
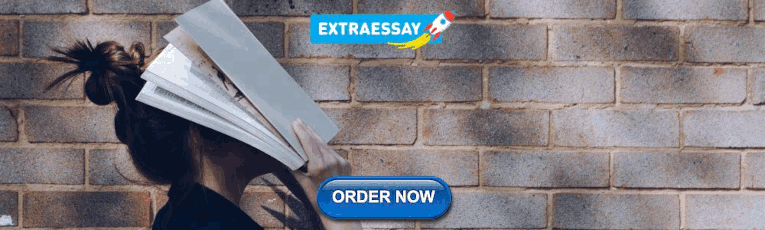
Typeerror: int object does not support item assignment
Today, we will explore the “typeerror: int object does not support item assignment” error message in Python.
If this error keeps bothering you, don’t worry! We’ve got your back.
In this article, we will explain in detail what this error is all about and why it occurs in your Python script.
Aside from that, we’ll hand you the different solutions that will get rid of this “typeerror: ‘int’ object does not support item assignment” error.
Please enable JavaScript
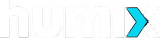
What is “typeerror: ‘int’ object does not support item assignment”?
The “typeerror int object does not support item assignment” is an error message in Python that occurs when you try to assign a value to an integer as if it were a list or dictionary, which is not allowed.
It is mainly because integers are immutable in Python, meaning their value cannot be changed after they are created.
In other words, once an integer is created, its value cannot be changed.
For example:
In this example, the code tries to assign the value 1 to the first element of sample , but sample is an integer, not a list or dictionary, and as a result, it throws an error:
This error message indicates that you need to modify your code to avoid attempting to change an integer’s value using item assignment.
What are the root causes of “typeerror: ‘int’ object does not support item assignment”
Here are the most common root causes of “int object does not support item assignment”, which include the following:
- Attempting to modify an integer directly
- Using an integer where a sequence is expected
- Not converting integer objects to mutable data types before modifying them
- Using an integer as a dictionary key
- Passing an integer to a function that expects a mutable object
- Mixing up variables
- Incorrect syntax
- Using the wrong method or function
How to fix “typeerror: int object does not support item assignment”
Here are the following solutions to fix the “typeerror: ‘int’ object does not support item assignment” error message:
Solution 1: Use a list instead of an integer
Since integers are immutable in Python, we cannot modify them.
Therefore, we can use a mutable data type such as a list instead to modify individual elements within a variable.
Solution 2: Convert the integer to a list first
You have to convert the integer first to a string using the str() function.
Then, convert the string to a list using the list() function.
We can also modify individual elements within the list and join it back into a string using the join() function.
Finally, we convert the string back to an integer using the int() function.
Solution 3: Use a dictionary instead of an integer
This solution is similar to using a list. We can use a dictionary data type to modify individual elements within a variable.
In this example code, we modify the value of the ‘b’ key within the dictionary.
Another example:
Solution 4: Use a different method or function
You can define sample as a list and append items to the list using the append() method .
How to avoid “typeerror: int object does not support item assignment”
- You have to use appropriate data types for the task at hand.
- You must convert immutable data types to mutable types before modifying it.
- You have to use the correct operators and functions for specific data types.
- Avoid modifying objects directly if they are not mutable.
Frequently Asked Question (FAQs)
You can easily resolve the error by converting the integer object to a mutable data type or creating a new integer object with the desired value.
The error occurs when you try to modify an integer object, which is not allowed since integer objects are immutable.
By executing the different solutions that this article has already given, you can definitely resolve the “typeerror: int object does not support item assignment” error message in Python.
We are hoping that this article provides you with sufficient solutions.
You could also check out other “ typeerror ” articles that may help you in the future if you encounter them.
- Uncaught typeerror: illegal invocation
- Typeerror slice none none none 0 is an invalid key
- Typeerror: cannot read property ‘getrange’ of null
Thank you very much for reading to the end of this article.

TypeError: 'tuple' object does not support item assignment
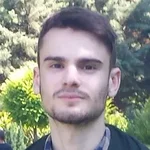
Last updated: Apr 8, 2024 Reading time · 4 min
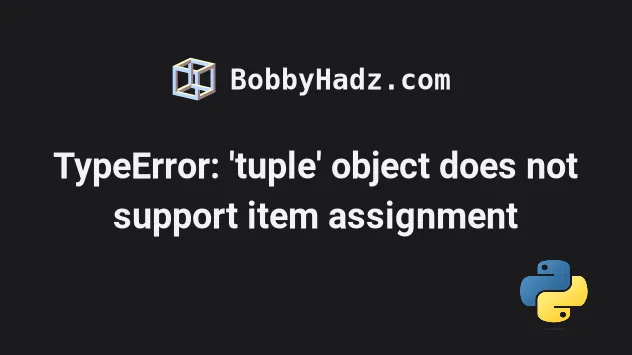
# TypeError: 'tuple' object does not support item assignment
The Python "TypeError: 'tuple' object does not support item assignment" occurs when we try to change the value of an item in a tuple.
To solve the error, convert the tuple to a list, change the item at the specific index and convert the list back to a tuple.

Here is an example of how the error occurs.
We tried to update an element in a tuple, but tuple objects are immutable which caused the error.
# Convert the tuple to a list to solve the error
We cannot assign a value to an individual item of a tuple.
Instead, we have to convert the tuple to a list.
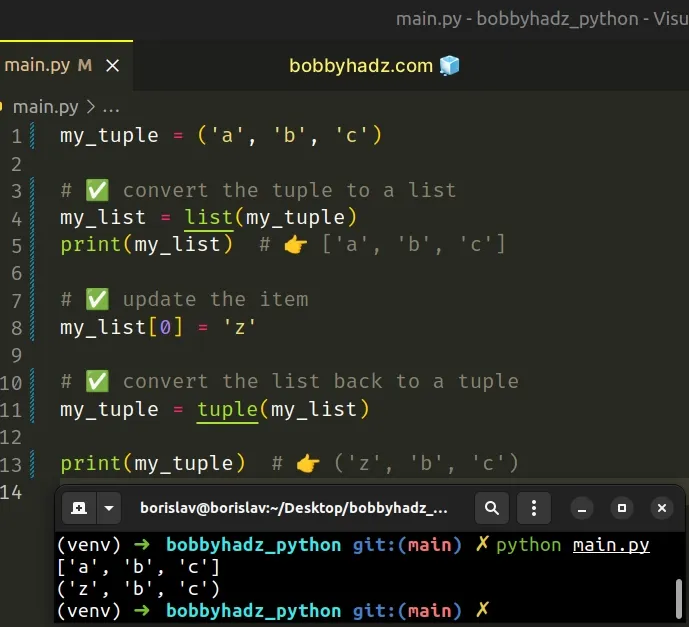
This is a three-step process:
- Use the list() class to convert the tuple to a list.
- Update the item at the specified index.
- Use the tuple() class to convert the list back to a tuple.
Once we have a list, we can update the item at the specified index and optionally convert the result back to a tuple.
Python indexes are zero-based, so the first item in a tuple has an index of 0 , and the last item has an index of -1 or len(my_tuple) - 1 .
# Constructing a new tuple with the updated element
Alternatively, you can construct a new tuple that contains the updated element at the specified index.
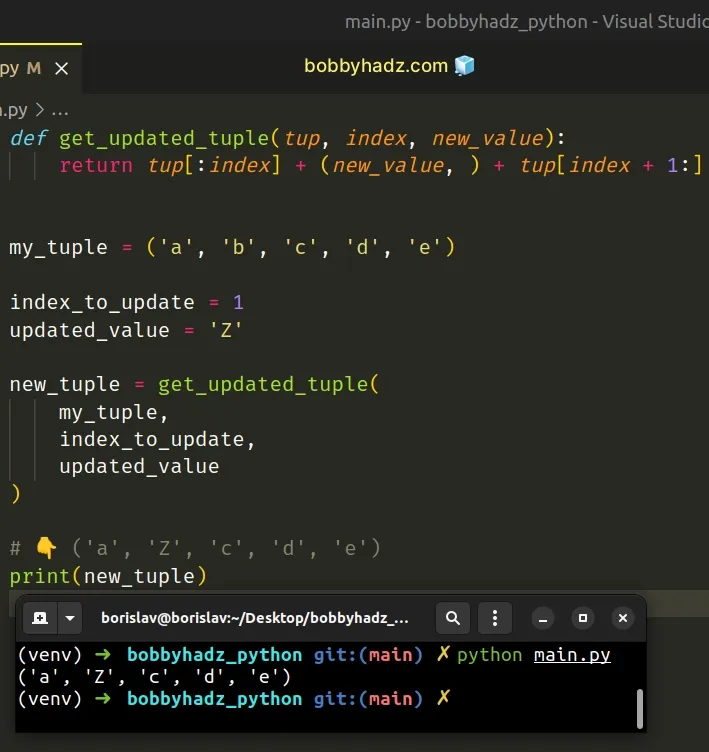
The get_updated_tuple function takes a tuple, an index and a new value and returns a new tuple with the updated value at the specified index.
The original tuple remains unchanged because tuples are immutable.
We updated the tuple element at index 1 , setting it to Z .
If you only have to do this once, you don't have to define a function.
The code sample achieves the same result without using a reusable function.
The values on the left and right-hand sides of the addition (+) operator have to all be tuples.
The syntax for tuple slicing is my_tuple[start:stop:step] .
The start index is inclusive and the stop index is exclusive (up to, but not including).
If the start index is omitted, it is considered to be 0 , if the stop index is omitted, the slice goes to the end of the tuple.
# Using a list instead of a tuple
Alternatively, you can declare a list from the beginning by wrapping the elements in square brackets (not parentheses).
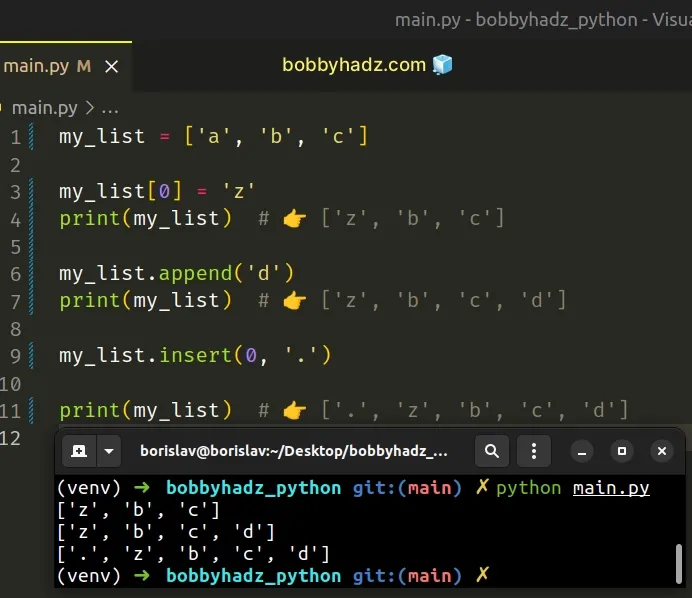
Declaring a list from the beginning is much more efficient if you have to change the values in the collection often.
Tuples are intended to store values that never change.
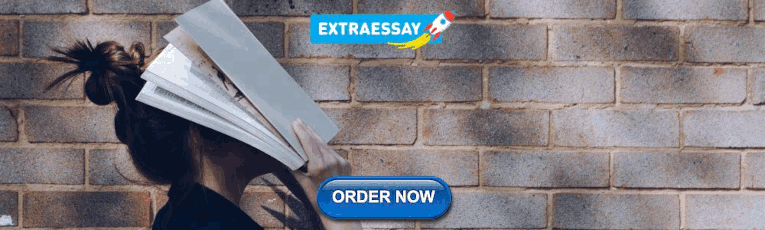
# How tuples are constructed in Python
In case you declared a tuple by mistake, tuples are constructed in multiple ways:
- Using a pair of parentheses () creates an empty tuple
- Using a trailing comma - a, or (a,)
- Separating items with commas - a, b or (a, b)
- Using the tuple() constructor
# Checking if the value is a tuple
You can also handle the error by checking if the value is a tuple before the assignment.
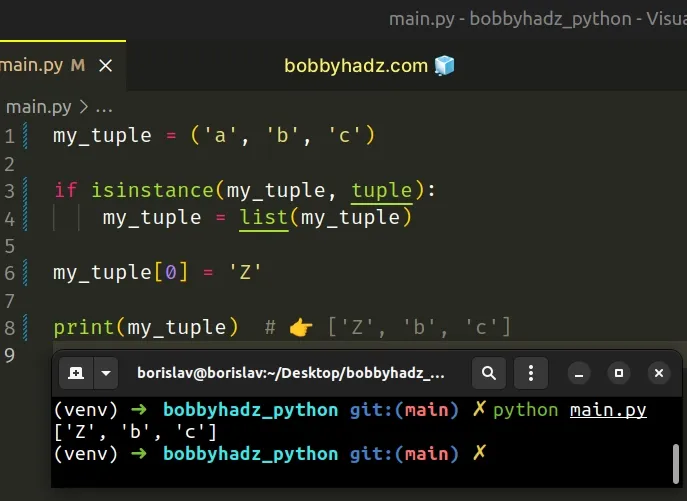
If the variable stores a tuple, we set it to a list to be able to update the value at the specified index.
The isinstance() function returns True if the passed-in object is an instance or a subclass of the passed-in class.
If you aren't sure what type a variable stores, use the built-in type() class.
The type class returns the type of an object.
# Additional Resources
You can learn more about the related topics by checking out the following tutorials:
- How to convert a Tuple to an Integer in Python
- How to convert a Tuple to JSON in Python
- Find Min and Max values in Tuple or List of Tuples in Python
- Get the Nth element of a Tuple or List of Tuples in Python
- Creating a Tuple or a Set from user Input in Python
- How to Iterate through a List of Tuples in Python
- Write a List of Tuples to a File in Python
- AttributeError: 'tuple' object has no attribute X in Python
- TypeError: 'tuple' object is not callable in Python [Fixed]
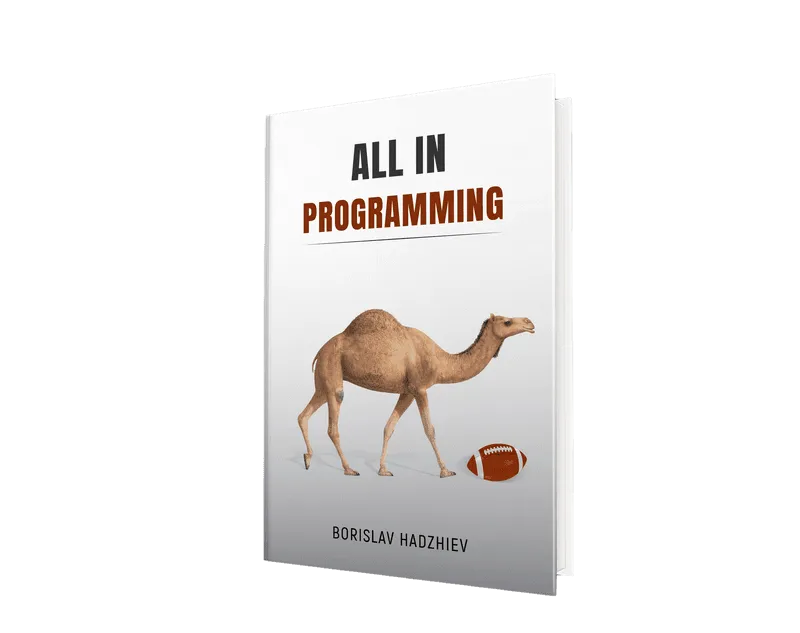
Borislav Hadzhiev
Web Developer
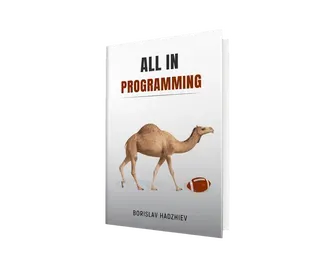
Copyright © 2024 Borislav Hadzhiev
How to Fix STR Object Does Not Support Item Assignment Error in Python
- Python How-To's
- How to Fix STR Object Does Not Support …
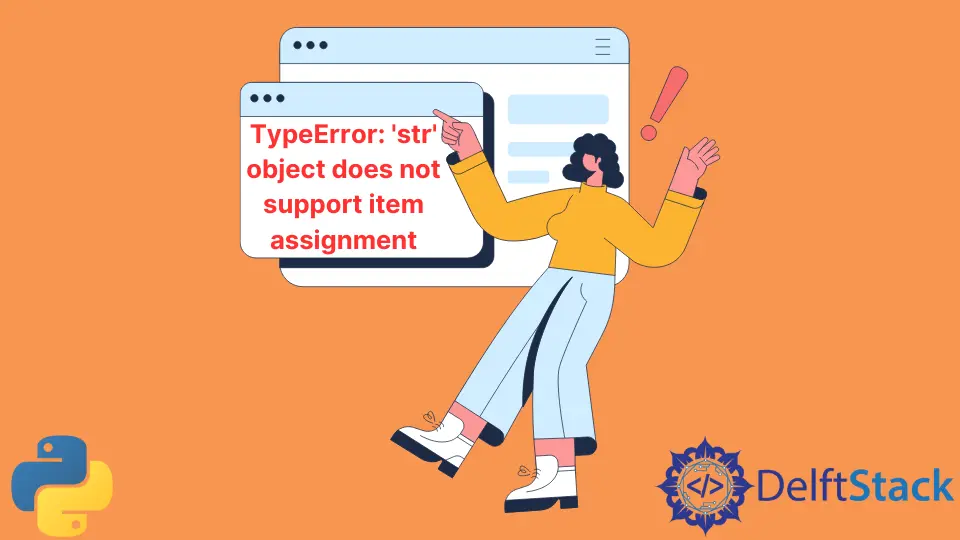
In Python, strings are immutable, so we will get the str object does not support item assignment error when trying to change the string.
You can not make some changes in the current value of the string. You can either rewrite it completely or convert it into a list first.
This whole guide is all about solving this error. Let’s dive in.
Fix str object does not support item assignment Error in Python
As the strings are immutable, we can not assign a new value to one of its indexes. Take a look at the following code.
The above code will give o as output, and later it will give an error once a new value is assigned to its fourth index.
The string works as a single value; although it has indexes, you can not change their value separately. However, if we convert this string into a list first, we can update its value.
The above code will run perfectly.
First, we create a list of string elements. As in the list, all elements are identified by their indexes and are mutable.
We can assign a new value to any of the indexes of the list. Later, we can use the join function to convert the same list into a string and store its value into another string.
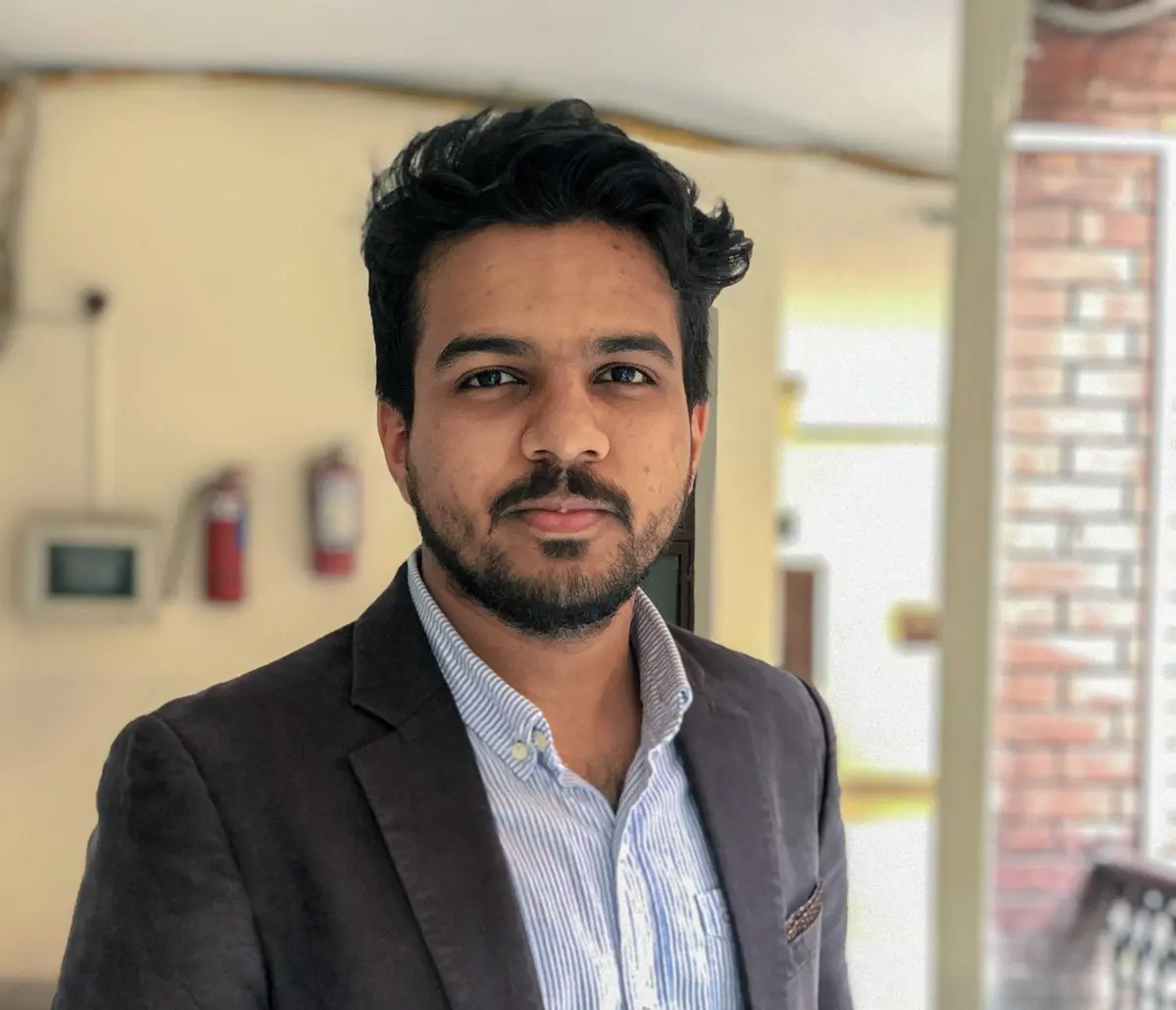
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
Related Article - Python Error
- Can Only Concatenate List (Not Int) to List in Python
- How to Fix Value Error Need More Than One Value to Unpack in Python
- How to Fix ValueError Arrays Must All Be the Same Length in Python
- Invalid Syntax in Python
- How to Fix the TypeError: Object of Type 'Int64' Is Not JSON Serializable
- How to Fix the TypeError: 'float' Object Cannot Be Interpreted as an Integer in Python
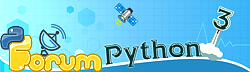
- View Active Threads
- View Today's Posts
- View New Posts
- My Discussions
- Unanswered Posts
- Unread Posts
- Active Threads
- Mark all forums read
- Member List
- Interpreter
Error: int object does not support item assignment
- Python Forum
- Python Coding
- General Coding Help
- 0 Vote(s) - 0 Average
- View a Printable Version
User Panel Messages
Announcements.
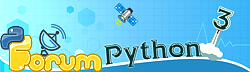
Login to Python Forum
Fix Python TypeError: 'str' object does not support item assignment
by Nathan Sebhastian
Posted on Jan 11, 2023
Reading time: 4 minutes
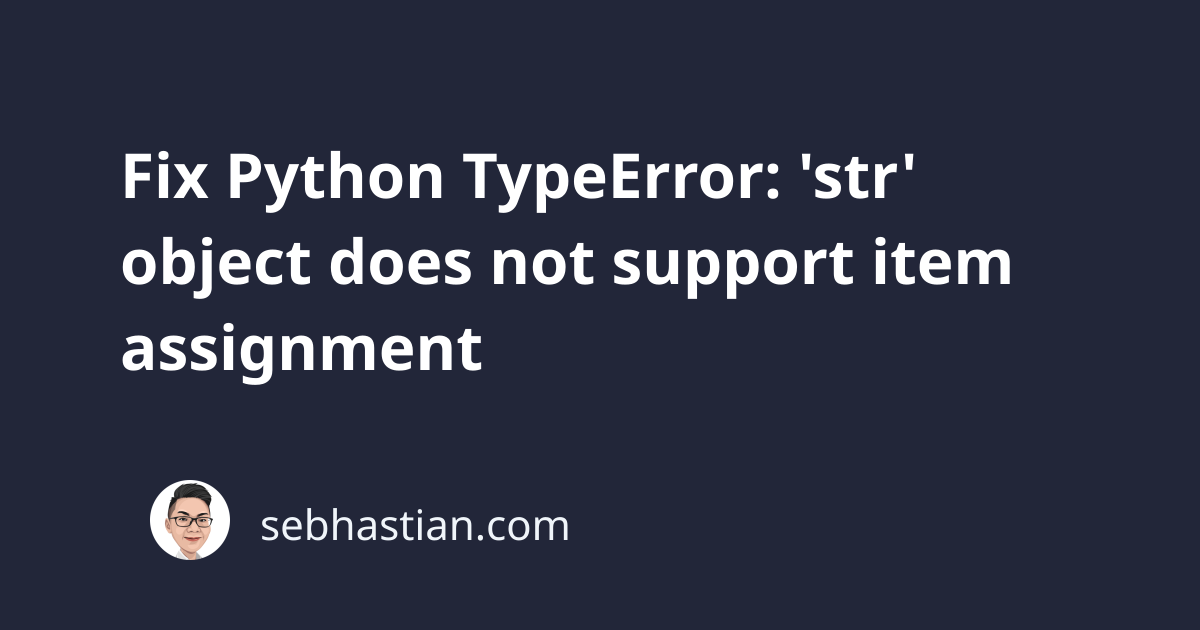
Python shows TypeError: 'str' object does not support item assignment error when you try to access and modify a string object using the square brackets ( [] ) notation.
To solve this error, use the replace() method to modify the string instead.
This error occurs because a string in Python is immutable, meaning you can’t change its value after it has been defined.
For example, suppose you want to replace the first character in your string as follows:
The code above attempts to replace the letter H with J by adding the index operator [0] .
But because assigning a new value to a string is not possible, Python responds with the following error:
To fix this error, you can create a new string with the desired modifications, instead of trying to modify the original string.
This can be done by calling the replace() method from the string. See the example below:
The replace() method allows you to replace all occurrences of a substring in your string.
This method accepts 3 parameters:
- old - the substring you want to replace
- new - the replacement for old value
- count - how many times to replace old (optional)
By default, the replace() method replaces all occurrences of the old string:
You can control how many times the replacement occurs by passing the third count parameter.
The code below replaces only the first occurrence of the old value:
And that’s how you can modify a string using the replace() method.
If you want more control over the modification, you can use a list.
Convert the string to a list first, then access the element you need to change as shown below:
After you modify the list element, merge the list back as a string by using the join() method.
This solution gives you more control as you can select the character you want to replace. You can replace the first, middle, or last occurrence of a specific character.
Another way you can modify a string is to use the string slicing and concatenation method.
Consider the two examples below:
In both examples, the string slicing operator is used to extract substrings of the old_str variable.
In the first example, the slice operator is used to extract the substring starting from index 1 to the end of the string with old_str[1:] and concatenates it with the character ‘J’ .
In the second example, the slice operator is used to extract the substring before index 7 with old_str[:7] and the substring after index 8 with old_str[8:] syntax.
Both substrings are joined together while putting the character x in the middle.
The examples show how you can use slicing to extract substrings and concatenate them to create new strings.
But using slicing and concatenation can be more confusing than using a list, so I would recommend you use a list unless you have a strong reason.
The Python error TypeError: 'str' object does not support item assignment occurs when you try to modify a string object using the subscript or index operator assignment.
This error happens because strings in Python are immutable and can’t be modified.
The solution is to create a new string with the required modifications. There are three ways you can do it:
- Use replace() method
- Convert the string to a list, apply the modifications, merge the list back to a string
- Use string slicing and concatenation to create a new string
Now you’ve learned how to modify a string in Python. Nice work!
Take your skills to the next level ⚡️
I'm sending out an occasional email with the latest tutorials on programming, web development, and statistics. Drop your email in the box below and I'll send new stuff straight into your inbox!
Hello! This website is dedicated to help you learn tech and data science skills with its step-by-step, beginner-friendly tutorials. Learn statistics, JavaScript and other programming languages using clear examples written for people.
Learn more about this website
Connect with me on Twitter
Or LinkedIn
Type the keyword below and hit enter
Click to see all tutorials tagged with:
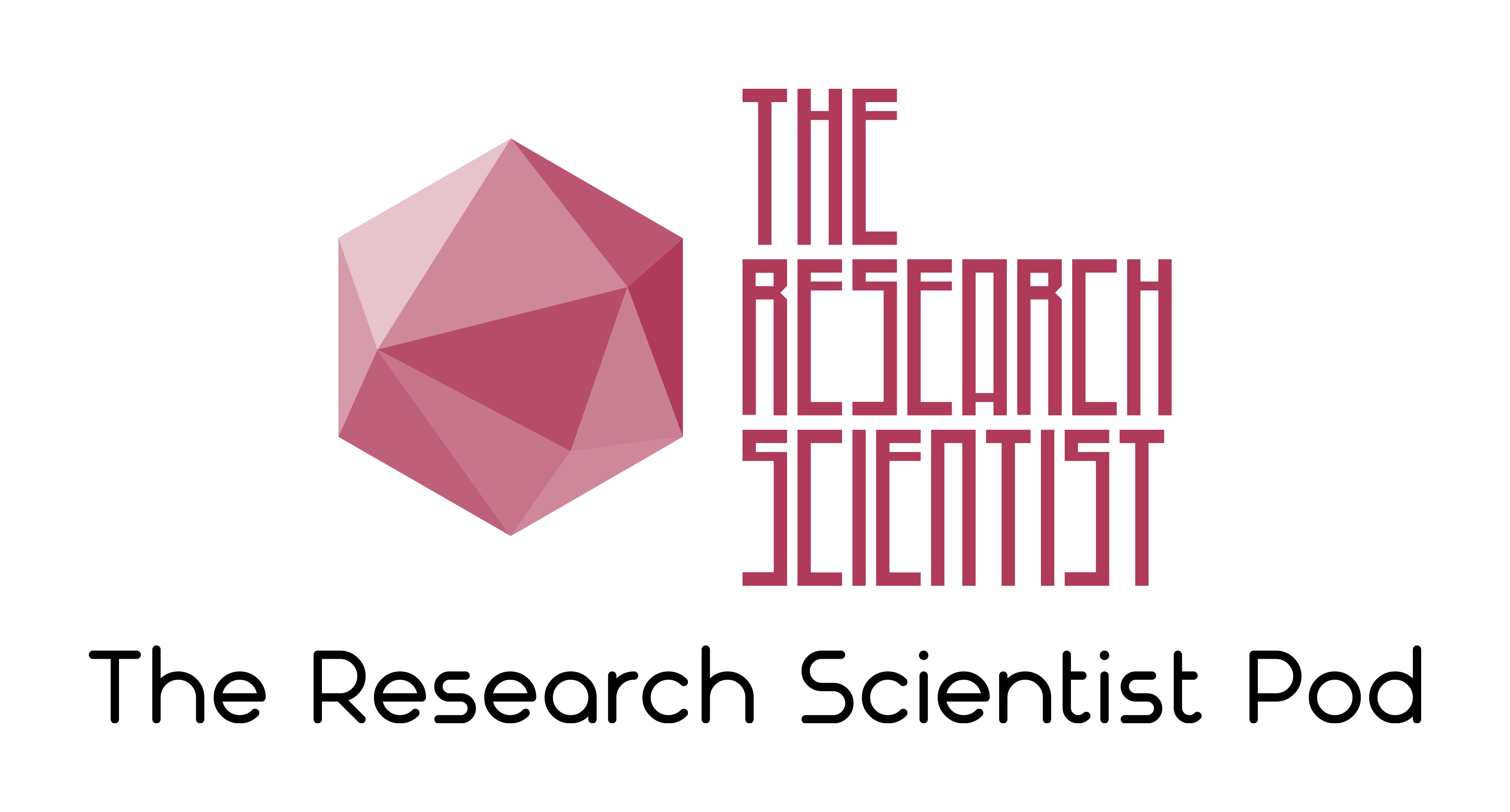
How to Solve Python TypeError: ‘tuple’ object does not support item assignment
by Suf | Programming , Python , Tips
Tuples are immutable objects, which means you cannot change them once created. If you try to change a tuple in place using the indexing operator [], you will raise the TypeError: ‘tuple’ object does not support item assignment.
To solve this error, you can convert the tuple to a list, perform an index assignment then convert the list back to a tuple.
This tutorial will go through how to solve this error and solve it with the help of code examples.
Table of contents
Typeerror: ‘tuple’ object does not support item assignment.
Let’s break up the error message to understand what the error means. TypeError occurs whenever you attempt to use an illegal operation for a specific data type.
The part 'tuple' object tells us that the error concerns an illegal operation for tuples.
The part does not support item assignment tells us that item assignment is the illegal operation we are attempting.
Tuples are immutable objects, which means we cannot change them once created. We have to convert the tuple to a list, a mutable data type suitable for item assignment.
Let’s look at an example of assigning items to a list. We will iterate over a list and check if each item is even. If the number is even, we will assign the square of that number in place at that index position.
Let’s run the code to see the result:
We can successfully do item assignments on a list.
Let’s see what happens when we try to change a tuple using item assignment:
We throw the TypeError because the tuple object is immutable.
To solve this error, we need to convert the tuple to a list then perform the item assignment. We will then convert the list back to a tuple. However, you can leave the object as a list if you do not need a tuple.
Let’s run the code to see the updated tuple:
Congratulations on reading to the end of this tutorial. The TypeError: ‘tuple’ object does not support item assignment occurs when you try to change a tuple in-place using the indexing operator [] . You cannot modify a tuple once you create it. To solve this error, you need to convert the tuple to a list, update it, then convert it back to a tuple.
For further reading on TypeErrors, go to the article:
- How to Solve Python TypeError: ‘str’ object does not support item assignment
To learn more about Python for data science and machine learning, go to the online courses page on Python for the most comprehensive courses available.
Have fun and happy researching!
Share this:
- Click to share on Facebook (Opens in new window)
- Click to share on LinkedIn (Opens in new window)
- Click to share on Reddit (Opens in new window)
- Click to share on Pinterest (Opens in new window)
- Click to share on Telegram (Opens in new window)
- Click to share on WhatsApp (Opens in new window)
- Click to share on Twitter (Opens in new window)
- Click to share on Tumblr (Opens in new window)
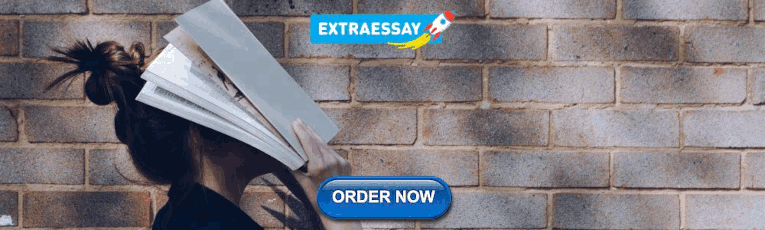
IMAGES
VIDEO
COMMENTS
array[0][0] = 0*0 >> TypeError: 'int' object does not support item assignment Since array[0] is an integer, you can't use the second [0]. There is nothing there to get. So, like Ashalynd said, the array = x*y seems to be the problem. Depending on what you really want to do, there could be many solutions.
However, tuples are immutable, and you cannot perform such an assignment: >>> x[0] = 0 Traceback (most recent call last): File "<stdin>", line 1, in <module> TypeError: 'tuple' object does not support item assignment You can fix this issue by using a list instead.
The TypeError: 'int' object does not support item assignment occurs when you try to change the elements of an integer using indexing. Integers are single values and are not indexable. ... How to Solve Python TypeError: 'tuple' object does not support item assignment; To learn more about Python for data science and machine learning, ...
In this video, we delve into the common Python error message: 'TypeError: 'int' object does not support item assignment.' Learn why this error occurs, how to...
Lists and tuples are arguably Python's most versatile, useful data types. You will find them in virtually every nontrivial Python program. Here's what you'll learn in this tutorial: You'll cover the important characteristics of lists and tuples. You'll learn how to define them and how to manipulate them.
I have a raster layer of Type Float32, and another raster output of Type Integer created from this raster layer outside the python code. I want to scan every column wise, and pick up the Float raster minimum value location within a 5 neighbourhood of Integer raster value of 1 in every column and assign the value 1 at this minimum value location ...
Here are the most common root causes of "int object does not support item assignment", which include the following: Attempting to modify an integer directly. Using an integer where a sequence is expected. Not converting integer objects to mutable data types before modifying them. Using an integer as a dictionary key.
In order to fix it, one need to know what expected output is. Currently that One is just you. You initialized app as an integer in app=0. Later in the code you called app in app [i], which you can only do with list items and dictionaries, not integers. I'm not 100% sure what you were trying to do, but if you want to create a list of numbers ...
#pythonforbeginners Learn how to solve the common Python error "TypeError 'int' object does not support item assignment" with practical examples and step-by-...
If the variable stores a None value, we set it to an empty dictionary. # Track down where the variable got assigned a None value You have to figure out where the variable got assigned a None value in your code and correct the assignment to a list or a dictionary.. The most common sources of None values are:. Having a function that doesn't return anything (returns None implicitly).
Re: 'int' object does not support item assignment. Wed May 24, 2017 10:46 am. Not sure how you figured out that `s1 = 60,2` would create a 60x2 array, that command creates a tuple with the value (60, 2) If you want to create an array of a pre-defined size where each element is preset to zero the following code will work. Code: Select all. w = 60.
We accessed the first nested array (index 0) and then updated the value of the first item in the nested array.. Python indexes are zero-based, so the first item in a list has an index of 0, and the last item has an index of -1 or len(a_list) - 1. # Checking what type a variable stores The Python "TypeError: 'float' object does not support item assignment" is caused when we try to mutate the ...
Once we have a list, we can update the item at the specified index and optionally convert the result back to a tuple. Python indexes are zero-based, so the first item in a tuple has an index of 0, and the last item has an index of -1 or len(my_tuple) - 1. # Constructing a new tuple with the updated element Alternatively, you can construct a new tuple that contains the updated element at the ...
Python Scipy Python Python Tkinter Batch PowerShell Python Pandas Numpy Python Flask Django Matplotlib Docker Plotly Seaborn Matlab Linux Git C Cpp HTML JavaScript jQuery Python Pygame TensorFlow TypeScript Angular React CSS PHP Java Go Kotlin Node.js Csharp Rust Ruby Arduino MySQL MongoDB Postgres SQLite R VBA Scala Raspberry Pi
27. You're passing an integer to your function as a. You then try to assign to it as: a[k] = ... but that doesn't work since a is a scalar... It's the same thing as if you had tried: That statement doesn't make much sense and python would yell at you the same way (presumably). Also, ++k isn't doing what you think it does -- it's parsed as ...
Traceback (most recent call last): File "C:\ProgramData\Anaconda3\lib\multiprocessing\pool.py", line 119, in worker result = (True, func(*args, **kwds)) File "C ...
The TypeError: 'set' object does not support item assignment occurs when you try to change the elements of a set using indexing. The set data type is not indexable. To perform item assignment you should convert the set to a list, perform the item assignment then convert the list back to a set.
BTW, personally, I would use a one-dimensional numpy array as your matrix rather than any kind of Python list at all, and access with something like matrix[(y*height) + x + subpixel_offset], where subpixel_offset is 0/1/2 for r/g/b. Or, depending on your access patterns, you might put the r/g/b offset at a different place and multiply (x*y) by it.
greet[0] = 'J'. TypeError: 'str' object does not support item assignment. To fix this error, you can create a new string with the desired modifications, instead of trying to modify the original string. This can be done by calling the replace() method from the string. See the example below: old_str = 'Hello, world!'.
5. Strings in Python are immutable (you cannot change them inplace). What you are trying to do can be done in many ways: Copy the string: foo = 'Hello'. bar = foo. Create a new string by joining all characters of the old string: new_string = ''.join(c for c in oldstring) Slice and copy:
Tuples are immutable objects, which means we cannot change them once created. We have to convert the tuple to a list, a mutable data type suitable for item assignment. Example. Let's look at an example of assigning items to a list. We will iterate over a list and check if each item is even.