- Trending Now
- Foundational Courses
- Data Science
- Practice Problem
- Machine Learning
- System Design
- DevOps Tutorial
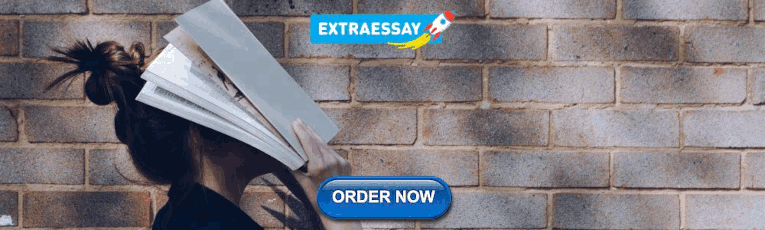
Assignment Operators in Programming
- Binary Operators in Programming
- Operator Associativity in Programming
- C++ Assignment Operator Overloading
- What are Operators in Programming?
- Assignment Operators In C++
- Bitwise AND operator in Programming
- Increment and Decrement Operators in Programming
- Types of Operators in Programming
- Logical AND operator in Programming
- Modulus Operator in Programming
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- Pre Increment and Post Increment Operator in Programming
- Right Shift Operator (>>) in Programming
- JavaScript Assignment Operators
- Move Assignment Operator in C++ 11
- Assignment Operators in Python
- Assignment Operators in C
- Subtraction Assignment( -=) Operator in Javascript
Assignment operators in programming are symbols used to assign values to variables. They offer shorthand notations for performing arithmetic operations and updating variable values in a single step. These operators are fundamental in most programming languages and help streamline code while improving readability.
Table of Content
What are Assignment Operators?
- Types of Assignment Operators
- Assignment Operators in C++
- Assignment Operators in Java
- Assignment Operators in C#
- Assignment Operators in Javascript
- Application of Assignment Operators
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign ( = ), which assigns the value on the right side of the operator to the variable on the left side.
Types of Assignment Operators:
- Simple Assignment Operator ( = )
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
Below is a table summarizing common assignment operators along with their symbols, description, and examples:
Assignment Operators in C:
Here are the implementation of Assignment Operator in C language:
Assignment Operators in C++:
Here are the implementation of Assignment Operator in C++ language:
Assignment Operators in Java:
Here are the implementation of Assignment Operator in java language:
Assignment Operators in Python:
Here are the implementation of Assignment Operator in python language:
Assignment Operators in C#:
Here are the implementation of Assignment Operator in C# language:
Assignment Operators in Javascript:
Here are the implementation of Assignment Operator in javascript language:
Application of Assignment Operators:
- Variable Initialization : Setting initial values to variables during declaration.
- Mathematical Operations : Combining arithmetic operations with assignment to update variable values.
- Loop Control : Updating loop variables to control loop iterations.
- Conditional Statements : Assigning different values based on conditions in conditional statements.
- Function Return Values : Storing the return values of functions in variables.
- Data Manipulation : Assigning values received from user input or retrieved from databases to variables.
Conclusion:
In conclusion, assignment operators in programming are essential tools for assigning values to variables and performing operations in a concise and efficient manner. They allow programmers to manipulate data and control the flow of their programs effectively. Understanding and using assignment operators correctly is fundamental to writing clear, efficient, and maintainable code in various programming languages.
Please Login to comment...
Similar reads.
- Programming
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
cppreference.com
Assignment operators.
Assignment and compound assignment operators are binary operators that modify the variable to their left using the value to their right.
[ edit ] Simple assignment
The simple assignment operator expressions have the form
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs .
Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non-lvalue (so that expressions such as ( a = b ) = c are invalid).
rhs and lhs must satisfy one of the following:
- both lhs and rhs have compatible struct or union type, or..
- rhs must be implicitly convertible to lhs , which implies
- both lhs and rhs have arithmetic types , in which case lhs may be volatile -qualified or atomic (since C11)
- both lhs and rhs have pointer to compatible (ignoring qualifiers) types, or one of the pointers is a pointer to void, and the conversion would not add qualifiers to the pointed-to type. lhs may be volatile or restrict (since C99) -qualified or atomic (since C11) .
- lhs is a (possibly qualified or atomic (since C11) ) pointer and rhs is a null pointer constant such as NULL or a nullptr_t value (since C23)
[ edit ] Notes
If rhs and lhs overlap in memory (e.g. they are members of the same union), the behavior is undefined unless the overlap is exact and the types are compatible .
Although arrays are not assignable, an array wrapped in a struct is assignable to another object of the same (or compatible) struct type.
The side effect of updating lhs is sequenced after the value computations, but not the side effects of lhs and rhs themselves and the evaluations of the operands are, as usual, unsequenced relative to each other (so the expressions such as i = ++ i ; are undefined)
Assignment strips extra range and precision from floating-point expressions (see FLT_EVAL_METHOD ).
In C++, assignment operators are lvalue expressions, not so in C.
[ edit ] Compound assignment
The compound assignment operator expressions have the form
The expression lhs @= rhs is exactly the same as lhs = lhs @ ( rhs ) , except that lhs is evaluated only once.
[ edit ] References
- C17 standard (ISO/IEC 9899:2018):
- 6.5.16 Assignment operators (p: 72-73)
- C11 standard (ISO/IEC 9899:2011):
- 6.5.16 Assignment operators (p: 101-104)
- C99 standard (ISO/IEC 9899:1999):
- 6.5.16 Assignment operators (p: 91-93)
- C89/C90 standard (ISO/IEC 9899:1990):
- 3.3.16 Assignment operators
[ edit ] See Also
Operator precedence
[ edit ] See also
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 19 August 2022, at 09:36.
- This page has been accessed 58,085 times.
- Privacy policy
- About cppreference.com
- Disclaimers


- school Campus Bookshelves
- menu_book Bookshelves
- perm_media Learning Objects
- login Login
- how_to_reg Request Instructor Account
- hub Instructor Commons
Margin Size
- Download Page (PDF)
- Download Full Book (PDF)
- Periodic Table
- Physics Constants
- Scientific Calculator
- Reference & Cite
- Tools expand_more
- Readability
selected template will load here
This action is not available.
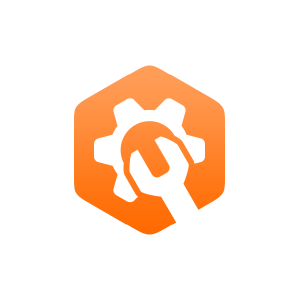
4.5: Assignment Operator
- Last updated
- Save as PDF
- Page ID 10258
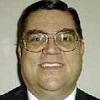
- Kenneth Leroy Busbee
- Houston Community College via OpenStax CNX
\( \newcommand{\vecs}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vecd}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash {#1}}} \)
\( \newcommand{\id}{\mathrm{id}}\) \( \newcommand{\Span}{\mathrm{span}}\)
( \newcommand{\kernel}{\mathrm{null}\,}\) \( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\) \( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\) \( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\inner}[2]{\langle #1, #2 \rangle}\)
\( \newcommand{\Span}{\mathrm{span}}\)
\( \newcommand{\id}{\mathrm{id}}\)
\( \newcommand{\kernel}{\mathrm{null}\,}\)
\( \newcommand{\range}{\mathrm{range}\,}\)
\( \newcommand{\RealPart}{\mathrm{Re}}\)
\( \newcommand{\ImaginaryPart}{\mathrm{Im}}\)
\( \newcommand{\Argument}{\mathrm{Arg}}\)
\( \newcommand{\norm}[1]{\| #1 \|}\)
\( \newcommand{\Span}{\mathrm{span}}\) \( \newcommand{\AA}{\unicode[.8,0]{x212B}}\)
\( \newcommand{\vectorA}[1]{\vec{#1}} % arrow\)
\( \newcommand{\vectorAt}[1]{\vec{\text{#1}}} % arrow\)
\( \newcommand{\vectorB}[1]{\overset { \scriptstyle \rightharpoonup} {\mathbf{#1}} } \)
\( \newcommand{\vectorC}[1]{\textbf{#1}} \)
\( \newcommand{\vectorD}[1]{\overrightarrow{#1}} \)
\( \newcommand{\vectorDt}[1]{\overrightarrow{\text{#1}}} \)
\( \newcommand{\vectE}[1]{\overset{-\!-\!\rightharpoonup}{\vphantom{a}\smash{\mathbf {#1}}}} \)
The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol. But bite your tongue, when you see the = symbol you need to start thinking: assignment. The assignment operator has two operands. The one to the left of the operator is usually an identifier name for a variable. The one to the right of the operator is a value.
The value 21 is moved to the memory location for the variable named: age. Another way to say it: age is assigned the value 21.
The item to the right of the assignment operator is an expression. The expression will be evaluated and the answer is 14. The value 14 would assigned to the variable named: total_cousins.
The expression to the right of the assignment operator contains some identifier names. The program would fetch the values stored in those variables; add them together and get a value of 44; then assign the 44 to the total_students variable.
Definitions

- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Pointer to Pointer
- C - Passing Pointers to Functions
- C - Return Pointer from Functions
- C - Function Pointers
- C - Pointer to an Array
- C - Pointers to Structures
- C - Chain of Pointers
- C - Pointer vs Array
- C - Character Pointers and Functions
- C - NULL Pointer
- C - void Pointer
- C - Dangling Pointers
- C - Dereference Pointer
- C - Near, Far and Huge Pointers
- C - Initialization of Pointer Arrays
- C - Pointers vs. Multi-dimensional Arrays
- C - Strings
- C - Array of Strings
- C - Special Characters
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Self-Referential Structures
- C - Lookup Tables
- C - Dot (.) Operator
- C - Enumeration (or enum)
- C - Nested Structures
- C - Structure Padding and Packing
- C - Anonymous Structure and Union
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
To Continue Learning Please Login
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
Assignment operators (C# reference)
- 11 contributors
The assignment operator = assigns the value of its right-hand operand to a variable, a property , or an indexer element given by its left-hand operand. The result of an assignment expression is the value assigned to the left-hand operand. The type of the right-hand operand must be the same as the type of the left-hand operand or implicitly convertible to it.
The assignment operator = is right-associative, that is, an expression of the form
is evaluated as
The following example demonstrates the usage of the assignment operator with a local variable, a property, and an indexer element as its left-hand operand:
The left-hand operand of an assignment receives the value of the right-hand operand. When the operands are of value types , assignment copies the contents of the right-hand operand. When the operands are of reference types , assignment copies the reference to the object.
This is called value assignment : the value is assigned.
ref assignment
Ref assignment = ref makes its left-hand operand an alias to the right-hand operand, as the following example demonstrates:
In the preceding example, the local reference variable arrayElement is initialized as an alias to the first array element. Then, it's ref reassigned to refer to the last array element. As it's an alias, when you update its value with an ordinary assignment operator = , the corresponding array element is also updated.
The left-hand operand of ref assignment can be a local reference variable , a ref field , and a ref , out , or in method parameter. Both operands must be of the same type.
Compound assignment
For a binary operator op , a compound assignment expression of the form
is equivalent to
except that x is only evaluated once.
Compound assignment is supported by arithmetic , Boolean logical , and bitwise logical and shift operators.
Null-coalescing assignment
You can use the null-coalescing assignment operator ??= to assign the value of its right-hand operand to its left-hand operand only if the left-hand operand evaluates to null . For more information, see the ?? and ??= operators article.
Operator overloadability
A user-defined type can't overload the assignment operator. However, a user-defined type can define an implicit conversion to another type. That way, the value of a user-defined type can be assigned to a variable, a property, or an indexer element of another type. For more information, see User-defined conversion operators .
A user-defined type can't explicitly overload a compound assignment operator. However, if a user-defined type overloads a binary operator op , the op= operator, if it exists, is also implicitly overloaded.
C# language specification
For more information, see the Assignment operators section of the C# language specification .
- C# operators and expressions
- ref keyword
- Use compound assignment (style rules IDE0054 and IDE0074)
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
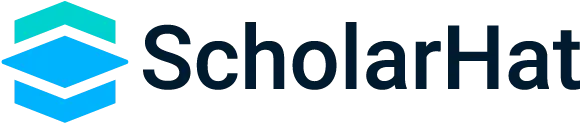
01 Career Opportunities
- Top 50 Mostly Asked C Interview Questions and Answers
02 Beginner
- If Statement in C
- Understanding do...while loop in C
- Understanding for loop in C
- if else if statements in C Programming
- If...else statement in C Programming
- Understanding realloc() function in C
- Understanding While loop in C
- Why C is called middle level language?
- Beginner's Guide to C Programming
- First C program and Its Syntax
- Escape Sequences and Comments in C
- Keywords in C: List of Keywords
- Identifiers in C: Types of Identifiers
- Data Types in C Programming - A Beginner Guide with examples
- Variables in C Programming - Types of Variables in C ( With Examples )
- 10 Reasons Why You Should Learn C
- Boolean and Static in C Programming With Examples ( Full Guide )
- Operators in C: Types of Operators
- Bitwise Operators in C: AND, OR, XOR, Shift & Complement
- Expressions in C Programming - Types of Expressions in C ( With Examples )
- Conditional Statements in C: if, if..else, Nested if
- Switch Statement in C: Syntax and Examples
- Ternary Operator in C: Ternary Operator vs. if...else Statement
- Loop in C with Examples: For, While, Do..While Loops
- Nested Loops in C - Types of Expressions in C ( With Examples )
- Infinite Loops in C: Types of Infinite Loops
- Jump Statements in C: break, continue, goto, return
- Continue Statement in C: What is Break & Continue Statement in C with Example
03 Intermediate
- Constants in C language
- Getting Started with Data Structures in C
- Functions in C Programming
- Call by Value and Call by Reference in C
- Recursion in C: Types, its Working and Examples
- Storage Classes in C: Auto, Extern, Static, Register
- Arrays in C Programming: Operations on Arrays
- Strings in C with Examples: String Functions
04 Advanced
- How to Dynamically Allocate Memory using calloc() in C?
- How to Dynamically Allocate Memory using malloc() in C?
- Pointers in C: Types of Pointers
- Multidimensional Arrays in C: 2D and 3D Arrays
- Dynamic Memory Allocation in C: Malloc(), Calloc(), Realloc(), Free()
05 Training Programs
- Java Programming Course
- C++ Programming Course
- C Programming Course
- Data Structures and Algorithms Training
- C Programming Assignment ..
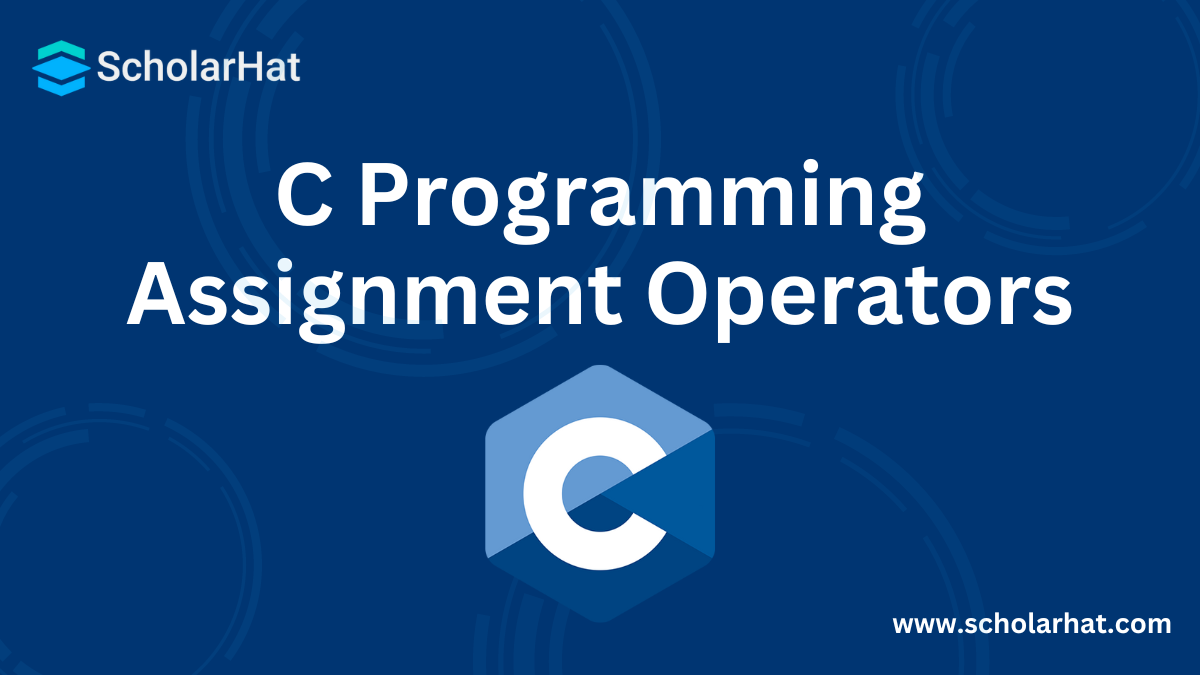
C Programming Assignment Operators
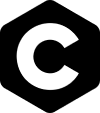
C Programming For Beginners Free Course
What is an assignment operator in c.
Assignment Operators in C are used to assign values to the variables. They come under the category of binary operators as they require two operands to operate upon. The left side operand is called a variable and the right side operand is the value. The value on the right side of the "=" is assigned to the variable on the left side of "=". The value on the right side must be of the same data type as the variable on the left side. Hence, the associativity is from right to left.
In this C tutorial , we'll understand the types of C programming assignment operators with examples. To delve deeper you can enroll in our C Programming Course .
Before going in-depth about assignment operators you must know about operators in C. If you haven't visited the Operators in C tutorial, refer to Operators in C: Types of Operators .
Types of Assignment Operators in C
There are two types of assignment operators in C:
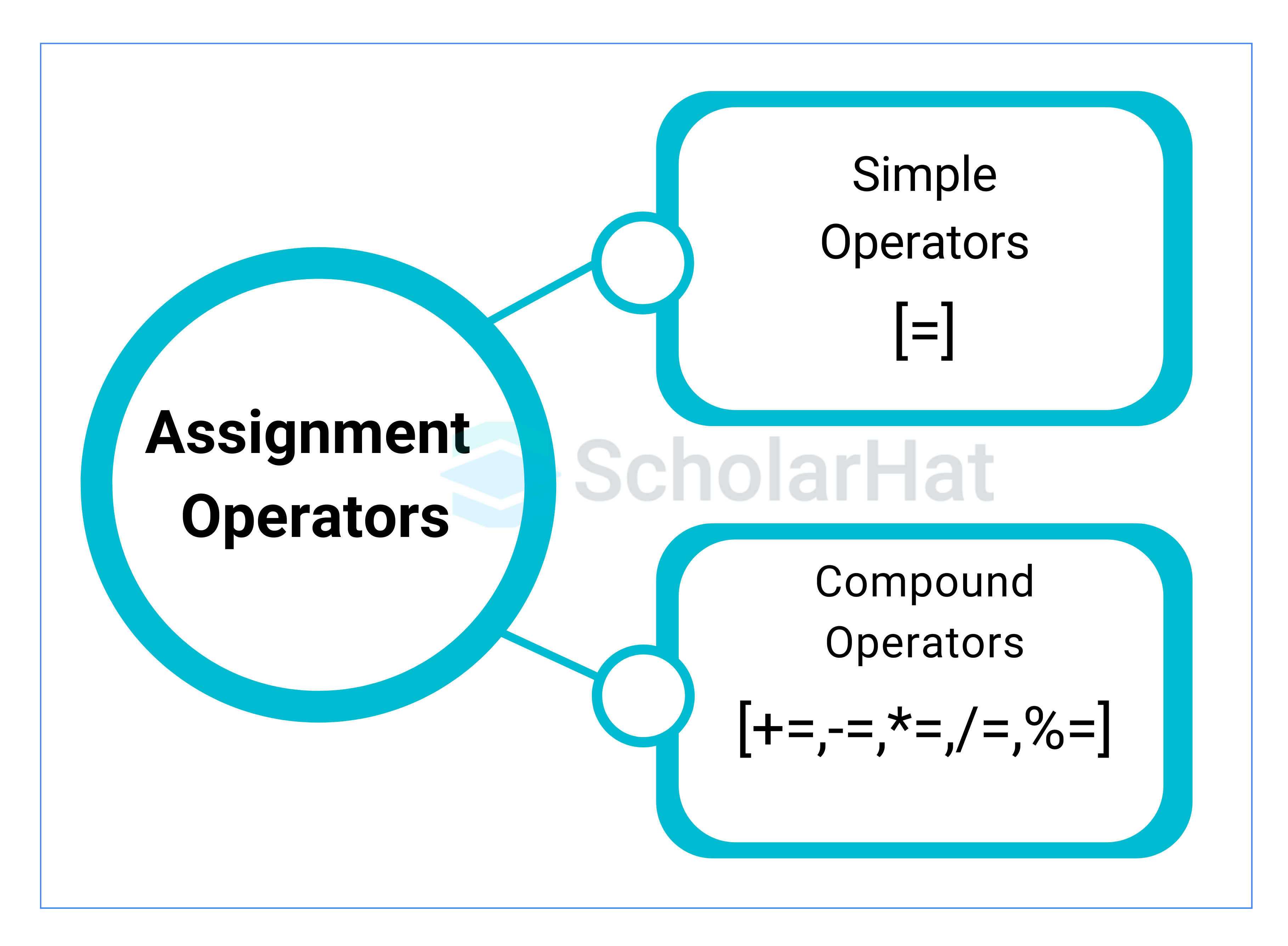
Example of Augmented Arithmetic and Assignment Operators
There can be five combinations of bitwise operators with the assignment operator, "=". Let's look at them one by one.
Example of Augmented Bitwise and Assignment Operators
Practice problems on assignment operators in c, 1. what will the value of "x" be after the execution of the following code.
The correct answer is 52. x starts at 50, increases by 5 to 55, then decreases by 3 to 52.
2. After executing the following code, what is the value of the number variable?
The correct answer is 144. After right-shifting 73 (binary 1001001) by one and then left-shifting the result by two, the value becomes 144 (binary 10010000).
Benefits of Using Assignment Operators
- Simplifies Code: For example, x += 1 is shorter and clearer than x = x + 1.
- Reduces Errors: They break complex expressions into simpler, more manageable parts thus reducing errors.
- Improves Readability: They make the code easier to read and understand by succinctly expressing common operations.
- Enhances Performance: They often operate in place, potentially reducing the need for additional memory or temporary variables.
Best Practices and Tips for Using the Assignment Operator
While performing arithmetic operations with the same variable, use compound assignment operators
- Initialize Variables When Declaring int count = 0 ; // Initialization
- Avoid Complex Expressions in Assignments a = (b + c) * (d - e); // Consider breaking it down: int temp = b + c; a = temp * (d - e);
- Avoid Multiple Assignments in a Single Statement // Instead of this a = b = c = 0 ; // Do this a = 0 ; b = 0 ; c = 0 ;
- Consistent Formatting int result = 0 ; result += 10 ;
When mixing assignments with other operations, use parentheses to ensure the correct order of evaluation.
Live Classes Schedule
Can't find convenient schedule? Let us know
About Author
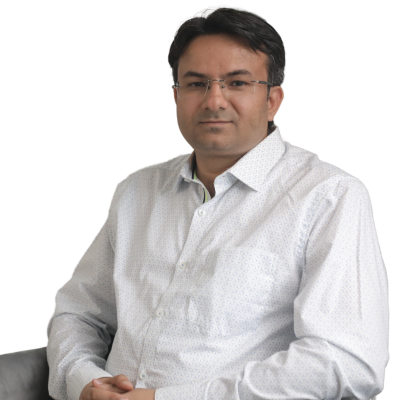
- 22+ Video Courses
- 800+ Hands-On Labs
- 400+ Quick Notes
- 55+ Skill Tests
- 45+ Interview Q&A Courses
- 10+ Real-world Projects
- Career Coaching Sessions
- Email Support
We use cookies to make interactions with our websites and services easy and meaningful. Please read our Privacy Policy for more details.
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
Assignment (=)
The assignment ( = ) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables.
A valid assignment target, including an identifier or a property accessor . It can also be a destructuring assignment pattern .
An expression specifying the value to be assigned to x .
Return value
The value of y .
Thrown in strict mode if assigning to an identifier that is not declared in the scope.
Thrown in strict mode if assigning to a property that is not modifiable .
Description
The assignment operator is completely different from the equals ( = ) sign used as syntactic separators in other locations, which include:
- Initializers of var , let , and const declarations
- Default values of destructuring
- Default parameters
- Initializers of class fields
All these places accept an assignment expression on the right-hand side of the = , so if you have multiple equals signs chained together:
This is equivalent to:
Which means y must be a pre-existing variable, and x is a newly declared const variable. y is assigned the value 5 , and x is initialized with the value of the y = 5 expression, which is also 5 . If y is not a pre-existing variable, a global variable y is implicitly created in non-strict mode , or a ReferenceError is thrown in strict mode. To declare two variables within the same declaration, use:
Simple assignment and chaining
Value of assignment expressions.
The assignment expression itself evaluates to the value of the right-hand side, so you can log the value and assign to a variable at the same time.
Unqualified identifier assignment
The global object sits at the top of the scope chain. When attempting to resolve a name to a value, the scope chain is searched. This means that properties on the global object are conveniently visible from every scope, without having to qualify the names with globalThis. or window. or global. .
Because the global object has a String property ( Object.hasOwn(globalThis, "String") ), you can use the following code:
So the global object will ultimately be searched for unqualified identifiers. You don't have to type globalThis.String ; you can just type the unqualified String . To make this feature more conceptually consistent, assignment to unqualified identifiers will assume you want to create a property with that name on the global object (with globalThis. omitted), if there is no variable of the same name declared in the scope chain.
In strict mode , assignment to an unqualified identifier in strict mode will result in a ReferenceError , to avoid the accidental creation of properties on the global object.
Note that the implication of the above is that, contrary to popular misinformation, JavaScript does not have implicit or undeclared variables. It just conflates the global object with the global scope and allows omitting the global object qualifier during property creation.
Assignment with destructuring
The left-hand side of can also be an assignment pattern. This allows assigning to multiple variables at once.
For more information, see Destructuring assignment .
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators in the JS guide
- Destructuring assignment
Python Operators Cheat Sheet

- python basics
- learn python
Discover the essential Python operators and how to effectively use them with our comprehensive cheat sheet. We cover everything from arithmetic to bitwise operations!
If you’ve ever written a few lines of Python code, you are likely familiar with Python operators. Whether you're doing basic arithmetic calculations, creating variables, or performing complex logical operations, chances are that you had to use a Python operator to perform the task. But just how many of them exist, and what do you use them for?
In this cheat sheet, we will cover every one of Python’s operators:
- Arithmetic operators.
- Assignment operators.
- Comparison operators.
- Logical operators.
- Identity operators.
- Membership operators.
- Bitwise operators.
Additionally, we will discuss operator precedence and its significance in Python.
If you're just starting out with Python programming, you may want to look into our Python Basics Track . Its nearly 40 hours of content covers Python operators and much more; you’ll get an excellent foundation to build your coding future on.
Without further ado, let's dive in and learn all about Python operators.
What Are Python Operators?
Python operators are special symbols or keywords used to perform specific operations. Depending on the operator, we can perform arithmetic calculations, assign values to variables, compare two or more values, use logical decision-making in our programs, and more.
How Operators Work
Operators are fundamental to Python programming (and programming as a whole); they allow us to manipulate data and control the flow of our code. Understanding how to use operators effectively enables programmers to write code that accomplishes a desired task.
In more specific terms, an operator takes two elements – called operands – and combines them in a given manner. The specific way that this combination happens is what defines the operator. For example, the operation A + B takes the operands A and B , performs the “sum” operation (denoted by the + operator), and returns the total of those two operands.
The Complete List of Python Operators
Now that we know the basic theory behind Python operators, it’s time to go over every single one of them.
In each section below, we will explain a family of operators, provide a few code samples on how they are used, and present a comprehensive table of all operators in that family. Let’s get started!
Python Arithmetic Operators
Arithmetic operators are used to perform mathematical calculations like addition, subtraction, multiplication, division, exponentiation, and modulus. Most arithmetic operators look the same as those used in everyday mathematics (or in spreadsheet formulas).
Here is the complete list of arithmetic operators in Python:
Most of these operators are self-explanatory, but a few are somewhat tricky. The floor division operator ( // ), for example, returns the integer portion of the division between two numbers.
The modulo operator ( % ) is also uncommon: it returns the remainder of an integer division, i.e. what remains when you divide a number by another. When dividing 11 by 4, the number 4 divides “perfectly” up to the value 8. This means that there’s a remainder of 3 left, which is the value returned by the modulo operator.
Also note that the addition ( + ) and subtraction ( - ) operators are special in that they can operate on a single operand; the expression +5 or -5 is considered an operation in itself. When used in this fashion, these operators are referred to as unary operators . The negative unary operator (as in -5 ) is used to invert the value of a number, while the positive unary operator (as in +5 ) was mostly created for symmetrical reasons, since writing +5 is effectively the same as just writing 5 .
Python Assignment Operators
Assignment operators are used to assign values to variables . They can also perform arithmetic operations in combination with assignments.
The canonical assignment operator is the equal sign ( = ). Its purpose is to bind a value to a variable: if we write x = 10 , we store the value 10 inside the variable x. We can then later refer to the variable x in order to retrieve its value.
The remaining assignment operators are collectively known as augmented assignment operators . They combine a regular assignment with an arithmetic operator in a single line. This is denoted by the arithmetic operator placed directly before the “vanilla” assignment operator.
Augmented assignment operators are simply used as a shortcut. Instead of writing x = x + 1 , they allow us to write x += 1 , effectively “updating” a variable in a concise manner. Here’s a code sample of how this works:
In the table below, you can find the complete list of assignment operators in Python. Note how there is an augmented assignment operator for every arithmetic operator we went over in the previous section:
Python Comparison Operators
Comparison operators are used to compare two values . They return a Boolean value ( True or False ) based on the comparison result.
These operators are often used in conjunction with if/else statements in order to control the flow of a program. For example, the code block below allows the user to select an option from a menu:
The table below shows the full list of Python comparison operators:
Note: Pay attention to the “equal to” operator ( == ) – it’s easy to mistake it for the assignment operator ( = ) when writing Python scripts!
If you’re coming from other programming languages, you may have heard about “ternary conditional operators”. Python has a very similar structure called conditional expressions, which you can learn more about in our article on ternary operators in Python . And if you want more details on this topic, be sure to check out our article on Python comparison operators .
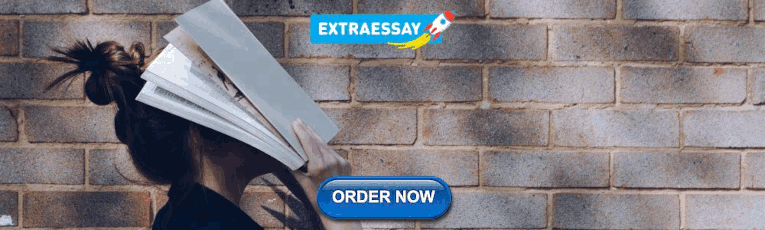
Python Logical Operators
Logical operators are used to combine and manipulate Boolean values . They return True or False based on the Boolean values given to them.
Logical operators are often used to combine different conditions into one. You can leverage the fact that they are written as normal English words to create code that is very readable. Even someone who isn’t familiar with Python could roughly understand what the code below attempts to do:
Here is the table with every logical operator in Python:
Note: When determining if a value falls inside a range of numbers, you can use the “interval notation” commonly used in mathematics; the expression x > 0 and x < 100 can be rewritten as 0 < x < 100 .
Python Identity Operators
Identity operators are used to query whether two Python objects are the exact same object . This is different from using the “equal to” operator ( == ) because two variables may be equal to each other , but at the same time not be the same object .
For example, the lists list_a and list_b below contain the same values, so for all practical purposes they are considered equal. But they are not the same object – modifying one list does not change the other:
The table below presents the two existing Python identity operators:
Python Membership Operators
Membership operators are used to test if a value is contained inside another object .
Objects that can contain other values in them are known as collections . Common collections in Python include lists, tuples, and sets.
Python Bitwise Operators
Bitwise operators in Python are the most esoteric of them all. They are used to perform bit-level operations on integers. Although you may not use these operators as often in your day to day coding, they are a staple in low-level programming languages like C.
As an example, let’s consider the numbers 5 (whose binary representation is 101 ), and the number 3 (represented as 011 in binary).
In order to apply the bitwise AND operator ( & ), we take the first digit from each number’s binary representation (in this case: 1 for the number 5, and 0 for the number 3). Then, we perform the AND operation, which works much like Python’s logical and operator except True is now 1 and False is 0 .
This gives us the operation 1 AND 0 , which results in 0 .
We then simply perform the same operation for each remaining pair of digits in the binary representation of 5 and 3, namely:
- 0 AND 1 = 0
- 1 AND 1 = 1
We end up with 0 , 0 , and 1 , which is then put back together as the binary number 001 – which is, in turn, how the number 1 is represented in binary. This all means that the bitwise operation 5 & 3 results in 1 . What a ride!
The name “bitwise” comes from the idea that these operations are performed on “bits” (the numbers 0 or 1), one pair at a time. Afterwards, they are all brought up together in a resulting binary value.
The table below presents all existing bitwise operations in Python:
Operator Precedence in Python
Operator precedence determines the order in which operators are evaluated in an expression. Operators with higher precedence are evaluated first.
For example, the fact that the exponentiation operator ( ** ) has a higher precedence than the addition operator ( + ) means that the expression 2 ** 3 + 4 is seen by Python as (2 ** 3) + 4 . The order of operation is exponentiation and then addition. To override operator precedence, you need to explicitly use parentheses to encapsulate a part of the expression, i.e. 2 ** (3 + 4) .
The table below illustrates the operator precedence in Python. Operators in the earlier rows have a higher precedence:
Want to Learn More About Python Operators?
In this article, we've covered every single Python operator. This includes arithmetic, assignment, comparison, logical, identity, membership, and bitwise operators. Understanding these operators is crucial for writing Python code effectively!
For those looking to dive deeper into Python, consider exploring our Learn Programming with Python track . It consists of five in-depth courses and over 400 exercises for you to master the language. You can also challenge yourself with our article on 10 Python practice exercises for beginners !
You may also like
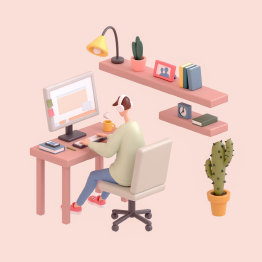
How Do You Write a SELECT Statement in SQL?
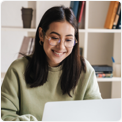
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
Python Operators
Python flow control.
Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers and Mathematics
- Python List
- Python Tuple
- Python String
- Python Sets
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
- Python datetime
- Python strftime()
- Python strptime()
- How to get current date and time in Python?
- Python Get Current Time
- Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python 3 Tutorial
- Python Strings
- Python any()
Operators are special symbols that perform operations on variables and values. For example,
Here, + is an operator that adds two numbers: 5 and 6 .
- Types of Python Operators
Here's a list of different types of Python operators that we will learn in this tutorial.
- Arithmetic Operators
- Assignment Operators
- Comparison Operators
- Logical Operators
- Bitwise Operators
- Special Operators
1. Python Arithmetic Operators
Arithmetic operators are used to perform mathematical operations like addition, subtraction, multiplication, etc. For example,
Here, - is an arithmetic operator that subtracts two values or variables.
Example 1: Arithmetic Operators in Python
In the above example, we have used multiple arithmetic operators,
- + to add a and b
- - to subtract b from a
- * to multiply a and b
- / to divide a by b
- // to floor divide a by b
- % to get the remainder
- ** to get a to the power b
2. Python Assignment Operators
Assignment operators are used to assign values to variables. For example,
Here, = is an assignment operator that assigns 5 to x .
Here's a list of different assignment operators available in Python.
Example 2: Assignment Operators
Here, we have used the += operator to assign the sum of a and b to a .
Similarly, we can use any other assignment operators as per our needs.
3. Python Comparison Operators
Comparison operators compare two values/variables and return a boolean result: True or False . For example,
Here, the > comparison operator is used to compare whether a is greater than b or not.
Example 3: Comparison Operators
Note: Comparison operators are used in decision-making and loops . We'll discuss more of the comparison operator and decision-making in later tutorials.
4. Python Logical Operators
Logical operators are used to check whether an expression is True or False . They are used in decision-making. For example,
Here, and is the logical operator AND . Since both a > 2 and b >= 6 are True , the result is True .
Example 4: Logical Operators
Note : Here is the truth table for these logical operators.
5. Python Bitwise operators
Bitwise operators act on operands as if they were strings of binary digits. They operate bit by bit, hence the name.
For example, 2 is 10 in binary, and 7 is 111 .
In the table below: Let x = 10 ( 0000 1010 in binary) and y = 4 ( 0000 0100 in binary)
6. Python Special operators
Python language offers some special types of operators like the identity operator and the membership operator. They are described below with examples.
- Identity operators
In Python, is and is not are used to check if two values are located at the same memory location.
It's important to note that having two variables with equal values doesn't necessarily mean they are identical.
Example 4: Identity operators in Python
Here, we see that x1 and y1 are integers of the same values, so they are equal as well as identical. The same is the case with x2 and y2 (strings).
But x3 and y3 are lists. They are equal but not identical. It is because the interpreter locates them separately in memory, although they are equal.
- Membership operators
In Python, in and not in are the membership operators. They are used to test whether a value or variable is found in a sequence ( string , list , tuple , set and dictionary ).
In a dictionary, we can only test for the presence of a key, not the value.
Example 5: Membership operators in Python
Here, 'H' is in message , but 'hello' is not present in message (remember, Python is case-sensitive).
Similarly, 1 is key, and 'a' is the value in dictionary dict1 . Hence, 'a' in y returns False .
- Precedence and Associativity of operators in Python
Table of Contents
- Introduction
- Python Arithmetic Operators
- Python Assignment Operators
- Python Comparison Operators
- Python Logical Operators
- Python Bitwise operators
- Python Special operators
Video: Operators in Python
Sorry about that.
Related Tutorials
Python Tutorial
JS Tutorial
Js versions, js functions, js html dom, js browser bom, js web apis, js vs jquery, js graphics, js examples, js references, javascript assignment, javascript assignment operators.
Assignment operators assign values to JavaScript variables.
Shift Assignment Operators
Bitwise assignment operators, logical assignment operators, the = operator.
The Simple Assignment Operator assigns a value to a variable.
Simple Assignment Examples
The += operator.
The Addition Assignment Operator adds a value to a variable.
Addition Assignment Examples
The -= operator.
The Subtraction Assignment Operator subtracts a value from a variable.
Subtraction Assignment Example
The *= operator.
The Multiplication Assignment Operator multiplies a variable.
Multiplication Assignment Example
The **= operator.
The Exponentiation Assignment Operator raises a variable to the power of the operand.
Exponentiation Assignment Example
The /= operator.
The Division Assignment Operator divides a variable.
Division Assignment Example
The %= operator.
The Remainder Assignment Operator assigns a remainder to a variable.
Remainder Assignment Example
Advertisement
The <<= Operator
The Left Shift Assignment Operator left shifts a variable.
Left Shift Assignment Example
The >>= operator.
The Right Shift Assignment Operator right shifts a variable (signed).
Right Shift Assignment Example
The >>>= operator.
The Unsigned Right Shift Assignment Operator right shifts a variable (unsigned).
Unsigned Right Shift Assignment Example
The &= operator.
The Bitwise AND Assignment Operator does a bitwise AND operation on two operands and assigns the result to the the variable.
Bitwise AND Assignment Example
The |= operator.
The Bitwise OR Assignment Operator does a bitwise OR operation on two operands and assigns the result to the variable.
Bitwise OR Assignment Example
The ^= operator.
The Bitwise XOR Assignment Operator does a bitwise XOR operation on two operands and assigns the result to the variable.
Bitwise XOR Assignment Example
The &&= operator.
The Logical AND assignment operator is used between two values.
If the first value is true, the second value is assigned.
Logical AND Assignment Example
The &&= operator is an ES2020 feature .
The ||= Operator
The Logical OR assignment operator is used between two values.
If the first value is false, the second value is assigned.
Logical OR Assignment Example
The ||= operator is an ES2020 feature .
The ??= Operator
The Nullish coalescing assignment operator is used between two values.
If the first value is undefined or null, the second value is assigned.
Nullish Coalescing Assignment Example
The ??= operator is an ES2020 feature .
Test Yourself With Exercises
Use the correct assignment operator that will result in x being 15 (same as x = x + y ).
Start the Exercise

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Boat operator charged with BUI on Old Hickory Lake

One year since school custodian was shot, killed

Man helps people after boat capsizes

Drivers fill gas tanks with mystery substance

Man wanted after one shot in Nashville

School board votes against punishing director of schools

2 killed, 4 in custody after shooting in La Vergne
- SI SWIMSUIT
- SI SPORTSBOOK
Ronald Acuna Jr. Delivers Heartbreaking Message on "X" After Tearing ACL
Brady farkas | may 27, 2024.
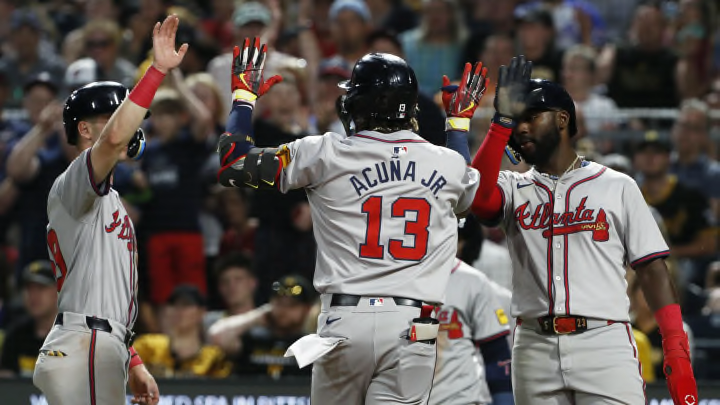
- Atlanta Braves
Atlanta Braves ' superstar and reigning National League MVP winner Ronald Acuna Jr. delivered a heartbreaking message to fans on social media after learning that he had torn his ACL on Sunday.
Acuna simply wrote "Sorry" on "X" with a broken heart emoji and a crying face.
Sorry💔😪 — Ronald Acuña Jr. (@ronaldacunajr24) May 27, 2024
One of the best players in baseball, this is already the second torn ACL for Acuna, who is just 26 years old. He also tore his ACL in 2021, missing the entire Braves' playoff and World Series run. Given the timing of this injury, Acuna is going to miss the rest of 2024 and will likely miss the early portion of 2025 as well.
Acuna Jr. was hitting .250 with four homers this season for the Braves. He had also stolen 16 bases.
Acuna Jr. produced one of the best years we've ever seen in 2023, when he won the MVP by hitting .337 with 41 homers, 106 RBI and a major league best 73 stolen bases. He is also a four-time All-Star who has represented Venezuela in the World Baseball Classic. He is a Rookie of the Year winner and a three-time Silver Slugger also.
In his absence, the Braves will have to rely on a still-potent offense, anchored by Matt Olson, Austin Riley, Marcell Ozuna and Michael Harris II.
The Braves enter play on Monday at 30-20 overall and in second place in the National League East. They will host the visiting Washington Nationals on Monday at 4:10 p.m. ET.
Washington has won two of its last three.
Follow Fastball on FanNation on social media
Continue to follow our Fastball on FanNation coverage on social media by liking us on Facebook and by following us on Twitter @FastballFN .
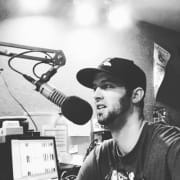
BRADY FARKAS
Brady Farkas is a baseball writer for Fastball on Sports Illustrated/FanNation and the host of 'The Payoff Pitch' podcast which can be found on Apple Podcasts and Spotify. Videos on baseball also posted to YouTube. Brady has spent nearly a decade in sports talk radio and is a graduate of Oswego State University. You can follow him on Twitter @WDEVRadioBrady.
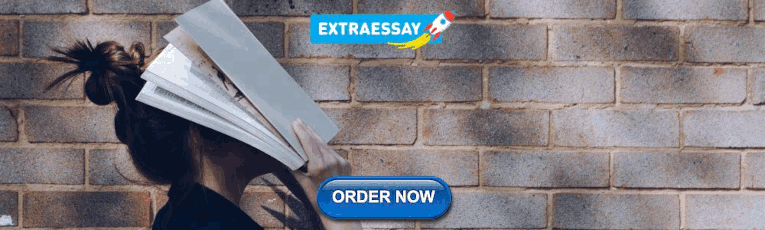
IMAGES
VIDEO
COMMENTS
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Variants. Assignment operators. Assignment operators modify the value of the object. All built-in assignment operators return *this, and most user-defined overloads also return *this so that the user-defined operators can be used in the same manner as the built-ins. However, in a user-defined operator overload, any type can be used as return ...
Assignment Operators. Assignment operators are used to assign values to variables. In the example below, we use the assignment operator ( =) to assign the value 10 to a variable called x:
The Assignment Operator. The central component of an assignment statement is the assignment operator. This operator is represented by the = symbol, which separates two operands: A variable ; A value or an expression that evaluates to a concrete value
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
The built-in assignment operators return the value of the object specified by the left operand after the assignment (and the arithmetic/logical operation in the case of compound assignment operators). The resultant type is the type of the left operand. The result of an assignment expression is always an l-value.
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: | =. In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place.
Discussion. The assignment operator allows us to change the value of a modifiable data object (for beginning programmers this typically means a variable). It is associated with the concept of moving a value into the storage location (again usually a variable). Within C++ programming language the symbol used is the equal symbol.
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
The left-hand operand of ref assignment can be a local reference variable, a ref field, and a ref, out, or in method parameter. Both operands must be of the same type. Compound assignment. For a binary operator op, a compound assignment expression of the form. x op= y is equivalent to. x = x op y except that x is only evaluated once.
Assignment Operators in C are used to assign values to the variables. They come under the category of binary operators as they require two operands to operate upon. The left side operand is called a variable and the right side operand is the value. The value on the right side of the "=" is assigned to the variable on the left side of "=".
The += assignment operator is a combination of + arithmetic operator and = simple assignment operator. For example, x += y; is equivalent to x = x+y;. It adds the right side value to the value of left side operand and assign the result back to the left-hand side operand. #include <stdio.h> int main () { int x = 100, y = 20, z = 50 ...
Python Assignment Operators. Assignment operators are used to assign values to variables: Operator. Example. Same As. Try it. =. x = 5. x = 5.
The assignment (=) operator is used to assign a value to a variable or property. The assignment expression itself has a value, which is the assigned value. This allows multiple assignments to be chained in order to assign a single value to multiple variables. Try it. Syntax. js. x = y
Augmented assignment operators are simply used as a shortcut. Instead of writing x = x + 1, they allow us to write x += 1, effectively "updating" a variable in a concise manner. Here's a code sample of how this works: # Initial assignment. x = 10. print(x) . # output: 10. # Augmented assignment operator: subtraction. x -= 2.
Assignment operators are used to assign values to variables. For example, # assign 5 to x x = 5. Here, = is an assignment operator that assigns 5 to x. Here's a list of different assignment operators available in Python.
Use the correct assignment operator that will result in x being 15 (same as x = x + y ). Start the Exercise. Well organized and easy to understand Web building tutorials with lots of examples of how to use HTML, CSS, JavaScript, SQL, Python, PHP, Bootstrap, Java, XML and more.
Assignment Operator: An assignment operator is the operator used to assign a new value to a variable, property, event or indexer element in C# programming language. Assignment operators can also be used for logical operations such as bitwise logical operations or operations on integral operands and Boolean operands. Unlike in C++, assignment ...
The difference in assignment operators is clearer when you use them to set an argument value in a function call. For example: median(x = 1:10) x. ## Error: object 'x' not found. In this case, x is declared within the scope of the function, so it does not exist in the user workspace. median(x <- 1:10)
Boat operator charged with BUI on Old Hickory Lake. Published: May. 28, 2024 at 3:31 AM PDT | Updated: moments ago. The man was visibly intoxicated after capsizing a boat with people aboard. News.
Atlanta Braves. Atlanta Braves ' superstar and reigning National League MVP winner Ronald Acuna Jr. delivered a heartbreaking message to fans on social media after learning that he had torn his ...