Learn Java practically and Get Certified .
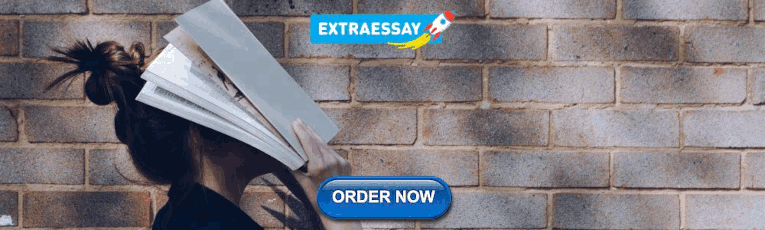
Popular Tutorials
Popular examples, reference materials, learn java interactively, java introduction.
- Get Started With Java
- Your First Java Program
- Java Comments
Java Fundamentals
- Java Variables and Literals
- Java Data Types (Primitive)
- Java Operators
- Java Basic Input and Output
Java Expressions, Statements and Blocks
Java Flow Control
Java if...else statement.
Java Ternary Operator
- Java for Loop
- Java for-each Loop
- Java while and do...while Loop
Java break Statement
- Java continue Statement
Java switch Statement
- Java Arrays
- Java Multidimensional Arrays
- Java Copy Arrays
Java OOP(I)
- Java Class and Objects
- Java Methods
- Java Method Overloading
- Java Constructors
- Java Static Keyword
- Java Strings
- Java Access Modifiers
- Java this Keyword
- Java final keyword
- Java Recursion
- Java instanceof Operator
Java OOP(II)
- Java Inheritance
- Java Method Overriding
- Java Abstract Class and Abstract Methods
- Java Interface
- Java Polymorphism
- Java Encapsulation
Java OOP(III)
- Java Nested and Inner Class
- Java Nested Static Class
- Java Anonymous Class
- Java Singleton Class
- Java enum Constructor
- Java enum Strings
- Java Reflection
- Java Package
- Java Exception Handling
- Java Exceptions
- Java try...catch
- Java throw and throws
- Java catch Multiple Exceptions
- Java try-with-resources
- Java Annotations
- Java Annotation Types
- Java Logging
- Java Assertions
- Java Collections Framework
- Java Collection Interface
- Java ArrayList
- Java Vector
- Java Stack Class
- Java Queue Interface
- Java PriorityQueue
- Java Deque Interface
- Java LinkedList
- Java ArrayDeque
- Java BlockingQueue
- Java ArrayBlockingQueue
- Java LinkedBlockingQueue
- Java Map Interface
- Java HashMap
- Java LinkedHashMap
- Java WeakHashMap
- Java EnumMap
- Java SortedMap Interface
- Java NavigableMap Interface
- Java TreeMap
- Java ConcurrentMap Interface
- Java ConcurrentHashMap
- Java Set Interface
- Java HashSet Class
- Java EnumSet
- Java LinkedHashSet
- Java SortedSet Interface
- Java NavigableSet Interface
- Java TreeSet
- Java Algorithms
- Java Iterator Interface
- Java ListIterator Interface
Java I/o Streams
- Java I/O Streams
- Java InputStream Class
- Java OutputStream Class
- Java FileInputStream Class
- Java FileOutputStream Class
- Java ByteArrayInputStream Class
- Java ByteArrayOutputStream Class
- Java ObjectInputStream Class
- Java ObjectOutputStream Class
- Java BufferedInputStream Class
- Java BufferedOutputStream Class
- Java PrintStream Class
Java Reader/Writer
- Java File Class
- Java Reader Class
- Java Writer Class
- Java InputStreamReader Class
- Java OutputStreamWriter Class
- Java FileReader Class
- Java FileWriter Class
- Java BufferedReader
- Java BufferedWriter Class
- Java StringReader Class
- Java StringWriter Class
- Java PrintWriter Class
Additional Topics
- Java Keywords and Identifiers
- Java Operator Precedence
- Java Bitwise and Shift Operators
- Java Scanner Class
- Java Type Casting
- Java Wrapper Class
- Java autoboxing and unboxing
- Java Lambda Expressions
- Java Generics
- Nested Loop in Java
- Java Command-Line Arguments
Java Tutorials
In programming, we use the if..else statement to run a block of code among more than one alternatives.
For example, assigning grades (A, B, C) based on the percentage obtained by a student.
- if the percentage is above 90 , assign grade A
- if the percentage is above 75 , assign grade B
- if the percentage is above 65 , assign grade C
1. Java if (if-then) Statement
The syntax of an if-then statement is:
Here, condition is a boolean expression such as age >= 18 .
- if condition evaluates to true , statements are executed
- if condition evaluates to false , statements are skipped
Working of if Statement
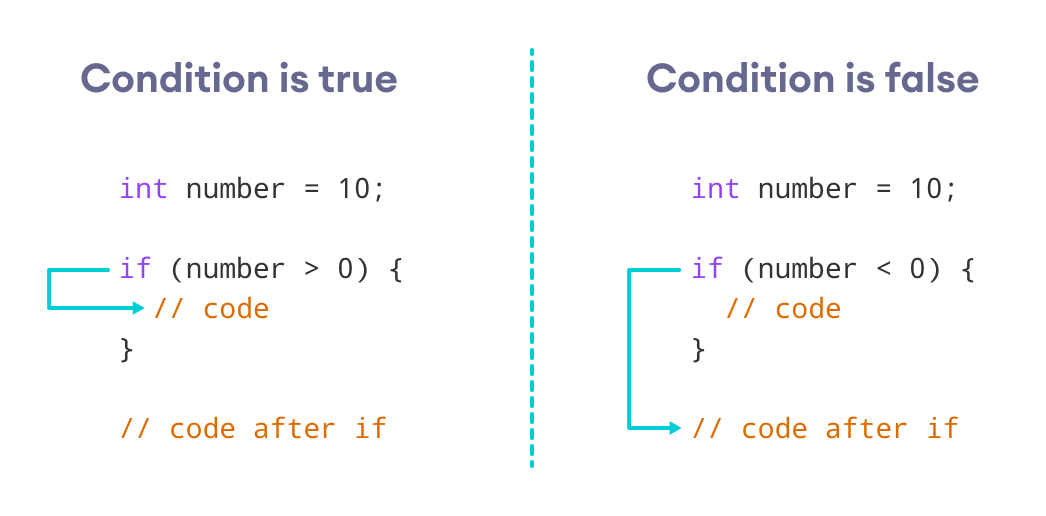
Example 1: Java if Statement
In the program, number < 0 is false . Hence, the code inside the body of the if statement is skipped .
Note: If you want to learn more about about test conditions, visit Java Relational Operators and Java Logical Operators .
We can also use Java Strings as the test condition.
Example 2: Java if with String
In the above example, we are comparing two strings in the if block.
2. Java if...else (if-then-else) Statement
The if statement executes a certain section of code if the test expression is evaluated to true . However, if the test expression is evaluated to false , it does nothing.
In this case, we can use an optional else block. Statements inside the body of else block are executed if the test expression is evaluated to false . This is known as the if-...else statement in Java.
The syntax of the if...else statement is:
Here, the program will do one task (codes inside if block) if the condition is true and another task (codes inside else block) if the condition is false .
How the if...else statement works?
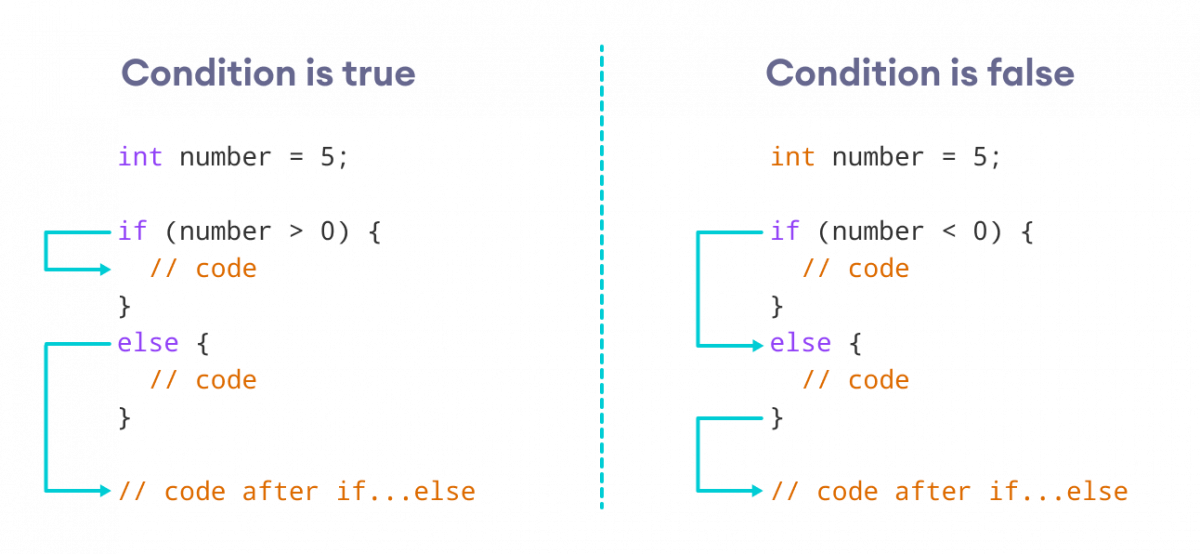
Example 3: Java if...else Statement
In the above example, we have a variable named number . Here, the test expression number > 0 checks if number is greater than 0.
Since the value of the number is 10 , the test expression evaluates to true . Hence code inside the body of if is executed.
Now, change the value of the number to a negative integer. Let's say -5 .
If we run the program with the new value of number , the output will be:
Here, the value of number is -5 . So the test expression evaluates to false . Hence code inside the body of else is executed.
3. Java if...else...if Statement
In Java, we have an if...else...if ladder, that can be used to execute one block of code among multiple other blocks.
Here, if statements are executed from the top towards the bottom. When the test condition is true , codes inside the body of that if block is executed. And, program control jumps outside the if...else...if ladder.
If all test expressions are false , codes inside the body of else are executed.
How the if...else...if ladder works?
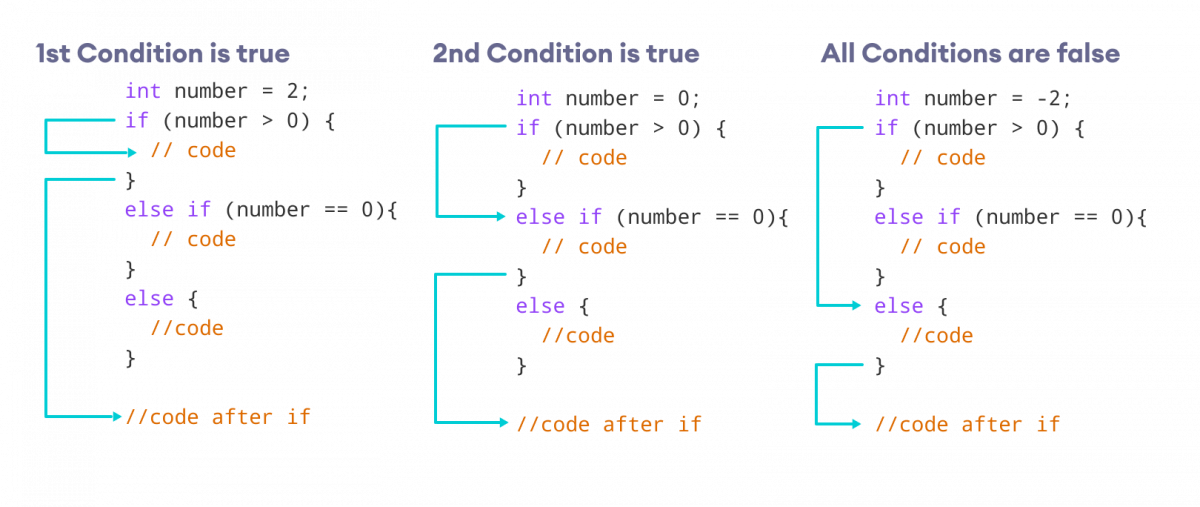
Example 4: Java if...else...if Statement
In the above example, we are checking whether number is positive, negative, or zero . Here, we have two condition expressions:
- number > 0 - checks if number is greater than 0
- number < 0 - checks if number is less than 0
Here, the value of number is 0 . So both the conditions evaluate to false . Hence the statement inside the body of else is executed.
Note : Java provides a special operator called ternary operator , which is a kind of shorthand notation of if...else...if statement. To learn about the ternary operator, visit Java Ternary Operator .
4. Java Nested if..else Statement
In Java, it is also possible to use if..else statements inside an if...else statement. It's called the nested if...else statement.
Here's a program to find the largest of 3 numbers using the nested if...else statement.
Example 5: Nested if...else Statement
In the above programs, we have assigned the value of variables ourselves to make this easier.
However, in real-world applications, these values may come from user input data, log files, form submission, etc.
Table of Contents
- Introduction
- Java if (if-then) Statement
- Example: Java if Statement
- Java if...else (if-then-else) Statement
- Example: Java if else Statement
- Java if..else..if Statement
- Example: Java if..else..if Statement
- Java Nested if..else Statement
Sorry about that.
Related Tutorials
Java Tutorial
Java Tutorial
Java methods, java classes, java file handling, java how to, java reference, java examples, java if ... else, java conditions and if statements.
You already know that Java supports the usual logical conditions from mathematics:
- Less than: a < b
- Less than or equal to: a <= b
- Greater than: a > b
- Greater than or equal to: a >= b
- Equal to a == b
- Not Equal to: a != b
You can use these conditions to perform different actions for different decisions.
Java has the following conditional statements:
- Use if to specify a block of code to be executed, if a specified condition is true
- Use else to specify a block of code to be executed, if the same condition is false
- Use else if to specify a new condition to test, if the first condition is false
- Use switch to specify many alternative blocks of code to be executed
The if Statement
Use the if statement to specify a block of Java code to be executed if a condition is true .
Note that if is in lowercase letters. Uppercase letters (If or IF) will generate an error.
In the example below, we test two values to find out if 20 is greater than 18. If the condition is true , print some text:
Try it Yourself »
We can also test variables:
Example explained
In the example above we use two variables, x and y , to test whether x is greater than y (using the > operator). As x is 20, and y is 18, and we know that 20 is greater than 18, we print to the screen that "x is greater than y".
Test Yourself With Exercises
Print "Hello World" if x is greater than y .
Start the Exercise

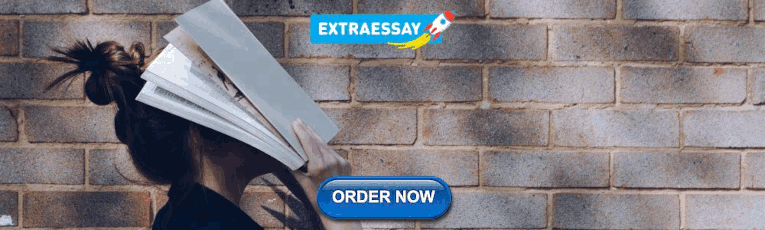
COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Java if-else Statements
Java programming tutorial index.
If else statements in Java is also used to control the program flow based on some condition, only the difference is: it's used to execute some statement code block if the expression is evaluated to true, otherwise executes else statement code block.
The basic format of if else statement is:
Figure - Flowchart of if-else Statement:
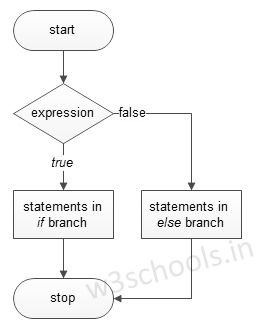
Example of a Java Program to Demonstrate If else statements
Program Output:
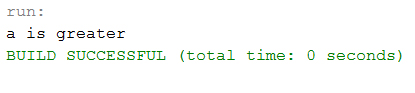
The Java Tutorials have been written for JDK 8. Examples and practices described in this page don't take advantage of improvements introduced in later releases and might use technology no longer available. See Java Language Changes for a summary of updated language features in Java SE 9 and subsequent releases. See JDK Release Notes for information about new features, enhancements, and removed or deprecated options for all JDK releases.
Equality, Relational, and Conditional Operators
The equality and relational operators.
The equality and relational operators determine if one operand is greater than, less than, equal to, or not equal to another operand. The majority of these operators will probably look familiar to you as well. Keep in mind that you must use " == ", not " = ", when testing if two primitive values are equal.
The following program, ComparisonDemo , tests the comparison operators:
The Conditional Operators
The && and || operators perform Conditional-AND and Conditional-OR operations on two boolean expressions. These operators exhibit "short-circuiting" behavior, which means that the second operand is evaluated only if needed.
The following program, ConditionalDemo1 , tests these operators:
Another conditional operator is ?: , which can be thought of as shorthand for an if-then-else statement (discussed in the Control Flow Statements section of this lesson). This operator is also known as the ternary operator because it uses three operands. In the following example, this operator should be read as: "If someCondition is true , assign the value of value1 to result . Otherwise, assign the value of value2 to result ."
The following program, ConditionalDemo2 , tests the ?: operator:
Because someCondition is true, this program prints "1" to the screen. Use the ?: operator instead of an if-then-else statement if it makes your code more readable; for example, when the expressions are compact and without side-effects (such as assignments).
The Type Comparison Operator instanceof
The instanceof operator compares an object to a specified type. You can use it to test if an object is an instance of a class, an instance of a subclass, or an instance of a class that implements a particular interface.
The following program, InstanceofDemo , defines a parent class (named Parent ), a simple interface (named MyInterface ), and a child class (named Child ) that inherits from the parent and implements the interface.
When using the instanceof operator, keep in mind that null is not an instance of anything.
About Oracle | Contact Us | Legal Notices | Terms of Use | Your Privacy Rights
Copyright © 1995, 2022 Oracle and/or its affiliates. All rights reserved.
Home » Java Tutorial » Java if-else
Java if-else
Summary : in this tutorial, you will learn how to use the Java if-else statement to execute a block of code if a condition is true and another code block if the condition is false.
Introduction to the Java if-else statement
The Java if-else statement evaluates a condition. If the condition is true , it executes a code block that follows the if keyword. If the condition is false , it executes another code block that follows the else keyword.
Here’s the syntax of the if-else statement:
In this syntax:
- if : This keyword marks the beginning of the if-else statement.
- (condition) : This is a condition that the if-else statement will evaluate. It can be true or false .
- {} This is the first pair of curly brace containing the code block that the if-else statement will execute if the condition is true .
- else : This else keyword introduces the alternate code block to be executed if the condition is false .
- {}: The second pair of curly braces enclose the block of code that the if-else statement will execute if the condition is false .
Java if-else statement examples
Let’s take some examples of using the Java if-else statement.
1) Simple Java if-else example
The following example shows how to use the if-else statement to display different messages based on the value of a variable temperature :
In this example, we have an integer variable temperature with a value of 25 . The if-else statement checks if the temperature is greater than 30.
Since this condition is false , the if-else statement executes the code block in the else part that displays the message "It's not too hot." .
2) Using the if-else statement to find the max of two numbers
The following uses the if-else statement to find the maximum number of two numbers:
How it works.
First, declare two integer variables x and y , and initialize their values to 10 and 20 respectively.
Second, declare the max variable that holds the maximum number.
Third, check if x is greater than y , and assign the x to the max if the condition is true. Otherwise, assign the value of y to max .
Finally, display the value of the max variable, which is 20 in this case.
Nest if-else statement
Like the if statement, you can nest an if-else statement inside an if or if-else statement.
In theory, you can have an unlimited level of nestings. But in practice, you should avoid nesting the if-else statement to make the code easier to understand.
Here’s the syntax for using nested if-else statements:
The following example illustrates how to use the nested if-else statement to find the maximum number of three numbers:
- First, define three integer variables x , y , and z and initialize their values to 10, 20, and 30 respectively.
- It checks if x is greater than y .
- If yes, it further checks if x is greater than z . If true, x is assigned to max , otherwise z is assigned to max .
- If x is not greater than y , it checks if y is greater than z . If true, y is assigned to max , otherwise z is assigned to max .
- Finally, print the value of max , which represents the maximum among x , y , and z . In this case, it will print 30, as z is the maximum value.
- Use Java if-else statement to execute a block of code if a condition is true or another block of code if the condition is false .
- Avoid nesting too many if-else statements to make your code more readable and easier to understand.
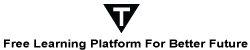
Java Tutorial
Control statements, java object class, java inheritance, java polymorphism, java abstraction, java encapsulation, java oops misc.
- Send your Feedback to [email protected]
Help Others, Please Share

Learn Latest Tutorials

Transact-SQL

Reinforcement Learning

R Programming

React Native

Python Design Patterns

Python Pillow

Python Turtle

Preparation

Verbal Ability

Interview Questions

Company Questions
Trending Technologies

Artificial Intelligence

Cloud Computing

Data Science

Machine Learning

B.Tech / MCA

Data Structures

Operating System

Computer Network

Compiler Design

Computer Organization

Discrete Mathematics

Ethical Hacking

Computer Graphics

Software Engineering

Web Technology

Cyber Security

C Programming

Control System

Data Mining

Data Warehouse

- Java One Line if Statement
- Java Howtos
Ternary Operator in Java
One line if-else statement using filter in java 8.
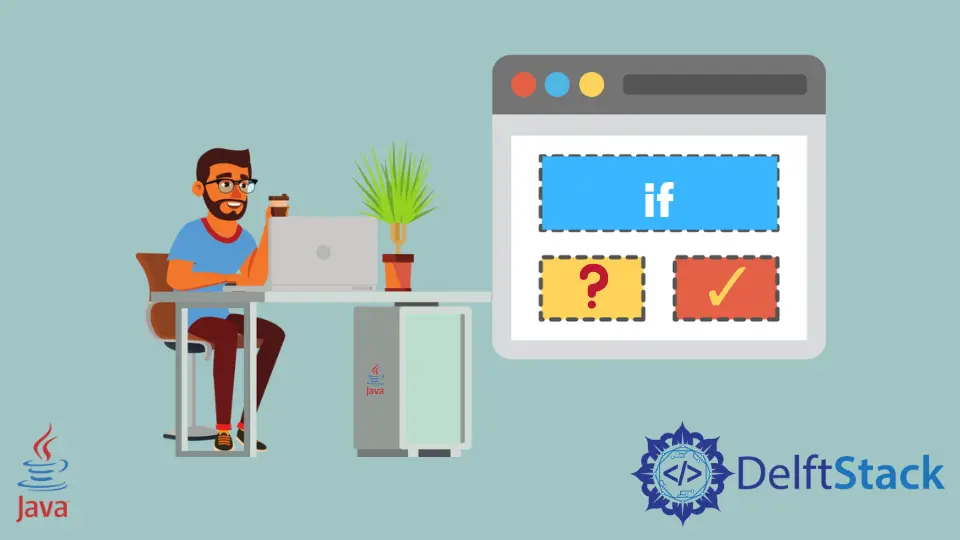
The if-else statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else block, which may not always be ideal, especially for concise and readable code.
Fortunately, Java provides a shorthand form called the ternary operator, which allows us to write a one-line if-else statement.
The ternary operator in Java, denoted as ? : , provides a compact way to represent an if-else statement. Its syntax is as follows:
Here, condition is evaluated first. If condition is true, expression1 is executed; otherwise, expression2 is executed.
Example 1: Assigning a Value Based on a Condition
This code uses the ternary operator to determine if a student has made a distinction based on their exam marks.
If marks is greater than 70 , the string Yes is assigned to the distinction variable and the output will be:
If marks is less than or equal to 70 , the string No is assigned to the distinction variable and the output will be:
Example 2: Printing a Message Conditionally
This code defines a Boolean variable isRaining with the value true and uses a ternary operator to print a message based on the value of isRaining .
If isRaining is true , it prints Bring an umbrella ; otherwise, it prints No need for an umbrella .
Example 3: Returning a Value from a Method
In this code, we’re assigning the maximum of a and b to the variable max using a ternary operator. The maximum value is then printed to the console.
You can try it yourself by replacing the values of a and b with your desired values to find the maximum between them.
Java 8 introduced streams and the filter method, which operates similarly to an if-else statement. It allows us to filter elements based on a condition.
Here’s its syntax:
Predicate is a functional interface that takes an argument and returns a Boolean. It’s often used with lambda expressions to define the condition for filtering.
Here’s a demonstration of a one-line if-else -like usage using the filter method in Java 8 streams.
As we can see, this example demonstrates a one-line usage of an if-else -like structure using the filter method. It first defines a list of words and then applies a stream to this list.
Within the map function, a lambda expression is used to check if each word starts with the letter b . If a word meets this condition, it remains unchanged; otherwise, it is replaced with the string Not available .
Finally, the resulting stream is collected back into a list using collect(Collectors.toList()) . The output shows the effect of this one-line if-else -like usage, modifying the words based on the specified condition.
Let’s see another example.
This example code also showcases the use of Java 8 streams to filter and print elements from a list based on a condition. The code begins by importing necessary packages and defining a class named Java8Streams .
Inside the main method, a list of strings, stringList , is created with elements 1 and 2 . The stream is then created from this list using the stream() method.
Next, the filter method is applied to this stream, utilizing a lambda expression as the predicate. The lambda expression checks if the string is equal to 1 .
If this condition is true, the string is allowed to pass through the filter; otherwise, it is discarded.
Finally, the forEach method is used to iterate over the filtered stream and print each element that passed the filter. It uses a method reference System.out::println to achieve this.
In summary, one-line if statements in Java offer a compact and efficient way to handle simple conditional logic. By understanding the syntax and appropriate usage, you can write concise code while maintaining readability and clarity.
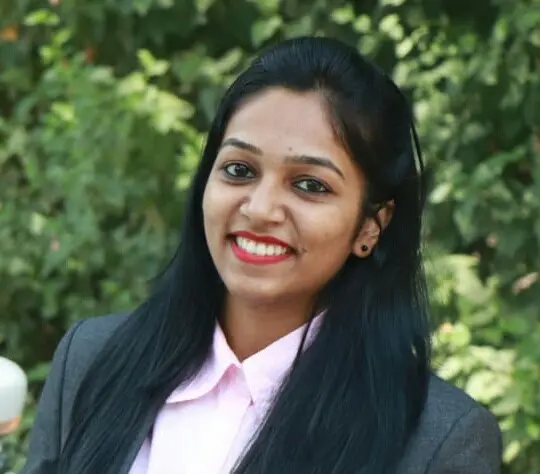
Rashmi is a professional Software Developer with hands on over varied tech stack. She has been working on Java, Springboot, Microservices, Typescript, MySQL, Graphql and more. She loves to spread knowledge via her writings. She is keen taking up new things and adopt in her career.
Related Article - Java Statement
- Difference Between break and continue Statements in Java
- The continue Statement in Java
- Java Arrays
- Java Strings
- Java Collection
- Java 8 Tutorial
- Java Multithreading
- Java Exception Handling
- Java Programs
- Java Project
- Java Collections Interview
- Java Interview Questions
- Spring Boot
Nested if in Java
- Nested if in C++
- || operator in Java
- For Loop in Java
- Loops in Java
- Break statement in Java
- Deque contains() method in Java
- Stack empty() Method in Java
- instanceof Keyword in Java
- Compare two Strings in Java
- Java Integer compare() method
- Pattern Matching for Switch in Java
- DecimalFormat equals() method in Java
- NavigableSet ceiling() method in Java
- String equals() Method in Java
- Short compare() method in Java
- BigInteger equals() Method in Java
- LongBuffer equals() method in Java
- Switch Statements in Java
- IntBuffer equals() method in Java
Generally, if condition works like yes or no type. If the condition satisfies, it executes some block of code. Otherwise, it doesn’t execute the code. Let us see the syntax of the simple if condition.
Syntax :
Nested if condition
Nested means within. Nested if condition means if-within-if. Nested if condition comes under decision-making statement in Java . There could be infinite if conditions inside an if condition. The below syntax represents the Nested if condition.
Syntax :
Note – If the inner condition satisfies then only outer if will be executed. Along with if conditions we can write else conditions also.
Example 1
Code explanation :
- In the first step, we have imported the required packages.
- In the next step, we have created a class names GFG
- In the next step, we have written the main method.
- Within the main method, we have assigned values to variables.
- Using nested if conditions, we have printed a statement.
- Here two statements are true. Hence statement was executed successfully.
Example 2 :
- Here inner if the condition is not true. Hence else part is executed.
Nested if condition comes under decision-making statement in Java. It contains several branches with an if condition inside another if condition. The syntax, code examples, and explanations of Nested-if statements are covered in detail in the above article.
Please Login to comment...
Similar reads.
- java-basics
- Java-Control-Flow
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
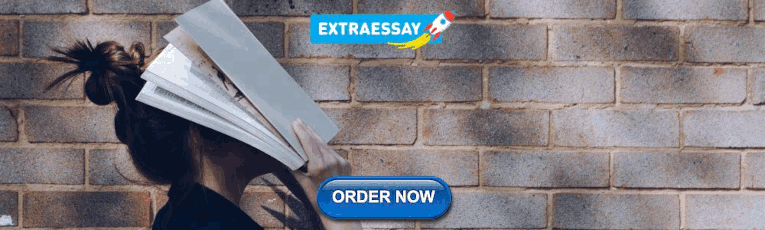
IMAGES
VIDEO
COMMENTS
Stack Overflow Public questions & answers; Stack Overflow for Teams Where developers & technologists share private knowledge with coworkers; Talent Build your employer brand ; Advertising Reach developers & technologists worldwide; Labs The future of collective knowledge sharing; About the company
Output. The number is positive. Statement outside if...else block. In the above example, we have a variable named number.Here, the test expression number > 0 checks if number is greater than 0.. Since the value of the number is 10, the test expression evaluates to true.Hence code inside the body of if is executed.. Now, change the value of the number to a negative integer.
Java has the following conditional statements: Use if to specify a block of code to be executed, if a specified condition is true. Use else to specify a block of code to be executed, if the same condition is false. Use else if to specify a new condition to test, if the first condition is false. Use switch to specify many alternative blocks of ...
Java if-else. Decision-making in Java helps to write decision-driven statements and execute a particular set of code based on certain conditions. The if statement alone tells us that if a condition is true it will execute a block of statements and if the condition is false it won't. In this article, we will learn about Java if-else.
The if-else statement is the most basic of all control structures, and it's likely also the most common decision-making statement in programming. It allows us to execute a certain code section only if a specific condition is met. 2. Syntax of If-Else. The if statement always needs a boolean expression as its parameter.
The if-then Statement. The if-then statement is the most basic of all the control flow statements. It tells your program to execute a certain section of code only if a particular test evaluates to true.For example, the Bicycle class could allow the brakes to decrease the bicycle's speed only if the bicycle is already in motion. One possible implementation of the applyBrakes method could be as ...
Working of if statement: Control falls into the if block. The flow jumps to Condition. Condition is tested. If Condition yields true, goto Step 4. If Condition yields false, goto Step 5. The if-block or the body inside the if is executed. Flow steps out of the if block. Flowchart if statement:
We will see how to write such type of conditions in the java program using control statements. In this tutorial, we will see four types of control statements that you can use in java programs based on the requirement: In this tutorial we will cover following conditional statements: a) if statement b) nested if statement c) if-else statement
Java if-else Statements. If else statements in Java is also used to control the program flow based on some condition, only the difference is: it's used to execute some statement code block if the expression is evaluated to true, otherwise executes else statement code block. The basic format of if else statement is:
Because someCondition is true, this program prints "1" to the screen. Use the ?: operator instead of an if-then-else statement if it makes your code more readable; for example, when the expressions are compact and without side-effects (such as assignments).. The Type Comparison Operator instanceof. The instanceof operator compares an object to a specified type.
Summary: in this tutorial, you will learn how to use the Java if-else statement to execute a block of code if a condition is true and another code block if the condition is false.. Introduction to the Java if-else statement. The Java if-else statement evaluates a condition. If the condition is true, it executes a code block that follows the if keyword. If the condition is false, it executes ...
These are used to cause the flow of execution to advance and branch based on changes to the state of a program. Java's Selection statements: if. if-else. nested-if. if-else-if. switch-case. jump - break, continue, return. 1. if: if statement is the most simple decision-making statement.
JLS 16 Definite Assignment; JLS 14.4.2 Scope of Local Variable Declarations; JLS 14.9.2 The if-then-else Statement; Math.abs(int) Wikipedia/Two's complement (read: the most negative number) Attempt #1: Declaring before the if. ... JAVA IF Else variables. Hot Network Questions
Java If-else Statement. The Java if statement is used to test the condition. It checks boolean condition: true or false. There are various types of if statement in Java. if statement; if-else statement; if-else-if ladder; nested if statement; Java if Statement. The Java if statement tests the condition. It executes the if block if condition is ...
Write a Java program to solve quadratic equations (use if, else if and else). Test Data Input a: 1 Input b: 5 Input c: 1 Expected Output: The roots are -0.20871215252208009 and -4.7912878474779195 Click me to see the solution. 3. Write a Java program that takes three numbers from the user and prints the greatest number. Test Data Input the 1st ...
Ternary Operator in Java One Line if-else Statement Using filter in Java 8 ; Conclusion The if-else statement in Java is a fundamental construct used to conditionally execute blocks of code based on certain conditions. However, it often requires multiple lines to define a simple if-else block, which may not always be ideal, especially for concise and readable code.
Assignment in a conditional statement is valid in javascript, because your just asking "if assignment is valid, do something which possibly includes the result of the assignment". But indeed, assigning before the conditional is also valid, not too verbose, and more commonly used. - okdewit.
Note: The compound assignment operator in Java performs implicit type casting. Let's consider a scenario where x is an int variable with a value of 5. int x = 5; If you want to add the double value 4.5 to the integer variable x and print its value, there are two methods to achieve this: Method 1: x = x + 4.5. Method 2: x += 4.5.
The reason it is not compiling is because of scoping. The variable you are declaring is inside an if statement. That gives its scope, ability to access it, to only within the if statement. In order to reference it outside the if statement, you must declare it within the scope you want to access it. Then you can assign values to it like as follows, activityFactor = 1.0;, within the if else ...
Assignment and else if statements. Ask Question Asked 11 years, 6 months ago. ... import java.awt.*; import java.awt.event.*; import javax.swing.*; class PizzaFrame extends JFrame { // The following components are defined (but not created). private JButton newButton, exitButton; private JLabel priceLabel, grandTotalLabel; private JCheckBox ...
Here inner if the condition is not true. Hence else part is executed. Nested if condition comes under decision-making statement in Java. It contains several branches with an if condition inside another if condition. The syntax, code examples, and explanations of Nested-if statements are covered in detail in the above article.