
- C Programming Tutorial
- C - Overview
- C - Features
- C - History
- C - Environment Setup
- C - Program Structure
- C - Hello World
- C - Compilation Process
- C - Comments
- C - Keywords
- C - Identifiers
- C - User Input
- C - Basic Syntax
- C - Data Types
- C - Variables
- C - Integer Promotions
- C - Type Conversion
- C - Booleans
- C - Constants
- C - Literals
- C - Escape sequences
- C - Format Specifiers
- C - Storage Classes
- C - Operators
- C - Arithmetic Operators
- C - Relational Operators
- C - Logical Operators
- C - Bitwise Operators
- C - Assignment Operators
- C - Unary Operators
- C - Increment and Decrement Operators
- C - Ternary Operator
- C - sizeof Operator
- C - Operator Precedence
- C - Misc Operators
- C - Decision Making
- C - if statement
- C - if...else statement
- C - nested if statements
- C - switch statement
- C - nested switch statements
- C - While loop
- C - For loop
- C - Do...while loop
- C - Nested loop
- C - Infinite loop
- C - Break Statement
- C - Continue Statement
- C - goto Statement
- C - Functions
- C - Main Functions
- C - Function call by Value
- C - Function call by reference
- C - Nested Functions
- C - Variadic Functions
- C - User-Defined Functions
- C - Callback Function
- C - Return Statement
- C - Recursion
- C - Scope Rules
- C - Static Variables
- C - Global Variables
- C - Properties of Array
- C - Multi-Dimensional Arrays
- C - Passing Arrays to Function
- C - Return Array from Function
- C - Variable Length Arrays
- C - Pointers
- C - Pointers and Arrays
- C - Applications of Pointers
- C - Pointer Arithmetics
- C - Array of Pointers
- C - Passing Pointers to Functions
- C - Strings
- C - Array of Strings
- C - Structures
- C - Structures and Functions
- C - Arrays of Structures
- C - Pointers to Structures
- C - Self-Referential Structures
- C - Nested Structures
- C - Bit Fields
- C - Typedef
- C - Input & Output
- C - File I/O
- C - Preprocessors
- C - Header Files
- C - Type Casting
- C - Error Handling
- C - Variable Arguments
- C - Memory Management
- C - Command Line Arguments
- C Programming Resources
- C - Questions & Answers
- C - Quick Guide
- C - Useful Resources
- C - Discussion
- Selected Reading
- UPSC IAS Exams Notes
- Developer's Best Practices
- Questions and Answers
- Effective Resume Writing
- HR Interview Questions
- Computer Glossary
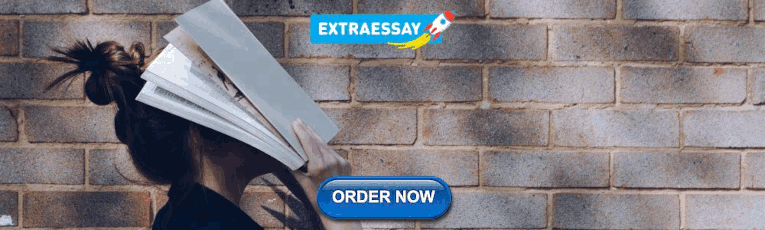
Assignment Operators in C
In C language, the assignment operator stores a certain value in an already declared variable. A variable in C can be assigned the value in the form of a literal, another variable, or an expression.
The value to be assigned forms the right-hand operand, whereas the variable to be assigned should be the operand to the left of the " = " symbol, which is defined as a simple assignment operator in C.
In addition, C has several augmented assignment operators.
The following table lists the assignment operators supported by the C language −
Simple Assignment Operator (=)
The = operator is one of the most frequently used operators in C. As per the ANSI C standard, all the variables must be declared in the beginning. Variable declaration after the first processing statement is not allowed.
You can declare a variable to be assigned a value later in the code, or you can initialize it at the time of declaration.
You can use a literal, another variable, or an expression in the assignment statement.
Once a variable of a certain type is declared, it cannot be assigned a value of any other type. In such a case the C compiler reports a type mismatch error.
In C, the expressions that refer to a memory location are called "lvalue" expressions. A lvalue may appear as either the left-hand or right-hand side of an assignment.
On the other hand, the term rvalue refers to a data value that is stored at some address in memory. A rvalue is an expression that cannot have a value assigned to it which means an rvalue may appear on the right-hand side but not on the left-hand side of an assignment.
Variables are lvalues and so they may appear on the left-hand side of an assignment. Numeric literals are rvalues and so they may not be assigned and cannot appear on the left-hand side. Take a look at the following valid and invalid statements −
Augmented Assignment Operators
In addition to the = operator, C allows you to combine arithmetic and bitwise operators with the = symbol to form augmented or compound assignment operator. The augmented operators offer a convenient shortcut for combining arithmetic or bitwise operation with assignment.
For example, the expression "a += b" has the same effect of performing "a + b" first and then assigning the result back to the variable "a".
Run the code and check its output −
Similarly, the expression "a <<= b" has the same effect of performing "a << b" first and then assigning the result back to the variable "a".
Here is a C program that demonstrates the use of assignment operators in C −
When you compile and execute the above program, it will produce the following result −
To Continue Learning Please Login
This browser is no longer supported.
Upgrade to Microsoft Edge to take advantage of the latest features, security updates, and technical support.
C Assignment Operators
- 6 contributors
An assignment operation assigns the value of the right-hand operand to the storage location named by the left-hand operand. Therefore, the left-hand operand of an assignment operation must be a modifiable l-value. After the assignment, an assignment expression has the value of the left operand but isn't an l-value.
assignment-expression : conditional-expression unary-expression assignment-operator assignment-expression
assignment-operator : one of = *= /= %= += -= <<= >>= &= ^= |=
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators:
In assignment, the type of the right-hand value is converted to the type of the left-hand value, and the value is stored in the left operand after the assignment has taken place. The left operand must not be an array, a function, or a constant. The specific conversion path, which depends on the two types, is outlined in detail in Type Conversions .
- Assignment Operators
Was this page helpful?
Coming soon: Throughout 2024 we will be phasing out GitHub Issues as the feedback mechanism for content and replacing it with a new feedback system. For more information see: https://aka.ms/ContentUserFeedback .
Submit and view feedback for
Additional resources
C Programming Tutorial
- Assignment Operator in C
Last updated on July 27, 2020
We have already used the assignment operator ( = ) several times before. Let's discuss it here in detail. The assignment operator ( = ) is used to assign a value to the variable. Its general format is as follows:
The operand on the left side of the assignment operator must be a variable and operand on the right-hand side must be a constant, variable or expression. Here are some examples:
The precedence of the assignment operator is lower than all the operators we have discussed so far and it associates from right to left.
We can also assign the same value to multiple variables at once.
here x , y and z are initialized to 100 .
Since the associativity of the assignment operator ( = ) is from right to left. The above expression is equivalent to the following:
Note that expressions like:
are called assignment expression. If we put a semicolon( ; ) at the end of the expression like this:
then the assignment expression becomes assignment statement.
Compound Assignment Operator #
Assignment operations that use the old value of a variable to compute its new value are called Compound Assignment.
Consider the following two statements:
Here the second statement adds 5 to the existing value of x . This value is then assigned back to x . Now, the new value of x is 105 .
To handle such operations more succinctly, C provides a special operator called Compound Assignment operator.
The general format of compound assignment operator is as follows:
where op can be any of the arithmetic operators ( + , - , * , / , % ). The above statement is functionally equivalent to the following:
Note : In addition to arithmetic operators, op can also be >> (right shift), << (left shift), | (Bitwise OR), & (Bitwise AND), ^ (Bitwise XOR). We haven't discussed these operators yet.
After evaluating the expression, the op operator is then applied to the result of the expression and the current value of the variable (on the RHS). The result of this operation is then assigned back to the variable (on the LHS). Let's take some examples: The statement:
is equivalent to x = x + 5; or x = x + (5); .
Similarly, the statement:
is equivalent to x = x * 2; or x = x * (2); .
Since, expression on the right side of op operator is evaluated first, the statement:
is equivalent to x = x * (y + 1) .
The precedence of compound assignment operators are same and they associate from right to left (see the precedence table ).
The following table lists some Compound assignment operators:
The following program demonstrates Compound assignment operators in action:
Expected Output:
Load Comments
- Intro to C Programming
- Installing Code Blocks
- Creating and Running The First C Program
- Basic Elements of a C Program
- Keywords and Identifiers
- Data Types in C
- Constants in C
- Variables in C
- Input and Output in C
- Formatted Input and Output in C
- Arithmetic Operators in C
- Operator Precedence and Associativity in C
- Increment and Decrement Operators in C
- Relational Operators in C
- Logical Operators in C
- Conditional Operator, Comma operator and sizeof() operator in C
- Implicit Type Conversion in C
- Explicit Type Conversion in C
- if-else statements in C
- The while loop in C
- The do while loop in C
- The for loop in C
- The Infinite Loop in C
- The break and continue statement in C
- The Switch statement in C
- Function basics in C
- The return statement in C
- Actual and Formal arguments in C
- Local, Global and Static variables in C
- Recursive Function in C
- One dimensional Array in C
- One Dimensional Array and Function in C
- Two Dimensional Array in C
- Pointer Basics in C
- Pointer Arithmetic in C
- Pointers and 1-D arrays
- Pointers and 2-D arrays
- Call by Value and Call by Reference in C
- Returning more than one value from function in C
- Returning a Pointer from a Function in C
- Passing 1-D Array to a Function in C
- Passing 2-D Array to a Function in C
- Array of Pointers in C
- Void Pointers in C
- The malloc() Function in C
- The calloc() Function in C
- The realloc() Function in C
- String Basics in C
- The strlen() Function in C
- The strcmp() Function in C
- The strcpy() Function in C
- The strcat() Function in C
- Character Array and Character Pointer in C
- Array of Strings in C
- Array of Pointers to Strings in C
- The sprintf() Function in C
- The sscanf() Function in C
- Structure Basics in C
- Array of Structures in C
- Array as Member of Structure in C
- Nested Structures in C
- Pointer to a Structure in C
- Pointers as Structure Member in C
- Structures and Functions in C
- Union Basics in C
- typedef statement in C
- Basics of File Handling in C
- fputc() Function in C
- fgetc() Function in C
- fputs() Function in C
- fgets() Function in C
- fprintf() Function in C
- fscanf() Function in C
- fwrite() Function in C
- fread() Function in C
Recent Posts
- Machine Learning Experts You Should Be Following Online
- 4 Ways to Prepare for the AP Computer Science A Exam
- Finance Assignment Online Help for the Busy and Tired Students: Get Help from Experts
- Top 9 Machine Learning Algorithms for Data Scientists
- Data Science Learning Path or Steps to become a data scientist Final
- Enable Edit Button in Shutter In Linux Mint 19 and Ubuntu 18.04
- Python 3 time module
- Pygments Tutorial
- How to use Virtualenv?
- Installing MySQL (Windows, Linux and Mac)
- What is if __name__ == '__main__' in Python ?
- Installing GoAccess (A Real-time web log analyzer)
- Installing Isso
PrepBytes Blog
ONE-STOP RESOURCE FOR EVERYTHING RELATED TO CODING
Sign in to your account
Forgot your password?
Login via OTP
We will send you an one time password on your mobile number
An OTP has been sent to your mobile number please verify it below
Register with PrepBytes
Assignment operator in c.
Last Updated on June 23, 2023 by Prepbytes
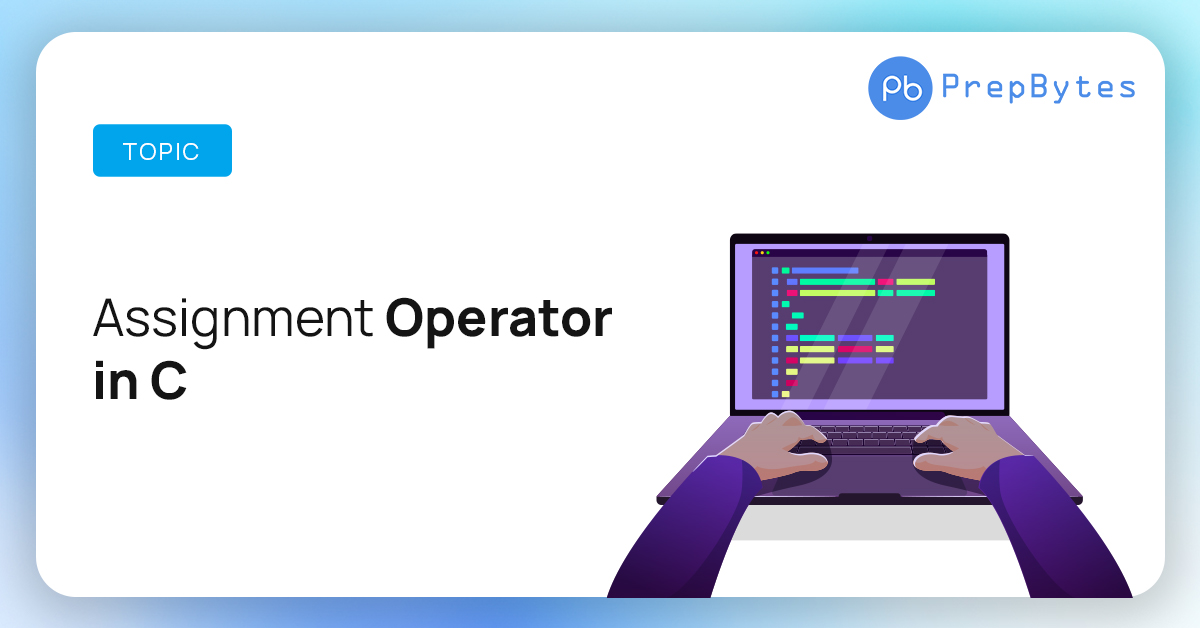
This type of operator is employed for transforming and assigning values to variables within an operation. In an assignment operation, the right side represents a value, while the left side corresponds to a variable. It is essential that the value on the right side has the same data type as the variable on the left side. If this requirement is not fulfilled, the compiler will issue an error.
What is Assignment Operator in C language?
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side. This fundamental operation allows developers to store and manipulate data effectively throughout their programs.
Example of Assignment Operator in C
For example, consider the following line of code:
Types of Assignment Operators in C
Here is a list of the assignment operators that you can find in the C language:
Simple assignment operator (=): This is the basic assignment operator, which assigns the value on the right-hand side to the variable on the left-hand side.
Addition assignment operator (+=): This operator adds the value on the right-hand side to the variable on the left-hand side and assigns the result back to the variable.
x += 3; // Equivalent to x = x + 3; (adds 3 to the current value of "x" and assigns the result back to "x")
Subtraction assignment operator (-=): This operator subtracts the value on the right-hand side from the variable on the left-hand side and assigns the result back to the variable.
x -= 4; // Equivalent to x = x – 4; (subtracts 4 from the current value of "x" and assigns the result back to "x")
* Multiplication assignment operator ( =):** This operator multiplies the value on the right-hand side with the variable on the left-hand side and assigns the result back to the variable.
x = 2; // Equivalent to x = x 2; (multiplies the current value of "x" by 2 and assigns the result back to "x")
Division assignment operator (/=): This operator divides the variable on the left-hand side by the value on the right-hand side and assigns the result back to the variable.
x /= 2; // Equivalent to x = x / 2; (divides the current value of "x" by 2 and assigns the result back to "x")
Bitwise AND assignment (&=): The bitwise AND assignment operator "&=" performs a bitwise AND operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x &= 3; // Binary: 0011 // After bitwise AND assignment: x = 1 (Binary: 0001)
Bitwise OR assignment (|=): The bitwise OR assignment operator "|=" performs a bitwise OR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x |= 3; // Binary: 0011 // After bitwise OR assignment: x = 7 (Binary: 0111)
Bitwise XOR assignment (^=): The bitwise XOR assignment operator "^=" performs a bitwise XOR operation between the value on the left-hand side and the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x ^= 3; // Binary: 0011 // After bitwise XOR assignment: x = 6 (Binary: 0110)
Left shift assignment (<<=): The left shift assignment operator "<<=" shifts the bits of the value on the left-hand side to the left by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x <<= 2; // Binary: 010100 (Shifted left by 2 positions) // After left shift assignment: x = 20 (Binary: 10100)
Right shift assignment (>>=): The right shift assignment operator ">>=" shifts the bits of the value on the left-hand side to the right by the number of positions specified by the value on the right-hand side. It then assigns the result back to the left-hand side variable.
x >>= 2; // Binary: 101 (Shifted right by 2 positions) // After right shift assignment: x = 5 (Binary: 101)
Conclusion The assignment operator in C, denoted by the equals sign (=), is used to assign a value to a variable. It is a fundamental operation that allows programmers to store data in variables for further use in their code. In addition to the simple assignment operator, C provides compound assignment operators that combine arithmetic or bitwise operations with assignment, allowing for concise and efficient code.
FAQs related to Assignment Operator in C
Q1. Can I assign a value of one data type to a variable of another data type? In most cases, assigning a value of one data type to a variable of another data type will result in a warning or error from the compiler. It is generally recommended to assign values of compatible data types to variables.
Q2. What is the difference between the assignment operator (=) and the comparison operator (==)? The assignment operator (=) is used to assign a value to a variable, while the comparison operator (==) is used to check if two values are equal. It is important not to confuse these two operators.
Q3. Can I use multiple assignment operators in a single statement? No, it is not possible to use multiple assignment operators in a single statement. Each assignment operator should be used separately for assigning values to different variables.
Q4. Are there any limitations on the right-hand side value of the assignment operator? The right-hand side value of the assignment operator should be compatible with the data type of the left-hand side variable. If the data types are not compatible, it may lead to unexpected behavior or compiler errors.
Q5. Can I assign the result of an expression to a variable using the assignment operator? Yes, it is possible to assign the result of an expression to a variable using the assignment operator. For example, x = y + z; assigns the sum of y and z to the variable x.
Q6. What happens if I assign a value to an uninitialized variable? Assigning a value to an uninitialized variable will initialize it with the assigned value. However, it is considered good practice to explicitly initialize variables before using them to avoid potential bugs or unintended behavior.
Leave a Reply Cancel reply
Your email address will not be published. Required fields are marked *
Save my name, email, and website in this browser for the next time I comment.
- Linked List
- Segment Tree
- Backtracking
- Dynamic Programming
- Greedy Algorithm
- Operating System
- Company Placement
- Interview Tips
- General Interview Questions
- Data Structure
- Other Topics
- Computational Geometry
- Game Theory
Related Post
Null character in c, ackermann function in c, median of two sorted arrays of different size in c, number is palindrome or not in c, implementation of queue using linked list in c, c program to replace a substring in a string.
Operators in C
Share this article
Postfix Operators
Frequently asked questions about operators in c.
Continuing on from my last article on the fundamentals of C , we’re now going to look at operators. An expression is a sequence of operators and operands that computes a value. An operator in C is a symbol that tells the computer to perform mathematical or logical manipulation on data. The data items that operators act upon are called operands. The different operators supported by C are: 1. Postfix Operators 2. Unary Operators 3. Cast Operators 4. Multiplicative Operators 5. Additive Operators 6. Bitwise Shift Operators 7. Relational Operators 8.Equality Operators 9.Bitwise AND Operator 10.Bitwise Exclusive OR Operator 11.Bitwise Inclusive OR Operator 12. Logical AND Operator 13. Logical OR Operator 14.Conditional Operator 15. Assignment Operators 16. Comma Operators
1. Array Subscripts
2. function calls, what is the difference between unary and binary operators in c.
Unary operators in C are those that require only one operand to perform their operation. Examples include increment (++), decrement (–), and the logical NOT (!) operator. On the other hand, binary operators require two operands. Examples include arithmetic operators like addition (+), subtraction (-), multiplication (*), and division (/), and relational operators like less than (<), greater than (>), etc. The main difference between the two lies in the number of operands they require to perform their operation.
How does the modulus operator work in C?
The modulus operator (%) in C is a mathematical operator that returns the remainder of a division operation. For example, if you have an expression like ’10 % 3′, the result would be 1, because when 10 is divided by 3, the remainder is 1. This operator is particularly useful when you need to determine if a number is even or odd, as even numbers will have a modulus of 0 when divided by 2.
What is the purpose of the assignment operator in C?
The assignment operator (=) in C is used to assign the value of the right-hand operand to the left-hand operand. For example, in the statement ‘int a = 10;’, the assignment operator is used to assign the value 10 to the variable ‘a’. It’s important to note that the assignment operator is different from the equality operator (==), which is used to compare two values.
How do logical operators work in C?
Logical operators in C are used to perform logical operations, such as AND, OR, and NOT. The AND operator (&&) returns true if both operands are true. The OR operator (||) returns true if either or both operands are true. The NOT operator (!) returns true if the operand is false, and vice versa. These operators are often used in conditional statements to combine or invert conditions.
What is the precedence of operators in C?
Operator precedence in C determines the order in which operations are performed in an expression. Operators with higher precedence are performed first. For example, multiplication and division operators have higher precedence than addition and subtraction operators. If an expression contains operators with the same precedence, they are performed from left to right. Parentheses can be used to change the order of operations in an expression.
What are bitwise operators in C?
Bitwise operators in C are used to perform operations on individual bits of a number. They include bitwise AND (&), OR (|), XOR (^), NOT (~), left shift (<<), and right shift (>>). These operators are often used in low-level programming, such as writing device drivers or embedded systems.
How does the ternary operator work in C?
The ternary operator in C is a shorthand way of writing an if-else statement. It’s represented as ‘? :’. The syntax is ‘condition ? expression_if_true : expression_if_false’. If the condition is true, the expression after the ‘?’ is executed. If the condition is false, the expression after the ‘:’ is executed.
What is the difference between the equality operator and the assignment operator in C?
The equality operator (==) in C is used to compare two values and returns true if they are equal and false if they are not. On the other hand, the assignment operator (=) is used to assign the value of the right-hand operand to the left-hand operand. It’s important not to confuse the two, as using the assignment operator when you mean to use the equality operator is a common mistake.
How do increment and decrement operators work in C?
The increment operator (++) and decrement operator (–) in C are used to increase or decrease the value of a variable by 1, respectively. They can be used in both postfix (i++) and prefix (++i) forms. In the postfix form, the value is first used in the expression and then incremented or decremented. In the prefix form, the value is first incremented or decremented and then used in the expression.
What are relational operators in C?
Relational operators in C are used to compare two values. They include less than (<), greater than (>), less than or equal to (<=), greater than or equal to (>=), equal to (==), and not equal to (!=). These operators return true if the comparison is true and false if it is not. They are often used in conditional statements to make decisions based on the relationship between two values.
My name is Surabhi Saxena, I am a graduate in computer applications. I have been a college topper, and have been awarded during college by the education minister for excellence in academics. I love programming and Linux. I have a blog on Linux at www.myblogonunix.wordpress.com.
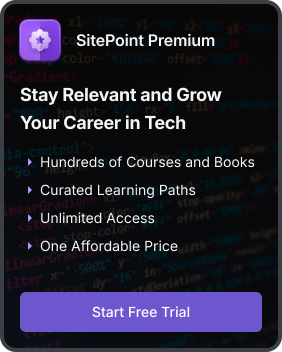
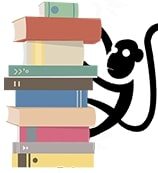
Assignment Operators in C
For complete youtube video: click here.
In this class, we will try to understand Assignment Operators in C.
The complete class on arithmetic operators .
There are two types of assignment operators.
- Simple Assignment Operator
- Compound Assignment Operator
The image below shows the classification of assignment operators.

Simple Assignment Operators
The simple operator ” = ” is used to store a constant value, the value of another variable or the result of expression evaluation into a variable.
For example, a = 15, b = c, or c = 20 + 40.
A critical point to understand is the assignment operator requires an Lvalue as its left operand.
An Lvalue represents an object stored in computer memory, not a constant or computation result.
An object stored in the computer memory means a variable.
The left operand of the variable should always be a variable.
It should not be an expression or a constant.
For example, 15 = b, a + b = 23, such a left operand use is not allowed.
Compound Operators
The other type of assignment operators is the compound assignment operators.
C supports a lot of compound assignment operators.
In this class, we will discuss only a few of them. [ +=, -=, *=, /=, %= ]
The compound assignment operator uses the old value of the variable to compute the new value.
For example, a += 2 is how we use the compound assignment operator.
Here a and 2 are operands, and += is a compound assignment operator.
+= means the old value of a is added with 2, and the new value is stored in the a.
a += 2 is a short form of a = a + 2 expression.
If the value of a is initialized to 12. [a = 12]
a += 2 or a = a + 2 results in 14.
The new value 14 will be stored in a.
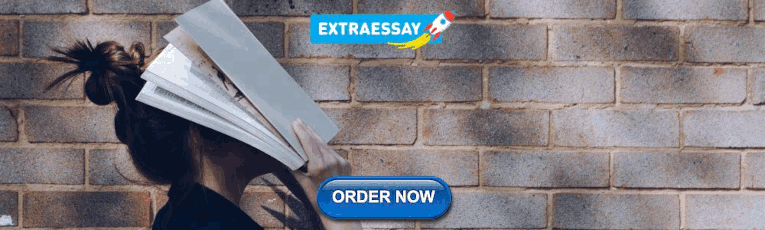
cppreference.com
Assignment operators.
Assignment operators modify the value of the object.
[ edit ] Definitions
Copy assignment replaces the contents of the object a with a copy of the contents of b ( b is not modified). For class types, this is performed in a special member function, described in copy assignment operator .
For non-class types, copy and move assignment are indistinguishable and are referred to as direct assignment .
Compound assignment replace the contents of the object a with the result of a binary operation between the previous value of a and the value of b .
[ edit ] Assignment operator syntax
The assignment expressions have the form
- ↑ target-expr must have higher precedence than an assignment expression.
- ↑ new-value cannot be a comma expression, because its precedence is lower.
[ edit ] Built-in simple assignment operator
For the built-in simple assignment, the object referred to by target-expr is modified by replacing its value with the result of new-value . target-expr must be a modifiable lvalue.
The result of a built-in simple assignment is an lvalue of the type of target-expr , referring to target-expr . If target-expr is a bit-field , the result is also a bit-field.
[ edit ] Assignment from an expression
If new-value is an expression, it is implicitly converted to the cv-unqualified type of target-expr . When target-expr is a bit-field that cannot represent the value of the expression, the resulting value of the bit-field is implementation-defined.
If target-expr and new-value identify overlapping objects, the behavior is undefined (unless the overlap is exact and the type is the same).
In overload resolution against user-defined operators , for every type T , the following function signatures participate in overload resolution:
For every enumeration or pointer to member type T , optionally volatile-qualified, the following function signature participates in overload resolution:
For every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signature participates in overload resolution:
[ edit ] Built-in compound assignment operator
The behavior of every built-in compound-assignment expression target-expr op = new-value is exactly the same as the behavior of the expression target-expr = target-expr op new-value , except that target-expr is evaluated only once.
The requirements on target-expr and new-value of built-in simple assignment operators also apply. Furthermore:
- For + = and - = , the type of target-expr must be an arithmetic type or a pointer to a (possibly cv-qualified) completely-defined object type .
- For all other compound assignment operators, the type of target-expr must be an arithmetic type.
In overload resolution against user-defined operators , for every pair A1 and A2 , where A1 is an arithmetic type (optionally volatile-qualified) and A2 is a promoted arithmetic type, the following function signatures participate in overload resolution:
For every pair I1 and I2 , where I1 is an integral type (optionally volatile-qualified) and I2 is a promoted integral type, the following function signatures participate in overload resolution:
For every optionally cv-qualified object type T , the following function signatures participate in overload resolution:
[ edit ] Example
Possible output:
[ edit ] Defect reports
The following behavior-changing defect reports were applied retroactively to previously published C++ standards.
[ edit ] See also
Operator precedence
Operator overloading
- Recent changes
- Offline version
- What links here
- Related changes
- Upload file
- Special pages
- Printable version
- Permanent link
- Page information
- In other languages
- This page was last modified on 25 January 2024, at 22:41.
- This page has been accessed 410,142 times.
- Privacy policy
- About cppreference.com
- Disclaimers

- Windows Programming
- UNIX/Linux Programming
- General C++ Programming
- copy assignment operator - arrays
copy assignment operator - arrays

- C++ Data Types
- C++ Input/Output
- C++ Pointers
- C++ Interview Questions
- C++ Programs
- C++ Cheatsheet
- C++ Projects
- C++ Exception Handling
- C++ Memory Management
Assignment Operators In C++
- Move Assignment Operator in C++ 11
- JavaScript Assignment Operators
- Assignment Operators in Programming
- Is assignment operator inherited?
- Solidity - Assignment Operators
- Augmented Assignment Operators in Python
- bitset operator[] in C++ STL
- C++ Assignment Operator Overloading
- Self assignment check in assignment operator
- Copy Constructor vs Assignment Operator in C++
- Operators in C++
- C++ Arithmetic Operators
- Bitwise Operators in C++
- Casting Operators in C++
- Assignment Operators in C
- Assignment Operators in Python
- Compound assignment operators in Java
- Arithmetic Operators in C
- Operators in C
In C++, the assignment operator forms the backbone of many algorithms and computational processes by performing a simple operation like assigning a value to a variable. It is denoted by equal sign ( = ) and provides one of the most basic operations in any programming language that is used to assign some value to the variables in C++ or in other words, it is used to store some kind of information.
The right-hand side value will be assigned to the variable on the left-hand side. The variable and the value should be of the same data type.
The value can be a literal or another variable of the same data type.
Compound Assignment Operators
In C++, the assignment operator can be combined into a single operator with some other operators to perform a combination of two operations in one single statement. These operators are called Compound Assignment Operators. There are 10 compound assignment operators in C++:
- Addition Assignment Operator ( += )
- Subtraction Assignment Operator ( -= )
- Multiplication Assignment Operator ( *= )
- Division Assignment Operator ( /= )
- Modulus Assignment Operator ( %= )
- Bitwise AND Assignment Operator ( &= )
- Bitwise OR Assignment Operator ( |= )
- Bitwise XOR Assignment Operator ( ^= )
- Left Shift Assignment Operator ( <<= )
- Right Shift Assignment Operator ( >>= )
Lets see each of them in detail.
1. Addition Assignment Operator (+=)
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way.
This above expression is equivalent to the expression:
2. Subtraction Assignment Operator (-=)
The subtraction assignment operator (-=) in C++ enables you to update the value of the variable by subtracting another value from it. This operator is especially useful when you need to perform subtraction and store the result back in the same variable.
3. Multiplication Assignment Operator (*=)
In C++, the multiplication assignment operator (*=) is used to update the value of the variable by multiplying it with another value.
4. Division Assignment Operator (/=)
The division assignment operator divides the variable on the left by the value on the right and assigns the result to the variable on the left.
5. Modulus Assignment Operator (%=)
The modulus assignment operator calculates the remainder when the variable on the left is divided by the value or variable on the right and assigns the result to the variable on the left.
6. Bitwise AND Assignment Operator (&=)
This operator performs a bitwise AND between the variable on the left and the value on the right and assigns the result to the variable on the left.
7. Bitwise OR Assignment Operator (|=)
The bitwise OR assignment operator performs a bitwise OR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
8. Bitwise XOR Assignment Operator (^=)
The bitwise XOR assignment operator performs a bitwise XOR between the variable on the left and the value or variable on the right and assigns the result to the variable on the left.
9. Left Shift Assignment Operator (<<=)
The left shift assignment operator shifts the bits of the variable on the left to left by the number of positions specified on the right and assigns the result to the variable on the left.
10. Right Shift Assignment Operator (>>=)
The right shift assignment operator shifts the bits of the variable on the left to the right by a number of positions specified on the right and assigns the result to the variable on the left.
Also, it is important to note that all of the above operators can be overloaded for custom operations with user-defined data types to perform the operations we want.
Please Login to comment...
Similar reads.
- Geeks Premier League 2023
- Geeks Premier League
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
Navigation Menu
Search code, repositories, users, issues, pull requests..., provide feedback.
We read every piece of feedback, and take your input very seriously.
Saved searches
Use saved searches to filter your results more quickly.
To see all available qualifiers, see our documentation .
- Notifications
Nondestructive assignment using = with { } #8114
dmchurch May 12, 2024
Beta Was this translation helpful? Give feedback.
Replies: 2 comments · 3 replies
Colejohnson66 may 12, 2024.
{{editor}}'s edit
Dmchurch may 12, 2024 author, iam3yal may 13, 2024, dmchurch may 13, 2024 author.
- Numbered list
- Unordered list
- Attach files
Select a reply
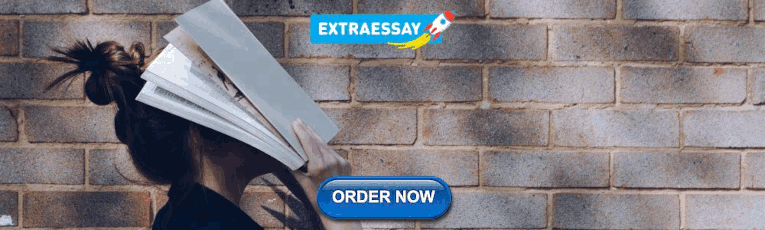
IMAGES
VIDEO
COMMENTS
a = b; } For an "assignable" array, you can use the array container-like class found in Boost ( boost::array ), C++ TR1 ( std::tr1::array ), or C++0x ( std::array ). It is actually a class that contains an array; it can be copied and it provides many of the benefits of the Standard Library containers plus the performance characteristics of an ...
The default assignment semantics are specified in clause 28 of § 12.8 [class.copy]. Specifically, the object's data members are assigned one by one. In the case of an array, this the array's elements are assigned one by one. The implicitly-defined copy/move assignment operator for a non-union class X performs memberwise copy- /move assignment ...
We can update the value of an element at the given index i in a similar way to accessing an element by using the array subscript operator [ ] and assignment operator =. array_name [i] = new_value; C Array Traversal. Traversal is the process in which we visit every element of the data structure. For C array traversal, we use loops to iterate ...
1. "=": This is the simplest assignment operator. This operator is used to assign the value on the right to the variable on the left. Example: a = 10; b = 20; ch = 'y'; 2. "+=": This operator is combination of '+' and '=' operators. This operator first adds the current value of the variable on left to the value on the right and ...
Assignment operators are used in programming to assign values to variables. We use an assignment operator to store and update data within a program. They enable programmers to store data in variables and manipulate that data. The most common assignment operator is the equals sign (=), which assigns the value on the right side of the operator to ...
Simple assignment operator. Assigns values from right side operands to left side operand. C = A + B will assign the value of A + B to C. +=. Add AND assignment operator. It adds the right operand to the left operand and assign the result to the left operand. C += A is equivalent to C = C + A. -=.
Assignment performs implicit conversion from the value of rhs to the type of lhs and then replaces the value in the object designated by lhs with the converted value of rhs . Assignment also returns the same value as what was stored in lhs (so that expressions such as a = b = c are possible). The value category of the assignment operator is non ...
The assignment operators in C can both transform and assign values in a single operation. C provides the following assignment operators: Operator Operation Performed = ... The left operand must not be an array, a function, or a constant. The specific conversion path, which depends on the two types, is outlined in detail in Type Conversions.
The Assignment operators in C are some of the Programming operators that are useful for assigning the values to the declared variables. Equals (=) operator is the most commonly used assignment operator. For example: int i = 10; The below table displays all the assignment operators present in C Programming with an example. C Assignment Operators.
Let's discuss it here in detail. The assignment operator ( = ) is used to assign a value to the variable. Its general format is as follows: variable = right_side. The operand on the left side of the assignment operator must be a variable and operand on the right-hand side must be a constant, variable or expression.
Assignment operators are used to assign value to a variable. The left side of an assignment operator is a variable and on the right side, there is a value, variable, or an expression. It computes the outcome of the right side and assign the output to the variable present on the left side. C supports following Assignment operators: 1.
In C, the assignment operator serves the purpose of assigning a value to a variable. It is denoted by the equals sign (=) and plays a vital role in storing data within variables for further utilization in code. When using the assignment operator, the value present on the right-hand side is assigned to the variable on the left-hand side.
There are five postfix operators in C:- 1. Array subscripts 2. Function Call 3. ... The assignment operator (=) in C is used to assign the value of the right-hand operand to the left-hand operand ...
Simple Assignment Operators. The simple operator " = " is used to store a constant value, the value of another variable or the result of expression evaluation into a variable. For example, a = 15, b = c, or c = 20 + 40. A critical point to understand is the assignment operator requires an Lvalue as its left operand.
for assignments to class type objects, the right operand could be an initializer list only when the assignment is defined by a user-defined assignment operator. removed user-defined assignment constraint. CWG 1538. C++11. E1 ={E2} was equivalent to E1 = T(E2) ( T is the type of E1 ), this introduced a C-style cast. it is equivalent to E1 = T{E2}
What you have above does not work on C arrays, they can't use the assignment operator. If they could, it would, presumably, work just like integers: int a; int b; a = b; //b still exists, of course, and still has its value! it would be unusual to make a custom operator for any type (arrays or not) that self-destructed the right hand side ...
5. In C you cannot assign arrays directly. At first I thought this might because the C facilities were supposed to be implementable with a single or a few instructions and more complicated functionality was offloaded to standard library functions. After all using memcpy() is not that hard.
In C++, the addition assignment operator (+=) combines the addition operation with the variable assignment allowing you to increment the value of variable by a specified expression in a concise and efficient way. Syntax. variable += value; This above expression is equivalent to the expression: variable = variable + value; Example.
And for the comma operator with lowest precedence of all, the syntax is actually: expression: assignment-expression. expression , assignment-expression. That is, the syntax for an expression in C. Now for some reason, C99 apparently didn't want to allow comma operators inside []. I don't know why - perhaps it would make qualified/static array ...
If not, I'd suggest reversing the syntax order in this case (because with= doesn't look great) and making it a three-token operator of =, with, and { that looks like the following: That syntax aligns it with = new for assigning a new instance, = ref for assigning a reference, and = from for assigning a LINQ query, so it feels pretty C#-like to me.
Array::operator [] should probably throw an exception if the index is out of bounds. Currently it just returns garbage memory. Inside Array::Array (int size ) the assignment to size assigns to the parameter, not to the member. Change the first line to: this->size = (size > 0 ? size : 10);
5. Your first overload must be in form: int operator() (int row, int col) const. Not. const int operator() (int row, int col) And it is not the read operation, it is used when an object of your type is created as const, this overload will be used, if not const, other overload will be used, both for reading and writing.