Python Tutorial
File handling, python modules, python numpy, python pandas, python matplotlib, python scipy, machine learning, python mysql, python mongodb, python reference, module reference, python how to, python examples, python datetime, python dates.
A date in Python is not a data type of its own, but we can import a module named datetime to work with dates as date objects.
Import the datetime module and display the current date:
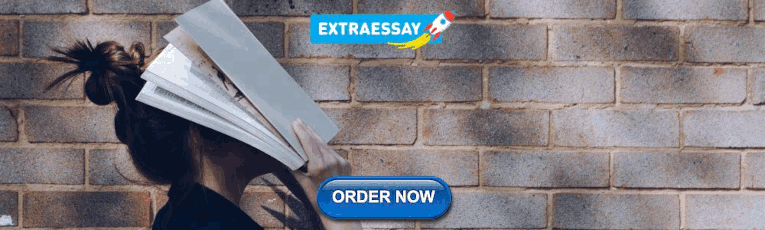
Date Output
When we execute the code from the example above the result will be:
The date contains year, month, day, hour, minute, second, and microsecond.
The datetime module has many methods to return information about the date object.
Here are a few examples, you will learn more about them later in this chapter:
Return the year and name of weekday:
Creating Date Objects
To create a date, we can use the datetime() class (constructor) of the datetime module.
The datetime() class requires three parameters to create a date: year, month, day.
Create a date object:
The datetime() class also takes parameters for time and timezone (hour, minute, second, microsecond, tzone), but they are optional, and has a default value of 0 , ( None for timezone).
Advertisement
The strftime() Method
The datetime object has a method for formatting date objects into readable strings.
The method is called strftime() , and takes one parameter, format , to specify the format of the returned string:
Display the name of the month:
A reference of all the legal format codes:

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
Learn Python practically and Get Certified .
Popular Tutorials
Popular examples, reference materials, learn python interactively, python introduction.
- Get Started With Python
- Your First Python Program
- Python Comments
Python Fundamentals
- Python Variables and Literals
- Python Type Conversion
- Python Basic Input and Output
- Python Operators
Python Flow Control
- Python if...else Statement
- Python for Loop
- Python while Loop
- Python break and continue
- Python pass Statement
Python Data types
- Python Numbers, Type Conversion and Mathematics
- Python List
- Python Tuple
- Python Sets
- Python Dictionary
- Python Functions
- Python Function Arguments
- Python Variable Scope
- Python Global Keyword
- Python Recursion
- Python Modules
- Python Package
- Python Main function
Python Files
- Python Directory and Files Management
- Python CSV: Read and Write CSV files
- Reading CSV files in Python
- Writing CSV files in Python
- Python Exception Handling
- Python Exceptions
- Python Custom Exceptions
Python Object & Class
- Python Objects and Classes
- Python Inheritance
- Python Multiple Inheritance
- Polymorphism in Python
- Python Operator Overloading
Python Advanced Topics
- List comprehension
- Python Lambda/Anonymous Function
- Python Iterators
- Python Generators
- Python Namespace and Scope
- Python Closures
- Python Decorators
- Python @property decorator
- Python RegEx
Python Date and Time
Python datetime
Python strftime()
- Python strptime()
How to get current date and time in Python?
- Python Get Current Time
Python timestamp to datetime and vice-versa
- Python time Module
- Python sleep()
Additional Topic
- Precedence and Associativity of Operators in Python
- Python Keywords and Identifiers
- Python Asserts
- Python Json
- Python *args and **kwargs
Python Tutorials
Python Get Current time
There are a number of ways we can take to get the current date. We will use the date class of the datetime module to accomplish this task.
- Example 1: Python get today's date
Here, we imported the date class from the datetime module. Then, we used the date.today() method to get the current local date.
- Example 2: Current date in different formats
- Get the current date and time in Python
If we need to get the current date and time, you can use the datetime class of the datetime module.
Here, we have used datetime.now() to get the current date and time.
Then, we used strftime() to create a string representing date and time in another format.
Table of Contents
- Introduction
Video: Dates and Times in Python
Sorry about that.
Related Tutorials
Python Tutorial
Creating and Parsing Datetime in Python with Delorean
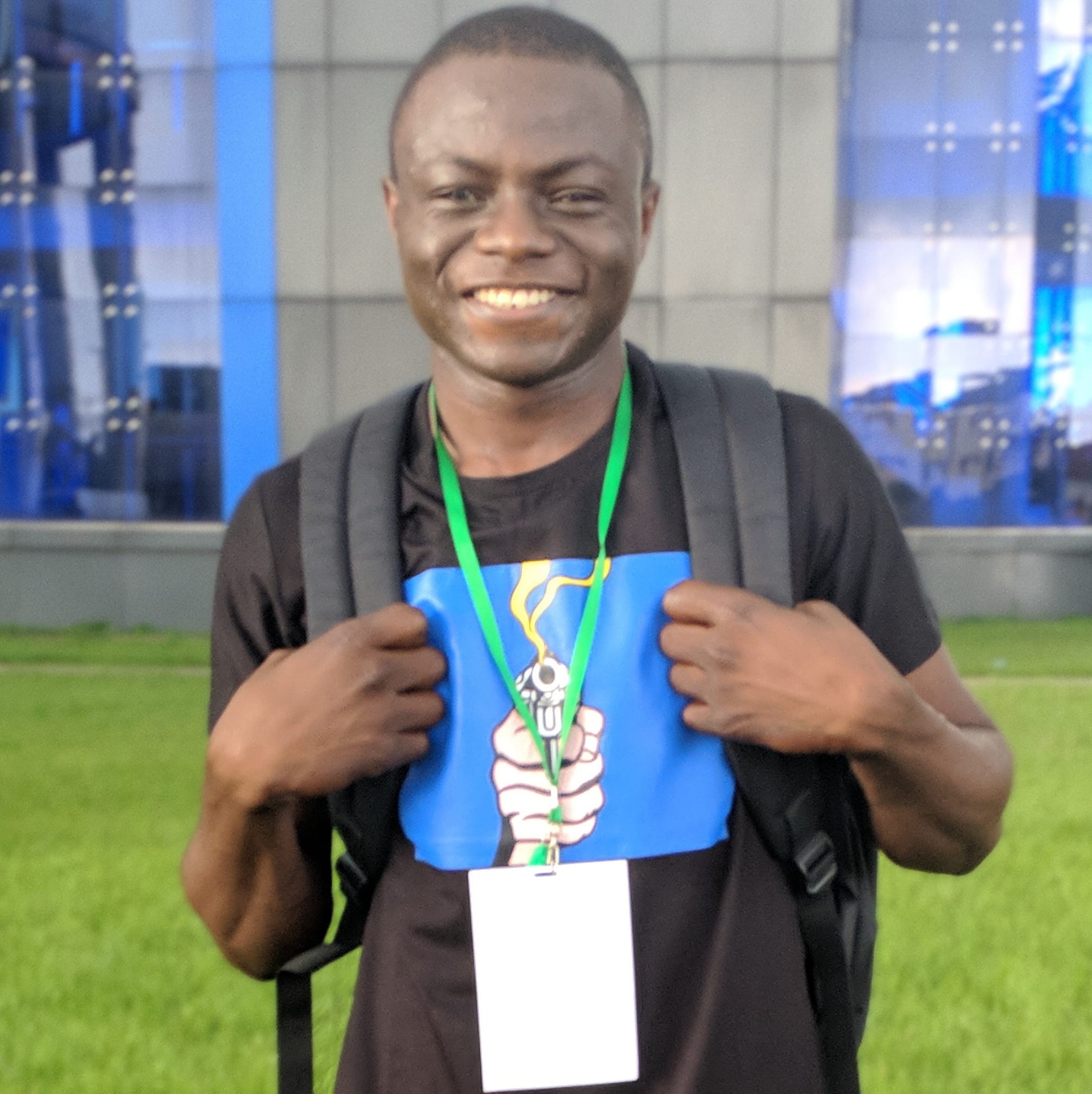
- Introduction
Working with datetime can be a bit daunting and challenging, and handling datetime in Python is no exception. Python's built-in datetime module introduced us to several classes - date , datetime , time , timezone and timedelta , and several external libraries have spawned to address the issues present in the official module, such as Arrow .
In this guide, we'll take a look at how to create and parse datetime objects in Delorean - a library built upon pytz which is the standard library for solving problems relating to time zones, and dateutil which is used to calculate deltas between any 2 given datetime objects.
- Installing Delorean Setting Up a Virtual Environment
Delorean is available for installation via pip . It is generally advisable to work in a virtual environment because it enables you to organize dependencies required by different projects isolated.
On Linux, or MacOS to create a virtual environment, we'd run:
Alternatively, on Windows we can run:
- Creating a Delorean Datetime Object
The main class we'll be working with, which represents all datetime objects is the Delorean() class. Let's go ahead and import it from the delorean module, and instantiate a datetime object:
After running the code, you should see the following on your terminal/command line:
As usual, it's a wrapper for the standard datetime object, which is situated inside the Delorean object assigned to it. The default timezone is 'UTC' , though, you can easily switch this by either defining the timezone while instantiating the object or by shifting the time to a different timezone .
Since the output is a bit hard to parse by humans - it makes sense to extract some of the data from the wrapper to make it easier to interpret. While the descending hierarchy of time is clear - it takes too long to go through it and parse it with our eyes. Let's get the date of this object and print just that:
This results in:
If you're interested in just the time, without much regard for the date itself, you can get both the timezone-aware time, as well as the timezone-naive time fairly easily:
To change the timezone, we either supply it to the constructor call, or shift the time:
To take a look at all the available timezones, since Delorean uses pytz under the hood - we can simply print them out:
Check out our hands-on, practical guide to learning Git, with best-practices, industry-accepted standards, and included cheat sheet. Stop Googling Git commands and actually learn it!
Which results in:
- Convert String to Delorean Datetime Object
No library would be complete without the ability to parse strings into datetime objects. Delorean is versatile with the string formats and assumes that the day comes first if there is any ambiguity in the parsed string. The parse() method is used to parse a string, and it optionally accepts timezone information - otherwise, 'UTC' is assumed:
Running this code results in:
You can notice how in the ambiguous case of 8/6/2019 , it was assumed that the day comes first, hence it was parsed as the 8th of June, instead of the 6th of August. Also, since we didn't provide the year of the final date - it's automatically assigned to the current year.
If you'd like to turn this behavior around, you can set the dayfirst argument to False . Additionally, you can also set the yearfirst argument to True , which is False by default, in which case, the first expected value will be the year :
- Epoch Timestamp to Delorean Object
Since all modern computers adopted the use of UNIX time , also known as Epoch time - it's needless to say that we can convert a UNIX/Epoch timestamp into a Delorean object. This is the underlying mechanism that allows for working with time anyway. To convert a Unix timestamp into a Delorean datetime object, we use the epoch() method of the delorean module:
We've inconspicuously used the first 10 digits of Pi to form a timestamp into the future, resulting in a time well beyond that of this guide:
- Generating a Sequence of Dates
A great way to produce a sequence of Delorean objects is via the stops() generator. You can generate N dates following a pattern, such as every Tuesday , or every hour or every 10 weeks . This is useful for creating, say, monthly payment plans or calculating ROI plans for instance.
Using this approach, you can also generate a series of dates on which something should occur, such as running a script every week at the same time, to gather data from an application for aggregation. Though, you can also use the python-crontab library for that, or the underlying crontab utility tool instead.
The stops() generator accepts a freq argument, denoting the frequency, a timezone and a count , denoting how many dates it should generate. The frequency can be set to any valid Delorean constant - SECONDLY , MINUTELY , HOURLY , DAILY , WEEKLY , MONTHLY or YEARLY :
This generates a sequence of datetime objects following this pattern:
If you're unsure how many datetime objects you want exactly but do have a target datetime in mind, you can also set it to loop until a given date occurs:
Note: The stops() method only accepts timezone-naive datetime instances for the start and stop arguments, and returns timezone-aware dates. But it also requires you to specify a timezone when instantiating a Delorean instance using the constructor. What we're left with is - defining a timezone for the instance, and then using the naive datetime for both instead.
Running this code will give us a date for each month until it reaches the first of January, 2022:
In this guide, we've taken a look at how to create and parse Delorean objects in Python. We've seen how to convert a string to datetime in various formats, how to convert an epoch timestamp into datetime, and how to generate a sequence of dates using the stops() generator.
You might also like...
- Hidden Features of Python
- Python Docstrings
- Handling Unix Signals in Python
- The Best Machine Learning Libraries in Python
- Guide to Sending HTTP Requests in Python with urllib3
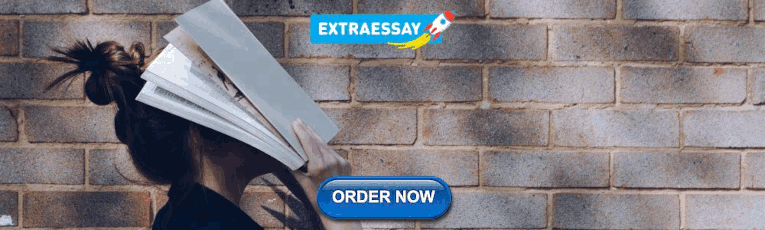
Improve your dev skills!
Get tutorials, guides, and dev jobs in your inbox.
No spam ever. Unsubscribe at any time. Read our Privacy Policy.
I am a software developer with interests in open source, android development (with kotlin), backend web development (with python), and data science (with python as well).
In this article
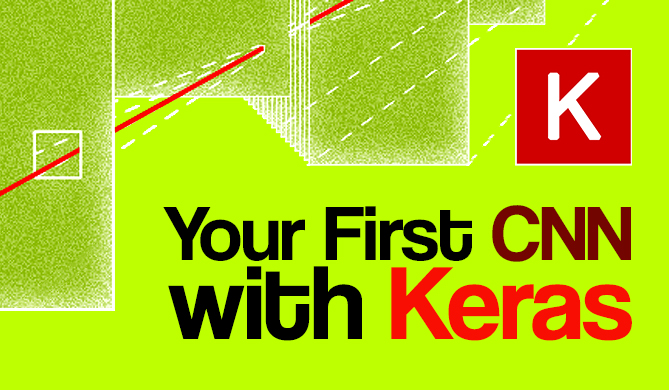
Building Your First Convolutional Neural Network With Keras
Most resources start with pristine datasets, start at importing and finish at validation. There's much more to know. Why was a class predicted? Where was...
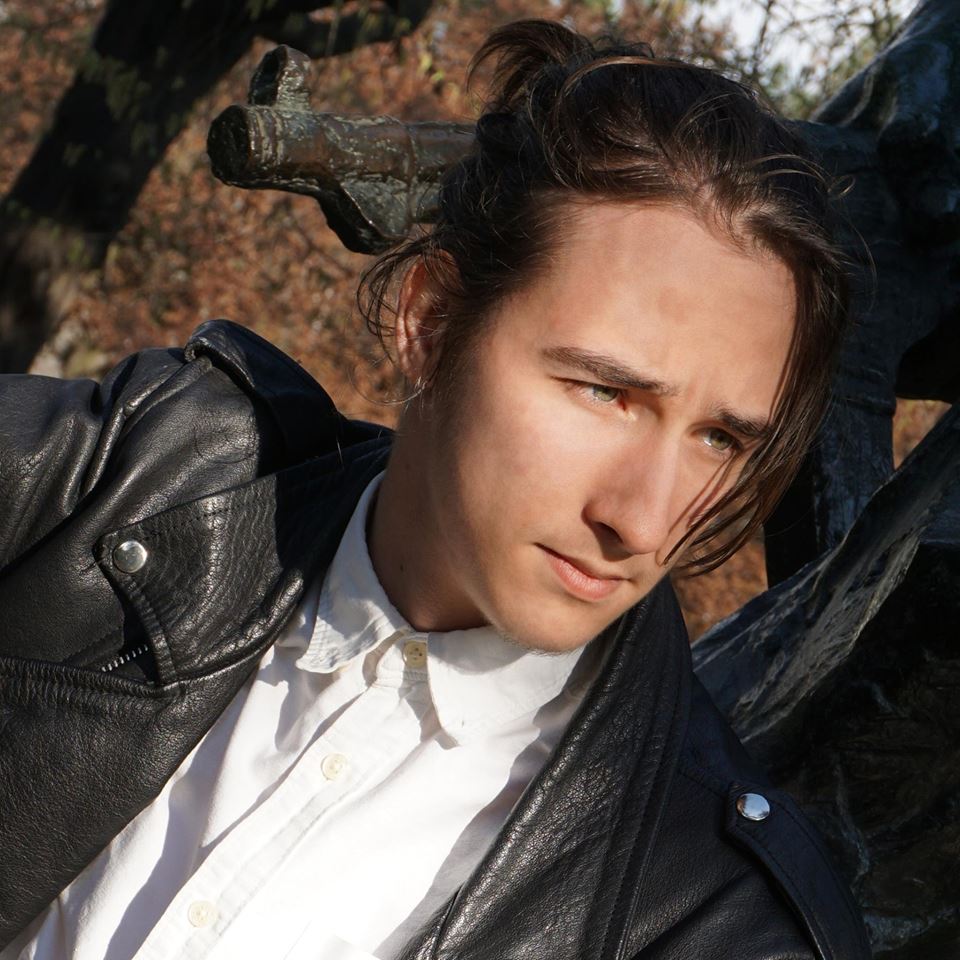
Data Visualization in Python with Matplotlib and Pandas
Data Visualization in Python with Matplotlib and Pandas is a course designed to take absolute beginners to Pandas and Matplotlib, with basic Python knowledge, and...
© 2013- 2024 Stack Abuse. All rights reserved.
- How it works
- Homework answers
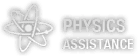
Answer to Question #178448 in Python for CHANDRASENA REDDY CHADA
Date Format - 2
Given seconds as input, write a program to print in D days H hours M minutes S seconds.Input
The input will be a single line containing an integer.Output
The output should be a single line containing the D Days H Hours M Minutes S Seconds format. Print only the non-zero values.Explanation
For example, if the given seconds are 200. As 200 seconds is equal to 3 minutes and 20 seconds, and days, hours are zero, so ignore the days and hours. So the output should be "3 Minutes 20 Seconds"
Sample Input 1
Sample Output 1
3 Minutes 20 Seconds
Sample Input 2
Sample Output 2
Need a fast expert's response?
and get a quick answer at the best price
for any assignment or question with DETAILED EXPLANATIONS !
Good explanation, thank you...
Leave a comment
Ask your question, related questions.
- 1. Right Angle TriangleGiven an integer N, write a program to print a right angle triangle pattern simi
- 2. Date Format - 2Given seconds as input, write a program to print in D days H hours M minutes S second
- 3. Date Format - 2 Given seconds as input, write a program to print in D days H hours M minutes S secon
- 4. Right Angle Triangle Given an integer N, write a program to print a right angle triangle pattern sim
- 5. You are going to create a quiz in python. Your program should allow the player to choose the subject
- 6. Write a function that finds the factorial of a number. The function should get a number from the use
- 7. Write a function named max that accepts two integer values as arguments and returns the value that i
- Programming
- Engineering
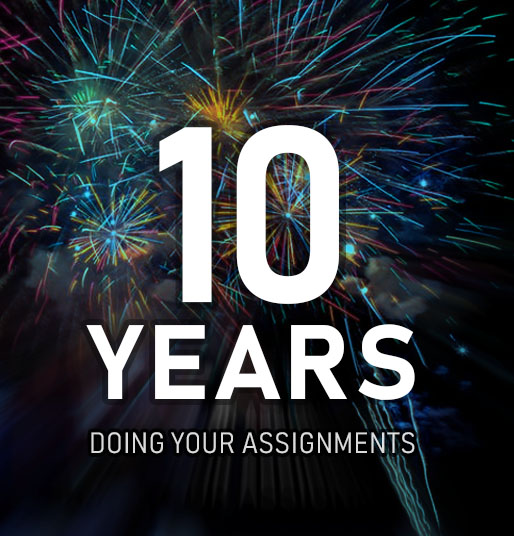
Who Can Help Me with My Assignment
There are three certainties in this world: Death, Taxes and Homework Assignments. No matter where you study, and no matter…
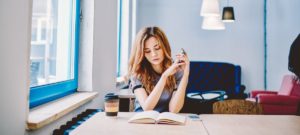
How to Finish Assignments When You Can’t
Crunch time is coming, deadlines need to be met, essays need to be submitted, and tests should be studied for.…
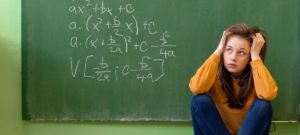
How to Effectively Study for a Math Test
Numbers and figures are an essential part of our world, necessary for almost everything we do every day. As important…

Getting the Weekday Name in Python

Python’s DateTime module offers a wide range of time-handling functions that make life a breeze. However, there’s no direct method to return a plain-English representation of a weekday name. Fortunately, there are several easy ways to get there.
- 1 Getting the Date + Weekday
- 5 Final Thoughts
Getting the Date + Weekday
Before we take a look at getting the weekday name, let’s consider how Python’s datetime class gets a date and, subsequently, determines the day of the week for said date. There’s nothing fancy about it—but it pays to know where you’re starting .
This code illustrates how far the datetime class will get you before you have to start getting creative. Before we move on, there are two things worth noting:
- The weekday method assigns Monday the value 0
- Another method, the isoweekday , assigns Sunday the value of 1
Check out the official datetime.weekday documentation for more info. Now, knowing how to get an integer representation of the weekday for a given datetime object, we can start scheming on how to produce a plain-English version. Below are three approaches ranked in order of dependencies.
Method 1: Do it Manually
This approach offers the flexibility of being able to tweak weekday naming and order on-the-fly. Need to truncate the date names? Just edit the list. Need to start with Sunday? Just re-arrange it. Need to reverse the list for some strange reason? Just reverse it!
- No added dependencies
- No added methods to remember
- Flexibility for change
- Explicit representation in code
- More typing
- Not immediately resistant to duplication issues (i.e. using it across a project)
- The fear of typos
Method 2: Use Strftime
This method is, arguably, the most succinct. It’s a reasonable one-liner, doesn’t stray from the datetime class, and offers some flexibility once familiar with the strftime documentation .
- No extra dependencies
- One-liner possible
- Semi-flexible
- No fear of typos
- Easy to integrate project-wide
- Need to be familiar with strftime to change format
Method 3: The Calendar Module
This is really just a round-about way to achieve the same result as the first method. The added benefit here is that someone else manages the weekday list (not really a burden in this case.) The calender.day_name object is just a fancy list of all weekday names. Indexing into this object is the same as indexing into a manual list.
- Built-in module
- Lightweight dependency
- Uses native Python syntax for indexing
- Extra dependency
- The syntax could be simpler
Final Thoughts
When I was first learning to code, one of the biggest surprises to me was how complex managing time and dates can be. The subtle length differences in months, leap years, converting strings to datetimes —it seemed like wading into the deep end for what was such a peripheral task.
Converting computer-friendly date representations like epoch timestamps into user-friendly representations is another annoyingly demanding task. In this case, Python’s datetime class makes it simple to get the weekday integer. After that, it’s all just syntactic sugar! Personally, I prefer method two here because it keeps everything tightly coupled to the DateTime module.
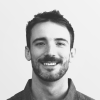
Python Reduce(): A Powerful Functional Programming Made Simple
Have you ever found yourself wondering what all the hype is about functional programming? This ...
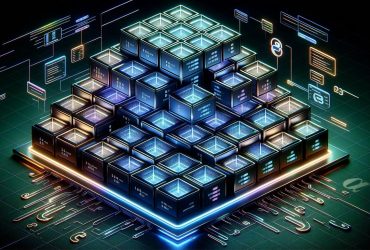
Python’s DefaultDict: Hash Tables Made Easy
Hash Tables do wonders for search operations but can quickly cause syntactic clutter when used ...
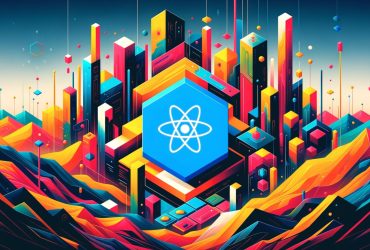
ESLint + React Functional Component Parameter Destructuring
React and ESLint are both amazing tools for building modern applications. Getting them to work ...

- Python Basics
- Interview Questions
- Python Quiz
- Popular Packages
- Python Projects
- Practice Python
- AI With Python
- Learn Python3
- Python Automation
- Python Web Dev
- DSA with Python
- Python OOPs
- Dictionaries
- Python datetime module
- Python DateTime - Date Class
- ctime() Function Of Datetime.date Class In Python
- fromisoformat() Function Of Datetime.date Class In Python
- Fromordinal() Function Of Datetime.date Class In Python
- fromtimestamp() Function Of Datetime.date Class In Python
- isocalendar() Function Of Datetime.date Class In Python
- Isoformat() Function Of Datetime.date Class In Python
- Isoweekday() Function Of Datetime.date Class In Python
- timetuple() Function Of Datetime.date Class In Python
- Get current date using Python
- toordinal() Function Of Datetime.date Class In Python
weekday() Function Of Datetime.date Class In Python
- Python DateTime - Time Class
- Python time.daylight() Function
- Python time.tzname() Function
Datetime class
- Python DateTime - DateTime Class
- Python DateTime astimezone() Method
- Python - time.ctime() Method
- Isocalendar() Method Of Datetime Class In Python
- Isoformat() Method Of Datetime Class In Python
- Isoweekday() Method Of Datetime Class In Python
- Datetime.replace() Function in Python
- Python DateTime - strptime() Function
- Python | time.time() method
- Python datetime.timetz() Method with Example
- Python - datetime.toordinal() Method with Example
- Get UTC timestamp in Python
- Python datetime.utcoffset() Method with Example
Timedelta class
- Python DateTime - Timedelta Class
- Python - Timedelta object with negative values
- Python | Difference between two dates (in minutes) using datetime.timedelta() method
- Python | datetime.timedelta() function
- Python timedelta total_seconds() Method with Example
- Python - datetime.tzinfo()
- Handling timezone in Python
The weekday() of datetime.date class function is used to return the integer value corresponding to the specified day of the week.
Syntax: weekday() Parameters: This function does not accept any parameter. Return values: This function returns the integer mapping corresponding to the specified day of the week. Below table shows the integer values corresponding to the day of the week.
Example 1: Getting the integer values corresponding to the specified days of the week.
Example 2: Getting the date and time along with the day’s name of the entire week.
Please Login to comment...
Similar reads.
- Python-datetime
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
CopyAssignment
We are Python language experts, a community to solve Python problems, we are a 1.2 Million community on Instagram, now here to help with our blogs.
- Weekend in Python
Checking whether a date is a weekday or weekend in Python is very easy. If you don’t know, weekend days are Saturday and Sunday . Check out the following code to learn how to find a date is a weekday or weekend.
- Hyphenate Letters in Python
- Earthquake in Python | Easy Calculation
- Striped Rectangle in Python
- Perpendicular Words in Python
- Free shipping in Python
- Raj has ordered two electronic items Python | Assignment Expert
- Team Points in Python
- Ticket selling in Cricket Stadium using Python | Assignment Expert
- Split the sentence in Python
- String Slicing in JavaScript
- First and Last Digits in Python | Assignment Expert
- List Indexing in Python
- Date Format in Python | Assignment Expert
- New Year Countdown in Python
- Add Two Polynomials in Python
- Sum of even numbers in Python | Assignment Expert
- Evens and Odds in Python
- A Game of Letters in Python
- Sum of non-primes in Python
- Smallest Missing Number in Python
- String Rotation in Python
- Secret Message in Python
- Word Mix in Python
- Single Digit Number in Python
- Shift Numbers in Python | Assignment Expert
- Temperature Conversion in Python
- Special Characters in Python
- Sum of Prime Numbers in the Input in Python
Author: Harry

Search….
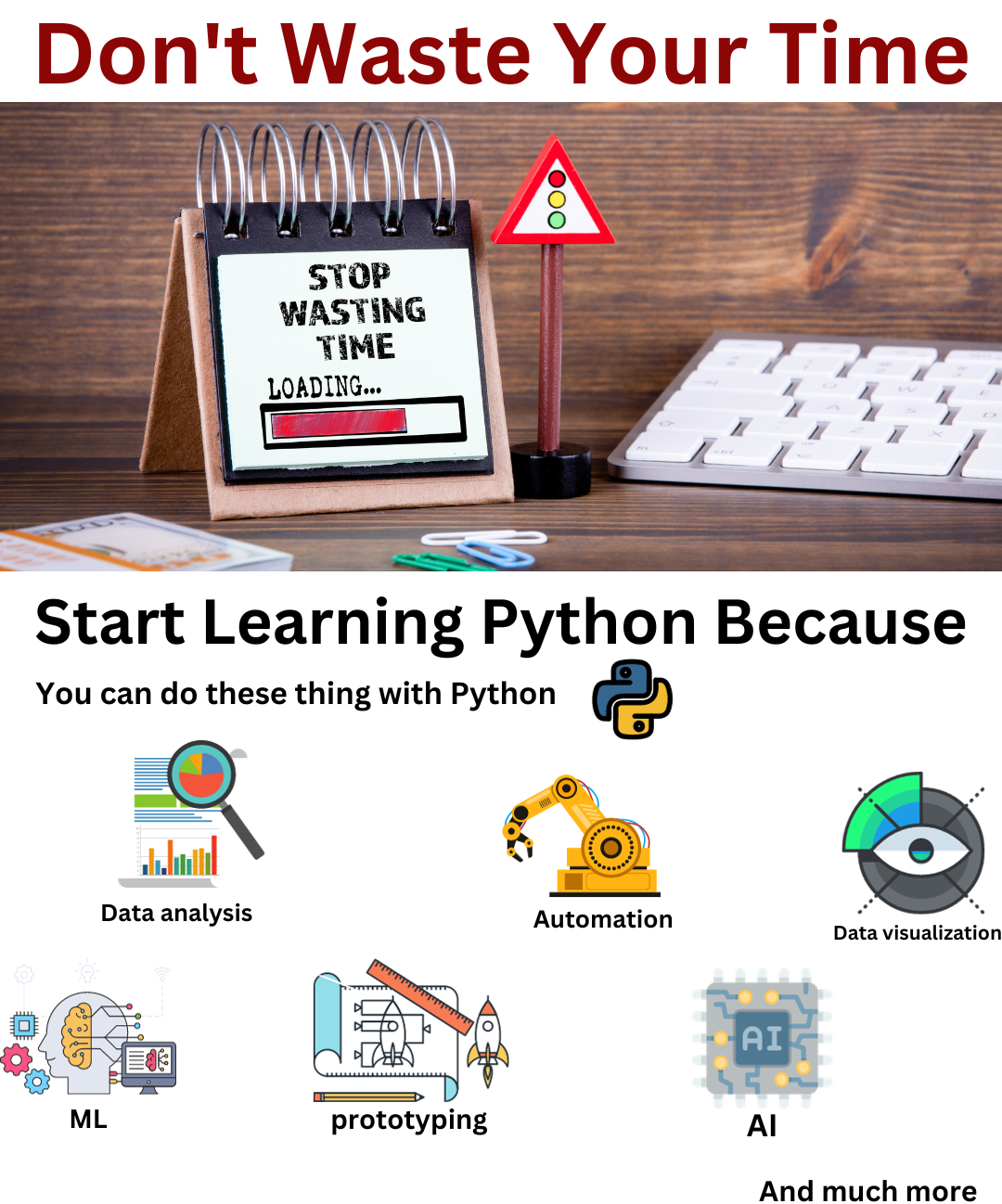
Machine Learning
Data Structures and Algorithms(Python)
Python Turtle
Games with Python
All Blogs On-Site
Python Compiler(Interpreter)
Online Java Editor
Online C++ Editor
Online C Editor
All Editors
Services(Freelancing)
Recent Posts
- Most Underrated Database Trick | Life-Saving SQL Command
- Python List Methods
- Top 5 Free HTML Resume Templates in 2024 | With Source Code
- How to See Connected Wi-Fi Passwords in Windows?
- 2023 Merry Christmas using Python Turtle
© Copyright 2019-2023 www.copyassignment.com. All rights reserved. Developed by copyassignment
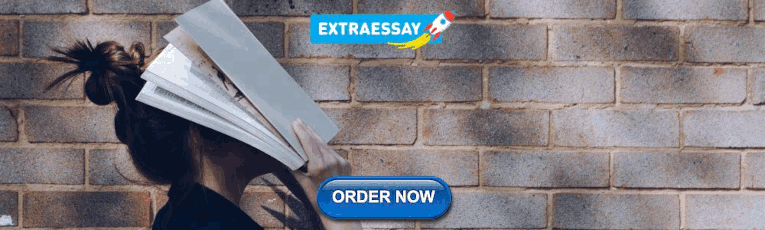
IMAGES
VIDEO
COMMENTS
Question #199892. Given date-time D, write a program to print the time left for the next New Year.Input. The input will be a single line containing the date-time in the string format similar to "Dec 30 2020 02:43 PM".Output. The output should be a single line containing hours and minutes left for the next new year in the format similar to "33 ...
The WHY: dates are objects. In Python, dates are objects. Therefore, when you manipulate them, you manipulate objects, not strings or timestamps. Any object in Python has TWO string representations: The regular representation that is used by print can be get using the str() function. It is most of the time the most common human readable format ...
Exercise 1: Print current date and time in Python. Exercise 2: Convert string into a datetime object. Exercise 3: Subtract a week (7 days) from a given date in Python. Exercise 4: Print a date in a the following format. Exercise 5: Find the day of the week of a given date. Exercise 6: Add a week (7 days) and 12 hours to a given date.
Date Output. When we execute the code from the example above the result will be: 2024-05-10 08:15:40.977341. The date contains year, month, day, hour, minute, second, and microsecond. The datetime module has many methods to return information about the date object. Here are a few examples, you will learn more about them later in this chapter:
Convert dates to strings using DateTime. Date and time are different from strings and thus many times it is important to convert the DateTime to string. For this, we use strftime() method. Syntax of datetime.strftime. Syntax: datetime.strftime(format, t) Parameters: format - This is of string type. i.e. the directives can be embedded in the ...
Parsing and formatting dates in very in Python with datetime. In this video I show you how to use the strftime and strptime functions to work with dates.Need...
In this tutorial, you will learn to get today's date and current date and time in Python with the help of examples. Courses Tutorials Examples Try Programiz PRO
Introduction. Working with datetime can be a bit daunting and challenging, and handling datetime in Python is no exception. Python's built-in datetime module introduced us to several classes - date, datetime, time, timezone and timedelta, and several external libraries have spawned to address the issues present in the official module, such as Arrow.. In this guide, we'll take a look at how to ...
Write a Python program to print yesterday, today, tomorrow. Click me to see the solution. 8. Write a Python program to convert the date to datetime (midnight of the date) in Python. Sample Output : 2015-06-22 00:00:00. Click me to see the solution. 9. Write a Python program to print the next 5 days starting today. Click me to see the solution. 10.
Question #178448. Date Format - 2. Given seconds as input, write a program to print in D days H hours M minutes S seconds.Input. The input will be a single line containing an integer.Output. The output should be a single line containing the D Days H Hours M Minutes S Seconds format. Print only the non-zero values.Explanation.
Python DateTime weekday () Method with Example. In this article, we will discuss the weekday () function in the DateTime module. weekday () function is used to get the week number based on given DateTime. It will return the number in the range of 0-6. It will take input as DateTime in the format of " (YYYY, MM, DD, HH, MM, SS)", where, We ...
Getting the Date + Weekday. Before we take a look at getting the weekday name, let's consider how Python's datetime class gets a date and, subsequently, determines the day of the week for said date. There's nothing fancy about it—but it pays to know where you're starting.. from datetime import datetime # Create datetime object date = datetime.now() >>> 2021-02-07 15:30:46.665318 ...
The author selected the COVID-19 Relief Fund to receive a donation as part of the Write for DOnations program.. Introduction. Python 3.8, released in October 2019, adds assignment expressions to Python via the := syntax. The assignment expression syntax is also sometimes called "the walrus operator" because := vaguely resembles a walrus with tusks. ...
The weekday () of datetime.date class function is used to return the integer value corresponding to the specified day of the week. Syntax: weekday () Parameters: This function does not accept any parameter. Return values: This function returns the integer mapping corresponding to the specified day of the week.
If you don't know, weekend days are Saturday and Sunday. Check out the following code to learn how to find a date is a weekday or weekend. Code: from datetime import datetime d = datetime (year, month, date); if d.weekday () > 4: print 'Date is weekend.' else: print 'Data is weekday.'. Also Read:
Technically you can "print" and assign on the same line by using sys. See the following: >>> import sys >>> a = sys.stdout.write("hello") hello >>> print(a) 5 As you can see, you "print" - by writing to standard out which is essentially the the same as print() - the string and assign the length of the string to the variable - not the string itself.
Since functions are no different than variables, you can reassign them however you please: def add(a, b): return a + b. add = "ha-ha, I'm a string". The parentheses after the = sign are the kind of parentheses you use in math: print = (1 + 2) # print == 3. Parentheses immediately after a name represent a function call.