Python Global Variables – How to Define a Global Variable Example
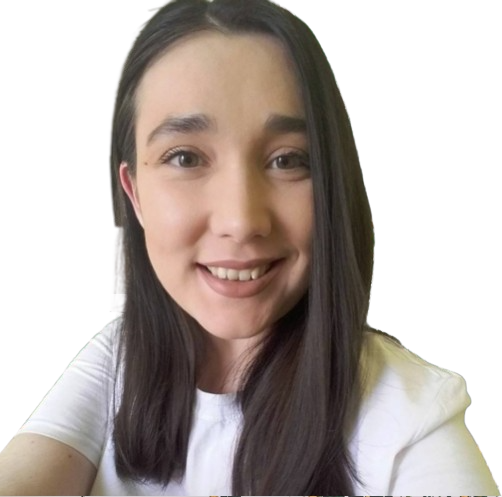
In this article, you will learn the basics of global variables.
To begin with, you will learn how to declare variables in Python and what the term 'variable scope' actually means.
Then, you will learn the differences between local and global variables and understand how to define global variables and how to use the global keyword.
Here is what we will cover:
- Variable scope explained
- How to create variables with local scope
- The global keyword
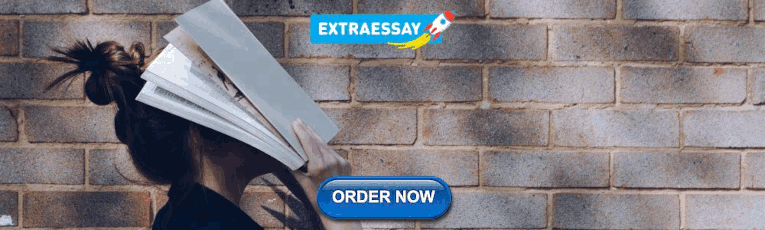
What Are Variables in Python and How Do You Create Them? An Introduction for Beginners
You can think of variables as storage containers .
They are storage containers for holding data, information, and values that you would like to save in the computer's memory. You can then reference or even manipulate them at some point throughout the life of the program.
A variable has a symbolic name , and you can think of that name as the label on the storage container that acts as its identifier.
The variable name will be a reference and pointer to the data stored inside it. So, there is no need to remember the details of your data and information – you only need to reference the variable name that holds that data and information.
When giving a variable a name, make sure that it is descriptive of the data it holds. Variable names need to be clear and easily understandable both for your future self and the other developers you may be working with.
Now, let's see how to actually create a variable in Python.
When declaring variables in Python, you don't need to specify their data type.
For example, in the C programming language, you have to mention explicitly the type of data the variable will hold.
So, if you wanted to store your age which is an integer, or int type, this is what you would have to do in C:
However, this is how you would write the above in Python:
The variable name is always on the left-hand side, and the value you want to assign goes on the right-hand side after the assignment operator.
Keep in mind that you can change the values of variables throughout the life of a program:
You keep the same variable name, my_age , but only change the value from 28 to 29 .
What Does Variable Scope in Python Mean?
Variable scope refers to the parts and boundaries of a Python program where a variable is available, accessible, and visible.
There are four types of scope for Python variables, which are also known as the LEGB rule :
- E nclosing,
For the rest of this article, you will focus on learning about creating variables with global scope, and you will understand the difference between the local and global variable scopes.
How to Create Variables With Local Scope in Python
Variables defined inside a function's body have local scope, which means they are accessible only within that particular function. In other words, they are 'local' to that function.
You can only access a local variable by calling the function.
Look at what happens when I try to access that variable with a local scope from outside the function's body:
It raises a NameError because it is not 'visible' in the rest of the program. It is only 'visible' within the function where it was defined.
How to Create Variables With Global Scope in Python
When you define a variable outside a function, like at the top of the file, it has a global scope and it is known as a global variable.
A global variable is accessed from anywhere in the program.
You can use it inside a function's body, as well as access it from outside a function:
What happens when there is a global and local variable, and they both have the same name?
In the example above, maybe you were not expecting that specific output.
Maybe you thought that the value of city would change when I assigned it a different value inside the function.
Maybe you expected that when I referenced the global variable with the line print(f" I want to visit {city} next year!") , the output would be #I want to visit London next year! instead of #I want to visit Athens next year! .
However, when the function was called, it printed the value of the local variable.
Then, when I referenced the global variable outside the function, the value assigned to the global variable was printed.
They didn't interfere with one another.
That said, using the same variable name for global and local variables is not considered a best practice. Make sure that your variables don't have the same name, as you may get some confusing results when you run your program.
How to Use the global Keyword in Python
What if you have a global variable but want to change its value inside a function?
Look at what happens when I try to do that:
By default Python thinks you want to use a local variable inside a function.
So, when I first try to print the value of the variable and then re-assign a value to the variable I am trying to access, Python gets confused.
The way to change the value of a global variable inside a function is by using the global keyword:
Use the global keyword before referencing it in the function, as you will get the following error: SyntaxError: name 'city' is used prior to global declaration .
Earlier, you saw that you couldn't access variables created inside functions since they have local scope.
The global keyword changes the visibility of variables declared inside functions.
And there you have it! You now know the basics of global variables in Python and can tell the differences between local and global variables.
I hope you found this article useful.
To learn more about the Python programming language, check out freeCodeCamp's Scientific Computing with Python Certification .
You'll start from the basics and learn in an interactive and beginner-friendly way. You'll also build five projects at the end to put into practice and help reinforce what you've learned.
Thanks for reading and happy coding!
Read more posts .
If this article was helpful, share it .
Learn to code for free. freeCodeCamp's open source curriculum has helped more than 40,000 people get jobs as developers. Get started
- Contributors
Global and Local Variables
Table of contents, scope of global variables in python, how global variables work in python, the role of non-local variables in python, the global keyword - python's global variables in function, advanced tips for python variable scopes and naming conventions.
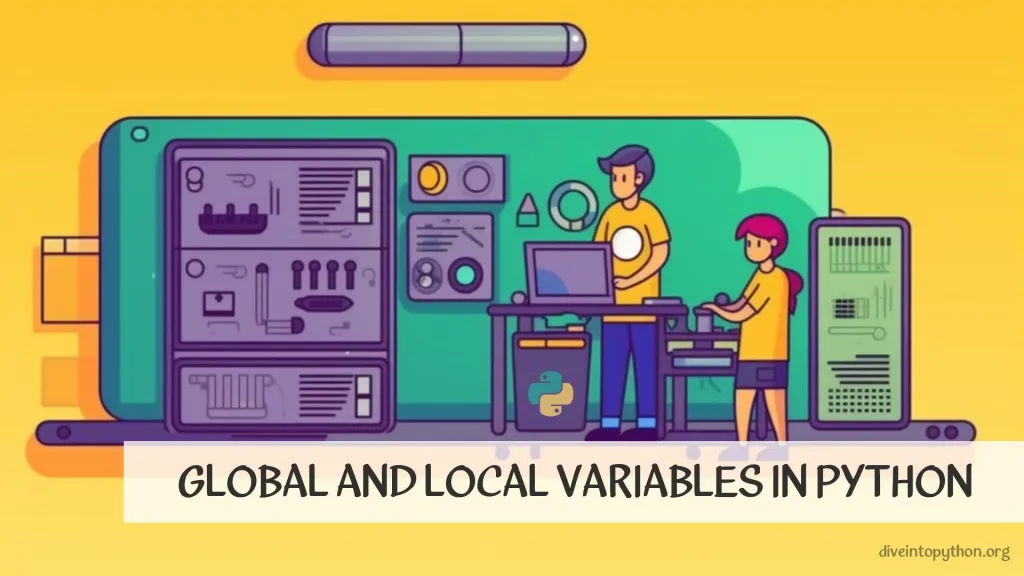
In Python, variables are used to store values that can be accessed and manipulated within a program. However, the scope of a variable can differ depending on whether it is a global variable or a local variable. Global variables in Python can be accessed from any part of the program, while local variables are limited to the function or block in which they are defined. Understanding the differences between variable scopes is important for developing efficient and effective Python code.
Global variables in Python are the variables that are defined outside of any function in a program. They can be accessed and modified by any function or module in the program.
The scope of a variable in Python defines its accessibility. There are two types of scopes in Python: Global scope and Local scope. A global scope means that a variable is accessible throughout the program, while a local scope means that a variable is accessible only within the function where it is defined.
Example 1: How to Define a Global Variable in Python
In Python, global variables can be accessed and modified from any function or module in the program. However, assigning a value to a global variable inside a function creates a new local variable within that function.
Here are some examples of how the scope of global variables works in Python:
Example 2: Accessing a Global Variable Inside a Function
In this example, the function func is accessing the global variable x which is defined outside of any function.
Example 3: Accessing the Global Variable Outside the Function
In this example, the function func is creating a new local variable x by assigning it a value of 10 . So, the print statement inside the function is referring to the local variable and not the global variable. However, when the print statement is called outside the function, it refers to the global variable x .
Global variables are variables that can be accessed and modified throughout the code, regardless of where they are declared. The variable scopes in Python determine the accessibility of variables in different parts of the code. Python supports three variable scopes - local, global, and nonlocal . Local variables are variables that are declared and used within a particular function or block of code, and their scope is limited to that particular function or block of code.
How to Change a Global Variable in Function
In Python, to set a global variable you need to declare and initialize a variable outside of any function or block. In the above code, a global variable named global_var is declared and initialized outside the function. The func() function accesses and modifies the value of global_var using the global keyword. Finally, the modified value of the global variable is printed.
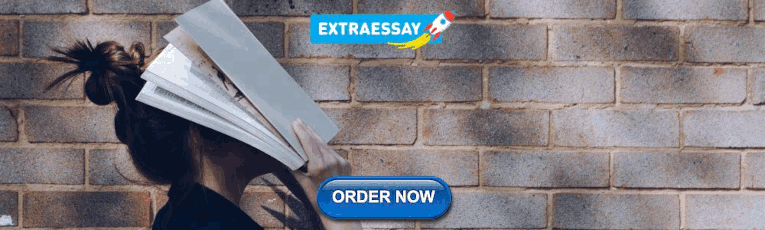
The Attempt to Access a Local Variable Beyond Its Function
In the above code, local_var is a local variable declared and initialized within the func() function. The scope of this variable is limited to the function only. When the function is called, the value of local_var is printed. However, when we try to access this variable outside the function, we get a NameError as the variable is not defined in that scope.
Global variables are variables that can be accessed and modified from anywhere in the program, whereas local variables are only accessible within a specific function or block of code. The scope of a variable refers to the area in which it can be accessed.
Non-local variables in Python are variables that are defined in an outer function but can be accessed in an inner function. The nonlocal keyword is used to declare a non-local variable in Python.
In this example, count is a global variable that can be accessed and modified from anywhere in the program. The global keyword is used inside the increment function to indicate that we are modifying the global variable count .
In this example, x is a local variable in the outer function. The inner function has access to this variable using the nonlocal keyword, so we can modify its value. When we call the outer function, the inner function is executed and the value of x is changed to nonlocal . This change is reflected in the outer function when we print the value of x after the inner function is executed.
Let's review how to use global variables in functions in Python . Global variables are variables that can be accessed and modified from any part of the program. In Python, a variable's scope determines where it can be accessed. The best practice for using global variables in Python is to minimize their usage, as too many global variables can make the program difficult to understand, debug, and maintain.
One example of a global variable is the power function in Python. We can use a loop to calculate the power of a number. Here is an example:
In this example, we declare a global variable power outside the function calculate_power() . Inside the function, we use this global variable to store the power of the number. We reset the value of the power variable to 1 for each new calculation.
Program to Count the Number of Times a Function is Called
In this example, we declare the global variable count outside the function my_function() . Inside the function, we increment the value of the count variable every time the function is called. We then print the value of count .
Overall, it is generally best to avoid global variables in favor of local variables with more limited scope. However, in some cases, global variables may be necessary or useful, and we can use them carefully with the global keyword to access them inside functions.
Advanced tips for Python variable scopes include avoiding global variables as much as possible to prevent naming conflicts and unexpected behavior. It is also recommended to use descriptive variable names that follow the PEP 8 naming conventions , such as using lowercase letters and underscores to separate words.
Function naming conventions in Python follow the same PEP 8 guidelines , using lowercase letters and underscores to separate words. Function names should also be descriptive and convey the purpose of the function.
In this example, we declare a local variable message inside the greet() function. This variable is only accessible within the function and cannot be accessed from outside. The function takes a name parameter and returns a greeting message with the name included.
Contribute with us!
Do not hesitate to contribute to Python tutorials on GitHub: create a fork, update content and issue a pull request.
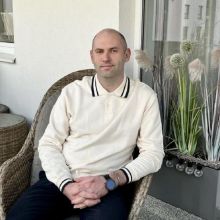
Assigning to global variables PREMIUM

Sign in to change your settings
Sign in to your Python Morsels account to save your screencast settings.
Don't have an account yet? Sign up here .
Let's talk about assigning to global variables in Python.
Variable assignments are local
Let's take a global variable (a variable defined outside of any function ) called message :
And let's define a function called set_message that assigns to the message variable:
If we call the set_message function with the name Trey , it will assign message to Hello Trey .
But if we read message now, it's not actually Hello Trey , it's Hello world :
This happens because assignments within a function only assign to local variables . So when we're inside set_message function, we're always assigning, to a local variable .
So that message variable in the set_message function is a local variable . When the function returns, that local variable disappears.
So calling set_message doesn't actually change our global variable message , it just shadowed the global variable message (meaning we made a local variable with the same name as a global variable).
The global variable promise
It is actually possible in to write to global variables in Python.
I'm going to show you how to write to global variables, but you must promise me that you won't actually every do this .
"I promise I will not assign to global variables from within a function"
Promised? Great! Read on...
Assigning to global variables
I've assigned to global variables (from within a function) very infrequently in my own Python code and you probably shouldn't do this in your code either. But Python does allow you to do this even though you often shouldn't.
The trick to writing a global variable is to use the global statement :
When we call this new set_message function you'll see that it actually updates the global message variable:
If we call set_message with a different name, message will change again:
Normally, all assignments assign to local variables and variables are either local or global in one scope. But you can think of the global statement as an escape hatch: it's a way of declaring that the message variable is a global variable within the set_message function.
Best practices with global variables
So, every time we read from it and (more importantly) every time we write to it, it should read from and write to the global variable message .
You don't need the global statement if you're just reading . Whenever you read a variable, Python will look for a local variable with that name and, if it doesn't find it, it will look for a global variable with the same name.
You only need global when you're writing to a global variable . And, you probably shouldn't be writing to global variables from within a function.
Functions are typically are seen as having inputs (which come from their arguments usually) and outputs (usually their return value).
Global state usually shouldn't be changed simply by calling a function. Functions usually provide information through their return value.
It's a common practice to make a function like this:
This get_message function doesn't assign to a message variable; it just returns a string which represents the message we actually want:
This get_message function doesn't change our global variable message :
But if we did want to change our global message variable, as long as we're in the global scope when we're calling the function, we can assign to that global variable directly:
Assigning to message while at the module-level (outside of any function), changes the global message variable directly:
If we wanted to change it to something else, we'd pass in a different argument, and continue to assign to the message variable:
Typically in Python we embrace the fact that all assignments are local ; unless you're in the global scope (you're outside of any function) because then assignment statements will assign to the global scope.
All assignments assign to local variables in Python, unless you use the global statement which is kind of our escape hatch for assigning to a global variable. Don't do it though! You don't usually want that escape hatch.
Typically instead of assigning to a global variable, you should return from your function and leave it up to the caller of your function to assign to a global variable , if they would like to.
A Python tip every week
Need to fill-in gaps in your Python skills?
Sign up for my Python newsletter where I share one of my favorite Python tips every week .
Series: Variable Scope
Python has 4 scopes: local, enclosing, global, and built-ins. Python's "global" variables are only global to the module they're in. The only truly universal variables are the built-ins.
To track your progress on this Python Morsels topic trail, sign in or sign up .
Remember the "two types of change" in Python? Scope is all about one of those two types of change: assignment . Python's scope rules are unrelated to mutation.
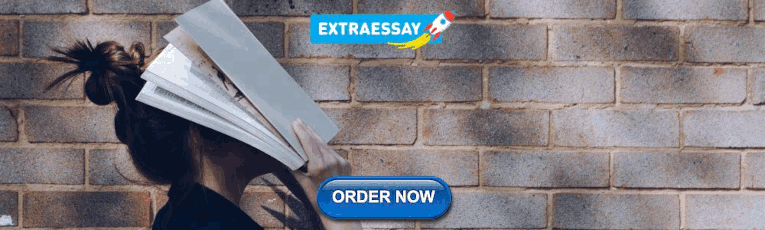
IMAGES
VIDEO
COMMENTS
In this tutorial, you'll learn how to use global variables in Python functions using the global keyword or the built-in globals() function. You'll also learn a few strategies to avoid relying on global variables because they can lead to code that's difficult to understand, debug, and maintain.
Get started. In this article, you will learn the basics of global variables. To begin with, you will learn how to declare variables in Python and what the term 'variable scope' actually means. Then, you will learn the differences between local and global variables and understand how to define global variables and.
189. Global variables are special. If you try to assign to a variable a = value inside of a function, it creates a new local variable inside the function, even if there is a global variable with the same name. To instead access the global variable, add a global statement inside the function: a = 7. def setA(value):
There are 2 ways to declare a variable as global: 1. assign variable inside functions and use global line. def declare_a_global_variable(): global global_variable_1 global_variable_1 = 1 # Note to use the function to global variables declare_a_global_variable() 2. assign variable outside functions: global_variable_2 = 2
It is used to pull in a variable that already exists outside of its scope. It's true that global itself doesn't create the variable, but it doesn't require that it already exist either. Inside a function, you can do global x and then x = 10 and that will create the global variable if it doesn't already exist.
Example 1: How to Define a Global Variable in Python. # Define a global variable. global_var = 10. In Python, global variables can be accessed and modified from any function or module in the program. However, assigning a value to a global variable inside a function creates a new local variable within that function.
Trey Hunner. 3 min. read • 4 min. video • Python 3.8—3.12 • Jan. 28, 2021. Let's talk about assigning to global variables in Python. Variable assignments are local. Let's take a global variable (a variable defined outside of any function) called message: >>> message = "Hello world"
In this tutorial, you’ll learn what is a global variable in Python and how to use them effectively. Goals of this lesson: Understand what is a global variable in Python with examples; Use global variables across multiple functions; Learn how to use the global keyword to modify the global variables; Learn to use global variables across Python ...