Home » JavaScript Tutorial » JavaScript Constructor Function
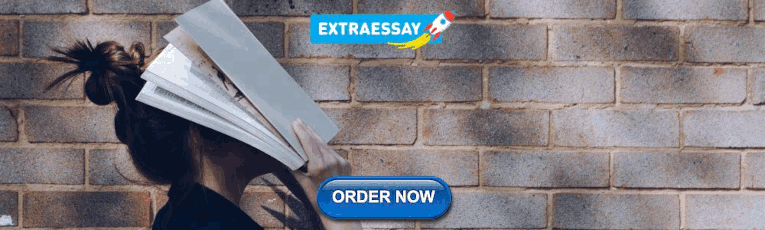
JavaScript Constructor Function
Summary : in this tutorial, you’ll learn about the JavaScript constructor function and how to use the new keyword to create an object.
Introduction to JavaScript constructor functions
In the JavaScript objects tutorial , you learned how to use the object literal syntax to create a new object.
For example, the following creates a new person object with two properties firstName and lastName :
In practice, you often need to create many similar objects like the person object.
To do that, you can use a constructor function to define a custom type and the new operator to create multiple objects from this type.
Technically speaking, a constructor function is a regular function with the following convention:
- The name of a constructor function starts with a capital letter like Person , Document , etc.
- A constructor function should be called only with the new operator.
Note that ES6 introduces the class keyword that allows you to define a custom type. Classes are just syntactic sugar over the constructor functions with some enhancements.
The following example defines a constructor function called Person :
In this example, the Person is the same as a regular function except that its name starts with the capital letter P .
To create a new instance of the Person , you use the new operator:
Basically, the new operator does the following:
- Create a new empty object and assign it to the this variable.
- Assign the arguments 'John' and 'Doe' to the firstName and lastName properties of the object.
- Return the this value.
It’s functionally equivalent to the following:
Therefore, the following statement:
… returns the same result as the following statement:
However, the constructor function Person allows you to create multiple similar objects. For example:
Adding methods to JavaScript constructor functions
An object may have methods that manipulate its data. To add a method to an object created via the constructor function, you can use the this keyword. For example:
Now, you can create a new Person object and invoke the getFullName() method:
The problem with the constructor function is that when you create multiple instances of the Person , the this.getFullName() is duplicated in every instance, which is not memory efficient.
To resolve this, you can use the prototype so that all instances of a custom type can share the same methods.
Returning from constructor functions
Typically, a constructor function implicitly returns this that set to the newly created object. But if it has a return statement, then here are the rules:
- If return is called with an object, the constructor function returns that object instead of this .
- If return is called with a value other than an object, it is ignored.
Calling a constructor function without the new keyword
Technically, you can call a constructor function like a regular function without using the new keyword like this:
In this case, the Person just executes like a regular function. Therefore, the this inside the Person function doesn’t bind to the person variable but the global object .
If you attempt to access the firstName or lastName property, you’ll get an error:
Similarly, you cannot access the getFullName() method since it’s bound to the global object.
To prevent a constructor function from being invoked without the new keyword, ES6 introduced the new.target property.
If a constructor function is called with the new keyword, the new.target returns a reference of the function. Otherwise, it returns undefined .
The following adds a statement to the Person function to show the new.target to the console:
The following returns undefined because the Person constructor function is called like a regular function:
However, the following returns a reference to the Person function because it’s called the new keyword:
By using the new.target , you can force the callers of the constructor function to use the new keyword. Otherwise, you can throw an error like this:
Alternatively, you can make the syntax more flexible by creating a new Person object if the users of the constructor function don’t use the new keyword:
This pattern is often used in JavaScript libraries and frameworks to make the syntax more flexible.
- JavaScript constructor function is a regular function used to create multiple similar objects.
Popular Tutorials
Popular examples, reference materials, learn python interactively, js introduction.
- Getting Started
- JS Variables & Constants
- JS console.log
- JavaScript Data types
- JavaScript Operators
- JavaScript Comments
- JS Type Conversions
JS Control Flow
- JS Comparison Operators
- JavaScript if else Statement
- JavaScript for loop
- JavaScript while loop
- JavaScript break Statement
- JavaScript continue Statement
- JavaScript switch Statement
JS Functions
- JavaScript Function
- Variable Scope
- JavaScript Hoisting
- JavaScript Recursion
JavaScript Objects
- JavaScript Methods & this
- JavaScript Constructor
- JavaScript Getter and Setter
- JavaScript Prototype
- JavaScript Array
- JS Multidimensional Array
- JavaScript String
- JavaScript for...in loop
- JavaScript Number
- JavaScript Symbol
Exceptions and Modules
- JavaScript try...catch...finally
- JavaScript throw Statement
- JavaScript Modules
- JavaScript ES6
- JavaScript Arrow Function
- JavaScript Default Parameters
- JavaScript Template Literals
- JavaScript Spread Operator
- JavaScript Map
- JavaScript Set
- Destructuring Assignment
JavaScript Classes
- JavaScript Inheritance
- JavaScript for...of
- JavaScript Proxies
JavaScript Asynchronous
- JavaScript setTimeout()
- JavaScript CallBack Function
- JavaScript Promise
- Javascript async/await
- JavaScript setInterval()
Miscellaneous
- JavaScript JSON
- JavaScript Date and Time
- JavaScript Closure
JavaScript this
- JavaScript use strict
- Iterators and Iterables
- JavaScript Generators
- JavaScript Regular Expressions
- JavaScript Browser Debugging
- Uses of JavaScript
JavaScript Tutorials
- JavaScript Object.getPrototypeOf()
JavaScript Class Inheritance
JavaScript Constructor Function
In JavaScript, a constructor function is used to create and initialize objects .
Here is a simple example of a constructor function. Read the rest of the tutorial for more.
Here, Person() is an object constructor function. And, we use the new keyword to create an object from a constructor function.
- Create Multiple Objects With Constructor Function
In JavaScript, you can create multiple objects from a constructor function. For example,
In the above program, we created two objects ( person1 and person2 ) using the same constructor function.
In JavaScript, when this keyword is used in a constructor function, this refers to the specific object in which it is created. For example,
Hence, when an object accesses the name property of the constructor function, it can directly access it as person1.name .
- JavaScript Constructor Function Parameters
You can also create a constructor function with parameters. For example,
In the above example, we have passed arguments to the constructor function during the creation of the object.
This allows each object to have different properties:
Constructor Function vs. Object Literal
Object literals are used to create a single object.
On the other hand, constructor functions are useful if you want to create multiple objects. For example,
Objects created from constructor functions are unique. Thus, you can add a new property to a particular object that isn't accessible to other objects. For example,
Here, the age property and the greet() method are unique to person1 and are thus unavailable to person2 .
On the other hand, when an object is created with an object literal, any object variable derived from that object will act as a clone of the original object.
Hence, changes you make in one object will be reflected in the other. For example,
- JavaScript Built-In Constructors
JavaScript also has built-in constructors to create objects of various types. Some of them are:
Example: JavaScript Built-In Constructors
Note : You should not declare strings , numbers , and boolean values as objects because they slow down the program. Instead, declare them as primitive types using code such as let name = "John" , let number = 57 , etc.
More on Constructor Functions
You can also add properties and methods to a constructor function using a prototype . For example,
To learn more about prototypes, visit JavaScript Prototype .
In JavaScript, the class keyword was introduced in ES6 (ES2015) to provide a more convenient syntax for defining objects and their behavior.
Classes serve as blueprints for creating objects with similar properties and methods. They resemble the functionality of constructor functions in JavaScript.
To learn more, visit JavaScript Classes .
- JavaScript Function and Function Expressions
Table of Contents
- Introduction
Sorry about that.
Related Tutorials
JavaScript Tutorial
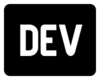
DEV Community

Posted on May 9, 2020 • Updated on Jun 12, 2020
An Easy Guide To Understanding Constructors In JavaScript
Table of contents.
- An Introduction To Constructors
- Functions, Constructors And The new Operator
- Constructors And Prototypal Inheritance
- JavaScript's Built-In Constructors
- Closing Thoughts
1. An Introduction To Constructors
In the previous article in this series, we looked at prototypal inheritance in JavaScript and talked about important object-oriented (OOP) JavaScript concepts like the prototype, the prototype chain, inheritance, and more. We also looked at how to set an object's prototype using its __proto__ property ( we noted that this is not the recommended way. ) and dealt with the this variable in detail. You can read through this article below:

Understanding Prototypal Inheritance In JavaScript
Lawrence eagles ・ apr 23 '20 ・ 13 min read.
In this article, we would pick up where we left off by looking at the recommended ways to set an object's prototype in JavaScript. While there are several ways to do this, our focus here is on function constructors.
✨ We will deal with the other recommended methods in the upcoming articles in this series. Talking in detail about all of them; in a single article would make it very long and grueling to read!
Constructors
Constructors in JavaScript are special functions that are used to construct objects. This topic may appear difficult and intimidating but it is actually very simple.
💡 The key to understanding constructors is to know that they are actually normal JavaScript functions. What makes them special is that they are always invoked along with a very powerful operator in JavaScript called the new operator.
Kindly run the code below and consider its result.
Our small contrieved example above creates a new object and stores a reference to it in the Lawrence variable. This object has all the properties specified in the Person constructor. The Person function itself is a normal JavaScript function; what gives it the power of a constructor ( the power to construct objects ) is this line of code:
✨ The new operator modifies the behaviour of the function it operates on. Using this along with some JavaScript design patterns, we are able to create powerful constructors. We will elaborate on this in the next section.
2. Functions, Constructors And The new Operator
In section 1 we learned that when the Person constructor, (or any other constructor) is invoked without the new operator it is invoked as a regular JavaScript function. In this section, we will elaborate on this with code examples. Kindly consider the code below.
Above is the declaration of the Person function. We can notice two things from it viz:
- It sets some properties e.g firstname, lastname, occupation, and gender to the object the this variable binds (or is pointing) to. In this case the global object.
💡 As mentioned in the previous article in this series, one rule of the this variable is that when it is used inside a function it points to the global object but when it is used inside a method (a function in an object) it would point to that object
If this is not very clear to you, feel free to visit my previous article on OOP JavaScript. I have already provided a link to it in section 1. However, here is a quick recap. Kindly run the code below and consider its result
The above example shows that the this variable inside a function is pointing to the global object.
- Another thing that should be pretty obvious about the Person function is that it does not have a return statement hence when invoked it would return undefined.
💡 The JavaScript engine would return undefined from any function that does not return a value. This behaviour is leveraged in creating constructors as we are able to modify what that function returns using the new operator. Hence it is a common pattern in JavaScript, that constructors do not have a return statement
The New Operator
This is a very powerful JavaScript operator that has the ability to modify certain behaviours of a function. The new operator can be very confusing and somewhat intimidating at first.
✨ The key to understanding it is to always see it as another regular JavaScript operator. Hence, a good understanding of operators in JavaScript is necessary to grasp the power of this operator .
Operators are special JavaScript functions that are syntactically different from regular functions. They are not like a regular JavaScript functions objects hence passing them to console.dir() would throw an error. You can see some code examples below. Kindly run the codes below and consider the results:
💡 The console.dir() displays an interactive list of the properties of the specified JavaScript object

You can see all the properties of the tellDevName function and the Date constructor when you run the code but if you uncomment the lines where I passed an operator as a parameter, and try to run the code, runkit would throw an error, this tells us that they are not regular function objects.
Operators much like regular functions take parameters (which are called operands) but unlike regular functions, they give us a convenient syntax which can be in the form of any of the three notations below:
- Infix Notation: In this notation, operators are placed between their operands. Kindly consider the code below:
In our examples above each operator sits between two parameters (operands) and returns a value. Learn more about the infix notation here
- Postfix Notation: In this Notation, the operators follow their operands. Kindly consider the codes below:
Above is a small example that finds the even number from a list of numbers. But what concerns us from this example is the increment operator. There is also the decrement operator. Learn more about the postfix notation Kindly consider the code below:
- Prefix Notation: In this notation, the operator precedes its operands. Learn more about the prefix notation Kindly consider the codes below:
From our examples above we can see that the new operator uses the prefix notation it takes a function (constructor) invocation and returns a newly constructed object.
🎉 I do hope that our succinct discourse on operators, would make you understand the new operator better and hence further your understanding of function constructors
With our understanding of operators, we can now clearly see that the new operator actually takes a function (constructor) invocation as its parameter(operand) it then performs some operations on it and returns a value. Below are the operations of the new operator on a function constructor.
- Creates an empty object and binds (points) the this variable to the newly created object.
- Returns the object the this variable binds to (the newly created object) if the function doesn't return its own object (this is why constructors should not have a return statement) . Kindly run the codes below and consider the results:
From the code example above I have deliberately given 2 of the 3 functions the same name but since JavaScript is case sensitive they are two different functions. Notice that the first letter of the constructor's name is capitalized while the regular function name is all lowercase.
💡 We use this pattern in JavaScript to differentiate constructors from regular functions. The first letter of a constructor's name is always capitalized. This would also serve as a reminder for you to use the new operator with every constructor. In our code example above, Person is the constructor and person is the regular function.
We can see from the result of the code above that the regular function returns undefined as expected but the constructor returns a new object created by the new operator which also binds the this variable in that constructor to this object.
💥 Also notice the BadPerson constructor that returns its own object fails to return the object created from the new operator. This is a pitiful we must avoid. Again, as a rule, a constructor should not have a return statement❗

JavaScript Design Patterns For Creating Constructors
With our knowledge of constructors and the new operator, we can easily add properties to the newly constructed object . Here is a common JavaScript pattern for this. Kindly consider the code below
The only limitation here is that any object created by this constructor is always going to have these properties. In other to make the object properties dynamic, we can pass them as parameters to the constructor (since constructors are regular functions in the first place). Kindly run the codes below and consider its result:
From the results of running the code above, we can see that the arguments passed to each constructor, when invoked with the new operator are used to set up the properties of the newly constructed objects. You can read more about the new operator at MDN .
- Lastly the new operator links (sets) the prototype of the newly created object to another object. In our introduction, we said we were going to talk about the recommended ways to set an object's prototype and our focus was on function constructors. This point brings our long discourse back to the subject matter. Let's talk more about it in the next section.
3. Constructors And Prototypal Inheritance
In JavaScript, every function has a property called the prototype . This sits as an empty object in the function and remains dormant throughout the life of that function. It would only become active and quite useful if that function is used as a constructor.
💡 The prototype property of a function (which is an object in JavaScript) is not the prototype of that function object but it acts as the prototype of any object constructed with that function when it is used as a constructor. The naming is a little confusing but we love our JavaScript 😉
Kindly run the code below and consider its result:
From the results of the code above we can see that all the objects constructed with the Person constructor have access to the getPersonbio method which sits in the prototype property of the Person constructor. As we have noted above this property becomes the prototype of each object.
✨ The details of how the search is made down the prototype chain in other for all the constructed object to access the getPersonBio method has already been discussed in the previous article. I would kindly suggest you take a look at it if this section is not very clear to you.
4. JavaScript's Built-In Constructors
JavaScript comes with some built-in constructors. If you are a JavaScript developer, there is a high probability that you have used some of them. Kindly run the code below and consider its result:
From running the codes above we can see that each returns an object because every constructor in JavaScript returns an object. You can learn more about each of these built-in constructors from the links below: Number Constructor String Constructor Date Constructor
✨ These are just a selected few you can get a more robots list here
5. Closing Thoughts
I do hope you followed through to this point. If you did you are really appreciated. It has been a long discussion, and I hope you got a thing or two. If so, I would now be looking forward to hearing your opinions, comments, questions, or requests (in case anything is not clear) in the comments section below.
Top comments (2)
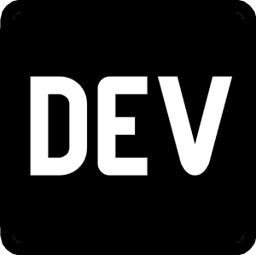
Templates let you quickly answer FAQs or store snippets for re-use.

- Joined Sep 30, 2022
Hello, First thanks a lot for these useful article, really help me understand the concepts. Just a little thing I can't figure out: in the example of section 3. why does the results log the following: "Hello my name is Eagles Lawrence I am a Software Developer" "Developer's bio:" undefined
I understand the first line but why is "Developer's bio:" after sentence "Hello my name is ..." ? And why does it returns undefined after? Hope you can help me see this clearer Thanks

- Joined Jan 23, 2023
Its because the function ( getPersonBio ) just has a console.log which gets executed first and not returning anything so undefined later
Are you sure you want to hide this comment? It will become hidden in your post, but will still be visible via the comment's permalink .
Hide child comments as well
For further actions, you may consider blocking this person and/or reporting abuse
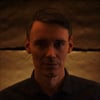
Composition in Rust and Python
Antonov Mike - May 12

Warum ist die Planung von Büroräumen wichtig?
Mark Albukerky - May 17

Complete portfolio using HTML
chaitanya kolla - May 12

Constructor, operator "new"
The regular {...} syntax allows us to create one object. But often we need to create many similar objects, like multiple users or menu items and so on.
That can be done using constructor functions and the "new" operator.
Constructor function
Constructor functions technically are regular functions. There are two conventions though:
- They are named with capital letter first.
- They should be executed only with "new" operator.
For instance:
When a function is executed with new , it does the following steps:
- A new empty object is created and assigned to this .
- The function body executes. Usually it modifies this , adds new properties to it.
- The value of this is returned.
In other words, new User(...) does something like:
So let user = new User("Jack") gives the same result as:
Now if we want to create other users, we can call new User("Ann") , new User("Alice") and so on. Much shorter than using literals every time, and also easy to read.
That’s the main purpose of constructors – to implement reusable object creation code.
Let’s note once again – technically, any function (except arrow functions, as they don’t have this ) can be used as a constructor. It can be run with new , and it will execute the algorithm above. The “capital letter first” is a common agreement, to make it clear that a function is to be run with new .
If we have many lines of code all about creation of a single complex object, we can wrap them in an immediately called constructor function, like this:
This constructor can’t be called again, because it is not saved anywhere, just created and called. So this trick aims to encapsulate the code that constructs the single object, without future reuse.
Constructor mode test: new.target
The syntax from this section is rarely used, skip it unless you want to know everything.
Inside a function, we can check whether it was called with new or without it, using a special new.target property.
It is undefined for regular calls and equals the function if called with new :
That can be used inside the function to know whether it was called with new , “in constructor mode”, or without it, “in regular mode”.
We can also make both new and regular calls to do the same, like this:
This approach is sometimes used in libraries to make the syntax more flexible. So that people may call the function with or without new , and it still works.
Probably not a good thing to use everywhere though, because omitting new makes it a bit less obvious what’s going on. With new we all know that the new object is being created.
Return from constructors
Usually, constructors do not have a return statement. Their task is to write all necessary stuff into this , and it automatically becomes the result.
But if there is a return statement, then the rule is simple:
- If return is called with an object, then the object is returned instead of this .
- If return is called with a primitive, it’s ignored.
In other words, return with an object returns that object, in all other cases this is returned.
For instance, here return overrides this by returning an object:
And here’s an example with an empty return (or we could place a primitive after it, doesn’t matter):
Usually constructors don’t have a return statement. Here we mention the special behavior with returning objects mainly for the sake of completeness.
By the way, we can omit parentheses after new :
Omitting parentheses here is not considered a “good style”, but the syntax is permitted by specification.
Methods in constructor
Using constructor functions to create objects gives a great deal of flexibility. The constructor function may have parameters that define how to construct the object, and what to put in it.
Of course, we can add to this not only properties, but methods as well.
For instance, new User(name) below creates an object with the given name and the method sayHi :
To create complex objects, there’s a more advanced syntax, classes , that we’ll cover later.
- Constructor functions or, briefly, constructors, are regular functions, but there’s a common agreement to name them with capital letter first.
- Constructor functions should only be called using new . Such a call implies a creation of empty this at the start and returning the populated one at the end.
We can use constructor functions to make multiple similar objects.
JavaScript provides constructor functions for many built-in language objects: like Date for dates, Set for sets and others that we plan to study.
In this chapter we only cover the basics about objects and constructors. They are essential for learning more about data types and functions in the next chapters.
After we learn that, we return to objects and cover them in-depth in the chapters Prototypes, inheritance and Classes .
Two functions – one object
Is it possible to create functions A and B so that new A() == new B() ?
If it is, then provide an example of their code.
Yes, it’s possible.
If a function returns an object then new returns it instead of this .
So they can, for instance, return the same externally defined object obj :
Create new Calculator
Create a constructor function Calculator that creates objects with 3 methods:
- read() prompts for two values and saves them as object properties with names a and b respectively.
- sum() returns the sum of these properties.
- mul() returns the multiplication product of these properties.
Run the demo
Open a sandbox with tests.
Open the solution with tests in a sandbox.
Create new Accumulator
Create a constructor function Accumulator(startingValue) .
Object that it creates should:
- Store the “current value” in the property value . The starting value is set to the argument of the constructor startingValue .
- The read() method should use prompt to read a new number and add it to value .
In other words, the value property is the sum of all user-entered values with the initial value startingValue .
Here’s the demo of the code:
- If you have suggestions what to improve - please submit a GitHub issue or a pull request instead of commenting.
- If you can't understand something in the article – please elaborate.
- To insert few words of code, use the <code> tag, for several lines – wrap them in <pre> tag, for more than 10 lines – use a sandbox ( plnkr , jsbin , codepen …)
Lesson navigation
- © 2007—2024 Ilya Kantor
- about the project
- terms of usage
- privacy policy
Full Stack Development Articles
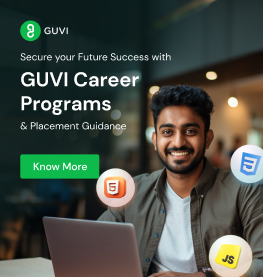
Get In Touch For Details! Request More Information
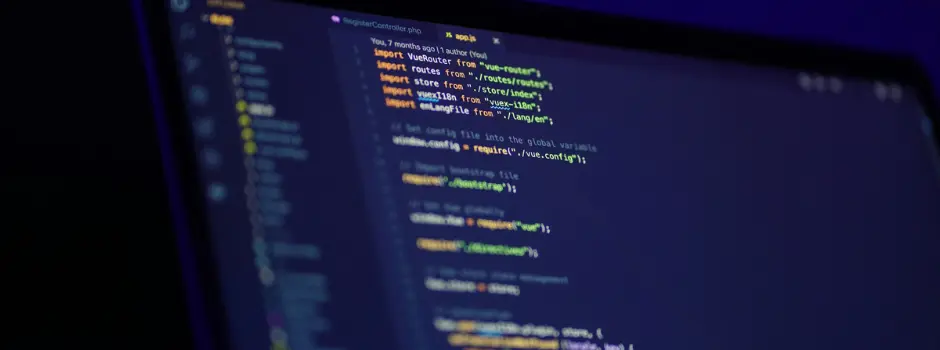
Constructors in JavaScript: 6 Uses Every Top Programmer Must Know
Mar 19, 2024 4 Min Read 752 Views
(Last Updated)
In the world of JavaScript programming, constructors play a crucial role. They are special functions that are used to create and initialize objects.
When an object is created using the new keyword, the constructor gets called, allowing you to set values for the object’s properties and perform any necessary initialization tasks.
In this comprehensive guide, we will explore the concept of constructors in JavaScript, understand how they work, and examine various examples and use cases.
Table of contents
- What is a Constructor in JavaScript?
- How Constructors in JavaScript Work?
- How to use Constructors in JavaScript:
- 1) Using the "this" Keyword in Constructors
- 2) Creating Multiple Objects with Constructors
- 3) Constructor with Parameters
- 4) Constructor vs Object Literal
- 5) Understanding Object Prototypes
- 6) Built-in Constructors in JavaScript
- Takeaways...
- What are constructors in JavaScript?
- What is the difference between a constructor and a class in JavaScript?
- What are constructors in JavaScript used for?
- Can we call multiple constructors?
A constructor is a special function in JavaScript that is used to create and initialize objects. It is typically defined within a class or an object blueprint. When a new object is created using the new keyword and a constructor function, the constructor gets invoked, and a new instance of the object is created.
The purpose of a constructor is to set the initial state of the object and define its properties and methods. It allows you to specify the values of the object’s properties when it is created, making it easier to work with and manipulate the object later on.
Must Read: Master JavaScript Frontend Roadmap: From Novice to Expert [2024]
Before we move to the next section, make sure that you are strong in the full-stack development basics. If not, consider enrolling for a professionally certified online full-stack web development course by a recognized institution that can also offer you an industry-grade certificate that boosts your resume.
When a constructor is called in JavaScript, several operations take place:
- A new empty object is created.
- The this keyword starts referring to the newly created object, making it the current instance object.
- The properties and methods defined within the constructor are attached to the object.
- The new object is returned as the result of the constructor call.
This sequence of operations ensures that a new object with the desired properties and behaviors is created and ready to be used.
Also Read: All About Loops in JavaScript: A Comprehensive Guide
Given below is the code to initialize two user instances as shown in the image above:
Let’s explore some examples of constructors in JavaScript to understand how they are used.
1) Using the “this” Keyword in Constructors
In a constructor, the this keyword refers to the newly created object. You can use the this keyword to define and assign values to the object’s properties. Here’s an example:
In the example above, the Car constructor takes three parameters: make , model , and year . The this keyword is used to assign the parameter values to the corresponding properties of the newly created myCar object.
By using the this keyword, you ensure that the values are specific to each instance of the Car object. If you were to create multiple car objects using the same constructor, each object would have its own unique set of property values.
Must Read: 4 Key Differences Between == and === Operators in JavaScript
Constructors are not limited to creating just a single object. You can create multiple objects using the same constructor. Each object will have its own set of properties and values. Here’s an example:
In the above example, the Circle constructor takes a radius parameter and defines a getArea method. The getArea method calculates and returns the area of the circle based on its radius.
By creating multiple circle objects using the new keyword and the Circle constructor, we can compute and output the areas of different circles. Each object has its own radius property and getArea method, ensuring that the calculations are specific to each circle.
Also Explore: Arrays in JavaScript: A Comprehensive Guide
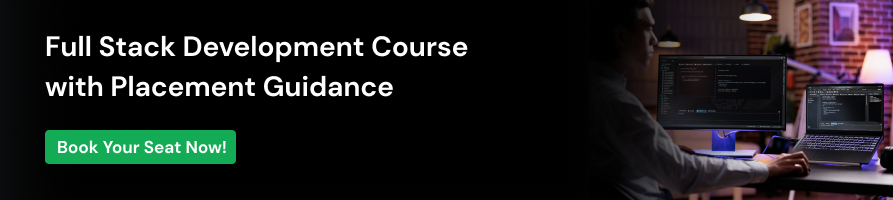
Constructors in JavaScript can accept parameters, allowing you to pass values during object creation. This enables you to set different property values for each object based on the provided arguments.
Let’s consider an example of a Rectangle constructor:
In the above example, the Rectangle constructor takes two parameters: width and height . The constructor assigns the respective parameter values to the width and height properties of the newly created rectangle objects.
By creating multiple rectangles using the new keyword and the Rectangle constructor, we can calculate and output the areas of different rectangles. Each object has its own width and height properties, ensuring that the calculations are specific to each rectangle.
Must Explore: What is jQuery? A Key Concept You Should Know
In JavaScript, you have multiple ways to create objects. While constructors are useful for creating multiple objects with similar properties and behaviors, object literals are handy for creating single objects with specific properties.
Let’s compare the two approaches using an example:
In the example above, we have created three objects: person1 and person2 using the Person constructor, and person3 using an object literal.
The objects created with the constructor have similar properties and behaviors, allowing us to create multiple instances easily. On the other hand, the object created with the object literal approach is a single, standalone object with specific properties.
When deciding whether to use a constructor or an object literal, consider the specific needs of your program. Constructors are ideal when you need to create multiple objects with shared properties and behaviors. Object literals are suitable for creating single, independent objects with unique properties.
Also Explore: HTML Tutorial: A Comprehensive Guide for Web Development
In JavaScript, object prototypes play a significant role in defining shared properties and methods for objects created with a constructor. Prototypes allow objects to inherit properties and methods from a common prototype object.
Let’s consider an example of a Person constructor with a shared method defined in the prototype:
In the above example, the Person constructor creates a person object with name and age properties. The shared greet method is defined in the Person.prototype , allowing all instances of Person objects to access and invoke the method.
By defining methods in the prototype, you ensure that they are shared across all instances of the objects created with the constructor. This improves memory efficiency and allows you to add or modify shared behaviors easily.
JavaScript provides several built-in constructors that you can use to create objects with specific types and functionalities. However, it is generally recommended to use primitive data types where possible for better performance. Here are some examples of built-in constructors:
In the above examples, we create objects using the built-in constructors String , Number , Boolean , Array , and Date . These constructors allow us to work with string, number, boolean, array, and date objects, respectively.
While using these constructors is possible, it is generally recommended to use primitive data types directly, as they offer better performance and are simpler to work with.
If you want to learn more about programming with JavaScript and make a successful career out of it, then you must sign up for the Certified Full Stack Development Course , offered by GUVI, which gives you in-depth knowledge of the practical implementation of all frontend as well as backend development through various real-life FSD projects.
Takeaways…
Constructors in JavaScript are essential for creating and initializing objects. They provide a way to define object blueprints and set initial property values.
By using constructors, you can create multiple objects with shared properties and behaviors, pass parameters during object creation, and leverage prototypes for shared methods.
Understanding constructors enables you to build more robust and flexible applications. Whether you’re creating simple objects or complex data structures, constructors play a vital role in JavaScript programming.
Remember to use constructors wisely, considering the specific needs of your program.
Must Read: Variables and Data Types in JavaScript: A Complete Guide
Constructors in JavaScript are special functions used for initializing objects created with a class or constructor function.
The main difference between a constructor and a class in JavaScript is that a constructor is a function used to create and initialize objects, while a class is a blueprint for creating objects.
Constructors in JavaScript are used to set initial values, assign properties, and perform other setup tasks when creating objects.
In JavaScript, a class can have only one constructor, but you can call multiple constructors using techniques like function overloading or using factory functions.
Career transition
About the Author
Jaishree Tomar
A recent CS Graduate with a quirk for writing and coding, a Data Science and Machine Learning enthusiast trying to pave my own way with tech. I have worked as a freelancer with a UK-based Digital Marketing firm writing various tech blogs, articles, and code snippets. Now, working as a Technical Writer at GUVI writing to my heart’s content!
Did you enjoy this article?
Recommended courses.
- Career Programs
- Micro Courses
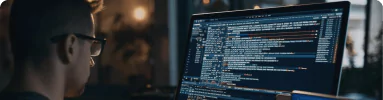
Most Popular

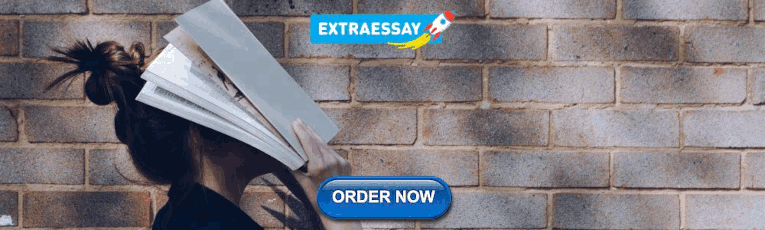
Java Full Stack Development Course
Available in

EMI Options Available

Placement Guidance

1:1 Mentor Doubt Clearing Sessions

MERN Full Stack Development
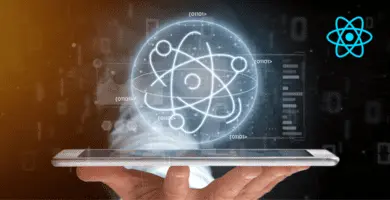
ReactJs Project
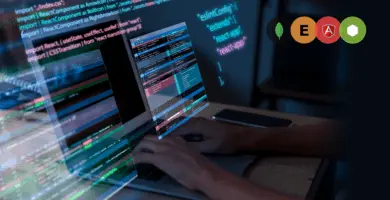
MEAN Stack Course
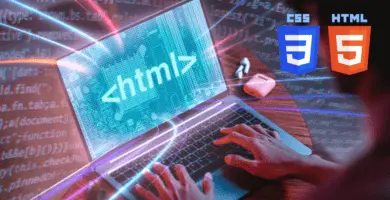
Advanced JavaScript
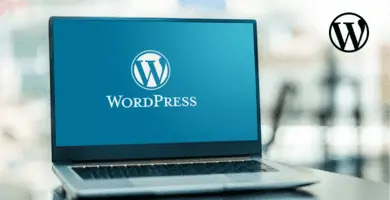
PHP with MySQL
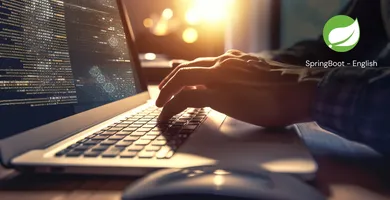
Spring Boot

HTML CSS Project
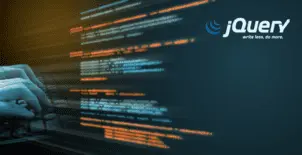
jQuery - Beginners to Advanced
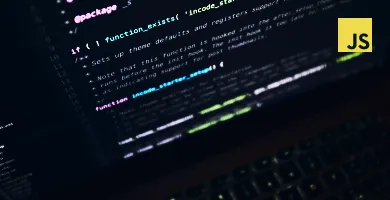
Schedule 1:1 free counselling
Similar Articles
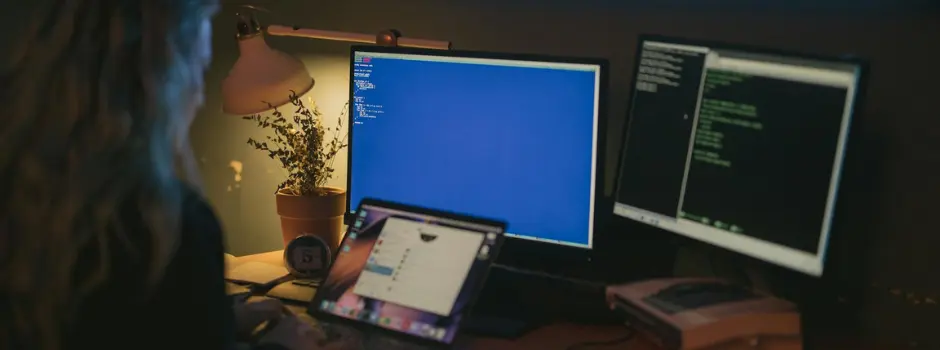
By Lukesh S
21 May, 2024
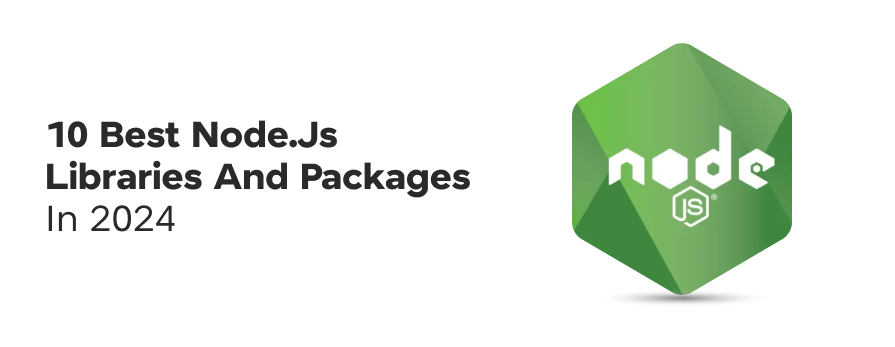
By Isha Sharma
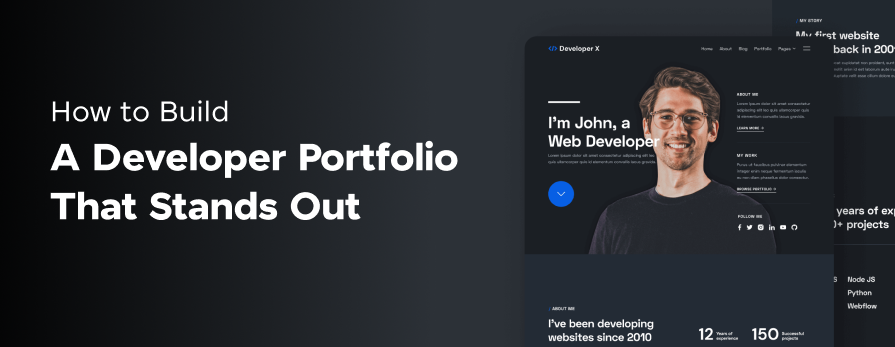
By Meghana D
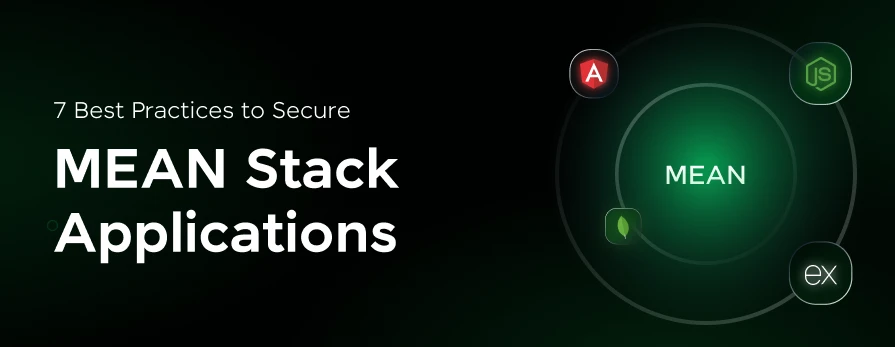
20 May, 2024

18 May, 2024
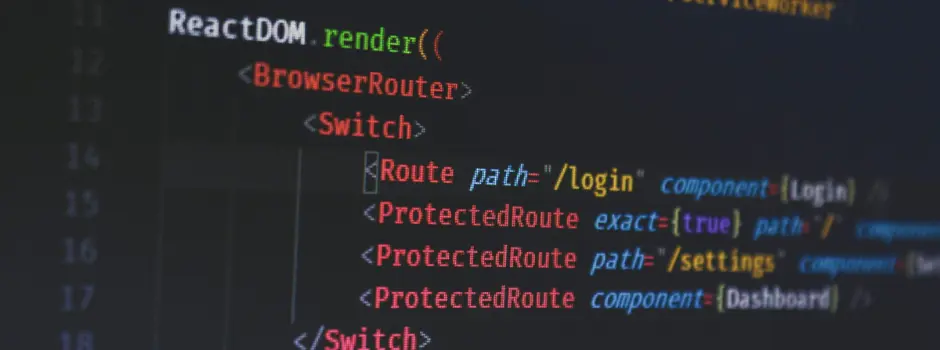
By Jaishree Tomar
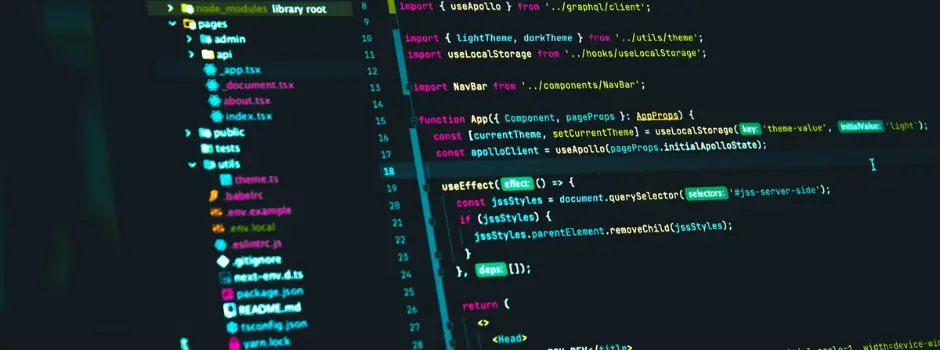
16 May, 2024
JS Reference
Html events, html objects, other references, javascript class constructor.
Create a Car class, and then create an object called "mycar" based on the Car class:
Try it Yourself »
More "Try it Yourself" examples below.
Description
The constructor() method is a special method for creating and initializing objects created within a class.
Note: A class cannot have more than one constructor() method. This will throw a SyntaxError .
You can use the super() method to call the constructor of a parent class (see "More Examples" below).
Browser Support
constructor() is an ECMAScript6 (ES6) feature.
ES6 (JavaScript 2015) is supported in all modern browsers since June 2017:
constructor() is not supported in Internet Explorer.
Technical Details
More examples.
To create a class inheritance, use the extends keyword.
A class created with a class inheritance inherits all the methods from another class:
Create a class named "Model" which will inherit the methods from the "Car" class:
The super() method refers to the parent class.
By calling the super() method in the constructor method, we call the parent's constructor method and get access to the parent's properties and methods.
Related Pages
JavaScript Tutorial: JavaScript Classes
JavaScript Tutorial: JavaScript ES6 (EcmaScript 2015)
JavaScript Reference: The extends Keyword
JavaScript Reference: The super Keyword

COLOR PICKER

Contact Sales
If you want to use W3Schools services as an educational institution, team or enterprise, send us an e-mail: [email protected]
Report Error
If you want to report an error, or if you want to make a suggestion, send us an e-mail: [email protected]
Top Tutorials
Top references, top examples, get certified.
- DSA with JS - Self Paced
- JS Tutorial
- JS Exercise
- JS Interview Questions
- JS Operator
- JS Projects
- JS Examples
- JS Free JS Course
- JS A to Z Guide
- JS Formatter
JavaScript Object Constructors
- JavaScript Set() Constructor
- JavaScript WeakSet() Constructor
- JavaScript String() Constructor
- JavaScript RegExp() Constructor
- JavaScript Symbol() Constructor
- JavaScript Object Accessors
- JavaScript Set constructor Property
- JavaScript Map constructor Property
- JavaScript Map() Constructor Property
- JavaScript Date constructor Property
- JavaScript Array constructor Property
- JavaScript Reflect construct() Method
- JavaScript Symbol constructor Property
- JavaScript String constructor Property
- JavaScript Number constructor Property
- JavaScript RegExp constructor Property
- JavaScript Array() Constructor
- Objects in Javascript
- JavaScript Boolean Constructor Property
Object : An object is the collection of related data or functionality in the form of key . These functionalities usually consist of several functions and variables. All JavaScript values are objects except primitives.
Here, subject and language are the keys and programming and JavaScript are the values . Class: In JavaScript, a class is a kind of function. These classes are similar to normal java classes. The classes are declared with the class keyword like other OOP languages. The class syntax has two components: class declarations and class expressions .
Class declarations:
Here class name is GFG .
Class expressions:
this keyword : The this keyword refers to the object it belongs to, like OOPs languages C++, C#, JAVA etc. this keyword is used in different ways in different areas. While executing a function in JavaScript that has a reference to its current execution context, that is the reference by which the function or data member is called. See the previous example.
Adding property to an object: The property can be added to the object by using dot(.) operator or square bracket. ,
The GFG has two properties “articles” and “quantity”. Now we wish to add one more property name called subject .
Using dot (.) operator
Using square bracket:
Here, subject is the property and ‘JavaScript’ is the value of the property. Adding a property to Constructor: We cannot add a property to an existing constructor like adding a property to an object (see previous point), for adding a property we need to declare under the constructor.
Here, we add a property name G with value “GEEK” , in this case the value “GEEK” is not passed as an argument. Adding a Method to an Object: We can add a new method to an existing object.
Here, the object is GFG. Adding a Method to Constructor:
Here, in the last line a method is added to an object.
Constructor: A constructor is a function that initializes an object. In JavaScript the constructors are more similar to normal java constructor. Object constructor : In JavaScript, there is a special constructor function known as Object() is used to create and initialize an object. The return value of the Object() constructor is assigned to a variable. The variable contains a reference to the new object. We need an object constructor to create an object “type” that can be used multiple times without redefining the object every time.
Here, GFG is the constructor name and A, B, C are the arguments of the constructor.
Instantiating an object constructor: There are two ways to instantiate object constructor,
In 1st method, the object is created by using new keyword like normal OOP languages, and “Java”, “JavaScript”, “C#” are the arguments, that are passed when the constructor is invoked. In 2nd method, the object is created by using curly braces “{ }” .
Assigning properties to the objects: There are two ways to assigning properties to the objects.
- Using dot (.) operator:
- Using third bracket:
Example 1: This example shows object creation by using new keyword and assigning properties to the object using dot(.) operator.
Example 2: This example shows object creation using curly braces and assigning properties to the object using third bracket “[]” operator.
Example 3: This example shows how to use function() with object constructor.
Example: Another way to create a function using function name.
Please Login to comment...
Similar reads.
- javascript-oop
- Web Technologies
Improve your Coding Skills with Practice
What kind of Experience do you want to share?
- skip navigation All Products Product Bundles DevCraft All Telerik .NET tools and Kendo UI JavaScript components in one package. Now enhanced with: NEW : Design Kits for Figma
How to Implement Function Overloading in TypeScript
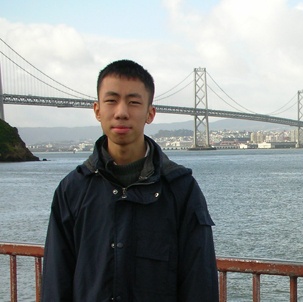
Function overloading is a TypeScript feature that lets us define a function that accepts different kinds of arguments.
TypeScript mainly adds data type-checking capabilities that are missing in JavaScript. This makes programming easier since we don’t have to check the data type of variables and other values ourselves.
We can write our JavaScript programs in TypeScript. Then we can compile the TypeScript code into JavaScript so it can be run by JavaScript runtimes like the browser and Node.js.
One useful feature that is available in TypeScript is function overloading. Function overloading is a feature that lets us define a function that accepts different kinds of arguments.
In this article, we will look at how to implement function overloading in our TypeScript code.
Function Overloading in TypeScript
With TypeScript, we can define a function that accepts different kinds of arguments with function overloading.
To use this, we just have to add all the possible signatures for a function for the combinations of arguments we want the function to accept.
For example, to implement function overloading in TypeScript, we can write something like the following:
We have two different signatures for the add function and they both have a return type set to number .
The first signature expects that both parameters a and b are numbers. And the second signature expects that both parameters a and b are strings.
To implement the function, we make a and b accept the unknown type, which lets us pass in anything for a and b .
In the function body, we check the data types of a and b with the typeof operator. If a and b are both strings, we convert a and b to numbers with the Number function and then get the sum of the numbers. If they’re both numbers, then we just return the sum of them. Otherwise, we return 0.
The unknown type is a type-safe counterpart of any . We can’t assign values with unknown type to any variable. But we can convert unknown type values to something else that matches the type of the variable.
With the typeof checks, we narrowed the types of a and b to one data type so TypeScript can infer the type and do the operations for the variables with the inferred type.
Then we can call add by writing:
We call add with the values that are expected by at least one of the signatures, so it passes the type checks done by the TypeScript compiler.
If we call add with values that aren’t expected by any of the signatures like:
Then the TypeScript compiler will raise a type-check error.
The function implementation’s signature must be more general than the overload signatures. This way, the function with overloads can accept different kinds of values expected by at least one of the signatures.
Method Overloading
We can also overload constructor or class methods.
For instance, we can write something like:
We define the Greeter class with the greet method in it. The greet method is overloaded with two different signatures. The first takes a string and returns a string . The second takes a string array and also returns a string .
In the implementation, we make the person function have an unknown type. We can add a return type or omit it since the TypeScript compiler can infer the return type from the implementation. We can check the value of person in the implementation with our own type checks.
First we check if person is a string with typeof person === "string" . Then we check if person is an array with Array.isArray .
Next, we can create a Greeter instance and call the greet method by writing:
We create a Greeter instance with new . And then we call greet on the instance either with a string or an array of strings we specified with the signatures.
When to Use Function Overloading?
Ideally, we use function overloading only when we expect required arguments that have different types.
The examples above all have required parameters only but they’re allowed to have different types. If we expect some arguments that are optional, then we can use the optional parameter syntax instead.
For instance, instead of writing something like:
We can replace the overloads with optional parameters by writing something like:
Since we accept param1 and param2 as optional parameters, we can use ? to denote them as such.
Function overloading is a feature in TypeScript that lets us define a function that accepts different kinds of arguments.
We can use function overloading for specifying any kinds of function parameters. But ideally, we use them only for required parameters since TypeScript already has a simple optional function parameters syntax.
We can have function overloads for regular functions and class methods.
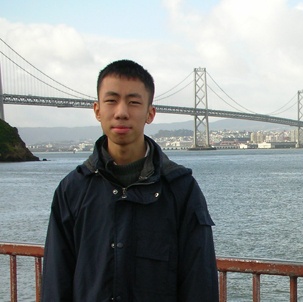
John Au-Yeung
John Au-Yeung is a frontend developer with 6+ years of experience. He is an avid blogger (visit his site at https://thewebdev.info/ ) and the author of Vue.js 3 By Example .
Related Posts
How my team accidentally moved to typescript and loved it, fully typed with trpc, pattern matching with typescript, all articles.
- ASP.NET Core
- ASP.NET MVC
- ASP.NET AJAX
- Blazor Desktop/.NET MAUI
- Design Systems
- Document Processing
- Accessibility
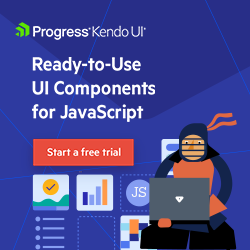
Latest Stories in Your Inbox
Subscribe to be the first to get our expert-written articles and tutorials for developers!
All fields are required
Progress collects the Personal Information set out in our Privacy Policy and the Supplemental Privacy notice for residents of California and other US States and uses it for the purposes stated in that policy.
You can also ask us not to share your Personal Information to third parties here: Do Not Sell or Share My Info
By submitting this form, I understand and acknowledge my data will be processed in accordance with Progress' Privacy Policy .
I agree to receive email communications from Progress Software or its Partners , containing information about Progress Software’s products. I understand I may opt out from marketing communication at any time here or through the opt out option placed in the e-mail communication received.
By submitting this form, you understand and agree that your personal data will be processed by Progress Software or its Partners as described in our Privacy Policy . You may opt out from marketing communication at any time here or through the opt out option placed in the e-mail communication sent by us or our Partners.
We see that you have already chosen to receive marketing materials from us. If you wish to change this at any time you may do so by clicking here .
Thank you for your continued interest in Progress. Based on either your previous activity on our websites or our ongoing relationship, we will keep you updated on our products, solutions, services, company news and events. If you decide that you want to be removed from our mailing lists at any time, you can change your contact preferences by clicking here .
- Skip to main content
- Skip to search
- Skip to select language
- Sign up for free
- Português (do Brasil)
Destructuring assignment
The destructuring assignment syntax is a JavaScript expression that makes it possible to unpack values from arrays, or properties from objects, into distinct variables.
Description
The object and array literal expressions provide an easy way to create ad hoc packages of data.
The destructuring assignment uses similar syntax but uses it on the left-hand side of the assignment instead. It defines which values to unpack from the sourced variable.
Similarly, you can destructure objects on the left-hand side of the assignment.
This capability is similar to features present in languages such as Perl and Python.
For features specific to array or object destructuring, refer to the individual examples below.
Binding and assignment
For both object and array destructuring, there are two kinds of destructuring patterns: binding pattern and assignment pattern , with slightly different syntaxes.
In binding patterns, the pattern starts with a declaration keyword ( var , let , or const ). Then, each individual property must either be bound to a variable or further destructured.
All variables share the same declaration, so if you want some variables to be re-assignable but others to be read-only, you may have to destructure twice — once with let , once with const .
In many other syntaxes where the language binds a variable for you, you can use a binding destructuring pattern. These include:
- The looping variable of for...in for...of , and for await...of loops;
- Function parameters;
- The catch binding variable.
In assignment patterns, the pattern does not start with a keyword. Each destructured property is assigned to a target of assignment — which may either be declared beforehand with var or let , or is a property of another object — in general, anything that can appear on the left-hand side of an assignment expression.
Note: The parentheses ( ... ) around the assignment statement are required when using object literal destructuring assignment without a declaration.
{ a, b } = { a: 1, b: 2 } is not valid stand-alone syntax, as the { a, b } on the left-hand side is considered a block and not an object literal according to the rules of expression statements . However, ({ a, b } = { a: 1, b: 2 }) is valid, as is const { a, b } = { a: 1, b: 2 } .
If your coding style does not include trailing semicolons, the ( ... ) expression needs to be preceded by a semicolon, or it may be used to execute a function on the previous line.
Note that the equivalent binding pattern of the code above is not valid syntax:
You can only use assignment patterns as the left-hand side of the assignment operator. You cannot use them with compound assignment operators such as += or *= .
Default value
Each destructured property can have a default value . The default value is used when the property is not present, or has value undefined . It is not used if the property has value null .
The default value can be any expression. It will only be evaluated when necessary.
Rest property
You can end a destructuring pattern with a rest property ...rest . This pattern will store all remaining properties of the object or array into a new object or array.
The rest property must be the last in the pattern, and must not have a trailing comma.
Array destructuring
Basic variable assignment, destructuring with more elements than the source.
In an array destructuring from an array of length N specified on the right-hand side of the assignment, if the number of variables specified on the left-hand side of the assignment is greater than N , only the first N variables are assigned values. The values of the remaining variables will be undefined.
Swapping variables
Two variables values can be swapped in one destructuring expression.
Without destructuring assignment, swapping two values requires a temporary variable (or, in some low-level languages, the XOR-swap trick ).
Parsing an array returned from a function
It's always been possible to return an array from a function. Destructuring can make working with an array return value more concise.
In this example, f() returns the values [1, 2] as its output, which can be parsed in a single line with destructuring.
Ignoring some returned values
You can ignore return values that you're not interested in:
You can also ignore all returned values:
Using a binding pattern as the rest property
The rest property of array destructuring assignment can be another array or object binding pattern. The inner destructuring destructures from the array created after collecting the rest elements, so you cannot access any properties present on the original iterable in this way.
These binding patterns can even be nested, as long as each rest property is the last in the list.
On the other hand, object destructuring can only have an identifier as the rest property.
Unpacking values from a regular expression match
When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if it is not needed.
Using array destructuring on any iterable
Array destructuring calls the iterable protocol of the right-hand side. Therefore, any iterable, not necessarily arrays, can be destructured.
Non-iterables cannot be destructured as arrays.
Iterables are only iterated until all bindings are assigned.
The rest binding is eagerly evaluated and creates a new array, instead of using the old iterable.
Object destructuring
Basic assignment, assigning to new variable names.
A property can be unpacked from an object and assigned to a variable with a different name than the object property.
Here, for example, const { p: foo } = o takes from the object o the property named p and assigns it to a local variable named foo .
Assigning to new variable names and providing default values
A property can be both
- Unpacked from an object and assigned to a variable with a different name.
- Assigned a default value in case the unpacked value is undefined .
Unpacking properties from objects passed as a function parameter
Objects passed into function parameters can also be unpacked into variables, which may then be accessed within the function body. As for object assignment, the destructuring syntax allows for the new variable to have the same name or a different name than the original property, and to assign default values for the case when the original object does not define the property.
Consider this object, which contains information about a user.
Here we show how to unpack a property of the passed object into a variable with the same name. The parameter value { id } indicates that the id property of the object passed to the function should be unpacked into a variable with the same name, which can then be used within the function.
You can define the name of the unpacked variable. Here we unpack the property named displayName , and rename it to dname for use within the function body.
Nested objects can also be unpacked. The example below shows the property fullname.firstName being unpacked into a variable called name .
Setting a function parameter's default value
Default values can be specified using = , and will be used as variable values if a specified property does not exist in the passed object.
Below we show a function where the default size is 'big' , default co-ordinates are x: 0, y: 0 and default radius is 25.
In the function signature for drawChart above, the destructured left-hand side has a default value of an empty object = {} .
You could have also written the function without that default. However, if you leave out that default value, the function will look for at least one argument to be supplied when invoked, whereas in its current form, you can call drawChart() without supplying any parameters. Otherwise, you need to at least supply an empty object literal.
For more information, see Default parameters > Destructured parameter with default value assignment .
Nested object and array destructuring
For of iteration and destructuring, computed object property names and destructuring.
Computed property names, like on object literals , can be used with destructuring.
Invalid JavaScript identifier as a property name
Destructuring can be used with property names that are not valid JavaScript identifiers by providing an alternative identifier that is valid.
Destructuring primitive values
Object destructuring is almost equivalent to property accessing . This means if you try to destruct a primitive value, the value will get wrapped into the corresponding wrapper object and the property is accessed on the wrapper object.
Same as accessing properties, destructuring null or undefined throws a TypeError .
This happens even when the pattern is empty.
Combined array and object destructuring
Array and object destructuring can be combined. Say you want the third element in the array props below, and then you want the name property in the object, you can do the following:
The prototype chain is looked up when the object is deconstructed
When deconstructing an object, if a property is not accessed in itself, it will continue to look up along the prototype chain.
Specifications
Browser compatibility.
BCD tables only load in the browser with JavaScript enabled. Enable JavaScript to view data.
- Assignment operators
- ES6 in Depth: Destructuring on hacks.mozilla.org (2015)
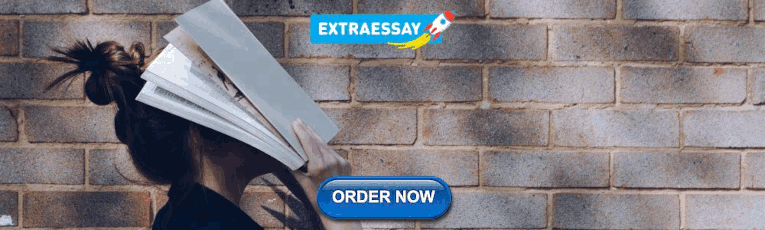
IMAGES
VIDEO
COMMENTS
Using new on a class goes through the following steps: (If it's a derived class) The constructor body before the super() call is evaluated. This part should not access this because it's not yet initialized. (If it's a derived class) The super() call is evaluated, which initializes the parent class through the same process.; The current class's fields are initialized.
Object Types (Blueprints) (Classes) The examples from the previous chapters are limited. They only create single objects. Sometimes we need a "blueprint" for creating many objects of the same "type".The way to create an "object type", is to use an object constructor function.. In the example above, function Person() is an object constructor function. ...
Introduction to JavaScript constructor functions. In the JavaScript objects tutorial, you learned how to use the object literal syntax to create a new object. For example, the following creates a new person object with two properties firstName and lastName: firstName: 'John' , lastName: 'Doe'. In practice, you often need to create many similar ...
Create Multiple Objects With Constructor Function. In JavaScript, you can create multiple objects from a constructor function. For example, this.name = "John", this.age = 23, this.greet = function () {. console.log("hello"); const person2 = new Person(); In the above program, we created two objects ( person1 and person2) using the same ...
4. Using Nick's sample above, you can create a constructor for objects without parameters using a return statement as the last statement in your object definition. Return your constructor function as below and it will run the code in __construct each time you create the object: var __construct = function() {.
2. Functions, Constructors And The new Operator. In section 1 we learned that when the Person constructor, (or any other constructor) is invoked without the new operator it is invoked as a regular JavaScript function. In this section, we will elaborate on this with code examples. Kindly consider the code below.
The function Car() is an object constructor function. Best practices for Javascript object constructors are: The new keyword must be used to instantiate an object. Constructor names begin with a capital letter. The constructor has three parts, two of which are done for you by Javascript: the assignment of this to the object address and ...
Create a constructor function Accumulator(startingValue).. Object that it creates should: Store the "current value" in the property value.The starting value is set to the argument of the constructor startingValue.; The read() method should use prompt to read a new number and add it to value.; In other words, the value property is the sum of all user-entered values with the initial value ...
1) Using the "this" Keyword in Constructors. In a constructor, the this keyword refers to the newly created object. You can use the this keyword to define and assign values to the object's properties. Here's an example: // Constructor. function Carss(making, model, year) {. this.make = making; this.model = model;
Constructors are Object Blueprints. The examples from the previous chapters are limited. They only create single objects. Sometimes we need a "blueprint" for creating many objects of the same "type".The way to create an "object type", is to use an object constructor function.. In the example below, function Person() is an object constructor function.
Description. The constructor() method is a special method for creating and initializing objects created within a class. The constructor() method is called automatically when a class is initiated, and it has to have the exact name "constructor", in fact, if you do not have a constructor method, JavaScript will add an invisible and empty ...
Instantiating an object constructor: There are two ways to instantiate object constructor, 1. var object_name = new Object(); or. var object_name = new Object("java", "JavaScript", "C#"); 2. var object_name = { }; In 1st method, the object is created by using new keyword like normal OOP languages, and "Java", "JavaScript", "C#" are ...
1. Instantiates a new instance object and binds this to it within the constructor. 2. Binds instance.__proto__ to Constructor.prototype. 3. As a side-effect of 2, binds instance.__proto__ ...
class Polygon { constructor({height, width}) { Object.assign(this, arguments[0]); } } Though, creating this object will require creating a "config" object which, depending on your view, either adding clutter to the code or making it more readable:
We can't assign values with unknown type to any variable. But we can convert unknown type values to something else that matches the type of the variable. With the typeof checks, we narrowed the types of a and b to one data type so TypeScript can infer the type and do the operations for the variables with the inferred type.
Unpacking values from a regular expression match. When the regular expression exec() method finds a match, it returns an array containing first the entire matched portion of the string and then the portions of the string that matched each parenthesized group in the regular expression. Destructuring assignment allows you to unpack the parts out of this array easily, ignoring the full match if ...
I've been trying to find a way to correctly define an array as one of the constructor values. Let's say we have a student and we need to have an array with his grades and then using the array we need to get an average from the student's grades.
The name property of a JavaScript class constructor is read-only once the class is defined, but it can be modified by a static field definition in the class body itself. ... because that won't check "read-only" the way a normal property assignment does. Thus this will also work: class Foo { static { Object.defineProperty(this, "name", { value ...