicates with presentation device via supported presentation control protocol, to control the presentation session and to transmit the message between two pages.
In controlling browsing context, the Presentation interface provides a mechanism to override the browser default behavior of launching presentation to external screen. In receiving browsing context, Presentation interface provides the access to the available presentation connections.
Initiates or reconnects to a presentation made by a controlling browsing context.
A PresentationAvailability object is associated with available presentation displays and represents the presentation display availability for a presentation request.
The PresentationConnectionAvailableEvent is fired on a PresentationRequest when a connection associated with the object is created.
Each presentation connection is represented by a PresentationConnection object.
A PresentationConnectionCloseEvent is fired when a presentation connection enters a closed state.
The PresentationReceiver allows a receiving browsing context to access the controlling browsing contexts and communicate with them.
PresentationConnectionList represents the collection of non-terminated presentation connections. It is also a monitor for the event of new available presentation connection.
Example codes below highlight the usage of main features of the Presentation API: controller.html implements the controller and presentation.html implements the presentation. Both pages are served from the domain https://example.org ( https://example.org/controller.html and https://example.org/presentation.html ). These examples assume that the controlling page is managing one presentation at a time. Please refer to the comments in the code examples for further details.
Monitor availability of presentation displays
In controller.html :
Starting a new presentation
Reconnect to a presentation.
In the controller.html file:
Presentation initiation by the controlling UA
Setting presentation.defaultRequest allows the page to specify the PresentationRequest to use when the controlling UA initiates a presentation.
Monitor connection's state and exchange data
Monitor available connection(s) and say hello.
In presentation.html :
Passing locale information with a message
In the presentation.html file:
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
Presentation API polyfill contains a JavaScript polyfill of the Presentation API specification under standardization within the Second Screen Working Group at W3C. The polyfill is mostly intended for exploring how the Presentation API may be implemented on top of different presentation mechanisms.
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
JavaScript API for PowerPoint
- 4 contributors
A PowerPoint add-in interacts with objects in PowerPoint by using the Office JavaScript API, which includes two JavaScript object models:
PowerPoint JavaScript API : The PowerPoint JavaScript API provides strongly-typed objects that you can use to access objects in PowerPoint.
Common APIs : Introduced with Office 2013, the Common API can be used to access features such as UI, dialogs, and client settings that are common across multiple types of Office applications.
Learn programming concepts
See PowerPoint add-ins overview for information about important programming concepts.
Learn about API capabilities
For hands-on experience using the Common API to interact with content in PowerPoint, complete the PowerPoint add-in tutorial .
For detailed information about the PowerPoint JavaScript API object model, see the PowerPoint JavaScript API reference documentation .
Try out code samples in Script Lab
Use Script Lab to get started quickly with a collection of built-in samples that show how to complete tasks with the API. You can run the samples in Script Lab to instantly see the result in the task pane or document, examine the samples to learn how the API works, and even use samples to prototype your own add-in.
- PowerPoint add-ins documentation
- PowerPoint add-ins overview
- PowerPoint JavaScript API reference
- Office client application and platform availability for Office Add-ins
- API Reference documentation
Office Add-ins
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
Your browser doesn't support the features required by impress.js, so you are presented with a simplified version of this presentation.
For the best experience please use the latest Chrome , Safari or Firefox browser.
impress.js *
It’s a presentation tool inspired by the idea behind prezi.com and based on the power of CSS3 transforms and transitions in modern browsers.
visualize your big thoughts
and tiny ideas
by positioning , rotating and scaling them on an infinite canvas
the only limit is your imagination
want to know more?
one more thing...
have you noticed it’s in 3D * ?
Use a spacebar or arrow keys to navigate. Press 'P' to launch speaker console.
The HTML Presentation Framework
Created by Hakim El Hattab and contributors
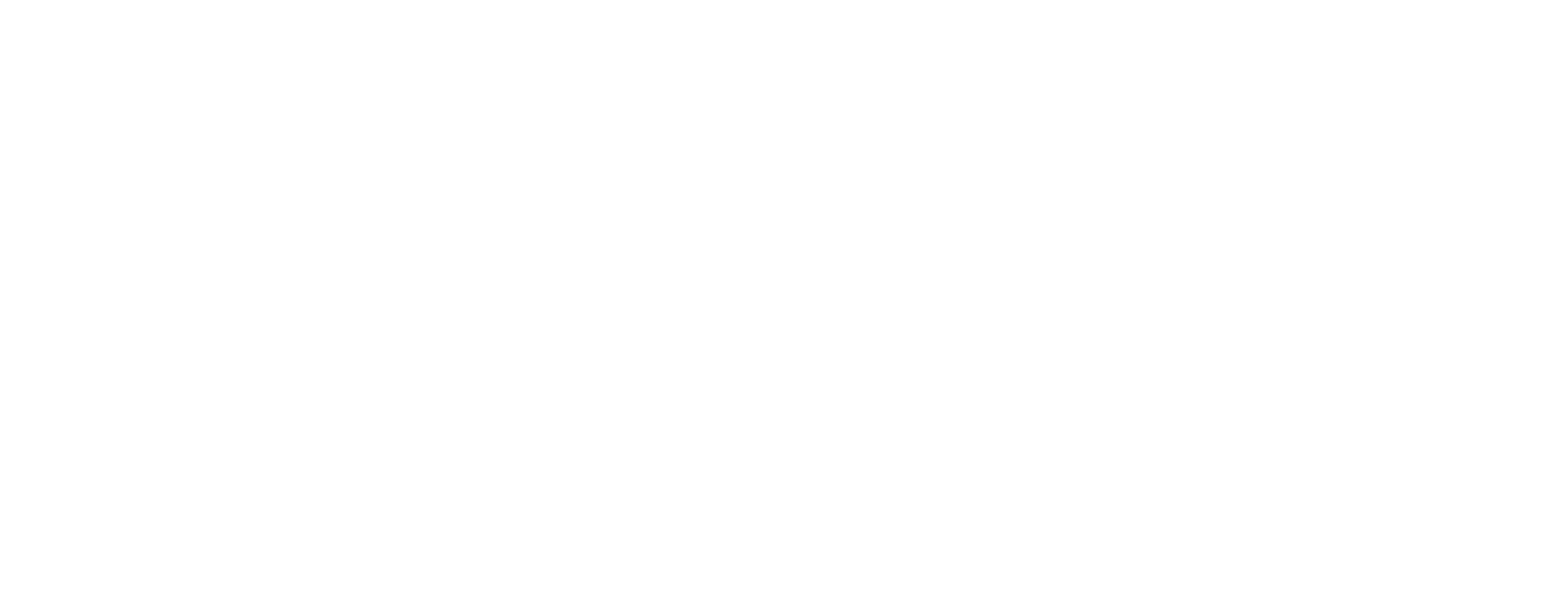
Hello There
reveal.js enables you to create beautiful interactive slide decks using HTML. This presentation will show you examples of what it can do.
Vertical Slides
Slides can be nested inside of each other.
Use the Space key to navigate through all slides.
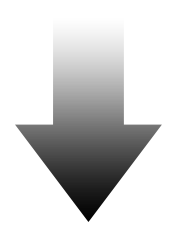
Basement Level 1
Nested slides are useful for adding additional detail underneath a high level horizontal slide.
Basement Level 2
That's it, time to go back up.
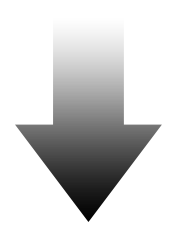
Not a coder? Not a problem. There's a fully-featured visual editor for authoring these, try it out at https://slides.com .
Pretty Code
Code syntax highlighting courtesy of highlight.js .
Even Prettier Animations
Point of view.
Press ESC to enter the slide overview.
Hold down the alt key ( ctrl in Linux) and click on any element to zoom towards it using zoom.js . Click again to zoom back out.
(NOTE: Use ctrl + click in Linux.)
Auto-Animate
Automatically animate matching elements across slides with Auto-Animate .
Touch Optimized
Presentations look great on touch devices, like mobile phones and tablets. Simply swipe through your slides.
Add the r-fit-text class to auto-size text
Hit the next arrow...
... to step through ...
... a fragmented slide.
Fragment Styles
There's different types of fragments, like:
fade-right, up, down, left
fade-in-then-out
fade-in-then-semi-out
Highlight red blue green
Transition Styles
You can select from different transitions, like: None - Fade - Slide - Convex - Concave - Zoom
Slide Backgrounds
Set data-background="#dddddd" on a slide to change the background color. All CSS color formats are supported.
Image Backgrounds
Tiled backgrounds, video backgrounds, ... and gifs, background transitions.
Different background transitions are available via the backgroundTransition option. This one's called "zoom".
You can override background transitions per-slide.
Iframe Backgrounds
Since reveal.js runs on the web, you can easily embed other web content. Try interacting with the page in the background.
Marvelous List
- No order here
Fantastic Ordered List
- One is smaller than...
- Two is smaller than...
Tabular Tables
Clever quotes.
These guys come in two forms, inline: The nice thing about standards is that there are so many to choose from and block:
“For years there has been a theory that millions of monkeys typing at random on millions of typewriters would reproduce the entire works of Shakespeare. The Internet has proven this theory to be untrue.”
Intergalactic Interconnections
You can link between slides internally, like this .
Speaker View
There's a speaker view . It includes a timer, preview of the upcoming slide as well as your speaker notes.
Press the S key to try it out.
Export to PDF
Presentations can be exported to PDF , here's an example:
Global State
Set data-state="something" on a slide and "something" will be added as a class to the document element when the slide is open. This lets you apply broader style changes, like switching the page background.
State Events
Additionally custom events can be triggered on a per slide basis by binding to the data-state name.
Take a Moment
Press B or . on your keyboard to pause the presentation. This is helpful when you're on stage and want to take distracting slides off the screen.
- Right-to-left support
- Extensive JavaScript API
- Auto-progression
- Parallax backgrounds
- Custom keyboard bindings
- Try the online editor - Source code & documentation
If you like Mexican food, it’s a good time to be eating in and around Washington. The choices have never been better or more varied. One restaurant mixes in Lebanese accents with delicious results.
If you live in the suburbs, lucky you. The slate of interesting new restaurants, especially in Northern Virginia — host to a vegetarian Vietnamese hot spot and a dining destination whose principals worked at t he Inn at Little Washington , among other attractions — is apt to keep you close to home for meals.
Now is also an opportunity to sink your teeth into hamburgers of all stripes, explore youthful mom-and-pops and see what some prominent names are up to. No surprise, but serial restaurateurs Stephen Starr and Peter Chang have rolled out more of what they do best: scenes and Chinese .
Welcome to another collection of spring favorites, based on recent visits to area newcomers.
While I was eating around, I noticed some significant changes on the dining front . Restaurants aren’t always open when we expect them to be, aged fish is being celebrated, and some members of the industry are looking to please themselves as much as their customers. Read here to learn about how restaurants across the country are mixing things up.
Not every fresh face was invited to the party. Some places didn’t make the cut because they were so young I haven’t had a chance to try them, or because a visit left me unimpressed. My spring guide is a celebration of what’s new, but also what’s worth your time and money. The following 26 names all qualify for the distinction.

About this story
Editing by Joe Yonan and Matt Brooks. Photo editing by Jennifer Beeson Gregory. Design by Marissa Vonesh. Development by Aadit Tambe. Design editing by Christine Ashack and Matthew Callahan. Additional design by Shikha Subramanium. Copy editing by Emily Morman and Jordan Melendrez. Photo research by Lucas Trevor. Research by Anna Luisa Rodriguez.
- Español – América Latina
- Português – Brasil
- Tiếng Việt
- Chrome for Developers
10 updates from Google I/O 2024: Unlocking the power of AI for every web developer
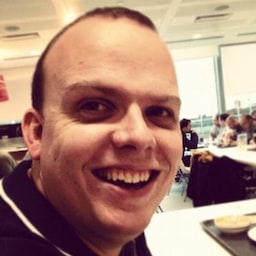
The web turns 35 years old this August. Generations of developers have shaped this incredible technology and brought countless innovations to our lives. Now, it's the dawn of a new generation, with AI. Here are 10 exciting features unveiled in our Developer Keynote and I/O sessions that will guide your path to better development–through a more powerful web made easier.
1. Gemini Nano integration in Chrome desktop to deliver new, on-device AI features
From Chrome 126, Gemini Nano will be built into the Chrome desktop . Why is this important? You'll be able to deliver powerful AI features to Chrome's billions of users without having to worry about prompt engineering, fine tuning, capacity, or cost. "Help me write" is available in Chrome with on-device power, to help users write short-form content.
Help us shape the future of the web by signing up for our early preview program .
2. Web Assembly and WebGPU enable on-device AI, no matter the AI model you use
We've invested heavily to ensure that AI models run quickly and efficiently by using WebGPU and Wasm–the backbone technologies that enable on-device AI on the web. New improvements like 16-bit floating point values in WebGPU, and Memory64 and JavaScript Promise Integration in Wasm, are making AI run even faster. With Wasm and WebGPU, your AI libraries will be able run models at scale, across a massive range of hardware.
3. AI-powered Chrome DevTools will streamline your debugging process
Chrome DevTools is one of the most popular ways to debug and tune your app. With AI, debugging is about to get so much easier. We're bringing Gemini to the Chrome DevTools Console to generate insights, help you understand what the problem is, and even figure out how to fix it!
Chrome DevTools Console insights is available today as an experimental feature in the US, and it's rolling out to more countries soon.
4. Speculation Rules API brings instant browsing experiences
With the new Speculation Rules API , we're enabling near-instant navigation, dramatically speeding up browsing by pre-fetching and pre-rendering pages in the background. Think milliseconds instead of seconds. Best of all? It only requires a few lines of code to get started, and AI can be used to intelligently predict navigation patterns.
5. View Transitions API for multi-page sites
We want to redefine how users experience the web. And for developers, we want to change how you build for the web. With the View Transitions API , you can easily animate between page states. And now, it works with multi-page apps , enabling you to create fluid navigation regardless of your site's architecture. When combined with Speculation Rules and AI, page transitions can be truly seamless.
6. Web Platform Dashboard for a unified view across browsers
We know it isn't easy keeping up with the latest platform changes, APIs, and frameworks across browsers when they're constantly updating. Baseline provides a way to understand which web features are supported on all browsers. Now, with the Web Platform Dashboard , you'll be able to see the entire web platform mapped out as a set of features, follow their development, and check their interop status.
7. Baseline tooling, right in your workflow
Baseline works best when it's integrated into your workflow . From today, Akamai's RUM Archive has a new tool for developers on RUM Archive Insights . For the first time, you can see the global user share of Baseline versions and features that are unlocked with that version of Baseline, side-by-side.
8. Partial hydration in Angular enables improved performance
We believe one of the best platforms for building and deploying web apps is Angular. We're now working on partial hydration , so JavaScript will only load and hydrate part of your app only when needed, significantly improving Core Web Vitals for performance sensitive apps. Check it out in developer preview in the next couple of weeks.
9. Angular fine-grained reactivity with Signals, right out of the box
We want to give you more granular control over the detection and management of changes in your apps. Enter: fine-grained reactivity with Signals. Angular provides a new set of reactive APIs that empower first-class developer experience with Signals. And Signals enable fine-grained change detection that will check only a fraction of your component tree to propagate state changes, so you no longer have to manually optimize the UI.
Signal-based reactive APIs are available today, right out of the box. Fine-grained change detection is coming later this year.
10. Build 3D immersive experiences in Maps JavaScript API
We're unlocking more ways of building immersive web experiences, by bringing Photorealistic 3D Maps to the familiar Maps JavaScript API from Google Maps Platform. Now, you can take advantage of Google's rendering technology to deliver rich 3D maps at the speed your users expect. The best part: you can get started with a single line of code.
We can't wait to see the stunning, immersive experiences you'll build! Learn more .
Every day, you're breaking through the complexity of development, pushing the limits of what's possible. We're excited to help you create new experiences for all of us to enjoy. Visit developer.chrome.com and web.dev to learn more about this powerful web, made easier. And be sure to connect with us on X , YouTube , and now LinkedIn .
See you at the next I/O!
Except as otherwise noted, the content of this page is licensed under the Creative Commons Attribution 4.0 License , and code samples are licensed under the Apache 2.0 License . For details, see the Google Developers Site Policies . Java is a registered trademark of Oracle and/or its affiliates.
Last updated 2024-05-14 UTC.
The Earthquake Event Page application supports most recent browsers, view supported browsers . Or, try our Real-time Notifications, Feeds, and Web Services .
This is a potential security issue, you are being redirected to https://csrc.nist.gov .
You have JavaScript disabled. This site requires JavaScript to be enabled for complete site functionality.
An official website of the United States government
Here’s how you know
Official websites use .gov A .gov website belongs to an official government organization in the United States.
Secure .gov websites use HTTPS A lock ( Lock Locked padlock icon ) or https:// means you’ve safely connected to the .gov website. Share sensitive information only on official, secure websites.
NIST Issues Updated Security Requirements and Assessment Procedures for Protecting Controlled Unclassified Information (CUI) May 14, 2024
NIST has published the final versions of Special Publication (SP) 800-171r3 (Revision 3) , Protecting Controlled Unclassified Information in Nonfederal Systems and Organizations , and SP 800-171Ar3 , Assessing Security Requirements for Controlled Unclassified Information . The security requirements and assessment procedures have been issued concurrently through the Cybersecurity and Privacy Reference Tool (CPRT) to give users additional ways to access the datasets (i.e., via browser, download as spreadsheet, and JSON).
Major updates to SP 800-171r3 include refinements for consistency with SP 800-53r5, such as:
- Restructured security requirements to show direct alignment with SP 800-53r5 controls
- Introduction of organization-defined parameters (ODP)
- New tailoring criteria to reduce potential redundancy and improve clarity
- Recategorization of controls based on the new tailoring criteria
SP 800-171r3 provides additional outcome-oriented guidance to reduce ambiguity and better support implementation.
NIST is also issuing a CUI Overlay that shows the direct SP 800-53 control item tailoring for the CUI security requirements. Other supplemental resources to assist implementers include an analysis of changes between SP 800-171r2 and SP 800-171r3 and an FAQ.
Similarly, SP 800-171Ar3 includes updates for consistency with the corresponding SP 800-171r3 security requirements and the source SP 800-53Ar3 assessment procedures, including:
- Modifications to the assessment procedure structure and syntax
- Inclusion of ODPs to facilitate traceability and usability
In response to the feedback received during the public comment period, additional guidance on conducting security requirement assessments was also included, and a one-time “revision number” change was made for consistency and alignment with SP 800-171r3.
NIST plans to release additional resources through the Online Informative References (OLIR), including crosswalks between SP 800-171r3 and SP 800-53r5, and the Cybersecurity Framework 2.0.
NIST has also issued a News Article, NIST Finalizes Updated Guidelines for Protecting Sensitive Information , about the release.
For more information about the NIST Protecting CUI Project and other resources, see: https://csrc.nist.gov/Projects/protecting-controlled-unclassified-information . Please direct questions and comments to [email protected] .
Parent Project
Related topics.
Security and Privacy: assurance , risk assessment , security controls
Laws and Regulations: Federal Information Security Modernization Act
Euro Convergence: Experts concerned about incompatibilities between AI Act and MDR

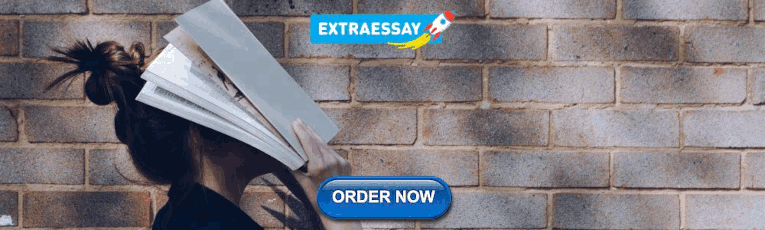
IMAGES
VIDEO
COMMENTS
JavaScript on a webpage may not read/write arbitrary files on the hard disk, copy them or execute programs. It has no direct access to OS functions. Modern browsers allow it to work with files, but the access is limited and only provided if the user does certain actions, like "dropping" a file into a browser window or selecting it via an ...
JavaScript is a very free-form language compared to Java. You do not have to declare all variables, classes, and methods. You do not have to be concerned with whether methods are public, private, or protected, and you do not have to implement interfaces. Variables, parameters, and function return types are not explicitly typed.
Introduction JavaScript is a scripting language most often used for client-side web development. JavaScript is an implementation of the ECMAScript standard. The ECMAScript only defines the syntax/characteristics of the language and a basic set of commonly used objects such as Number, Date, Regular Expression, etc. The JavaScript supported in ...
JavaScript is a scripting language that enables you to create dynamically updating content, control multimedia, animate images, and pretty much everything else. (Okay, not everything, but it is amazing what you can achieve with a few lines of JavaScript code.) The three layers build on top of one another nicely.
4. Starting to program • The following slides should appear familiar as you have done some programming before - If you are familiar with basic programming concepts such as variables, arrays, conditional statements and loops, you should be able to understand and use JavaScript quite rapidly. 5.
JavaScript overview. Interpreted language. But browsers are getting very good at running it quickly. Native execution in browser. No (exposed) underlying "assembly" language. Dynamically typed (like Python) No declared type, but values have types Variables can change types. Object-oriented. Everything is an object, including primitives and ...
It's essential to remember that JavaScript is a key tool for one job - websites. While JavaScript statements can run without being deployed to a site, dynamic web page presentation is really what JavaScript is all about. And JavaScript script execution typically occurs on the client system.
JavaScript was invented by Brendan Eich in 1995, and became an ECMA standard in 1997. ECMA-262 is the official name of the standard. ECMAScript is the official name of the language. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
In this video called "Presentation: Introduction To Javascript", Woz-U instructor Steve Bishop goes over what Javascript is, how we use Javascript, and how t...
Learn how to create your first JavaScript application with this beginner-friendly tutorial. Build a simple program to display a message to the user and start exploring the possibilities of this versatile language. Welcome to JavaScript! If you're brand new to JavaScript or Programming here's where you can start.
We want to make this open-source project available for people all around the world. Help to translate the content of this tutorial to your language!
JavaScript is the world's most popular programming language. JavaScript is the programming language of the Web. JavaScript is easy to learn. This tutorial will teach you JavaScript from basic to advanced. Start learning JavaScript now »
the canonical clickable UI control (inline) <button> Click me! </button>. button's text appears inside tag; can also contain images. To make a responsive button or other UI control: choose the control (e.g. button) and event (e.g. mouse click) of interest. write a JavaScript function to run when the event occurs.
What can JavaScript Do? • JavaScript can dynamically modify an HTML page • JavaScript can react to user input • JavaScript can validate user input • JavaScript can be used to create cookies (yum!) • JavaScript is a full-featured programming language • JavaScript user interaction does not require any communication with the server
introduction to JavaScript while also illustrating the context of when and where it should be used." —R. S. Doiel, Senior Software Engineer, USC Web Services "Learni ng JavaScript is a great introduction into modern JavaScript development. From covering the history to its exciting future, Learning JavaScript equips the novice developer
JAVA SCRIPT Introduction JavaScript is a object-based scripting language and it is light weighted. It is first implemented by Netscape (with help from Sun Microsystems). JavaScript was created by Brendan Eich at Netscape in 1995 for the purpose of allowing code in web-pages (performing logical operation on client side). It is not compiled but translated. JavaScript Translator is responsible to ...
JavaScript is a programming language used to create dynamic content for websites. It achieves this by adding new HTML elements while modifying existing ones. Many coders enhance web development skills using JavaScript to create user-friendly and interactive websites.
Create Stunning Presentations on the Web. reveal.js is an open source HTML presentation framework. It's a tool that enables anyone with a web browser to create fully-featured and beautiful presentations for free. Presentations made with reveal.js are built on open web technologies. That means anything you can do on the web, you can do in your ...
Presentation frameworks are tools or libraries that can help you create presentations using web technologies that you are familiar with, such as HTML, CSS, JavaScript, Markdown, Vue, React, and more. You'll have full control over the appearance and layout of your slides.
Flowtime.js is a framework for easily building HTML presentations or websites. It's built with web standards in mind and on top of a solid full page grid layout. The animations are managed with native and accelerated CSS3 transitions. Javascript takes care of the navigation behaviour and adds advanced functionalities and configuration options.
The Presentation API lets a user agent (such as a Web browser) effectively display web content through large presentation devices such as projectors and network-connected televisions. Supported types of multimedia devices include both displays which are wired using HDMI, DVI, or the like, or wireless, using DLNA, Chromecast, AirPlay, or Miracast.. In general, a web page uses the Presentation ...
A PowerPoint add-in interacts with objects in PowerPoint by using the Office JavaScript API, which includes two JavaScript object models: PowerPoint JavaScript API: The PowerPoint JavaScript API provides strongly-typed objects that you can use to access objects in PowerPoint. Common APIs: Introduced with Office 2013, the Common API can be used ...
impress.js is a presentation tool based on the power of CSS3 transforms and transitions in modern browsers and inspired by the idea behind prezi.com.
Presentations can be exported to PDF, here's an example: Global State. Set data-state="something" on a slide and "something" will be added as a class to the document element when the slide is open. This lets you apply broader style changes, like switching the page background. ... Extensive JavaScript API; Auto-progression; Parallax backgrounds ...
Research by Anna Luisa Rodriguez. The 26 top new restaurants in the D.C. area include Pastis, Moon Rabbit, Pascual, Bar Del Monte, Maple Room, Chay, El Presidente, Cielo Rojo and more.
Build 3D immersive experiences in Maps JavaScript API. We're unlocking more ways of building immersive web experiences, by bringing Photorealistic 3D Maps to the familiar Maps JavaScript API from Google Maps Platform. Now, you can take advantage of Google's rendering technology to deliver rich 3D maps at the speed your users expect. The best ...
The Earthquake Event Page application supports most recent browsers, view supported browsers.Or, try our Real-time Notifications, Feeds, and Web Services.Real-time Notifications, Feeds, and Web Services.
NIST is also issuing a CUI Overlay that shows the direct SP 800-53 control item tailoring for the CUI security requirements. Other supplemental resources to assist implementers include an analysis of changes between SP 800-171r2 and SP 800-171r3 and an FAQ. Similarly, SP 800-171Ar3 includes updates for consistency with the corresponding SP 800 ...
BERLIN - Experts are concerned that the recently adopted European Artificial Intelligence (AI) Act is in some ways incompatible with the Medical Device Regulation (MDR), in part due to conflicting terminology across the two documents. During the 2024 RAPS Euro Convergence, a panel of experts discussed how medical devices under the AI Act ...