- Engineering Mathematics
- Discrete Mathematics
- Operating System
- Computer Networks
- Digital Logic and Design
- C Programming
- Data Structures
- Theory of Computation
- Compiler Design
- Computer Org and Architecture
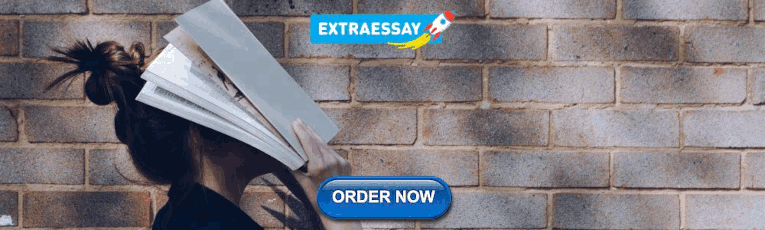
Operating System Tutorial
- What is an Operating System?
- Functions of Operating System
- Types of Operating Systems
- Need and Functions of Operating Systems
- Commonly Used Operating System
Structure of Operating System
- Operating System Services
- Introduction of System Call
- System Programs in Operating System
- Operating Systems Structures
- History of Operating System
- Booting and Dual Booting of Operating System
Types of OS
- Batch Processing Operating System
- Multiprogramming in Operating System
- Time Sharing Operating System
- What is a Network Operating System?
- Real Time Operating System (RTOS)
Process Management
- Introduction of Process Management
- Process Table and Process Control Block (PCB)
- Operations on Processes
- Process Schedulers in Operating System
- Inter Process Communication (IPC)
- Context Switching in Operating System
- Preemptive and Non-Preemptive Scheduling
CPU Scheduling in OS
- CPU Scheduling in Operating Systems
- CPU Scheduling Criteria
- Multiple-Processor Scheduling in Operating System
- Thread Scheduling
Threads in OS
- Thread in Operating System
- Threads and its types in Operating System
- Multithreading in Operating System
- Process Synchronization
- Introduction of Process Synchronization
- Race Condition Vulnerability
- Critical Section in Synchronization
- Mutual Exclusion in Synchronization
Critical Section Problem Solution
- Peterson's Algorithm in Process Synchronization
- Semaphores in Process Synchronization
- Semaphores and its types
- Producer Consumer Problem using Semaphores | Set 1
- Readers-Writers Problem | Set 1 (Introduction and Readers Preference Solution)
- Dining Philosopher Problem Using Semaphores
- Hardware Synchronization Algorithms : Unlock and Lock, Test and Set, Swap
Deadlocks & Deadlock Handling Methods
- Introduction of Deadlock in Operating System
- Conditions for Deadlock in Operating System
- Banker's Algorithm in Operating System
- Wait For Graph Deadlock Detection in Distributed System
- Handling Deadlocks
- Deadlock Prevention And Avoidance
- Deadlock Detection And Recovery
- Deadlock Ignorance in Operating System
- Recovery from Deadlock in Operating System
- Memory Management
- Memory Management in Operating System
- Implementation of Contiguous Memory Management Techniques
- Non-Contiguous Allocation in Operating System
- Compaction in Operating System
- Best-Fit Allocation in Operating System
- Worst-Fit Allocation in Operating Systems
- First-Fit Allocation in Operating Systems
- Fixed (or static) Partitioning in Operating System
- Variable (or Dynamic) Partitioning in Operating System
- Paging in Operating System
- Segmentation in Operating System
- Virtual Memory in Operating System
- Page Replacement Algorithms
- Page Replacement Algorithms in Operating Systems
- Program for Page Replacement Algorithms | Set 2 (FIFO)
- Belady's Anomaly in Page Replacement Algorithms
- Optimal Page Replacement Algorithm
- Program for Least Recently Used (LRU) Page Replacement algorithm
- Techniques to handle Thrashing
- Storage Management
- File Systems in Operating System
- File Allocation Methods
- Free space management in Operating System
- Disk Scheduling Algorithms
- RAID (Redundant Arrays of Independent Disks)
OS Interview Questions
- Last Minute Notes – Operating Systems
- Operating System Interview Questions
OS Quiz and GATE PYQ's
- OS Process Management
- OS Memory Management
- OS Input Output Systems
- OS CPU Scheduling
- 50 Operating System MCQs with Answers
An Operating System(OS) is software that manages and handles the hardware and software resources of a computer system. It provides interaction between users of computers and computer hardware. An operating system is responsible for managing and controlling all the activities and sharing of computer resources. An operating system is a low-level Software that includes all the basic functions like processor management, memory management, Error detection, etc.
This Operating System tutorial will cover all the basic to advance operating system concepts like System Structure, CPU Scheduling, Deadlock, file and disk management, and many more.
Recent Articles on Operating Systems
- System Structure
- CPU Scheduling
- Processes & Threads
- File and Disk Management
- Introduction of Operating System
- Real time systems
- Tasks in Real Time systems
- Difference between multitasking, multithreading and multiprocessing
- Types of computer memory (RAM and ROM)
- Difference between 32-bit and 64-bit operating systems
- What happens when we turn on computer?
- UEFI(Unified Extensible Firmware Interface) and how is it different from BIOS
System Structure :
- Microkernel
- Kernel I/O Subsystem (I/O System)
- Monolithic Kernel and key differences from Microkernel
- Get/Set process resource limits in C
- Privileged and Non-Privileged Instructions
CPU Scheduling :
- Process | (Introduction and different states)
- States of a process
- Process Scheduler
- Measure the time spent in context switch?
- Difference between dispatcher and scheduler
- FCFS Scheduling | Set 1
- FCFS Scheduling | Set 2
- Convoy Effect in Operating Systems
- Shortest Job First (or SJF) scheduling | Set 1 (Non- preemptive)
- Program for Shortest Job First (SJF) scheduling | Set 2 (Preemptive)
- Shortest Job First scheduling with predicted burst time
- Longest Remaining Time First (LRTF) Program
- Longest Remaining Time First (LRTF) algorithm
- Round Robin scheduling
- Selfish Round Robin Scheduling
- Round Robin Scheduling with different arrival times
- Priority Scheduling
- Program for Preemptive Priority CPU Scheduling
- Priority Scheduling with different arrival time – Set 2
- Starvation and Aging in Operating Systems</a
- Highest Response Ratio Next (HRRN) Scheduling
- Multilevel Queue Scheduling
- Multilevel Feedback Queue Scheduling
- Lottery Process Scheduling
- Multiple-Processor Scheduling
Process Synchronization :
- Process Synchronization | Introduction
- Process Synchronization | Set 2
- Critical Section
- Inter Process Communication
- Interprocess Communication: Methods
- IPC through shared memory
- IPC using Message Queues
- Message based Communication in IPC (inter process communication)
- Communication between two process using signals in C
- Semaphores in operating system
- Mutex vs. Semaphore
- Process Synchronization | Monitors
- Peterson’s Algorithm for Mutual Exclusion | Set 1 (Basic C implementation)
- Peterson’s Algorithm for Mutual Exclusion | Set 2 (CPU Cycles and Memory Fence)
- Peterson’s Algorithm (Using processes and shared memory)
- Dekker’s algorithm
- Bakery Algorithm
- Dining-Philosophers Solution Using Monitors
- Reader-Writers solution using Monitors
- Lock variable synchronization mechanism
- Mutex lock for Linux Thread Synchronization
- Priority Inversion : What the heck !
- What’s difference between Priority Inversion and Priority Inheritance ?
- Deadlock Introduction
- Deadlock, Starvation, and Livelock
- Banker’s Algorithm
- Resource Allocation Graph (RAG)
- Program for Banker’s Algorithm
- Banker’s Algorithm : Print all the safe state (or safe sequences)
- Deadlock detection algorithm
Processes & Threads :
- Operating System | Thread
- Threads and its types
- Operating System | User Level thread Vs Kernel Level thread
- Process-based and Thread-based Multitasking
- Multi threading models
- Benefits of Multithreading
- Zombie Processes and their Prevention
- Maximum number of Zombie process a system can handle
- Operating System | Remote Procedure call (RPC)
Memory Management :
- Memory Hierarchy Design and its Characteristics
- Introduction to memory and memory units
- Different Types of RAM (Random Access Memory)
- Buddy System: Memory allocation technique
- Memory Management | Partition Allocation Method
- Variable (or dynamic) Partitioning in Operating System
- Requirements of memory management system
- Memory management – mapping virtual address to physical addresses
- Page Table Entries
- Virtual Memory
- Memory Interleaving
- Virtual Memory Questions
- Inverted Page Table
- Page Fault Handling
- Segmentation
- Memory Segmentation in 8086 Microprocessor
- Program for Next Fit algorithm in Memory Management
- Overlays in Memory Management
- Program for Page Replacement Algorithms | Set 1 ( LRU)
- Program for Optimal Page Replacement Algorithm
- LFU (Least Frequently Used) Cache Implementation
- Second Chance (or Clock) Page Replacement Policy
- Allocating kernel memory (buddy system and slab system)
- Program for buddy memory allocation scheme in Operating Systems | Set 1 (Allocation)
- Program for buddy memory allocation scheme in Operating Systems | Set 2 (Deallocation)
- Static and Dynamic Libraries | Set 1
- Working with Shared Libraries | Set 1
- Working with Shared Libraries | Set 2
- Named Pipe or FIFO with example C program
- Tracing memory usage in Linux
Disk Management
- File Systems
- Unix File System
- File Directory | Path Name
- Structures of Directory
- File Access Methods
- Secondary memory
- Secondary memory – Hard disk drive
- Free space management
- Important Linux Commands (leave, diff, cal, ncal, locate and ln)
- Introduction to Linux Shell and Shell Scripting
- ‘crontab’ in Linux with Examples
- indepth and maxdepth in Linux find() command for limiting search to a specific directory.
Types of Operating System
- Batch OS (e.g. Transactions Process, Payroll System, etc.)
- Multi-programmed OS(e.g. Windows, UNIX, macOS, etc.)
- Timesharing OS(e.g. Multics, Linux, etc.)
- Real-Time OS(e.g. PSOS, VRTX, etc.)
- Distributed OS(e.g. LOCUS, Solaris, etc.)
Operating System Functions
- Memory and processor Management
- Network Management
- Security Management
- File Management
- Error Detection
- Job Accounting
FAQs on Operating System
Q.1 why learn operating systems.
OS is the most important part of a computer. Through OS users can interact with computer software. It provides an interface between Hardware and CPU. It also provides a platform for the program to run on it and services to users. It performs all the basic tasks required in an application.
Q.2 Write the top 10 Operating System Examples?
Some most popular OS examples are given below: Windows Linux MacOS Ios Android Ubuntu CentOS Solaris Chrome OS Fedora
Q.3 What are the Advantages of a multiprocessor system?
A multiprocessor system involves the processing of two or more computer programs simultaneously that share the same memory area. It increases reliability.
Q.4 What is a thread in OS?
A thread is a lightweight process or subprogram which is part of the process or a program. A thread has its own Registers, Stack, State, and Program counter.
Quick Links :
- Last Minute Notes (LMNs) | Operating Systems
- Commonly Asked Operating Systems Interview Questions
- Gate Practice Questions
- ‘Quizzes’ on Operating Systems !
- ‘Practice Problems’ on Operating Systems !
Please Login to comment...
Similar reads, improve your coding skills with practice.
What kind of Experience do you want to share?
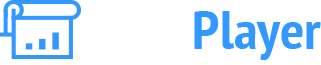
- My presentations
Auth with social network:
Download presentation
We think you have liked this presentation. If you wish to download it, please recommend it to your friends in any social system. Share buttons are a little bit lower. Thank you!
Presentation is loading. Please wait.
Chapter 1 Introduction to Computer Operating System.
Published by Paul Dugdale Modified over 9 years ago
Similar presentations
Presentation on theme: "Chapter 1 Introduction to Computer Operating System."— Presentation transcript:
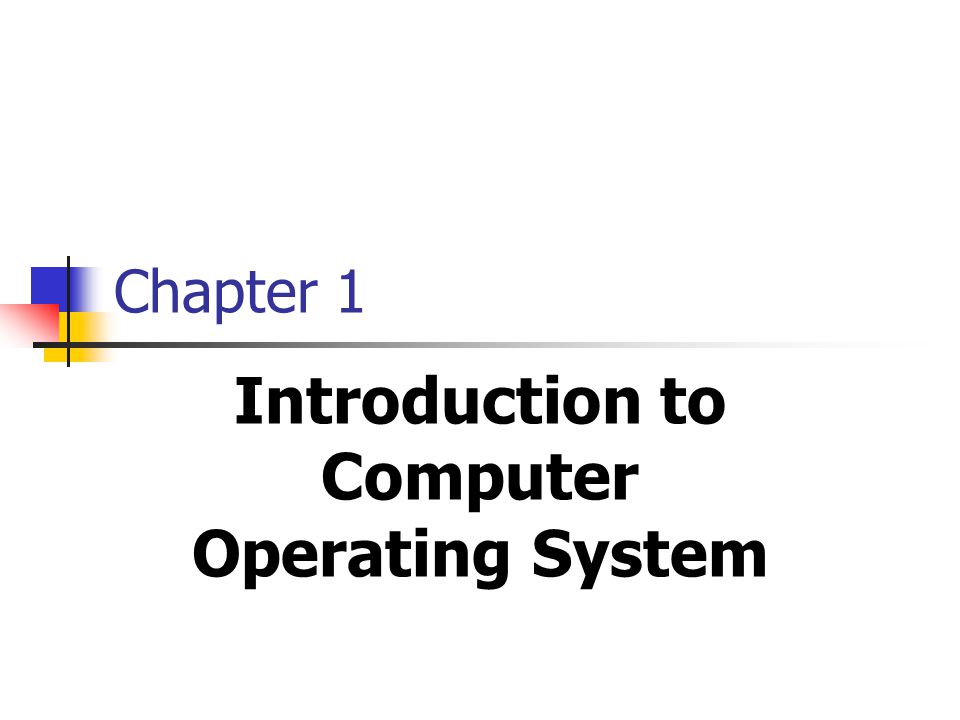
Objectives Overview Define an operating system
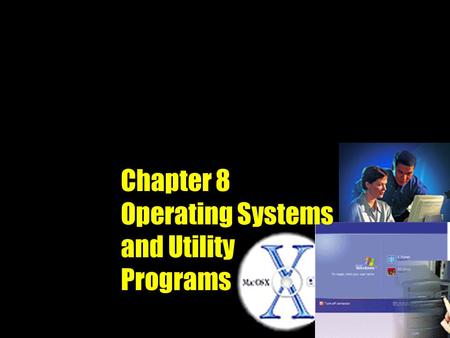
Chapter 8 Operating Systems and Utility Programs
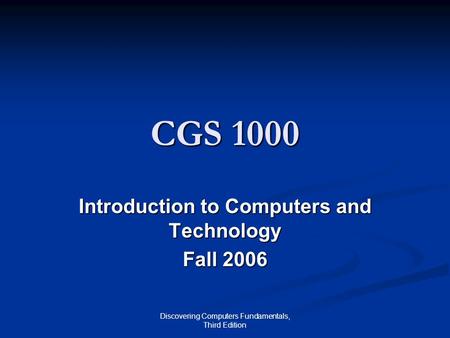
Discovering Computers Fundamentals, Third Edition CGS 1000 Introduction to Computers and Technology Fall 2006.
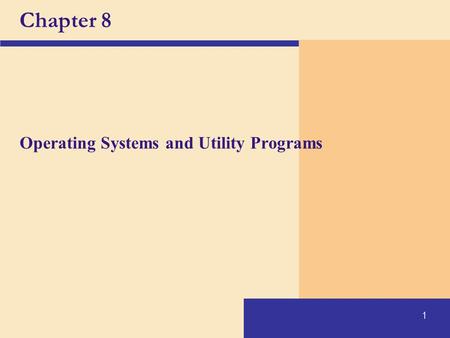
Chapter 8 Operating Systems and Utility Programs.
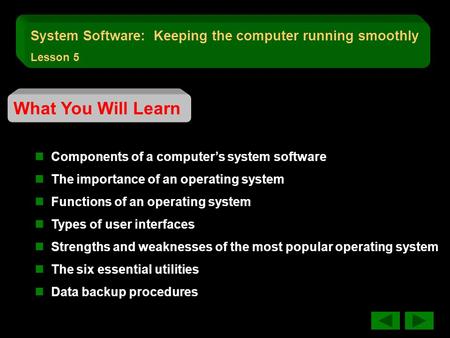
What You Will Learn Components of a computer’s system software The importance of an operating system Functions of an operating system Types of user interfaces.
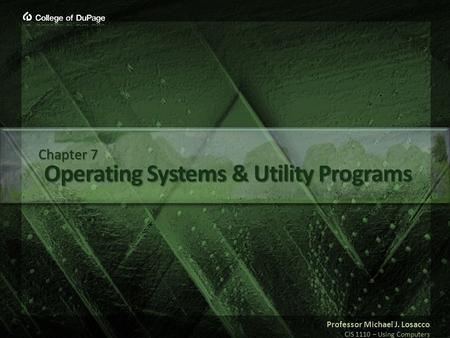
Professor Michael J. Losacco CIS 1110 – Using Computers Operating Systems & Utility Programs Chapter 7.
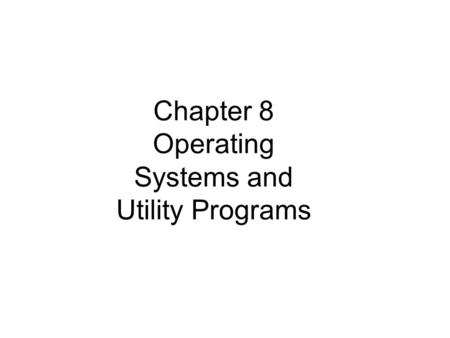
Operating Systems: Software in the Background
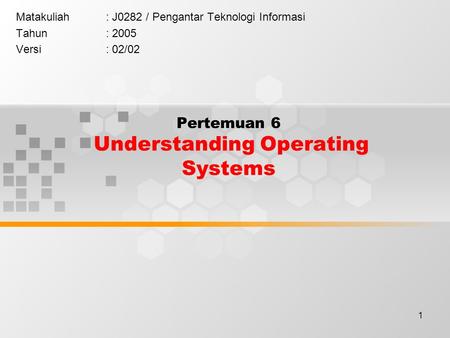
1 Pertemuan 6 Understanding Operating Systems Matakuliah: J0282 / Pengantar Teknologi Informasi Tahun: 2005 Versi: 02/02.
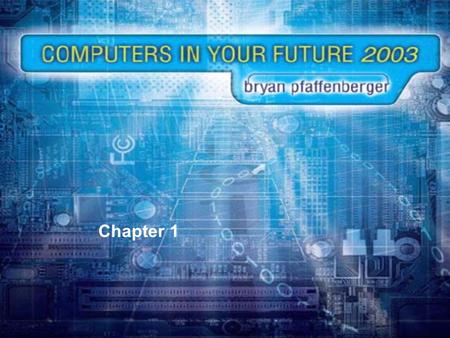
Chapter 1. What is computer fluency? The knowledge possessed by people who are able to navigate the digital world successfully NOT THIS.
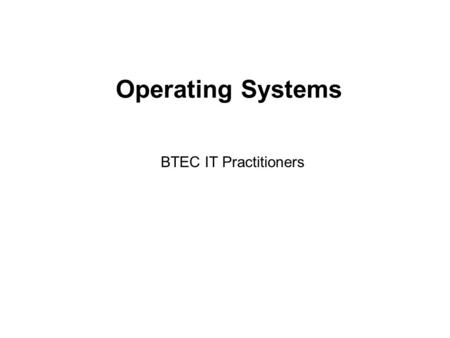
Operating Systems BTEC IT Practitioners.
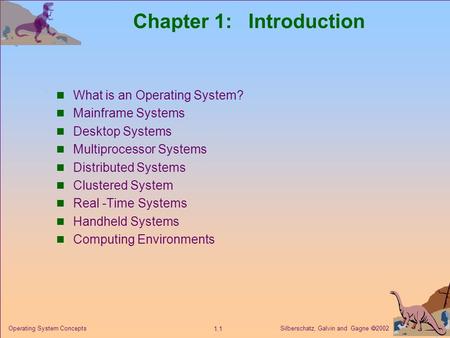
Silberschatz, Galvin and Gagne Operating System Concepts Chapter 1: Introduction What is an Operating System? Mainframe Systems Desktop Systems.
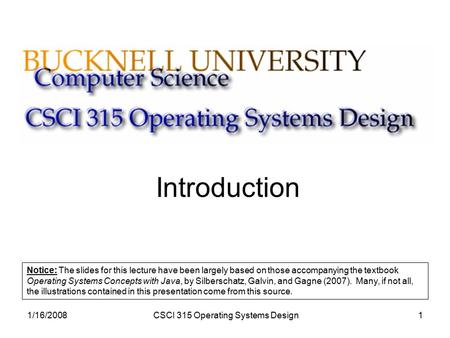
1/16/2008CSCI 315 Operating Systems Design1 Introduction Notice: The slides for this lecture have been largely based on those accompanying the textbook.
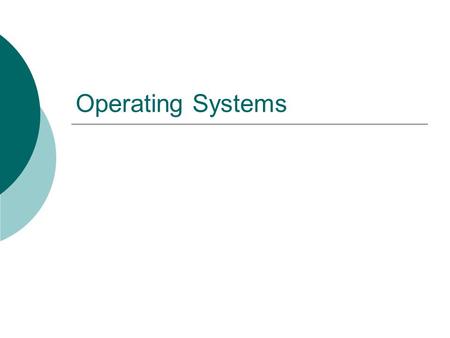
Operating Systems.
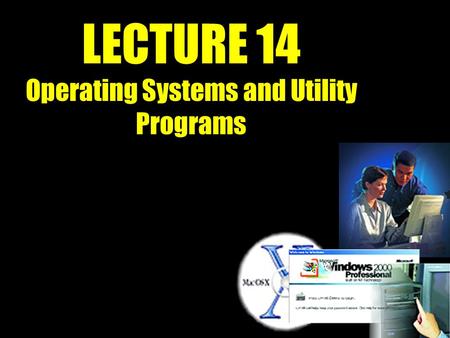
LECTURE 14 Operating Systems and Utility Programs
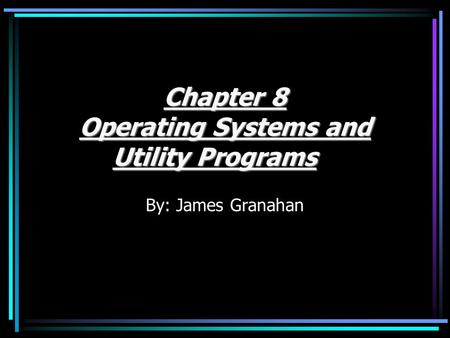
Chapter 8 Operating Systems and Utility Programs By: James Granahan.
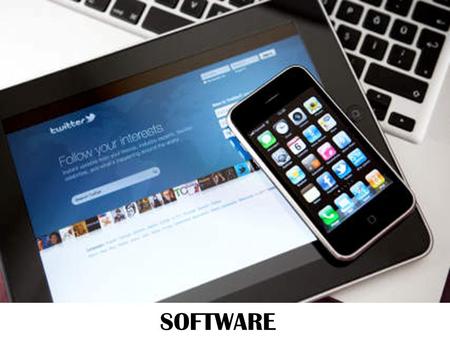
Lesson 4 Computer Software
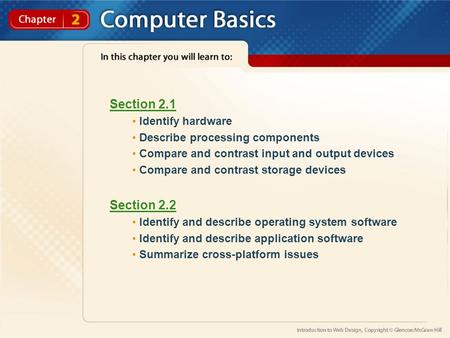
Section 2.1 Identify hardware Describe processing components Compare and contrast input and output devices Compare and contrast storage devices Section.
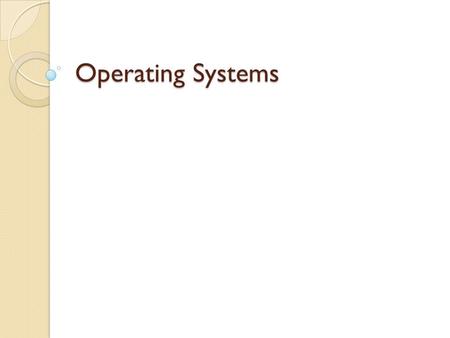
About project
© 2024 SlidePlayer.com Inc. All rights reserved.
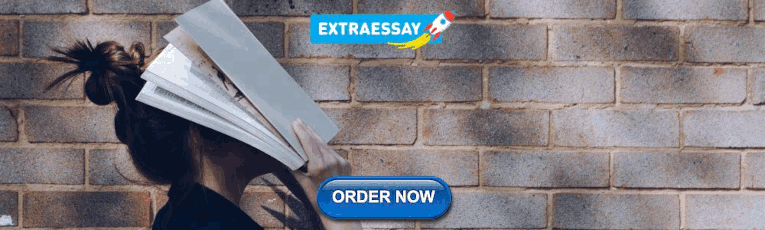
Copyright Note
Errors and additions.
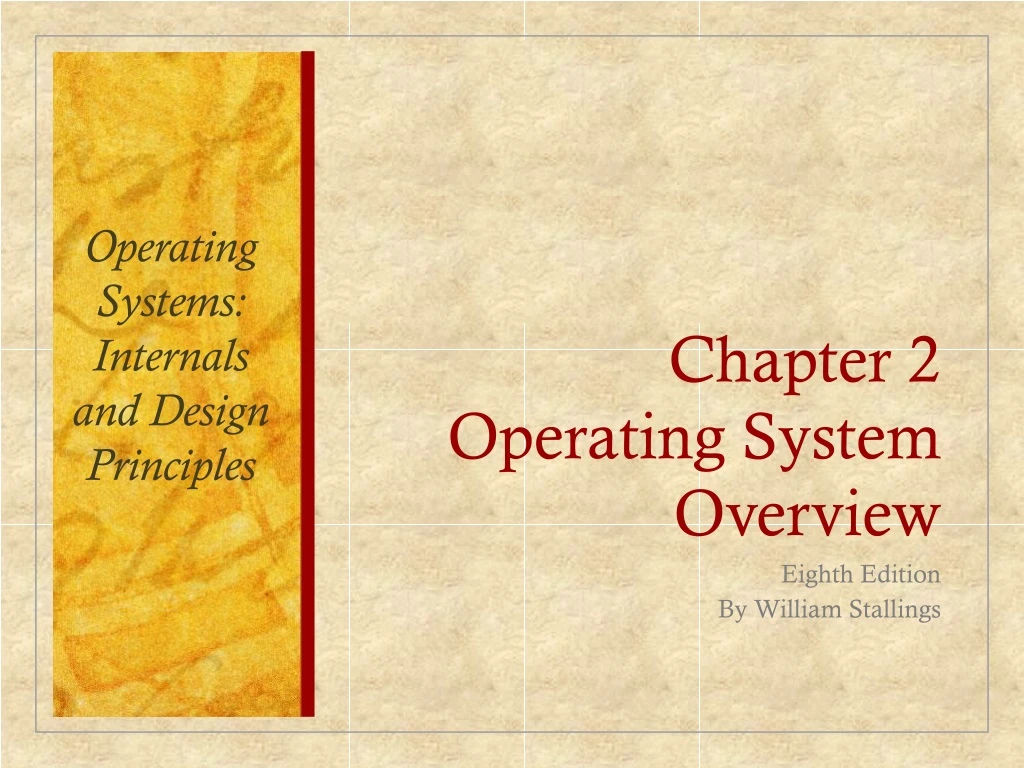
Chapter 2 Operating System Overview
Oct 29, 2019
1.79k likes | 2.47k Views
Operating Systems: Internals and Design Principles. Chapter 2 Operating System Overview. Eighth Edition By William Stallings. Operating System. A program that controls the execution of application programs An interface between applications and hardware. Operating System Services.
Share Presentation
- time sharing
- operating systems
- resident monitor
- processor time alternates
- compatible time sharing systems
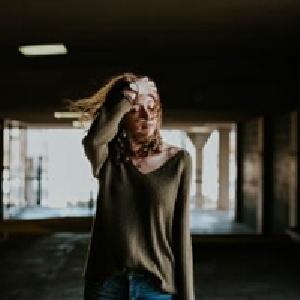
Presentation Transcript
Operating Systems:Internals and Design Principles Chapter 2Operating System Overview Eighth Edition By William Stallings
Operating System A program that controls the execution of application programs An interface between applications and hardware
Operating System Services Program development Program execution Access I/O devices Controlled access to files System access Error detection and response Accounting
Key Interfaces • Instruction set architecture (ISA) • Application binary interface (ABI) • Application programming interface (API)
The Role of an OS A computer is a set of resources for the movement, storage, and processing of data The OS is responsible for managing these resources
Operating System as Software Functions in the same way as ordinary computer software Program, or suite of programs, executed by the processor Frequently relinquishes control and must depend on the processor to allow it to regain control
Evolution of Operating Systems • A major OS will evolve over time for a number of reasons:
Evolution of Operating Systems • Stages include:
Serial Processing Earliest Computers: Problems: Scheduling: most installations used a hardcopy sign-up sheet to reserve computer time time allocations could run short or long, resulting in wasted computer time Setup time a considerable amount of time was spent just on setting up the program to run • No operating system • programmers interacted directly with the computer hardware • Computers ran from a console with display lights, toggle switches, some form of input device, and a printer • Users have access to the computer in “series”
Simple Batch Systems • Early computers were very expensive • important to maximize processor utilization • Monitor • user no longer has direct access to processor • job is submitted to computer operator who batches them together and places them on an input device • program branches back to the monitor when finished
Monitor Point of View Monitor controls the sequence of events Resident Monitor is software always in memory Monitor reads in job and gives control Job returns control to monitor
Processor Point of View • Processor executes instruction from the memory containing the monitor • Executes the instructions in the user program until it encounters an ending or error condition • “control is passed to a job” means processor is fetching and executing instructions in a user program • “control is returned to the monitor” means that the processor is fetching and executing instructions from the monitor program
Job Control Language (JCL)
Desirable Hardware Features
Modes of Operation
Simple Batch System Overhead • Processor time alternates between execution of user programs and execution of the monitor • Sacrifices: • some main memory is now given over to the monitor • some processor time is consumed by the monitor • Despite overhead, the simple batch system improves utilization of the computer
Multiprogrammed Batch Systems • Processor is often idle • even with automatic job sequencing • I/O devices are slow compared to processor
Uniprogramming The processor spends a certain amount of time executing, until it reaches an I/O instruction; it must then wait until that I/O instruction concludes before proceeding
Multiprogramming There must be enough memory to hold the OS (resident monitor) and one user program When one job needs to wait for I/O, the processor can switch to the other job, which is likely not waiting for I/O
Multiprogramming also known as multitasking memory is expanded to hold three, four, or more programs and switch among all of them Multiprogramming
Multiprogramming Example Table 2.1 Sample Program Execution Attributes
Effects on Resource Utilization Table 2.2 Effects of Multiprogramming on Resource Utilization
Time-Sharing Systems • Can be used to handle multiple interactive jobs • Processor time is shared among multiple users • Multiple users simultaneously access the system through terminals, with the OS interleaving the execution of each user program in a short burst or quantum of computation
Batch Multiprogramming vs. Time Sharing Table 2.3 Batch Multiprogramming versus Time Sharing
Compatible Time-Sharing Systems CTSS Time Slicing System clock generates interrupts at a rate of approximately one every 0.2 seconds At each interrupt OS regained control and could assign processor to another user At regular time intervals the current user would be preempted and another user loaded in Old user programs and data were written out to disk Old user program code and data were restored in main memory when that program was next given a turn • One of the first time-sharing operating systems • Developed at MIT by a group known as Project MAC • Ran on a computer with 32,000 36-bit words of main memory, with the resident monitor consuming 5000 of that • To simplify both the monitor and memory management a program was always loaded to start at the location of the 5000th word
Major Achievements • Operating Systems are among the most complex pieces of software ever developed
Process Fundamental to the structure of operating systems
Development of the Process • Three major lines of computer system development created • problems in timing and synchronization that contributed to • the development:
Causes of Errors • Nondeterminate program operation • program execution is interleaved by the processor when memory is shared • the order in which programs are scheduled may affect their outcome • Deadlocks • it is possible for two or more programs to be hung up waiting for each other • may depend on the chance timing of resource allocation and release • Improper synchronization • a program must wait until the data are available in a buffer • improper design of the signaling mechanism can result in loss or duplication • Failed mutual exclusion • more than one user or program attempts to make use of a shared resource at the same time • only one routine at a time allowed to perform an update against the file
Components of a Process • The execution context is essential: • it is the internal data by which the OS is able to supervise and control the process • includes the contents of the various process registers • includes information such as the priority of the process and whether the process is waiting for the completion of a particular I/O event • A process contains three components: • an executable program • the associated data needed by the program (variables, work space, buffers, etc.) • the execution context (or “process state”) of the program
Process Management The entire state of the process at any instant is contained in its context New features can be designed and incorporated into the OS by expanding the context to include any new information needed to support the feature
Memory Management • The OS has five principal storage management responsibilities:
Virtual Memory A facility that allows programs to address memory from a logical point of view, without regard to the amount of main memory physically available Conceived to meet the requirement of having multiple user jobs reside in main memory concurrently
Paging • Allows processes to be comprised of a number of fixed-size blocks, called pages • Program references a word by means of a virtual address • consists of a page number and an offset within the page • each page may be located anywhere in main memory • Provides for a dynamic mapping between the virtual address used in the program and a real (or physical) address in main memory
Information Protection and Security • The nature of the threat that concerns an organization will vary greatly depending on the circumstances • The problem involves controlling access to computer systems and the information stored in them
Scheduling andResource Management • Key responsibility of an OS is managing resources • Resource allocation policies must consider:
Different Architectural Approaches • Demands on operating systems require new ways of organizing the OS
Microkernel Architecture • Assigns only a few essential functions to the kernel: • The approach:
Multithreading • Technique in which a process, executing an application, is divided into threads that can run concurrently
Symmetric Multiprocessing (SMP) • Term that refers to a computer hardware architecture and also to the OS behavior that exploits that architecture • Several processes can run in parallel • Multiple processors are transparent to the user • these processors share same main memory and I/O facilities • all processors can perform the same functions • The OS takes care of scheduling of threads or processes on individual processors and of synchronization among processors
SMP Advantages
OS Design Distributed Operating System Object-Oriented Design Used for adding modular extensions to a small kernel Enables programmers to customize an operating system without disrupting system integrity Eases the development of distributed tools and full-blown distributed operating systems • Provides the illusion of • a single main memory space • single secondary memory space • unified access facilities • State of the art for distributed operating systems lags that of uniprocessor and SMP operating systems
- More by User
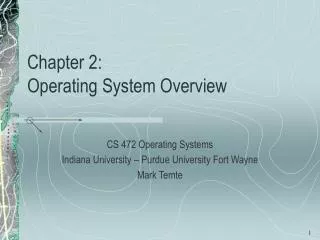
Chapter 2: Operating System Overview
Chapter 2: Operating System Overview CS 472 Operating Systems Indiana University – Purdue University Fort Wayne Mark Temte Operating system overview An example of computing without an operating system Session scheduling and set-up time Front panel lights and toggle switches
1.05k views • 58 slides
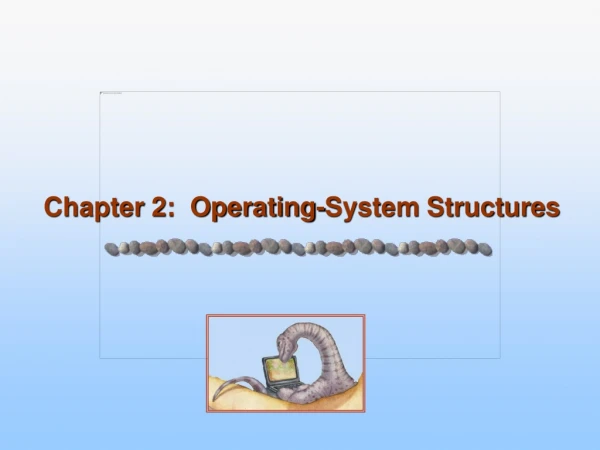
Chapter 2: Operating-System Structures
Chapter 2: Operating-System Structures. Chapter 2: Operating-System Structures. Operating System Services User Operating System Interface System Calls Types of System Calls System Programs Operating System Design and Implementation Operating System Structure Virtual Machines
740 views • 44 slides
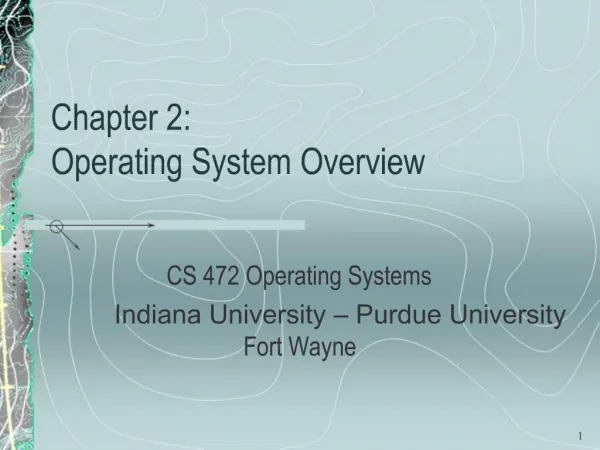
2. Operating system overview. An example of computing without an operating systemSession scheduling and set-up timeFront panel lights and toggle switchesBootstrap loader on paper tapeCompiler on cardsSource deckPaper tape object fileData deckExecution. 3. Operating system objectives. ConvenienceMakes the computer more convenient to useEfficiencyAllows computer system resources to be used in an efficient mannerAbility to evolvePermit effective development, testing, and introduction o9446
962 views • 57 slides
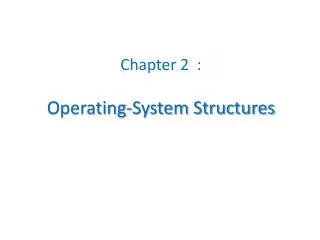
Chapter 2 : Operating-System Structures
Chapter 2 : Operating-System Structures. Chapter 2: Operating-System Structures. Operating System Services User Operating System Interface System Calls Types of System Calls System Programs Operating System Design and Implementation Operating System Structure Virtual Machines
587 views • 42 slides
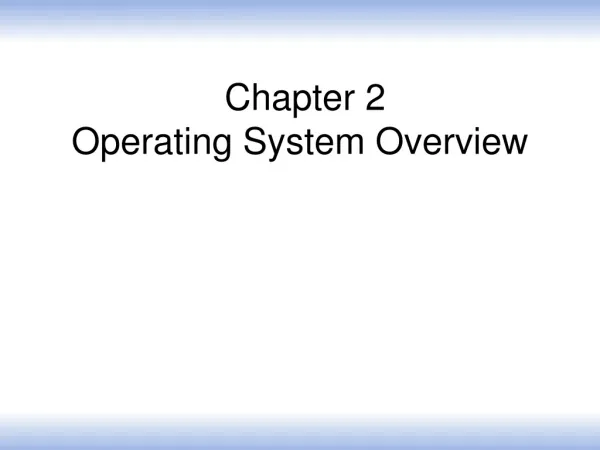
Chapter 2 Operating System Overview. Operating System. A program that controls the execution of application programs An interface between applications and hardware Convenience Efficiency Ability to evolve. Layers and Views. Services Provided by the OS. Program development
542 views • 31 slides
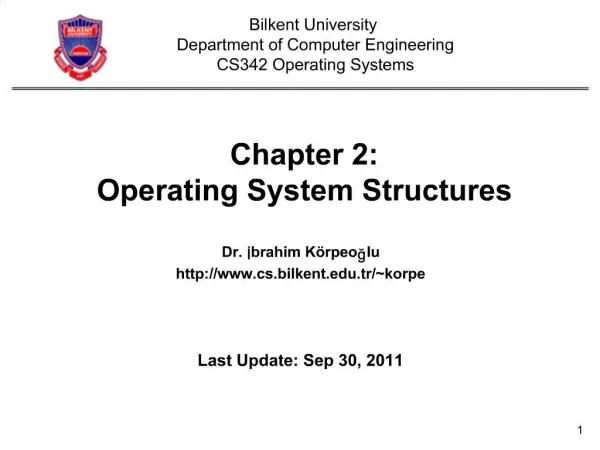
Chapter 2: Operating System Structures
2. Outline. OutlineOperating System ServicesUser Operating System InterfaceSystem CallsSystem ProgramsOperating System Structure. ObjectivesTo describe the services an operating system provides to users, processes, and other systemsTo discuss the various ways of structuring an operating syst
446 views • 43 slides
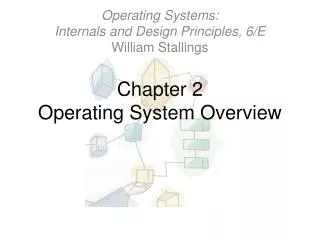
Operating Systems: Internals and Design Principles, 6/E William Stallings. Chapter 2 Operating System Overview. Operating System. A program that controls the execution of application programs An interface between applications and hardware. Evolution of Operating Systems. Serial processing
749 views • 38 slides
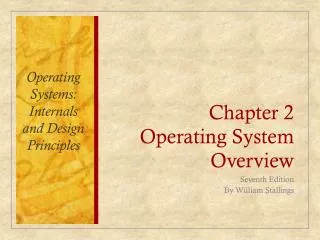
Operating Systems: Internals and Design Principles. Chapter 2 Operating System Overview. Seventh Edition By William Stallings. Operating Systems: Internals and Design Principles.
1.1k views • 81 slides
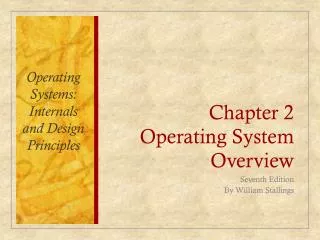
Operating Systems: Internals and Design Principles. Chapter 2 Operating System Overview. Seventh Edition By William Stallings. Operating System. A program that controls the execution of application programs An interface between applications and hardware.
701 views • 50 slides
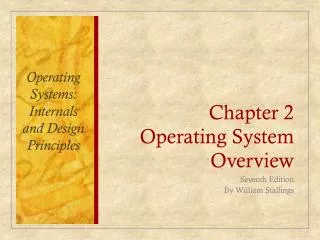
Operating Systems: Internals and Design Principles. Chapter 2 Operating System Overview. Seventh Edition By William Stallings. Operating Systems: Internals and Design Principles. Operating systems are those programs that interface the machine with
1.04k views • 81 slides
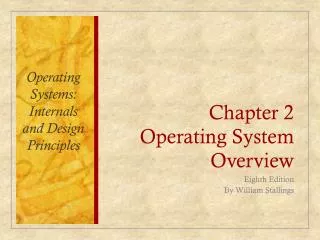
1.29k views • 91 slides
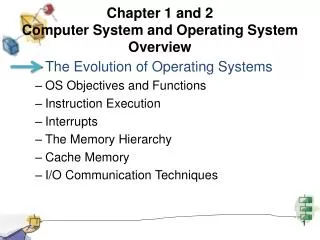
Chapter 1 and 2 Computer System and Operating System Overview
Chapter 1 and 2 Computer System and Operating System Overview. The Evolution of Operating Systems OS Objectives and Functions Instruction Execution Interrupts The Memory Hierarchy Cache Memory I/O Communication Techniques. Evolution of Operating Systems.
798 views • 54 slides
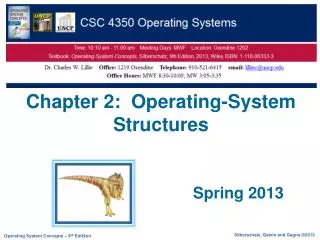
Chapter 2: Operating-System Structures. Chapter 2: Operating-System Structures. Operating System Services User Operating System Interface System Calls Types of System Calls System Programs Operating System Design and Implementation Operating System Structure Operating System Debugging
848 views • 56 slides
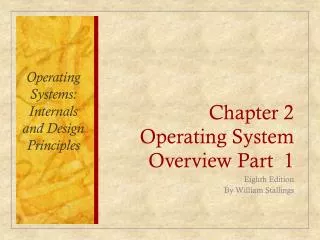
Chapter 2 Operating System Overview Part 1
Operating Systems: Internals and Design Principles. Chapter 2 Operating System Overview Part 1. Eighth Edition By William Stallings. Operating System. A program that controls the execution of application programs An interface between applications and hardware. Operating System Services.
2.18k views • 56 slides
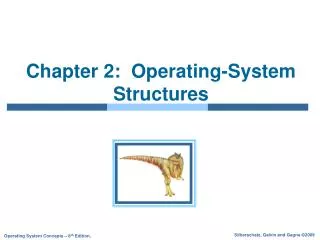
Chapter 2: Operating-System Structures. Chapter 2: Operating-System Structures. Operating System Services User Operating System Interface System Calls Types of System Calls System Programs Operating System Structure Virtual Machines. Objectives.
573 views • 42 slides
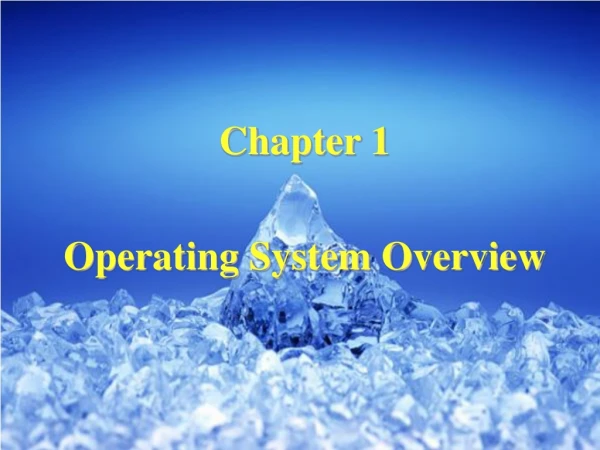
Chapter 1 Operating System Overview
Chapter 1 Operating System Overview. Contents. Operating System Objectives and Functions The Evolution of Operating System Major Achievements Characteristics of Modern OS Examples ( NT 、 UNIX ). 1.1 Objectives and Functions (p45). 1.1.1 Overview
830 views • 52 slides
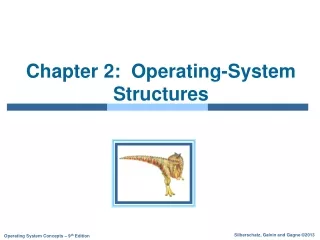
563 views • 55 slides
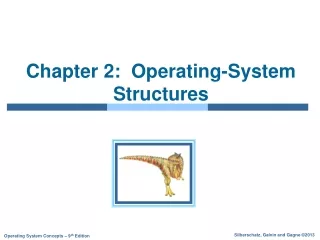
Chapter 2: Operating-System Structures. Chapter 2: Operating-System Structures. Operating System Services User and Operating System Interface System Calls Types of System Calls System Programs Operating System Design and Implementation Operating System Structure
664 views • 66 slides
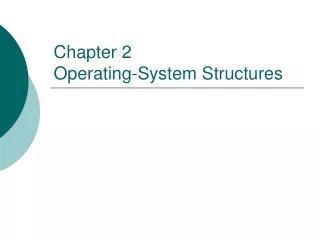
Chapter 2 Operating-System Structures
Chapter 2 Operating-System Structures. Operating-System Structures. Internally, operating systems vary greatly in their makeup The goals of the system should be well defined before the design begins These goals form the basis for choices among various algorithms and strategies.
1.26k views • 122 slides
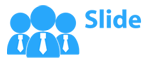
- Operating System
- Popular Categories
Powerpoint Templates
Icon Bundle
Kpi Dashboard
Professional
Business Plans
Swot Analysis
Gantt Chart
Business Proposal
Marketing Plan
Project Management
Business Case
Business Model
Cyber Security
Business PPT
Digital Marketing
Digital Transformation
Human Resources
Product Management
Artificial Intelligence
Company Profile
Acknowledgement PPT
PPT Presentation
Reports Brochures
One Page Pitch
Interview PPT
All Categories
Powerpoint Templates and Google slides for Operating System
Save your time and attract your audience with our fully editable ppt templates and slides..
Item 1 to 60 of 16666 total items
- You're currently reading page 1

Engage buyer personas and boost brand awareness by pitching yourself using this prefabricated set. This Operating System Process Research Segment Ideal Time Accounting Information is a great tool to connect with your audience as it contains high-quality content and graphics. This helps in conveying your thoughts in a well-structured manner. It also helps you attain a competitive advantage because of its unique design and aesthetics. In addition to this, you can use this PPT design to portray information and educate your audience on various topics. With thirty five slides, this is a great design to use for your upcoming presentations. Not only is it cost-effective but also easily pliable depending on your needs and requirements. As such color, font, or any other design component can be altered. It is also available for immediate download in different formats such as PNG, JPG, etc. So, without any further ado, download it now.
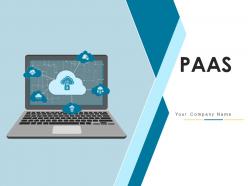
Engage buyer personas and boost brand awareness by pitching yourself using this prefabricated set. This PAAS Cloud Resources Operating System Service Applications is a great tool to connect with your audience as it contains high-quality content and graphics. This helps in conveying your thoughts in a well-structured manner. It also helps you attain a competitive advantage because of its unique design and aesthetics. In addition to this, you can use this PPT design to portray information and educate your audience on various topics. With twelve slides, this is a great design to use for your upcoming presentations. Not only is it cost-effective but also easily pliable depending on your needs and requirements. As such color, font, or any other design component can be altered. It is also available for immediate download in different formats such as PNG, JPG, etc. So, without any further ado, download it now.
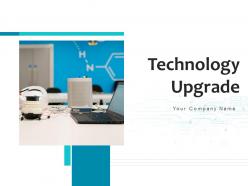
Deliver a lucid presentation by utilizing this Technology Upgrade Operating System Consumer Finalize Device Management. Use it to present an overview of the topic with the right visuals, themes, shapes, and graphics. This is an expertly designed complete deck that reinforces positive thoughts and actions. Use it to provide visual cues to your audience and help them make informed decisions. A wide variety of discussion topics can be covered with this creative bundle such as Operating System, Consumer Finalize, Device Management. All the eleven slides are available for immediate download and use. They can be edited and modified to add a personal touch to the presentation. This helps in creating a unique presentation every time. Not only that, with a host of editable features, this presentation can be used by any industry or business vertical depending on their needs and requirements. The compatibility with Google Slides is another feature to look out for in the PPT slideshow.
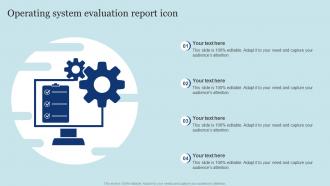
Presenting our set of slides with name Operating System Evaluation Report Icon. This exhibits information on four stages of the process. This is an easy-to-edit and innovatively designed PowerPoint template. So download immediately and highlight information on Operating System, Evaluation Report, Icon.

This slide provides information regarding ethical operating system as a responsible tech approach that improves product development process. The approach is utilized at product delivery or discovery stage by stakeholders and clients. Introducing Building Responsible Organization Ethical Operating System OS Overview About Duration And People to increase your presentation threshold. Encompassed with two stages, this template is a great option to educate and entice your audience. Dispence information on Risks And Analyze Existing Solutions, Project Development, Development And Effective Innovation, using this template. Grab it now to reap its full benefits.
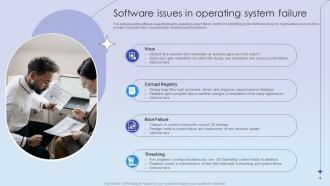
This slide presents software issues that lead to operating system failure, helpful in highlighting problems that are faced by organisations and should be avoided. It includes virus, corrupt registry, thrashing and boot failure. Presenting our set of slides with Software Issues In Operating System Failure. This exhibits information on four stages of the process. This is an easy to edit and innovatively designed PowerPoint template. So download immediately and highlight information on Windows Update, Deadlock Results, Websites.
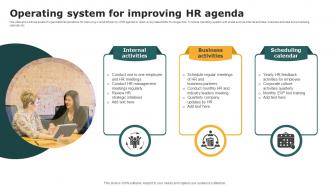
This slide shows three areas of organizational operations for improving overall efficiency of HR agenda to retain every stakeholder for longer time. It include operating system with areas such as internal activities, business activities and scheduling calendar etc.Presenting our set of slides with Operating system for improving HR agenda. This exhibits information on three stages of the process. This is an easy to edit and innovatively designed PowerPoint template. So download immediately and highlight information on Internal Activities, Business Activities, Scheduling Calendar.
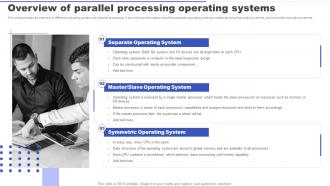
This slide provides an overview of different operating systems for parallel processing. It also shares information about the separate operating systems, master and slave operating systems, and symmetric operating systems. Increase audience engagement and knowledge by dispensing information using Overview Of Parallel Processing Operating Systems Ppt Slides Example File. This template helps you present information on three stages. You can also present information on Slave Operating System, Separate Operating System, Symmetric Operating System using this PPT design. This layout is completely editable so personaize it now to meet your audiences expectations.
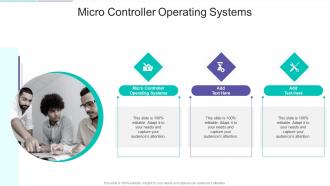
Presenting our Micro Controller Operating Systems In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases three stages. It is useful to share insightful information on Micro Controller Operating Systems This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
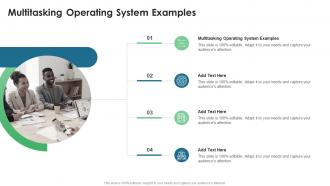
Presenting our Multitasking Operating System Examples In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases four stages. It is useful to share insightful information on Multitasking Operating System Examples This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
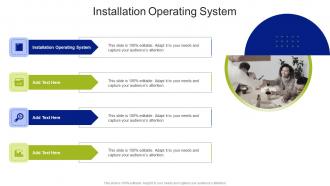
Presenting our Installation Operating System In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases four stages. It is useful to share insightful information on Installation Operating System This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
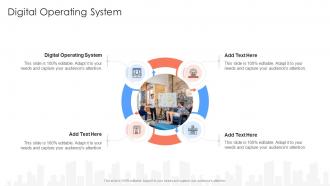
Presenting Digital Operating System In Powerpoint And Google Slides Cpb slide which is completely adaptable. The graphics in this PowerPoint slide showcase four stages that will help you succinctly convey the information. In addition, you can alternate the color, font size, font type, and shapes of this PPT layout according to your content. This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Digital Operating System. This well-structured design can be downloaded in different formats like PDF, JPG, and PNG. So, without any delay, click on the download button now.
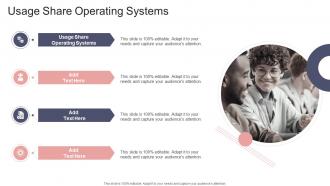
Presenting our Usage Share Operating Systems In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases four stages. It is useful to share insightful information on Usage Share Operating Systems. This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
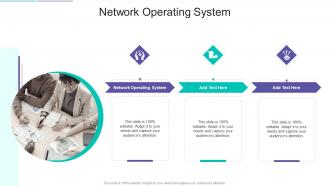
Presenting our Network Operating System In Powerpoint And Google Slides Cpb PowerPoint template design. This PowerPoint slide showcases three stages. It is useful to share insightful information on Network Operating System This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
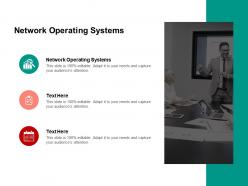
Presenting our Network Operating Systems Ppt Powerpoint Presentation Professional Graphics Pictures Cpb PowerPoint template design. This PowerPoint slide showcases three stages. It is useful to share insightful information on Network Operating Systems This PPT slide can be easily accessed in standard screen and widescreen aspect ratios. It is also available in various formats like PDF, PNG, and JPG. Not only this, the PowerPoint slideshow is completely editable and you can effortlessly modify the font size, font type, and shapes according to your wish. Our PPT layout is compatible with Google Slides as well, so download and edit it as per your knowledge.
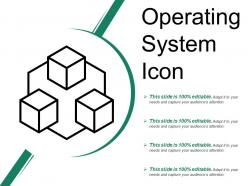
Presenting this Operating System Icon PowerPoint presentation. You can include charts and graphs for a clear representation of data. The PPT supports the standard (4:3) and widescreen (16:9) sizes. It is also compatible with Google Slides. Transform this into common images or document formats like JPEG, PNG or PDF. High-quality graphics ensure that quality is always maintained.
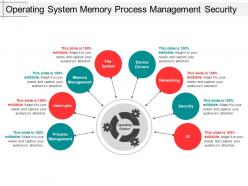
Presenting this Operating System Memory Process Management Security PowerPoint presentation. Add charts and graphs for a wonderful representation of information. The PPT also supports the standard (4:3) and widescreen (16:9) aspect ratios. It is compatible with Google Slides. Convert this into popular images or document formats such as JPEG, PNG or PDF. High-quality graphics ensure that graphics quality is maintained at every stage of presentation.
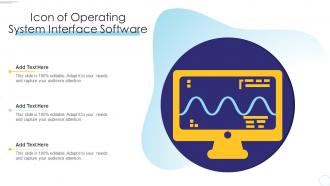
Presenting our set of slides with name Icon Of Operating System Interface Software. This exhibits information on three stages of the process. This is an easy-to-edit and innovatively designed PowerPoint template. So download immediately and highlight information on Icon Of Operating System, Interface Software.
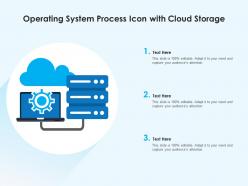
Showcasing this set of slides titled Operating System Process Icon With Cloud Storage. The topics addressed in these templates are Operating System Process Icon With Cloud Storage. All the content presented in this PPT design is completely editable. Download it and make adjustments in color, background, font etc. as per your unique business setting.
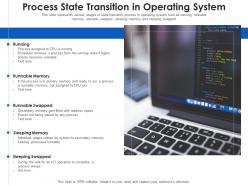
Presenting Process State Transition In Operating System to dispense important information. This template comprises one stages. It also presents valuable insights into the topics including Runnable Memory, Runnable Swapped, Sleeping Memory. This is a completely customizable PowerPoint theme that can be put to use immediately. So, download it and address the topic impactfully.
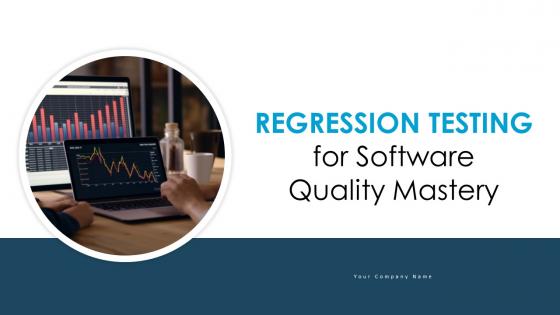
Enthrall your audience with this Regression Testing For Software Quality Mastery Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising ninety seven slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
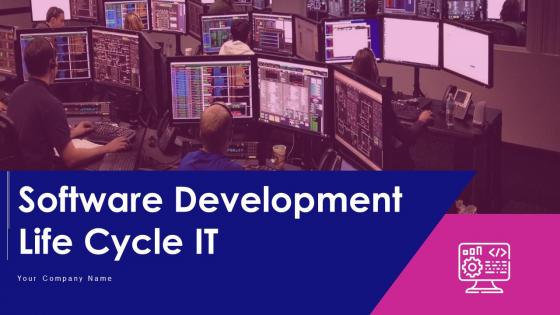
Enthrall your audience with this Software Development Life Cycle IT Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well crafted template. It acts as a great communication tool due to its well researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention grabber. Comprising ninty five slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
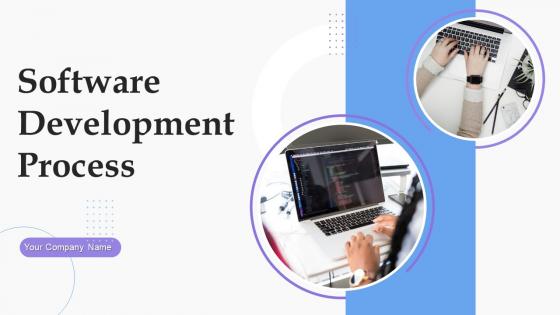
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Software Development Process Powerpoint Presentation Slides and has templates with professional background images and relevant content. This deck consists of total of ninty two slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
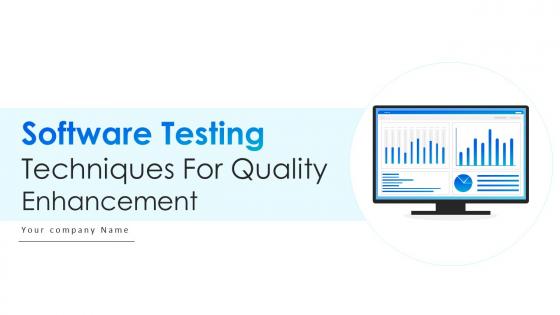
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of eighty six slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
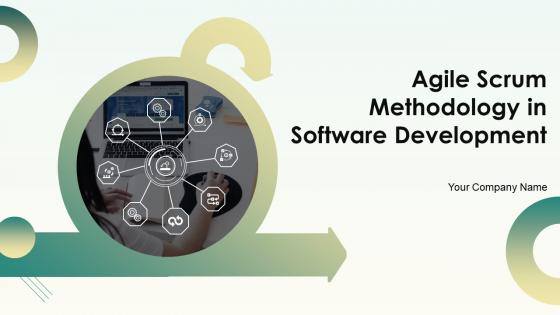
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Agile Scrum Methodology In Software Development Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the eighty five slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
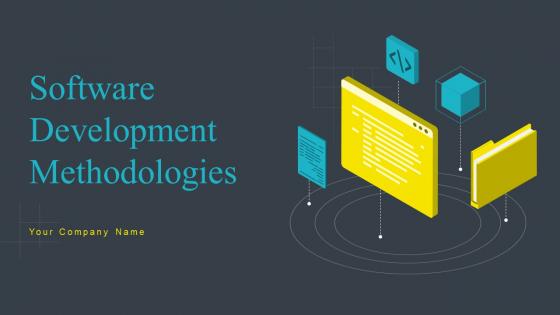
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of eighty four slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
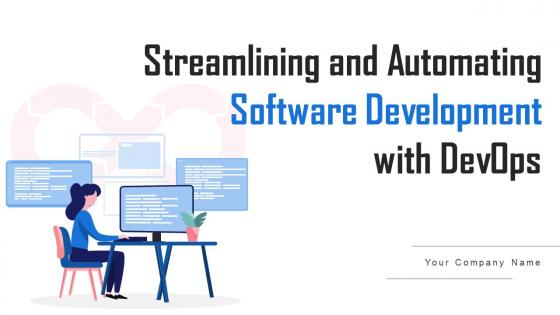
Enthrall your audience with this Streamlining And Automating Software Development With Devops Complete Deck. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising seventy six slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
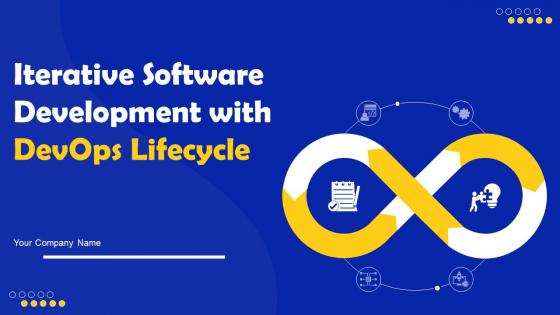
Enthrall your audience with this Iterative Software Development With Devops Lifecycle Powerpoint Presentation Slides Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising seventy six slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
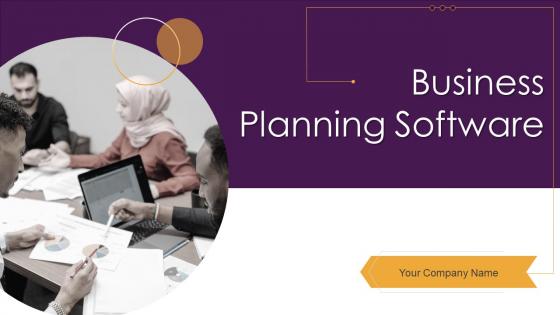
Enthrall your audience with this Business Planning Software Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising seventy eight slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
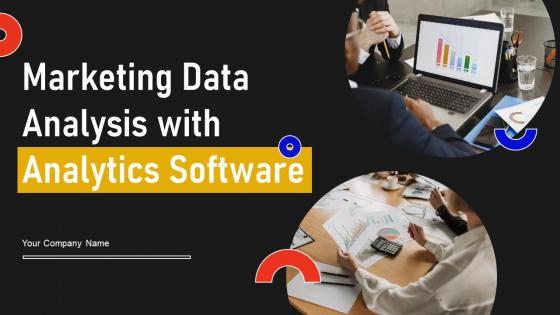
Deliver an informational PPT on various topics by using this Marketing Data Analysis With Analytics Software MKT CD V. This deck focuses and implements best industry practices, thus providing a birds-eye view of the topic. Encompassed with seventy three slides, designed using high-quality visuals and graphics, this deck is a complete package to use and download. All the slides offered in this deck are subjective to innumerable alterations, thus making you a pro at delivering and educating. You can modify the color of the graphics, background, or anything else as per your needs and requirements. It suits every business vertical because of its adaptable layout.
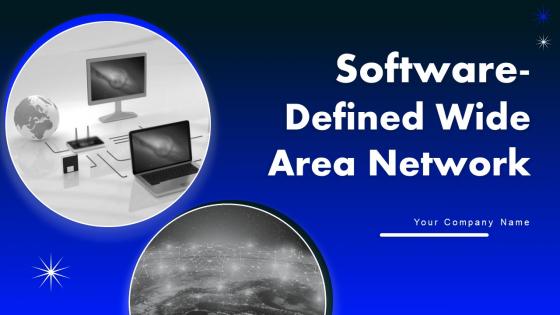
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Software Defined Wide Area Network Powerpoint Presentation Slides and has templates with professional background images and relevant content. This deck consists of total of seventy three slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
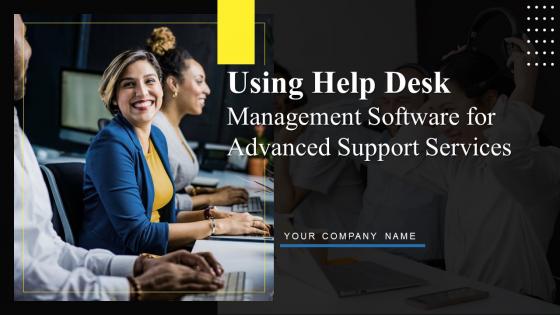
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of seventy seven slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.

Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this CDP Software Guide For Comprehensive Database MKT CD V is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the seventy slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
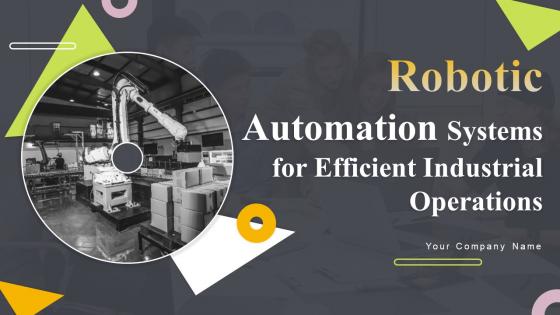
Deliver an informational PPT on various topics by using this Robotic Automation Systems For Efficient Industrial Operations Powerpoint Presentation Slides. This deck focuses and implements best industry practices, thus providing a birds-eye view of the topic. Encompassed with seventy slides, designed using high-quality visuals and graphics, this deck is a complete package to use and download. All the slides offered in this deck are subjective to innumerable alterations, thus making you a pro at delivering and educating. You can modify the color of the graphics, background, or anything else as per your needs and requirements. It suits every business vertical because of its adaptable layout.
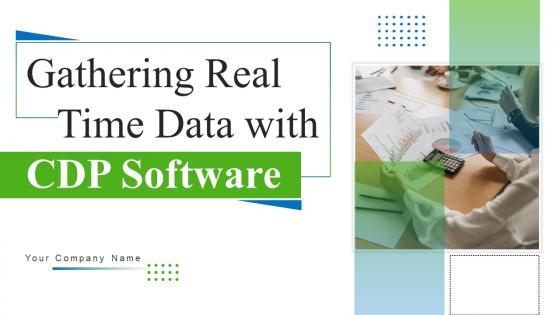
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Gathering Real Time Data With CDP Software Mkt Cd V and has templates with professional background images and relevant content. This deck consists of total of sixty nine slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
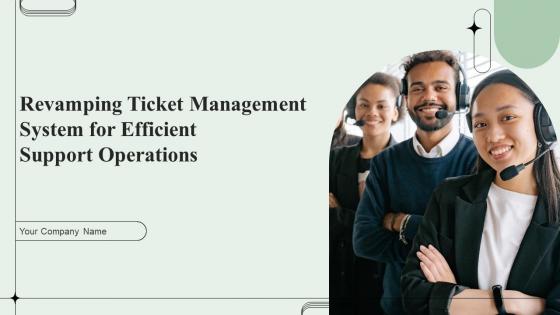
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of seventy three slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
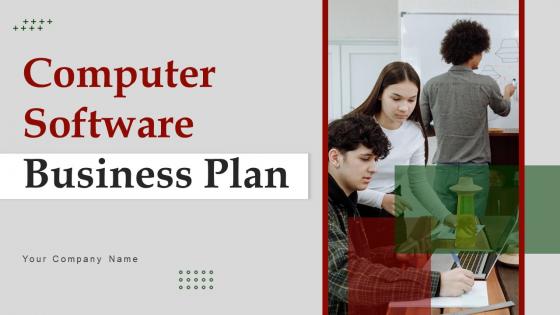
You can survive and sail through cut throat competition if you have the right skills and products at hand. If a business plan is on your upcoming agenda, then it will not be wise of you to proceed in absence of our well designed Computer Software Business Plan Powerpoint Presentation Slides. Our PowerPoint presentation swears by in depth detailing and thus answers every question that may hit you or your audience at any point of time. Whats more, are the multi fold benefits that our PowerPoint offers. Made up of high resolution graphics, this PPT does not hamper when projected on a wide screen. Being pre designed and thoroughly editable this ready made business plan saves a lot of the presenters time and efforts which otherwise get wasted in designing the business plan from scratch. We make our business plan PowerPoint presentation available to you keeping in mind the competitive edge. Join your hands with us now.
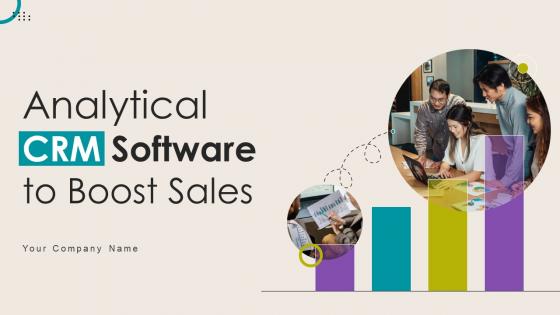
While your presentation may contain top-notch content, if it lacks visual appeal, you are not fully engaging your audience. Introducing our Analytical CRM Software To Boost Sales Powerpoint Presentation Slides SA CD deck, designed to engage your audience. Our complete deck boasts a seamless blend of Creativity and versatility. You can effortlessly customize elements and color schemes to align with your brand identity. Save precious time with our pre-designed template, compatible with Microsoft versions and Google Slides. Plus, it is downloadable in multiple formats like JPG, JPEG, and PNG. Elevate your presentations and outshine your competitors effortlessly with our visually stunning 100 percent editable deck.
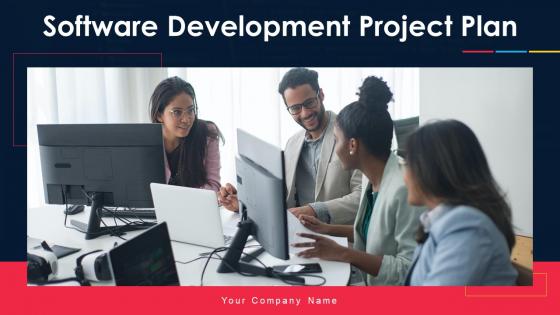
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of seventy slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
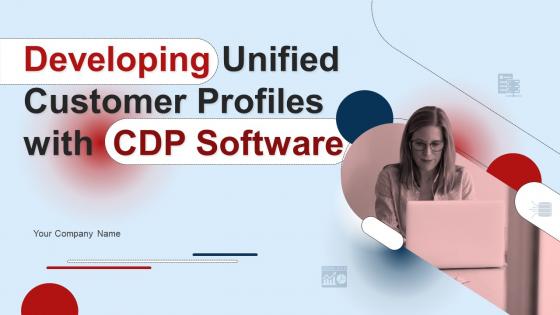
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Developing Unified Customer Profiles with CDP Software MKT CD V is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty six slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
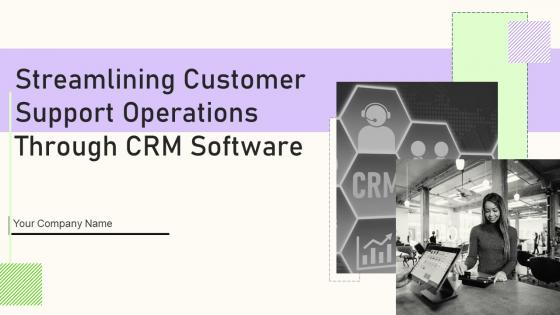
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Streamlining Customer Support Operations Through CRM Software Powerpoint Presentation Slides and has templates with professional background images and relevant content. This deck consists of total of sixty six slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
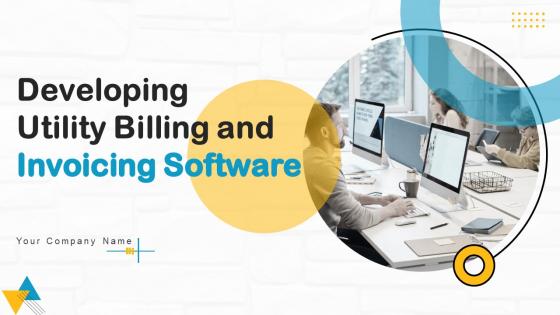
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Developing Utility Billing And Invoicing Software Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty five slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
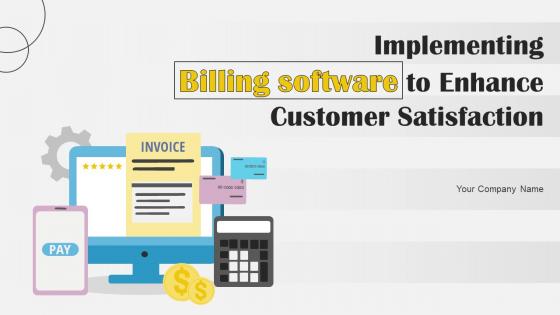
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Implementing Billing Software To Enhance Customer Satisfaction Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty five slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
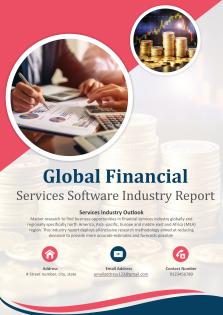
You can survive and sail through cut throat competition if you have the right skills and products at hand. If a business plan is on your upcoming agenda, then it will not be wise of you to proceed in absence of our well designed Global Financial Services Software Industry Report Pdf Word Document IR V document. Our business plan word document swears by in depth detailing and thus answers every question that may hit you or your audience at any point of time. Whats more, are the multi fold benefits that our word document offers. Made up of high resolution graphics, this document does not hamper when projected on a A4 screen. Being pre designed and thoroughly editable this ready made business plan saves a lot of the presenters time and efforts which otherwise get wasted in designing the business plan from scratch. We make our business plan word documents available to you keeping in mind the competitive edge. Join your hands with us now.
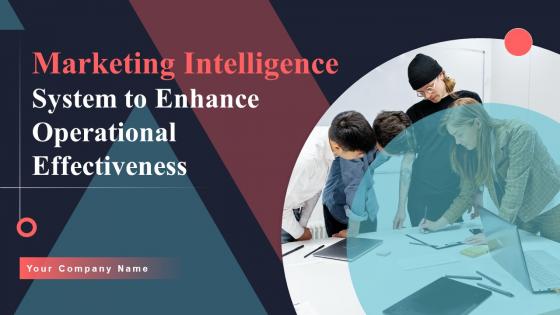
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Marketing Intelligence System To Enhance Operational Effectiveness MKT CD V and has templates with professional background images and relevant content. This deck consists of total of sixty three slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
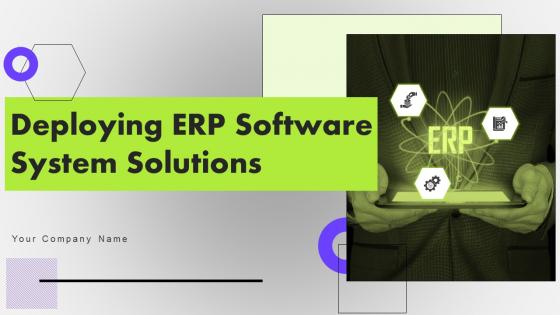
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Deploying ERP Software System Solutions Complete Deck is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty three slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
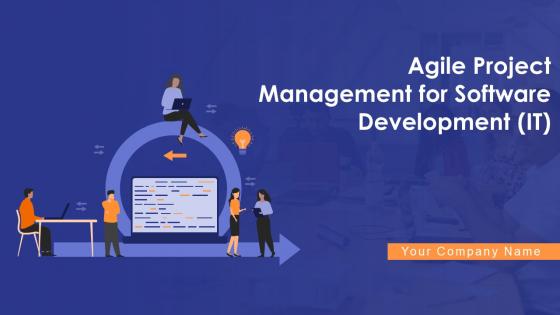
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Agile Project Management For Software Development IT Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty three slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
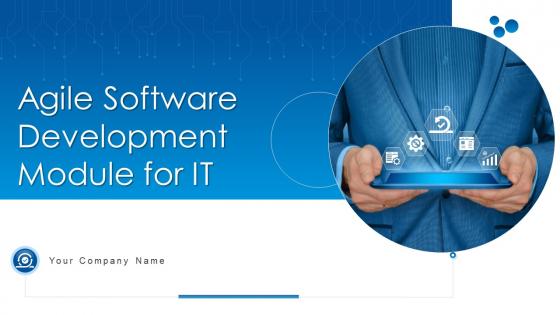
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of sixty seven slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
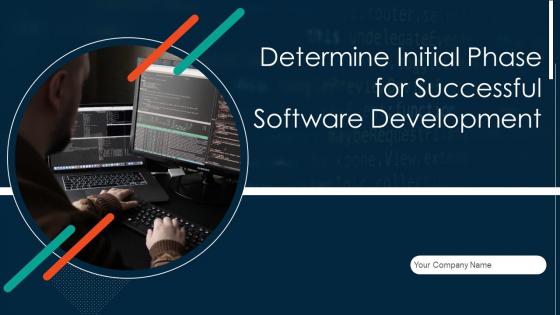
Enthrall your audience with this Determine Initial Phase For Successful Software Development Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising sixty six slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
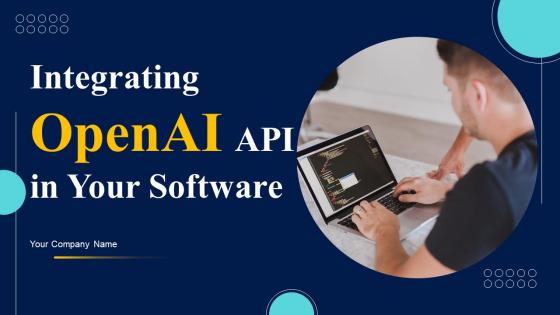
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of sixty two slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
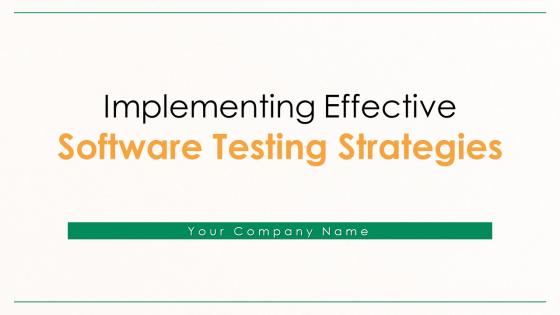
Deliver an informational PPT on various topics by using this Implementing Effective Software Testing Strategies Powerpoint Presentation Slides. This deck focuses and implements best industry practices, thus providing a birds-eye view of the topic. Encompassed with sixty two slides, designed using high-quality visuals and graphics, this deck is a complete package to use and download. All the slides offered in this deck are subjective to innumerable alterations, thus making you a pro at delivering and educating. You can modify the color of the graphics, background, or anything else as per your needs and requirements. It suits every business vertical because of its adaptable layout.
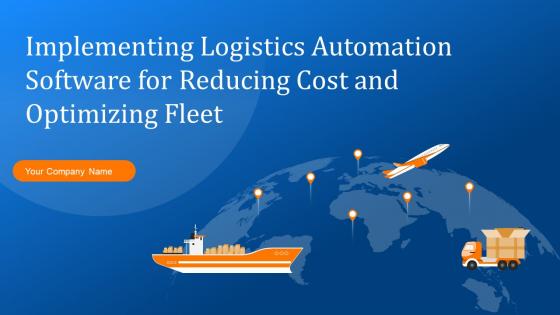
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Implementing Logistics Automation Software For Reducing Cost And Optimizing Fleet Complete Deck is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty six slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
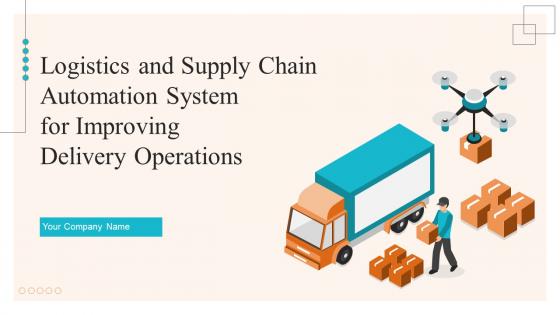
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of sixty six slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.
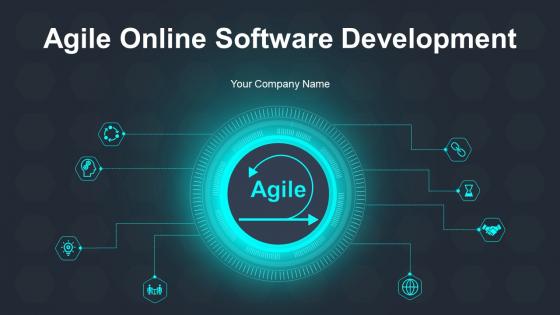
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Agile Online Software Development Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty three slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
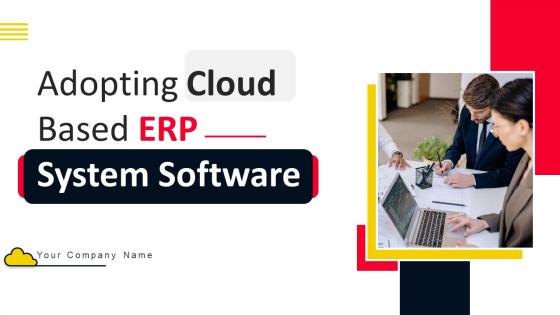
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Adopting Cloud Based ERP System Software Complete Deck is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty two slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
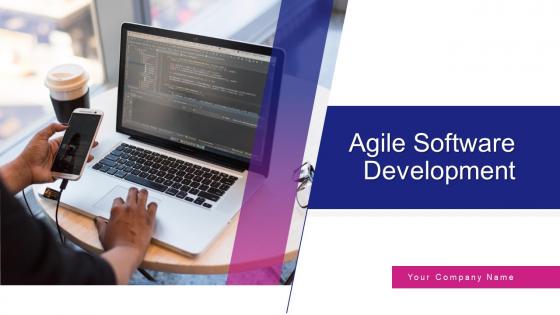
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Agile Software Development Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty two slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
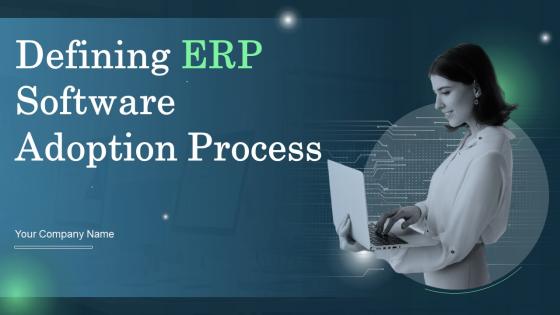
Enthrall your audience with this Defining ERP Software Adoption Process Complete Deck. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising fifty seven slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
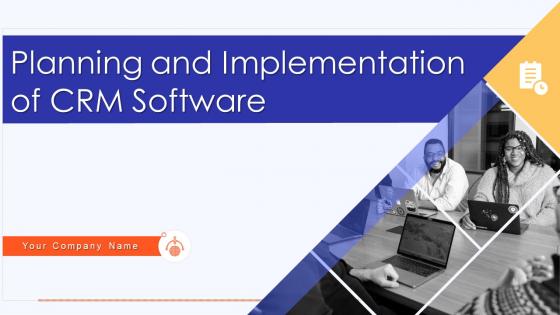
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Planning And Implementation Of CRM Software Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the sixty one slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
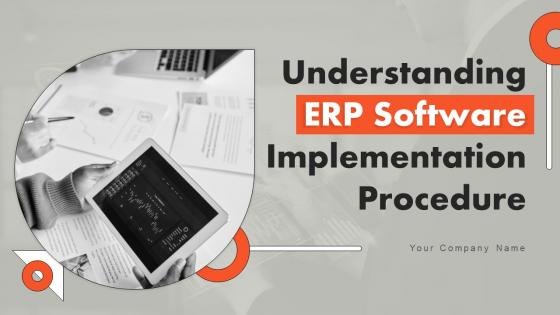
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Understanding ERP Software Implementation Procedure Complete Deck is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty six slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
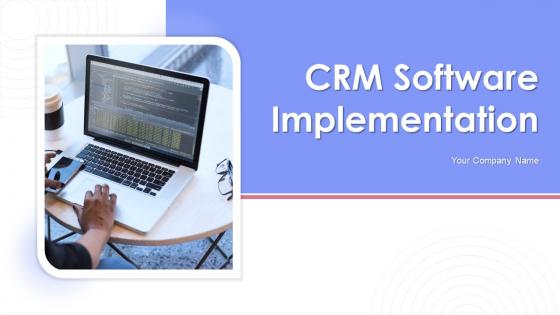
Enthrall your audience with this CRM Software Implementation Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising sixty slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
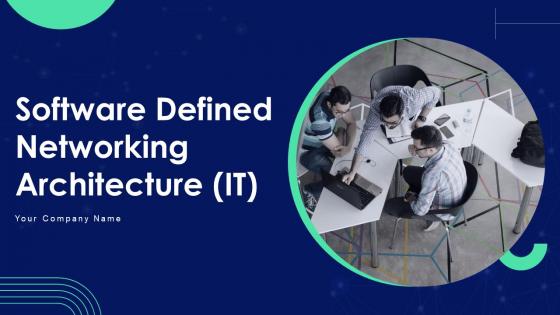
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Software Defined Networking Architecture IT Powerpoint Presentation Slides and has templates with professional background images and relevant content. This deck consists of total of fifty nine slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
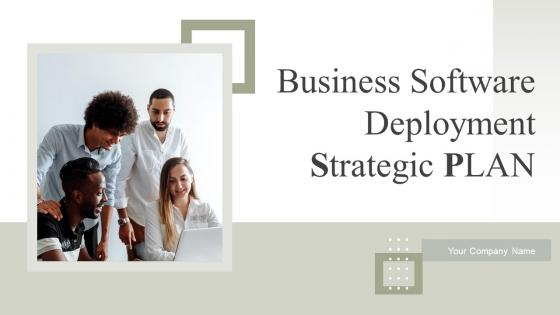
Enthrall your audience with this Business Software Deployment Strategic Plan Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising fifty nine slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
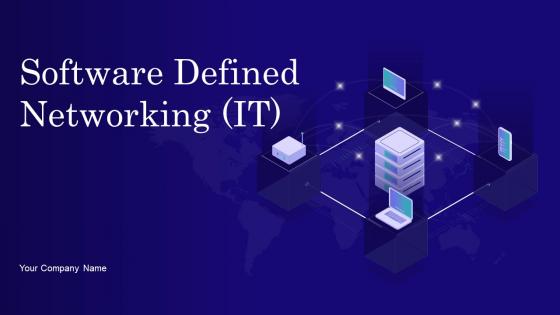
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Software Defined Networking IT Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty nine slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
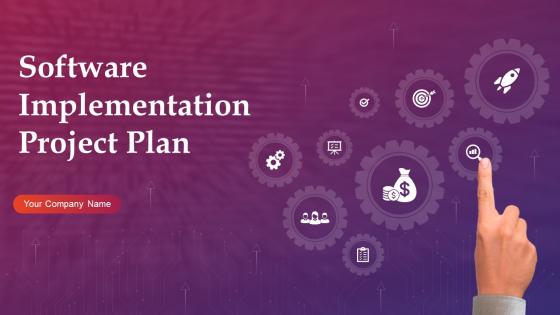
Enthrall your audience with this Software Implementation Project Plan Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising fifty eight slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
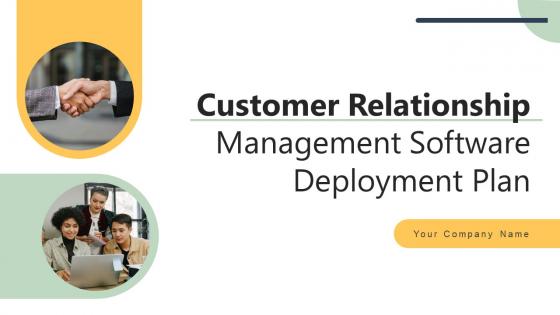
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Customer Relationship Management Software Deployment Plan SA CD is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty four slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
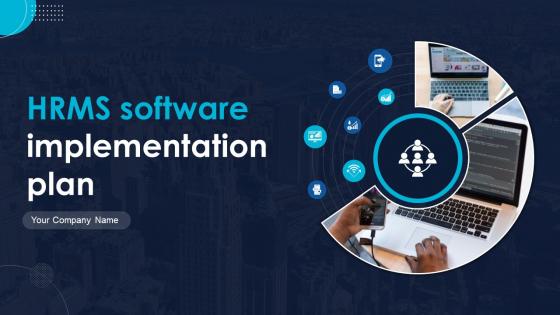
Enthrall your audience with this HRMS Software Implementation Plan Powerpoint Presentation Slides. Increase your presentation threshold by deploying this well-crafted template. It acts as a great communication tool due to its well-researched content. It also contains stylized icons, graphics, visuals etc, which make it an immediate attention-grabber. Comprising fifty four slides, this complete deck is all you need to get noticed. All the slides and their content can be altered to suit your unique business setting. Not only that, other components and graphics can also be modified to add personal touches to this prefabricated set.
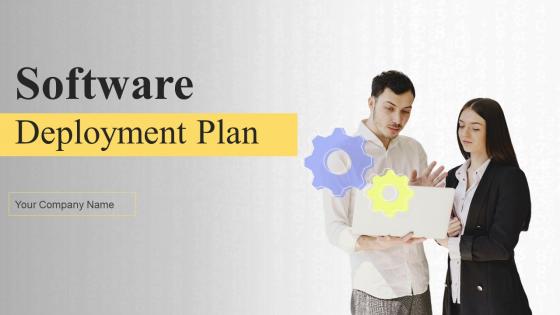
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes Software Deployment Plan Powerpoint Presentation Slides and has templates with professional background images and relevant content. This deck consists of total of fifty eight slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
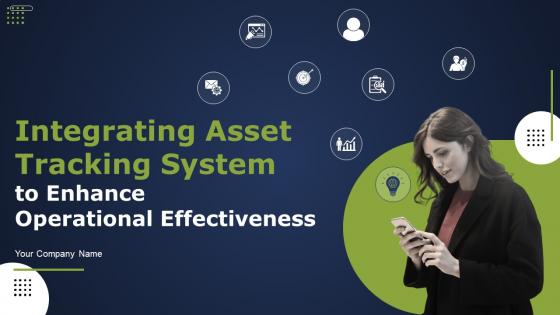
Deliver an informational PPT on various topics by using this Integrating Asset Tracking System To Enhance Operational Effectiveness Complete Deck. This deck focuses and implements best industry practices, thus providing a birds-eye view of the topic. Encompassed with fifty eight slides, designed using high-quality visuals and graphics, this deck is a complete package to use and download. All the slides offered in this deck are subjective to innumerable alterations, thus making you a pro at delivering and educating. You can modify the color of the graphics, background, or anything else as per your needs and requirements. It suits every business vertical because of its adaptable layout.
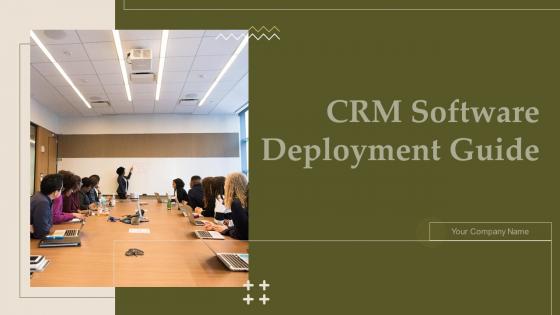
This complete deck covers various topics and highlights important concepts. It has PPT slides which cater to your business needs. This complete deck presentation emphasizes CRM Software Deployment Guide Powerpoint Presentation Slides and has templates with professional background images and relevant content. This deck consists of total of fifty seven slides. Our designers have created customizable templates, keeping your convenience in mind. You can edit the color, text and font size with ease. Not just this, you can also add or delete the content if needed. Get access to this fully editable complete presentation by clicking the download button below.
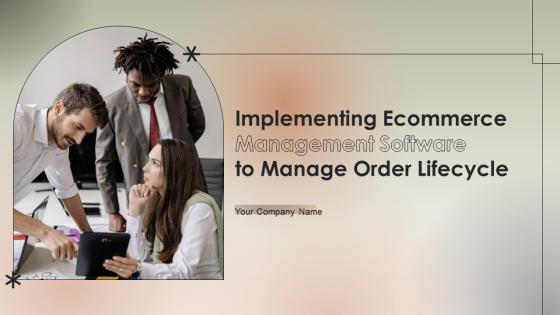
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Implementing Ecommerce Management Software To Manage Order Lifecycle Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty seven slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
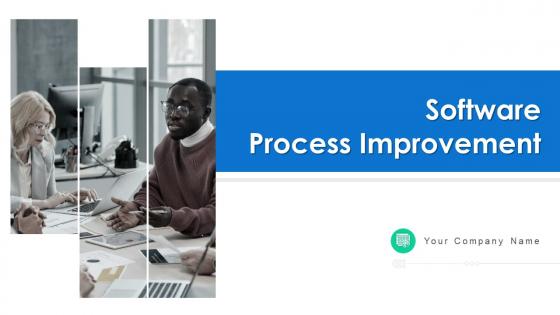
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Software Process Improvement Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty three slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
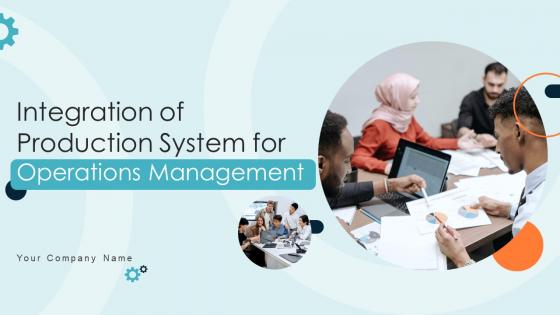
Ditch the Dull templates and opt for our engaging Integration Of Production System For Operations Management Strategy CD deck to attract your audience. Our visually striking design effortlessly combines creativity with functionality, ensuring your content shines through. Compatible with Microsoft versions and Google Slides, it offers seamless integration of presentation. Save time and effort with our pre-designed PPT layout, while still having the freedom to customize fonts, colors, and everything you ask for. With the ability to download in various formats like JPG, JPEG, and PNG, sharing your slides has never been easier. From boardroom meetings to client pitches, this deck can be the secret weapon to leaving a lasting impression.
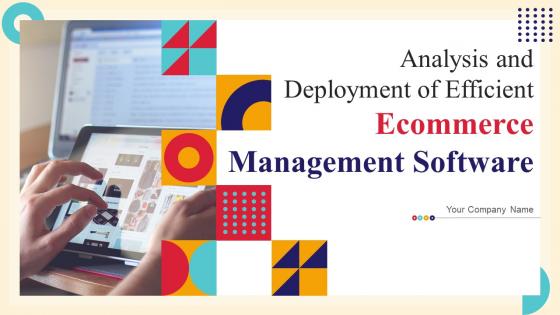
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Analysis And Deployment Of Efficient Ecommerce Management Software Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty six slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
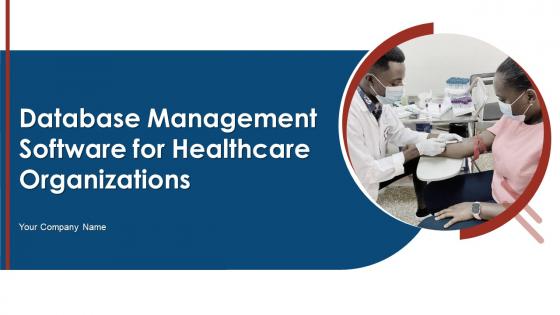
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Database Management Software For Healthcare Organizations Complete Deck is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty six slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
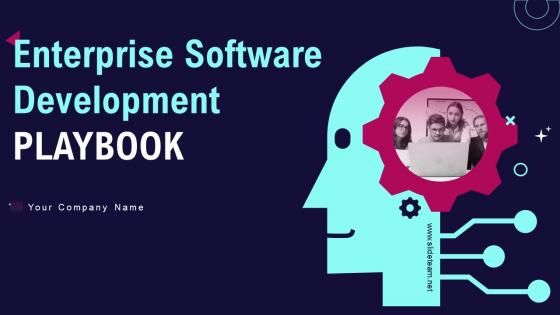
Deliver an informational PPT on various topics by using this Enterprise Software Development Playbook Powerpoint Presentation Slides. This deck focuses and implements best industry practices, thus providing a birds-eye view of the topic. Encompassed with fifty four slides, designed using high-quality visuals and graphics, this deck is a complete package to use and download. All the slides offered in this deck are subjective to innumerable alterations, thus making you a pro at delivering and educating. You can modify the color of the graphics, background, or anything else as per your needs and requirements. It suits every business vertical because of its adaptable layout.
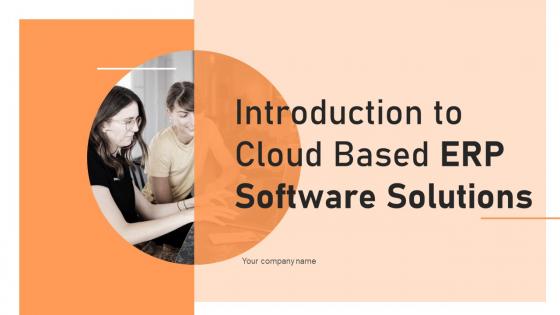
This complete presentation has PPT slides on wide range of topics highlighting the core areas of your business needs. It has professionally designed templates with relevant visuals and subject driven content. This presentation deck has total of fifty four slides. Get access to the customizable templates. Our designers have created editable templates for your convenience. You can edit the color, text and font size as per your need. You can add or delete the content if required. You are just a click to away to have this ready-made presentation. Click the download button now.

Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Agile Aided Software Development Playbook Ppt Template is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty four slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
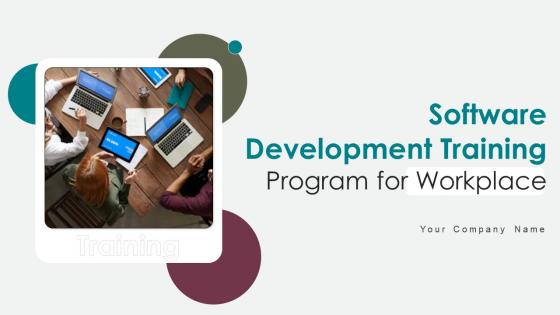
Do not compromise on a template that erodes your messages impact. Introducing our engaging Software Development Training Program For Workplace Complete Deck DTE CD complete deck, thoughtfully crafted to grab your audiences attention instantly. With this deck, effortlessly download and adjust elements, streamlining the customization process. Whether you are using Microsoft versions or Google Slides, it fits seamlessly into your workflow. Furthermore, its accessible in JPG, JPEG, PNG, and PDF formats, facilitating easy sharing and editing. Not only that you also play with the color theme of your slides making it suitable as per your audiences preference.
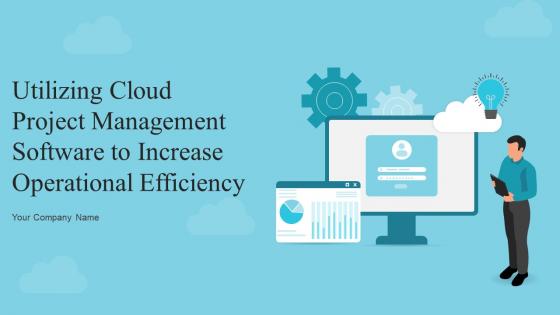
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Utilizing Cloud Project Management Software To Increase Operational Efficiency Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the fifty three slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.
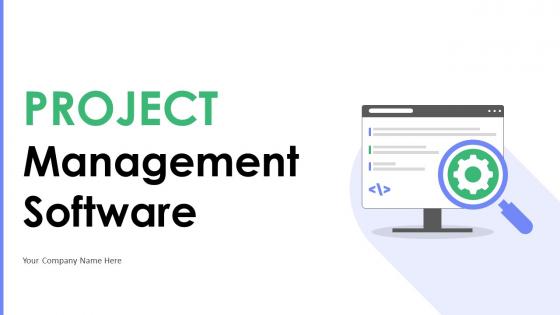
Deliver this complete deck to your team members and other collaborators. Encompassed with stylized slides presenting various concepts, this Project Management Software Powerpoint Presentation Slides is the best tool you can utilize. Personalize its content and graphics to make it unique and thought-provoking. All the forty eight slides are editable and modifiable, so feel free to adjust them to your business setting. The font, color, and other components also come in an editable format making this PPT design the best choice for your next presentation. So, download now.

Introducing Copilot+ PCs
May 20, 2024 | Yusuf Mehdi - Executive Vice President, Consumer Chief Marketing Officer
- Share on Facebook (opens new window)
- Share on Twitter (opens new window)
- Share on LinkedIn (opens new window)

An on-demand recording of our May 20 event is available .
Today, at a special event on our new Microsoft campus, we introduced the world to a new category of Windows PCs designed for AI, Copilot+ PCs.
Copilot+ PCs are the fastest, most intelligent Windows PCs ever built. With powerful new silicon capable of an incredible 40+ TOPS (trillion operations per second), all – day battery life and access to the most advanced AI models, Copilot+ PCs will enable you to do things you can’t on any other PC. Easily find and remember what you have seen in your PC with Recall, generate and refine AI images in near real-time directly on the device using Cocreator, and bridge language barriers with Live Captions, translating audio from 40+ languages into English .
These experiences come to life on a set of thin, light and beautiful devices from Microsoft Surface and our OEM partners Acer, ASUS, Dell, HP, Lenovo and Samsung, with pre-orders beginning today and availability starting on June 18. Starting at $999, Copilot+ PCs offer incredible value.
This first wave of Copilot+ PCs is just the beginning. Over the past year, we have seen an incredible pace of innovation of AI in the cloud with Copilot allowing us to do things that we never dreamed possible. Now, we begin a new chapter with AI innovation on the device. We have completely reimagined the entirety of the PC – from silicon to the operating system, the application layer to the cloud – with AI at the center, marking the most significant change to the Windows platform in decades.
YouTube Video
The fastest, most secure Windows PCs ever built
We introduced an all-new system architecture to bring the power of the CPU, GPU, and now a new high performance Neural Processing Unit (NPU) together. Connected to and enhanced by the large language models (LLMs) running in our Azure Cloud in concert with small language models (SLMs), Copilot+ PCs can now achieve a level of performance never seen before. They are up to 20x more powerful [1] and up to 100x as efficient [2] for running AI workloads and deliver industry-leading AI acceleration. They outperform Apple’s MacBook Air 15” by up to 58% in sustained multithreaded performance [3] , all while delivering all-day battery life. With incredible efficiency, Copilot+ PCs can deliver up to 22 hours of local video playback or 15 hours of web browsing on a single charge. [4] That is up to 20% more battery in local video playback than the MacBook Air 15”. [5]
Windows now has the best implementation of apps on the fastest chip, starting with Qualcomm. We now offer more native Arm64 experiences than ever before, including our fastest implementation of Microsoft 365 apps like Teams, PowerPoint, Outlook, Word, Excel, OneDrive and OneNote. Chrome, Spotify, Zoom, WhatsApp, Adobe Photoshop, Adobe Lightroom, Blender, Affinity Suite, DaVinci Resolve and many more now run natively on Arm to give you great performance with additional apps, like Slack, releasing later this year. In fact, 87% of the total app minutes people spend in apps today have native Arm versions. [6] With a powerful new emulator, Prism, your apps run great, whether native or emulated.
Every Copilot+ PC comes secured out of the box. The Microsoft Pluton Security processor will be enabled by default on all Copilot+ PCs and we have introduced a number of new features, updates and defaults to Windows 11 that make it easy for users to stay secure. And, we’ve built in personalized privacy controls to help you protect what’s important to you. You can read more about how we are making Windows more secure here .
Entirely new, powerful AI experiences
Copilot+ PCs leverage powerful processors and multiple state-of-the-art AI models, including several of Microsoft’s world-class SLMs, to unlock a new set of experiences you can run locally, directly on the device. This removes previous limitations on things like latency, cost and even privacy to help you be more productive, creative and communicate more effectively.
Recall instantly
We set out to solve one of the most frustrating problems we encounter daily – finding something we know we have seen before on our PC. Today, we must remember what file folder it was stored in, what website it was on, or scroll through hundreds of emails trying to find it.
Now with Recall, you can access virtually what you have seen or done on your PC in a way that feels like having photographic memory. Copilot+ PCs organize information like we do – based on relationships and associations unique to each of our individual experiences. This helps you remember things you may have forgotten so you can find what you’re looking for quickly and intuitively by simply using the cues you remember. [7]
You can scroll across time to find the content you need in your timeline across any application, website, document, or more. Interact intuitively using snapshots with screenray to help you take the next step using suggested actions based on object recognition. And get back to where you were, whether to a specific email in Outlook or the right chat in Teams.
Recall leverages your personal semantic index, built and stored entirely on your device. Your snapshots are yours; they stay locally on your PC. You can delete individual snapshots, adjust and delete ranges of time in Settings, or pause at any point right from the icon in the System Tray on your Taskbar. You can also filter apps and websites from ever being saved. You are always in control with privacy you can trust.
Cocreate with AI-powered image creation and editing, built into Windows
Since the launch of Image Creator, almost 10 billion images have been generated, helping more people bring their ideas to life easily by using natural language to describe what they want to create. Yet, today’s cloud offerings may limit the number of images you can create, keep you waiting while the artwork processes or even present privacy concerns. By using the Neural Processing Units (NPUs) and powerful local small language models, we are bringing innovative new experiences to your favorite creative applications like Paint and Photos.
Combine your ink strokes with text prompts to generate new images in nearly real time with Cocreator. As you iterate, so does the artwork, helping you more easily refine, edit and evolve your ideas. Powerful diffusion-based algorithms optimize for the highest quality output over minimum steps to make it feel like you are creating alongside AI. Use the creativity slider to choose from a range of artwork from more literal to more expressive. Once you select your artwork, you can continue iterating on top of it, helping you express your ideas, regardless of your creative skills.
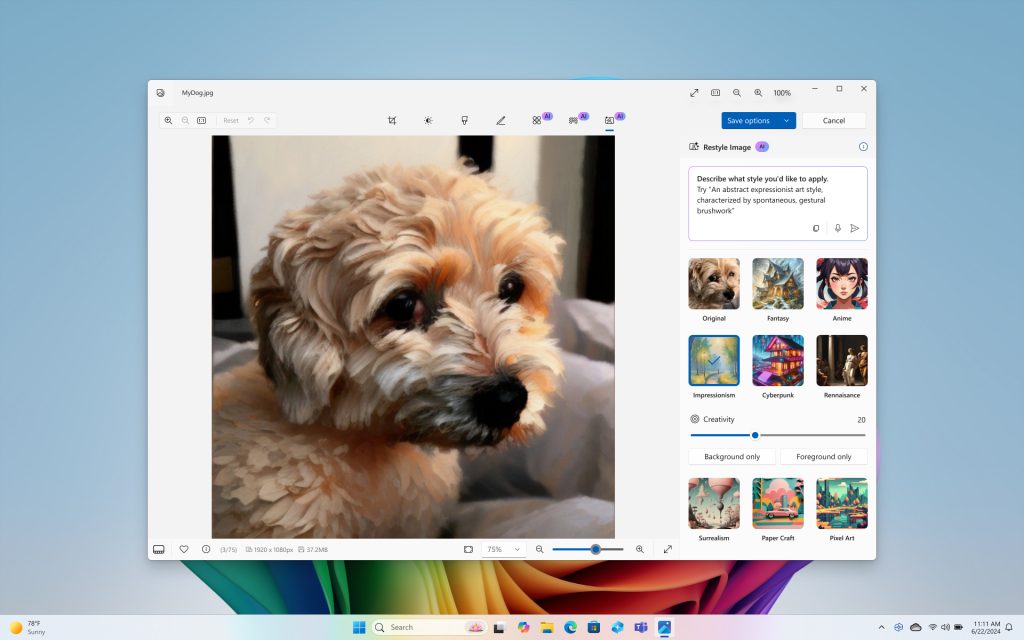
Take photo editing and image creation to the next level. With Restyle Image, you can reimagine your personal photos with a new style combining image generation and photo editing in Photos. Use a pre-set style like Cyberpunk or Claymation to change the background, foreground or full picture to create an entirely new image. Or jumpstart your next creative project and get visual inspiration with Image Creator in Photos. On Copilot+ PCs you can generate endless images for free, fast, with the ability to fine tune images to your liking and to save your favorites to collections.
Innovative AI experiences from the creative apps you love
We are also partnering with some of the biggest and most-loved applications on the planet to leverage the power of the NPU to deliver new innovative AI experiences.
Together with Adobe, we are thrilled to announce Adobe’s flagship apps are coming to Copilot+ PCs, including Photoshop, Lightroom and Express – available today. Illustrator, Premiere Pro and more are coming this summer. And we’re continuing to partner to optimize AI in these apps for the NPU. For Adobe Creative Cloud customers, they will benefit from the full performance advantages of Copilot+ PCs to express their creativity faster than ever before.
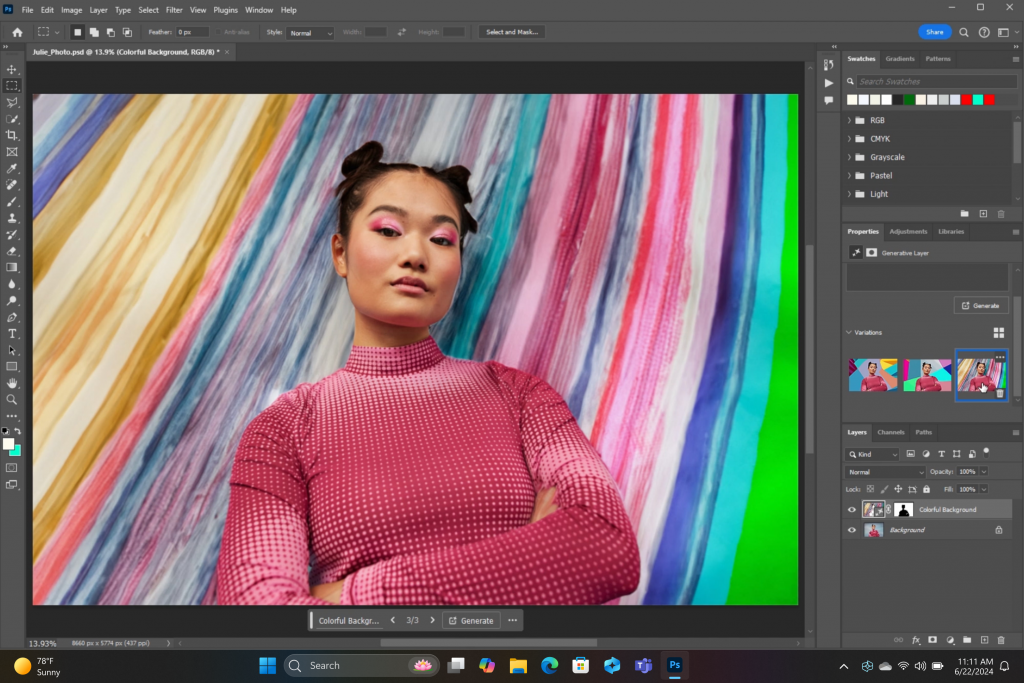
DaVinci Resolve Studio
Effortlessly apply visual effects to objects and people using NPU-accelerated Magic Mask in DaVinci Resolve Studio.
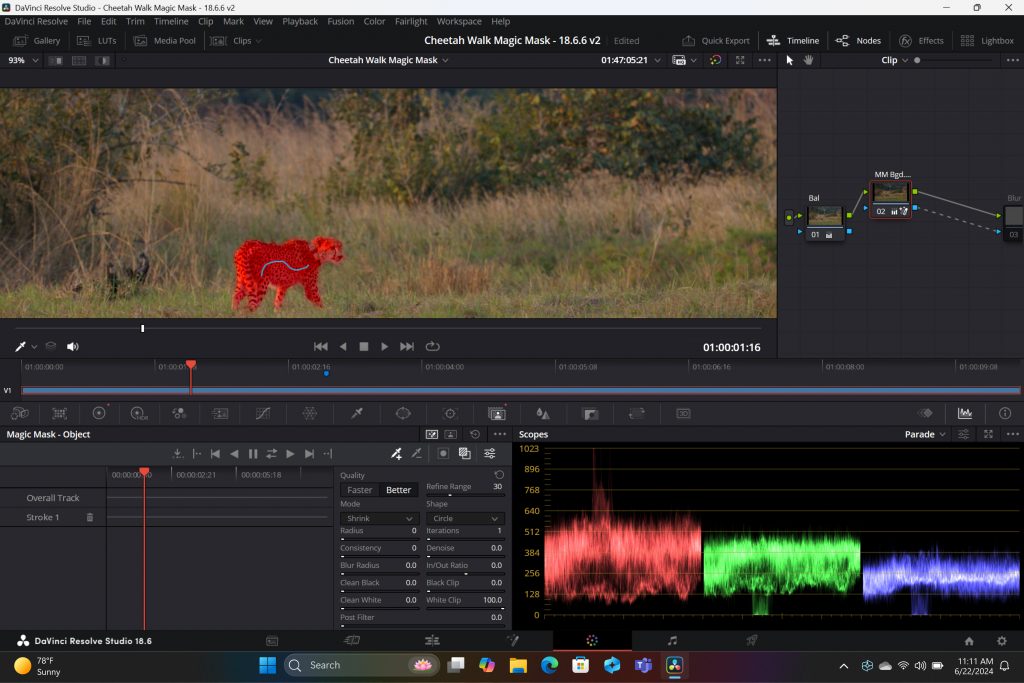
Remove the background from any video clip in a snap using Auto Cutout running on the NPU in CapCut.
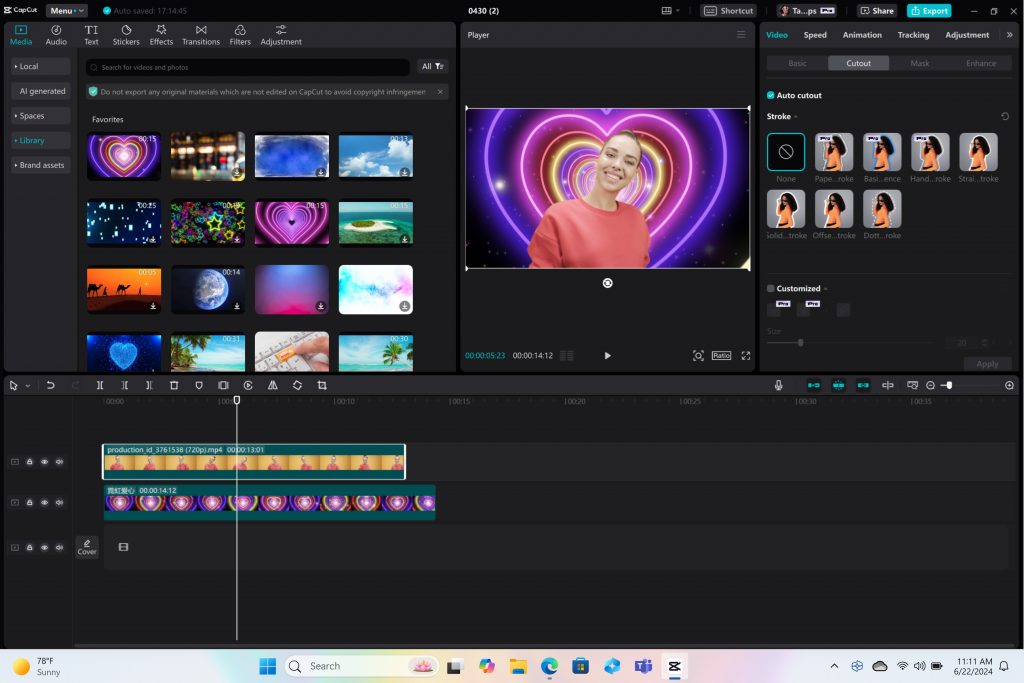
Stay in your flow with faster, more responsive adaptive input controls, like head movement or facial expressions via the new NPU-powered camera pipeline in Cephable.
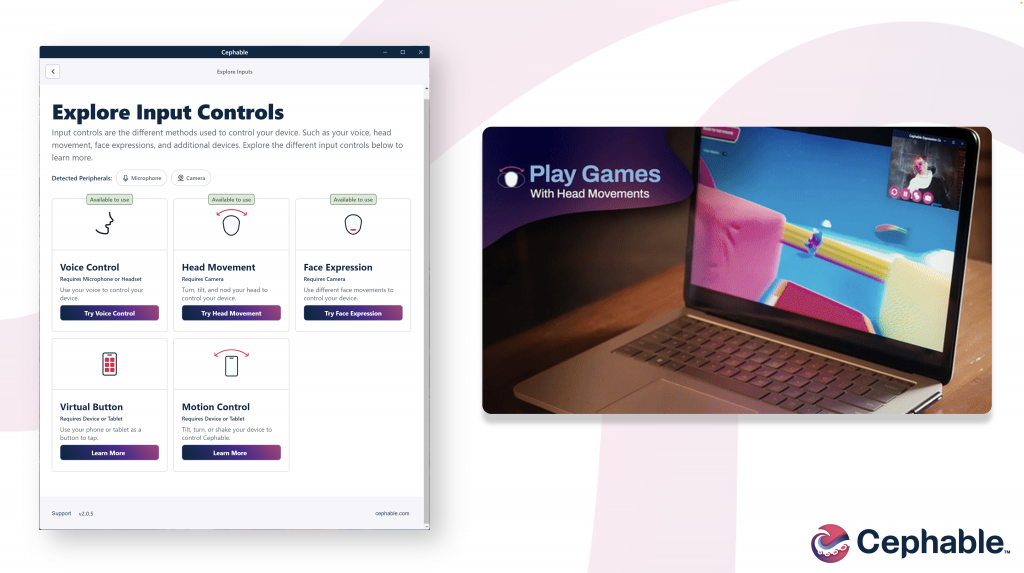
LiquidText
Make quicker and smarter annotations to documents, using AI features that run entirely on-device via NPU, so data stays private in LiquidText.
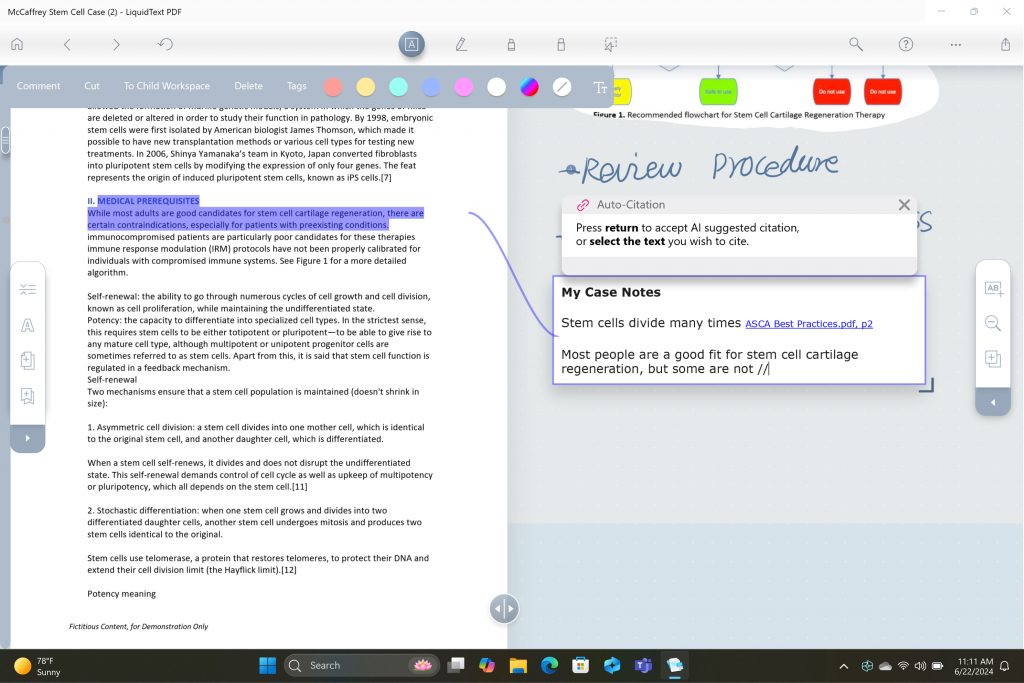
Have fun breaking down and remixing any music track, with a new, higher-quality version of NeuralMix™ that’s exclusive to NPU in Algoriddim’s djay Pro.

Connect and communicate effortlessly with live captions
In an increasingly connected and global world, Windows wants to bring people closer together. Whether catching up on your favorite podcast from a different country, or watching your favorite international sports team, or even collaborating with friends and colleagues across the world, we want to make more content accessible to more people.
Live Captions now has live translations and will turn any audio that passes through your PC into a single, English-language caption experience, in real time on your screen across all your apps consistently. You can translate any live or pre-recorded audio in any app or video platform from over 40 languages into English subtitles instantly, automatically and even while you’re offline. Powered by the NPU and available across all Copilot+ PCs, now you can have confidence your words are understood as intended.
New and enhanced Windows Studio Effects
Look and sound your best automatically with easily accessible controls at your fingertips in Quick Settings. Portrait light automatically adjusts the image to improve your perceived illumination in a dark environment or brighten the foreground pixels when in a low-light environment. Three new creative filters (illustrated, animated or watercolor) add an artistic flare. Eye contact teleprompter helps you maintain eye contact while reading your screen. New improvements to voice focus and portrait blur help ensure you’re always in focus.
Copilot, your everyday AI companion
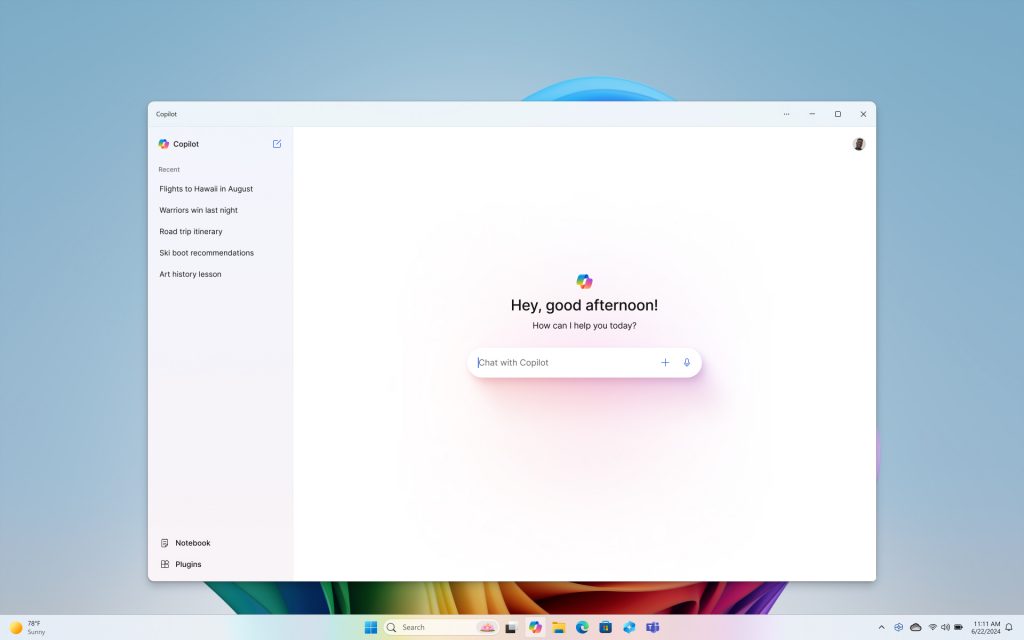
Every Copilot+ PC comes with your personal powerful AI agent that is just a single tap away on keyboards with the new Copilot key. [8] Copilot will now have the full application experience customers have been asking for in a streamlined, simple yet powerful and personal design. Copilot puts the most advanced AI models at your fingertips. In the coming weeks, get access to the latest models including GPT-4o from our partners at OpenAI, so you can have voice conversations that feel more natural.
Advancing AI responsibly
At Microsoft, we have a company-wide commitment to develop ethical, safe and secure AI. Our responsible AI principles guided the development of these new experiences, and all AI features are aligned with our standards. Learn more here .
New Copilot+ PCs from Microsoft Surface and our partners
We have worked with each of the top OEMs — Acer, ASUS, Dell, HP, Lenovo, Samsung — and of course Surface, to bring exciting new Copilot+ PCs that will begin to launch on June 18. Starting at $999, these devices are up to $200 less than similar spec’d devices [9] .
Surface plays a key role in the Windows ecosystem, as we design software and hardware together to deliver innovative designs and meaningful experiences to our customers and fans. We are introducing the first-ever Copilot+ PCs from Surface: The all-new Surface Pro and Surface Laptop.
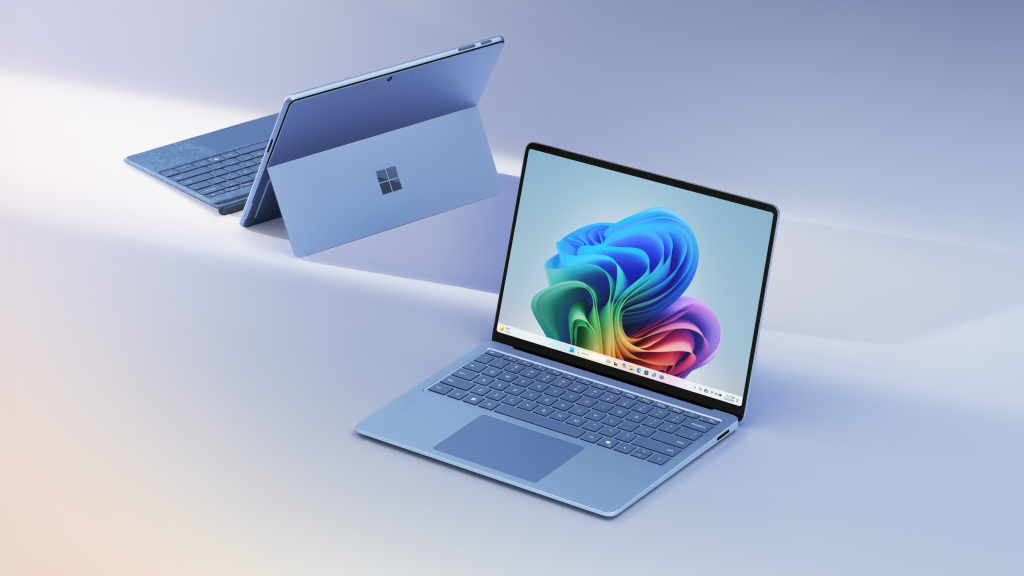
The new Surface Laptop is a powerhouse in an updated, modern laptop design with razor-thin bezels, a brilliant touchscreen display, AI-enhanced camera, premium audio, and now with a haptic touchpad.
Choose between a 13.8” and 15” display and four stunning colors. Enjoy up to 22 hours of local video playback on Surface Laptop 15” or up to 20 hours on Surface Laptop13.8” on top of incredible performance and all-new AI experiences.
The new Surface Pro is the most flexible 2-in-1 laptop, now reimagined with more speed and battery life to power all-new AI experiences. It introduces a new, optional OLED with HDR display, and ultrawide field of view camera perfect for Windows Studio Effects. The new Surface Pro Flex Keyboard is the first 2-in-1 keyboard designed to be used both attached or detached. It delivers enhanced stability, with Surface Slim Pen storage and charging integrated seamlessly, as well as a quiet, haptic touchpad. Learn more here.
New Copilot+ PCs from the biggest brands available starting June 18:
- Acer : Acer’s Swift 14 AI 2.5K touchscreen enables you to draw and edit your vision with greater accuracy and with color-accurate imagery. Launch and discover AI-enhanced features, like Acer PurifiedVoice 2.0 and Purified View, with a touch of the dedicated AcerSense button.
- ASUS : The ASUS Vivobook S 15 is a powerful device that brings AI experiences to life with its Snapdragon X Elite Platform and built-in Qualcomm® AI. It boasts 40+ NPU TOPS, a dual-fan cooling system, and up to 1 TB of storage. Next-gen AI enhancements include Windows Studio effects v2 and ASUS AiSense camera, with presence-detection capabilities for Adaptive Dimming and Lock. Built for portability, it has an ultra-slim and light all-metal design, a high-capacity battery, and premium styling with a single-zone RGB backlit keyboard.
- Dell : Dell is launching five new Copilot+ PCs, including the XPS 13, Inspiron 14 Plus, Inspiron 14, Latitude 7455, and Latitude 5455, offering a range of consumer and commercial options that deliver groundbreaking battery life and unique AI experiences. The XPS 13 is powered by Snapdragon X Elite processors and features a premium, futuristic design, while the Latitude 7455 boasts a stunning QHD+ display and quad speakers with AI noise reduction. The Inspiron14 and Inspiron 14 Plus feature a Snapdragon X Plus 1and are crafted with lightweight, low carbon aluminum and are energy efficient with EPEAT Gold rating.
- HP : HP’s OmniBook X AI PC and HP EliteBook Ultra G1q AI PC with Snapdragon X Elite are slim and sleek designs, delivering advanced performance and mobility for a more personalized computing experience. Features include long-lasting battery life and AI-powered productivity tools, such as real-time transcription and meeting summaries. A 5MP camera with automatic framing and eye focus is supported by Poly Studio’s crystal-clear audio for enhanced virtual interactions.
- Lenovo : Lenovo is launching two AI PCs: one built for consumers, Yoga Slim 7x, and one for commercial, ThinkPad T14s Gen 6. The Yoga Slim 7x brings efficiency for creatives, featuring a 14.5” touchscreen with 3K Dolby Vision and optimized power for 3D rendering and video editing. The T14s Gen 6 brings enterprise-level experiences and AI performance to your work tasks, with features including a webcam privacy shutter, Wi-Fi 7 connectivity and up to 64GB RAM.
- Samsung : Samsung’s new Galaxy Book4 Edge is ultra-thin and light, with a 3K resolution 2x AMOLED display and Wi-Fi 7 connectivity. It has a long-lasting battery that provides up to 22 hours of video playback, making it perfect for work or entertainment on the go.
Learn more about new Copilot+ PCs and pre-order today at Microsoft.com and from major PC manufacturers, as well as other leading global retailers.
Start testing for commercial deployment today
Copilot+ PCs offer businesses the most performant Windows 11 devices with unique AI capabilities to unlock productivity, improve collaboration and drive efficiency. As a Windows PC, businesses can deploy and manage a Copilot+ PC with the same tools and processes used today including IT controls for new features and AppAssure support. We recommend IT admins begin testing and readying for deployment to start empowering your workforce with access to powerful AI features on these high-performance devices. You can read more about our commercial experiences here .
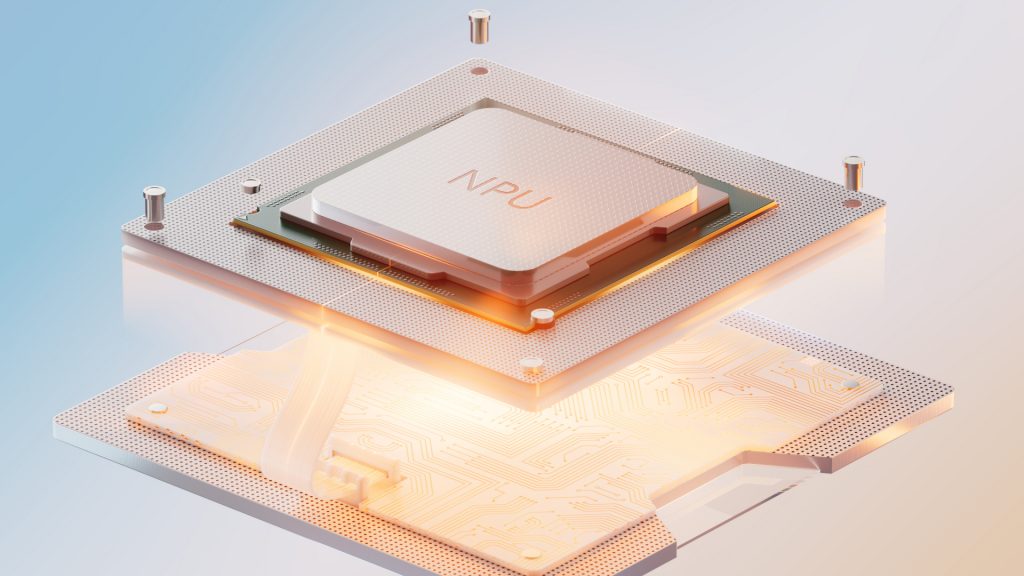
AI innovation across the Windows ecosystem
Like we’ve always done with Windows, we have built a platform for our ecosystem partners to build on.
The first Copilot+ PCs will launch with both the Snapdragon® X Elite and Snapdragon® X Plus processors and feature leading performance per watt thanks to the custom Qualcomm Oryon™ CPU, which delivers unrivaled performance and battery efficiency. Snapdragon X Series delivers 45 NPU TOPS all-in-one system on a chip (SoC). The premium integrated Qualcomm® Adreno ™ GPU delivers stunning graphics for immersive entertainment. We look forward to expanding through deep partnerships with Intel and AMD, starting with Lunar Lake and Strix Point. We will bring new Copilot+ PC experiences at a later date. In the future we expect to see devices with this silicon paired with powerful graphics cards like NVIDIA GeForce RTX and AMD Radeon™, bringing Copilot+ PC experiences to reach even broader audiences like advanced gamers and creators.
We are at an inflection point where the PC will accelerate AI innovation. We believe the richest AI experiences will only be possible when the cloud and device work together in concert. Together with our partners, we’re setting the frame for the next decade of Windows innovation.
[1] Based on snapshot of aggregated, non-gaming app usage data as of April 2024 for iGPU-based laptops and 2-in-1 devices running Windows 10 and Windows 11 in US, UK, CA, FR, AU, DE, JP.
[2] Tested April 2024 using Phi SLM workload running 512-token prompt processing in a loop with default settings comparing pre-release Copilot+ PC builds with Snapdragon Elite X 12 Core and Snapdragon X Plus 10 core configurations (QNN build) to Windows 11 PC with NVIDIA 4080 GPU configuration (CUDA build).
[3] Tested May 2024 using Cinebench 2024 Multi-Core benchmark comparing Copilot+ PCs with Snapdragon X Elite 12 core and Snapdragon X Plus 10 core configurations to MacBook Air 15” with M3 8 core CPU / 10 Core GPU configuration. Performance will vary significantly between device configuration and usage.
[4] *Battery life varies significantly by device and with settings, usage and other factors. See aka.ms/cpclaims*
[5] *Battery life varies significantly based on device configuration, usage, network and feature configuration, signal strength, settings and other factors. Testing conducted May 2024 using the prelease Windows ADK full screen local video playback assessment under standard testing conditions, with the device connected to Wi-Fi and screen brightness set to 150 nits, comparing Copilot+ PCs with Snapdragon X Elite 12 core and Snapdragon X Plus 10 core configurations running Windows Version 26097.5003 (24H2) to MacBook Air 15” M3 8-Core CPU/ 10 Core GPU running macOS 14.4 with similar device configurations and testing scenario.
[6] Based on snapshot of aggregated, non-gaming app usage data as of April 2024 for iGPU-based laptops and 2-in-1 devices running Windows 10 and Windows 11 in US, UK, CA, FR, AU, DE, JP.
[7] Recall is optimized for select languages (English, Chinese (simplified), French, German, Japanese, and Spanish.) Content-based and storage limitations apply. Learn more here .
[8] Copilot key functionality may vary. See aka.ms/keysupport
[9] Based on MSRPs; actual savings may vary
Tags: AI , Copilot+ PC
- Check us out on RSS
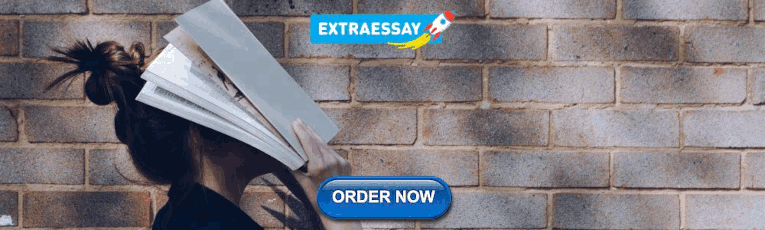
IMAGES
VIDEO
COMMENTS
In this , I add the Some Basic Idea of Operating System. It Include :- 1) Introduction and Background 2) Structure and Background 3) Main Function Of Operating System 4) Some Popular Operating System 5) Objective Of Operating System 6) Conclusion Presentation On Operating system
Operating System Concepts - slides. Avi Silberschatz. Peter Baer Galvin. Greg Gagne. We provide a set of slides to accompany each chapter. Click on the links below to download the slides in Powerpoint format. We also provide zip files of the all Powerpoint files , PDF files, and all figures used in the text. Chapter.
The slides are authorized for personal use, and for use in conjunction with a course for which Operating System Concepts is the prescribed text. Instructors are free to modify the slides to their taste, as long as the modified slides acknowledge the source and the fact that they have been modified. Paper copies of the slides may be sold ...
The slides below are copyright Silberschatz, Galvin and Gagne, 2008. The slides are authorized for personal use, and for use in conjunction with a course for which Operating System Concepts is the prescribed text. Instructors are free to modify the slides to their taste, as long as the modified slides acknowledge the source and the fact that ...
History of operating systems • Started out as a library to provide common functionality across programs • Later, evolved from procedure call to system call: what's the difference? • When a system call is made to run OS code, the CPU executes at a higher privilege level • Evolved from running a single program to multiple processes ...
Chapter 1: Introduction to Operating System. The document provides an overview of operating system concepts, including: - The four main components of a computer system: hardware, operating system, applications, and users. - What operating systems do, such as managing resources and controlling programs.
The slides below are copyright Silberschatz, Galvin and Gagne, 2018. The slides are authorized for personal use, and for use in conjunction with a course for which Operating System Concepts is the prescribed text. Instructors are free to modify the slides to their taste, as long as the modified slides acknowledge the source and the fact that ...
Operating System Hardware App 1 App 2 App N • The Operating System (OS): - controls all execution. - multiplexes resources between applications. - abstracts away from complexity. • Typically also have some libraries and some tools provided with OS. • Are these part of the OS? Is IE a tool? - no-one can agree. . .
A program that acts as an intermediary between a user of a computer and the computer hardware Operating system goals: O Execute user programs and make solving user problems easier Make the computer system convenient to use Use the computer hardware in an efficient manner. Computer System Structure Computer system can be divided into four ...
An Operating System (OS) is software that manages and handles the hardware and software resources of a computer system. It provides interaction between users of computers and computer hardware. An operating system is responsible for managing and controlling all the activities and sharing of computer resources. An operating system is a low-level ...
The slides below are copyright Silberschatz, Galvin and Gagne, 2018. The slides are authorized for personal use, and for use in conjunction with a course for which Operating System Concepts is the prescribed text. Instructors are free to modify the slides to their taste, as long as the modified slides acknowledge the source and the fact that ...
OS support tasks 1. Provides the facilities to create, modification of program and data files using an editor. 2. Access to the compiler for translating the user program from high level language to machine language. 3. Provide a loader program to move the compiled program code to computer's memory for execution.
Download presentation. Presentation on theme: "Chapter 1 Introduction to Computer Operating System."—. Presentation transcript: 1 Chapter 1 Introduction to Computer Operating System. 2 2 Objectives When finish this chapter, you will understand: what an operating system is. a brief history of operating systems. goals of operating systems ...
Data Visualization. Infographics. Charts. Blog. April 18, 2024. Use Prezi Video for Zoom for more engaging meetings. April 16, 2024. Understanding 30-60-90 sales plans and incorporating them into a presentation. April 13, 2024.
Yes. Slide Formats. 16:9. 4:3. Our picture-perfect Operating System Concepts PPT template is the best pick for software and hardware experts to impart knowledge on the environment created by an operating system to help users execute and manage programs. You can also capitalize on the set to illustrate how operating systems make it convenient ...
The slides are authorized for personal use, and for use in conjunction with a course for which Operating System Concepts is the prescribed text. Instructors are free to modify the slides to their taste, as long as the modified slides acknowledge the source and the fact that they have been modified. Paper copies of the slides may be sold ...
Presentation Transcript. Operating Systems:Internals and Design Principles Chapter 2Operating System Overview Eighth Edition By William Stallings. Operating System A program that controls the execution of application programs An interface between applications and hardware. Operating System Services Program development Program execution Access I ...
Get hold of our 100% authentic Operating System Concepts presentation template, designed for PowerPoint and Google Slides, to describe how the operating system manages different tasks, processes, and applications in a computer. You can also explain how it distributes resources to several computer programs.
This PPT presentation can be accessed with Google Slides and is available in both standard screen and widescreen aspect ratios. It is also a useful set to elucidate topics like Digital Operating System. This well-structured design can be downloaded in different formats like PDF, JPG, and PNG.
We have completely reimagined the entirety of the PC - from silicon to the operating system, ... It boasts 40+ NPU TOPS, a dual-fan cooling system, and up to 1 TB of storage. Next-gen AI enhancements include Windows Studio effects v2 and ASUS AiSense camera, with presence-detection capabilities for Adaptive Dimming and Lock. ...