Next: Unions , Previous: Overlaying Structures , Up: Structures [ Contents ][ Index ]
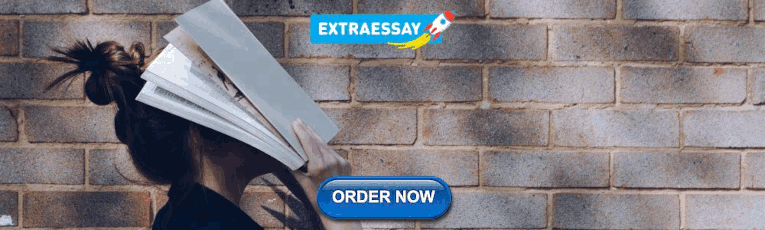
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
Search anything:
Copy struct in C [Shallow & Deep Copy]
C programming.
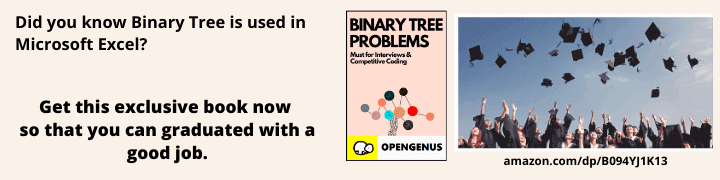
Open-Source Internship opportunity by OpenGenus for programmers. Apply now.
In this article, we have explained how to copy a struct in C Programming Language to another struct. We have covered different cases including Shallow and Deep copy.
Table of Contents
- Introduction
Copy by assignment to structure variable
Deep copy and shallow copy, deep copy of struct in c, shallow copy of struct in c, introduction.
What is a struct?
A structure is a collection of variables of various data types that can be manipulated together. Using a structure has the advantage of making coding easier and making the code easier to understand. In this article we will learn different ways to copy struct in C language.
Copying is to reuse some or all of the data of the original object, and create a new object by copying the original object. In programming it is the process of making an exact copy of the object or variable, i.e., both the object or variables must have the same value.
When you create a copy of something, you expect it to be an independent object and similar to the thing you copied. In programming that definition can vary in many ways. So, we are also going to study in depth about shallow and deep copy.
Approach Explained:
A variable defined in a structure is called a structure variable . Since this structure variable is a variable, it can be assigned to another variable. If the assignment destination variable has the same structure as the assignment source variable, the assignment expression can copy the structure variable as shown in the following implemented code.
Implemented Code:
In programming to make a copy means to store the data in different variables.
However there is an extra factor to consider in all of this. That is whether the copy is a deep copy or a shallow copy. To understand copying in C language we have to first understand how C stores data first.
A deep copy means the data saved to a variable is independent of the original data.
A shallow copy means the data is still in someway connected to the original variable.
What is a deep copy? Deep copy refers to copying the specific content of the object. Deep copy opens up a new memory address in the computer to store the copied object. Changes to the source data do not affect the replicated data.
Why use deep copy? We hope that when changing the new array (object), the original array (object) will not be changed.
Code Explained: In order to avoid for the structure containing pointer variables, and the pointer variable members in the structure point to a section of dynamically allocated memory space, we can use deep copy in the process of copying each other.We copy std1 to std2, the member name of std2 points to a new space again, and assigns the content of the space pointed to by std1.name to the space pointed to by std2.name. The "copy" of the structure is successful.
What is a shallow copy?
Code Explained: First, we define a structure Student, and declare two structure variables t1 and t2 in the main function. Next, we open up a space in the heap area for the name of t1, and use the strcpy function to copy to the space area pointed to by name. Finally we use t1 to assign directly to t2. The member names of t1 and t2 point to the same memory area in our computer.
With this article at OpenGenus, you must have the complete idea of how to copy struct in C.
Copying is to reuse some or all of the properties of the original object and create a new object based on the meta object.
When copying, all properties in an object are copied, including Object and array properties. This copy is called deep copy.
When copying, only part of the properties are copied, and Object and array properties are not copied, this kind of copy is called shallow copy.
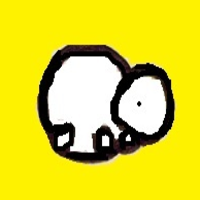
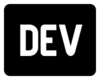
DEV Community

Posted on Jul 12, 2022
Ways to copy struct in c/c++
Today I am gonna show some of the ways to copy struct in c/c++ .
In this approach we will copy attribute by attribute of struct into another struct to make a copy. The rest code is self explainable.
Disadvantage in this approach
Although It is very clear that this code is written for copying the struct. But as the size of struct increase It becomes more difficult to type all the attributes and So the chance of typo also increases.
So what's the solution for this. Let's discuss our next approach.
Instead of copying attribute by attributes We can also copy whole struct using memcpy and rest code will be same. Just copy whole struct in one line only.
We can also use assignment operator to make copy of struct.
A lot of people don't even realize that they can copy a struct this way because one can't do same it with an array. Similarly one can return struct from a function and assign it but not array. The compiler do all the copying stuff behind the scene for us.
NOTE : When dealing with struct pointers approach 3 doesn't work as assigning one pointer to another doesn't make copy instead just point to new memory address. So if we modify new variable, original one is also affected.
❤️Thank you so much for reading it completely. If you find any mistake please let me know in comments.
Also consider sharing and giving a thumbs up If this post help you in any way.
Top comments (0)
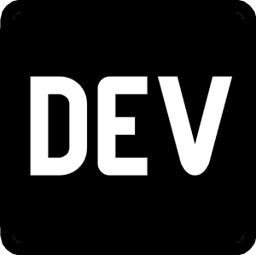
Templates let you quickly answer FAQs or store snippets for re-use.
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
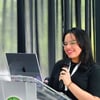
Supabase Auth now supports Anonymous Sign-ins
Yuri - Apr 17
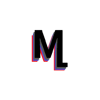
GEMINI 💎 VS CHATGPT ⚔️
Mince - Apr 23

Difference between var and let in Javascript
Amal Satheesan - Apr 10
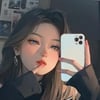
Insertion Sort in Java (With Intuition + Dry run + Code)
Alysa - Apr 23
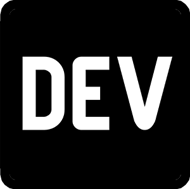
We're a place where coders share, stay up-to-date and grow their careers.
Previous: Temporaries May Vanish Before You Expect , Up: Common Misunderstandings with GNU C++ [ Contents ][ Index ]
14.7.4 Implicit Copy-Assignment for Virtual Bases ¶
When a base class is virtual, only one subobject of the base class belongs to each full object. Also, the constructors and destructors are invoked only once, and called from the most-derived class. However, such objects behave unspecified when being assigned. For example:
The C++ standard specifies that ‘ Base::Base ’ is only called once when constructing or copy-constructing a Derived object. It is unspecified whether ‘ Base::operator= ’ is called more than once when the implicit copy-assignment for Derived objects is invoked (as it is inside ‘ func ’ in the example).
G++ implements the “intuitive” algorithm for copy-assignment: assign all direct bases, then assign all members. In that algorithm, the virtual base subobject can be encountered more than once. In the example, copying proceeds in the following order: ‘ name ’ (via strdup ), ‘ val ’, ‘ name ’ again, and ‘ bval ’.
If application code relies on copy-assignment, a user-defined copy-assignment operator removes any uncertainties. With such an operator, the application can define whether and how the virtual base subobject is assigned.
C Functions
C structures, c structures (structs).
Structures (also called structs) are a way to group several related variables into one place. Each variable in the structure is known as a member of the structure.
Unlike an array , a structure can contain many different data types (int, float, char, etc.).
Create a Structure
You can create a structure by using the struct keyword and declare each of its members inside curly braces:
To access the structure, you must create a variable of it.
Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable:
Create a struct variable with the name "s1":
Access Structure Members
To access members of a structure, use the dot syntax ( . ):
Now you can easily create multiple structure variables with different values, using just one structure:
What About Strings in Structures?
Remember that strings in C are actually an array of characters, and unfortunately, you can't assign a value to an array like this:
An error will occur:
However, there is a solution for this! You can use the strcpy() function and assign the value to s1.myString , like this:
Simpler Syntax
You can also assign values to members of a structure variable at declaration time, in a single line.
Just insert the values in a comma-separated list inside curly braces {} . Note that you don't have to use the strcpy() function for string values with this technique:
Note: The order of the inserted values must match the order of the variable types declared in the structure (13 for int, 'B' for char, etc).
Copy Structures
You can also assign one structure to another.
In the following example, the values of s1 are copied to s2:
Modify Values
If you want to change/modify a value, you can use the dot syntax ( . ).
And to modify a string value, the strcpy() function is useful again:
Modifying values are especially useful when you copy structure values:
Ok, so, how are structures useful?
Imagine you have to write a program to store different information about Cars, such as brand, model, and year. What's great about structures is that you can create a single "Car template" and use it for every cars you make. See below for a real life example.
Real-Life Example
Use a structure to store different information about Cars:
C Exercises
Test yourself with exercises.
Fill in the missing part to create a Car structure:
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Copy assignment operator
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default .
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The implicitly-declared copy assignment operator for class T is trivial if all of the following is true:
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std:: memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std:: memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std:: move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
Learn C practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn c interactively, introduction.
- Getting Started with C
- Your First C Program
- C Variables, Constants and Literals
- C Data Types
- C Input Output (I/O)
- C Programming Operators
Flow Control
- C if...else Statement
- C while and do...while Loop
- C break and continue
- C switch Statement
- C goto Statement
- C Functions
- C User-defined functions
- Types of User-defined Functions in C Programming
- C Recursion
- C Storage Class
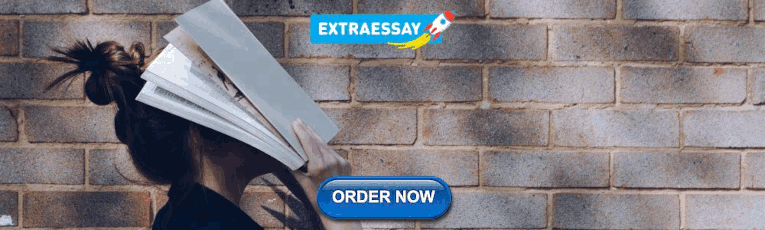
Programming Arrays
- C Multidimensional Arrays
- Pass arrays to a function in C
Programming Pointers
- Relationship Between Arrays and Pointers
- C Pass Addresses and Pointers
- C Dynamic Memory Allocation
- C Array and Pointer Examples
Programming Strings
- C Programming Strings
- String Manipulations In C Programming Using Library Functions
- String Examples in C Programming
Structure and Union
C structs and Pointers
C Structure and Function
Programmimg Files
- C File Handling
- C Files Examples
Additional Topics
- C Keywords and Identifiers
- C Precedence And Associativity Of Operators
- C Bitwise Operators
- C Preprocessor and Macros
- C Standard Library Functions
C Struct Examples
- Add Two Complex Numbers by Passing Structure to a Function
- Add Two Distances (in inch-feet system) using Structures
In C programming, a struct (or structure) is a collection of variables (can be of different types) under a single name.
- Define Structures
Before you can create structure variables, you need to define its data type. To define a struct, the struct keyword is used.
Syntax of struct
For example,
Here, a derived type struct Person is defined. Now, you can create variables of this type.
Create struct Variables
When a struct type is declared, no storage or memory is allocated. To allocate memory of a given structure type and work with it, we need to create variables.
Here's how we create structure variables:
Another way of creating a struct variable is:
In both cases,
- person1 and person2 are struct Person variables
- p[] is a struct Person array of size 20.
Access Members of a Structure
There are two types of operators used for accessing members of a structure.
- . - Member operator
- -> - Structure pointer operator (will be discussed in the next tutorial)
Suppose, you want to access the salary of person2 . Here's how you can do it.
Example 1: C structs
In this program, we have created a struct named Person . We have also created a variable of Person named person1 .
In main() , we have assigned values to the variables defined in Person for the person1 object.
Notice that we have used strcpy() function to assign the value to person1.name .
This is because name is a char array ( C-string ) and we cannot use the assignment operator = with it after we have declared the string.
Finally, we printed the data of person1 .
- Keyword typedef
We use the typedef keyword to create an alias name for data types. It is commonly used with structures to simplify the syntax of declaring variables.
For example, let us look at the following code:
We can use typedef to write an equivalent code with a simplified syntax:
Example 2: C typedef
Here, we have used typedef with the Person structure to create an alias person .
Now, we can simply declare a Person variable using the person alias:
- Nested Structures
You can create structures within a structure in C programming. For example,
Suppose, you want to set imag of num2 variable to 11 . Here's how you can do it:
Example 3: C Nested Structures
Why structs in c.
Suppose you want to store information about a person: his/her name, citizenship number, and salary. You can create different variables name , citNo and salary to store this information.
What if you need to store information of more than one person? Now, you need to create different variables for each information per person: name1 , citNo1 , salary1 , name2 , citNo2 , salary2 , etc.
A better approach would be to have a collection of all related information under a single name Person structure and use it for every person.
More on struct
- Structures and pointers
- Passing structures to a function
Table of Contents
- C struct (Introduction)
- Create struct variables
- Access members of a structure
- Example 1: C++ structs
Sorry about that.
Related Tutorials
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Copy constructors and copy assignment operators (C++)
- 8 contributors
Starting in C++11, two kinds of assignment are supported in the language: copy assignment and move assignment . In this article "assignment" means copy assignment unless explicitly stated otherwise. For information about move assignment, see Move Constructors and Move Assignment Operators (C++) .
Both the assignment operation and the initialization operation cause objects to be copied.
Assignment : When one object's value is assigned to another object, the first object is copied to the second object. So, this code copies the value of b into a :
Initialization : Initialization occurs when you declare a new object, when you pass function arguments by value, or when you return by value from a function.
You can define the semantics of "copy" for objects of class type. For example, consider this code:
The preceding code could mean "copy the contents of FILE1.DAT to FILE2.DAT" or it could mean "ignore FILE2.DAT and make b a second handle to FILE1.DAT." You must attach appropriate copying semantics to each class, as follows:
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x); .
Use the copy constructor.
If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you. Similarly, if you don't declare a copy assignment operator, the compiler generates a member-wise copy assignment operator for you. Declaring a copy constructor doesn't suppress the compiler-generated copy assignment operator, and vice-versa. If you implement either one, we recommend that you implement the other one, too. When you implement both, the meaning of the code is clear.
The copy constructor takes an argument of type ClassName& , where ClassName is the name of the class. For example:
Make the type of the copy constructor's argument const ClassName& whenever possible. This prevents the copy constructor from accidentally changing the copied object. It also lets you copy from const objects.
Compiler generated copy constructors
Compiler-generated copy constructors, like user-defined copy constructors, have a single argument of type "reference to class-name ." An exception is when all base classes and member classes have copy constructors declared as taking a single argument of type const class-name & . In such a case, the compiler-generated copy constructor's argument is also const .
When the argument type to the copy constructor isn't const , initialization by copying a const object generates an error. The reverse isn't true: If the argument is const , you can initialize by copying an object that's not const .
Compiler-generated assignment operators follow the same pattern for const . They take a single argument of type ClassName& unless the assignment operators in all base and member classes take arguments of type const ClassName& . In this case, the generated assignment operator for the class takes a const argument.
When virtual base classes are initialized by copy constructors, whether compiler-generated or user-defined, they're initialized only once: at the point when they are constructed.
The implications are similar to the copy constructor. When the argument type isn't const , assignment from a const object generates an error. The reverse isn't true: If a const value is assigned to a value that's not const , the assignment succeeds.
For more information about overloaded assignment operators, see Assignment .
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
cppreference.com
Copy constructors.
A copy constructor is a constructor which can be called with an argument of the same class type and copies the content of the argument without mutating the argument.
[ edit ] Syntax
[ edit ] explanation.
The copy constructor is called whenever an object is initialized (by direct-initialization or copy-initialization ) from another object of the same type (unless overload resolution selects a better match or the call is elided ), which includes
- initialization: T a = b ; or T a ( b ) ; , where b is of type T ;
- function argument passing: f ( a ) ; , where a is of type T and f is void f ( T t ) ;
- function return: return a ; inside a function such as T f ( ) , where a is of type T , which has no move constructor .
[ edit ] Implicitly-declared copy constructor
If no user-defined copy constructors are provided for a class type, the compiler will always declare a copy constructor as a non- explicit inline public member of its class. This implicitly-declared copy constructor has the form T :: T ( const T & ) if all of the following are true:
- each direct and virtual base B of T has a copy constructor whose parameters are of type const B & or const volatile B & ;
- each non-static data member M of T of class type or array of class type has a copy constructor whose parameters are of type const M & or const volatile M & .
Otherwise, the implicitly-declared copy constructor is T :: T ( T & ) .
Due to these rules, the implicitly-declared copy constructor cannot bind to a volatile lvalue argument.
A class can have multiple copy constructors, e.g. both T :: T ( const T & ) and T :: T ( T & ) .
The implicitly-declared (or defaulted on its first declaration) copy constructor has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17) .
[ edit ] Implicitly-defined copy constructor
If the implicitly-declared copy constructor is not deleted, it is defined (that is, a function body is generated and compiled) by the compiler if odr-used or needed for constant evaluation (since C++11) . For union types, the implicitly-defined copy constructor copies the object representation (as by std::memmove ). For non-union class types, the constructor performs full member-wise copy of the object's direct bases and non-static data members, in their initialization order, using direct initialization.
[ edit ] Deleted copy constructor
The implicitly-declared or explicitly-defaulted (since C++11) copy constructor for class T is undefined (until C++11) defined as deleted (since C++11) if any of the following conditions is satisfied:
- T has a potentially constructed subobject of class type M (or possibly multi-dimensional array thereof) such that
- M has a destructor that is deleted or (since C++11) inaccessible from the copy constructor, or
- the overload resolution as applied to find M 's copy constructor
- does not result in a usable candidate, or
- in the case of the subobject being a variant member , selects a non-trivial function.
[ edit ] Trivial copy constructor
The copy constructor for class T is trivial if all of the following are true:
- it is not user-provided (that is, it is implicitly-defined or defaulted);
- T has no virtual member functions;
- T has no virtual base classes;
- the copy constructor selected for every direct base of T is trivial;
- the copy constructor selected for every non-static class type (or array of class type) member of T is trivial;
A trivial copy constructor for a non-union class effectively copies every scalar subobject (including, recursively, subobject of subobjects and so forth) of the argument and performs no other action. However, padding bytes need not be copied, and even the object representations of the copied subobjects need not be the same as long as their values are identical.
TriviallyCopyable objects can be copied by copying their object representations manually, e.g. with std::memmove . All data types compatible with the C language (POD types) are trivially copyable.
[ edit ] Eligible copy constructor
Triviality of eligible copy constructors determines whether the class is an implicit-lifetime type , and whether the class is a trivially copyable type .
[ edit ] Notes
In many situations, copy constructors are optimized out even if they would produce observable side-effects, see copy elision .
[ edit ] Example
[ edit ] defect reports.
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
- converting constructor
- copy assignment
- copy elision
- default constructor
- aggregate initialization
- constant initialization
- copy initialization
- default initialization
- direct initialization
- initializer list
- list initialization
- reference initialization
- value initialization
- zero initialization
- move assignment
- move constructor
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 2 February 2024, at 09:23.
- This page has been accessed 1,248,882 times.
- Privacy policy
- About cppreference.com
- Disclaimers

Copy assignment operator
A copy assignment operator of class T is a non-template non-static member function with the name operator = that takes exactly one parameter of type T , T & , const T & , volatile T & , or const volatile T & . A type with a public copy assignment operator is CopyAssignable .
[ edit ] Syntax
[ edit ] explanation.
- Typical declaration of a copy assignment operator when copy-and-swap idiom can be used
- Typical declaration of a copy assignment operator when copy-and-swap idiom cannot be used
- Forcing a copy assignment operator to be generated by the compiler
- Avoiding implicit copy assignment
The copy assignment operator is called whenever selected by overload resolution , e.g. when an object appears on the left side of an assignment expression.
[ edit ] Implicitly-declared copy assignment operator
If no user-defined copy assignment operators are provided for a class type ( struct , class , or union ), the compiler will always declare one as an inline public member of the class. This implicitly-declared copy assignment operator has the form T & T :: operator = ( const T & ) if all of the following is true:
- each direct base B of T has a copy assignment operator whose parameters are B or const B& or const volatile B &
- each non-static data member M of T of class type or array of class type has a copy assignment operator whose parameters are M or const M& or const volatile M &
Otherwise the implicitly-declared copy assignment operator is declared as T & T :: operator = ( T & ) . (Note that due to these rules, the implicitly-declared copy assignment operator cannot bind to a volatile lvalue argument)
A class can have multiple copy assignment operators, e.g. both T & T :: operator = ( const T & ) and T & T :: operator = ( T ) . If some user-defined copy assignment operators are present, the user may still force the generation of the implicitly declared copy assignment operator with the keyword default . (since C++11)
Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. If a using-declaration is used to bring in the assignment operator from the base class, and its argument type could be the same as the argument type of the implicit assignment operator of the derived class, the using-declaration is also hidden by the implicit declaration.
[ edit ] Deleted implicitly-declared copy assignment operator
The implicitly-declared or defaulted copy assignment operator for class T is defined as deleted in any of the following is true:
- T has a non-static data member that is const
- T has a non-static data member of a reference type.
- T has a non-static data member that cannot be copy-assigned (has deleted, inaccessible, or ambiguous copy assignment operator)
- T has direct or virtual base class that cannot be copy-assigned (has deleted, inaccessible, or ambiguous move assignment operator)
- T has a user-declared move constructor
- T has a user-declared move assignment operator
[ edit ] Trivial copy assignment operator
The copy assignment operator for class T is trivial if all of the following is true:
- The operator is not user-provided (meaning, it is implicitly-defined or defaulted), and if it is defaulted, its signature is the same as implicitly-defined
- T has no virtual member functions
- T has no virtual base classes
- The copy assignment operator selected for every direct base of T is trivial
- The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial
A trivial copy assignment operator makes a copy of the object representation as if by std::memmove . All data types compatible with the C language (POD types) are trivially copy-assignable.
[ edit ] Implicitly-defined copy assignment operator
If the implicitly-declared copy assignment operator is not deleted or trivial, it is defined (that is, a function body is generated and compiled) by the compiler. For union types, the implicitly-defined copy assignment copies the object representation (as by std::memmove ). For non-union class types ( class and struct ), the operator performs member-wise copy assignment of the object's bases and non-static members, in their initialization order, using, using built-in assignment for the scalars and copy assignment operator for class types.
The generation of the implicitly-defined copy assignment operator is deprecated (since C++11) if T has a user-declared destructor or user-declared copy constructor.
[ edit ] Notes
If both copy and move assignment operators are provided, overload resolution selects the move assignment if the argument is an rvalue (either prvalue such as a nameless temporary or xvalue such as the result of std::move ), and selects the copy assignment if the argument is lvalue (named object or a function/operator returning lvalue reference). If only the copy assignment is provided, all argument categories select it (as long as it takes its argument by value or as reference to const, since rvalues can bind to const references), which makes copy assignment the fallback for move assignment, when move is unavailable.
[ edit ] Copy and swap
Copy assignment operator can be expressed in terms of copy constructor, destructor, and the swap() member function, if one is provided:
T & T :: operator = ( T arg ) { // copy/move constructor is called to construct arg swap ( arg ) ; // resources exchanged between *this and arg return * this ; } // destructor is called to release the resources formerly held by *this
For non-throwing swap(), this form provides strong exception guarantee . For rvalue arguments, this form automatically invokes the move constructor, and is sometimes referred to as "unifying assignment operator" (as in, both copy and move).
[ edit ] Example
Next: Unions , Previous: Overlaying Different Structures , Up: Structures [ Contents ][ Index ]
15.13 Structure Assignment
Assignment operating on a structure type copies the structure. The left and right operands must have the same type. Here is an example:
Notionally, assignment on a structure type works by copying each of the fields. Thus, if any of the fields has the const qualifier, that structure type does not allow assignment:
See Assignment Expressions .
When a structure type has a field which is an array, as here,
structure assigment such as r1 = r2 copies array fields’ contents just as it copies all the other fields.
This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct . You can’t copy the contents of the data field as an array, because
would convert the array objects (as always) to pointers to the zeroth elements of the arrays (of type struct record * ), and the assignment would be invalid because the left operand is not an lvalue.
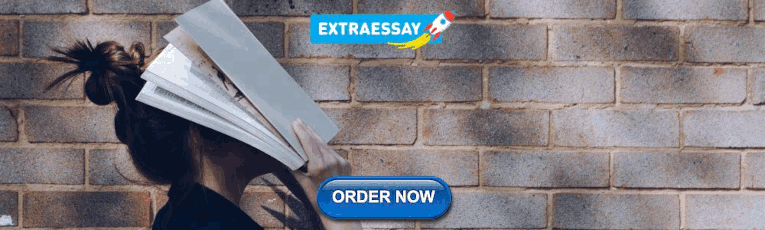
IMAGES
VIDEO
COMMENTS
4. Yes, you can assign one instance of a struct to another using a simple assignment statement. In the case of non-pointer or non pointer containing struct members, assignment means copy. In the case of pointer struct members, assignment means pointer will point to the same address of the other pointer.
the copy assignment operator selected for every non-static class type (or array of class type) member of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
structure assigment such as r1 = r2 copies array fields' contents just as it copies all the other fields. This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct. You can't copy the contents of the data field as an array, because.
With this article at OpenGenus, you must have the complete idea of how to copy struct in C. Copying is to reuse some or all of the properties of the original object and create a new object based on the meta object. When copying, all properties in an object are copied, including Object and array properties. This copy is called deep copy.
Today I am gonna show some of the ways to copy struct in c/c++. Approach 1 In this approach we will copy attribute by attribute of struct into another struct to make a copy. The rest code is self explainable. ... We can also use assignment operator to make copy of struct. Code. int main () ...
The C++ standard specifies that 'Base::Base' is only called once when constructing or copy-constructing a Derived object.It is unspecified whether 'Base::operator=' is called more than once when the implicit copy-assignment for Derived objects is invoked (as it is inside 'func' in the example). G++ implements the "intuitive" algorithm for copy-assignment: assign all direct ...
You can create a structure by using the struct keyword and declare each of its members inside curly braces: To access the structure, you must create a variable of it. Use the struct keyword inside the main() method, followed by the name of the structure and then the name of the structure variable: Create a struct variable with the name "s1":
193. For simple structures you can either use memcpy like you do, or just assign from one to the other: RTCclk = RTCclkBuffert; The compiler will create code to copy the structure for you. An important note about the copying: It's a shallow copy, just like with memcpy.
struct Copy Operation. In C, variables are copied in three situations: - when used as the right side of an assignment operation. - when used as a parameter in a function call. - when used as the return value from a function. In most cases, the default copy mechanism for struct types is adequate. struct LocationType { int X, Y; }; typedef struct ...
The copy assignment operator selected for every non-static class type (or array of class type) memeber of T is trivial. A trivial copy assignment operator makes a copy of the object representation as if by std::memmove. All data types compatible with the C language (POD types) are trivially copy-assignable.
Example 1: C structs ... This is because name is a char array and we cannot use the assignment operator = with it after we have declared the string. Finally, we printed the data of person1. Keyword typedef. We use the typedef keyword to create an alias name for data types. It is commonly used with structures to simplify the syntax of declaring ...
C17 6.5.16: An assignment operator stores a value in the object designated by the left operand. An assignment expression has the value of the left operand after the assignment, but is not an lvalue. The type of an assignment expression is the type the left operand would have after lvalue conversion. (Lvalue conversion in this case isn't ...
Use an assignment operator operator= that returns a reference to the class type and takes one parameter that's passed by const reference—for example ClassName& operator=(const ClassName& x);. Use the copy constructor. If you don't declare a copy constructor, the compiler generates a member-wise copy constructor for you.
The implicitly-declared (or defaulted on its first declaration) copy constructor has an exception specification as described in dynamic exception specification (until C++17) noexcept specification (since C++17). [] Implicitly-defined copy constructoIf the implicitly-declared copy constructor is not deleted, it is defined (that is, a function body is generated and compiled) by the compiler if ...
If no user-defined copy assignment operators are provided for a class type (struct, class, or union), the compiler will always declare one as an inline public member of the class. ... (since C++11) Because the copy assignment operator is always declared for any class, the base class assignment operator is always hidden. ...
Just be careful of memory allocation inside the struct. For example if you have a struct that contains a pointer to a string and you allocate memory for the string. That memory does not get copied. the pointer to the memory gets copied, but not the memory itself. In other word this type of assignment is not a deep copy.
In C++, the only difference between a class and a struct is that members of structs by default are public and members in classes by default are private. In C#, classes and structs are fundamentally different. Classes have reference semantics and structs have value semantics. As VersionSchemeState is a struct, result = state makes a copy (shallow).
structure assigment such as r1 = r2 copies array fields' contents just as it copies all the other fields.. This is the only way in C that you can operate on the whole contents of a array with one operation: when the array is contained in a struct.You can't copy the contents of the data field as an array, because
Classes and Objects are part of object-oriented methods and typically provide features such as properties and methods. One of the great features of an object orientated language like C++ is a copy assignment operator that is used with operator= to create a new object from an existing one. In this post, we explain what a copy assignment operator is and its types in usage with some C++ examples.
4. std::strings by themselves can be copied without any problems. When you define a class (or struct), C++ will generate a number of methods for you by default, including a copy constructor and an assignment operator. I believe that the generated copy constructor will call the copy constructor on each of fields, and the generated assignment ...