Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python exercises.
You can test your Python skills with W3Schools' Exercises.
We have gathered a variety of Python exercises (with answers) for each Python Chapter.
Try to solve an exercise by filling in the missing parts of a code. If you're stuck, hit the "Show Answer" button to see what you've done wrong.
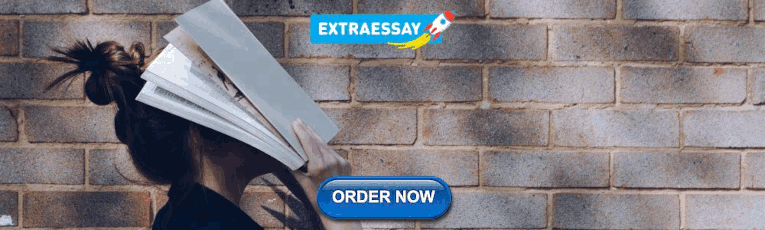
Count Your Score
You will get 1 point for each correct answer. Your score and total score will always be displayed.
Start Python Exercises
Start Python Exercises ❯
If you don't know Python, we suggest that you read our Python Tutorial from scratch.
Kickstart your career
Get certified by completing the course

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
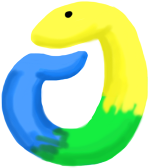
Practice Python
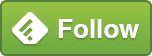
Beginner Python exercises
- Why Practice Python?
- Why Chilis?
- Resources for learners
All Exercises

All Solutions
- 1: Character Input Solutions
- 2: Odd Or Even Solutions
- 3: List Less Than Ten Solutions
- 4: Divisors Solutions
- 5: List Overlap Solutions
- 6: String Lists Solutions
- 7: List Comprehensions Solutions
- 8: Rock Paper Scissors Solutions
- 9: Guessing Game One Solutions
- 10: List Overlap Comprehensions Solutions
- 11: Check Primality Functions Solutions
- 12: List Ends Solutions
- 13: Fibonacci Solutions
- 14: List Remove Duplicates Solutions
- 15: Reverse Word Order Solutions
- 16: Password Generator Solutions
- 17: Decode A Web Page Solutions
- 18: Cows And Bulls Solutions
- 19: Decode A Web Page Two Solutions
- 20: Element Search Solutions
- 21: Write To A File Solutions
- 22: Read From File Solutions
- 23: File Overlap Solutions
- 24: Draw A Game Board Solutions
- 25: Guessing Game Two Solutions
- 26: Check Tic Tac Toe Solutions
- 27: Tic Tac Toe Draw Solutions
- 28: Max Of Three Solutions
- 29: Tic Tac Toe Game Solutions
- 30: Pick Word Solutions
- 31: Guess Letters Solutions
- 32: Hangman Solutions
- 33: Birthday Dictionaries Solutions
- 34: Birthday Json Solutions
- 35: Birthday Months Solutions
- 36: Birthday Plots Solutions
- 37: Functions Refactor Solution
- 38: f Strings Solution
- 39: Character Input Datetime Solution
- 40: Error Checking Solution
Practice 140 exercises in Python
Learn and practice Python by completing 140 exercises that explore different concepts and ideas.
Explore the Python exercises on Exercism
Unlock more exercises as you progress. They’re great practice and fun to do!
- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
Python Exercise with Practice Questions and Solutions
- Python List Exercise
- Python String Exercise
- Python Tuple Exercise
- Python Dictionary Exercise
- Python Set Exercise
Python Matrix Exercises
- Python program to a Sort Matrix by index-value equality count
- Python Program to Reverse Every Kth row in a Matrix
- Python Program to Convert String Matrix Representation to Matrix
- Python - Count the frequency of matrix row length
- Python - Convert Integer Matrix to String Matrix
- Python Program to Convert Tuple Matrix to Tuple List
- Python - Group Elements in Matrix
- Python - Assigning Subsequent Rows to Matrix first row elements
- Adding and Subtracting Matrices in Python
- Python - Convert Matrix to dictionary
- Python - Convert Matrix to Custom Tuple Matrix
- Python - Matrix Row subset
- Python - Group similar elements into Matrix
- Python - Row-wise element Addition in Tuple Matrix
- Create an n x n square matrix, where all the sub-matrix have the sum of opposite corner elements as even
Python Functions Exercises
- Python splitfields() Method
- How to get list of parameters name from a function in Python?
- How to Print Multiple Arguments in Python?
- Python program to find the power of a number using recursion
- Sorting objects of user defined class in Python
- Assign Function to a Variable in Python
- Returning a function from a function - Python
- What are the allowed characters in Python function names?
- Defining a Python function at runtime
- Explicitly define datatype in a Python function
- Functions that accept variable length key value pair as arguments
- How to find the number of arguments in a Python function?
- How to check if a Python variable exists?
- Python - Get Function Signature
- Python program to convert any base to decimal by using int() method
Python Lambda Exercises
- Python - Lambda Function to Check if value is in a List
- Difference between Normal def defined function and Lambda
- Python: Iterating With Python Lambda
- How to use if, else & elif in Python Lambda Functions
- Python - Lambda function to find the smaller value between two elements
- Lambda with if but without else in Python
- Python Lambda with underscore as an argument
- Difference between List comprehension and Lambda in Python
- Nested Lambda Function in Python
- Python lambda
- Python | Sorting string using order defined by another string
- Python | Find fibonacci series upto n using lambda
- Overuse of lambda expressions in Python
- Python program to count Even and Odd numbers in a List
- Intersection of two arrays in Python ( Lambda expression and filter function )
Python Pattern printing Exercises
- Simple Diamond Pattern in Python
- Python - Print Heart Pattern
- Python program to display half diamond pattern of numbers with star border
- Python program to print Pascal's Triangle
- Python program to print the Inverted heart pattern
- Python Program to print hollow half diamond hash pattern
- Program to Print K using Alphabets
- Program to print half Diamond star pattern
- Program to print window pattern
- Python Program to print a number diamond of any given size N in Rangoli Style
- Python program to right rotate n-numbers by 1
- Python Program to print digit pattern
- Print with your own font using Python !!
- Python | Print an Inverted Star Pattern
- Program to print the diamond shape
Python DateTime Exercises
- Python - Iterating through a range of dates
- How to add time onto a DateTime object in Python
- How to add timestamp to excel file in Python
- Convert string to datetime in Python with timezone
- Isoformat to datetime - Python
- Python datetime to integer timestamp
- How to convert a Python datetime.datetime to excel serial date number
- How to create filename containing date or time in Python
- Convert "unknown format" strings to datetime objects in Python
- Extract time from datetime in Python
- Convert Python datetime to epoch
- Python program to convert unix timestamp string to readable date
- Python - Group dates in K ranges
- Python - Divide date range to N equal duration
- Python - Last business day of every month in year
Python OOPS Exercises
- Get index in the list of objects by attribute in Python
- Python program to build flashcard using class in Python
- How to count number of instances of a class in Python?
- Shuffle a deck of card with OOPS in Python
- What is a clean and Pythonic way to have multiple constructors in Python?
- How to Change a Dictionary Into a Class?
- How to create an empty class in Python?
- Student management system in Python
- How to create a list of object in Python class
Python Regex Exercises
- Validate an IP address using Python without using RegEx
- Python program to find the type of IP Address using Regex
- Converting a 10 digit phone number to US format using Regex in Python
- Python program to find Indices of Overlapping Substrings
- Python program to extract Strings between HTML Tags
- Python - Check if String Contain Only Defined Characters using Regex
- How to extract date from Excel file using Pandas?
- Python program to find files having a particular extension using RegEx
- How to check if a string starts with a substring using regex in Python?
- How to Remove repetitive characters from words of the given Pandas DataFrame using Regex?
- Extract punctuation from the specified column of Dataframe using Regex
- Extract IP address from file using Python
- Python program to Count Uppercase, Lowercase, special character and numeric values using Regex
- Categorize Password as Strong or Weak using Regex in Python
- Python - Substituting patterns in text using regex
Python LinkedList Exercises
- Python program to Search an Element in a Circular Linked List
- Implementation of XOR Linked List in Python
- Pretty print Linked List in Python
- Python Library for Linked List
- Python | Stack using Doubly Linked List
- Python | Queue using Doubly Linked List
- Program to reverse a linked list using Stack
- Python program to find middle of a linked list using one traversal
- Python Program to Reverse a linked list
Python Searching Exercises
- Binary Search (bisect) in Python
- Python Program for Linear Search
- Python Program for Anagram Substring Search (Or Search for all permutations)
- Python Program for Binary Search (Recursive and Iterative)
- Python Program for Rabin-Karp Algorithm for Pattern Searching
- Python Program for KMP Algorithm for Pattern Searching
Python Sorting Exercises
- Python Code for time Complexity plot of Heap Sort
- Python Program for Stooge Sort
- Python Program for Recursive Insertion Sort
- Python Program for Cycle Sort
- Bisect Algorithm Functions in Python
- Python Program for BogoSort or Permutation Sort
- Python Program for Odd-Even Sort / Brick Sort
- Python Program for Gnome Sort
- Python Program for Cocktail Sort
- Python Program for Bitonic Sort
- Python Program for Pigeonhole Sort
- Python Program for Comb Sort
- Python Program for Iterative Merge Sort
- Python Program for Binary Insertion Sort
- Python Program for ShellSort
Python DSA Exercises
- Saving a Networkx graph in GEXF format and visualize using Gephi
- Dumping queue into list or array in Python
- Python program to reverse a stack
- Python - Stack and StackSwitcher in GTK+ 3
- Multithreaded Priority Queue in Python
- Python Program to Reverse the Content of a File using Stack
- Priority Queue using Queue and Heapdict module in Python
- Box Blur Algorithm - With Python implementation
- Python program to reverse the content of a file and store it in another file
- Check whether the given string is Palindrome using Stack
- Take input from user and store in .txt file in Python
- Change case of all characters in a .txt file using Python
- Finding Duplicate Files with Python
Python File Handling Exercises
- Python Program to Count Words in Text File
- Python Program to Delete Specific Line from File
- Python Program to Replace Specific Line in File
- Python Program to Print Lines Containing Given String in File
- Python - Loop through files of certain extensions
- Compare two Files line by line in Python
- How to keep old content when Writing to Files in Python?
- How to get size of folder using Python?
- How to read multiple text files from folder in Python?
- Read a CSV into list of lists in Python
- Python - Write dictionary of list to CSV
- Convert nested JSON to CSV in Python
- How to add timestamp to CSV file in Python
Python CSV Exercises
- How to create multiple CSV files from existing CSV file using Pandas ?
- How to read all CSV files in a folder in Pandas?
- How to Sort CSV by multiple columns in Python ?
- Working with large CSV files in Python
- How to convert CSV File to PDF File using Python?
- Visualize data from CSV file in Python
- Python - Read CSV Columns Into List
- Sorting a CSV object by dates in Python
- Python program to extract a single value from JSON response
- Convert class object to JSON in Python
- Convert multiple JSON files to CSV Python
- Convert JSON data Into a Custom Python Object
- Convert CSV to JSON using Python
Python JSON Exercises
- Flattening JSON objects in Python
- Saving Text, JSON, and CSV to a File in Python
- Convert Text file to JSON in Python
- Convert JSON to CSV in Python
- Convert JSON to dictionary in Python
- Python Program to Get the File Name From the File Path
- How to get file creation and modification date or time in Python?
- Menu driven Python program to execute Linux commands
- Menu Driven Python program for opening the required software Application
- Open computer drives like C, D or E using Python
Python OS Module Exercises
- Rename a folder of images using Tkinter
- Kill a Process by name using Python
- Finding the largest file in a directory using Python
- Python - Get list of running processes
- Python - Get file id of windows file
- Python - Get number of characters, words, spaces and lines in a file
- Change current working directory with Python
- How to move Files and Directories in Python
- How to get a new API response in a Tkinter textbox?
- Build GUI Application for Guess Indian State using Tkinter Python
- How to stop copy, paste, and backspace in text widget in tkinter?
- How to temporarily remove a Tkinter widget without using just .place?
- How to open a website in a Tkinter window?
Python Tkinter Exercises
- Create Address Book in Python - Using Tkinter
- Changing the colour of Tkinter Menu Bar
- How to check which Button was clicked in Tkinter ?
- How to add a border color to a button in Tkinter?
- How to Change Tkinter LableFrame Border Color?
- Looping through buttons in Tkinter
- Visualizing Quick Sort using Tkinter in Python
- How to Add padding to a tkinter widget only on one side ?
- Python NumPy - Practice Exercises, Questions, and Solutions
- Pandas Exercises and Programs
- How to get the Daily News using Python
- How to Build Web scraping bot in Python
- Scrape LinkedIn Using Selenium And Beautiful Soup in Python
- Scraping Reddit with Python and BeautifulSoup
- Scraping Indeed Job Data Using Python
Python Web Scraping Exercises
- How to Scrape all PDF files in a Website?
- How to Scrape Multiple Pages of a Website Using Python?
- Quote Guessing Game using Web Scraping in Python
- How to extract youtube data in Python?
- How to Download All Images from a Web Page in Python?
- Test the given page is found or not on the server Using Python
- How to Extract Wikipedia Data in Python?
- How to extract paragraph from a website and save it as a text file?
- Automate Youtube with Python
- Controlling the Web Browser with Python
- How to Build a Simple Auto-Login Bot with Python
- Download Google Image Using Python and Selenium
- How To Automate Google Chrome Using Foxtrot and Python
Python Selenium Exercises
- How to scroll down followers popup in Instagram ?
- How to switch to new window in Selenium for Python?
- Python Selenium - Find element by text
- How to scrape multiple pages using Selenium in Python?
- Python Selenium - Find Button by text
- Web Scraping Tables with Selenium and Python
- Selenium - Search for text on page
- Python Projects - Beginner to Advanced
Python Exercise: Practice makes you perfect in everything. This proverb always proves itself correct. Just like this, if you are a Python learner, then regular practice of Python exercises makes you more confident and sharpens your skills. So, to test your skills, go through these Python exercises with solutions.
Python is a widely used general-purpose high-level language that can be used for many purposes like creating GUI, web Scraping, web development, etc. You might have seen various Python tutorials that explain the concepts in detail but that might not be enough to get hold of this language. The best way to learn is by practising it more and more.
The best thing about this Python practice exercise is that it helps you learn Python using sets of detailed programming questions from basic to advanced. It covers questions on core Python concepts as well as applications of Python in various domains. So if you are at any stage like beginner, intermediate or advanced this Python practice set will help you to boost your programming skills in Python.
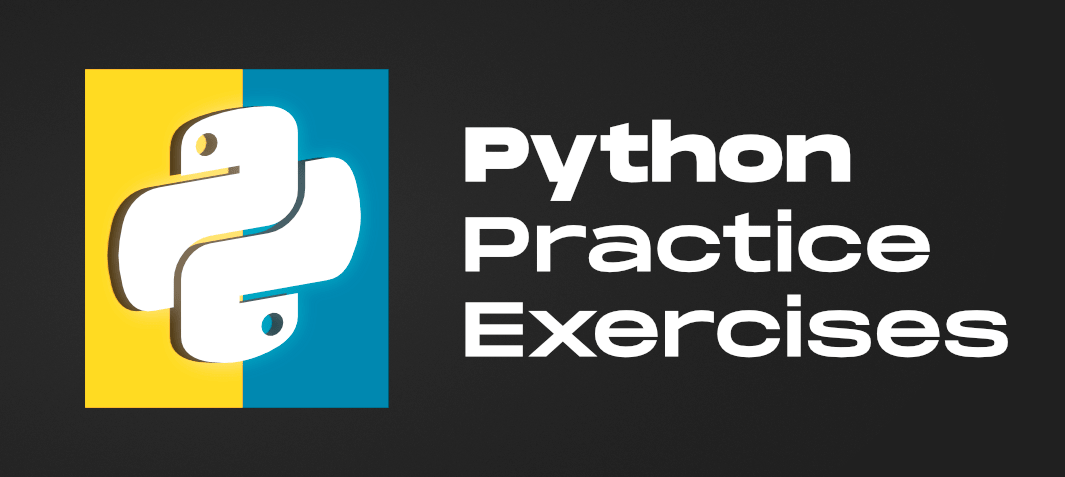
List of Python Programming Exercises
In the below section, we have gathered chapter-wise Python exercises with solutions. So, scroll down to the relevant topics and try to solve the Python program practice set.
Python List Exercises
- Python program to interchange first and last elements in a list
- Python program to swap two elements in a list
- Python | Ways to find length of list
- Maximum of two numbers in Python
- Minimum of two numbers in Python
>> More Programs on List
Python String Exercises
- Python program to check whether the string is Symmetrical or Palindrome
- Reverse words in a given String in Python
- Ways to remove i’th character from string in Python
- Find length of a string in python (4 ways)
- Python program to print even length words in a string
>> More Programs on String
Python Tuple Exercises
- Python program to Find the size of a Tuple
- Python – Maximum and Minimum K elements in Tuple
- Python – Sum of tuple elements
- Python – Row-wise element Addition in Tuple Matrix
- Create a list of tuples from given list having number and its cube in each tuple
>> More Programs on Tuple
Python Dictionary Exercises
- Python | Sort Python Dictionaries by Key or Value
- Handling missing keys in Python dictionaries
- Python dictionary with keys having multiple inputs
- Python program to find the sum of all items in a dictionary
- Python program to find the size of a Dictionary
>> More Programs on Dictionary
Python Set Exercises
- Find the size of a Set in Python
- Iterate over a set in Python
- Python – Maximum and Minimum in a Set
- Python – Remove items from Set
- Python – Check if two lists have atleast one element common
>> More Programs on Sets
- Python – Assigning Subsequent Rows to Matrix first row elements
- Python – Group similar elements into Matrix
>> More Programs on Matrices
>> More Programs on Functions
- Python | Find the Number Occurring Odd Number of Times using Lambda expression and reduce function
>> More Programs on Lambda
- Programs for printing pyramid patterns in Python
>> More Programs on Python Pattern Printing
- Python program to get Current Time
- Get Yesterday’s date using Python
- Python program to print current year, month and day
- Python – Convert day number to date in particular year
- Get Current Time in different Timezone using Python
>> More Programs on DateTime
>> More Programs on Python OOPS
- Python – Check if String Contain Only Defined Characters using Regex
>> More Programs on Python Regex
>> More Programs on Linked Lists
>> More Programs on Python Searching
- Python Program for Bubble Sort
- Python Program for QuickSort
- Python Program for Insertion Sort
- Python Program for Selection Sort
- Python Program for Heap Sort
>> More Programs on Python Sorting
- Program to Calculate the Edge Cover of a Graph
- Python Program for N Queen Problem
>> More Programs on Python DSA
- Read content from one file and write it into another file
- Write a dictionary to a file in Python
- How to check file size in Python?
- Find the most repeated word in a text file
- How to read specific lines from a File in Python?
>> More Programs on Python File Handling
- Update column value of CSV in Python
- How to add a header to a CSV file in Python?
- Get column names from CSV using Python
- Writing data from a Python List to CSV row-wise
>> More Programs on Python CSV
>> More Programs on Python JSON
- Python Script to change name of a file to its timestamp
>> More Programs on OS Module
- Python | Create a GUI Marksheet using Tkinter
- Python | ToDo GUI Application using Tkinter
- Python | GUI Calendar using Tkinter
- File Explorer in Python using Tkinter
- Visiting Card Scanner GUI Application using Python
>> More Programs on Python Tkinter
NumPy Exercises
- How to create an empty and a full NumPy array?
- Create a Numpy array filled with all zeros
- Create a Numpy array filled with all ones
- Replace NumPy array elements that doesn’t satisfy the given condition
- Get the maximum value from given matrix
>> More Programs on NumPy
Pandas Exercises
- Make a Pandas DataFrame with two-dimensional list | Python
- How to iterate over rows in Pandas Dataframe
- Create a pandas column using for loop
- Create a Pandas Series from array
- Pandas | Basic of Time Series Manipulation
>> More Programs on Python Pandas
>> More Programs on Web Scraping
- Download File in Selenium Using Python
- Bulk Posting on Facebook Pages using Selenium
- Google Maps Selenium automation using Python
- Count total number of Links In Webpage Using Selenium In Python
- Extract Data From JustDial using Selenium
>> More Programs on Python Selenium
- Number guessing game in Python
- 2048 Game in Python
- Get Live Weather Desktop Notifications Using Python
- 8-bit game using pygame
- Tic Tac Toe GUI In Python using PyGame
>> More Projects in Python
In closing, we just want to say that the practice or solving Python problems always helps to clear your core concepts and programming logic. Hence, we have designed this Python exercises after deep research so that one can easily enhance their skills and logic abilities.
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
Say "Hello, World!" With Python Easy Max Score: 5 Success Rate: 96.24%
Python if-else easy python (basic) max score: 10 success rate: 89.71%, arithmetic operators easy python (basic) max score: 10 success rate: 97.41%, python: division easy python (basic) max score: 10 success rate: 98.68%, loops easy python (basic) max score: 10 success rate: 98.10%, write a function medium python (basic) max score: 10 success rate: 90.31%, print function easy python (basic) max score: 20 success rate: 97.27%, list comprehensions easy python (basic) max score: 10 success rate: 97.69%, find the runner-up score easy python (basic) max score: 10 success rate: 94.17%, nested lists easy python (basic) max score: 10 success rate: 91.70%, cookie support is required to access hackerrank.
Seems like cookies are disabled on this browser, please enable them to open this website
Holy Python
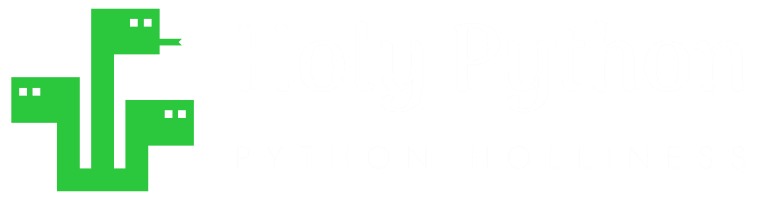
Intermediate Python Exercises
Congratulations on finishing the Beginner Python Exercises !
Here are the intermediate exercises. They are slightly more challenging and there are some bulkier concepts for new programmer, such as: List Comprehensions , For Loops , While Loops , Lambda and Conditional Statements .
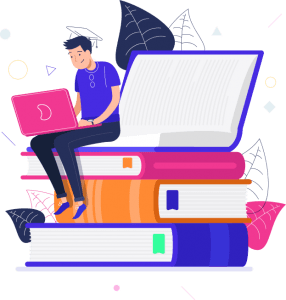
Holy Python is reader-supported. When you buy through links on our site, we may earn an affiliate commission.
Choose the topics you'd like to practice from our extensive exercise list.
Exercise 1: .format() | (6)
Exercise 2: .join() | (5)
Exercise 3: .split() | (3)
Exercise 4: .strip() | (4)
Exercise 5: dir() | (3)
Exercise 6: Nested Data | (5)
Exercise 7: Conditionals – if | (7)
Exercise 8: For Loop | (9)
Exercise 9: While Loop | (4)
Exercise 10: Break & Continue | (2)
Exercise 11: Lambda | (9)
Exercise 12: zip() | (5)
Exercise 13: map() | (7)
Exercise 14: filter() | (6)
Exercise 15: sorted() | (8)
Exercise 16: List Comprehension | (7)
Exercise 17: Dict Comprehension | (3)
Exercise 18: help() | (/)
Exercise 19: Debugging | (/)
Exercise 20: pass | (4)
Congratulations! You’re almost there.
Good luck on this final stretch. Soon you will be a more refined & confident coder and your efforts will start paying off.
One question we encounter on the daily basis is “I think I learned lots of Python so far. What do I do next?” It’s a very understandable question when you’re new to computer programming so, we’ve created an article specifically to address that:
- I’ve been learning coding. What’s Next? (Python Edition)
You can make a great life with coding, not just for you but also for the whole world and you will make it, if you stay at it! Guaranteed almost 100%.
We will continue providing premium, top-quality content to support you in this journey and to give back to the community.
Thank you so much for practicing with HolyPython.com.
FREE ONLINE PYTHON COURSES
Choose from over 100+ free online Python courses.
Python Lessons
Beginner lessons.
Simple builtin Python functions and fundamental concepts.
Intermediate Lessons
More builtin Python functions and slightly heavier fundamental coding concepts.
Advanced Lessons
Python concepts that let you apply coding in the real world generally implementing multiple methods.
Python Exercises
Beginner exercises.
Basic Python exercises that are simple and straightforward.
Intermediate Exercises
Slightly more complex Python exercises and more Python functions to practice.
Advanced Exercises
Project-like Python exercises to connect the dots and prepare for real world tasks.
Don’t forget to check out our Python Tutorials and News page. And, if you found Holypython.com useful please share it with others.

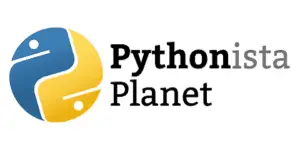
35 Python Programming Exercises and Solutions
To understand a programming language deeply, you need to practice what you’ve learned. If you’ve completed learning the syntax of Python programming language, it is the right time to do some practice programs.
In this article, I’ll list down some problems that I’ve done and the answer code for each exercise. Analyze each problem and try to solve it by yourself. If you have any doubts, you can check the code that I’ve provided below. I’ve also attached the corresponding outputs.
1. Python program to check whether the given number is even or not.
2. python program to convert the temperature in degree centigrade to fahrenheit, 3. python program to find the area of a triangle whose sides are given, 4. python program to find out the average of a set of integers, 5. python program to find the product of a set of real numbers, 6. python program to find the circumference and area of a circle with a given radius, 7. python program to check whether the given integer is a multiple of 5, 8. python program to check whether the given integer is a multiple of both 5 and 7, 9. python program to find the average of 10 numbers using while loop, 10. python program to display the given integer in a reverse manner, 11. python program to find the geometric mean of n numbers, 12. python program to find the sum of the digits of an integer using a while loop, 13. python program to display all the multiples of 3 within the range 10 to 50, 14. python program to display all integers within the range 100-200 whose sum of digits is an even number, 15. python program to check whether the given integer is a prime number or not, 16. python program to generate the prime numbers from 1 to n, 17. python program to find the roots of a quadratic equation, 18. python program to print the numbers from a given number n till 0 using recursion, 19. python program to find the factorial of a number using recursion, 20. python program to display the sum of n numbers using a list, 21. python program to implement linear search, 22. python program to implement binary search, 23. python program to find the odd numbers in an array, 24. python program to find the largest number in a list without using built-in functions, 25. python program to insert a number to any position in a list, 26. python program to delete an element from a list by index, 27. python program to check whether a string is palindrome or not, 28. python program to implement matrix addition, 29. python program to implement matrix multiplication, 30. python program to check leap year, 31. python program to find the nth term in a fibonacci series using recursion, 32. python program to print fibonacci series using iteration, 33. python program to print all the items in a dictionary, 34. python program to implement a calculator to do basic operations, 35. python program to draw a circle of squares using turtle.
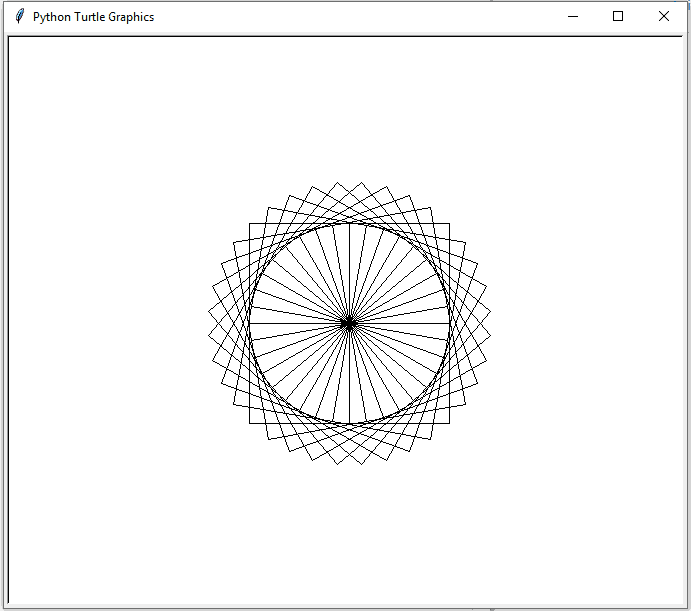
For practicing more such exercises, I suggest you go to hackerrank.com and sign up. You’ll be able to practice Python there very effectively.
Once you become comfortable solving coding challenges, it’s time to move on and build something cool with your skills. If you know Python but haven’t built an app before, I suggest you check out my Create Desktop Apps Using Python & Tkinter course. This interactive course will walk you through from scratch to building clickable apps and games using Python.
I hope these exercises were helpful to you. If you have any doubts, feel free to let me know in the comments.
Happy coding.
I'm the face behind Pythonista Planet. I learned my first programming language back in 2015. Ever since then, I've been learning programming and immersing myself in technology. On this site, I share everything that I've learned about computer programming.
11 thoughts on “ 35 Python Programming Exercises and Solutions ”
I don’t mean to nitpick and I don’t want this published but you might want to check code for #16. 4 is not a prime number.
Thanks man for pointing out the mistake. I’ve updated the code.
# 8. Python program to check whether the given integer is a multiple of both 5 and 7:
You can only check if integer is a multiple of 35. It always works the same – just multiply all the numbers you need to check for multiplicity.
For reverse the given integer n=int(input(“enter the no:”)) n=str(n) n=int(n[::-1]) print(n)
very good, tnks
Please who can help me with this question asap
A particular cell phone plan includes 50 minutes of air time and 50 text messages for $15.00 a month. Each additional minute of air time costs $0.25, while additional text messages cost $0.15 each. All cell phone bills include an additional charge of $0.44 to support 911 call centers, and the entire bill (including the 911 charge) is subject to 5 percent sales tax.
We are so to run the code in phyton
this is best app
Hello Ashwin, Thanks for sharing a Python practice
May be in a better way for reverse.
#”’ Reverse of a string
v_str = str ( input(‘ Enter a valid string or number :- ‘) ) v_rev_str=” for v_d in v_str: v_rev_str = v_d + v_rev_str
print( ‘reverse of th input string / number :- ‘, v_str ,’is :- ‘, v_rev_str.capitalize() )
#Reverse of a string ”’
Problem 15. When searching for prime numbers, the maximum search range only needs to be sqrt(n). You needlessly continue the search up to //n. Additionally, you check all even numbers. As long as you declare 2 to be prime, the rest of the search can start at 3 and check every other number. Another big efficiency improvement.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name and email in this browser for the next time I comment.
Recent Posts
Introduction to Modular Programming with Flask
Modular programming is a software design technique that emphasizes separating the functionality of a program into independent, interchangeable modules. In this tutorial, let's understand what modular...
Introduction to ORM with Flask-SQLAlchemy
While Flask provides the essentials to get a web application up and running, it doesn't force anything upon the developer. This means that many features aren't included in the core framework....
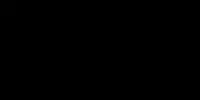
25 Python Projects for Beginners – Easy Ideas to Get Started Coding Python
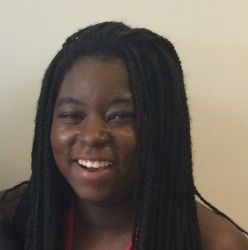
The best way to learn a new programming language is to build projects with it.
I have created a list of 25 beginner friendly project tutorials in Python.
My advice for tutorials would be to watch the video, build the project, break it apart and rebuild it your own way. Experiment with adding new features or using different methods.
That will test if you have really learned the concepts or not.
You can click on any of the projects listed below to jump to that section of the article.
If you are not familiar with the basics of Python, then I would suggest watching this beginner freeCodeCamp Python tutorial .
Python Projects You Can Build
- Guess the Number Game (computer)
- Guess the Number Game (user)
- Rock, paper, scissors
- Countdown Timer
- Password Generator
- QR code encoder / decoder
- Tic-Tac-Toe
- Tic-Tac-Toe AI
- Binary Search
- Minesweeper
- Sudoku Solver
- Photo manipulation in Python
- Markov Chain Text Composer
- Connect Four
- Online Multiplayer Game
- Web Scraping Program
- Bulk file renamer
- Weather Program
Code a Discord Bot with Python - Host for Free in the Cloud
- Space invaders game
Mad libs Python Project
In this Kylie Ying tutorial, you will learn how to get input from the user, work with f-strings, and see your results printed to the console.
This is a great starter project to get comfortable doing string concatenation in Python.
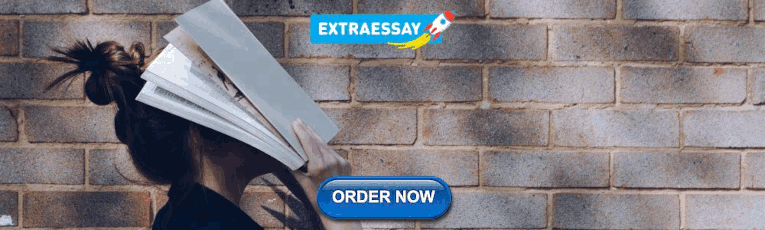
Guess the Number Game Python Project (computer)
In this Kylie Ying tutorial, you will learn how to work with Python's random module , build functions, work with while loops and conditionals, and get user input.
Guess the Number Game Python Project (user)
In this Kylie Ying tutorial, you will build a guessing game where the computer has to guess the correct number. You will work with Python's random module , build functions, work with while loops and conditionals, and get user input.
Rock, paper, scissors Python Project
In this Kylie Ying tutorial , you will work with random.choice() , if statements, and getting user input. This is a great project to help you build on the fundamentals like conditionals and functions.
Hangman Python Project
In this Kylie Ying tutorial, you will learn how to work with dictionaries, lists, and nested if statements. You will also learn how to work with the string and random Python modules.
Countdown Timer Python Project
In this Code With Tomi tutorial , you will learn how to build a countdown timer using the time Python module. This is a great beginner project to get you used to working with while loops in Python.
Password Generator Python Project
In this Code With Tomi tutorial , you will learn how to build a random password generator. You will collect data from the user on the number of passwords and their lengths and output a collection of passwords with random characters.
This project will give you more practice working with for loops and the random Python module.
QR code encoder / decoder Python Project
In this Code With Tomi tutorial , you will learn how to create your own QR codes and encode/decode information from them. This project uses the qrcode library.
This is a great project for beginners to get comfortable working with and installing different Python modules.
Tic-Tac-Toe Python Project
In this Kylie Ying tutorial, you will learn how to build a tic-tac-toe game with various players in the command line. You will learn how to work with Python's time and math modules as well as get continual practice with nested if statements.
Tic-Tac-Toe AI Python Project
In this Kylie Ying tutorial, you will learn how to build a tic-tac-toe game where the computer never loses. This project utilizes the minimax algorithm which is a recursive algorithm used for decision making.
Binary Search Python Project
In this Kylie Ying tutorial, you will learn how to implement the divide and conquer algorithm called binary search. This is a common searching algorithm which comes up in job interviews, which is why it is important to know how to implement it in code.
Minesweeper Python Project
In this Kylie Ying tutorial, you will build the classic minesweeper game in the command line. This project focuses on recursion and classes.
Sudoku Solver Python Project
In this Kylie Ying tutorial, you will learn how to build a sudoku solver which utilizes the backtracking technique. Backtracking is a recursive technique that searches for every possible combination to help solve the problem.
Photo Manipulation in Python Project
In this Kylie Ying tutorial, you will learn how to create an image filter and change the contrast, brightness, and blur of images. Before starting the project, you will need to download the starter files .
Markov Chain Text Composer Python Project
In this Kylie Ying tutorial, you will learn about the Markov chain graph model and how it can be applied the relationship of song lyrics. This project is a great introduction into artificial intelligence in Python.
Pong Python Project
In this Christian Thompson tutorial , you will learn how to recreate the classic pong game in Python. You will be working with the os and turtle Python modules which are great for creating graphics for games.
Snake Python Project
In this Tech with Tim tutorial, you will learn how to recreate the classic snake game in Python. This project uses Object-oriented programming and Pygame which is a popular Python module for creating games.
Connect Four Python Project
In this Keith Galli tutorial, you will learn how to build the classic connect four game. This project utilizes the numpy , math , pygame and sys Python modules.
This project is great if you have already built some smaller beginner Python projects. But if you haven't built any Python projects, then I would highly suggest starting with one of the earlier projects on the list and working your way up to this one.
Tetris Python Project
In this Tech with Tim tutorial, you will learn how to recreate the classic Tetris game. This project utilizes Pygame and is great for beginner developers to take their skills to the next level.
Online Multiplayer Game Python Project
In this Tech with Tim tutorial, you will learn how to build an online multiplayer game where you can play with anyone around the world. This project is a great introduction to working with sockets, networking, and Pygame.
Web Scraping Program Python Project
In this Code With Tomi tutorial , you will learn how to ask for user input for a GitHub user link and output the profile image link through web scraping. Web scraping is a technique that collects data from a web page.
Bulk File Re-namer Python Project
In this Code With Tomi tutorial , you will learn how to build a program that can go into any folder on your computer and rename all of the files based on the conditions set in your Python code.
Weather Program Python Project
In this Code With Tomi tutorial , you will learn how to build a program that collects user data on a specific location and outputs the weather details of that provided location. This is a great project to start learning how to get data from API's.
In this Beau Carnes tutorial , you will learn how to build your own bot that works in Discord which is a platform where people can come together and chat online. This project will teach you how to work with the Discord API and Replit IDE.
After this video was released, Replit changed how you can store your environments variables in your program. Please read through this tutorial on how to properly store environment variables in Replit.
Space Invaders Game Python Project
In this buildwithpython tutorial , you will learn how to build a space invaders game using Pygame. You will learn a lot of basics in game development like game loops, collision detection, key press events, and more.
I am a musician and a programmer.
If you read this far, thank the author to show them you care. Say Thanks
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively.
Python Examples
The best way to learn Python is by practicing examples. This page contains examples on basic concepts of Python. We encourage you to try these examples on your own before looking at the solution.
All the programs on this page are tested and should work on all platforms.
Want to learn Python by writing code yourself? Enroll in our Interactive Python Course for FREE.
- Python Program to Check Prime Number
- Python Program to Add Two Numbers
- Python Program to Find the Factorial of a Number
- Python Program to Make a Simple Calculator
- Python Program to Print Hello world!
- Python Program to Find the Square Root
- Python Program to Calculate the Area of a Triangle
- Python Program to Solve Quadratic Equation
- Python Program to Swap Two Variables
- Python Program to Generate a Random Number
- Python Program to Convert Kilometers to Miles
- Python Program to Convert Celsius To Fahrenheit
- Python Program to Check if a Number is Positive, Negative or 0
- Python Program to Check if a Number is Odd or Even
- Python Program to Check Leap Year
- Python Program to Find the Largest Among Three Numbers
- Python Program to Print all Prime Numbers in an Interval
- Python Program to Display the multiplication Table
- Python Program to Print the Fibonacci sequence
- Python Program to Check Armstrong Number
- Python Program to Find Armstrong Number in an Interval
- Python Program to Find the Sum of Natural Numbers
- Python Program to Display Powers of 2 Using Anonymous Function
- Python Program to Find Numbers Divisible by Another Number
- Python Program to Convert Decimal to Binary, Octal and Hexadecimal
- Python Program to Find ASCII Value of Character
- Python Program to Find HCF or GCD
- Python Program to Find LCM
- Python Program to Find the Factors of a Number
- Python Program to Shuffle Deck of Cards
- Python Program to Display Calendar
- Python Program to Display Fibonacci Sequence Using Recursion
- Python Program to Find Sum of Natural Numbers Using Recursion
- Python Program to Find Factorial of Number Using Recursion
- Python Program to Convert Decimal to Binary Using Recursion
- Python Program to Add Two Matrices
- Python Program to Transpose a Matrix
- Python Program to Multiply Two Matrices
- Python Program to Check Whether a String is Palindrome or Not
- Python Program to Remove Punctuations From a String
- Python Program to Sort Words in Alphabetic Order
- Python Program to Illustrate Different Set Operations
- Python Program to Count the Number of Each Vowel
- Python Program to Merge Mails
- Python Program to Find the Size (Resolution) of an Image
- Python Program to Find Hash of File
- Python Program to Create Pyramid Patterns
- Python Program to Merge Two Dictionaries
- Python Program to Safely Create a Nested Directory
- Python Program to Access Index of a List Using for Loop
- Python Program to Flatten a Nested List
- Python Program to Slice Lists
- Python Program to Iterate Over Dictionaries Using for Loop
- Python Program to Sort a Dictionary by Value
- Python Program to Check If a List is Empty
- Python Program to Catch Multiple Exceptions in One Line
- Python Program to Copy a File
- Python Program to Concatenate Two Lists
- Python Program to Check if a Key is Already Present in a Dictionary
- Python Program to Split a List Into Evenly Sized Chunks
- Python Program to Parse a String to a Float or Int
- Python Program to Print Colored Text to the Terminal
- Python Program to Convert String to Datetime
- Python Program to Get the Last Element of the List
- Python Program to Get a Substring of a String
- Python Program to Print Output Without a Newline
- Python Program Read a File Line by Line Into a List
- Python Program to Randomly Select an Element From the List
- Python Program to Check If a String Is a Number (Float)
- Python Program to Count the Occurrence of an Item in a List
- Python Program to Append to a File
- Python Program to Delete an Element From a Dictionary
- Python Program to Create a Long Multiline String
- Python Program to Extract Extension From the File Name
- Python Program to Measure the Elapsed Time in Python
- Python Program to Get the Class Name of an Instance
- Python Program to Convert Two Lists Into a Dictionary
- Python Program to Differentiate Between type() and isinstance()
- Python Program to Trim Whitespace From a String
- Python Program to Get the File Name From the File Path
- Python Program to Represent enum
- Python Program to Return Multiple Values From a Function
- Python Program to Get Line Count of a File
- Python Program to Find All File with .txt Extension Present Inside a Directory
- Python Program to Get File Creation and Modification Date
- Python Program to Get the Full Path of the Current Working Directory
- Python Program to Iterate Through Two Lists in Parallel
- Python Program to Check the File Size
- Python Program to Reverse a Number
- Python Program to Compute the Power of a Number
- Python Program to Count the Number of Digits Present In a Number
- Python Program to Check If Two Strings are Anagram
- Python Program to Capitalize the First Character of a String
- Python Program to Compute all the Permutation of the String
- Python Program to Create a Countdown Timer
- Python Program to Count the Number of Occurrence of a Character in String
- Python Program to Remove Duplicate Element From a List
- Python Program to Convert Bytes to a String
10 if-else Practice Problems in Python

- learn python
- python programming
If you're trying to learn Python, you need to practice! These ten if-else Python practice problems provide you some hands-on experience. And don’t worry – we’ve provided full code solutions and detailed explanations!
Python is particularly good for beginners to learn. Its clear syntax can be read almost as clearly as a normal sentence. The if-else statement is a good example of this; it lets you build logic into your programs. This article will walk you through ten i f-else practice exercises in Python. Each one is specifically designed for beginners, helping you hone your understanding of if-else statements.
The exercises in this article are taken directly from our courses, including Python Basics: Part 1 . This course helps absolute beginners understand computer programming with Python; if you’ve never written a line of code, this course is a great place to start.
As the saying goes, practice makes perfect. Learning Python is no exception. As we discuss in Different Ways to Practice Python , doing online courses is a great way to practice a variety of topics. The more you practice writing code, the more comfortable and confident you'll become in your abilities. So – whether you aim to build your own software, delve into data science, or automate mundane tasks – dedicating time to practice is key.
The Python if-else Statement
Before we dive into the exercises, let's briefly discuss the workings of if-else statements in Python. An if-else statement allows your program to make decisions based on certain conditions. The syntax is straightforward:
- You provide a condition to evaluate within the if
- If that condition is true, the corresponding block of code is executed.
- If the condition is false, the code within the optional else block is executed instead.
Here's a simple example:
In this example, if the value of x is greater than 5, the program will print "x is greater than 5"; otherwise, it will print "x is not greater than 5".
This concept forms the backbone of decision-making in Python programs.
To get the most benefit from this article, attempt the problems before reading the solutions. Some of these exercises will have several possible solutions, so also try to think about alternative ways to solve the same problem. Let’s get started.
10 if-else Python Practice Exercises
Exercise 1: are you tall.
Exercise: Write a program that will ask the user for their height in centimeters. Use the input() built-in function to do this. If the height is more than 185 centimeters, print the following line of code:
Explanation: This is a similar syntax to the above template example. The height variable stores the user input as an integer and tests if it’s greater than 185 cm. If so, the message is printed. Notice there is no else; this means if the height is less than or equal to 185, nothing happens.
Exercise 2: More Options
Exercise: Write a program that will ask the user the following question:
- If the user answers y , print: Wrong! Canberra is the capital!
- If the user answers n , print: Correct!
- If the user answers anything else, print: I do not understand your answer!
Explanation: The if statement can be extended with an elif (i.e. else if) to check any answer that returns False to the first condition. If the elif statement also returns False , the indented block under the else is executed.
Exercise 3: Check If a Character Is in a String
Exercise: Write a program to ask the user to do the following:
- Provide the name of a country that does not contain any lowercase a or e letters. (Use the prompt: The country is : )
- If the user provides a correct string (i.e. one with no a or e inside it), print: You won... unless you made this name up!
- Otherwise, print: You lost!
(By the way, possible answers include Hong Kong, Cyprus, and Togo.)
Explanation: This code prompts the user to input a country name using the built-in input() function. Then, it checks if the letters 'a' or 'e' is present in the inputted country name using the in operator. If either letter is found, it prints "You lost!". Otherwise, it prints "You won... unless you made this name up!" using the else statement.
Exercise 4: Count Letter Frequency in a String
Exercise: The letter e is said to be the most frequent letter in the English language. Count and print how many times this letter appears in the poem below:
John Knox was a man of wondrous might, And his words ran high and shrill, For bold and stout was his spirit bright, And strong was his stalwart will. Kings sought in vain his mind to chain, And that giant brain to control, But naught on plain or stormy main Could daunt that mighty soul. John would sit and sigh till morning cold Its shining lamps put out, For thoughts untold on his mind lay hold, And brought but pain and doubt. But light at last on his soul was cast, Away sank pain and sorrow, His soul is gay, in a fair to-day, And looks for a bright to-morrow. (Source: "Unidentified," in Current Opinion, July 1888)
Explanation: The solution starts by initializing a counter with the value of 0. Then a for loop is used to loop through every letter in the string variable poem. The if statement tests if the current letter is an e; if it is, the counter is incremented by 1. Finally, the result of the counter is printed. Note that there is no else needed in this solution, since nothing should happen if the letter is not an e.
Exercise 5: Format Recipes
Exercise: Anne loves cooking and she tries out various recipes from the Internet. Sometimes, though, she finds recipes that are written as a single paragraph of text. For instance:
When you're in the middle of cooking, such a paragraph is difficult to read. Anne would prefer an ordered list, like this:
- Cut a slit into the chicken breast.
- Stuff it with mustard, mozzarella and cheddar.
- Secure the whole thing with rashers of bacon.
- Roast for 20 minutes at 200C.
Write a Python script that accepts a recipe string from the user ('Paste your recipe: ') and prints an ordered list of sentences, just like in the example above.
Each sentence of both the input and output should end with a single period.
Explanation: The solution takes a recipe input from the user with input() , then iterates through each character in the recipe. Whenever it encounters a period ( '.' ), it increments a counter, adds a new line character ( '\n' ) and the incremented counter followed by a period to an ordered list. If it encounters any other character, it simply adds that character to the ordered list. Finally, it calculates the length of the counter, removes the last part of the ordered list to exclude the unnecessary counter, and prints the modified ordered list, which displays the recipe with numbered steps.
Exercise 6: Nested if Statements
Exercise: A leap year is a year that consists of 366 (not 365) days. It occurs roughly every four years. More specifically, a year is considered leap if it is either divisible by 4 but not by 100 or it is divisible by 400.
Write a program that asks the user for a year and replies with either leap year or not a leap year.
Explanation: This program takes a year from the user as input. It checks if the year is divisible by 4. If it is, it then checks if the year is divisible by 100. If it is, it further checks if the year is divisible by 400. If it is, it prints 'leap year' because it satisfies all the conditions for a leap year. This is all achieved with nesting.
If it's divisible by 4, but not by 100, or if it’s not divisible by 400, the program prints 'not a leap year'. If the year is not divisible by 100, it directly prints 'leap year' because it satisfies the basic condition for a leap year (being divisible by 4). If the year is not divisible by 4, it prints 'not a leap year'.
Exercise 7: Scrabble
Exercise: Create a function called can_build_word(letters_list, word) that takes a list of letters and a word. The function should return True if the word uses only the letters from the list.
Like in real Scrabble, you cannot use one tile twice in the same word. For example, if a list of letters contains only one 'a', ‘banana’ returns False. The function should also return False if the word contains any letters not in the list.
For example …
… should return True. On the other hand …
… should return False because there is only one 'f'.
One thing to keep in mind: Neither the provided letter list nor the word should be changed by your function. It may be tempting to remove letters from the letters list to remove the used tiles. This should not be the case. Instead, you should copy the passed list. Simply use the list() function and pass it another list:
This way we can safely operate on a copy of the list without changing the contents of the original list.
Explanation: The function starts by making a copy of the list of letters to avoid modifying the original. Then, it iterates through each letter in the word. For each letter, it checks if that letter exists in the list copy. If it does, it removes that letter from the copy, which ensures each letter is used only once.
If the letter doesn't exist in the copy of the letters list, it means the word cannot be formed using the available letters; it returns False. If all the letters in the word can be found and removed from the list copy, it means the word can indeed be formed; it returns True .
If you are looking for additional material to help you learn how to work with Python lists, our article 12 Beginner-Level Python List Exercises with Solutions has got you covered.
Exercise 8: for Loops and if Statements
Exercise: Write a program that asks for a positive integer and prints all of its divisors, one by one, on separate lines in ascending order.
A divisor is a number that divides a particular number with no remainder. For example, the divisors of 4 are 1, 2, and 4.
To check if a is a divisor of b , you can use the modulo operator %. a % b returns the remainder of dividing a by b . For example, 9 % 2 is 1 because 9 = (4 * 2) + 1, and 9 % 3 is 0 because 9 divides into 3 with no remainder. If a % b == 0 , then b is a divisor of a .
Explanation: This solution takes an input number from the user and then iterates through numbers from 1 to that input number (inclusive). For each number i in this range, it checks if the input number is divisible by i with no remainder ( number % i == 0 ). If the condition is satisfied, it means that i is a factor of the input number.
Looping is another important skill in Python. This problem could also be solved with a while loop. Take a look at 10 Python Loop Exercises with Solutions to learn more about for and while loops.
Exercise 9: if Statements and Data Structures
Exercise: Write a function named get_character_frequencies() that takes a string as input and returns a dictionary of lowercase characters as keys and the number of their occurrences as values. Ignore the case of a character.
Explanation: This function initializes an empty dictionary ( freq_dict ) to store the frequencies of characters. It iterates through each character in the input word using a for loop. Within the loop, it converts each character to lowercase using the lower() method; this ensures case-insensitive counting.
The program checks if the lowercase character is already a key in the dictionary. If it's not, it adds the character as a key and sets its frequency to 1. If it is already a key, it increments the frequency count for that character by 1 and returns the dictionary.
If you’re not familiar with how Python dictionaries work, Top 10 Python Dictionary Exercises for Beginners will give you experience working with this important data structure.
Exercise 10: if Statements and Multiple Returns
Exercise: Write a function named return_bigger(a, b) that takes two numbers and returns the bigger one. If the numbers are equal, return 0.
Explanation: When using if statements in a function, you can define a return value in the indented block of code under the if-else statement. This makes your code simpler and more readable – another great example of the clear syntax which makes Python such an attractive tool for all programmers.
Do You Want More Python Practice Exercises?
Learning anything new is a challenge. Relying on a variety of resources is a good way to get a broad background. Read some books and blogs to get a theoretical understanding, watch videos online, take advantage of the active Python community on platforms like Stack Overflow . And most importantly, do some hands-on practice. We discuss these points in detail in What’s the Best Way to Practice Python?
The Python practice exercises and solutions shown here were taken directly from several of our interactive online courses, including Python Basics Practice , Python Practice: Word Games , and Working with Strings in Python . The courses build up your skills and knowledge, giving you exposure to a variety of topics.
And if you’re already motivated to take your skills to the next level, have a go at another 10 Python Practice Exercises for Beginners with Solutions . Happy coding!
You may also like
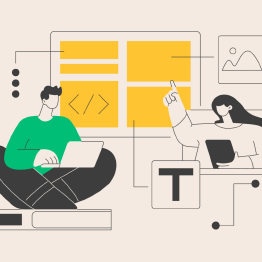
How Do You Write a SELECT Statement in SQL?
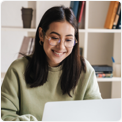
What Is a Foreign Key in SQL?

Enumerate and Explain All the Basic Elements of an SQL Query
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
python-practice
Here are 55 public repositories matching this topic..., jassics / learning-python.
Learning Python Concepts with easy to understand code examples, lab exercises, real-world examples.
- Updated May 13, 2024
Ram-95 / Python-Competitive-Programming
💻 [Competitive Programming] This Repo consists of my solutions in Python 3 to various problems of [HackerRank, Leetcode, Codeforces, Code Chef etc.]
- Updated Dec 17, 2021
Nishad00 / Python-Practice-Solved-Programs
This Repository is a collection of all of my solved problems on Hacker rank in Python course. This repository contain basic program from hello world to some advanced program like puzzle or Game
- Updated Oct 1, 2020
HackBinghamtonArchives / Intermediate-Python-Tutorial
🐍 Repo for a HackBU workshop on some Intermediate Python practices, just a step above basic for loops and if statements and what not.
- Updated Apr 20, 2018
- Jupyter Notebook
shouhaddo / Python-Training
This is a repository to share my students who are undergoing python training under me codes of everyday lectures provided by me.
- Updated Apr 18, 2021
devs-in-tech / Python-Bootcamp-Notes
This repository contains all the notes, assignments and resources of my Python Bootcamp on YouTube Channel.
- Updated Mar 11, 2022
JalanJiang / python-100-practice
😼 Python 100 天从新手到大师进击练习
- Updated May 30, 2019
flyhigher139 / python_practices
python practices
- Updated Dec 22, 2016
RisingLight / Python_Practice
Repository for challenging Python problems we came across.
- Updated Nov 15, 2019
raihan-uddin / Python-Project-Based-Practice
Python Practice!
- Updated Apr 26, 2017
matta174 / python_practice
My journey into teaching myself Python
- Updated Feb 13, 2017
rajeshkumarkarra / LearnAI
Learn AI in a easy way
- Updated Jul 3, 2019
aiyogg / pdm-demo
Python template project useing PDM.
- Updated Jun 6, 2022
dlujHub / Automate-the-boring-stuff-with-Python
Automate the Boring Stuff with Python. Practice Projects
- Updated Mar 9, 2019
arthurosipyan / Python-Programming-Bootcamp
Derek Banas Python Course from Udemy ( https://www.udemy.com/course/ultimate-python-tutorial/ )
- Updated Aug 18, 2021
irsol / python-exercises
- Updated Apr 8, 2020
jlomeli71 / python-notes
Files upload for backup about python
- Updated Jan 7, 2021
kHarshit / python-projects
This is a series of python projects.
- Updated Oct 2, 2019
himanshu0113 / pythonCrap
Python practice, basics and few advance matrix operations. Mostly numpy.
- Updated Sep 18, 2017
sci-c0 / python-practice-projects
A collection of python projects for practice
- Updated May 19, 2022
Improve this page
Add a description, image, and links to the python-practice topic page so that developers can more easily learn about it.
Curate this topic
Add this topic to your repo
To associate your repository with the python-practice topic, visit your repo's landing page and select "manage topics."
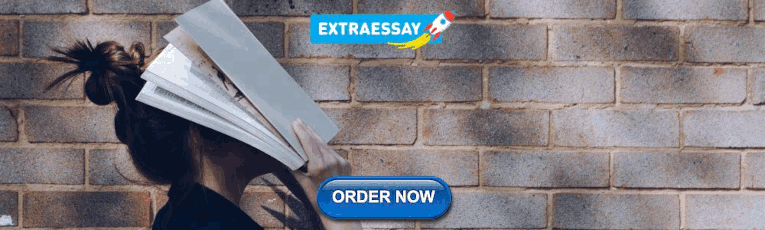
IMAGES
VIDEO
COMMENTS
Practice Python programming skills with free coding exercises for beginners and intermediate developers. Each exercise covers a specific Python topic and provides solutions and hints.
Improve your Python skills with these 15 exercises covering fundamental concepts, data structures, and algorithms. Each problem is followed by a solution and explanation, and some problems are taken from our Python courses.
Test your Python skills with interactive exercises for each chapter. Fill in the missing code and get instant feedback on your score and errors.
Learn and practice Python with interactive exercises and solutions. Choose from 40 topics ranging from basic syntax to advanced topics such as functions, files, web scraping, and plots.
Solve programming problems in Python with step-by-step instructions and tests. Learn how to use search engines, error messages, and code examples to discover how to do things in Python.
Edabit offers over 2,500 Python challenges for different levels and topics, from language fundamentals to algorithms. You can write code, check your answer, and see the solution in the same web page.
Learn Python online by writing actual code with free exercises, courses, projects, and tutorials. Find out how to practice Python at home, on mobile, or in 30 days.
Improve your Python skills with 10 exercises covering user input, strings, loops, functions, and more. Each exercise has a solution with detailed explanations and examples.
Learn how to write code for interview-style problems in Python with this tutorial. You'll solve five problems, from sum of a range of integers to Sudoku solver, and see the solutions and discussions.
1. 2. ». Explore project-based Python tutorials and gain practical coding skills. Work on Python projects that help you gain real-world programming experience. These projects include full source code and step-by-step instructions, and will make you more confident in tackling real-world coding challenges.
Exercism offers 140 exercises in Python that explore different concepts and ideas. You can unlock more exercises as you progress and get feedback from mentors.
Learn Python using sets of detailed programming questions from basic to advanced. This web page covers questions on core Python concepts as well as applications of Python in various domains, with solutions and explanations.
This Python essential exercise is to help Python beginners to learn necessary Python skills quickly.. Immerse yourself in the practice of Python's foundational concepts, such as loops, control flow, data types, operators, list, strings, input-output, and built-in functions.
Join over 23 million developers in solving code challenges on HackerRank, one of the best ways to prepare for programming interviews.
Intermediate Python Exercises. Congratulations on finishing the Beginner Python Exercises! Here are the intermediate exercises. They are slightly more challenging and there are some bulkier concepts for new programmer, such as: List Comprehensions, For Loops, While Loops, Lambda and Conditional Statements. Holy Python is reader-supported.
This Python functions exercise aims to help Python developers to learn and practice how to define functions. Also, you will practice how to create and use the nested functions and the function arguments effectively. ... It contains Python function assignments, programs, questions, and challenges. Total 10 questions. The solution is provided for ...
Learn and practice Python syntax with 35 problems and code examples. Find out how to check even or odd numbers, convert temperature, find area, average, product, circumference, and more.
Python Projects You Can Build. Mad Libs. Guess the Number Game (computer) Guess the Number Game (user) Rock, paper, scissors. Hangman. Countdown Timer. Password Generator. QR code encoder / decoder.
Guessing Game — This is another beginner-level project that'll help you learn and practice the basics. Mad Libs — Use Python code to make interactive Python Mad Libs! Hangman — Another childhood classic that you can make to stretch your Python skills. Snake — This is a bit more complex, but it's a classic (and surprisingly fun) game ...
All Examples. Files. Python Program to Print Hello world! Python Program to Add Two Numbers. Python Program to Find the Square Root. Python Program to Calculate the Area of a Triangle. Python Program to Solve Quadratic Equation. Python Program to Swap Two Variables. Python Program to Generate a Random Number.
Python Exercises, Practice, Solution: Python is a widely used high-level, general-purpose, interpreted, dynamic programming language. Its design philosophy emphasizes code readability, and its syntax allows programmers to express concepts in fewer lines of code than possible in languages such as C++ or Java.
The syntax is straightforward: You provide a condition to evaluate within the if. If that condition is true, the corresponding block of code is executed. If the condition is false, the code within the optional else block is executed instead. Here's a simple example: x = 10. if x > 5: print("x is greater than 5") else:
python notes python3 python-programming python-practice python-book assignments python-basics python-bootcamp python-notes python-project-beginner python-projects Updated Mar 11, 2022; Python ... Python practice, basics and few advance matrix operations. Mostly numpy. numpy coding python-2 python-practice Updated Sep 18, 2017;
However, assertions for input validation are not a best practice as they can disabled easily and will lead to unexpected behaviour in production. The use of explicit Python conditional expressions is preferable for input validation and enforcing pre-conditions, post-conditions, and code invariants. 3. Lazy Loading with Generators and Yield ...