How to Find the Smallest Number in a Python List [8 Ways]
In this Python tutorial, I will explain how to find the smallest number in a Python list . We will use different methods to write a Python program to find smallest number in a list .
We aim to create a Python program to generate the smallest number in the given list of numbers.
We’ll review multiple techniques that make finding the smallest number in a Python list easy.
During my research, I found eight methods to find the smallest number in a list in Python . Here is the list:
- Using the min() function
- Using a loop
- Using the sort() function
- Using sorted() function
- Using lambda function
- Using reduce() function
- Using recursion
- Using the heapq module
Table of Contents
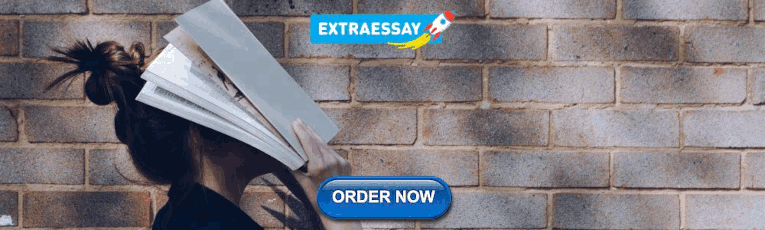
Find the smallest number in a Python list
A list is a collection of items in Python. To find the smallest number in a list, we can use the min() function , which gives the smallest number from the passed list.
We can also sort the list and then extract the first or last element according to the order using different methods in Python.
Let’s see them all in detail with examples:
1. Python program to find smallest number in a list using the min() function
Python has a built-in min() function , which returns the smallest item in an iterable or the smallest of two or more arguments. We can use the min() function in the Python list to find the smallest number.
Here is an instance:
For the above code, we have created a Python list called zip_code_USA that contains several zip codes.
- It then uses the built-in min() function to find the smallest number in the list and stores the result in a variable called new_output .
After implementing the code in the Pycharm editor, the screenshot is mentioned below.

This is how to find the smallest number in a Python list using the min() function.
2. Find smallest number in Python using for loop
We can also use a for loop in Python to iterate through each element of the Python list and compare it with the smallest number found.
We will use the if conditional statements in Python to check for the smallest number while comparing.
Here is an example to find smallest number in Python using for loop :
The code creates a list of integers called populations and initializes a variable called smallest_population to the first element of the list.
- It then iterates over each element of the list using a for loop in Python and checks if the current element is smaller than the current value of the smallest_population variable.
- If it is, the smallest_population variable is updated with the current element. Finally, the code prints the smallest element in the list using the print() function.
Below is a screenshot displaying the output after implementing the Pycharm editor’s code.

3. Python program to find smallest number in a list using the sort() function
We can also use the Python sort() function to sort the elements of a list in ascending order. We can find the smallest number by sorting the list and selecting the first element by indexing in Python.
Let’s see an example of using the sort() function to find the smallest number in a Python list.
The above code creates a list of integers called temperatures_nyc .
- Then, sort the list in ascending order using the sort() method in Python and extract the first element from the sorted list.
Upon running the code in Pycharm, the resulting output is displayed in the screenshot below.

In this example, we have understood how to find the smallest number in a Python list using a sort() function.
4. How to find smallest number in list Python using the sorted() function
We can use the sorted() function in Python to sort the list in ascending order and then take the first element, which will be the smallest, similar to the last method.
The sorted() function returns a sorted list of the specified iterable object. We can also sort the list in descending order and extract the last element, as it will be the smallest element
Here is an instance to get the smallest item in the Python list:
The above code creates a list of ages in numbers called employee_ages .
We use the built-in sorted() function with a reverse parameter to sort the list in descending order, storing the result in a new variable called sorted_employee_age .
Then, we access the last element of the sorted list by indexing in Python.
- The len() function in Python returns the length of the list, and as the indexing starts from 0, we subtract one (1) from the length to get the last element.
- Note: We can also use the pop() method to get the last element from the sorted list in Python.
The screenshot below presents the output after the code was successfully implements the Pycharm editor.

5. How to find smallest element in list Python using lambda function
- This section will discuss how to find the smallest number in a Python list using the lambda function .
- A lambda function only has one expression. They can be used to create a function object, which might or might not yield results.
- We can combine it with the min() function to find the lowest number in the Python list.
Let’s take an example and check how to find the smallest number in a Python list using the lambda function:
Source Code:
Here, we have used the lambda function to filter the list of integers and then find the minimum value among the filtered list elements in Python using the min() function .
Here is the execution of the following given code:

6. How to find the smallest number in a list Python using the reduce() function
You can use the reduce() function from the functools module of Python to iteratively apply a function to pairs of elements in the list until we are left with a single value, i.e., the smallest number from a list in Python.
Below is an example that explains how to use the reduce() function in Python to get the smallest number in a list.
The above code imports the reduce() function from the functools library in Python.
- The reduce() function applies a lambda function to find the smallest element in the list using comparison operators and stores the result in a variable called lowest_production .
- Finally, the code prints the smallest element in the list using the print() function.
The screenshot below features the output after executing the Pycharm editor’s code.

7. Find smallest number in the list Python using recursion
We can also use the recursion technique to find the smallest number in a list in Python.
Recursion is a technique where a function calls itself in its definition.
Here is an example:
Here, we recursively compare the first element of the list to the rest of the elements using the min() function in Python until we are left with a single element in the list, which is the smallest number in the Python list.
After executing the code in Pycharm, one can see the output in the below screenshot.

8. How to find the smallest number in a list Python using the heapq module
If you have a large list and want to find the smallest element without sorting the entire list, you can use the heapq module in Python to create a heap and find the smallest element from a Python list in constant time.
Here is an instance to find the smallest number in the Python list:
The above code imports the heapq library, which provides an efficient way to find the smallest elements in a list. It then creates a list of integers called my_list .
- The code uses the heapify() function from the heapq library in Python to transform a regular list into a heap. In other words, it rearranges the list in place into a Min heap.
- Then, the heappop() function pops the smallest element while preserving the heap property.

This tutorial contains eight methods to find the smallest number in a Python list, like the min() function, a for loop, sort() function, sorted() function, lambda function, reduce() function, recursion, and different functions of the heapq module .
Depending on the size of the Python list and specific requirements, one can choose the most suitable method for their task.
You may also like the following Python list tutorials:
- How to Split String by Space into List in Python
- How to Prepend to a List in Python
- How to find the Mean of a List in Python
- How to Get the Index of the Minimum Element of a List in Python
- How to Get the First and Last Digit of a Number in Python

I am Bijay Kumar, a Microsoft MVP in SharePoint. Apart from SharePoint, I started working on Python, Machine learning, and artificial intelligence for the last 5 years. During this time I got expertise in various Python libraries also like Tkinter, Pandas, NumPy, Turtle, Django, Matplotlib, Tensorflow, Scipy, Scikit-Learn, etc… for various clients in the United States, Canada, the United Kingdom, Australia, New Zealand, etc. Check out my profile .

- Online Python Compiler
- Hello World
- Console Operations
- Conditional Statements
- Loop Statements
- Builtin Functions
- Type Conversion
Collections
- Classes and Objects
- File Operations
- Global Variables
- Regular Expressions
- Multi-threading
- phonenumbers
- Breadcrumbs
- ► Python Examples
- ► ► List Operations
- ► ► ► Python Program to Find Smallest Number in List
- Python Strings
- Python List Tutorials
- Python Lists
- Python List Operations
- Create Lists
- Python – Create an empty list
- Python – Create a list of size n
- Python – Create a list of numbers from 1 to n
- Python – Create a list of strings
- Python – Create a list of objects
- Python – Create a list of empty lists
- Access Lists
- Python List – Access elements
- Python List – Get item at specific index
- Python List – Get first element
- Python List – Get last element
- Python List – Iterate with index
- Python List – While loop
- Python List – For loop
- Python List – Loop through items
- Python – Unpack List
- List Properties
- Python – List length
- List Methods
- Python List Methods
- Python List append()
- Python List clear()
- Python List copy()
- Python List count()
- Python List extend()
- Python List index()
- Python List insert()
- Python List pop()
- Python List remove()
- Python List reverse()
- Python List sort()
- Update / Transform Lists
- Python – Add item to list
- Python – Remove specific item from list
- Python – Remove item at specific index from list
- Python – Remove all occurrences of an item from list
- Python – Remove duplicates from list
- Python – Append a list to another list
- Python – Reverse list
- Python – Sort list
- Python – Sort list in descending order
- Python – Shuffle list
- Python – Convert list into list of lists
- Checking Operations on Lists
- Python - Check if list is empty
- Python - Check if element is present in list
- Python - Check if value is in list using "in" operator
- Python - Check if list contains all elements of another list
- List Finding Operations
- Python – Count the occurrences of items in a List with a specific value
- Python – Find duplicates in list
- Python – Find unique elements in list
- Python – Find index of specific item in list
- Python – Insert item at specific position in list
- Python – Find element with most number of occurrences in the list
- Python – Find element with least number of occurrences in the list
- List Comprehension Operations
- Python List Comprehension
- Python List Comprehension with two lists
- Python List Comprehension with If condition
- Python List Comprehension with multiple conditions
- Python List Comprehension with List of Lists
- Python List Comprehension with nested For loops
- List Filtering
- Python – Filter list
- Python – Filter list of strings that start with specific prefix string
- Python – Filter list based on datatype
- Python – Get list without last element
- Python – Traverse list except last element
- Python List – Get first N elements
- Python List – Get last N elements
- Python List – Slice
- Python List – Slice first N elements
- Python List – Slice last N elements
- Python List – Slice all but last
- Python List – Slice reverse
- List Conversions to other Types
- Python – Convert List to Dictionary
- Python – Convert dictionary keys to list
- Python – Convert dictionary values to list
- Python – Convert list to tuple
- Python – Convert tuple to list
- Python – Convert list to set
- Python – Convert set to list
- Python – Convert list to string
- Python – Convert CSV string to list
- Python – Convert list to CSV string
- Python – Convert string (numeric range) to a list
- Python – Convert list of lists to a flat list
- Python - Convert list of strings into list of integers
- Python - Convert list of strings into list of floats
- Python - Convert list of integers into list of strings
- Python - Convert list of floats into list of strings
- Numeric Lists
- Python – Find average of numbers in list
- Python – Find largest number in list
- Python – Find smallest number in list
- Python – Find sum of all numbers in numeric list
- Python – Find product of all numbers in numeric list
- Python – Sort numbers
- String Lists
- Python – Compare lists lexicographically
- Python – Find shortest string in list
- Python – Find longest string in list
- Python – Find most occurred string in a list
- Python – Find least occurred string in a list
- Python – Sort list of strings based on length
- Python – Sort a list alphabetically
- Python - Join list with new line
- Python - Join list with comma
- Python - Join list with space
- Printing Lists
- Python - Print list
- Python - Print list without brackets
- Python - Print list without commas
- Python - Print list without quotes
- Python - Print list without spaces
- Python - Print list without brackets and commas
- Python - Print list without brackets and quotes
- Python - Print list without quotes and commas
- Python - Print list in reverse order
- Python - Print list in JSON format
- Python - Print list with double quotes
- Python - Print list with index
- Python - Print list as column
- Python - Print list as lines
- Python - Print elements at even indices in List
- Python - Print elements at odd indices in List
- Exceptions with Lists
- Python List ValueError: too many values to unpack
- Python List ValueError: not enough values to unpack
- List Other Topics
- Python List of Lists
- Python – Print a list
- Python List vs Set
- Python Dictionary
Python Program to Find Smallest Number in List
- Find the smallest number in given list using min()
- Find the smallest number in given list using sort() function
- Find the smallest number in given list using For loop
Find Smallest Number in Python List
You can find the smallest number of a list in Python using min() function, sort() function or for loop.
We shall look into the following processes to find the smallest number in a list, with examples.
- Use min() builtin function
- Use List sort() function
- Use Python For Loop
Choose one, based on your program requirements or your own personal performance recommendations.
1. Find the smallest number in given list using min()
min() function can take a list as argument and return the minimum of the elements in the list.
In this example, we will take a list of numbers and find the smallest of the list using min() function.
Python Program
2. Find the smallest number in given list using sort() function
We know that sort() function sorts a list in ascending or descending order. After you sort a list, you have your smallest number at the start of the list if you have sorted in ascending order or at the end of the list if you have sorted in descending order.
3. Find the smallest number in given list using For loop
Though finding the smallest number using sort() function is easy, using Python For Loop does it relatively faster with less number of operations. And also, we do not change the order of elements in the given list.
In this example,
- We have assumed a list and initialized largest number variable to first element of the list. If the list is empty, smallest number will be None.
- During each iteration, we checked if the smallest number is greater than the element. If that is the case, we assigned our smallest number with the element.
- When you complete traversing the list, you will end up with the smallest number of the list in your variable.
In this tutorial of Python Examples , we learned some of the ways to find the smallest number in a Python list with the help of well detailed Python example programs.
Related Tutorials
- Selection Sort
- Python Program To
- Iterative Merge Sort
- Find Largest Prime Factor
- Maximum and Minimum Elements from a tuple
- Iterative QuickSort
- Binary Search
- Linear Search
- Insertion Sort
- Insertion sort
- Bubble Sort
- Counting sort
- Topological sort
- Binary Insertion Sort
- Bitonic sort
- Print Own Font
- Print Current Time
- Swap two numbers using third variable
- Swap two numbers without using third variable
- Binary Anagram
- Find Mirror Characters
- Python Convert Snake
- Area of Square
- Add Two Numbers
- Handle Missing Keys
- Find Factorial
- Simple Interest
- Compound Interest
- Armstrong Number
- Area of Circle
- Prime Number
- Find nth Fibonacci Number
- Print ASCII
- Find Sum of Squares
- Find Sum of Cubes
- Find Most Occurring Char
- Python Regex to
- List of Tuples
- Display Keys associated with Values in Dictionary
- Find Closest Pair to Kth index element in Tuple
- Sum of Array
- Largest in Array
- Array Rotation
- Rotate Array by Reversal
- Split and Merge Array
- Monotonic Array
- Key-Values List To Dictionary
- Print Anagrams in LIst
- Interchange First and Last
- Swap List Elements
- Find List Length
- Find Element in List
- Clear a List
- Reverse a List
- Sum of List Elements
- Product of List Elements
- FInd Small in List
- Find Largest in List
- Find Second Largest
- Find N Largest in List
- Find Even in List
- Find Odd in List
- FInd All Even in List
- All Positive in List
- Negative in List
- Remove Multiple From List
- Clone a List
- Find Occurrence in List
- Remove Empty Tuple
- Duplicate in List
- Merge Dictionaries
- Extract Unique From Dictionary
- Sort Dictionary
- Keys having multiple inputs
- Sum of Dictionary Values
- Remove Key from Dictionary
- Insert at Start in Dictionary
- Counting the frequencies
- Add Space between Words
- Python Program to
- Python Program for
- Rotate String
- Palindrome String
- Replace String Words
- Duplicate String
- Substring String
- Binary String
- Max Frequent Char
- String Remove Char
- Remove Duplicate
- Count Words Frequency
- Symmetrical String
- Generating Random String
- String Split and Join
- Reverse String Words
- Find Words using Chars
- Special Chars
- Find length of
- Remove Char
- String Char Order
- Even String
- Vowels in String
- Count Matching Char
- Remove Duplicates in String
- Find Greater Words
- Find URL in String
- Pigeonhole Sort
- BogoSort Algorithm
- Stooge Sort
- Heap Sort Program
- Python program for Insertion sort
- Join Tuples If Similar
- Extract Digit from Tuples List
- Pair Combinations of 2 tuples
- Remove Tuples of Length K
- Adding Tuple To List
- Transpose Matrix
- Matrix Multiplication
- Matrix Add | Sub
- Print G Pattern
- Printed Inverted Star
- Double-sided staircase Pattern
- String Contains Specified Chars
- Check if string start with same char
- Count Uppercase Chars
- Print Current Date
- Find Dates of yesterday today tomorrow
- Time 12 hours to 24 hours
- Difference of Current Time and Given Time
- Create Lap Timer
- Binary search
- Bubble Sort Program
- create Circular Linked List of N nodes and count No. of node
- Create Circular Link List of and display it in reverse order
Python program to find smallest number in a list
In this tutorial, you will learn how to find the smallest number in a list. List is an ordered set of values enclosed in square brackets [ ]. List stores some values called elements in it, which can be accessed by their particular index. In this tutorial, we will be discussing the various approaches by which we can find the smallest element in the given list.
For a given list of numbers, the task is to find the smallest number in the list.
Input: [10, 3, 20, 9, 11, 15, 23, 6]
Approach to find smallest number in a list
For executing this program in Python, there are multiple approaches we can follow:
- By comparing each element for finding the smallest number
- By using min() function
- By sorting the list using sort() function and printing the smallest element
Let us look at each approach in detail.
Approach 1: Comparing elements
In this approach, we will run a loop from the starting to the ending of the list and compare each element individually to find the minimum element. We will use for loop in this approach.
Follow the algorithm to understand the approach better
Step 1- Define a function that will find the smallest number
Step 2- Declare a variable that will store the smallest value
Step 3- Initialise it to the first value in list
Step 4- Run a loop for all elements in list
Step 5- Compare each element with the variable which stores the smallest value
Step 6- If the element is smaller than the value in the variable
Step 7- Copy the element in the variable
Step 8- Return the variable
Step 9- Declare and initialize list or take input
Step 10- Call function and print output
Python Program 1
Look at the program to understand the implementation of the above-mentioned approach.
smallest in [3, 9, 7, 3, 6, 5, 7, 24, 6] is 3
We have used '\n' for printing the statement in the next line. It is an escape sequence new line character.
Approach 2: min() function
In this approach, we will use min() method which is a built-in function in the Python library. It will return the smallest value in the list.
Step 1- Declare a function that will find the smallest number
Step 2- Use min() method and store the value returned by it in a variable
Step 3- Return the variable
Step 4- Declare and initialize a list or take input
Step 5- Call the function and print the value returned by it
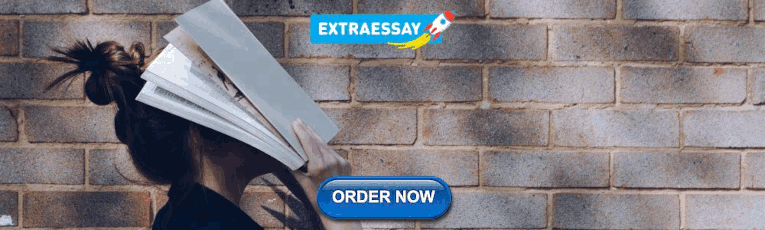
Python Program 2
Enter size of list4 Enter element of list 9 Enter element of list 4 Enter element of list 29 Enter element of list 4 smallest in [9, 4, 29, 4] is 4
Approach 3: by sorting and printing the smallest number
In this approach, we will first sort the elements of the list. For sorting the list we will use the predefined sort() function in Python. It will sort the elements in the list in ascending order. We will print the element present at the first position after sorting. This will be the smallest element in the list.
Step 1- Declare a function for finding the smallest number
Step 2- Use sort() method to sort the list
Step 3- Return the first element in the list after sorting
Step 4- Declare a list and take input or initialize values
Step 5- Call the function
Step 6- Print the value returned by the function
Python Program3
Enter size of list 5 Enter element of list 3 Enter element of list 9 Enter element of list 6 Enter element of list 0 Enter element of list 6 smallest in [3, 9, 6, 0, 6] is 0
So far, we have discussed three ways by which we can find the smallest element in the list. We have discussed how to use a for loop for comparing each element in the list and finding the minimum element. We have also used built-in functions like min() and sort() to find the smallest element in the list.
- ← Product of List Elements ← PREV
- Find Largest in List → NEXT →
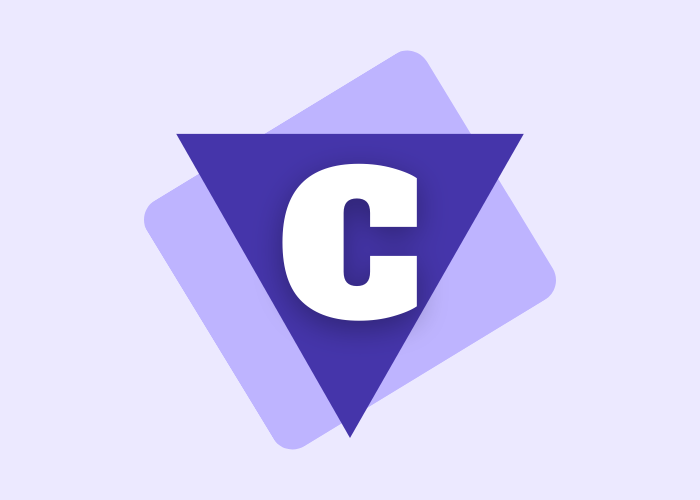
3 Easy Methods to Find the Smallest Number in Python
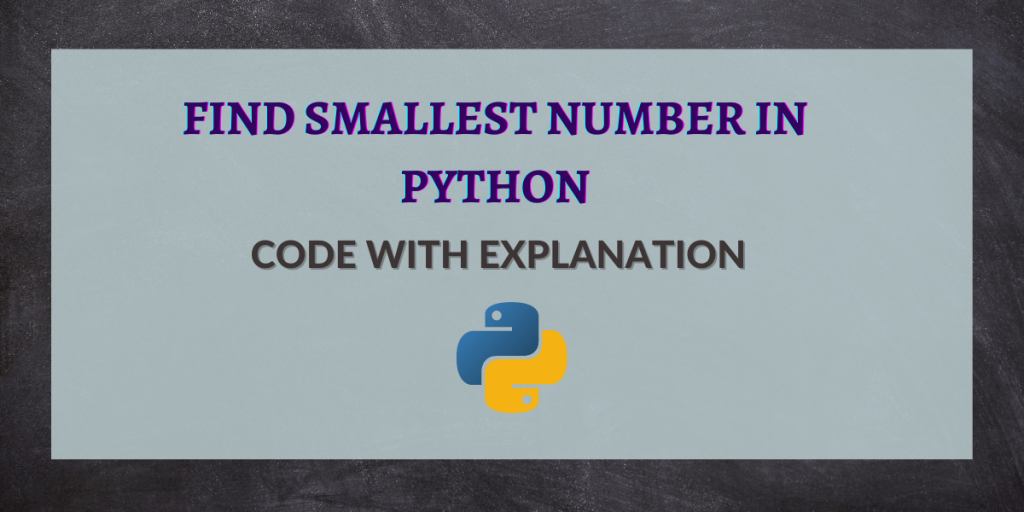
Hello there! This article is for beginners who wish to understand the basic code for finding the smallest number in Python. So let’s begin.
How to Find the Smallest Number in Python?
We aim to find the smallest number in Python of all the numbers given in a list.
Say if the list is: [32, 54, 67, 21]
The output should be: 21
In this article, we will understand 3 different methods to do this.
1. Using Python min()
Min() is a built-in function in python that takes a list as an argument and returns the smallest number in the list. An example is given below-
This is one of the simplest methods to find the smallest number. All you need to do is to pass the list to min() as an argument.
2. Using Python sort()
Sort() is another inbuilt method in python that doesn’t return the smallest number of the list . Instead, it sorts the list in ascending order.
So by sorting the list, we can access the first element of the list using indexing and that will be the smallest number in that list. Let’s see the code:
3. Using the ‘for’ loop
In the above code, we are using two for loops , one for getting the elements of the list from the user and the second one for finding the smallest number from the list.
After getting the elements from the user, we define the first element of the list (at 0 index) as the smallest number (min). Then with the for loop, we compare each element of the list to the min and if any element is smaller than min , it becomes the new min .
This is how we get the smallest number from the user-given list.
The output for the above code is:
So, these were some methods to find the smallest number from the given list in python. Hope you understood this! Feel free to ask questions below, if any. Thank you! 🙂
Finding the smallest number in a list can be simple with Python.
Finding the smallest number in a list in python is often required for many tasks like finding outliers, identifying min and max values etc. Here’s how you do it. …
Finding the smallest number in a list in python is often required for many tasks like finding outliers, identifying min and max values etc. Here’s how you do it.
This code snippet initializes a list of numbers and then applies the min() function to find the smallest number. This function returns the smallest value in an iterable or raises ValueError if given an empty iterable. The result is printed on the screen.
In the context of this problem, ‘outliers’ refers to data points that are distant from the majority and can distort the results. For example, if we have a list [1,2,3,4,5] and suddenly include 999 into it, the min function will still correctly return 1 as the smallest number, but this ‘outlier’ value might not be what we expect in some contexts.
The min() function can also be used to find out the minimum and maximum values in a list by passing multiple parameters:
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Sort the values of first list using second list in Python
- Python | Sort a list according to the second element in sublist
- Get Second Largest Number in Python List Using Bubble Sort
- Second largest value in a Python Dictionary
- Python program to find second largest number in a list
- Python program to sort a list of tuples by second Item
- Python Program to Print Largest Even and Largest Odd Number in a List
- Python - Sort by Frequency of second element in Tuple List
- Python | Sort dictionary by value list length
- Python | Ways to sort list of float values
- Python - Sort Records by Kth Index List
- Python - Keys with shortest length lists in dictionary
- Python - Group first elements by second elements in Tuple list
- Python - Split List on next larger value
- Rearrange an array in order - smallest, largest, 2nd smallest, 2nd largest, ..
- Find the largest and second largest value in a Linked List
- Second Smallest Element in a Linked List
- Sudo Placement[1.5] | Second Smallest in Range
- Largest and smallest digit of a number
Python | Largest, Smallest, Second Largest, Second Smallest in a List
Since, unlike other programming languages, Python does not have arrays, instead, it has list. Using lists is more easy and comfortable to work with in comparison to arrays. Moreover, the vast inbuilt functions of Python, make the task easier. So using these techniques, let’s try to find the various ranges of the number in a given list.
Input : list = [12, 45, 2, 41, 31, 10, 8, 6, 4] Output : Largest element is: 45 Smallest element is: 2 Second Largest element is: 41 Second Smallest element is: 4 Input : list = [22, 85, 62, 40, 55, 12, 39, 2, 43] Output : Largest element is: 85 Smallest element is: 2 Second Largest element is: 62 Second Smallest element is: 12
Approach#1: The approach is simple. Python allows us to sort a list using the list() function. Using this we can find various ranges of numbers in a list, from their position, after being sorted. Like the first position must contain the smallest and the last element must be the greatest.
Time Complexity: O(n*log(n)) Auxiliary Space: O(1)
Approach#2: Below is another traditional method to do the following calculation. The algorithm is simple, we take a number and compare it with all other numbers present in the list and get the largest, smallest, second largest, and second smallest element.
Time Complexity: O(N) Auxiliary Space: O(1)
Approach#3: This task can be performed using max and pop methods of list. We can find largest and smallest element of list using max and min method after getting min and max element pop outs the elements from list and again use min and max element to get the second largest and second smallest element.
The time complexity of this code is O(n) as it makes two linear scans of the list, one for finding the largest and smallest elements and another for finding the second largest and smallest elements. The pop operation is O(n) so the overall complexity is O(n). The space complexity is O(1) as the code only uses a constant amount of extra space.
Approach #3 : Using sort() and extend() methods
Time Complexity : O(NlogN) Auxiliary Space : O(N)
Approach#5: Using min and max
This approach finds the smallest, largest, second smallest, and second largest elements in a list by using the min and max functions and removing the elements from the list. The approach is concise and uses built-in functions for efficiency.
1. Use the min() and max() functions to find the smallest and largest elements. 2. Remove the smallest and largest elements from the list. 3. Use min() and max() functions again to find the second smallest and second largest elements.
Time complexity: O(n^2) due to the removal operation which takes O(n) time. Auxiliary Space: O(1)
Please Login to comment...
Similar reads.
- Python list-programs
- python-list
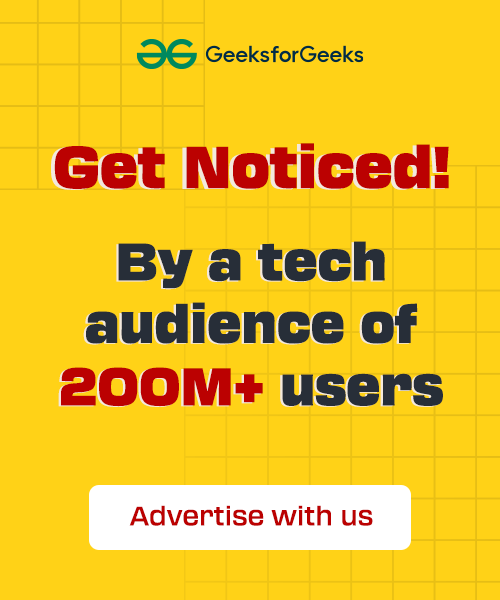
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
- Smallest Missing Number in Python
Problem Statement:
In the smallest missing number in Python, we are given a list of numbers, and we need to find the smallest missing number using the Python program. For example, [1, 2, 3, 3, 5, 7, 7, 4, 4, 9, 8] =>sorting=> [1, 2, 3, 3, 4, 4, 5, 7, 7, 8, 9] , 6 is the smallest missing number.
Code for Smallest Missing Number in Python:
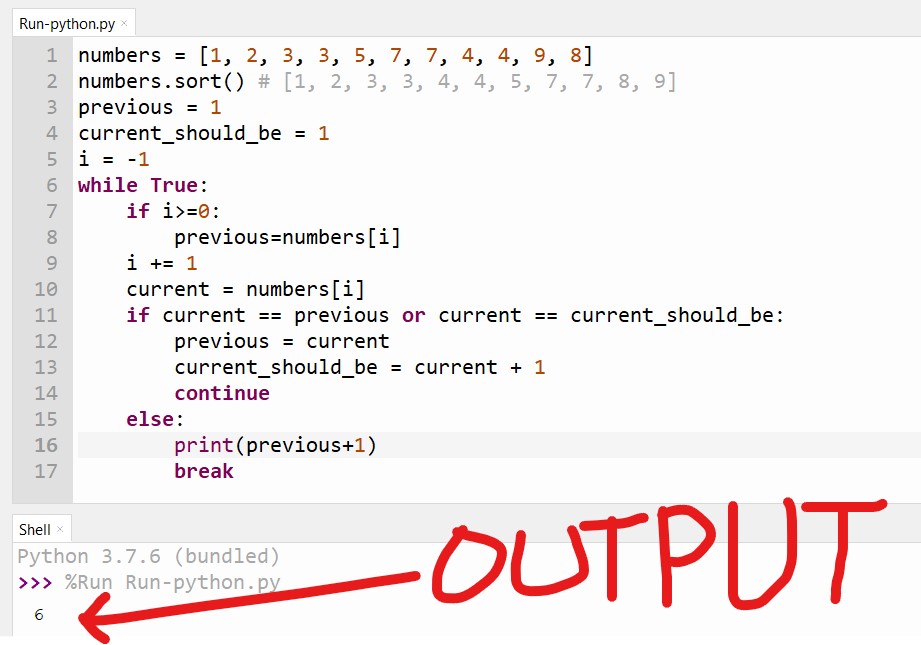
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Weekend in Python
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
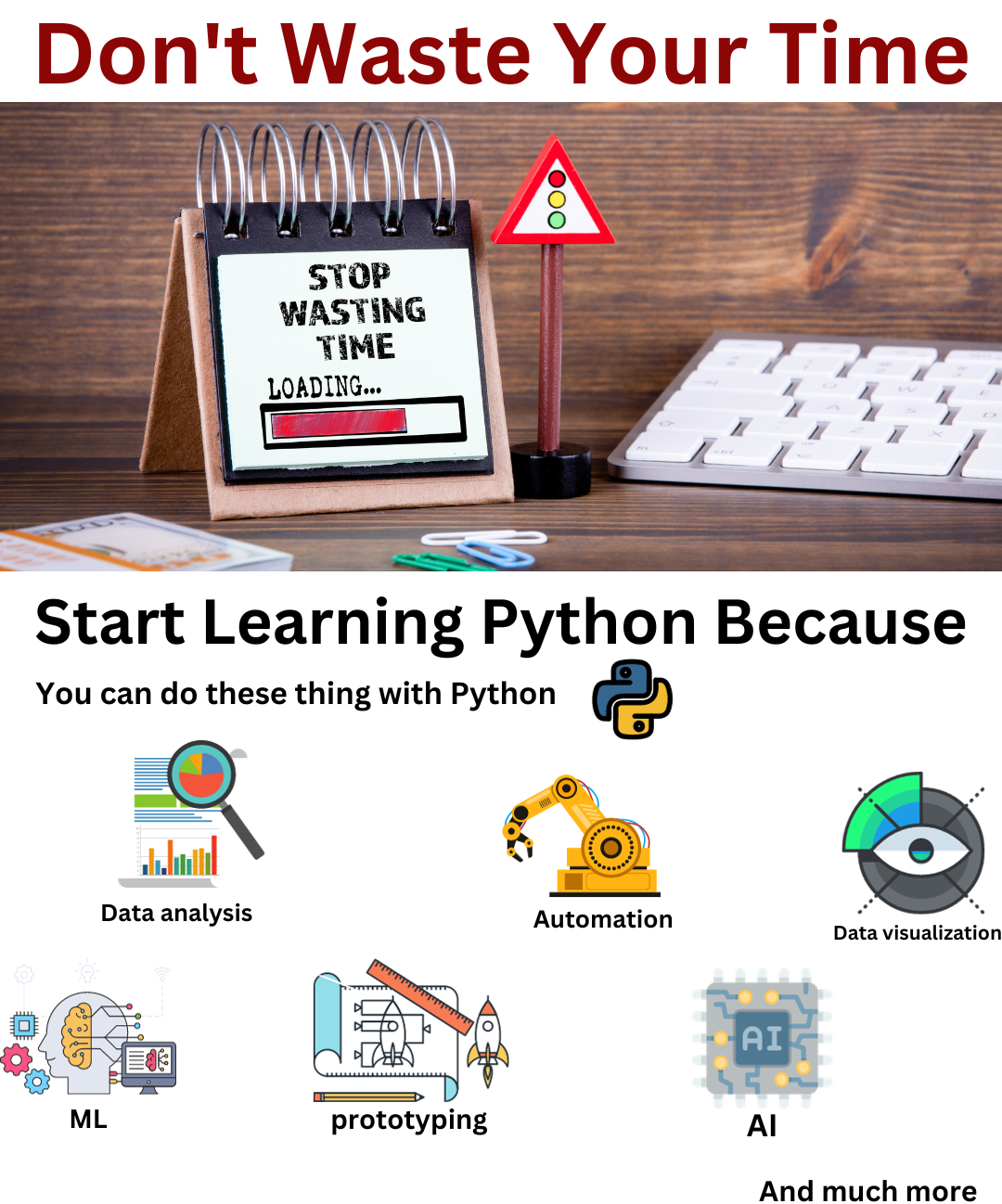
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
© Copyright 2019-2023 www.copyassignment.com. All rights reserved. Developed by copyassignment
- How it works
- Homework answers
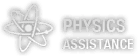
Answer to Question #228701 in Python for srikanth
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Leave a comment
Ask your question, related questions.
- 1. Replacing Characters of Sentence You are given a string S. Write a program to replace each letter
- 2. Diamond Given an integer value N, write a program to print a number diamond of 2*N -1 rows as sho
- 3. Diamond Crystal Given an integer value N, write a program to print a diamond pattern of 2*N rows
- 4. Number Diamond Given an integer N as input, write a program to print a number diamond of 2*N -1 r
- 5. Product of the Given NumbersGiven an integer N, write a program which reads N inputs and prints the
- 6. Day Name - 2Given the weekday of the first day of the month, determine the day of the week of the gi
- 7. Denominations - 2The possible denominations of currency notes are 100, 50, 20 and 10. The amount A t
- Programming
- Engineering
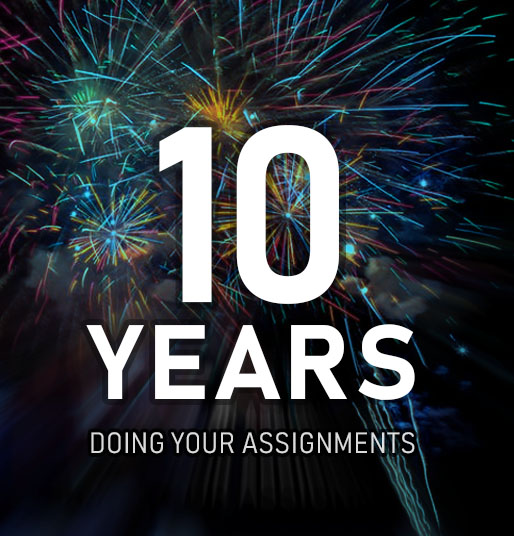
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
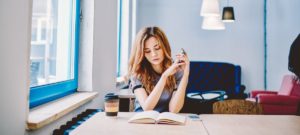
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
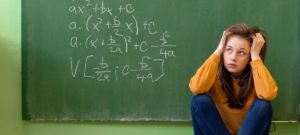
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…
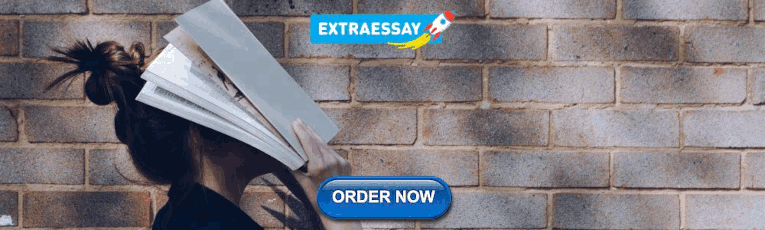
IMAGES
VIDEO
COMMENTS
We are given a list of numbers and our task is to write a Python program to find the smallest number in given list. For the following program we can use various methods including the built-in min method, sorting the array and returning the last element, etc. Example: Input : list1 = [10, 20, 4] Output : 4. Input : list2 = [20, 10, 20, 1, 100]
Question #187021. Smallest Missing Number. Given a list of numbers, write a program to print the smallest positive integer missing in the given numbers. Input. The input will be a single line containing numbers separated by space. Output.
2. Find smallest number in Python using for loop. We can also use a for loop in Python to iterate through each element of the Python list and compare it with the smallest number found.. We will use the if conditional statements in Python to check for the smallest number while comparing.. Here is an example to find smallest number in Python using for loop: ...
Find Smallest Number in Python List. You can find the smallest number of a list in Python using min() function, sort() function or for loop. We shall look into the following processes to find the smallest number in a list, with examples. Use min() builtin function; Use List sort() function; Use Python For Loop
Step 1- Define a function that will find the smallest number. Step 2- Declare a variable that will store the smallest value. Step 3- Initialise it to the first value in list. Step 4- Run a loop for all elements in list. Step 5- Compare each element with the variable which stores the smallest value. Step 6- If the element is smaller than the ...
you want the n first or 2 first? this is one way to get the 2 first lowest numbers in a list: list1=[1,15,9,3,6,21,10,11] list1.sort() twoFirst = list1[:2] nFirst = list1[:n] I am probably deleting my answer as someone suggested while I was writing my answer. This was return the same number multiple times if there are relevant duplicates.
In this article, we will understand 3 different methods to do this. 1. Using Python min () Min () is a built-in function in python that takes a list as an argument and returns the smallest number in the list. An example is given below-. #declaring a list. list1 = [-1, 65, 49, 13, -27] print ("list = ", list1)
Python Program to find the Smallest Number in a List using the sort function. The sort function sorts List elements in ascending order. Next, we are using Index position 0 to print the first element in a List. a = [100, 50, 60, 80, 20, 15] a.sort() print(a[0]) 20. This list's smallest number is the same as above.
Use Python's min() and max() to find smallest and largest values in your data. Call min() and max() with a single iterable or with any number of regular arguments. Use min() and max() with strings and dictionaries. Tweak the behavior of min() and max() with the key and default arguments.
Finding the smallest number in a list in python is often required for many tasks like finding outliers, identifying min and max values etc. Here's how you do it. # Initializing a sample list of numbers numbers = [ 45 , 67 , 23 , 10 , 89 , 64 , 98 , 5 ] # Using the min() function to find out the smallest number in the list smallest_number ...
Question #182869. Smallest Missing Number. Given a list of numbers, write a program to print the smallest positive integer missing in the given numbers.Input. The input will be a single line containing numbers separated by space.Output. The output should be a single line containing the smallest missing number from given numbers.Explanation.
This function will be used to find the smallest number in the list. min = list [ 0 ] -> We assume the list is not empty. This line initializes a variable called 'min' to the first element of the list. for a in list: -> This line starts a loop that will iterate over each element in the list, one at a time. The loop variable 'a' will take on the ...
Question #189799. Smallest Missing Number. Given a list of numbers, write a program to print the smallest positive integer missing in the given numbers. Input The input will be a single line containing numbers separated by space. Output The output should be a single line containing the smallest missing number from given numbers.
The algorithm is simple, we take a number and compare it with all other numbers present in the list and get the largest, smallest, second largest, and second smallest element. Python3. # second largest and second smallest in a. # list with complexity O(n) def Range(list1): largest = list1[0] lowest = list1[0]
Jason's right: rather than elif smallest is None, which won't be tested if any of the first three tests are true, use if smallest is None-- largest > number is always true if the number is a candidate for being smallest, and both the continue and elif will mean the next lines don't get run.; if you want an explicit "does nothing" clause, you might want pass instead
Problem Statement: In the smallest missing number in Python, we are given a list of numbers, and we need to find the smallest missing number using the Python program.
Question #228701. Largest Number in the List. You are given space -separated integers as input. Write a program to print the maximum number among the given numbers. Input. The first line of input contains space -separated integers. Explanation. In the example, the integers given are. 1, 0, 3, 2, 9, 8.
I have seen things on how to sort out a list but I am having trouble figuring out how print the smallest number that a user inputs from from a total of 3 numbers that they input. I have found some answers on here for other languages and I am sure this has been asked before but I have had trouble finding anything that helps this particular ...
With our help, you can master Python programming and tackle any assignment with confidence. In conclusion, mastering Python programming requires dedication, practice, and expert guidance.
I need to create a function that will print the smallest, largest,and average of n amount of numbers inputed by user. ... sm += x is augmented assignment which the same as doing sm = sm + x. ... largest and smallest number using input while loop python. 0. Calculate Maximun, Minimun, Average of n numbers ...